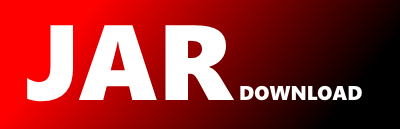
com.ibm.cp4waiops.connectors.sdk.Util Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of connectors-sdk Show documentation
Show all versions of connectors-sdk Show documentation
A developer SDK for creating connectors for CP4WAIOps.
package com.ibm.cp4waiops.connectors.sdk;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.nio.charset.StandardCharsets;
import com.google.protobuf.ByteString;
import com.google.protobuf.InvalidProtocolBufferException;
import io.cloudevents.core.format.EventDeserializationException;
import io.cloudevents.core.format.EventFormat;
import io.cloudevents.core.format.EventSerializationException;
import io.cloudevents.jackson.JsonFormat;
import io.cloudevents.protobuf.ProtobufFormat;
import java.util.logging.Logger;
public class Util {
private static final EventFormat _jsonFormat = new JsonFormat();
private static final EventFormat _protoFormat = new ProtobufFormat();
private static final Logger logger = Logger.getLogger(Util.class.getName());
public static io.cloudevents.v1.proto.CloudEvent convertCloudEventToProto(io.cloudevents.CloudEvent event)
throws EventSerializationException, InvalidProtocolBufferException {
// TODO get rid of the extra conversion step
byte[] tmp = _protoFormat.serialize(event);
io.cloudevents.v1.proto.CloudEvent converted = io.cloudevents.v1.proto.CloudEvent.parseFrom(tmp);
// Workaround for structured cloud events serialized to kafka
if (event.getDataContentType() == Constant.JSON_CONTENT_TYPE && converted.hasTextData()) {
ByteString data = ByteString.copyFrom(converted.getTextData(), StandardCharsets.UTF_8);
converted = converted.toBuilder().setBinaryData(data).build();
}
return converted;
}
public static io.cloudevents.CloudEvent convertCloudEventFromProto(io.cloudevents.v1.proto.CloudEvent proto)
throws EventDeserializationException {
// TODO get rid of the extra conversion step
byte[] tmp = proto.toByteArray();
return _protoFormat.deserialize(tmp);
}
public static String convertCloudEventToJSON(io.cloudevents.CloudEvent event) throws EventSerializationException {
byte[] tmp = _jsonFormat.serialize(event);
return new String(tmp, StandardCharsets.UTF_8);
}
/**
* Provide more details error diagnostic information to assist the connector developer.
*
* @param t
* - the throwable containing the stack trace to be analyzed.
*
* @return a more detailed diagnostic message. If none can be found, null is returned
*/
public static String getDiagnosticMessage(Throwable t) {
if (t != null) {
try {
StringWriter sw = new StringWriter();
t.printStackTrace(new PrintWriter(sw));
String exceptionAsString = sw.toString();
String resultString = null;
// The most common error message seen contains "UNAVAILABLE: io exception". However, that can be caused
// by many scenarios. This stack trace analyzer looks deeper into the stack trace to help provide better
// diagnostic information to the connector developer to fix the problem.
if (exceptionAsString.contains("java.io.IOException: Connection reset by peer")) {
resultString = "CAUSE: connector-bridge loses connection due to lack of activity on the gRPC channel.\n FIX: add a keep-alive to the connector by adding the Liberty config file /resources/META-INF/microprofile-config.properties with grpc-bridge.grpc-client-keep-alive-time-seconds=60";
} else if (exceptionAsString.contains("UNAVAILABLE: Network closed for unknown reason")) {
resultString = "CAUSE: connector-bridge pod was unavailable, most often caused by the connector-bridge restarting and being temporarily unavailable.\n FIX: Ensure the Kafka and Zookeeper pods are not in a restart case. Check if the connector-bridge has restarted several times to see what the cause was.";
} else if (exceptionAsString.contains("java.net.ConnectException: Connection refused")) {
resultString = "CAUSE: connector-bridge pod cannot be reached, most often caused by a certificate issue.\n FIX: Ensure the certificate used by the connector is up-to-date. Restarting the pod can also refresh the certificate on the connector to resolve the problem.";
} else if (exceptionAsString
.contains("io.netty.channel.ConnectTimeoutException: connection timed out")) {
resultString = "CAUSE: connector-bridge pod cannot be reached, most often caused by the pod or GitApp being unavailable.\n FIX: Ensure the connector-bridge is in a running state and has no restarts. Check the connector-bridge logs for more details.";
}
return resultString;
} catch (Exception e) {
// Do nothing, this is to prevent any unforseen error
logger.info("Could not parse stack trace due to " + e.getMessage());
}
}
return null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy