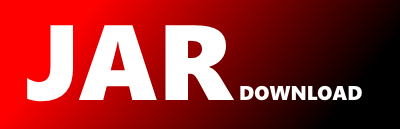
com.ibm.optim.oaas.client.job.JobClient.html Maven / Gradle / Ivy
Show all versions of api_java_client Show documentation
JobClient (Java Client for DOcplexcloud 1.0 API)
com.ibm.optim.oaas.client.job
Interface JobClient
-
public interface JobClient
Interface of a job client used to create, submit and monitor optimization
jobs.
-
-
Method Summary
Methods
Modifier and Type
Method and Description
void
abortJob(String jobid)
Aborts execution of a job.
String
copyJob(String jobid,
JobCreationData data,
boolean shallow)
Creates a new job by copying an existing job.
String
createJob(JobCreationData data)
Creates a new job.
String
createJobAttachment(String jobid,
JobAttachmentCreationData att)
Creates a job attachment for a given job.
void
deleteAllJobs()
Deletes all jobs.
boolean
deleteJob(String jobid)
Deletes a job.
boolean
deleteJobAttachment(String jobid,
String attid)
Deletes a job attachment.
void
deleteJobAttachments(String jobid)
Deletes all job attachments of a given job.
InputStream
downloadJobAttachment(String jobid,
String attid)
Downloads job attachment contents as a stream.
void
downloadJobAttachment(String jobid,
String attid,
File file)
Downloads job attachment contents and stores them as a file.
<T> T
downloadJobAttachment(String jobid,
String attid,
com.fasterxml.jackson.databind.ObjectMapper mapper,
Class<T> type)
Downloads job attachment contents as a JSON object.
<T> T
downloadJobAttachment(String jobid,
String attid,
com.fasterxml.jackson.databind.ObjectMapper mapper,
com.fasterxml.jackson.core.type.TypeReference<T> type)
Downloads job attachment contents as a JSON object.
void
downloadJobAttachment(String jobid,
String attid,
OutputStream stream)
Downloads job attachment contents and copies them to an output stream.
InputStream
downloadLog(String jobid)
Downloads the log as a stream.
void
downloadLog(String jobid,
File file)
Downloads the log and stores it as a file.
void
downloadLog(String jobid,
OutputStream stream)
Downloads the log and copies it to an output stream.
void
executeJob(String jobid)
Submits a job for execution.
List<? extends Job>
getAllJobs()
Returns all the jobs.
JobFailureInfo
getFailureInfo(String jobid)
Returns job failure information for the given job.
Job
getJob(String jobid)
Returns a given job.
JobAttachment
getJobAttachment(String jobid,
String attid)
Returns the job attachment (if any) of a given job.
List<? extends JobAttachment>
getJobAttachments(String jobid)
Returns the list of job attachments for a job.
List<? extends JobAttachment>
getJobAttachments(String jobid,
JobAttachmentType type)
Returns the list of job attachments of a given type for a job.
JobExecutionStatus
getJobExecutionStatus(String jobid)
Returns the job execution status.
List<? extends JobLogItem>
getJobLogItems(String jobid)
Returns the list of the log items of a given job.
List<? extends JobLogItem>
getJobLogItems(String jobid,
long start)
Returns the list of the log items of a given job starting at a given index
(included).
List<? extends JobLogItem>
getJobLogItems(String jobid,
long start,
boolean continuous)
Returns the list of log items of a given job starting at a given index
(included).
void
killJob(String jobid)
Kills the execution of a job.
JobAttachmentCreationData
newInputJobAttachment(String name)
Creates new input attachment data to be added to a job.
JobCreationData
newJobCreationData()
Creates a new object to create and initialize a new job.
JobRequestBuilder
newRequest()
Creates a new job request builder using this client.
String
recreateJob(String jobid,
JobCreationData data,
boolean execute)
Creates a new job by replacing an existing job.
void
uploadJobAttachment(String jobid,
String attid,
AttachmentContentWriter writer)
Uploads job attachment contents with a custom writer.
void
uploadJobAttachment(String jobid,
String attid,
File file)
Uploads job attachment contents as a file.
void
uploadJobAttachment(String jobid,
String attid,
InputStream stream)
Uploads job attachment contents as a stream.
void
uploadJobAttachment(String jobid,
String attid,
com.fasterxml.jackson.databind.ObjectMapper mapper,
Object obj)
Uploads job attachment contents as a JSON stream.
-
-
Method Detail
-
deleteAllJobs
void deleteAllJobs()
throws OperationException
Deletes all jobs. Jobs currently being executed will be killed before being deleted.
- Throws:
OperationException
- if any other remote exception was raised.
-
getAllJobs
List<? extends Job> getAllJobs()
throws OperationException
Returns all the jobs.
- Returns:
- The jobs.
- Throws:
OperationException
- if any other remote exception was raised.
-
createJob
String createJob(JobCreationData data)
throws OperationException,
SubscriptionException,
ValidationException
Creates a new job. Data can be passed to initialize the job. The
application ID refers to the type of engine (for example opl
or cplex
). There are two ways to manage the application ID:
-
The application ID can be specified explicitly at creation time. In this
case, the attachments can be declared and uploaded incrementally. When
the job is submitted, the list of attachments must be compatible with the
application ID, otherwise an exception will be returned.
-
The application ID can be left unspecified at creation time. In this case, the full
list of attachments must be declared, and the application ID will be deduced
from the attachment extensions.
Note that when the attachments are declared and length is specified, the
subscription limits are also pre-checked so that an exception will be
raised before actually uploading the content.
- Parameters:
data
- The data used to initialize the job.
- Returns:
- The ID of the newly created job.
- Throws:
SubscriptionException
- if the subscription is invalid or a limit was reached.
ValidationException
- if the application or attachments are invalid.
OperationException
- if any other remote exception is raised such as a system
issue or authentication issue.
-
copyJob
String copyJob(String jobid,
JobCreationData data,
boolean shallow)
throws OperationException,
SubscriptionException,
ValidationException,
JobNotFoundException
Creates a new job by copying an existing job. The existing job
cannot currently be running or waiting for execution. All creation data
is copied over to the new job. By default, input attachments are copied
to the new job. If a shallow copy is requested, the contents will point to the existing job
and if it is deleted, accessing the referenced input attachments will raise an exception. Output
attachments are ignored. Optionally, job creation data can be passed
to override the parameters and declare additional or replacement input attachments.
- Parameters:
jobid
- The job ID of the job to copy.data
- The data used to override the template value or null
if not specified.shallow
- Indicates if a shallow copy is requested. If true
, the job contents point to the existing job.
- Returns:
- The created job ID.
- Throws:
JobNotFoundException
- if the job was not found.
SubscriptionException
- if the subscription is invalid or a limit was reached.
ValidationException
- if the application or attachments are invalid.
OperationException
- if any other remote exception is raised such as a system
issue or authentication issue.- See Also:
recreateJob(String, JobCreationData, boolean)
-
recreateJob
String recreateJob(String jobid,
JobCreationData data,
boolean execute)
throws OperationException,
SubscriptionException,
ValidationException,
JobNotFoundException
Creates a new job by replacing an existing job.
This can be used to resubmit a failed job for example. The existing job
cannot currently be running or waiting for execution. All creation data
is copied over to the new job. Input attachments are declared
in the new job and the contents are transferred to the new job. Output
attachments are ignored. Optionally, job creation data can be passed
to override the parameters and declare additional input attachments.
The existing job is automatically deleted.
- Parameters:
jobid
- The job ID of the template job.data
- The data used to override the existing values, or null
if not specified.execute
- Indicates if the job should be immediately submitted for execution.
- Returns:
- The created job id.
- Throws:
JobNotFoundException
- if the job was not found.
SubscriptionException
- if the subscription is invalid or a limit was reached.
ValidationException
- if the application or attachments are invalid.
OperationException
- if any other remote exception is raised, such as a system
issue or authentication issue.
-
getJob
Job getJob(String jobid)
throws OperationException,
JobNotFoundException
Returns a given job.
- Parameters:
jobid
- The job ID.
- Returns:
- The job.
- Throws:
OperationException
- if any other remote exception was raised.
JobNotFoundException
- if the job was not found.
-
deleteJob
boolean deleteJob(String jobid)
throws OperationException
Deletes a job. If the job is currently running, it will be killed before being
deleted. If the job cannot be found, no action is taken.
- Parameters:
jobid
- The job ID.
- Returns:
true
if it was deleted, false
otherwise.
- Throws:
OperationException
- if any other remote exception was raised.
-
getJobExecutionStatus
JobExecutionStatus getJobExecutionStatus(String jobid)
throws OperationException,
JobNotFoundException
Returns the job execution status. Depending on the status, additional
information will be available in the job description. For example,
if the status is FAILED
, additional information will be available in the
failure
property of the job.
- Parameters:
jobid
- The job ID.
- Returns:
- The job execution status.
- Throws:
OperationException
- if any other remote exception was raised.
JobNotFoundException
- if the job was not found.- See Also:
getJob(String)
-
executeJob
void executeJob(String jobid)
throws OperationException,
JobNotFoundException,
SubscriptionException,
ValidationException
Submits a job for execution. If the job does not currently have the state
CREATED
(for example, it is running or has already completed),
an exception will be returned.
- Parameters:
jobid
- The job ID.
- Throws:
OperationException
- if any other remote exception was raised.
JobNotFoundException
- if the job was not found.
SubscriptionException
- if the subscription is invalid or a limit was reached.
ValidationException
- if the application or attachments are invalid.
-
abortJob
void abortJob(String jobid)
throws OperationException,
JobNotFoundException
Aborts execution of a job. If the job is currently not running, nothing is done. When
a job is aborted, a clean stop is attempted.
- Parameters:
jobid
- The job ID.
- Throws:
OperationException
- if any other remote exception was raised.
JobNotFoundException
- if the job was not found.
-
killJob
void killJob(String jobid)
throws OperationException,
JobNotFoundException
Kills the execution of a job. If the job is currently not running, no action is taken.
- Parameters:
jobid
- The job ID.
- Throws:
OperationException
- if any other remote exception was raised.
JobNotFoundException
- if the job was not found.
-
getJobAttachments
List<? extends JobAttachment> getJobAttachments(String jobid)
throws OperationException,
JobNotFoundException
Returns the list of job attachments for a job.
- Parameters:
jobid
- The job ID.
- Returns:
- The list of attachments.
- Throws:
OperationException
- if any other remote exception was raised.
JobNotFoundException
- if the job was not found.
-
getJobAttachments
List<? extends JobAttachment> getJobAttachments(String jobid,
JobAttachmentType type)
throws OperationException,
JobNotFoundException
Returns the list of job attachments of a given type for a job.
- Parameters:
jobid
- The job ID.type
- The type of the job attachment (an input attachment or an output attachment).
- Returns:
- The list of attachments.
- Throws:
OperationException
- if any other remote exception was raised.
JobNotFoundException
- if the job was not found.
-
deleteJobAttachments
void deleteJobAttachments(String jobid)
throws OperationException,
JobNotFoundException
Deletes all job attachments of a given job.
- Parameters:
jobid
- The job ID.
- Throws:
OperationException
- if any other remote exception was raised.
JobNotFoundException
- if the job was not found.
-
createJobAttachment
String createJobAttachment(String jobid,
JobAttachmentCreationData att)
throws OperationException,
JobNotFoundException,
SubscriptionException,
ValidationException
Creates a job attachment for a given job.
- Parameters:
jobid
- The job ID.att
- The attachment data used to initialize the attachment.
- Returns:
- The attachment ID (attachment name).
- Throws:
OperationException
- if any other remote exception was raised.
JobNotFoundException
- if the job was not found.
SubscriptionException
- if the subscription is invalid or a limit was reached.
ValidationException
- if the application or attachments are invalid.
-
getJobAttachment
JobAttachment getJobAttachment(String jobid,
String attid)
throws OperationException,
JobNotFoundException,
AttachmentNotFoundException
Returns the job attachment (if any) of a given job.
- Parameters:
jobid
- The job ID.attid
- The job attachment ID.
- Returns:
- The attachment.
- Throws:
OperationException
- if any other remote exception was raised.
JobNotFoundException
- if the job was not found.
AttachmentNotFoundException
- if the attachment was not found.
-
deleteJobAttachment
boolean deleteJobAttachment(String jobid,
String attid)
throws OperationException,
JobNotFoundException
Deletes a job attachment.
- Parameters:
jobid
- The job ID.attid
- The attachment ID.
- Returns:
true
if the job attachment was deleted.
- Throws:
OperationException
- if any other remote exception was raised.
JobNotFoundException
- if the job was not found.
-
uploadJobAttachment
void uploadJobAttachment(String jobid,
String attid,
File file)
throws IOException,
OperationException,
JobNotFoundException,
AttachmentNotFoundException,
SubscriptionException
Uploads job attachment contents as a file.
- Parameters:
jobid
- The job ID.attid
- The attachment ID.file
- The content as a file.
- Throws:
IOException
- if an exception occurred while accessing the file.
JobNotFoundException
- if the job was not found.
AttachmentNotFoundException
- if the attachment was not found.
SubscriptionException
- if the subscription is invalid or a limit was reached.
OperationException
- if any other remote exception was raised.
-
uploadJobAttachment
void uploadJobAttachment(String jobid,
String attid,
InputStream stream)
throws IOException,
OperationException,
JobNotFoundException,
AttachmentNotFoundException,
SubscriptionException
Uploads job attachment contents as a stream.
- Parameters:
jobid
- The job ID.attid
- The attachment ID.stream
- The content as a stream.
- Throws:
IOException
- if an exception occurred while accessing the stream.
OperationException
- if any other remote exception was raised.
JobNotFoundException
- if the job was not found.
AttachmentNotFoundException
- if the attachment was not found.
SubscriptionException
- if the subscription is invalid or a limit was reached.
-
uploadJobAttachment
void uploadJobAttachment(String jobid,
String attid,
AttachmentContentWriter writer)
throws IOException,
OperationException,
JobNotFoundException,
AttachmentNotFoundException,
SubscriptionException
Uploads job attachment contents with a custom writer.
- Parameters:
jobid
- The job ID.attid
- The attachment ID.writer
- The content writer.
- Throws:
IOException
- if an exception occurred while accessing the stream.
OperationException
- if any other remote exception was raised.
JobNotFoundException
- if the job was not found.
AttachmentNotFoundException
- if the attachment was not found.
SubscriptionException
- if the subscription is invalid or a limit was reached.
-
uploadJobAttachment
void uploadJobAttachment(String jobid,
String attid,
com.fasterxml.jackson.databind.ObjectMapper mapper,
Object obj)
throws IOException,
OperationException,
JobNotFoundException,
AttachmentNotFoundException,
SubscriptionException
Uploads job attachment contents as a JSON stream.
- Parameters:
jobid
- The job ID.attid
- The attachment ID.mapper
- The JSON mapper.obj
- The object to be serialized as JSON.
- Throws:
IOException
- if an exception occurred while serializing the object.
OperationException
- if any other remote exception was raised.
JobNotFoundException
- if the job was not found.
AttachmentNotFoundException
- if the attachment was not found.
SubscriptionException
- if the subscription is invalid or a limit was reached.
-
downloadJobAttachment
void downloadJobAttachment(String jobid,
String attid,
File file)
throws IOException,
OperationException,
JobNotFoundException,
AttachmentNotFoundException
Downloads job attachment contents and stores them as a file.
- Parameters:
jobid
- The job ID.attid
- The attachment ID.file
- The content as a file.
- Throws:
IOException
- if an exception occurred while accessing the file.
OperationException
- if any other remote exception was raised.
JobNotFoundException
- if the job was not found.
AttachmentNotFoundException
- if the attachment was not found.
-
downloadJobAttachment
void downloadJobAttachment(String jobid,
String attid,
OutputStream stream)
throws IOException,
OperationException,
JobNotFoundException,
AttachmentNotFoundException
Downloads job attachment contents and copies them to an output stream.
- Parameters:
jobid
- The job ID.attid
- The attachment ID.stream
- The content as a stream.
- Throws:
IOException
- if an exception occurred while accessing the stream.
OperationException
- if any other remote exception was raised.
JobNotFoundException
- if the job was not found.
AttachmentNotFoundException
- if the attachment was not found.
-
downloadJobAttachment
<T> T downloadJobAttachment(String jobid,
String attid,
com.fasterxml.jackson.databind.ObjectMapper mapper,
com.fasterxml.jackson.core.type.TypeReference<T> type)
throws IOException,
OperationException,
JobNotFoundException,
AttachmentNotFoundException
Downloads job attachment contents as a JSON object.
- Type Parameters:
T
- The type of the return object.- Parameters:
jobid
- The job ID.attid
- The attachment ID.mapper
- The JSON mapper.type
- The type to deserialize.
- Returns:
- The stream.
- Throws:
IOException
- if an exception occurred while accessing the stream.
OperationException
- if any other remote exception was raised.
JobNotFoundException
- if the job was not found.
AttachmentNotFoundException
- if the attachment was not found.
-
downloadJobAttachment
<T> T downloadJobAttachment(String jobid,
String attid,
com.fasterxml.jackson.databind.ObjectMapper mapper,
Class<T> type)
throws IOException,
OperationException,
JobNotFoundException,
AttachmentNotFoundException
Downloads job attachment contents as a JSON object.
- Type Parameters:
T
- The type of the return object.- Parameters:
jobid
- The job ID.attid
- The attachment ID.mapper
- The JSON mapper.type
- The type to deserialize.
- Returns:
- The stream.
- Throws:
IOException
- if an exception occurred while accessing the stream.
OperationException
- if any other remote exception was raised.
JobNotFoundException
- if the job was not found.
AttachmentNotFoundException
- if the attachment was not found.
-
downloadJobAttachment
InputStream downloadJobAttachment(String jobid,
String attid)
throws IOException,
OperationException,
JobNotFoundException,
AttachmentNotFoundException
Downloads job attachment contents as a stream. The stream must be closed
to release resources.
- Parameters:
jobid
- The job ID.attid
- The attachment ID.
- Returns:
- The stream.
- Throws:
IOException
- if an exception occurred while accessing the stream.
OperationException
- if any other remote exception was raised.
JobNotFoundException
- if the job was not found.
AttachmentNotFoundException
- if the attachment was not found.
-
downloadLog
void downloadLog(String jobid,
File file)
throws IOException,
OperationException,
JobNotFoundException
Downloads the log and stores it as a file.
- Parameters:
jobid
- The job ID.file
- The log file.
- Throws:
IOException
- if an exception occurred while accessing the file.
JobNotFoundException
- if the job was not found.
OperationException
- if any other remote exception was raised.
-
downloadLog
void downloadLog(String jobid,
OutputStream stream)
throws IOException,
OperationException,
JobNotFoundException
Downloads the log and copies it to an output stream.
- Parameters:
jobid
- The job ID.stream
- The log stream.
- Throws:
IOException
- if an exception occurred while accessing the stream.
JobNotFoundException
- if the job was not found.
OperationException
- if any other remote exception was raised.
-
downloadLog
InputStream downloadLog(String jobid)
throws IOException,
OperationException,
JobNotFoundException
Downloads the log as a stream. The stream must be closed to release
resources.
- Parameters:
jobid
- The job ID.
- Returns:
- The log stream.
- Throws:
IOException
- if an exception occurred while accessing the stream.
OperationException
- if any other remote exception was raised.
JobNotFoundException
- if the job was not found.
-
getFailureInfo
JobFailureInfo getFailureInfo(String jobid)
throws OperationException,
JobNotFoundException
Returns job failure information for the given job.
- Parameters:
jobid
- The job ID.
- Returns:
- The failure information.
- Throws:
OperationException
- if any other remote exception was raised.
JobNotFoundException
- if the job was not found.
-
getJobLogItems
List<? extends JobLogItem> getJobLogItems(String jobid)
throws OperationException,
JobNotFoundException
Returns the list of the log items of a given job.
Each job generates log items with an index sequence number
starting at 0.
This list can be very large
and only a maximum number of log items, defined internally by the service,
will be returned at once.
The returned list is always ordered by sequence index.
To know if more log items are available, specify the next
starting sequence index.
- Parameters:
jobid
- The job ID.
- Returns:
- The list of log items.
- Throws:
OperationException
- if any other remote exception was raised.
JobNotFoundException
- if the job was not found.- See Also:
getJobLogItems(String, long)
,
getJobLogItems(String, long, boolean)
-
getJobLogItems
List<? extends JobLogItem> getJobLogItems(String jobid,
long start)
throws OperationException,
JobNotFoundException
Returns the list of the log items of a given job starting at a given index
(included).
Each job generates log items with an index sequence number
starting at 0.
This list can be very large
and only a maximum number of log items, defined internally by the service,
will be returned at once.
The returned list is always ordered by sequence index.
To know if more log items are available, specify the
starting sequence index.
- Parameters:
jobid
- The job ID.start
- The start index.
- Returns:
- The list of log items.
- Throws:
OperationException
- if any other remote exception was raised.
JobNotFoundException
- if the job was not found.- See Also:
getJobLogItems(String, long, boolean)
-
getJobLogItems
List<? extends JobLogItem> getJobLogItems(String jobid,
long start,
boolean continuous)
throws OperationException,
JobNotFoundException
Returns the list of log items of a given job starting at a given index
(included).
This list can be very large
and only a maximum number of log items, defined internally by the service,
will be returned at once.
The returned list is always ordered by sequence index.
To know if more log items are available, specify the
starting sequence index as the last sequence index + 1.
The continuous mode is designed to iterate over the log items as they become available until
the job ends to retrieve and display the live logs.
If the continuous flag is set, the server will return the next elements with no gap. It will also
set the missing flag of a log item if the log item is still not available after an internal timeout.
It will also set the log item stop flag when the sequence is completed.
Here is a code snippet showing how to use the continuous mode.
boolean stop = false;
long index = 0;
while (!stop) {
List<? extends JobLogItem> items = jobclient.getJobLogItems(jobid, index, true);
if ((items != null) && !items.isEmpty()) {
display(items);
JobLogItem last = items.get(items.size() - 1);
stop = last.stop();
index = last.getSeqid() + 1;
} else {
Thread.sleep(1000); // no new log so wait a little
}
}
- Parameters:
jobid
- The job ID.start
- The start index.continuous
- Indicates if server will try to return log elements in a continuous sequence
- Returns:
- The list of log items.
- Throws:
OperationException
- if any other remote exception was raised.
JobNotFoundException
- if the job was not found.
-
newJobCreationData
JobCreationData newJobCreationData()
Creates a new object to create and initialize a new job.
- Returns:
- A job creation data instance.
-
newInputJobAttachment
JobAttachmentCreationData newInputJobAttachment(String name)
Creates new input attachment data to be added to a job.
- Parameters:
name
- The attachment name.
- Returns:
- The attachment data.
-
newRequest
JobRequestBuilder newRequest()
Creates a new job request builder using this client.
- Returns:
- The job request.
Copyright © 2015–2017 IBM Corporation. Licensed under the Apache License, Version 2.0.