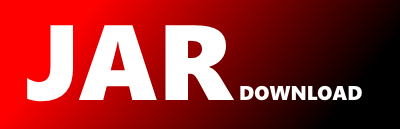
com.ibm.ims.connect.ConnectionFactory Maven / Gradle / Ivy
Show all versions of IMSConnectAPI Show documentation
/*
* File: ConnectionFactory.java
* ==========================================================================
* Licensed Material - Property of IBM
*
* IBM Confidential
*
* OCO Source Materials
*
* 5655-TDA
*
* (C) Copyright IBM Corp. 2009,2014 All Rights Reserved.
*
* The source code for this program is not published or
* otherwise divested of its trade secrets, irrespective
* of what has been deposited with the U.S. Copyright
* Office.
*
* US Government Users Restricted Rights - Use, duplication or
* disclosure restricted by GSA ADP Schedule Contract with
* IBM Corp.
* ===========================================================================
*/
package com.ibm.ims.connect;
import com.ibm.ims.connect.impl.ConnectionImpl;
import java.io.InputStream;
import java.net.URL;
import java.util.logging.Logger;
/**
* Factory class for creating connections to IMS Connect. Connection
objects generated by the
* ConnectionFactory
class are application-level handles to underlying physical TCP/IP socket connections.
*
* A client application can manually configure a connection factory by providing the connection attributes as input.
*
*
ConnectionFactory myCF = new ConnectionFactory();
myCF.loadConnectionFactoryAttributesFromFile("myConnectionAttributes.txt");
myCF.setSocketType(ApiProperties.SOCKET_TYPE_TRANSACTION);
myCF.setHostName("testsrvr.svl.ibm.com");
myCF.setClientId("myClntId");
myCF.setSslEncryptionType(ApiProperties.SSL_ENCRYPTYPE_NONE);
...
Connection myConn = myCF.getConnection();
*
*
* @see Connection
*/
public final class ConnectionFactory implements ApiProperties
// implements java.io.Serializable
{
@SuppressWarnings("unused")
private static final String copyright =
"Licensed Material - Property of IBM "
+ "5655-TDA"
+ "(C) Copyright IBM Corp. 2009, 2013 All Rights Reserved. "
+ "US Government Users Restricted Rights - Use, duplication or "
+ "disclosure restricted by GSA ADP Schedule Contract with IBM Corp. ";
private Logger logger;
@SuppressWarnings("unused")
private static String traceFileName;
static
{
String os = java.lang.System.getProperty("os.name").toUpperCase();
if ( os.indexOf("WINDOWS") >= 0)
{
traceFileName = ApiProperties.DEFAULT_TRACE_FILE_NAME_WINDOWS;
}
else if ((os.indexOf("Z/OS") >= 0) || (os.indexOf("OS/390") >= 0))
{
traceFileName = ApiProperties.DEFAULT_TRACE_FILE_NAME_USS;
}
else
{
// traceFileName = ApiProperties.DEFAULT_TRACE_FILE_NAME_UNIX;
}
}
/*** Connection properties ***/
/**
* hostName is a String variable whose value is the hostname (or IP address???)
* of the target IMS Connect for this connection. The default value is
* "myHostNm". Values must be a 1 to 8 character String set to the correct
* hostname of the target IMS Connect (or a string containing the IP address
* of the target IMS Connect (e.g., nnn.nnn.nnn.nnn or nnnn.nnnn.nnnn.nnnn.nnnn.nnnn
* or some permutations of these)
*/
private String hostName = ApiProperties.DEFAULT_HOSTNAME;
/**
* portNumber is an int variable whose value is the port number of the
* target IMS Connect for this connection. The default value is
* 9999. Values must be a valid port number set to the port number
* on which the target IMS Connect is listening.
*/
private int portNumber = ApiProperties.DEFAULT_PORTNUMBER;
/**
* socketType is an int variable whose value is used to determine whether a
* socket connection is a transaction socket which can only be disconnected
* by IMS Connect (either after a single non-conversational IMS transaction
* interaction has completed, after an IMS conversation (most likely
* including multiple iterations of the conversation) has completed, or
* after IMS itself has terminated or after an error has occured) or a
* persistent socket (left connected by IMS Connect until a fatal error
* has occured on that connection while IMS Connect is processing an
* interaction or until a disconnect request has been received by IMS
* Connect.)
* valid values for socketType:
* ApiProperties.SOCKET_TYPE_TRANSACTION
* ApiProperties.SOCKET_TYPE_PERSISTENT
* ApiProperties.DEFAULT_SOCKET_TYPE (SOCKET_TYPE_PERSISTENT)
*/
private byte socketType = ApiProperties.DEFAULT_SOCKET_TYPE;
/**
* clientID is a String variable whose value is used to identify a connection.
* This value can either be created by the client application or, if not
* specified (that is, the string is set to 1 to 8 blanks,) or if
* setGenerateClientID is set to "true," the clientID will be assigned by IMS
* Connect.
*/
private String clientId = ApiProperties.DEFAULT_CLIENTID;
/*** SSL properties ***/
/**
* sslEncryptionType is the Encryption Type for the SSL connection. It can have a value of
* "Strong" for strong encryption, i.e encryption with ciphers that have large key
* sizes or it can have a value of "Weak" for weak encryption with ciphers that have
* small key sizes, or "none" for no encryption.
*/
private byte sslEncryptionType = ApiProperties.DEFAULT_SSL_ENCRYPTIONTYPE;
/**
* sslKeystoreInputStream is an InputStream which wraps a keystore file that
* contains keys required during an SSL handshake. It usually holds public
* keys or certificates but it can also be used to store private keys and
* trusted certificates for the client. When TmInteraction.execute() is
* called, if a value is specified for the sslKeystoreName, the
* sslKeystoreUrl and the sslKeystoreInputStream, the sslKeystoreInputStream
* value takes precedence and will be used by the Connect API to load the
* keystore. If the sslKeystoreInputStream value is null, the sslKeystoreUrl
* value, if non-null, will be used. Only if both the sslKeystoreInputStream
* and sslKeystoreUrl values are null will the sslKeystoreName be used by the
* Connect API to load the keystore.
*/
private InputStream sslKeystoreInputStream = ApiProperties.DEFAULT_SSL_KEYSTORE_INPUT_STREAM;
/**
* sslKeystoreUrl is a URL that wraps a keystore file which contains keys
* required during an SSL handshake. It usually holds public keys or
* certificates but it can also be used to store private keys and trusted
* certificates client. When TmInteraction.execute() is called, if a value
* is specified for the sslKeystoreName, the sslKeystoreUrl and the
* sslKeystoreInputStream, the sslKeystoreInputStream value takes precedence
* and will be used by the Connect API to load the keystore. If the
* sslKeystoreInputStream value is null, the sslKeystoreUrl value, if
* non-null, will be used. Only if both the sslKeystoreInputStream and
* sslKeystoreUrl values are null will the sslKeystoreName be used by the
* API to load the keystore.
*/
private URL sslKeystoreUrl = ApiProperties.DEFAULT_SSL_KEYSTORE_URL;
/**
* sslKeystoreName is the filename (qualified or un-qualified) of a keyStore
* which contains keys required during the SSL handshake. It usually holds
* public keys or certificates but can also be used to store private keys
* and trusted certificates for the client. When TmInteraction.execute() is
* called, if a value is specified for the sslKeystoreName, the
* sslKeystoreUrl and the sslKeystoreInputStream, the sslKeystoreInputStream
* value takes precedence and will be used by the Connect API to load the
* keystore. If the sslKeystoreInputStream value is null, the sslKeystoreUrl
* value, if non-null, will be used. Only if both the sslKeystoreInputStream
* and sslKeystoreUrl values are null will the sslKeystoreName be used by the
* API to load the keystore.
*/
private String sslKeystoreName = ApiProperties.DEFAULT_SSL_KEYSTORE_NAME;
/**
* sslKeystorePassword is the password for the keyStore file which contains keys.
*/
private String sslKeystorePassword = ApiProperties.DEFAULT_SSL_KEYSTORE_PASSWORD;
/**
* sslTruststoreInputStream is an InputStream that wraps a truststore file which
* contains keys required during an SSL handshake. It usually holds private or
* trusted keys or certificates, but can also be used to store public keys and
* and certificates for the client. When TmInteraction.execute() is called, if
* a value is specified for the sslKeystoreName, the sslKeystoreUrl and the
* sslKeystoreInputStream, the sslKeystoreInputStream value takes precedence and
* will be used by the Connect API to load the keystore. If the
* sslKeystoreInputStream value is null, the sslKeystoreUrl value, if non-null,
* will be used. Only if both the sslKeystoreInputStream and sslKeystoreUrl
* values are null will the sslKeystoreName be used by the API to load the
* keystore.
*/
private InputStream sslTruststoreInputStream = ApiProperties.DEFAULT_SSL_TRUSTSTORE_INPUT_STREAM;
/**
* sslTruststoreUrl is a URL which wraps a truststore file that contains keys
* required during an SSL handshake. It usually holds private or trusted keys or
* certificates, but it can also be used to store private keys for the client. If
* a value is specified for the sslTruststoreName, the sslTruststoreUrl and the
* sslTruststoreInputStream, the sslTruststoreInputStream value takes precedence and
* will be used by the Connect API to load the truststore. If the
* sslTruststoreInputStream value is null, the sslTruststoreUrl value, if non-null,
* will be used. Only if both the sslTruststoreInputStream and sslTruststoreUrl
* values are null will the sslTruststoreName be used.
*/
private URL sslTruststoreUrl = ApiProperties.DEFAULT_SSL_TRUSTSTORE_URL;
/**
* sslTruststoreName is the filename (qualified or un-qualified) of a keystore which
* contains keys required during an SSL handshake. It usually holds private or
* trusted keys or certificates, but it can also be used to store private keys for
* the client. If a value is specified for the sslTruststoreName, the
* sslTruststoreUrl and the sslTruststoreInputStream, the sslTruststoreInputStream
* value takes precedence and will be used by the Connect API to load the
* truststore. If the sslTruststoreInputStream value is null, the sslTruststoreUrl
* value, if non-null, will be used. Only if both the sslTruststoreInputStream and
* sslTruststoreUrl values are null will the sslTruststoreName be used.
*/
private String sslTruststoreName = ApiProperties.DEFAULT_SSL_TRUSTSTORE_NAME;
/**
* sslTruststorePassword is the password for the keyStore file which contains
* keys for trusted entities.
*/
private String sslTruststorePassword = ApiProperties.DEFAULT_SSL_TRUSTSTORE_PASSWORD;
/**
* useSslConnection is a Boolean variable whose value indictes whether or not this
* connection is an SSL connection. A true value indicates that this connection is
* or will be an SSL connection while a false value indicates that SSL is not being
* or will not be used.
*/
private boolean useSslConnection = ApiProperties.DEFAULT_USE_SSL_CONNECTION;
/*** Timeout properties ***/
/*
* The value of interactionTimeout will be set automatically during an TmInteraction.execute()
* call to the value of the interactionTimeout property of that TmInteraction instance. This
* value will be used to control the amount of time that the API will wait for a response from
* IMS Connect for interactions such as a resumeTpipe or receive interaction. Note that a
* receive interaction is invoked internally as part of a sendreceive interaction.
*/
private int interactionTimeout = ApiProperties.DEFAULT_INTERACTION_TIMEOUT;
/*
* The value of socketConnectTimeout will be used internally by the API during a
* Connection.connect() call set the time that TCP/IP should wait for a socket connect request
* to complete successfully. However it should be noted that this value is used by TCP/IP in
* conjunction with the TCP/IP maximum socket connect retries value (which may or may not be
* user-customizable depending on runtime platform) to determine the amount of time that
* TCP/IP will actually wait for a socket connect request to complete successfully. Whichever
* event occurs first, the socket connect timeout or the maximum socket connect retries
* exceeded will determine when the connect attemp will be aborted by TCP/IP and an error
* returned to the calling program.
*/
private int socketConnectTimeout = ApiProperties.DEFAULT_SOCKET_CONNECT_TIMEOUT;
/*** Connection management properties ***/
/**
* useConnectionManager is a boolean variable whose value determines whether
* the IMS Connect API runtime instantiates a Connection Manager to manage
* connection pools.
*/
// private boolean useConnectionPoolManager = ApiProperties.DEFAULT_USE_CONNECTION_POOL_MANAGER;
/*** Other properties ***/
String validChars = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890-";
String validLetters = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ";
/**
* Default constructor.
* @throws IMSConnectAPIException
*/
public ConnectionFactory() throws ImsConnectApiException
{
logger = Logger.getLogger("com.ibm.ims.connect");
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
// if (logger.getLevel().intValue()<=ApiProperties.TRACE_LEVEL_ENTRY_EXIT.intValue())
logger.finer("<-> ConnectionImpl()...");
}
/**
* Create a ConnectionFactory instance using a ConnectionManager
instance.
*
* @param aConnectionManager the ConnectionManager
instance to manage the
* connections created by this ConnectionFactory instance.
*/
/* public ConnectionFactory(ConnectionManager aConnectionManager)
{
this.connectionManager = aConnectionManager;
}
*/
/**
* Creates a ConnectionAttributes
instance containing default values.
* The ConnectionAttributes
instance can be set with user-defined values
* and used to configure the connection properties on ConnectionFactory
or
* Connection
instances.
* Setting the properties by using a ConnectionAttributes
instance this way can be more
* efficient than loading a connection properties text file containing name-value pairs
* or setting the property values programmatically for each ConnectionFactory
* or Connection
instance.
* @return a ConnectionAttributes
instance
* @see Connection
*/
/* public ConnectionAttributes createConnectionAttributes()
{
ConnectionAttributes connAttr = new ConnectionAttributes();
return connAttr;
}
*/
/**
* Obtains a connection to a particular IMS Connect instance.
* A Connection
instance encapsulates a physical connection to IMS Connect and has methods
* to open and close the connection and to send and receive data over that connection.
* @return a Connection
instance
* @see Connection
*/
public Connection getConnection() throws ImsConnectApiException
{
try
{
Connection conn = null;
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
// if (logger.getLevel().intValue()<=ApiProperties.TRACE_LEVEL_ENTRY_EXIT.intValue())
logger.finer("--> ConnectionFactory.getConnection()...");
conn = ((Connection) new ConnectionImpl(this));
return conn;
}
finally
{
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
// if (logger.getLevel().intValue()<=ApiProperties.TRACE_LEVEL_ENTRY_EXIT.intValue())
logger.finer("<-- ConnectionFactory.getConnection()...");
}
}
/**
* Reads in connection property values from a connection properties text file. The
* loaded connection property values will be used to configure new Connection
instances
* that are generated by this ConnectionFactory
.
* @param aConnectionAttributesFileName text file containing the connection properties
* @see Connection
*/
public void loadConnectionFactoryAttributesFromFile(String aConnectionAttributesFileName)
throws Exception
{
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("--> ConnectionFactory.loadConnectionFactoryAttributesFromFile(String)...");
PropertiesFileLoader myPropertiesFileLoader = new PropertiesFileLoader();
myPropertiesFileLoader.loadPropertiesFile(this, aConnectionAttributesFileName);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("<-- ConnectionFactory.loadConnectionFactoryAttributesFromFile(String)...");
}
// Getter and setter methods
/**
* Gets the clientId property value.
* @return the clientId property value as a String
.
* @see Connection#setClientId(String)
*/
public String getClientId()
{
return this.clientId;
}
/**
* Sets the clientId field of this Connection
object, which is used by IMS Connect and IMS
* to identify IMS Connect clients. The clientId is also used for the names of asynchronous message queues
* (OTMA Tpipes) and other internal data structures in IMS Connect and OTMA.
* The clientId value is a string of 1 to 8 uppercase alphanumeric (A through Z, 0 to 9) or special
* (@, #, $) characters, left justified, and padded with blanks.
*
This value can be created by the client application or assigned
* by IMS Connect. If, on the first input message of a transaction, this string is not supplied by the client
* or if the value supplied is all blanks, or if setGenerateClientID is set to true, the clientID will be
* assigned by IMS Connect.
*
The clientID value will only be used by the API and IMS Connect
* while creating a connection. After the connection has been created, the clientID value specified by the
* client for subsequent interactions on that connection including re-use of the connection by other clients
* will be ignored by IMS Connect. The API will pass the specified clientID in the input message but IMS
* Connect will return the correct clientID (the original clientID for that connection) in the response message.
* @param aclientID the clientID to be used for this interaction.
* @see ApiProperties#DEFAULT_CLIENTID
* @throws ImsConnectApiException
*/
public final void setClientId(String aClientId) throws ImsConnectApiException
{
if (PropertiesFileLoader.isValidHostStyleName(aClientId) == true)
this.clientId = aClientId.toUpperCase();
else if (aClientId.trim().equals(""))
this.clientId = " ";
else if (aClientId.length() > 8)
{
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0026E, new Object[] { aClientId,
"clientId",
"8" });
ImsConnectApiException e = new ImsConnectApiException(ImsConnectErrorMessage.HWS0026E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception thrown in ConnectionFactory.setClientId(String). Exception thrown was: " + e.toString());
throw e;
}
else
{
String errMsg = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0029E,
new Object[] {"clientID",
aClientId});
ImsConnectApiException e = new ImsConnectApiException(ImsConnectErrorMessage.HWS0029E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception thrown in Connection.setClientId(String). Exception thrown was: " + e.toString());
throw e;
}
}
/**
* Gets the hostName property value.
* @return the hostName property value as a String
.
* @see Connection#setHostName(String)
*/
public String getHostName()
{
return this.hostName;
}
/**
* Sets the value of the hostName field of this Connection
. The hostName property is a String
* variable whose value is the hostname or IP address of the target IMS Connect for this connection.
*
The input String
must be either:
*
* - a 1 to 8 character
String
set to the correct hostname of the target IMS Connect, or
* - a
String
containing the IP V4 or IP V6 address of the target IMS Connect
* (e.g. "nnn.nnn.nnn.nnn" or "nnn.nnn.nnn.nnn.nnn.nnn").
*
* If a value for this property is not supplied by the client, the default value ("HOSTNAME") will be used.
* @param aHostName the hostName or IP address to be used for this interaction.
* @see ApiProperties#DEFAULT_HOSTNAME
* @throws ImsConnectApiException
*/
public void setHostName(String aHostName) throws ImsConnectApiException
{
int octet;
String[] result;
boolean valid = true;
boolean ipAddress = true;
int i, numberOfNodes;
if (this.hostName.equalsIgnoreCase(aHostName))
{
return;
}
else
{
result = aHostName.split("\\.");
numberOfNodes = result.length;
for (i = 0; i < numberOfNodes; i++)
{
try
{
octet = Integer.parseInt(result[i]);
if ((octet < 0) || (octet > 255))
{
valid = false;
break;
}
}
catch (Exception e)
{
valid = false;
ipAddress = false;
break;
}
}
if ((valid == true) && // nodes are all numbers between 0 and 255, inclusive
(ipAddress == true) && // hostname should be an IP address
((numberOfNodes != 4) && (numberOfNodes != 6))) // it is not a valid IPV4 or IPV6 address
{
valid = false;
}
if (ipAddress == false)
{
char[] charBuf;
int strLen;
if ((aHostName.length() <= 255) && (validLetters.indexOf(aHostName.charAt(0)) != -1))
{
result = aHostName.split("\\.");
if (numberOfNodes <= 127)
{
valid = true;
for (i = 0; i < numberOfNodes; i++)
{
int j;
strLen = result[i].length();
if(strLen > 63)
{
i = numberOfNodes;
j = strLen;
}
charBuf = new char[strLen];
result[i].getChars(0, strLen, charBuf, 0);
for (j = 0; j < strLen; j++)
{
if(validChars.indexOf(charBuf[j]) == -1)
{
valid = false;
i = numberOfNodes;
j = strLen;
}
}
}
}
}
else
valid = false;
}
}
if (valid)
{
this.hostName = aHostName;
}
else
{
String validHostnameString = "";
try
{
validHostnameString = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.VALID_PROPERTY_VALUE_HOSTNAME);
}
catch (Exception e1)
{
}
String errMsg = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0030E,
new Object[] {"hostName",
aHostName,
validHostnameString });
ImsConnectApiException e1 = new ImsConnectApiException(ImsConnectErrorMessage.HWS0030E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception caught in Connection.setHostName(String). Exception caught was: " + e1.toString());
throw e1;
}
}
/**
* Gets the interactionTimeout property value.
* @return the interactionTimeout property value as an int
in milliseconds.
*/
public int getInteractionTimeout()
{
return this.interactionTimeout;
}
/**
* Sets the interactionTimeout field of this Connection
instance and the
* SO_TIMEOUT value of the underlying socket.
*
The interaction timeout value is in milliseconds. A positive non-zero value for
* interactionTimeout causes the SO_TIMEOUT value to be set to that number of milliseconds.
* An interactionTimeout value of -1 disables the SO_TIMEOUT function. If the SO_TIMEOUT
* function is disabled, a socket will block forever or until data available to be read on
* that socket by the IMS Connect API on behalf of the client application.
* @param anInteractionTimeout the interaction timeout value in milliseconds. You can
* specify this parameter by using the constants defined in ApiProperties or by providing
* a valid non-negative integer value.
* @see ApiProperties#TIMEOUT_100_MILLISECONDS
* @see ApiProperties#TIMEOUT_10_MILLISECONDS
* @see ApiProperties#TIMEOUT_10_MINUTES
* @see ApiProperties#TIMEOUT_10_SECONDS
* @see ApiProperties#TIMEOUT_12_HOURS
* @see ApiProperties#TIMEOUT_1_DAY
* @see ApiProperties#TIMEOUT_1_HOUR
* @see ApiProperties#TIMEOUT_1_MILLISECOND
* @see ApiProperties#TIMEOUT_1_MINUTE
* @see ApiProperties#TIMEOUT_1_SECOND
* @see ApiProperties#TIMEOUT_1_WEEK
* @see ApiProperties#TIMEOUT_200_MILLISECONDS
* @see ApiProperties#TIMEOUT_20_MILLISECONDS
* @see ApiProperties#TIMEOUT_2_DAYS
* @see ApiProperties#TIMEOUT_2_HOURS
* @see ApiProperties#TIMEOUT_2_MILLISECONDS
* @see ApiProperties#TIMEOUT_2_MINUTES
* @see ApiProperties#TIMEOUT_2_SECONDS
* @see ApiProperties#TIMEOUT_2_WEEKS
* @see ApiProperties#TIMEOUT_30_MINUTES
* @see ApiProperties#TIMEOUT_30_SECONDS
* @see ApiProperties#TIMEOUT_45_SECONDS
* @see ApiProperties#TIMEOUT_45_MINUTES
* @see ApiProperties#TIMEOUT_4_HOURS
* @see ApiProperties#TIMEOUT_500_MILLISECONDS
* @see ApiProperties#TIMEOUT_50_MILLISECONDS
* @see ApiProperties#TIMEOUT_5_DAYS
* @see ApiProperties#TIMEOUT_5_MILLISECONDS
* @see ApiProperties#TIMEOUT_5_MINUTES
* @see ApiProperties#TIMEOUT_5_SECONDS
* @see ApiProperties#TIMEOUT_8_HOURS
* @see ApiProperties#INTERACTION_TIMEOUT_MAX
* @see ApiProperties#TIMEOUT_WAIT_FOREVER
* @throws ImsConnectCommunicationException
*/
public void setInteractionTimeout(int anInteractionTimeout) throws ImsConnectApiException
{
if(anInteractionTimeout <= 0 && anInteractionTimeout != -1)
{
String errMsg = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0007E,
new Object[] {"ConnectionFactory.setInteractionTimeout(int)", String.valueOf(anInteractionTimeout)});
ImsConnectApiException e = new ImsConnectApiException(ImsConnectErrorMessage.HWS0007E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception thrown in Connection.setInteractionTimeout(int). Exception thrown was: " + e.toString());
throw e;
}
else
{
this.interactionTimeout = anInteractionTimeout;
}
}
/**
* Gets the portNumber property value.
* @return the portNumber value.
* @see Connection#setPortNumber(int)
*/
public int getPortNumber()
{
return this.portNumber;
}
/**
* Sets the value of the portNumber field of this Connection object. The portNumber property specifies
* a TCP/IP port where the target IMS Connect is listening for requests for
* new connections from clients.
*
If a value for this property is not supplied by the client, the default value (9999) will be used.
* @param aPortNumber a valid port number on which the target IMS Connect is listening for incoming
* requests from IMS Connnect clients.
* @see ApiProperties#DEFAULT_PORTNUMBER
* @throws ImsConnectApiException
*/
public void setPortNumber(int aPortNumber) throws ImsConnectApiException
{
if(aPortNumber > 0 && aPortNumber <= 65535)
if(this.getPortNumber() == aPortNumber)
return;
else
{
this.portNumber = aPortNumber;
}
else
{
String validPortNumberString = "";
try
{
validPortNumberString = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.VALID_PROPERTY_VALUE_PORTNUMBER);
}
catch (Exception e1)
{
}
String errMsg = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0030E,
new Object[] {"portNumber",
String.valueOf(aPortNumber),
validPortNumberString });
ImsConnectApiException e = new ImsConnectApiException(ImsConnectErrorMessage.HWS0030E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception thrown in Connection.setPortNumber(int). Exception thrown was: " + e.toString());
throw e;
}
}
/**
* Gets the socketConnectTimeout property value.
* @return the socketConnectTimeout
*/
public int getSocketConnectTimeout()
{
return this.socketConnectTimeout;
}
/**
* Sets the socketConnectTimeout field of this Connection
.
*
The socketConnectTimeout determines the amount of time that the IMS Connect API will wait for
* a socket.connect() call to complete. Only one value can be specified per socket.
*
The valid input values, for some of which, constants are defined in ApiProperties, are:
*
* - -1 - API will wait forever (or until the connect request times out due to exceeding the maximum
* number of connection retry attempts defined in the TCP/IP properties.)
*
- > 0 - API will wait either until the maximum number of connection retry attempts defined in the
* TCP/IP properties has been attempted or until the socketConnectTimeout period has elapsed before
* throwing a java.net.SocketTimeoutException.
*
* Specifying a value less than -1 will result in an ImsConnectCommunicationException being thrown
* back to the client application. It should be noted that the actual timeout may be affected by the
* maximum number of connect retries allowed by TCP/IP. If the maximum number of connect retries is
* exceeded before the timeout pops, an exception will be thrown by TCP/IP.
* @param aSocketConnectTimeout the socketConnectTimeout to set in milliseconds
* @see ApiProperties#DEFAULT_SOCKET_CONNECT_TIMEOUT
* @see ApiProperties#TIMEOUT_100_MILLISECONDS
* @see ApiProperties#TIMEOUT_10_MILLISECONDS
* @see ApiProperties#TIMEOUT_10_MINUTES
* @see ApiProperties#TIMEOUT_10_SECONDS
* @see ApiProperties#TIMEOUT_12_HOURS
* @see ApiProperties#TIMEOUT_1_DAY
* @see ApiProperties#TIMEOUT_1_HOUR
* @see ApiProperties#TIMEOUT_1_MILLISECOND
* @see ApiProperties#TIMEOUT_1_MINUTE
* @see ApiProperties#TIMEOUT_1_SECOND
* @see ApiProperties#TIMEOUT_1_WEEK
* @see ApiProperties#TIMEOUT_200_MILLISECONDS
* @see ApiProperties#TIMEOUT_20_MILLISECONDS
* @see ApiProperties#TIMEOUT_2_DAYS
* @see ApiProperties#TIMEOUT_2_HOURS
* @see ApiProperties#TIMEOUT_2_MILLISECONDS
* @see ApiProperties#TIMEOUT_2_MINUTES
* @see ApiProperties#TIMEOUT_2_SECONDS
* @see ApiProperties#TIMEOUT_2_WEEKS
* @see ApiProperties#TIMEOUT_30_MINUTES
* @see ApiProperties#TIMEOUT_30_SECONDS
* @see ApiProperties#TIMEOUT_45_SECONDS
* @see ApiProperties#TIMEOUT_45_MINUTES
* @see ApiProperties#TIMEOUT_4_HOURS
* @see ApiProperties#TIMEOUT_500_MILLISECONDS
* @see ApiProperties#TIMEOUT_50_MILLISECONDS
* @see ApiProperties#TIMEOUT_5_DAYS
* @see ApiProperties#TIMEOUT_5_MILLISECONDS
* @see ApiProperties#TIMEOUT_5_MINUTES
* @see ApiProperties#TIMEOUT_5_SECONDS
* @see ApiProperties#TIMEOUT_8_HOURS
* @see ApiProperties#INTERACTION_TIMEOUT_MAX
* @see ApiProperties#TIMEOUT_WAIT_FOREVER
* @throws ImsConnectCommunicationException
*/
public void setSocketConnectTimeout(int aSocketConnectTimeout) throws ImsConnectApiException
{
if (aSocketConnectTimeout > 0 || aSocketConnectTimeout == -1)
this.socketConnectTimeout = aSocketConnectTimeout;
else
{
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0007E, new Object[] {"ConnectionFactory.setSocketConnectTimeout(int)", aSocketConnectTimeout});
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0007E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception thrown in Connection.setSocketConnectTimeout(). Exception thrown was: " + e.toString());
throw e;
}
}
/**
* Gets the socketType property value.
* @return the socketType value.
* @see ApiProperties#SOCKET_TYPE_PERSISTENT
* @see ApiProperties#SOCKET_TYPE_TRANSACTION
*/
public byte getSocketType()
{
return this.socketType;
}
/**
* Sets the socketType field of this Connection
.
*
The type of socket connection of a message is set by the client in the initial input message and
* maintained in the message until the interaction has completed. Only one value can be specified per
* interaction.
*
The valid input values, for which the following constants are defined in ApiProperties, are:
*
* - ApiProperties.SOCKET_TYPE_TRANSACTION = 0x00. A transaction socket can only be disconnected by IMS Connect.
* Transaction sockets are used for one transaction (including and required ACKs or NAKs) for non-conversational
* transactions or for one complete conversation (until the conversation is ended by the client or host application)
* for conversational transactions. Once the non-conversational IMS transaction interaction or IMS conversation
* has completed, the transaction socket is automatically closed by both IMS Connect and the API. The
* transaction socket also closes automatically after IMS itself has terminated or after an error has occured.
*
- ApiProperties.SOCKET_TYPE_PERSISTENT = 0x10. A persistent socket is left connected by IMS Connect until a fatal error
* has occured on that connection while IMS Connect is processing an interaction, or until a disconnect request
* has been received by IMS Connect. Any open persistent connections will be closed automatically by the API
* when the client application ends.
*
- ApiProperties.DEFAULT_SOCKET_TYPE (same as ApiProperties.SOCKET_TYPE_PERSISTENT)
*
* If a value for this property is not supplied by the client, the default value (SOCKET_TYPE_PERSISTENT)
* will be used.
* @param aSocketType the socket type to be used for this interaction. Use the constants defined
* in ApiProperties to specify this parameter.
* @see ApiProperties#DEFAULT_SOCKET_TYPE
* @see ApiProperties#SOCKET_TYPE_PERSISTENT
* @see ApiProperties#SOCKET_TYPE_TRANSACTION
* @throws ImsConnectApiException
*/
public void setSocketType(byte aSocketType) throws ImsConnectApiException
{
if(this.getSocketType() == aSocketType)
return;
else
{
this.socketType = aSocketType;
}
}
/**
* Returns the SSL encryption type property.
* @return the sslEncryptionType.
*/
public byte getSslEncryptionType()
{
return this.sslEncryptionType;
}
/**
* Sets the sslEncryptionType field of this ConnectionFactory
. The sslEncryptionType
* property is a byte value used to set the encryption type for the underlying connection when that
* connnection is an SSL connection.
*
The valid values, for which the following constants are defined in ApiProperties
, are:
*
* - ApiProperties.SSL_ENCRYPTIONTYPE_STRONG = 0x02
*
- ApiProperties.SSL_ENCRYPTIONTYPE_WEAK = 0x01
*
- ApiProperties.SSL_ENCRYPTYPE_NONE = 0x00
*
- ApiProperties.DEFAULT_SSL_ENCRYPTIONTYPE (same as ApiProperties.SSL_ENCRYPTIONTYPE_WEAK)
*
* If a value for this property is not supplied by the client, the default value (SSL_ENCRYPTIONTYPE_WEAK)
* will be used.
*
ApiProperties.SSL_ENCRYPTIONTYPE_STRONG and ApiProperties.SSL_ENCRYPTIONTYPE_WEAK reflect the strength of the cipher used which
* is related to the key length. All those ciphers that can be used for export are in the
* ApiProperties.SSL_ENCRYPTIONTYPE_WEAK category and the rest go into the strong category. The IMS Connect API will
* negotiate with IMS Connect to use a strong (greater than 64-bit encryption key) or weak (64-bit
* or smaller encryption key) cipher specification accordingly if either of these two settings are specified.
*
When ApiProperties.SSL_ENCRYPTIONTYPE_NONE is specified, the IMS Connect API will negotiate with IMS Connect to
* use a cipher spec whose name contains the string "NULL". Null encryption will allow for
* authentication to take place during the SSL handshaking process. Once the handshaking process for a
* socket has completed including authentication as required, all messages will flow in the clear (without
* encryption) over that socket.
* @param anSslEncryptionType the sslEncryptionType to be used for this interaction. Use the constants defined
* in ApiProperties to specify this parameter.
* @see ApiProperties#DEFAULT_SSL_ENCRYPTIONTYPE
* @see ApiProperties#SSL_ENCRYPTIONTYPE_NONE
* @see ApiProperties#SSL_ENCRYPTIONTYPE_STRONG
* @see ApiProperties#SSL_ENCRYPTIONTYPE_WEAK
* @throws ImsConnectApiException
*/
public void setSslEncryptionType(byte anSslEncryptionType) throws ImsConnectApiException
{
if(this.getSslEncryptionType() == anSslEncryptionType)
return;
else
{
this.sslEncryptionType = anSslEncryptionType;
}
}
/**
* Gets the input stream for the SSL key store.
* @return the sslKeystoreInputStream
*/
public InputStream getSslKeystoreInputStream()
{
return this.sslKeystoreInputStream;
}
/**
* Sets the sslKeystoreInputStream field of this ConnectionFactory
.
*
An SSL keystore is a password-protected database intended to contain private key
* material, such as private keys and their associated private key certificates. The private key
* in your keystore is used for encrypting/signing outgoing messages that can then only be
* decrytped using your public key which you have distributed to your partners. For your
* convenience, the IMS Connect API allows both private keys (and their associated private key
* certificates) as well as trusted certificates (usually stored in your truststore) to be stored
* in the same keystore in which case the sslTruststoreInputStream, sslTruststoreUrl and/or
* sslTruststoreName property can either be null or can point to the keystore InputStream, URL or
* file, respectively. Likewise, private keys or certificates could be stored in the truststore
* along with your trusted certificates, in which case the sslKeystoreInputStream, sslKeystoreUrl
* and/or sslKeystoreName properties could either be null or could point to the same or a different
* keystore or truststore InputStream, URL or file, respectively.
*
The sslKeystoreInputStream property can be used to specify an InputStream that wraps a JKS
* keystore file. When TmInteraction.execute() is called, if a value is specified for the
* sslKeystoreName, the sslKeystoreUrl and the sslKeystoreInputStream, the sslKeystoreInputStream
* value takes precedence and will be used by the Connect API to load the keystore. If the
* sslKeystoreInputStream value is null, the sslKeystoreUrl value, if non-null, will be used. Only
* when both the sslKeystoreInputStream and sslKeystoreUrl values are null, will the sslKeystoreName
* be used. If a value for this property is not set by the client application, the default value
* (null) will be used for this property.
*
It is important to note that an InputStream, because it does not support the mark or reset
* features, can only be used once. In fact, due to this restriction, the API internally closes an
* InputStream after it has loaded the keystore from that InputStream.
* @param anSslKeystoreInputStream a java.io.InputStream
to be used to set the
* sslKeystoreInputStream value for this interaction
* @see ApiProperties#DEFAULT_SSL_KEYSTORE_INPUT_STREAM
* @see Connection#setSslKeystoreName(String anSslKeystoreName)
*/
public void setSslKeystoreInputStream(InputStream anSslKeystoreInputStream)
{
this.sslKeystoreInputStream = anSslKeystoreInputStream;
}
/**
* Gets the URL for the SSL key store.
* @return the sslKeystoreUrl
*/
public URL getSslKeystoreUrl()
{
return this.sslKeystoreUrl;
}
/**
* Sets the sslKeystoreUrl field of this ConnectionFactory
.
*
An SSL keystore is a password-protected database intended to contain private key
* material, such as private keys and their associated private key certificates. The private key
* in your keystore is used for encrypting/signing outgoing messages that can then only be
* decrytped using your public key which you have distributed to your partners. For your
* convenience, the IMS Connect API allows both private keys (and their associated private key
* certificates) as well as trusted certificates (usually stored in your truststore) to be stored
* in the same keystore in which case the sslTruststoreInputStream, sslTruststoreUrl and/or
* sslTruststoreName property can either be null or can point to the keystore InputStream, URL or
* file, respectively. Likewise, private keys or certificates could be stored in the truststore
* along with your trusted certificates, in which case the sslKeystoreInputStream, sslKeystoreUrl
* and/or sslKeystoreName properties could either be null or could point to the same or a different
* keystore or truststore InputStream, URL or file, respectively.
*
The sslKeystoreUrl property can be used to specify a URL that wraps a JKS keystore file. When
* TmInteraction.execute() is called, if a value is specified for the sslKeystoreName, the
* sslKeystoreUrl and the sslKeystoreInputStream, the sslKeystoreInputStream value takes precedence
* and will be used by the Connect API to load the keystore. If the sslKeystoreInputStream value is
* null, the sslKeystoreUrl value, if non-null, will be used. Only when both the
* sslKeystoreInputStream and sslKeystoreUrl values are null, will the sslKeystoreName be used. If
* a value for this property is not set by the client application, the default value (null) will be
* used for this property.
* @param anSslKeystoreUrl a java.net.URL
to be used to set the sslKeystoreUrl value for
* this interaction
* @see ApiProperties#DEFAULT_SSL_KEYSTORE_URL
* @see ConnectionFactory#setSslKeystoreName(String anSslKeystoreName)
*/
public void setSslKeystoreUrl(URL anSslKeystoreUrl)
{
this.sslKeystoreUrl = anSslKeystoreUrl;
}
/**
* Returns the SSL keystore name as a String
.
* @return the sslKeystoreName.
*/
public String getSslKeystoreName()
{
return this.sslKeystoreName;
}
/**
* Sets the sslKeystoreName field of this ConnectionFactory
.
*
An SSL keystore is a password-protected database intended to contain private key
* material, such as private keys and their associated private key certificates. The private key
* in your keystore is used for encrypting/signing outgoing messages that can then only be
* decrytped using your public key which you have distributed to your partners. For your
* convenience, the IMS Connect API allows both private keys (and their associated private key
* certificates) as well as trusted certificates (usually stored in your truststore) to be stored
* in the same keystore in which case the sslTruststoreInputStream, sslTruststoreUrl and/or
* sslTruststoreName property can either be null or can point to the keystore InputStream, URL or
* file, respectively. Likewise, private keys or certificates could be stored in the truststore
* along with your trusted certificates, in which case the sslKeystoreInputStream, sslKeystoreUrl
* and/or sslKeystoreName properties could either be null or could point to the same or a different
* keystore or truststore InputStream, URL or file, respectively.
*
The sslKeystoreName property can be used to specify either a JKS keystore or, when running on
* z/OS, a RACF keyring. For non-z/OS platforms, specify the fully-qualified path name of your JKS
* keystore file. For z/OS, you can specify either the name of your JKS keystore file or a special
* string that provides the information needed to access your RACF keyring.
*
An example of a fully-qualified path name of your JKS keystore file is "c:\keystore\MyKeystore.ks".
*
A RACF keyring is specified as: "keystore_type:keyring_name:racfid". The keystore_type must be
* either JCERACFKS when software encryption is used for SSL or JCE4758RACFKS if hardware encryption
* is used. Replace keyring_name with the name of the RACF keyring that you are using as your
* keystore and racfid with a RACF ID that is authorized to access the specified keyring. Examples of
* RACF keyring specifications are "JCERACFKS:myKeyring:kruser01" or "JCE4758RACFKS:myKeyring:kruser01".
*
When running on z/OS, if the sslKeystoreName matches the above RACF keyring format, the IMS
* Connect API will use the specified RACF keyring as its keystore. If the keystore type specified is
* anything other than JCERACFKS or JCE4758RACFKS, the IMS Connect API attempts to interpret the
* sslKeystoreName specified as the name and location of a JKS keystore file.
*
When TmInteraction.execute() is called, if a value is specified for the sslKeystoreName, the
* sslKeystoreUrl and the sslKeystoreInputStream, the sslKeystoreInputStream value takes precedence
* and will be used by the Connect API to load the keystore. If the sslKeystoreInputStream value is
* null, the sslKeystoreUrl value, if non-null, will be used. Only when both the
* sslKeystoreInputStream and sslKeystoreUrl values are null, will the sslKeystoreName be used. If
* a value for this property is not supplied by the client, nor are values supplied for the
* sslKeystoreInputStream or sslKeystoreUrl, the default value from the ApiProperties.java file
* (DEFAULT_SSL_KEYSTORE_NAME = "c:\\MySSLKeystores\\myKeystore.ks") will be used.
*
Note that the JKS keystore file can have other file extensions; it does not have to use the
* ".ks" file name extension.
* @param anSslKeystoreName a java.lang.String
containing the name of the JKS keystore file to be
* used for this interaction
* @see ApiProperties#DEFAULT_SSL_KEYSTORE_NAME
* @throws ImsConnectApiException
*/
public void setSslKeystoreName(String anSslKeystoreName) throws ImsConnectApiException
{
if(this.getSslKeystoreName() == anSslKeystoreName)
return;
else
{
this.sslKeystoreName = anSslKeystoreName;
}
}
/**
* Returns the SSL keystore password as a String
.
* @return the sslKeystorePassword.
*/
public String getSslKeystorePassword()
{
return this.sslKeystorePassword;
}
/**
* Sets the sslKeystorePassword field of this ConnectionFactory
. The
* sslKeystorePassword specifies the password for the JKS keystore pointed to by
* sslKeystoreInputStream, sslKeystoreUrl or sslKeystoreName.
*
If a value for this property is not supplied by the client, the default value
* ("keystrPw") will be used.
* @param anSslKeystorePassword a String
specifying the SSL keystore
* password to be used for this interaction
* @see ApiProperties#DEFAULT_SSL_KEYSTORE_PASSWORD
* @throws ImsConnectApiException
*/
public void setSslKeystorePassword(String anSslKeystorePassword) throws ImsConnectApiException
{
if(this.getSslKeystoreName() == anSslKeystorePassword)
return;
else
{
this.sslKeystorePassword = anSslKeystorePassword;
}
}
/**
* Gets the input stream for the SSL trust store.
* @return the sslTruststoreInputStream
*/
public InputStream getSslTruststoreInputStream()
{
return this.sslTruststoreInputStream;
}
/**
* Sets the sslTruststoreInputStream field of this ConnectionFactory
.
*
An SSL truststore is a password-protected database intended to contain certificates of
* trusted certificate authorities. These certificates are used to validate the authenticity of a
* public key certificate during the handshaking process. For your convenience, the IMS Connect
* API allows both private key material as well as the trusted certificates usually stored in a
* truststore to be stored in the same truststore, in which case the sslKeystoreInputStream,
* sslKeytstoreUrl and/or sslKeystoreName properties can either be null or can point to the
* truststore InputStream, URL or file, respectively. Likewise, both private key material and
* trusted certificates could be stored in the same keystore, in which case the
* sslTruststoreInputStream, sslTruststoreUrl and/or sslTruststoreName properties could either be
* null or could point to the same or a different keystore or truststore InputStream, URL or file,
* respectively.
*
The sslTruststoreInputStream property can be used to specify an InputStream that wraps a JKS
* keystore file which will be used as a keystore which contains trusted certificates. When
* TmInteraction.execute() is called, if a value is specified for the sslTruststoreName, the
* sslTruststoreUrl and the sslTruststoreInputStream, the sslTruststoreInputStream value takes
* precedence and will be used by the Connect API to load the truststore. If the
* sslTruststoreInputStream value is null, the sslTruststoreUrl value, if non-null, will be used.
* Only when both the sslTruststoreInputStream and sslTruststoreUrl values are null, will the
* sslTruststoreName be used. If a value for this property is not supplied by the client, the
* default value (null) will be used for this property.
*
It is important to note that an InputStream, because it does not support the mark or reset
* features, can only be used once. In fact, due to this restriction, the API internally closes an
* InputStream after it has loaded the truststore from that InputStream.
* @param anSslTruststoreInputStream an java.io.InputStream
specifying the SSL truststore
* to be used for this interaction.
* @see ApiProperties#DEFAULT_SSL_TRUSTSTORE_INPUT_STREAM
* @see Connection#setSslKeystoreName(String anSslKeystoreName)
*/
public void setSslTruststoreInputStream(InputStream anSslTruststoreInputStream)
{
this.sslTruststoreInputStream = anSslTruststoreInputStream;
}
/**
* Gets the URL of the SSL truststore.
* @return the sslTruststoreUrl
*/
public URL getSslTruststoreUrl()
{
return this.sslTruststoreUrl;
}
/**
* Sets the sslTruststoreUrl field of this ConnectionFactory
.
*
An SSL truststore is a password-protected database intended to contain certificates of
* trusted certificate authorities. These certificates are used to validate the authenticity of a
* public key certificate during the handshaking process. For your convenience, the IMS Connect
* API allows both private key material as well as the trusted certificates usually stored in a
* truststore to be stored in the same truststore, in which case the sslKeystoreInputStream,
* sslKeytstoreUrl and/or sslKeystoreName properties can either be null or can point to the
* truststore InputStream, URL or file, respectively. Likewise, both private key material and
* trusted certificates could be stored in the same keystore, in which case the
* sslTruststoreInputStream, sslTruststoreUrl and/or sslTruststoreName properties could either be
* null or could point to the same or a different keystore or truststore InputStream, URL or file,
* respectively.
*
The sslTruststoreUrl property can be used to specify a URL that wraps a JKS keystore file.
* When TmInteraction.execute() is called, if a value is specified for the sslTruststoreName, the
* sslTruststoreUrl and the sslTruststoreInputStream, the sslTruststoreInputStream value takes
* precedence and will be used by the Connect API to load the truststore. If the
* sslTruststoreInputStream value is null, the sslTruststoreUrl value, if non-null, will be used.
* Only when both the sslTruststoreInputStream and sslTruststoreUrl values are null, will the
* sslTruststoreName be used. If a value for this property is not supplied by the client, the
* default value (null) will be used for this property.
* @param anSslTruststoreUrl a java.net.URL
pointing to the SSL truststore to be used
* for this interaction.
* @see ApiProperties#DEFAULT_SSL_TRUSTSTORE_URL
* @see Connection#setSslKeystoreName(String anSslKeystoreName)
*/
public void setSslTruststoreUrl(URL anSslTruststoreUrl)
{
this.sslTruststoreUrl = anSslTruststoreUrl;
}
/**
* Returns the SSL truststore name as a String
.
* @return the sslTruststoreName.
*/
public String getSslTruststoreName()
{
return this.sslTruststoreName;
}
/**
* Sets the sslTruststoreName field of this ConnectionFactory
.
*
An SSL truststore is a password-protected database intended to contain certificates of
* trusted certificate authorities. These certificates are used to validate the authenticity of a
* public key certificate during the handshaking process. For your convenience, the IMS Connect
* API allows both private key material as well as the trusted certificates usually stored in a
* truststore to be stored in the same truststore, in which case the sslKeystoreInputStream,
* sslKeytstoreUrl and/or sslKeystoreName properties can either be null or can point to the
* truststore InputStream, URL or file, respectively. Likewise, both private key material and
* trusted certificates could be stored in the same keystore, in which case the
* sslTruststoreInputStream, sslTruststoreUrl and/or sslTruststoreName properties could either be
* null or could point to the same or a different keystore or truststore InputStream, URL or file,
* respectively.
*
The same format is used for the values of the sslKeystoreName and sslTruststoreName properties.
* See the description of {@link ConnectionFactory#setSslKeystoreName(String)} for a discussion of
* this format. The sslTruststoreName property can either be empty (all blanks) or can point to the
* keystore file.
*
The sslTruststoreName property can be used to point to a JKS trusted keystore file.
* When TmInteraction.execute() is called, if a value is specified for the sslTruststoreName, the
* sslTruststoreUrl and the sslTruststoreInputStream, the sslTruststoreInputStream value takes
* precedence and will be used by the Connect API to load the truststore. If the
* sslTruststoreInputStream value is null, the sslTruststoreUrl value, if non-null, will be used.
* Only when both the sslTruststoreInputStream and sslTruststoreUrl values are null, will the
* sslTruststoreName be used.
*
If a value for this property is not supplied by the client, nor are values supplied for the
* sslKeystoreInputStream or sslKeystoreUrl, the default value from the ApiProperties.java file
* (DEFAULT_SSL_KEYSTORE_NAME = "c:\\MySSLKeystores\\myKeystore.ks") will be used.
*
Note that the JKS truststore file can have other file extensions; it does not have to use
* the ".ks" file name extension.
* @param anSslTrustStoreName a java.lang.String
containing the name of the JKS trust
* store file to be used for this interaction
* @see ApiProperties#DEFAULT_SSL_TRUSTSTORE_NAME
* @see Connection#setSslKeystoreName(String anSslKeystoreName)
* @throws ImsConnectApiException
*/
public void setSslTruststoreName(String anSslTruststoreName) throws ImsConnectApiException
{
if(this.getSslTruststoreName() == anSslTruststoreName)
return;
else
{
this.sslTruststoreName = anSslTruststoreName;
}
}
/**
* Returns the SSL trust store password as a String
.
* @return the sslTruststorePassword.
*/
public String getSslTruststorePassword()
{
return this.sslTruststorePassword;
}
/**
* Sets the sslTruststorePassword field of this Connection object which specifies the
* password for the JKS truststore pointed to by sslTruststoreName. This value is ignored if
* sslTruststoreName is null or blanks or if sslTruststoreName points to a RACF keyring. The
* sslTruststorePassword value is the password for the truststore file specified in the
* truststore pointed to by the sslTruststoreName value.
*
If a value for this property is not supplied by the client, the default value
* of "trststPw" will be used.
* @param anSslTruststorePassword the JKS truststore password to be used for this interaction.
* @see ApiProperties#DEFAULT_SSL_TRUSTSTORE_PASSWORD
* @throws ImsConnectApiException
*/
public void setSslTruststorePassword(String anSslTruststorePassword) throws ImsConnectApiException
{
if(this.getSslKeystoreName() == anSslTruststorePassword)
return;
else
{
this.sslTruststorePassword = anSslTruststorePassword;
}
}
/**
* Gets the useSslConnection property value.
* If this method returns true
, the underlying connection is an SSL connection
* or will be when connected.
* @return true
if the connection uses SSL. Otherwise, false
.
*/
public boolean isUseSslConnection()
{
return this.useSslConnection;
}
/**
* Configures this Connection
instance to connect to IMS Connect using an SSL connection.
* A true
value sets the connection as an SSL connection, while a false
* value indicates that the connection will be a non-SSL TCP/IP connection.
* If a value for this property is not supplied by the client, the default value of false
will
* be used.
* @param aUseSslConnection
* Set the input to true
to set the connection to be an SSL connection.
* Set the input to false
to set the connection to be an non-SSL connection.
* @see ApiProperties#DEFAULT_USE_SSL_CONNECTION
* @throws ImsConnectApiException
*/
public void setUseSslConnection(boolean aUseSslConnection) throws ImsConnectApiException
{
if(this.isUseSslConnection() == aUseSslConnection)
return;
else
{
this.useSslConnection = aUseSslConnection;
}
}
/**
* @param isStaleConnection The isStaleConnection value to set.
*/
/* protected void setStaleConnection(boolean isStaleConnection)
{
this.staleConnection = isStaleConnection;
}
*/
/**
* Sets the internal connection manager to be enabled or disabled for this interaction.
* @param aUseConnectionPoolManager
* Set the input to true
to enable the internal connection manager.
* Set the input to false
to disable the internal connection manager.
*/
/* public void setUseConnectionPoolManager(boolean aUseConnectionPoolManager)
{
this.useConnectionPoolManager = aUseConnectionPoolManager;
}
*/
/**
* Validates the input string.
* @param inStr the input String
object to be tested.
* @return true
if the input string is not null
and has a length greater than 0 after leading and
* trailing whitespace is removed. Otherwise, it returns false
.
*/
public boolean hasValue(String inStr)
{
if ((inStr != null) & (inStr.trim().length() > 0))
return true;
else
return false;
}
}