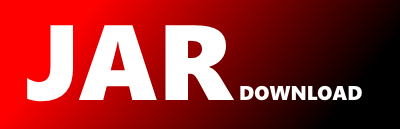
com.ibm.ims.connect.Imsout Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of IMSConnectAPI Show documentation
Show all versions of IMSConnectAPI Show documentation
API that allows Java applications to interface with IMS Connect
/**
* File: Imsout.java
* ==========================================================================
* Licensed Material - Property of IBM
*
* IBM Confidential
*
* OCO Source Materials
*
* 5655-TDA
*
* (C) Copyright IBM Corp. 2011, 2013 All Rights Reserved.
*
* The source code for this program is not published or
* otherwise divested of its trade secrets, irrespective
* of what has been deposited with the U.S. Copyright
* Office.
*
* US Government Users Restricted Rights - Use, duplication or
* disclosure restricted by GSA ADP Schedule Contract with
* IBM Corp.
* ===========================================================================
*/
package com.ibm.ims.connect;
/**
* Represents the imsout
element of a type-2 command response message. This element is returned for
* any Operations Manager (OM) XML response message. The Imsout object contains getter methods for all of the child
* elements and included response data.
*
* @since Enterprise Suite 2.1
*/
public interface Imsout
{
/* response message structure
imsout
ctl
omname?
omvsn?
xmlvsn?
statime
stotime
staseq
stoseq
rqsttkn1?
rqsttkn2?
rc
rsn
rsnmsg?
rsntxt?
uom?
cmdclients?
mbr+
typ
styp
(vsn, jobname) | (rc, rsn, rsntxt)) | msg
vsn?
jobname?
rc?
sn?
rsntxt?
msg?
cmdsyntax?
cmddtd?
cmdtext?
cmderr?
mbr*
(typ, styp, ((vsn, jobname) | (rc, rsn, rsntxt))) | msg
typ?
styp?
vsn?
jobname?
rc?
rsn?
rsntxt?
msg?
cmdsecerr?
exit
rc
userdata
saf
rc
racfrc
racfrsn
cmd
master?
userid?
verb
kwd
input
cmdrsphdr (we used to ignore in API processing since the response is not formatted for display in the API - added for internal user requirement)
hdr* (hdr element is saved as a string which must be parsed by the getter methods)
slbl
llbl
scope
sort
key
scroll
len
dtype
skipb?
align
cmdrspdata (used for IMS and IMS Connect Type2 commands - submitted through OM)
rsp*
msgdata? (used for IMS Type1 commands submitted as Type2 commands through OM)
mbr (only one instance for any type 1 command???)
typ?
styp?
(vsn, jobname) | (rc, rsn, rsntxt)) | msg
vsn?
jobname?
rc?
rsn?
rsntxt?
msg?
Key:
* = 0 or more occurrences
+ = 1 or more occurrences
? = 0 or 1 occurrences
*/
/**
* Gets any plain text encapsulated by the element.
* @return the elementText
*/
public String getElementText();
/**
* Gets the Ctl object that represents the ctl
element of the response message.
* @return the ctl
*/
public Ctl getCtl();
/**
* Gets the Cmdclients object that represents the cmdclients
element of the response message.
* This object contains information about all of the OM command processing clients that are registered
* with the OM that processed a QUERY(CMDCLIENTS) request.
* @return the cmdclients
*/
public Cmdclients getCmdclients();
/**
* Gets the text from the cmdsyntax
element of the response message. This element contains
* the XML definitions for the type-2 commands that each command processing client has registered with OM. This
* element is returned for a QUERY(CMDSYNTAX) request.
* @return the cmdsyntax
*/
public String getCmdsyntax();
/**
* Gets the text from the cmddtd
element of the response message. This element contains the XML
* Document Type Definition (DTD) for OM commands and command responses. This element is returned for a
* QUERY(CMDSYNTAX) request.
* @return the cmddtd
*/
public String getCmddtd();
/**
* Gets the translatable text strings that are associated with the XML command syntax definition.
* This element is returned for a QUERY(CMDSYNTAX) request.
* @return the cmdtext
*/
public String getCmdtext();
/**
* Gets the Cmderr object for the response message from the cmderr
element.
* This object contains information about errors that were returned by OM command processing clients.
* @return the cmderr
*/
public Cmderr getCmderr();
/**
* Gets the Cmdsecerr object for the response message from the cmdsecerr
element.
* This object contains information about command security failures from the OM command security exit routine
* or from the SAF that controls access to the command.
* @return the cmdsecerr
*/
public Cmdsecerr getCmdsecerr();
/**
* Gets the Cmd object for the response message from the cmd
element. This object contains
* information about the original type-2 command that was sent to OM.
* @return the cmd
*/
public Cmd getCmd();
/**
* Gets the Cmdrsphdr object for the response message from the Cmdrsphdr
element. This object
* contains formatting information for the response message.
* @return the cmdrsphdr
*/
public Cmdrsphdr getCmdrsphdr();
/**
* Gets the Cmdrspdata object for the response message from the Cmdrspdata
element. This object
* contains the response message data from all of the command processing clients that successfully processed
* the type-2 command.
* @return the cmdrspdata
*/
public Cmdrspdata getCmdrspdata();
/**
* Gets the Msgdata object for the response message from the msgdata
element. This object
* contains any IMS messages generated by command processing clients while they were processing the command.
* @return the msgdata
*/
public Msgdata getMsgdata();
/*
* Internal method
* @param mbr the mbr to set
*/
public boolean isMbrComplete();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy