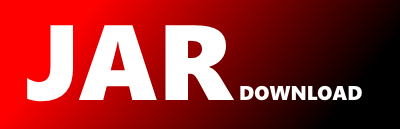
com.ibm.ims.connect.InputMessageProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of IMSConnectAPI Show documentation
Show all versions of IMSConnectAPI Show documentation
API that allows Java applications to interface with IMS Connect
/*
* File: InputMessageProperties.java
* ==========================================================================
* Licensed Material - Property of IBM
*
* IBM Confidential
*
* OCO Source Materials
*
* 5655-TDA
*
* (C) Copyright IBM Corp. 2009,2014 All Rights Reserved.
*
* The source code for this program is not published or
* otherwise divested of its trade secrets, irrespective
* of what has been deposited with the U.S. Copyright
* Office.
*
* US Government Users Restricted Rights - Use, duplication or
* disclosure restricted by GSA ADP Schedule Contract with
* IBM Corp.
* ===========================================================================
*/
package com.ibm.ims.connect;
/**
*
* Input Message Properties
*
*/
public class InputMessageProperties implements ApiProperties
{
public static final String copyright =
"Licensed Material - Property of IBM "
+ "5655-TDA"
+ "(C) Copyright IBM Corp. 2009, 2013 All Rights Reserved. "
+ "US Government Users Restricted Rights - Use, duplication or "
+ "disclosure restricted by GSA ADP Schedule Contract with IBM Corp. ";
public final static short IRM_LEN_DEFAULT = 0;
public final static short IRM_LEN_ARCH0 = 0x58; // (Dec. 88)
public final static short IRM_LEN_ARCH1 = 0x60; // (Dec. 96)
public final static short IRM_LEN_ARCH2 = 0x70; // (Dec. 112)
//For Sync callout Support
public final static short IRM_LEN_ARCH3 = 0xA0; // (Dec. 160)
public final static byte IRM_ARCH0 = (byte) 0x00;
public final static byte IRM_ARCH1 = (byte) 0x01;
public final static byte IRM_ARCH2 = (byte) 0x02;
// For sync callout support
public final static byte IRM_ARCH3 = (byte) 0x03;
public final static byte IRM_F0_NOFLAG = (byte) 0x00;
public final static byte IRM_F0_XML_TD = (byte) 0x01;
public final static byte IRM_F0_XML_D = (byte) 0x02;
public final static String IRM_ID_DEFAULT = EIGHT_BLANKS;
public final static int IRM_ID_LEN = 8;
public final static short IRM_RES_HALFWORD_DEFAULT = 0;
public final static byte IRM_F5_NOFLAG = (byte) 0x00;
public final static byte IRM_F5_OTMA = (byte) 0x80;
public final static byte IRM_F5_TRANS = (byte) 0x40;
public final static byte IRM_F5_SWAIT = (byte) 0x10;
public final static byte IRM_F5_SINGLE = (byte) 0x01;
public final static byte IRM_F5_AUTO = (byte) 0x02;
public final static byte IRM_F5_NOAUTO = (byte) 0x04;
public final static byte IRM_F5_XID = (byte) 0x08;
public final static byte IRM_TIME_QS = (byte) 0x00;
public final static byte IRM_TIME_ZERO = (byte) 0xE9;
public final static byte IRM_TIME_FF = (byte) 0xFF;
public final static byte IRM_SOCT_PER = (byte) 0x10;
public final static byte IRM_SOCT_NPER = (byte) 0x40;
public final static byte IRM_SOCT_TRAN = (byte) 0x00;
public final static byte IRM_ES_NOFLAG = (byte) 0x00;
public final static byte IRM_ES_UTF8 = (byte) 0x01;
public final static byte IRM_ES_UCS2 = (byte) 0x02;
public final static byte IRM_ES_UTF16 = (byte) 0x02;
public final static String IRM_CLIENTID_DEFAULT = EIGHT_BLANKS;
public final static int IRM_CLIENTID_LEN = 8;
public final static byte IRM_F1_NOFLAG = (byte) 0x00;
public final static byte IRM_F1_TRANEXP = (byte) 0x01;
public final static byte IRM_F1_MFSREQ = (byte) 0x80;
public final static byte IRM_F1_CIDREQ = (byte) 0x40;
public final static byte IRM_F1_UC = (byte) 0x20;
public final static byte IRM_F1_UCTC = (byte) 0x10;
public final static byte IRM_F1_CM0ANW = (byte) 0x02;
public final static byte IRM_F2_CMODE0 = (byte) 0x40;
public final static byte IRM_F2_CMODE1 = (byte) 0x20;
public final static byte IRM_F3_SYNC_NONE = (byte) 0x00;
public final static byte IRM_F3_SYNC_CONFIRM = (byte) 0x01;
public final static byte IRM_F3_SYNC_SYNCPT = (byte) 0x02;
public final static byte IRM_F3_REROUTE_FLAG = (byte) 0x08; // himakar
public final static char IRM_F4_DATA = (char) ' ';
public final static char IRM_F4_ACK = (char) 'A';
public final static char IRM_F4_NACK = (char) 'N';
public final static char IRM_F4_CANTIMER = (char) 'C';
public final static char IRM_F4_DEALLOC = (char) 'D';
public final static char IRM_F4_RESUMET = (char) 'R';
public final static char IRM_F4_SENDONLY = (char) 'S';
public final static char IRM_F4_SENDOACK = (char) 'K';
public final static char IRM_F4_SENDRECV = (char) ' ';
//------------------Added for Sync callout------------------------------////
public final static char IRM_F4_SENDONLY_CALLOUT = (char) 'M';
public final static char IRM_F4_SENDONLYACK_CALLOUT = (char) 'L';
public final static String IRM_TRANCODE_DEFAULT = EIGHT_BLANKS;
public final static int IRM_TRANCODE_MAX_LEN = 8;
public final static String IRM_IMSDESTID_DEFAULT = EIGHT_BLANKS;
public final static int IRM_IMSDESTID_MAX_LEN = 8;
public final static String IRM_LTERM_DEFAULT = EIGHT_BLANKS;
public final static int IRM_LTERM_MAX_LEN = 8;
public final static String IRM_RACF_USERID_DEFAULT = EIGHT_BLANKS;
public final static int IRM_RACF_USERID_MAX_LEN = 8;
public final static String IRM_RACF_GRNAME_DEFAULT = EIGHT_BLANKS;
public final static int IRM_RACF_GRNAME_MAX_LEN = 8;
public final static String IRM_RACF_PW_DEFAULT = EIGHT_BLANKS;
public final static int IRM_RACF_PW_MAX_LEN = 8;
public final static String IRM_APPL_NM_DEFAULT = EIGHT_BLANKS;
public final static int IRM_APPL_NM_MAX_LEN = 8;
public final static String IRM_REROUT_NM_DEFAULT = EIGHT_BLANKS;
public final static int IRM_REROUT_NM_MAX_LEN = 8;
public final static String IRM_TAG_ADAPT_DEFAULT = EIGHT_BLANKS;
public final static int IRM_TAG_ADAPT_MAX_LEN = 8;
public final static String IRM_TAG_MAP_DEFAULT = EIGHT_BLANKS;
public final static int IRM_TAG_MAP_MAX_LEN = 8;
public final static byte[] MESSAGE_END = { (byte) 0x00, (byte) 0x04,
(byte) 0x00, (byte) 0x00 };
public final static short IRM_NAK_REASONCODE_LOW = 2001;
public final static short IRM_NAK_REASONCODE_HIGH = 3000;
//------------------Added for Sync callout------------------------------////
public final static int IRM_INPUT_MOD_NAME_MAX_LEN = 8;
//--------------End--------------------------------------------------------/////
// begin: himakar 03/05/07 Callout changes
/*
* public static final byte RESP_MODE_NOFLAG = (byte) 0x20; // no response
* public static final byte RESP_MODE_ACK = (byte) 0x40; // positive
* response public static final byte RESP_MODE_NACK = (byte) 0x80; //
* negative responce // end: himakar 03/05/07 Callout changes
*/
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy