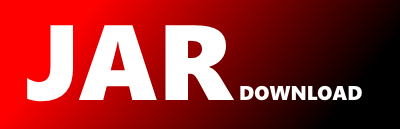
com.ibm.ims.connect.NumberConversion Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of IMSConnectAPI Show documentation
Show all versions of IMSConnectAPI Show documentation
API that allows Java applications to interface with IMS Connect
/*
* File: NumberConversion.java
* ==========================================================================
* Licensed Material - Property of IBM
*
* IBM Confidential
*
* OCO Source Materials
*
* 5655-TDA
*
* (C) Copyright IBM Corp. 2009, 2013 All Rights Reserved.
*
* The source code for this program is not published or
* otherwise divested of its trade secrets, irrespective
* of what has been deposited with the U.S. Copyright
* Office.
*
* US Government Users Restricted Rights - Use, duplication or
* disclosure restricted by GSA ADP Schedule Contract with
* IBM Corp.
* ===========================================================================
*/
package com.ibm.ims.connect;
/**
* @author kevin
*
*/
public class NumberConversion
{
@SuppressWarnings("unused")
private static final String copyright =
"Licensed Material - Property of IBM "
+ "5655-TDA"
+ "(C) Copyright IBM Corp. 2010, 2013 All Rights Reserved. "
+ "US Government Users Restricted Rights - Use, duplication or "
+ "disclosure restricted by GSA ADP Schedule Contract with IBM Corp. ";
/**
* IMSNumConverter constructor comment.
*/
public NumberConversion() {
super();
}
/**
* Parse the bytearray argument as an integer. It takes the first 4 bytes
* from the given offset argument and return its value as an integer.
*
* @param byteVal The bytearray containing the binary number
* @param startOffset The starting offset in the bytearray to parse.
* @return short
*/
public static int parseByteArrayToInt( byte[] byteVal, int startOffset )
{
int result = 0;
for (int i=startOffset; i < startOffset+4; i++)
result = (result << 8) + (byteVal[i] & 0xff);
return result;
}
/**
* Parse the bytearray argument as a short. Takes the first 2
* bytes at the given offset argument and returns its value as a
* short. If the offset points to the last element of the
* bytearray, a value of -1 is returned indicating that the
* method was not able to parse two bytes beginning at startOffset.
*
* @param byteVal The bytearray containing the binary number
* @param startOffset The starting offset in the bytearray to parse
* @return short The two bytes beginning at startOffset in byteVal
* converted to a short value or -1 if startOffset
* points to the last element of byteVal
*/
public static short parseByteArrayToShort( byte[] byteVal, int startOffset )
{
int result = 0;
for (int i=startOffset; i < startOffset+2; i++) {
if (i < byteVal.length)
result = (result << 8) + (byteVal[i] & 0xff);
else
return (short)-1;
}
return (short)result;
}
/**
* Return 2's complement representation of the number, in BigEndian format.
* @return byte[]
*/
public static byte[] parseIntToByteArray( int val )
{
byte[] result = new byte[4];
for( int i = 0; i < 4; i++, val >>= 8 )
{
result[3-i] = (byte)val;
}
return result;
}
/**
* Return 2's complement representation of the number, in BigEndian format.
* @return byte[]
*/
public static byte[] parseShortToByteArray( short val )
{
byte[] result = new byte[2];
for( int i = 0; i < 2; i++, val >>= 8 )
{
result[1-i] = (byte)val;
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy