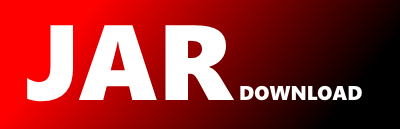
com.ibm.ims.connect.PropertiesFileLoader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of IMSConnectAPI Show documentation
Show all versions of IMSConnectAPI Show documentation
API that allows Java applications to interface with IMS Connect
/*
* File: PropertiesFileLoader.java
* ==========================================================================
* Licensed Material - Property of IBM
*
* IBM Confidential
*
* OCO Source Materials
*
* 5655-TDA
*
* (C) Copyright IBM Corp. 2009, 2014 All Rights Reserved.
*
* The source code for this program is not published or
* otherwise divested of its trade secrets, irrespective
* of what has been deposited with the U.S. Copyright
* Office.
*
* US Government Users Restricted Rights - Use, duplication or
* disclosure restricted by GSA ADP Schedule Contract with
* IBM Corp.
* ===========================================================================
*/
package com.ibm.ims.connect;
import com.ibm.ims.connect.impl.ConnectionImpl;
import com.ibm.ims.connect.impl.TmInteractionImpl;
import java.io.BufferedReader;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.lang.NoSuchMethodException;
import java.net.URL;
import java.util.logging.Logger;
public class PropertiesFileLoader
{
private static final String copyright =
"Licensed Material - Property of IBM "
+ "5655-TDA"
+ "(C) Copyright IBM Corp. 2009, 2013 All Rights Reserved. "
+ "US Government Users Restricted Rights - Use, duplication or "
+ "disclosure restricted by GSA ADP Schedule Contract with IBM Corp. ";
/*** Local variables used when loading an attributes file ***/
private int equalsPosition =-1;
private String key = null;
private String value = null;
private String strVal = "";
private String lowerValue = "";
private String upperValue = "";
private Boolean booleanVal;
private byte bytVal;
private Byte byteVal;
private int intVal;
private Integer integerVal;
private String apiPropVal;
private Class type;
private String setMethodName;
private Method setMethod;
private Object callingObject;
private Class callingObjectClass;
private static String validSpecialCharacters = "@#$";
private int numPropertiesSet = 0;
private Logger logger;
private FileInputStream fileInStream;
private BufferedReader bufFileReader;
//Private variables for the apiBufferReadLine method
private int maxLinesforReader;//Maximum lines for the reader
private int maxLineLen;//Maximum length for a line
private int line = 1;
//Initializing Default values for apiBufferReadLine method
private static final int DEFAULT_API_READER__LINES_MAX = 4096;
private static final int DEFAULT_API_READER_LINE_LENGTH_MAX = 4096;
private static final int CARRIAGE_RETURN = 13;
private static final int LINE_FEED = 10;
/**
* Populates a class's properties with values read in from an attributes file
*
*/
public void loadPropertiesFile(Object theCallingObject, String anAttributesFileName)
throws Exception
{
logger = Logger.getLogger("com.ibm.ims.connect");
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("--> PropertiesFileLoader.loadPropertiesFile(Object, String)...");
callingObject = theCallingObject;
callingObjectClass = theCallingObject.getClass();
numPropertiesSet = 0;
//open FileInputStream and BufferedReader for anAttributesFileName
try
{
fileInStream = new FileInputStream(anAttributesFileName);
bufFileReader = new BufferedReader(new InputStreamReader(fileInStream));
}
catch (Exception e)
{
String fileTypeString = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.UNKNOWN_TYPE_FILE);
try
{
if(callingObjectClass.equals(ConnectionImpl.class) ||
callingObjectClass.equals(ConnectionFactory.class) ||
callingObjectClass.equals(ConnectionAttributes.class))
fileTypeString = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.CONNECTION_PROPERTIES_FILE);
else if(callingObjectClass.equals(TmInteractionImpl.class) ||
callingObjectClass.equals(TmInteractionAttributes.class))
fileTypeString = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.TMINTERACTION_PROPERTIES_FILE);
}
catch (Exception e1)
{
// do nothing - this exception should never occur unless the API JAR is corrupted
}
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0002E, new Object[] {fileTypeString, anAttributesFileName, ImsConnectErrorMessage.getExceptionMessage(e) });
ImsConnectCommunicationException e1 = new ImsConnectCommunicationException(
ImsConnectErrorMessage.HWS0002E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception thrown in PropertiesFileLoader.loadPropertiesFile(Object, String). Exception thrown was: " + e1.toString());
throw e;
}
try
{
int i = 0;
// String line = bufFileReader.readLine();
// String line = this.apiBufferReadLine(bufFileReader);
String line = this.readLine(bufFileReader);
i++;
while(line != null)
{
// strip out slash-star comments
int slash_star_pos = line.indexOf("/*");
int star_slash_pos = line.indexOf("*/");
while(slash_star_pos > -1)
{
while((slash_star_pos > -1) && (star_slash_pos == -1))
{
//String nextLine = bufFileReader.readLine();
//String nextLine = this.apiBufferReadLine(bufFileReader);
String nextLine = this.readLine(bufFileReader);
i++;
if(nextLine != null)
{
line += nextLine;
star_slash_pos = line.indexOf("*/");
}
else
{
String errMsg = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0032E,
new Object[] {anAttributesFileName,
i,
slash_star_pos});
ImsConnectApiException e = new ImsConnectApiException(ImsConnectErrorMessage.HWS0032E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception caught in PropertiesFileLoader.loadPropertiesFile(Object, String). Exception caught was: " + e.toString());
throw e;
}
}
line = line.substring(0, slash_star_pos) + line.substring(star_slash_pos + 2);
slash_star_pos = line.indexOf("/*");
star_slash_pos = line.indexOf("*/");
}
// strip out slash-slash comments
int slash_slash_pos = line.indexOf("//");
if(slash_slash_pos > -1)
line = line.substring(0, slash_slash_pos);
equalsPosition = line.indexOf("=");
if(equalsPosition > -1)
{
key = line.substring(0, equalsPosition);
key = key.trim();
if((key.charAt(0) == '"') || (key.charAt(0) == '\''))
key = key.substring(1);
int keyLen = key.length();
if((key.charAt(keyLen - 1) == '"') || (key.charAt(keyLen - 1) == '\''))
key = key.substring(0,keyLen - 2);
keyLen = key.length();
if(keyLen != 0)
{
try
{
if(callingObjectClass.equals(ConnectionImpl.class) ||
theCallingObject.getClass().equals(ConnectionFactory.class))
type = ConnectionImpl.class.getDeclaredField(String.valueOf(key)).getType();
else if(callingObjectClass.equals(TmInteractionImpl.class))
type = TmInteractionImpl.class.getDeclaredField(String.valueOf(key)).getType();
}
catch (Exception e) // Invalid key
{
String errMsg = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0028E,
new Object[] {key});
ImsConnectApiException e1 = new ImsConnectApiException(ImsConnectErrorMessage.HWS0028E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception caught in PropertiesFileLoader.loadPropertiesFile(Object, String). Exception caught was: " + e.toString());
throw e1;
}
}
value = line.substring(equalsPosition + 1);
value = value.replaceAll("\t", " ");
String tmpVal = value.trim();
if (tmpVal.length() > 0)
value = tmpVal;
int valueLen = value.length();
if ((valueLen == 0) && (type == String.class))
{
value = " ";
valueLen = 8;
}
if(valueLen > 0)
{
if((value.charAt(0) == '"') || (value.charAt(0) == '\''))
value = value.substring(1);
valueLen = value.length();
if((value.charAt(valueLen - 1) == '"') || (value.charAt(valueLen - 1) == '\''))
{
value = value.substring(0,valueLen - 1);
valueLen = value.length();
}
if(type == String.class) // this value should be a String
{
lowerValue = value.toLowerCase();
upperValue = value.toUpperCase();
if((lowerValue.equals("true") || lowerValue.equals("false"))) // should not be a boolean value if we are looking for string value
{
String validStringString = "";
try
{
validStringString = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.VALID_PROPERTY_VALUE_STRING);
}
catch (Exception e)
{
}
String errMsg = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0030E,
new Object[] {key,
value,
validStringString});
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0030E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception caught in PropertiesFileLoader.loadPropertiesFile(Object, String). Exception caught was: " + e.toString());
throw e;
}
else
{
try // type = String and value is not a boolean value - see if it is an ApiProperties equate
{
Field targetField = ApiProperties.class.getDeclaredField(String.valueOf(upperValue));
Class classType = targetField.getType();
if(classType == String.class)
{
apiPropVal = (String)targetField.get(targetField);
setStringVal(key, apiPropVal);
}
else // if(classType != String.class) - should not be a byte, an int or anything else if we are looking for a string value
{
String validStringString = "";
try
{
validStringString = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.VALID_PROPERTY_VALUE_STRING);
}
catch (Exception e)
{
}
String errMsg = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0030E,
new Object[] {key,
value,
validStringString });
ImsConnectApiException e = new ImsConnectApiException(ImsConnectErrorMessage.HWS0030E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception caught in PropertiesFileLoader.loadPropertiesFile(Object, String). Exception caught was: " + e.toString());
throw e;
}
}
catch (Exception e1)
{
try
{
setStringVal(key, value);
}
catch (Exception e)
{
if (e instanceof InvocationTargetException)
{
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception caught in PropertiesFileLoader.loadPropertiesFile(Object, String). Exception caught was: " + e.getCause().toString());
throw (ImsConnectApiException) e.getCause();
}
else
{
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception caught in PropertiesFileLoader.loadPropertiesFile(Object, String). Exception caught was: " + e.toString());
throw e;
}
}
}
}
} // end of if(type == String.class) // this value should be a String
else if(type == boolean.class) // value should be a boolean
{
lowerValue = value.toLowerCase();
upperValue = value.toUpperCase();
if((lowerValue.equals("true") || lowerValue.equals("false"))) // should not be a boolean value if we are looking for string value
{
booleanVal = new Boolean(lowerValue);
setBoolVal(key, booleanVal);
}
else
{
try // type = boolean and value is not a boolean value - see if it is an ApiProperties equate that is a boolean value
{
Field targetField = ApiProperties.class.getDeclaredField(String.valueOf(upperValue));
Class classType = targetField.getType();
if(classType == boolean.class)
{
apiPropVal = targetField.get(targetField).toString();
booleanVal = new Boolean(apiPropVal);
setBoolVal(key, booleanVal);
}
else // if(classType != boolean.class) //should not be an int or anything else if we are looking for a string value
{
String validBooleanString = "";
try
{
validBooleanString = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.VALID_PROPERTY_VALUE_BOOLEAN);
}
catch (Exception e)
{
}
String errMsg = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0030E,
new Object[] {key,
value,
validBooleanString });
ImsConnectApiException e = new ImsConnectApiException(ImsConnectErrorMessage.HWS0030E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception caught in PropertiesFileLoader.loadPropertiesFile(Object, String). Exception caught was: " + e.toString());
throw e;
}
}
catch (Exception e1)
{
String validBooleanString = "";
try
{
validBooleanString = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.VALID_PROPERTY_VALUE_BOOLEAN);
}
catch (Exception e2)
{
}
String errMsg = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0031E,
new Object[] {key,
value,
validBooleanString,
e1});
ImsConnectApiException e2 = new ImsConnectApiException(ImsConnectErrorMessage.HWS0031E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception caught in PropertiesFileLoader.loadPropertiesFile(Object, String). Exception caught was: " + e2.toString());
throw e2;
}
}
} // end of else if(type == boolean.class) // value should be a boolean
else if(type == int.class) // value should be a int
{
try // try interpreting value as an int and using that to set the value of the current property
{
integerVal = (new Integer(value)).intValue();
setIntVal(key, integerVal);
}
catch (Exception e)
{
if ((e instanceof NoSuchMethodException) || (e instanceof NumberFormatException))
{
try
{
int multiplier = 1;
String multiplierString = "";
char multiplierChar = value.charAt(0);
int d = 0; // number of numeric digits in multiplier (e.g., d=2 for "ddApiProperties.ABCDEF")
while(Character.isDigit(multiplierChar))
{
multiplierString += multiplierChar; // used to build string consisting of digits of multiplier
value = value.substring(d++); // move beginning of value beyond multiplier digits
multiplier = (Integer.valueOf(multiplierString)).intValue(); // calculate multiplier each time through loop
// the multiplier is correct after last time thru loop
}
Field numTargetField = ApiProperties.class.getDeclaredField(String.valueOf(value));
Class numClassType = numTargetField.getType();
if(numClassType == int.class)
{
intVal = numTargetField.getInt(numTargetField);
intVal *= multiplier;
integerVal = new Integer((int)(intVal));
setIntVal(key, integerVal);
}
else
{
String validIntegerString = "";
try
{
validIntegerString = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.VALID_PROPERTY_VALUE_INTEGER);
}
catch (Exception e1)
{
}
String errMsg = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0030E,
new Object[] {key,
value,
validIntegerString });
ImsConnectApiException e2 = new ImsConnectApiException(ImsConnectErrorMessage.HWS0030E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception caught in PropertiesFileLoader.loadPropertiesFile(Object, String). Exception caught was: " + e2.toString());
throw e2;
}
}
catch (Exception e2)
{
String validIntegerString = "";
try
{
validIntegerString = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.VALID_PROPERTY_VALUE_INTEGER);
}
catch (Exception e1)
{
}
String errMsg = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0031E,
new Object[] {key,
strVal,
validIntegerString,
e2});
ImsConnectApiException e3 = new ImsConnectApiException(ImsConnectErrorMessage.HWS0031E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception caught in PropertiesFileLoader.loadPropertiesFile(Object, String). Exception caught was: " + e3.toString());
throw e3;
}
}
else if (e instanceof InvocationTargetException)
{
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception caught in PropertiesFileLoader.loadPropertiesFile(Object, String). Exception caught was: " + e.getCause().toString());
throw (ImsConnectApiException) e.getCause();
}
else
{
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception caught in PropertiesFileLoader.loadPropertiesFile(Object, String). Exception caught was: " + e.toString());
throw e;
}
}
} // end of else if(type == int.class) // value should be an int
else if(type == byte.class) // value should be a byte
{
try
{
byteVal = (new Byte(value)).byteValue();
setByteVal(key, byteVal);
}
catch (Exception e)
{
if ((e instanceof NoSuchMethodException) || (e instanceof NumberFormatException))
{
try
{
byte multiplier = 1;
String multiplierString = "";
char multiplierChar = value.charAt(0);
int d = 0; // number of numeric digits in multiplier (e.g., d=2 for "ddApiProperties.ABCDEF")
while(Character.isDigit(multiplierChar))
{
multiplierString += multiplierChar; // used to build string consisting of digits of multiplier
value = value.substring(d++); // move beginning of value beyond multiplier digits
multiplier = (Byte.valueOf(multiplierString)).byteValue(); // calculate multiplier each time through loop
} // the multiplier is correct after last time thru loop
Field numTargetField = ApiProperties.class.getDeclaredField(String.valueOf(value));
Class numClassType = numTargetField.getType();
if(numClassType == byte.class)
{
bytVal = numTargetField.getByte(numTargetField);
bytVal *= multiplier;
byteVal = new Byte((byte)(bytVal));
setByteVal(key, byteVal);
}
else
{
String validByteString = "";
try
{
validByteString = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.VALID_PROPERTY_VALUE_BYTE);
}
catch (Exception e1)
{
}
String errMsg = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0030E,
new Object[] {key,
value,
validByteString});
ImsConnectApiException e1 = new ImsConnectApiException(ImsConnectErrorMessage.HWS0030E, errMsg);
throw e1;
}
}
catch (Exception e2)
{
String validByteString = "";
try
{
validByteString = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.VALID_PROPERTY_VALUE_BYTE);
}
catch (Exception e1)
{
}
String errMsg = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0031E,
new Object[] {key,
value,
validByteString,
e2});
ImsConnectApiException e3 = new ImsConnectApiException(ImsConnectErrorMessage.HWS0031E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception caught in PropertiesFileLoader.loadPropertiesFile(Object, String). Exception caught was: " + e3.toString());
throw e3;
}
}
else if (e instanceof InvocationTargetException)
{
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception caught in PropertiesFileLoader.loadPropertiesFile(Object, String). Exception caught was: " + e.getCause().toString());
throw (ImsConnectApiException) e.getCause();
}
else
{
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception caught in PropertiesFileLoader.loadPropertiesFile(Object, String). Exception caught was: " + e.toString());
throw e;
}
}
} // end of "else if(type == byte.class) // value should be a byte"
} // end of else (after "if (valueLen > 0)" block)
} // end of "if(equalsPosition > -1)"
//line = bufFileReader.readLine();
//line = ((ApiBufferReader) bufFileReader).apiBufferReadLine();
//line = this.apiBufferReadLine(bufFileReader);
line = this.readLine(bufFileReader);
i++;
} // end of while(line != null)
}
catch(IOException e)
{
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0001E, new Object[] {ImsConnectErrorMessage.getExceptionMessage(e) });
ImsConnectApiException e1 = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0001E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception thrown in PropertiesFileLoader.loadPropertiesFile(Object, String). Exception thrown was: " + e1.toString());
throw e;
}
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL))
logger.finest(" " + numPropertiesSet + " properties set in " + theCallingObject.getClass().getSimpleName() + "...");
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("<-- PropertiesFileLoader.loadPropertiesFile(Object, String)...");
}
/**
* setBoolVal puts a Boolean property value into the propertiesTable and then uses the
* appropriate setxxx method to set this value in the corresponding field in this object.
*
*/
private void setBoolVal(String aKey, Boolean aBooleanVal) throws ClassNotFoundException,
NoSuchMethodException, IllegalAccessException, InvocationTargetException
{
Class[] argTypes = {boolean.class};
Object[] valueToBeSet = {aBooleanVal};
invokeSetMethod(aKey, argTypes, valueToBeSet);
}
/**
* setByteVal puts a Integer property value into the propertiesTable and then uses the
* appropriate setxxx method to set this value in the corresponding field in this object.
*
*/
private void setByteVal(String aKey, Byte aByteVal) throws ClassNotFoundException,
NoSuchMethodException, IllegalAccessException, InvocationTargetException
{
Class[] argTypes = {byte.class};
Object[] valueToBeSet = {aByteVal};
invokeSetMethod(aKey, argTypes, valueToBeSet);
}
/**
* setIntVal puts a Integer property value into the propertiesTable and then uses the
* appropriate setxxx method to set this value in the corresponding field in this object.
*
*/
private void setIntVal(String aKey, Integer anIntegerVal) throws ClassNotFoundException,
NoSuchMethodException, IllegalAccessException, InvocationTargetException
{
Class[] argTypes = {int.class};
Object[] valueToBeSet = {anIntegerVal};
invokeSetMethod(aKey, argTypes, valueToBeSet);
}
/**
* setStringVal puts a String property value into the propertiesTable and then uses the
* appropriate setxxx method to set this value in the corresponding field in this object.
*
*/
private void setStringVal(String aPropertyName, String aStringVal) throws ClassNotFoundException,
NoSuchMethodException, IllegalAccessException, InvocationTargetException
{
// propertiesTable.put(aPropertyName, aStringVal);
Class[] argTypes = {String.class};
Object[] valueToBeSet = {aStringVal};
invokeSetMethod(aPropertyName, argTypes, valueToBeSet);
}
/**
* invokeSetMethod creates the name of the method to set the property represented by the
* value of the key and then creates and invokes that method using the passed in argTypes
* and valueToBeSet.
*
* @param String aPropertyName The name of the property to be set
* @param Class[] argTypes An array containing the Classes of each of the set method
* parameters (there will only be one parameter since we are
* using this method to set the value of key-value pairs)
* @param Object[] valueToBeSet An array containing the values to be used for each of the
* set method parameters (there will only be one parameter
* since we are using this method to set the value of key-
* value pairs)
*/
private void invokeSetMethod(String aKey, Class[] argTypes, Object[] valueToBeSet) throws ClassNotFoundException,
NoSuchMethodException, IllegalAccessException, InvocationTargetException
{
//setMethodName = "set" + (aKey.substring(0,1)).toUpperCase() + aKey.substring(1);
//setMethod = callingObject.getClass().getMethod(setMethodName, argTypes);
//setMethod.invoke(callingObject, valueToBeSet);
//Setting ConnectionImpl methods
//Removing above semethodName and putting the below if statements to fix vulnerability
if(callingObjectClass.equals(ConnectionImpl.class))
this.callConnectionImplSetMethod(aKey, valueToBeSet[0], callingObject);
else if(callingObjectClass.equals(ConnectionFactory.class))
this.callConnectionFactorySetMethod(aKey, valueToBeSet[0], callingObject);
else if(callingObjectClass.equals(ConnectionAttributes.class))
this.callConnectionAttributesSetMethod(aKey, valueToBeSet[0], callingObject);
else if(callingObjectClass.equals(TmInteractionImpl.class))
this.callTmInteractionImplSetMethod(aKey, valueToBeSet[0], callingObject);
else if(callingObjectClass.equals(TmInteractionAttributes.class))
this.callTmInteractionAttributesSetMethod(aKey, valueToBeSet[0], callingObject);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL))
logger.finest(" " + aKey + " set to [" + valueToBeSet[0].toString() + "]");
numPropertiesSet++;
}
/**
* Extracts multiplier from value String
*/
private String extractValueMultiplier(String aValue)
{
int multiplier = 1;
String multiplierString = "";
char multiplierChar = aValue.charAt(0);
int d = 0; // number of numeric digits in multiplier (e.g., d=2 for "ddApiProperties.ABCDEF")
while(Character.isDigit(multiplierChar))
{
multiplierString += multiplierChar; // used to build string consisting of digits of multiplier
aValue = aValue.substring(d++); // move beginning of value beyond multiplier digits
}
return multiplierString;
}
/**
* isValidHostStyleName validates the name supplied as an input parameter against the
* standard host naming convention, 1 to 8 alphanumeric characters plus '@', '#' and '$'
* (with no spaces).
*
* #param String aName
*/
public static boolean isValidHostStyleName(String aName)
{
if ((aName != null) && (aName.length() > 0))
{
int i = 0;
for ( ; i < aName.length(); i++)
{
char c = aName.charAt(i);
if((!Character.isLetterOrDigit(c)) && (validSpecialCharacters.indexOf(c) == -1))
i = 9;
}
if(i < 9)
return true;
}
return false;
}
//Method created to remove vulnerability for BufferedReader.readline problem
protected String readLine(BufferedReader bufferedReader) throws ImsConnectApiException{
char[] lineBreak = System.getProperty("line.separator").toCharArray(); //OS specific file separator
int bufferMax = DEFAULT_API_READER_LINE_LENGTH_MAX; //Prevent the exploitation of a large file and use our own buffer size
char[] buffer = new char[bufferMax];
int charRead;
int pos = 0;
try{
if(line <= DEFAULT_API_READER__LINES_MAX){
while ((charRead = bufferedReader.read()) != -1 && charRead != lineBreak[0]) {
if(pos < bufferMax){
buffer[pos] = (char)charRead;
pos++;
}else{
break;
}
}
line++;
return new String(buffer,0,pos);
}
return null;
}catch(IOException e)
{
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0001E, new Object[] {ImsConnectErrorMessage.getExceptionMessage(e) });
ImsConnectApiException e1 = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0001E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception thrown in PropertiesFileLoader.apiBufferReadLine(). Exception thrown was: " + e1.toString());
throw e1;
}
}
private void callConnectionImplSetMethod(String key, Object value, Object conImpl) {
try {
ConnectionImpl cImpl = (ConnectionImpl)conImpl;
if (key.equals("hostName")) {
cImpl.setHostName((String) value);
} else if (key.equals("portNumber")) {
int portNum = (Integer) value;
cImpl.setPortNumber(portNum);
} else if (key.equals("socketType")) {
Byte sockType = (Byte) value;
cImpl.setSocketType(sockType.byteValue());
} else if (key.equals("clientId")) {
cImpl.setClientId((String) value);
} else if (key.equals("sslEncryptionType")) {
Byte encryptType = (Byte) value;
cImpl.setSslEncryptionType(encryptType.byteValue());
} else if (key.equals("sslKeystoreInputStream")) {
InputStream keyStoreIS = (InputStream) value;
cImpl.setSslKeystoreInputStream(keyStoreIS);
} else if (key.equals("sslKeystoreUrl")) {
URL keystoreURL = (URL) value;
cImpl.setSslKeystoreUrl(keystoreURL);
} else if (key.equals("sslKeystoreName")) {
cImpl.setSslKeystoreName((String) value);
} else if (key.equals("sslKeystorePassword")) {
cImpl.setSslKeystorePassword((String) value);
} else if (key.equals("sslTruststoreInputStream")) {
InputStream trustStoreIS = (InputStream) value;
cImpl.setSslTruststoreInputStream(trustStoreIS);
} else if (key.equals("sslTruststoreUrl")) {
URL storeURL = (URL) value;
cImpl.setSslTruststoreUrl(storeURL);
} else if (key.equals("sslTruststoreName")) {
cImpl.setSslTruststoreName((String) value);
} else if (key.equals("sslTruststorePassword")) {
cImpl.setSslTruststorePassword((String) value);
} else if (key.equals("useSslConnection")) {
Boolean sslConnect = (Boolean) value;
cImpl.setUseSslConnection(sslConnect.booleanValue());
} else if (key.equals("interactionTimeout")) {
int timeOut = (Integer) value;
cImpl.setInteractionTimeout(timeOut);
}
} catch (Exception ex) {
// TODO catch exception
}
}
private void callConnectionFactorySetMethod(String key, Object value, Object conFactory) {
try {
ConnectionFactory cF = (ConnectionFactory)conFactory;
if (key.equals("hostName")) {
String hostName = (String) value;
cF.setHostName(hostName);
} else if (key.equals("portNumber")) {
int portNum = (Integer) value;
cF.setPortNumber(portNum);
} else if (key.equals("socketType")) {
Byte sockType = (Byte) value;
cF.setSocketType(sockType.byteValue());
} else if (key.equals("clientId")) {
cF.setClientId((String) value);
} else if (key.equals("sslEncryptionType")) {
Byte encryptType = (Byte) value;
cF.setSslEncryptionType(encryptType.byteValue());
} else if (key.equals("sslKeystoreInputStream")) {
InputStream keyStoreIS = (InputStream) value;
cF.setSslKeystoreInputStream(keyStoreIS);
} else if (key.equals("sslKeystoreUrl")) {
URL keystoreURL = (URL) value;
cF.setSslKeystoreUrl(keystoreURL);
} else if (key.equals("sslKeystoreName")) {
cF.setSslKeystoreName((String) value);
} else if (key.equals("sslKeystorePassword")) {
cF.setSslKeystorePassword((String) value);
} else if (key.equals("sslTruststoreInputStream")) {
InputStream trustStoreIS = (InputStream) value;
cF.setSslTruststoreInputStream(trustStoreIS);
} else if (key.equals("sslTruststoreUrl")) {
URL storeURL = (URL) value;
cF.setSslTruststoreUrl(storeURL);
} else if (key.equals("sslTruststoreName")) {
cF.setSslTruststoreName((String) value);
} else if (key.equals("sslTruststorePassword")) {
cF.setSslTruststorePassword((String) value);
} else if (key.equals("useSslConnection")) {
Boolean sslConnect = (Boolean) value;
cF.setUseSslConnection(sslConnect.booleanValue());
} else if (key.equals("interactionTimeout")) {
int timeOut = (Integer) value;
cF.setInteractionTimeout(timeOut);
}
} catch (Exception ex) {
System.out.println(ex.toString());
// TODO catch exception
}
}
private void callConnectionAttributesSetMethod(String key, Object value, Object conAttributes) {
try {
ConnectionAttributes cAttr = (ConnectionAttributes)conAttributes;
if (key.equals("hostName")) {
cAttr.setHostName((String) value);
} else if (key.equals("portNumber")) {
int portNum = (Integer) value;
cAttr.setPortNumber(portNum);
} else if (key.equals("socketType")) {
Byte sockType = (Byte) value;
cAttr.setSocketType(sockType.byteValue());
} else if (key.equals("clientId")) {
cAttr.setClientId((String) value);
} else if (key.equals("sslEncryptionType")) {
Byte encryptType = (Byte) value;
cAttr.setSslEncryptionType(encryptType.byteValue());
} else if (key.equals("sslKeystoreInputStream")) {
InputStream keyStoreIS = (InputStream) value;
cAttr.setSslKeystoreInputStream(keyStoreIS);
} else if (key.equals("sslKeystoreUrl")) {
URL keystoreURL = (URL) value;
cAttr.setSslKeystoreUrl(keystoreURL);
} else if (key.equals("sslKeystoreName")) {
cAttr.setSslKeystoreName((String) value);
} else if (key.equals("sslKeystorePassword")) {
cAttr.setSslKeystorePassword((String) value);
} else if (key.equals("sslTruststoreInputStream")) {
InputStream trustStoreIS = (InputStream) value;
cAttr.setSslTruststoreInputStream(trustStoreIS);
} else if (key.equals("sslTruststoreUrl")) {
URL storeURL = (URL) value;
cAttr.setSslTruststoreUrl(storeURL);
} else if (key.equals("sslTruststoreName")) {
cAttr.setSslTruststoreName((String) value);
} else if (key.equals("sslTruststorePassword")) {
cAttr.setSslTruststorePassword((String) value);
} else if (key.equals("useSslConnection")) {
Boolean sslConnect = (Boolean) value;
cAttr.setUseSslConnection(sslConnect.booleanValue());
} else if (key.equals("interactionTimeout")) {
int timeOut = (Integer) value;
cAttr.setInteractionTimeout(timeOut);
}
} catch (Exception ex) {
// TODO catch exception
}
}
private void callTmInteractionImplSetMethod(String key, Object value, Object tmInterImpl) {
try {
TmInteractionImpl interImpl = (TmInteractionImpl)tmInterImpl;
if (key.equals("ackNakProvider")) {
Byte ackProvider = (Byte) value;
interImpl.setAckNakProvider(ackProvider);
} else if (key.equals("commitMode")) {
Byte cMode = (Byte) value;
interImpl.setCommitMode(cMode);
} else if (key.equals("cm0IgnorePurge")) {
Boolean cm0IgrPurge = (Boolean) value;
interImpl.setCm0IgnorePurge(cm0IgrPurge);
}else if (key.equals("inputMessageDataSegmentsIncludeLlzzAndTrancode")) {
Boolean includeLlzzTrancode = (Boolean) value;
interImpl.setInputMessageDataSegmentsIncludeLlzzAndTrancode(includeLlzzTrancode);
}else if (key.equals("imsConnectUserMessageExitIdentifier")) {
interImpl.setImsConnectUserMessageExitIdentifier((String)value);
}else if (key.equals("inputMessageOptions")) {
int iMesOptions = (Integer) value;
interImpl.setInputMessageOptions(iMesOptions);
}else if (key.equals("interactionTypeDescription")) {
interImpl.setInteractionTypeDescription((String)value);
}else if (key.equals("ltermOverrideName")) {
interImpl.setLtermOverrideName((String)value);
}else if (key.equals("returnMfsModname")) {
Boolean rMfsModName = (Boolean) value;
interImpl.setReturnMfsModname( rMfsModName);
} else if (key.equals("syncLevel")) {
Byte sLevel = (Byte) value;
interImpl.setSyncLevel(sLevel);
}else if (key.equals("imsConnectCodepage")) {
interImpl.setImsConnectCodepage((String)value);
}else if (key.equals("purgeUndeliverableOutput")) {
Boolean pOutput = (Boolean) value;
interImpl.setPurgeUndeliverableOutput(pOutput);
}else if (key.equals("rerouteUndeliverableOutput")) {
Boolean pOutput = (Boolean) value;
interImpl.setRerouteUndeliverableOutput(pOutput);
}else if (key.equals("rerouteName")) {
interImpl.setRerouteName((String)value);
}else if (key.equals("resumeTpipeAlternateClientId")) {
interImpl.setResumeTpipeAlternateClientId((String)value);
}else if (key.equals("resumeTpipeProcessing")) {
int rtpProc = (Integer) value;
interImpl.setResumeTpipeProcessing(rtpProc);
}else if (key.equals("calloutRequestNakProcessing")) {
interImpl.setCalloutRequestNakProcessing((String)value);
}
else if (key.equals("calloutResponseMessageType")) {
interImpl.setCalloutResponseMessageType((String)value);
}else if (key.equals("resumeTPipeRetrievalType")) {
Byte retType = (Byte) value;
interImpl.setResumeTpipeRetrievalType(retType);
}else if (key.equals("nakReasonCode")) {
short nakRC = (Short) value;
interImpl.setResumeTpipeProcessing(nakRC);
}else if (key.equals("inputModName")) {
interImpl.setInputModName((String)value);
}else if (key.equals("correlatorTkn")) {
byte[] cTkn = (byte[]) value;
interImpl.setCorrelatorToken(cTkn);
}else if (key.equals("racfApplName")) {
interImpl.setRacfApplName((String)value);
}else if (key.equals("racfGroupName")) {
interImpl.setRacfGroupName((String)value);
}else if (key.equals("racfPassword")) {
interImpl.setRacfPassword((String)value);
}else if (key.equals("racfUserId")) {
interImpl.setRacfUserId((String)value);
}else if (key.equals("imsDatastoreName")) {
interImpl.setImsDatastoreName((String)value);
}else if (key.equals("trancode")) {
interImpl.setTrancode((String)value);
}else if (key.equals("interactionTimeout")) {
int iTimeout = (Integer) value;
interImpl.setInteractionTimeout(iTimeout);
}else if (key.equals("imsConnectTimeout")) {
int cTimeout = (Integer) value;
interImpl.setImsConnectTimeout(cTimeout);
}else if (key.equals("otmaTransactionExpiration")) {
Boolean tExp = (Boolean) value;
interImpl.setOtmaTransactionExpiration(tExp);
}else if (key.equals("useCM0AckNoWait")) {
Boolean noWait = (Boolean) value;
interImpl.setUseCM0AckNoWait(noWait);
}else if (key.equals("responseIncludesLlll")) {
Boolean iLlll = (Boolean) value;
interImpl.setResponseIncludesLlll(iLlll);
}
} catch (Exception ex) {
// TODO catch exception
}
}
private void callTmInteractionAttributesSetMethod(String key, Object value, Object tmInterAttr) {
try {
TmInteractionAttributes interAttr = (TmInteractionAttributes)tmInterAttr;
if (key.equals("ackNakProvider")) {
Byte ackProvider = (Byte) value;
interAttr.setAckNakProvider(ackProvider);
} else if (key.equals("commitMode")) {
Byte cMode = (Byte) value;
interAttr.setCommitMode(cMode);
} else if (key.equals("cm0IgnorePurge")) {
Boolean cm0IgrPurge = (Boolean) value;
interAttr.setCm0IgnorePurge(cm0IgrPurge);
}else if (key.equals("inputMessageDataSegmentsIncludeLlzzAndTrancode")) {
Boolean includeLlzzTrancode = (Boolean) value;
interAttr.setInputMessageDataSegmentsIncludeLlzzAndTrancode(includeLlzzTrancode);
}else if (key.equals("imsConnectUserMessageExitIdentifier")) {
interAttr.setImsConnectUserMessageExitIdentifier((String)value);
}else if (key.equals("inputMessageOptions")) {
int iMesOptions = (Integer) value;
interAttr.setInputMessageOptions(iMesOptions);
}else if (key.equals("interactionTypeDescription")) {
interAttr.setInteractionTypeDescription((String)value);
}else if (key.equals("ltermOverrideName")) {
interAttr.setLtermOverrideName((String)value);
}else if (key.equals("returnMfsModname")) {
Boolean rMfsModName = (Boolean) value;
interAttr.setReturnMfsModname( rMfsModName);
} else if (key.equals("syncLevel")) {
Byte sLevel = (Byte) value;
interAttr.setSyncLevel(sLevel);
}else if (key.equals("imsConnectCodepage")) {
interAttr.setImsConnectCodepage((String)value);
}else if (key.equals("purgeUndeliverableOutput")) {
Boolean pOutput = (Boolean) value;
interAttr.setPurgeUndeliverableOutput(pOutput);
}else if (key.equals("rerouteUndeliverableOutput")) {
Boolean pOutput = (Boolean) value;
interAttr.setRerouteUndeliverableOutput(pOutput);
}else if (key.equals("rerouteName")) {
interAttr.setRerouteName((String)value);
}else if (key.equals("resumeTpipeAlternateClientId")) {
interAttr.setResumeTpipeAlternateClientId((String)value);
}else if (key.equals("resumeTpipeProcessing")) {
int rtpProc = (Integer) value;
interAttr.setResumeTpipeProcessing(rtpProc);
}else if (key.equals("calloutRequestNakProcessing")) {
interAttr.setCalloutRequestNakProcessing((String)value);
}
else if (key.equals("calloutResponseMessageType")) {
interAttr.setCalloutResponseMessageType((String)value);
}else if (key.equals("resumeTPipeRetrievalType")) {
Byte retType = (Byte) value;
interAttr.setResumeTpipeRetrievalType(retType);
}else if (key.equals("nakReasonCode")) {
short nakRC = (Short) value;
interAttr.setResumeTpipeProcessing(nakRC);
}else if (key.equals("inputModName")) {
//interAttr.setInputModName((String)value);
}else if (key.equals("correlatorTkn")) {
byte[] cTkn = (byte[]) value;
interAttr.setCorrelatorToken(cTkn);
}else if (key.equals("racfApplName")) {
interAttr.setRacfApplName((String)value);
}else if (key.equals("racfGroupName")) {
interAttr.setRacfGroupName((String)value);
}else if (key.equals("racfPassword")) {
interAttr.setRacfPassword((String)value);
}else if (key.equals("racfUserId")) {
interAttr.setRacfUserId((String)value);
}else if (key.equals("imsDatastoreName")) {
interAttr.setImsDatastoreName((String)value);
}else if (key.equals("trancode")) {
interAttr.setTrancode((String)value);
}else if (key.equals("interactionTimeout")) {
int iTimeout = (Integer) value;
interAttr.setInteractionTimeout(iTimeout);
}else if (key.equals("imsConnectTimeout")) {
int cTimeout = (Integer) value;
interAttr.setImsConnectTimeout(cTimeout);
}else if (key.equals("otmaTransactionExpiration")) {
Boolean tExp = (Boolean) value;
interAttr.setOtmaTransactionExpiration(tExp);
}else if (key.equals("useCM0AckNoWait")) {
//Boolean noWait = (Boolean) value;
//interAttr.setUseCM0AckNoWait(noWait);
}else if (key.equals("responseIncludesLlll")) {
Boolean iLlll = (Boolean) value;
interAttr.setResponseIncludesLlll(iLlll);
}
} catch (Exception ex) {
// TODO catch exception
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy