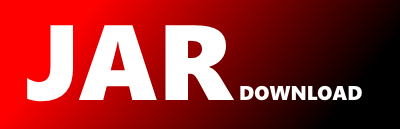
com.ibm.ims.connect.ResponseProcessor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of IMSConnectAPI Show documentation
Show all versions of IMSConnectAPI Show documentation
API that allows Java applications to interface with IMS Connect
/**
* File: ResponseProcessor.java
* ==========================================================================
* Licensed Material - Property of IBM
*
* IBM Confidential
*
* OCO Source Materials
*
* 5655-TDA
*
* (C) Copyright IBM Corp. 2011, 2013 All Rights Reserved.
*
* The source code for this program is not published or
* otherwise divested of its trade secrets, irrespective
* of what has been deposited with the U.S. Copyright
* Office.
*
* US Government Users Restricted Rights - Use, duplication or
* disclosure restricted by GSA ADP Schedule Contract with
* IBM Corp.
* ===========================================================================
*/
package com.ibm.ims.connect;
import java.io.ByteArrayInputStream;
import java.io.InputStream;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.util.logging.*;
import java.util.InvalidPropertiesFormatException;
import java.util.Properties;
//import java.util.regex.Pattern;
/**
* This class provides a processor which parses the response from a Type-2
* command received from IMS or IMS Connect into a Properties object which
* contains separate elements for each field returned in the response data.
*
* @author kevin
*
*/
public class ResponseProcessor
{
@SuppressWarnings("unused")
private static final String copyright =
"Licensed Material - Property of IBM "
+ "5655-T62"
+ "(C) Copyright IBM Corp. 2011 All Rights Reserved. "
+ "US Government Users Restricted Rights - Use, duplication or "
+ "disclosure restricted by GSA ADP Schedule Contract with IBM Corp. ";
private Properties myProperties = new Properties();
InputStream is = null;
String processedResponse = null;
private Logger logger;
ResponseProcessor()
{
logger = Logger.getLogger("com.ibm.ims.connect");
}
protected Properties processProperties(String aResponse, String anEncoding)
throws ImsConnectApiException
{
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("--> ResponseProcessor.processProperties(String aResponse, String anEncoding)");
// String processedResponse = "response after conversion to tagged XML format " + aResponse;
processedResponse = "" + // encoding=\"UTF-8\"?>" +
""); // replace first [\(] with [">]
processedResponse = processedResponse.replaceFirst("\\(", "\">"); // replace first [\(] with [">]
StringBuffer regexBuf2 = new StringBuffer();
regexBuf2.append("\\"); // regexBuf2 = "\"
regexBuf2.append(") "); // regexBuf2 = "\) "
String regex2 = regexBuf2.toString();
processedResponse = processedResponse.replaceFirst(regex2, " "); // replace first [\) ] with []
int startLoc = processedResponse.lastIndexOf("");
while (processedResponse.indexOf("(", startLoc) > 0)
{
int posNextLeftParen = processedResponse.indexOf("(", startLoc);
int posNextRightParen = processedResponse.indexOf("(", startLoc);
if((posNextLeftParen < posNextRightParen) && (posNextLeftParen != -1))
continue;
String leftPartOfString = processedResponse.substring(0, startLoc);
String restOfString = processedResponse.substring(startLoc);
restOfString = restOfString.replaceFirst("", ""); // replace first [\(] with [">]
restOfString = restOfString.replaceFirst("\\(", "\">"); // replace first [\(] with [">]
// restOfString = restOfString.replaceFirst(regex2, " "); // replace first [\) ] with []
restOfString = restOfString.replaceFirst("\\) ", ""); // replace first [\) ] with []
processedResponse = leftPartOfString + restOfString;
startLoc = processedResponse.lastIndexOf("");
}
/*if(!processedResponse.endsWith("")||processedResponse.endsWith("\\)"))
processedResponse =processedResponse.replaceFirst("\\)","");*/
processedResponse += " ";
///// // for debug only
// System.out.println("processedResponse (a element):");
// System.out.println(processedResponse);
/////
processedResponse.replaceAll("< ", "<");
processedResponse.replaceAll(" entry>", "entry>");
/////
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL))
logger.finer(" processedResponse (a element after removing unwanted spaces):\n" +
" " + processedResponse );
/////
try
{
byte[] bytesBuffer = processedResponse.getBytes(anEncoding);
InputStream is = new ByteArrayInputStream(bytesBuffer);
// InputStream is = new ByteArrayInputStream(processedResponse.getBytes(anEncoding));
// reset myProperties object
myProperties.clear();
// load the xml file into properties format
myProperties.loadFromXML(is);
}
catch (UnsupportedEncodingException usee)
{
String errMsg = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0017E,
new Object[] {"Type-2 command XML response", anEncoding});
ImsConnectApiException e = new ImsConnectApiException(ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception caught in ResponseProcessor.processProperties(String aResponse, String anEncoding): [" + e.toString() + "]");
throw e;
}
catch (InvalidPropertiesFormatException ipfe)
{
String errMsg = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0044E,
new Object[] {ipfe.toString()});
ImsConnectApiException e = new ImsConnectApiException(ImsConnectErrorMessage.HWS0044E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception caught in ResponseProcessor.processProperties(String aResponse, String anEncoding): [" + ipfe.toString() + "]");
throw e;
}
catch (IOException ioe)
{
String errMsg = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0044E,
new Object[] {ioe.toString()});
ImsConnectApiException e = new ImsConnectApiException(ImsConnectErrorMessage.HWS0044E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception caught in ResponseProcessor.processProperties(String aResponse, String anEncoding): [" + e.toString() + "]+");
throw e;
}
catch (Exception e)
{
String errMsg = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0044E,
new Object[] {e.toString()});
ImsConnectApiException e1 = new ImsConnectApiException(ImsConnectErrorMessage.HWS0044E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception caught in ResponseProcessor.processProperties(String aResponse, String anEncoding): [" + e1.toString() + "]+");
throw e1;
}
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("<-- ResponseProcessor.processProperties(String aResponse, String anEncoding)");
return myProperties;
}
public String getProcessedResponse()
{
return processedResponse;
}
static protected String formatBufferForTracing(byte[] buffer, String anImsConnectCodepage)
{
String imsConnectCodepage = anImsConnectCodepage;
StringBuffer strbuf =
new StringBuffer(buffer.length * 4 + (buffer.length / 16) * 6);
strbuf.append(" ");
// String passwordObfuscationChar = "*";
// byte passwordObfuscationByte = 0x00;
int hiByte;
int lowByte;
int rowByteCount = 0;
int totalByteCount = 0;
int numberOfBytesInRow = 32;
int startOfData = 116;
// int positionOfPasswordBegin = 76;
// int positionOfPasswordEnd = 83;
String str = "";
byte[] rowBytes;
byte[] irmRowBytes;
byte[] dataRowBytes;
/*
try
{
passwordObfuscationByte = (passwordObfuscationChar.getBytes(imsConnectCodepage))[0];
}
catch (UnsupportedEncodingException usee)
{
}
*/
for (int i = 0; i < buffer.length; i++)
{
hiByte = (int) ((buffer[i] & 0xF0) >> 4);
lowByte = (int) (buffer[i] & 0x0F);
strbuf.append(Integer.toString(hiByte, 16)); // append high-order nibble of a byte
strbuf.append(Integer.toString(lowByte, 16)); // append low-order nibble of a byte
rowByteCount++;
totalByteCount++;
if (rowByteCount % 4 == 0)
strbuf.append(" "); // add a space after every 4th byte
if (rowByteCount % 16 == 0)
strbuf.append(" "); // add an extra space after every 16th byte
if (rowByteCount == numberOfBytesInRow) // after row has been processed, format that row's bytes in human-readable form
{
try
{
int startOfRow = i - numberOfBytesInRow + 1;
rowBytes = new byte[numberOfBytesInRow];
System.arraycopy(buffer, i - numberOfBytesInRow + 1, rowBytes, 0, numberOfBytesInRow);
if (i < 116)
{
str = new String(rowBytes, 0, rowByteCount, imsConnectCodepage);
}
else if((startOfRow) >= 116)
{
str = new String(rowBytes, 0, rowByteCount, imsConnectCodepage);
// str = new String(rowBytes, 0, rowByteCount);
}
else
{
int startOfDataInRowString = startOfData - (i - numberOfBytesInRow);
int irmInRowLen = startOfDataInRowString;
int dataInRowLen = numberOfBytesInRow - irmInRowLen;
int dataInRowLen1 = buffer.length - startOfData;
if (dataInRowLen1 < dataInRowLen)
dataInRowLen = dataInRowLen1;
irmRowBytes = new byte[irmInRowLen];
dataRowBytes = new byte[dataInRowLen];
System.arraycopy(buffer, i - numberOfBytesInRow + 1, irmRowBytes, 0, irmInRowLen);
System.arraycopy(buffer, startOfData + 1, dataRowBytes, 0, dataInRowLen);
str = new String(irmRowBytes, 0, irmInRowLen, imsConnectCodepage) +
// str = new String(irmRowBytes, 0, irmInRowLen) +
new String(dataRowBytes, 0, dataInRowLen, imsConnectCodepage);
// new String(dataRowBytes, 0, dataInRowLen);
}
strbuf.append("|");
for (int m = 0; m < rowByteCount; m++)
{
if (isPrintableChar(str.charAt(m)))
strbuf.append(str.charAt(m));
else
strbuf.append(".");
}
strbuf.append("|");
}
catch (Exception e)
{
// System.out.println(e.getMessage());
}
strbuf.append(" : ");
strbuf.append(totalByteCount);
strbuf.append("\n ");
rowByteCount = 0;
}
}
if (rowByteCount != 0) // last row is not a full row of 32 bytes
{
for (int l = rowByteCount; l < 32; l++)
{
strbuf.append(" ");
if((l + 1) % 4 == 0)
strbuf.append(" "); // add an extra space after every 4th byte
if((l + 1) % 16 == 0)
strbuf.append(" "); // add an extra space after every 16th byte
}
try
{
str = new String(buffer, buffer.length - rowByteCount, rowByteCount, imsConnectCodepage);
// str = new String(buffer, buffer.length - rowByteCount, rowByteCount);
strbuf.append("|");
int m;
for (m = 0; m < rowByteCount; m++)
{
if (isPrintableChar(str.charAt(m)))
strbuf.append(str.charAt(m));
else
strbuf.append(".");
}
for(; m < 32; m++)
strbuf.append(" ");
strbuf.append("|");
}
catch (Exception e)
{
}
strbuf.append(" : ");
strbuf.append(totalByteCount);
}
return new String(strbuf);
}
private static boolean isPrintableChar(char ch)
{
if (Character.isISOControl(ch))
return false;
return !(Character.isIdentifierIgnorable(ch));
}
/////
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy