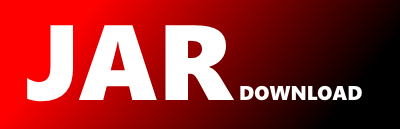
com.ibm.ims.connect.TmInteractionAttributes Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of IMSConnectAPI Show documentation
Show all versions of IMSConnectAPI Show documentation
API that allows Java applications to interface with IMS Connect
/*
* File: TmInteractionAttributes.java
* ==========================================================================
* Licensed Material - Property of IBM
*
* IBM Confidential
*
* OCO Source Materials
*
* 5655-TDA
*
* (C) Copyright IBM Corp. 2009, 2013 All Rights Reserved.
*
* The source code for this program is not published or
* otherwise divested of its trade secrets, irrespective
* of what has been deposited with the U.S. Copyright
* Office.
*
* US Government Users Restricted Rights - Use, duplication or
* disclosure restricted by GSA ADP Schedule Contract with
* IBM Corp.
* ===========================================================================
*/
package com.ibm.ims.connect;
/**
* This class represents the properties that are used to perform an interaction
* with IMS Connect for running an IMS transaction.
*
*/
public class TmInteractionAttributes implements ApiProperties
{
public static final String copyright =
"Licensed Material - Property of IBM "
+ "5655-TDA"
+ "(C) Copyright IBM Corp. 2009, 2013 All Rights Reserved. "
+ "US Government Users Restricted Rights - Use, duplication or "
+ "disclosure restricted by GSA ADP Schedule Contract with IBM Corp. ";
//---------------------------------------------------
// Set up default TmInteractionAttributes properties
//---------------------------------------------------
//Input properties
private byte ackNakProvider = ApiProperties.DEFAULT_ACK_NAK_PROVIDER;
private byte commitMode = ApiProperties.DEFAULT_COMMIT_MODE;
private boolean cm0IgnorePurge = ApiProperties.DEFAULT_CM0_IGNORE_PURGE;
private boolean cancelClientId = ApiProperties.DEFAULT_CANCEL_CLIENTID;
private boolean returnClientId = ApiProperties.DEFAULT_RETURN_CLIENTID;
private boolean generateClientIdWhenDuplicate = ApiProperties.DEFAULT_IMS_CONNECT_GENERATE_CLIENTID;
private boolean inputMessageDataSegmentsIncludeLlzzAndTrancode = ApiProperties.DEFAULT_INPUT_MESSAGE_DATA_SEGMENTS_INCLUDE_LLZZ_AND_TRANCODE;
private String imsConnectUserMessageExitIdentifier = ApiProperties.DEFAULT_IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER;
private int inputMessageOptions = ApiProperties.DEFAULT_INPUT_MESSAGE_OPTIONS;
private String interactionTypeDescription = ApiProperties.DEFAULT_INTERACTION_TYPE_DESC;
private String ltermOverrideName = ApiProperties.DEFAULT_LTERM_OVERRIDE_NAME;
private boolean returnMfsModname = ApiProperties.DEFAULT_RETURN_MFS_MODNAME;
private byte syncLevel = ApiProperties.DEFAULT_SYNC_LEVEL;
// Message encoding properties
private String imsConnectCodepage = ApiProperties.DEFAULT_IMS_CONNECT_CODEPAGE; //?????
// private byte imsConnectUnicodeEncodingSchema = ApiProperties.DEFAULT_IMS_CONNECT_UNICODE_ENCODING_SCHEMA;
// private byte imsConnectUnicodeUsage = ApiProperties.DEFAULT_IMS_CONNECT_UNICODE_USAGE;
// ResumeTpipe and Message routing properties
private boolean purgeUndeliverableOutput = ApiProperties.DEFAULT_PURGE_UNDELIVERABLE_OUTPUT;
private boolean rerouteUndeliverableOutput = ApiProperties.DEFAULT_REROUTE_UNDELIVERABLE_OUTPUT;
private String rerouteName = ApiProperties.DEFAULT_REROUTE_NAME;
private String resumeTpipeAlternateClientId = ApiProperties.DEFAULT_RESUMETPIPE_ALTERNATE_CLIENTID;
private int resumeTpipeProcessing = ApiProperties.DEFAULT_RESUME_TPIPE_PROCESSING;
// Security properties
private String racfApplName = ApiProperties.DEFAULT_RACF_APPL_NAME;
private String racfGroupName = ApiProperties.DEFAULT_RACF_GROUP_NAME;
private String racfPassword = ApiProperties.DEFAULT_RACF_PASSWORD;
private String racfUserId = ApiProperties.DEFAULT_RACF_USERID;
// Target IMS properties
private String imsDatastoreName = ApiProperties.DEFAULT_IMS_DATASTORE_NAME;
private String trancode = ApiProperties.DEFAULT_TRANCODE;
// Timeout properties
private int imsConnectTimeout = ApiProperties.DEFAULT_IMS_CONNECT_TIMEOUT;
private int interactionTimeout = ApiProperties.DEFAULT_INTERACTION_TIMEOUT;
private boolean otmaTransactionExpiration = ApiProperties.DEFAULT_OTMA_TRANSACTION_EXPIRATION;
// Response Properties
private boolean responseIncludesLlll = ApiProperties.DEFAULT_RESPONSE_INCLUDES_LLLL;
//------------------Added for Sync callout------------------------------////
private String calloutRequestNakProcessing = ApiProperties.DEFAULT_CALLOUT_REQUEST_NAK_PROCESSING;
private String calloutResponseMessageType = ApiProperties.DEFAULT_CALLOUT_RESPONSE_MESSAGE_TYPE;
private byte resumeTpipeRetrievalType = ApiProperties.DEFAULT_RESUME_TPIPE_RETRIEVAL_TYPE;
private short nakReasonCode = ApiProperties.IRM_NAK_REASONCODE_DEFAULT;
private byte[] correlatorTkn = CORRELATOR_TOKEN_DEFAULT;
//-----------------End-------------------------------------------------////
/* // IMS Connect XML properties
private String xmlAdapterName = ApiProperties.DEFAULT_XML_ADAPTER_NAME;
private String xmlConverterName = ApiProperties.DEFAULT_XML_CONVERTER_NAME;
private byte xmlAdapterType = ApiProperties.DEFAULT_XML_ADAPTER_TYPE;
private byte xmlMessageType = ApiProperties.DEFAULT_XML_MESSAGE_TYPE;
*/
// Getter and setter methods
//Input properties
/**
* Gets the ackNakProvider property value.
* @return byte
* @see TmInteraction#getAckNakProvider()
*/
public byte getAckNakProvider()
{
return this.ackNakProvider;
}
/**
* Set clientAckNak property value.
*
* @param anAckNakProvider byte
* @see TmInteraction#setAckNakProvider(byte)
*/
public void setAckNakProvider(byte anAckNakProvider)
{
this.ackNakProvider = anAckNakProvider;
}
/**
* Gets the the cm0IgnorePurge property value.
*
* @return boolean
* @see TmInteraction#isCm0IgnorePurge()
*/
public boolean isCm0IgnorePurge()
{
return this.cm0IgnorePurge;
}
/**
* Sets the cm0IgnorePurge property value for this interaction.
* @param cm0IgnorePurge the cm0IgnorePurge value to use for this interaction.
* Use the constants defined in ApiProperties
to specify this parameter.
* @see TmInteraction#setCm0IgnorePurge(boolean)
* @see ApiProperties#DEFAULT_CM0_IGNORE_PURGE
* @see ApiProperties#CM0_DO_IGNORE_PURGE
* @see ApiProperties#CM0_DO_NOT_IGNORE_PURGE
*/
public void setCm0IgnorePurge(boolean cm0IgnorePurge)
{
this.cm0IgnorePurge = cm0IgnorePurge;
}
/**
* Sets the cancelClientId property value for this interaction. Use this property to indicate whether
* an existing connection with the same clientID should be canceled or retained for the interaction.
* The valid values, for which the following constants are defined in ApiProperties
, are:
*
* DO_CANCEL_CLIENTID
- Indicates that if there is another connection with the same client ID
* that connection should be closed and the requesting connection should be granted.
* DO_NOT_CANCEL_CLIENTID
- Indicates that that if there is another connection
* with the same client ID that connection should be kept live
* and the current request denied.
* DEFAULT_CANCEL_CLIENTID
- same as DO_CANCEL_CLIENTID
*
*
* If a value for this property is not supplied by the client, the default value
* (DO_CANCEL_CLIENTID
) will be used.
* @param aCancelClientID the cancelClientID value to use for this interaction.
* Use the constants defined in ApiProperties
to specify this parameter.
* @see ApiProperties#DEFAULT_CANCEL_CLIENTID
* @see ApiProperties#DO_CANCEL_CLIENTID
* @see ApiProperties#DO_NOT_CANCEL_CLIENTID
* @see TmInteraction#setCancelClientId(boolean)
*/
public void setCancelClientId(boolean aCancelClientId)
{
this.cancelClientId = aCancelClientId;
}
/**
* Gets the cancelClientId property value.
* @return a boolean indicating if an existing connection with the same
* client ID should be canceled or not.
*
* @see TmInteraction#isCancelClientId()
*/
public boolean isCancelClientId()
{
return this.cancelClientId;
}
/**
* Specifies to IMS Connect whether to generate a unique client ID for the connection if a duplicate client ID already exists.
* The valid values, for which the following constants are defined in ApiProperties
, are:
*
* DO_GENERATE_CLIENTID
- Indicates to IMS Connect to generate the client ID for the connection.
* DO_NOT_GENERATE_CLIENTID
- Indicates to IMS Connect not to generate the client ID for the connection.
*
* DEFAULT_IMS_CONNECT_GENERATE_CLIENTID
- Same as DO_NOT_GENERATE_CLIENTID
.
*
*
* If a value for this property is not supplied by the client, the default value
* (DO_NOT_GENERATE_CLIENTID
) is used.
* @param aGenerateValue the generateClientIdWhenDuplicate value to use for this interaction.
* Use the constants defined in ApiProperties
to specify this parameter.
* @see ApiProperties#DEFAULT_IMS_CONNECT_GENERATE_CLIENTID
* @see ApiProperties#DO_GENERATE_CLIENTID
* @see ApiProperties#DO_NOT_GENERATE_CLIENTID
* @see TmInteraction#isGenerateClientIdWhenDuplicate()
*/
public void setGenerateClientIdWhenDuplicate(boolean aGenerateClientIdValue)
{
this.generateClientIdWhenDuplicate = aGenerateClientIdValue;
}
/**
* Gets the generateClientIdWhenDuplicate property value.
* @return a boolean indicating if a client ID will be generated by IMS Connect for a given duplicate client ID.
* @see TmInteraction#setGenerateClientIdWhenDuplicate(boolean)
*/
public boolean isGenerateClientIdWhenDuplicate()
{
return this.generateClientIdWhenDuplicate;
}
/**
* Identifies if IMS Connect would generate a unique client ID for the connection when no client ID is
* specified by the client, or a duplicate client ID already exists for the connection.
* The valid values, for which the following constants are defined in ApiProperties
, are:
*
* DO_RETURN_CLIENTID
- Indicates to IMS Connect to return the client ID for the connection.
* DO_NOT_RETURN_CLIENTID
- Indicates to IMS Connect not to return the client ID for the connection.
* DEFAULT_RETURN_CLIENTID
- Same as DO_NOT_RETURN_CLIENTID
*
*
* If a value for this property is not supplied by the client, the default value
* (DO_NOT_RETURN_CLIENTID
) is used.
* @param aReturnClientIdValue the returnClientID value to use for this interaction.
* Use the constants defined in ApiProperties
to specify this parameter.
* @see ApiProperties#DEFAULT_RETURN_CLIENTID
* @see ApiProperties#DO_RETURN_CLIENTID
* @see ApiProperties#DO_NOT_RETURN_CLIENTID
* @see TmInteraction#isReturnClientId()
*/
public void setReturnClientId(boolean aReturnClientId)
{
this.returnClientId = aReturnClientId;
}
/**
* Gets the returnClientId property value.
* @return a boolean indicating if a client Id will be returned with the output
* client ID should be canceled or not.
*
* @see TmInteraction#setReturnClientId(boolean)
*/
public boolean isReturnClientId()
{
return this.returnClientId;
}
/**
* Gets the commitMode property value.
* @return an byte
value containing the commitMode property value
* @see TmInteraction#getCommitMode()
* @see ApiProperties#DEFAULT_COMMIT_MODE
* @see ApiProperties#COMMIT_MODE_0
* @see ApiProperties#COMMIT_MODE_1
*/
public byte getCommitMode()
{
return this.commitMode;
}
/**
* Sets the commitMode property value to be used for this interaction.
* @param aCommitMode A byte value representing the commit mode. Use the constants defined in ApiProperties to specify this parameter.
* @see TmInteraction#setCommitMode(byte)
* @see ApiProperties#COMMIT_MODE_0
* @see ApiProperties#COMMIT_MODE_1
*/
public void setCommitMode(byte aCommitMode)
{
this.commitMode = aCommitMode;
}
/**
* Gets the imsConnectCodepage property value for this interaction.
* @return String
* @see TmInteraction#getImsConnectCodepage()
*/
public String getImsConnectCodepage()
{
return imsConnectCodepage;
}
/**
* Sets the imsConnectCodepage property value for this interaction.
* @param aCodepage String
* @see TmInteraction#setImsConnectCodepage(String)
*/
public void setImsConnectCodepage(String aCodepage)
{
this.imsConnectCodepage = aCodepage;
}
/**
* Gets the imsConnectTimeout property value.
* @return int
* @see TmInteraction#getImsConnectTimeout()
*/
public int getImsConnectTimeout()
{
return imsConnectTimeout;
}
/**
* Sets the imsConnectTimeout property value.
* @param anImsConnectTimeout
* the imsConnectTimeout value to be used for this interaction.
* Use the constants defined in ApiProperties
to specify this parameter.
* @see TmInteraction#setImsConnectTimeout(int)
*/
public void setImsConnectTimeout(int anImsConnectTimeout)
{
this.imsConnectTimeout = anImsConnectTimeout;
}
/**
* Gets the imsConectUserMessageExitIdentifier property value.
* @return String
* @see TmInteraction#getImsConnectUserMessageExitIdentifier()
*/
public String getImsConnectUserMessageExitIdentifier()
{
return this.imsConnectUserMessageExitIdentifier;
}
/**
* Sets the imsConnectUserMessageExitIdentifier property value.
* @param anIConUserMessageExitIdentifier String
* Use the constants defined in ApiProperties to specify this parameter.
* @see TmInteraction#setImsConnectUserMessageExitIdentifier(String)
*/
public void setImsConnectUserMessageExitIdentifier(String anIConUserMessageExitIdentifier)
{
imsConnectUserMessageExitIdentifier = anIConUserMessageExitIdentifier;
}
/**
* Gets imsDatastoreName property value
* @return String
* @see TmInteraction#getImsDatastoreName()
*/
public String getImsDatastoreName()
{
return this.imsDatastoreName;
}
/**
* Sets the imsDatastoreName property value.
* @param anImsDatastoreName
* the IMS datastore name to be used for this interaction.
* @see TmInteraction#setImsDatastoreName(String)
*/
public void setImsDatastoreName(String anImsDatastoreName)
{
this.imsDatastoreName = anImsDatastoreName;
}
/**
* Gets a boolean value representing whether the data segments in the input messages includes
* an LLZZ prefix.
* @return a boolean
value containing the inputMessageDataSegmentsIncludeLlzzAndTrancode
* property value
* @see TmInteraction#isInputMessageDataSegmentsIncludeLlzzAndTrancode()
* @see ApiProperties#INPUT_MESSAGE_DATA_SEGMENTS_DO_NOT_INCLUDE_LLZZ_AND_TRANCODE
* @see ApiProperties#INPUT_MESSAGE_DATA_SEGMENTS_DO_INCLUDE_LLZZ_AND_TRANCODE
*/
public boolean isInputMessageDataSegmentsIncludeLlzzAndTrancode()
{
return this.inputMessageDataSegmentsIncludeLlzzAndTrancode;
}
/**
* Sets the inputMessageDataSegmentsIncludeLlzzAndTrancode property value for this interaction.
* @param anInputMessageDataSegmentsIncludeLlzzAndTrancode
* a boolean value representing whether or not the data segments
* in the input messages include an LLZZ prefix and a trancode if required
* @see TmInteraction#setInputMessageDataSegmentsIncludeLlzzAndTrancode(boolean)
* @see TmInteractionAttributes#isInputMessageDataSegmentsIncludeLlzzAndTrancode()
*/
public void setInputMessageDataSegmentsIncludeLlzzAndTrancode(boolean anInputMessageDataSegmentsIncludeLlzzAndTrancode)
{
inputMessageDataSegmentsIncludeLlzzAndTrancode = anInputMessageDataSegmentsIncludeLlzzAndTrancode;
}
/**
* Get inputMessageOptions property value.
*
* @return the inputMessageOptions
* @see TmInteraction#getInputMessageOptions()
* @see ApiProperties#INPUT_MESSAGE_OPTIONS_NO_OPTIONS
* @see ApiProperties#INPUT_MESSAGE_OPTIONS_EBCDIC
*/
public int getInputMessageOptions()
{
return this.inputMessageOptions;
}
/**
* Sets the InputMessageOptions property value.
* @param anInputMessageOptions The inputMessageOptions to set. Use the constants defined in ApiProperties to specify this parameter.
* @see TmInteraction#setInputMessageOptions(int)
* @see TmInteraction#getInputMessageOptions()
* @see ApiProperties#INPUT_MESSAGE_OPTIONS_NO_OPTIONS
* @see ApiProperties#INPUT_MESSAGE_OPTIONS_EBCDIC
*/
public void setInputMessageOptions(int anInputMessageOptions)
{
this.inputMessageOptions = anInputMessageOptions;
}
/**
* Gets the interaction timeout value.
* @return an int
value containing the interactionTimeout property value
*/
public int getInteractionTimeout()
{
return interactionTimeout;
}
/**
* Sets the interactionTimeout property value.
* @param interactionTimeout
* the interactionTimeout to be used for this interaction.
* Use the constants defined in ApiProperties
to specify this parameter.
* @see TmInteraction#setInteractionTimeout(int)
* @see TmInteraction#getInteractionTimeout()
* @see ApiProperties#DEFAULT_INTERACTION_TIMEOUT
* @see ApiProperties#TIMEOUT_1_MILLISECOND
* @see ApiProperties#TIMEOUT_2_MILLISECONDS
* @see ApiProperties#TIMEOUT_5_MILLISECONDS
* @see ApiProperties#TIMEOUT_10_MILLISECONDS
* @see ApiProperties#TIMEOUT_20_MILLISECONDS
* @see ApiProperties#TIMEOUT_50_MILLISECONDS
* @see ApiProperties#TIMEOUT_100_MILLISECONDS
* @see ApiProperties#TIMEOUT_200_MILLISECONDS
* @see ApiProperties#TIMEOUT_500_MILLISECONDS
* @see ApiProperties#TIMEOUT_1_SECOND
* @see ApiProperties#TIMEOUT_2_SECONDS
* @see ApiProperties#TIMEOUT_5_SECONDS
* @see ApiProperties#TIMEOUT_10_SECONDS
* @see ApiProperties#TIMEOUT_30_SECONDS
* @see ApiProperties#TIMEOUT_45_SECONDS
* @see ApiProperties#TIMEOUT_1_MINUTE
* @see ApiProperties#TIMEOUT_2_MINUTES
* @see ApiProperties#TIMEOUT_5_MINUTES
* @see ApiProperties#TIMEOUT_10_MINUTES
* @see ApiProperties#TIMEOUT_30_MINUTES
* @see ApiProperties#TIMEOUT_45_MINUTES
* @see ApiProperties#TIMEOUT_1_HOUR
* @see ApiProperties#TIMEOUT_2_HOURS
* @see ApiProperties#TIMEOUT_4_HOURS
* @see ApiProperties#TIMEOUT_8_HOURS
* @see ApiProperties#TIMEOUT_12_HOURS
* @see ApiProperties#TIMEOUT_1_DAY
* @see ApiProperties#TIMEOUT_2_DAYS
* @see ApiProperties#TIMEOUT_5_DAYS
* @see ApiProperties#TIMEOUT_1_WEEK
* @see ApiProperties#TIMEOUT_2_WEEKS
* @see ApiProperties#INTERACTION_TIMEOUT_MAX
* @see ApiProperties#TIMEOUT_WAIT_FOREVER
*/
public void setInteractionTimeout(int interactionTimeout)
{
this.interactionTimeout = interactionTimeout;
}
/**
* Gets the interactionTypeDescription property value.
* @return a String
value containing the InteractionTypeDescription property value
* @see TmInteraction#setInteractionTypeDescription(String)
* @see TmInteraction#getInteractionTypeDescription()
* @see ApiProperties#INTERACTION_TYPE_DESC_ACK
* @see ApiProperties#INTERACTION_TYPE_DESC_CANCELTIMER
* @see ApiProperties#INTERACTION_TYPE_DESC_ENDCONVERSATION
* @see ApiProperties#INTERACTION_TYPE_DESC_NAK
* @see ApiProperties#INTERACTION_TYPE_DESC_RECEIVE
* @see ApiProperties#INTERACTION_TYPE_DESC_RESUMETPIPE
* @see ApiProperties#INTERACTION_TYPE_DESC_SENDONLY
* @see ApiProperties#INTERACTION_TYPE_DESC_SENDONLYACK
* @see ApiProperties#INTERACTION_TYPE_DESC_SENDRECV
*/
public String getInteractionTypeDescription()
{
return this.interactionTypeDescription;
}
/**
* Sets the interactionTypeDescription property value which specifies the function to be performed in
* IMS Connect and OTMA in this interaction. Only one function can be specified at a time.
* The valid values re defined in ApiProperties
.
* @param anInterTypeDesc
* type of interaction to be executed. Use the constants defined in ApiProperties to specify this parameter.
* @see TmInteraction#setInteractionTypeDescription(String)
* @see TmInteraction#setInteractionTypeDescription(String)
* @see ApiProperties#INTERACTION_TYPE_DESC_ACK
* @see ApiProperties#INTERACTION_TYPE_DESC_CANCELTIMER
* @see ApiProperties#INTERACTION_TYPE_DESC_ENDCONVERSATION
* @see ApiProperties#INTERACTION_TYPE_DESC_NAK
* @see ApiProperties#INTERACTION_TYPE_DESC_RECEIVE
* @see ApiProperties#INTERACTION_TYPE_DESC_RESUMETPIPE
* @see ApiProperties#INTERACTION_TYPE_DESC_SENDONLY
* @see ApiProperties#INTERACTION_TYPE_DESC_SENDONLYACK
* @see ApiProperties#INTERACTION_TYPE_DESC_SENDONLYXCFORDEREDDELIVERY
* @see ApiProperties#INTERACTION_TYPE_DESC_SENDRECV
*/
public void setInteractionTypeDescription(String anInterTypeDesc)
{
this.interactionTypeDescription = anInterTypeDesc;
}
/**
* Gets the LTERM override name.
* @return a String
value containing the ltermOverrideName property value
*/
public String getLtermOverrideName()
{
return this.ltermOverrideName;
}
/**
* Sets the ltermOverrideName property value.
* @param anLtermOverrideName the ltermOverrideName to set.
* @see TmInteraction#setLtermOverrideName(String)
* @see TmInteraction#getLtermOverrideName()
*/
public void setLtermOverrideName(String anLtermOverrideName)
{
this.ltermOverrideName = anLtermOverrideName;
}
/**
* Gets the otmaTransactionExpiration property value.
* @return a boolean
value indicating whether IMS Connect will pass a transaction expiration
* time to OTMA for this interaction. If this value is true, OTMA halts processing of the
* transaction for this interaction if the transaction has not yet completed or IMS Connect
* has not yet received the transaction response when the IMS Connect timeout expires.
* @see TmInteractionAttributes#setOtmaTransactionExpiration(boolean)
*/
public boolean isOtmaTransactionExpiration()
{
return this.otmaTransactionExpiration;
}
/**
* Sets the otmaTransactionExpiration property that controls the transaction expiration
* time that OTMA uses for the current interaction.
* @param otmaTransactionExpiration
* the otmaTransactionExpiration to set. Use the constants defined in ApiProperties to specify this parameter.
* @see ApiProperties#DO_EXPIRE_OTMA_TRANSACTION_ON_IMS_CONNECT_TIMEOUT
* @see ApiProperties#DO_NOT_EXPIRE_OTMA_TRANSACTION_ON_IMS_CONNECT_TIMEOUT
* @see ApiProperties#DEFAULT_OTMA_TRANSACTION_EXPIRATION
* @see TmInteractionAttributes#isOtmaTransactionExpiration()
*/
public void setOtmaTransactionExpiration(boolean otmaTransactionExpiration)
{
this.otmaTransactionExpiration = otmaTransactionExpiration;
}
/**
* Gets a boolean value representing whether undeliverable output will be purged.
* @return a boolean
value containing the purgeUndeliverableOutput property value
*/
public boolean isPurgeUndeliverableOutput()
{
return purgeUndeliverableOutput;
}
/**
* Sets the purgeUndeliverableOutput property value. The valid values are defined in ApiProperties
.
* @param aPurgeUndeliverableOutput the purgeUndeliverableOutput to set. Use the constants defined in ApiProperties to specify this parameter.
* @see TmInteraction#setPurgeUndeliverableOutput(boolean)
* @see TmInteraction#isPurgeUndeliverableOutput()
* @see ApiProperties#PURGE_UNDELIVERABLE_OUTPUT
* @see ApiProperties#DO_NOT_PURGE_UNDELIVERABLE_OUTPUT
*/
public void setPurgeUndeliverableOutput(boolean aPurgeUndeliverableOutput)
{
this.purgeUndeliverableOutput = aPurgeUndeliverableOutput;
}
/**
* Gets the RACF APPL name.
* @return a String
value containing the RACF APPL name property value
*/
public String getRacfApplName()
{
return this.racfApplName;
}
/**
* Sets the racfApplName property value.
* @param aRacfApplName
* the RACF application name to be used for this interaction
* @see TmInteraction#setRacfApplName(String)
* @see TmInteraction#getRacfApplName()
*/
public void setRacfApplName(String aRacfApplName)
{
this.racfApplName = aRacfApplName;
}
/**
* Gets the RACF Group name.
* @return a String
value containing the RACF group name property value
*/
public String getRacfGroupName()
{
return this.racfGroupName;
}
/**
* Set RACF Group name Sets the racfGroupName property value.
* @param aRacfGroupName
* the RACF group name to be used for this interaction.
* @see TmInteraction#setRacfGroupName(String)
* @see TmInteraction#getRacfGroupName()
*/
public void setRacfGroupName(String aRacfGroupName)
{
this.racfGroupName = aRacfGroupName;
}
/**
* Gets the RACF password.
* @return a String
value containing the RACF password property value
* @see TmInteraction#getRacfPassword()
*/
public String getRacfPassword()
{
return this.racfPassword;
}
/**
* Sets the racfPassword property value.
* @param aRacfPassword
* the RACF password to be used for this interaction.
* @see TmInteraction#setRacfPassword(String)
* @see TmInteraction#getRacfPassword()
*/
public void setRacfPassword(String aRacfPassword)
{
this.racfPassword = aRacfPassword;
}
/**
* Gets the RACF userID.
* @return a String
value containing the RACF userID property value
*/
public String getRacfUserId()
{
return this.racfUserId;
}
/**
* Sets the racfUserId property value.
* @param aRacfUserId
* the RACF userId to be used for this interaction.
* @see TmInteraction#setRacfUserId(String)
* @see TmInteraction#getRacfUserId()
*/
public void setRacfUserId(String aRacfUserId)
{
this.racfUserId = aRacfUserId;
}
/**
* Gets the reroute name property value.
* @return a String
value containing the rerouteName property
*/
public String getRerouteName()
{
return this.rerouteName;
}
/**
* Sets the rerouteName property value which specifies the optional name of a reroute destination
* (an OTMA Tpipe asynchronous hold queue).
* @param aRerouteName
* the rerouteName to be used for this interaction.
* @see TmInteraction#setRerouteName(String)
* @see TmInteraction#getRerouteName()
* @see ApiProperties#REROUTE_NAME_IMS_CONNECT_DEAD_LETTER_QUEUE
* @see ApiProperties#REROUTE_NAME_NONE
*/
public void setRerouteName(String aRerouteName)
{
this.rerouteName = aRerouteName;
}
/**
* Gets a boolean value representing whether undeliverable output will be rerouted to a
* different asynchronous hold queue.
* @return a boolean
value containing the rerouteUndeliverableOutput
* property value
*/
public boolean isRerouteUndeliverableOutput()
{
return rerouteUndeliverableOutput;
}
/**
* Sets the rerouteUndeliverableOutput property value.
* @param aRerouteUndeliverableOutput the rerouteUndeliverableOutput to set.
* Use the constants defined in ApiProperties to specify this parameter.
* @see TmInteraction#setRerouteUndeliverableOutput(boolean)
* @see TmInteraction#isRerouteUndeliverableOutput()
* @see ApiProperties#REROUTE_UNDELIVERABLE_OUTPUT
* @see ApiProperties#DO_NOT_REROUTE_UNDELIVERABLE_OUTPUT
*/
public void setRerouteUndeliverableOutput(boolean aRerouteUndeliverableOutput)
{
this.rerouteUndeliverableOutput = aRerouteUndeliverableOutput;
}
/**
* Gets the resumeTpipeAlternateClientID property value.
* @return a String
value containing the resumeTpipeAlternateClientId
* property value
* @see TmInteraction#getResumeTpipeAlternateClientId()
*/
public String getResumeTpipeAlternateClientId()
{
return resumeTpipeAlternateClientId;
}
/**
* Sets the resumeTpipeAlternateClientId property value.
* @param aResumeTpipeAlternateClientId
* the alternate client ID to set.
* @see TmInteraction#setResumeTpipeAlternateClientId(String)
* @see TmInteraction#getResumeTpipeAlternateClientId()
*/
public void setResumeTpipeAlternateClientId(String aResumeTpipeAlternateClientId)
{
this.resumeTpipeAlternateClientId = aResumeTpipeAlternateClientId;
}
/**
* Gets the resumeTpipeProcessing property value.
* @return an int
value containing the resumeTpipeProcessing property value.
* The meanings are defined in {@link ApiProperties}.
*/
public int getResumeTpipeProcessing()
{
return resumeTpipeProcessing;
}
/**
* Sets the resumeTpipeProcessing property value.
* @param resumeTpipeProcessing
* the resumeTpipeProcessing value to set. Use the constants defined in @lin {@link ApiProperties} to specify this parameter.
* @see TmInteraction#getResumeTpipeProcessing()
* @see TmInteraction#setResumeTpipeProcessing(int)
* @see ApiProperties#RESUME_TPIPE_SINGLE_NOWAIT
* @see ApiProperties#RESUME_TPIPE_NOAUTO
* @see ApiProperties#RESUME_TPIPE_SINGLE_WAIT
* @see ApiProperties#RESUME_TPIPE_AUTO
*/
public void setResumeTpipeProcessing(int resumeTpipeProcessing)
{
this.resumeTpipeProcessing = resumeTpipeProcessing;
}
/**
* Gets the returnMfsModname property value.
* @return a boolean
value containing the returnMfsModname property value
* @see TmInteraction#isReturnMfsModname()
* @see TmInteraction#setReturnMfsModname(boolean)
* @see ApiProperties#DO_RETURN_MFS_MODNAME
* @see ApiProperties#DO_NOT_RETURN_MFS_MODNAME
*/
public boolean isReturnMfsModname()
{
return this.returnMfsModname;
}
/**
* Sets the returnMfsModname property value.
* @param aReturnMfsModname the returnMfsModname to set. Use the constants defined in {@link ApiProperties} to specify this parameter.
* @see TmInteraction#isReturnMfsModname()
* @see TmInteraction#setReturnMfsModname(boolean)
* @see ApiProperties#DO_NOT_RETURN_MFS_MODNAME
* @see ApiProperties#DO_RETURN_MFS_MODNAME
*/
public void setReturnMfsModname(boolean aReturnMfsModname)
{
this.returnMfsModname = aReturnMfsModname;
}
/**
* Gets the syncLevel property value.
* @return a byte
value containing the syncLevel property value
* @see TmInteraction#getSyncLevel()
* @see TmInteraction#setSyncLevel(byte)
* @see ApiProperties#SYNC_LEVEL_CONFIRM
* @see ApiProperties#SYNC_LEVEL_NONE
*/
public byte getSyncLevel()
{
return this.syncLevel;
}
/**
* Sets the syncLevel property value.
* @param aSyncLevel
* a byte value representing the syncLevel. Use the constants defined in ApiProperties to specify this parameter.
* @see TmInteraction#setSyncLevel(byte)
* @see TmInteraction#getSyncLevel()
* @see ApiProperties#SYNC_LEVEL_CONFIRM
* @see ApiProperties#SYNC_LEVEL_NONE
*/
public void setSyncLevel(byte aSyncLevel)
{
this.syncLevel = aSyncLevel;
}
/**
* Gets the trancode property value.
* @return a String
value containing the trancode property value
* @see TmInteraction#setTrancode(String)
* @see TmInteraction#getTrancode()
*/
public String getTrancode()
{
return trancode;
}
/**
* Sets the trancode property value.
* @param aTrancode
* the trancode to be used for this interaction.
* @see TmInteraction#setTrancode(String)
* @see TmInteraction#getTrancode()
*/
public void setTrancode(String aTrancode)
{
this.trancode = aTrancode;
}
/**
* Gets the name of the XML Adapter to be used in the IMS Connect Adapter Task Manager
* @return a String
value containing the xmlAdapterName property value
*/
/* public String getXmlAdapterName()
{
return this.xmlAdapterName;
}
*/
/**
* Sets the name of the XML Adapter to be used in the IMS Connect Adapter Task Manager
*
* @param anXmlAdapterName
* the IMS Connect Adapter Task Manager adapter name
*/
/* public void setXmlAdapterName(String anXmlAdapterName)
{
this.xmlAdapterName = anXmlAdapterName;
}
*/
/**
* Gets the type of the adapter to be used in the IMS Connect Adapter Task Manager
* @return a byte
value containing the mlAdapterType property value
*/
/* public byte getXmlAdapterType()
{
return this.xmlAdapterType;
}
*/
/**
* Sets the type of the adapter to be used in the IMS Connect Adapter Task Manager
*
* @param anXmlAdapterType
* the IMS Connect Adapter Task Manager adapter type
*/
/* public void setXmlAdapterType(String anXmlAdapterType)
{
this.xmlAdapterType = anXmlAdapterType;
}
*/
/**
* Gets the name of the XML Converter to be used in the IMS Connect Adapter Task Manager
* @return a String
value containing the xmlConverterName property value
*/
/* public String getXmlConverterName()
{
return this.xmlConverterName;
}
*/
/**
* Sets the name of the XML Converter to be used in the IMS Connect Adapter Task Manager
*
* @param anXmlConverterName
* the IMS Connect Adapter Task Manager converter name
*/
/* public void setXmlConverterName(String anXmlConverterName)
{
this.xmlConverterName = anXmlConverterName;
}
*/
/**
* Gets the type of the XML message to be converted in IMS Connect
* @return a byte
value containing the xmlMessageType property value
*/
/* public byte getXmlMessageType()
{
return this.xmlMessageType;
}
*/
/**
* Sets the type of the XML message to be converted in IMS Connect
*
* @param anXmlMessageType
* the XML message type
*/
/* public void setXmlMessageType(String anXmlMessageType)
{
this.xmlMessageType = anXmlMessageType;
}
*/
// Other properties
/**
* Gets a boolean
value indicating whether each response message does or
* does not include LLLL
* @return a boolean
value containing whether the response messages
* returned to the IMS Connect API by IMS Connect start with LLLL (true -
* response messages processed using HWSSMPL1 in IMS Connect do start with
* LLLL) or do not start with LLLL (false - response messages processed
* using HWSSMPL0 do not start with LLLL)
*/
public boolean isResponseIncludesLlll()
{
return this.responseIncludesLlll;
}
/**
* Set a boolean
to include (true) or not include (false) the llll field value
* in the response message.
*
* @param responseIncludesLlll the responseIncludesLlll to set
*/
public void setResponseIncludesLlll(boolean responseIncludesLlll)
{
this.responseIncludesLlll = responseIncludesLlll;
}
//------------------Added for Sync callout------------------------------////
/**
* Sets the calloutRequestNakProcessing property value.
* @param aCalloutRequestNakProcessing the calloutRequestNakProcessing to set
* @see TmInteraction#setCalloutRequestNakProcessing(String)
*/
public void setCalloutRequestNakProcessing(
String aCalloutRequestNakProcessing) {
this.calloutRequestNakProcessing = aCalloutRequestNakProcessing;
}
/**
* Gets the calloutRequestNakProcessing property value.
* @return the calloutRequestNakProcessing
* @see TmInteraction#getCalloutRequestNakProcessing()
* @see TmInteraction#setCalloutRequestNakProcessing(String)
*/
public String getCalloutRequestNakProcessing() {
return calloutRequestNakProcessing;
}
/**
* Sets the calloutResponseMessageType property value.
*
* @param aCalloutResponseMessageType the calloutResponseMessageType to set
* @see TmInteraction#setCalloutResponseMessageType(String)
*/
public void setCalloutResponseMessageType(String aCalloutResponseMessageType) {
this.calloutResponseMessageType = aCalloutResponseMessageType;
}
/**
* Gets the calloutResponseMessageType property value.
* @return the calloutResponseMessageType
* @see TmInteraction#getCalloutResponseMessageType()
* @see TmInteraction#setCalloutResponseMessageType(String)
*/
public String getCalloutResponseMessageType() {
return calloutResponseMessageType;
}
/**
* Sets the resumeTpipeRetrievalType property value.
* @param aRetrievalType the resumeTpipeRetrievalType to set
* @see TmInteraction#setResumeTpipeRetrievalType(byte)
* @see TmInteraction#getResumeTpipeRetrievalType()
*/
public void setResumeTpipeRetrievalType(byte aRetrievalType) {
this.resumeTpipeRetrievalType = (byte) aRetrievalType;
}
/**
* Gets the current setting for the tpipe retrieval type.
* @return the resumeTpipeRetrievalType
* @see TmInteraction#getResumeTpipeRetrievalType()
*/
public byte getResumeTpipeRetrievalType() {
return resumeTpipeRetrievalType;
}
/**
* Sets the correlator token value.
* @param aCorrelatorToken
* @see TmInteraction#setCorrelatorToken(byte[])
*/
public void setCorrelatorToken(byte[] aCorrelatorToken){
this.correlatorTkn = aCorrelatorToken;
}
/**
* Gets the correlator token value.
* @return the correlatorTkn
*/
public byte[] getCorrelatorToken() {
return this.correlatorTkn;
}
/**
* Sets the nakReasonCode property value.
* @param aNakReasonCode the nakReasonCode to set
* @see TmInteraction#setNakReasonCode(short)
* @see TmInteraction#getNakReasonCode()
*/
public void setNakReasonCode(short aNakReasonCode) {
this.nakReasonCode = aNakReasonCode;
}
/**
* Gets the current NAK response reason code.
* @return the nakReasonCode
* @see TmInteraction#setNakReasonCode(short)
* @see TmInteraction#getNakReasonCode()
*/
public short getNakReasonCode() {
return nakReasonCode;
}
//--------------------End-----------------------------------------------////
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy