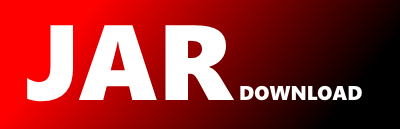
com.ibm.ims.connect.XmlParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of IMSConnectAPI Show documentation
Show all versions of IMSConnectAPI Show documentation
API that allows Java applications to interface with IMS Connect
/**
* File: XmlParser.java
* ==========================================================================
* Licensed Material - Property of IBM
*
* IBM Confidential
*
* OCO Source Materials
*
* 5655-TDA
*
* (C) Copyright IBM Corp. 2011, 2014 All Rights Reserved.
*
* The source code for this program is not published or
* otherwise divested of its trade secrets, irrespective
* of what has been deposited with the U.S. Copyright
* Office.
*
* US Government Users Restricted Rights - Use, duplication or
* disclosure restricted by GSA ADP Schedule Contract with
* IBM Corp.
* ===========================================================================
*/
package com.ibm.ims.connect;
import java.io.ByteArrayInputStream;
import java.io.InputStream;
import java.util.Arrays;
import java.util.List;
import java.util.logging.*;
import javax.xml.parsers.SAXParser;
import javax.xml.parsers.SAXParserFactory;
import org.xml.sax.InputSource;
import org.xml.sax.SAXParseException;
/**
* @author kevin
*
*/
public class XmlParser
{
private Logger logger;
public XmlParser()
{
logger = Logger.getLogger("com.ibm.ims.connect");
}
/*
* (non-Javadoc)
*
*/
public void parseXml(Type2CmdResponse aType2CmdRsp, byte[] aParsedOutDataByteArray, String anImsConnectCodepage) throws ImsConnectApiException
{
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("--> XmlParser.parseXml(Type2CmdResponse aType2CmdRsp, byte[] aParsedOutDataByteArray, String anImsConnectCodepage)");
/////All the below mentioned workaround has been revoed for moved to applyWorkaroundToResponseByteArray
///// workaround code to change \"skipb=\" to \" skipb=\" (insert a blank space after the first quote in the string \"skipb=\" wherever found
///// workaround code to change \"key=\" to \" key=\" (insert a blank space after the first quote in the string \"key=\" wherever found
///// workaround code to change \"align=\" to \" align=\" (insert a blank space after the first quote in the string \"align=\" wherever found
///// workaround code to change \"len=\" to \" len=\" (insert a blank space after the first quote in the string \"len=\" wherever found
///// workaround code to change "< " to "<" (as in < xmlvsn> to - remove a blank space after the '<' wherever found
///// workaround code to remove any binary 0's or 1's found - it's a temporary workaround and should be removed as soon as all of the problems in Connect and IMS are fixed
// int i = 0, j = 0, k = 0;
// int byteArrayLength = cleanedUpResponseByteArray.length;
// while(i < byteArrayLength)
// {
// if((cleanedUpResponseByteArray[i] == (byte) 0x00) || (cleanedUpResponseByteArray[i] == (byte) 0x01))
// {
// int cleanedUpResponseByteArrayLength = cleanedUpResponseByteArray.length - 1;
// byte[] newCleanedUpResponseByteArray = new byte[cleanedUpResponseByteArrayLength];
// System.arraycopy(cleanedUpResponseByteArray, 0, newCleanedUpResponseByteArray, 0, i);
// System.arraycopy(cleanedUpResponseByteArray, i+1, newCleanedUpResponseByteArray, i, cleanedUpResponseByteArrayLength - i);
// k = i + j++;
//// System.out.println("\"" + cleanedUpResponseByteArray[i] + "\" removed from response byte array at offset " + k + " in response message");
// cleanedUpResponseByteArray = newCleanedUpResponseByteArray.clone();
// byteArrayLength = cleanedUpResponseByteArray.length;
// }
// else
// {
// i++;
// j = 0;
// }
// }
///// end workaround
/////// debug println
// System.out.println("cleanedUpResponseByteArray:");
// System.out.println(ResponseProcessor.formatBufferForTracing(cleanedUpResponseByteArray, ApiProperties.DEFAULT_CODEPAGE_EBCDIC_US));
/////// end debug println
// byte[] cleanedUpResponseByteArray = applyWorkaroundToResponseByteArray(aParsedOutDataByteArray,anImsConnectCodepage);
// InputStream inStrm = new ByteArrayInputStream(cleanedUpResponseByteArray);
InputStream inStrm = new ByteArrayInputStream(aParsedOutDataByteArray);
SAXParser saxParser = null;
try
{
SAXParserFactory factory = SAXParserFactory.newInstance();
saxParser = factory.newSAXParser();
MyDefaultHandler handler = new MyDefaultHandler();
InputSource inSrc = new InputSource(inStrm);
inSrc.setSystemId(this.getClass().getClassLoader().getResource("imsout.dtd").toString()); // modified to point to DTD in API JAR file
handler.setMyT2CmdRsp(aType2CmdRsp);
saxParser.parse(inSrc, handler);
}
catch(SAXParseException spe)
{
StringBuffer sb = new StringBuffer( spe.toString() );
sb.append("\n Line number: " + spe.getLineNumber());
sb.append("\nColumn number: " + spe.getColumnNumber() );
sb.append("\n Public ID: " + spe.getPublicId() );
sb.append("\n System ID: " + spe.getSystemId() + "\n");
//System.out.println( sb.toString() );
String errMsg = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0044E,
new Object[] {sb.toString()});
ImsConnectApiException e = new ImsConnectApiException(ImsConnectErrorMessage.HWS0044E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception caught in XmlParser.parseXml(Type2CmdResponse aType2CmdRsp, byte[] aParsedOutDataByteArray, String anImsConnectCodepage): [" + sb.toString() + "]");
throw e;
}
catch (Throwable e)
{
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0001E, new Object[] {ImsConnectErrorMessage.getExceptionMessage(e) });
ImsConnectApiException e1 = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0001E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception thrown in parseXml(Type2CmdResponse aType2CmdRsp, byte[] aParsedOutDataByteArray, String anImsConnectCodepage). Exception thrown was: " + e1.toString());
throw e1;
}
finally
{
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("<-- XmlParser.parseXml(Type2CmdResponse aType2CmdRsp, byte[] aParsedOutDataByteArray, String anImsConnectCodepage)");
}
}
private byte[] applyWorkaroundToResponseByteArray(byte[] aParsedOutDataByteArray, String anImsConnectCodepage) throws ImsConnectApiException
{
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("--> XmlParser.applyWorkaroundToResponseByteArray(byte[] aParsedOutDataByteArray, String aBadString, String aReplaceString, String anImsConnectCodepage)");
String responseString = null;
String cleanedUpResponseString = null;
byte[] cleanedUpResponseByteArray = null;
List badString = Arrays.asList("\"skipb=\"","\"key=\"","\"align=\"","\"len=\"","< ");
List replacementString = Arrays.asList("\" skipb=\"","\" key=\"","\" align=\"","\" len=\"","<");
try
{
responseString = new String (aParsedOutDataByteArray, anImsConnectCodepage);
for(int i =0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy