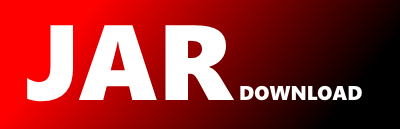
com.ibm.ims.connect.impl.CtlImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of IMSConnectAPI Show documentation
Show all versions of IMSConnectAPI Show documentation
API that allows Java applications to interface with IMS Connect
/**
* File: CtlImpl.java
* ==========================================================================
* Licensed Material - Property of IBM
*
* IBM Confidential
*
* OCO Source Materials
*
* 5655-TDA
*
* (C) Copyright IBM Corp. 2011, 2013 All Rights Reserved.
*
* The source code for this program is not published or
* otherwise divested of its trade secrets, irrespective
* of what has been deposited with the U.S. Copyright
* Office.
*
* US Government Users Restricted Rights - Use, duplication or
* disclosure restricted by GSA ADP Schedule Contract with
* IBM Corp.
* ===========================================================================
*/
package com.ibm.ims.connect.impl;
import com.ibm.ims.connect.Ctl;
/**
* @author kevin
*
*/
public class CtlImpl implements Ctl
{
private String ctlElementText;
private String omname = null;
private String omvsn = null;
private String xmlvsn = null;
private String statime = null;
private String stotime = null;
private String staseq = null;
private String stoseq = null;
private byte[] rqsttkn1 = null;
private byte[] rqsttkn2 = null;
private String rc = null;
private String rsn = null;
private String rsnmsg = null;
private String rsntxt = null;
private String uom = null;
/**
* @return the ctlElementText
*/
public String getCtlElementText()
{
return this.ctlElementText;
}
/**
* @param aCtlElementText the ctlElementText to set
*/
public void setCtlElementText(String aCtlElementText)
{
this.ctlElementText = aCtlElementText;
}
/**
* @return the omname
*/
public String getOmname()
{
return this.omname;
}
/**
* @param anOmname the omname to set
*/
public void setOmname(String anOmname)
{
this.omname = anOmname;
}
/**
* @return the omvsn
*/
public String getOmvsn()
{
return this.omvsn;
}
/**
* @param anOmvsn the omvsn to set
*/
public void setOmvsn(String anOmvsn)
{
this.omvsn = anOmvsn;
}
/**
* @return the rc
*/
public String getRc()
{
return this.rc;
}
/**
* @param aRc the rc to set
*/
public void setRc(String aRc)
{
this.rc = aRc;
}
/**
* @return the rqsttkn1
*/
public byte[] getRqsttkn1()
{
return this.rqsttkn1;
}
/**
* @param aRqsttkn1 the rqsttkn1 to set
*/
public void setRqsttkn1(byte[] aRqsttkn1)
{
this.rqsttkn1 = aRqsttkn1;
}
/**
* @return the rqsttkn2
*/
public byte[] getRqsttkn2()
{
return this.rqsttkn2;
}
/**
* @param aRqsttkn2 the rqsttkn2 to set
*/
public void setRqsttkn2(byte[] aRqsttkn2)
{
this.rqsttkn2 = aRqsttkn2;
}
/**
* @return the rsn
*/
public String getRsn()
{
return this.rsn;
}
/**
* @param aRsn the rsn to set
*/
public void setRsn(String aRsn)
{
this.rsn = aRsn;
}
/**
* @return the rsnmsg
*/
public String getRsnmsg()
{
return this.rsnmsg;
}
/**
* @param aRsnmsg the rsnmsg to set
*/
public void setRsnmsg(String aRsnmsg)
{
this.rsnmsg = aRsnmsg;
}
/**
* @return the rsntxt
*/
public String getRsntxt()
{
return this.rsntxt;
}
/**
* @param aRsntxt the rsntxt to set
*/
public void setRsntxt(String aRsntxt)
{
this.rsntxt = aRsntxt;
}
/**
* @return the staseq
*/
public String getStaseq()
{
return this.staseq;
}
/**
* @param aStaseq the staseq to set
*/
public void setStaseq(String aStaseq)
{
this.staseq = aStaseq;
}
/**
* @return the statime
*/
public String getStatime()
{
return this.statime;
}
/**
* @param aStatime the statime to set
*/
public void setStatime(String aStatime)
{
this.statime = aStatime;
}
/**
* @return the stoseq
*/
public String getStoseq()
{
return this.stoseq;
}
/**
* @param aStoseq the stoseq to set
*/
public void setStoseq(String aStoseq)
{
this.stoseq = aStoseq;
}
/**
* @return the stotime
*/
public String getStotime()
{
return this.stotime;
}
/**
* @param aStotime the stotime to set
*/
public void setStotime(String aStotime)
{
this.stotime = aStotime;
}
/**
* @return the uom
*/
public String getUom()
{
return this.uom;
}
/**
* @param aUom the uom to set
*/
public void setUom(String aUom)
{
this.uom = aUom;
}
/**
* @return the xmlvsn
*/
public String getXmlvsn()
{
return this.xmlvsn;
}
/**
* @param anXmlvsn the xmlvsn to set
*/
public void setXmlvsn(String anXmlvsn)
{
this.xmlvsn = anXmlvsn;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy