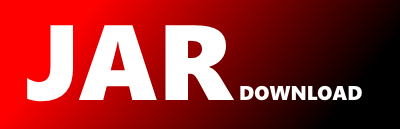
com.ibm.ims.connect.impl.HdrImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of IMSConnectAPI Show documentation
Show all versions of IMSConnectAPI Show documentation
API that allows Java applications to interface with IMS Connect
/**
* File: HdrImpl.java
* ==========================================================================
* Licensed Material - Property of IBM
*
* IBM Confidential
*
* OCO Source Materials
*
* 5655-TDA
*
* (C) Copyright IBM Corp. 2011, 2013 All Rights Reserved.
*
* The source code for this program is not published or
* otherwise divested of its trade secrets, irrespective
* of what has been deposited with the U.S. Copyright
* Office.
*
* US Government Users Restricted Rights - Use, duplication or
* disclosure restricted by GSA ADP Schedule Contract with
* IBM Corp.
* ===========================================================================
*/
package com.ibm.ims.connect.impl;
import com.ibm.ims.connect.Hdr;
import com.ibm.ims.connect.ApiProperties;
import com.ibm.ims.connect.ImsConnectApiException;
import com.ibm.ims.connect.ImsConnectErrorMessage;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.UnsupportedEncodingException;
import java.util.InvalidPropertiesFormatException;
import java.util.logging.*;
import java.util.Properties;
import java.util.Vector;
import org.xml.sax.Attributes;
/**
* @author kevin
*
*/
public class HdrImpl implements Hdr
{
private String elementText = null;
private String uri = null;
private Attributes attributes = null;
private Properties myProperties = new Properties();
private String slbl = null;
private String llbl = null;
private String scope = null;
private String sort = null;
private String key = null;
private String scroll = null;
private String len = null;
private String dtype = null;
private String skipb = null;
private String align = null;
private Logger logger;
/**
* @param String anElementText the elementText to set
*/
public HdrImpl(String anElementText)
{
logger = Logger.getLogger("com.ibm.ims.connect");
this.setElementText(anElementText);
}
/**
* @return the elementText
*/
public String getHdrAttributesAsXmlString()
{
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("--> Hdr.getHdrAttributesAsXmlString()");
StringBuffer hdrAttributesAsXmlStringBuffer = new StringBuffer();
hdrAttributesAsXmlStringBuffer.append("" + // encoding=\"UTF-8\"?>" +
"");
if (this.getSlbl() != null)
hdrAttributesAsXmlStringBuffer.append("" + this.getSlbl() + " " );
if (this.getLlbl() != null)
hdrAttributesAsXmlStringBuffer.append("" + this.getLlbl() + " " );
if (this.getScope() != null)
hdrAttributesAsXmlStringBuffer.append("" + this.getScope() + " " );
if (this.getSort() != null)
hdrAttributesAsXmlStringBuffer.append("" + this.getSort() + " " );
if (this.getKey() != null)
hdrAttributesAsXmlStringBuffer.append("" + this.getKey() + " " );
if (this.getScroll() != null)
hdrAttributesAsXmlStringBuffer.append("" + this.getScroll() + " " );
if (this.getLen() != null)
hdrAttributesAsXmlStringBuffer.append("" + this.getLen() + " " );
if (this.getDtype() != null)
hdrAttributesAsXmlStringBuffer.append("" + this.getDtype() + " " );
if (this.getSkipb() != null)
hdrAttributesAsXmlStringBuffer.append("" + this.getKey() + " " );
if (this.getAlign() != null)
hdrAttributesAsXmlStringBuffer.append("" + this.getKey() + " " );
hdrAttributesAsXmlStringBuffer.append(" ");
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("<-- Hdr.getHdrAttributesAsXmlString()");
return hdrAttributesAsXmlStringBuffer.toString();
}
/**
* @return the elementText
*/
public Properties getHdrElementAttributesAsPropertiesObject(String anEncoding)
throws ImsConnectApiException
{
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("--> Hdr.getHdrAttributesAsPropertiesObject(String anEncoding)");
try
{
InputStream is = new ByteArrayInputStream(this.getHdrAttributesAsXmlString().getBytes(anEncoding));
// reset myProperties object
myProperties.clear();
// load the xml file into properties format
myProperties.loadFromXML(is);
}
catch (UnsupportedEncodingException usee)
{
String errMsg = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0017E,
new Object[] {"Type-2 command cmdrsphdr", anEncoding});
ImsConnectApiException e = new ImsConnectApiException(ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception caught in Hdr.getHdrAttributesAsPropertiesObject(String anEncoding): [" + e.toString() + "]");
throw e;
}
catch (InvalidPropertiesFormatException ipfe)
{
String errMsg = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0044E,
new Object[] {ipfe.toString()});
ImsConnectApiException e = new ImsConnectApiException(ImsConnectErrorMessage.HWS0044E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception caught in Hdr.getHdrAttributesAsPropertiesObject(String anEncoding): [" + ipfe.toString() + "]");
throw e;
}
catch (IOException ioe)
{
String errMsg = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0044E,
new Object[] {ioe.toString()});
ImsConnectApiException e = new ImsConnectApiException(ImsConnectErrorMessage.HWS0044E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception caught in Hdr.getHdrAttributesAsPropertiesObject(String anEncoding): [" + e.toString() + "]+");
throw e;
}
catch (Exception e)
{
String errMsg = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0044E,
new Object[] {e.toString()});
ImsConnectApiException e1 = new ImsConnectApiException(ImsConnectErrorMessage.HWS0044E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception caught in Hdr.getHdrAttributesAsPropertiesObject(String anEncoding): [" + e1.toString() + "]+");
throw e1;
}
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("<-- Hdr.getHdrAttributesAsPropertiesObject(String anEncoding)");
return myProperties;
}
/**
* @return the elementText
*/
public String getElementText()
{
return this.elementText;
}
/**
* @param anElementText the elementText to set
*/
public void setElementText(String anElementText)
{
this.elementText = anElementText;
}
/**
* @return the uri
*/
public String getUri()
{
return this.uri;
}
/**
* @param uri the uri to set
*/
public void setUri(String uri)
{
this.uri = uri;
}
/**
* @return the attributes
*/
public Attributes getAttributes()
{
return this.attributes;
}
/**
* @param attributes the attributes to set
*/
public void setAttributes(Attributes attributes)
{
this.attributes = attributes;
}
/**
* @return the slbl
*/
public String getSlbl()
{
return this.slbl;
}
/**
* @param slbl the slbl to set
*/
public void setSlbl(String slbl)
{
this.slbl = slbl;
}
/**
* @return the llbl
*/
public String getLlbl()
{
return this.llbl;
}
/**
* @param llbl the llbl to set
*/
public void setLlbl(String llbl)
{
this.llbl = llbl;
}
/**
* @return the scope
*/
public String getScope()
{
return this.scope;
}
/**
* @param scope the scope to set
*/
public void setScope(String scope)
{
this.scope = scope;
}
/**
* @return the sort
*/
public String getSort()
{
return this.sort;
}
/**
* @param sort the sort to set
*/
public void setSort(String sort)
{
this.sort = sort;
}
/**
* @return the key
*/
public String getKey()
{
return this.key;
}
/**
* @param key the key to set
*/
public void setKey(String key)
{
this.key = key;
}
/**
* @return the scroll
*/
public String getScroll()
{
return this.scroll;
}
/**
* @param scroll the scroll to set
*/
public void setScroll(String scroll)
{
this.scroll = scroll;
}
/**
* @return the len
*/
public String getLen()
{
return this.len;
}
/**
* @param len the len to set
*/
public void setLen(String len)
{
this.len = len;
}
/**
* @return the dtype
*/
public String getDtype()
{
return this.dtype;
}
/**
* @param dtype the dtype to set
*/
public void setDtype(String dtype)
{
this.dtype = dtype;
}
/**
* @return the skipb
*/
public String getSkipb()
{
return this.skipb;
}
/**
* @param skipb the skipb to set
*/
public void setSkipb(String skipb)
{
this.skipb = skipb;
}
/**
* @return the align
*/
public String getAlign()
{
return this.align;
}
/**
* @param align the align to set
*/
public void setAlign(String align)
{
this.align = align;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy