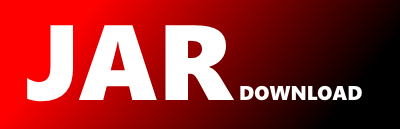
com.ibm.ims.connect.impl.InputMessageImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of IMSConnectAPI Show documentation
Show all versions of IMSConnectAPI Show documentation
API that allows Java applications to interface with IMS Connect
/*
* File: InputMessageImpl.java
* ==========================================================================
* Licensed Material - Property of IBM
*
* IBM Confidential
*
* OCO Source Materials
*
* 5655-TDA
*
* (C) Copyright IBM Corp. 2009, 2014 All Rights Reserved.
*
* The source code for this program is not published or
* otherwise divested of its trade secrets, irrespective
* of what has been deposited with the U.S. Copyright
* Office.
*
* US Government Users Restricted Rights - Use, duplication or
* disclosure restricted by GSA ADP Schedule Contract with
* IBM Corp.
* ===========================================================================
*/
package com.ibm.ims.connect.impl;
import java.io.UnsupportedEncodingException;
import java.nio.ByteBuffer;
import java.util.ArrayList;
import java.util.Formatter;
import java.util.logging.Logger;
import com.ibm.ims.connect.ApiProperties;
import com.ibm.ims.connect.ImsConnectApiException;
import com.ibm.ims.connect.ImsConnectErrorMessage;
import com.ibm.ims.connect.InputMessage;
import com.ibm.ims.connect.InputMessageProperties;
import com.ibm.ims.connect.TmInteraction;
/**
* The class represents the input message for the IMS transaction. It provides
* setter and getter methods to provide values for the LL and ZZ values along
* with the data for each segment in the input message to be sent to IMS Connect
* to invoke the IMS transaction.
*
* @author Kevin Flanigan
*
*/
public class InputMessageImpl implements InputMessage {
@SuppressWarnings("unused")
private static final String copyright = "Licensed Material - Property of IBM "
+ "5655-T62"
+ "(C) Copyright IBM Corp. 2009,2012 All Rights Reserved. "
+ "US Government Users Restricted Rights - Use, duplication or "
+ "disclosure restricted by GSA ADP Schedule Contract with IBM Corp. ";
// IRM fields
private int llll = 0;
private int irmLen = 0;
private byte irmArch;
private byte irmF0;
private byte[] irmId;
private short irmResWord;
private int irmF5;
private int irmTimer;
private byte irmSocType;
private byte irmEncodingSchema;
private byte[] irmClientId;
private byte irmF1;
private byte irmF2;
private byte irmF3;
private char irmF4;
private byte[] irmTrancode;
private byte[] irmDestId;
private byte[] irmLTerm;
private byte[] irmRacfUserId;
private byte[] irmRacfGroupName;
private byte[] irmRacfPassword;
private byte[] irmRacfApplName;
private byte[] irmRerouteName;
private byte[] irmTagAdapter;
private byte[] irmTagMap;
// ------------------Added for Sync
// callout------------------------------////
private short irmNakReasonCode = 0x00;
private byte[] irmInputModname;
private byte[] irmCorelatorToken;
// --------------End--------------------------------------------------------/////
// fields in data portion of input message
private byte[] trancodeInData;
private int trancodeInDataLength = 0;
@SuppressWarnings("unused")
private byte[] trancodeInDataToBeUsed; // set and used in getBytes() to
// build message
private byte[] data = null; // a 1-dimensional bytearray containing the
// input data including LLZZ and trancode only
// if they are supplied by the user in the input
// data
// private byte[][] dataSegmentsWithoutLlzzAndTrancode = null;
private String imsConnectCodepage = DEFAULT_CODEPAGE_EBCDIC_US;
private boolean inputMessageDataSegmentsIncludeLlzzAndTrancode = false;
private boolean connectionPropertiesAlreadySet = false;
private boolean tmInteractionPropertiesAlreadySet = false;
// Other fields
private int i = 0;
private Logger logger;
private TmInteractionImpl myTmInteractionImpl;
private String aCodepage;
private byte[] message = null; // input message built in
// buildInputMessageByteArray() method and
// sent to IMS Connect (includes LLLL, LLZZ,
// and trancode and data as needed)
int messageLength = 0;
private ArrayList inputData = new ArrayList(); // Vector of
// byte[]'s
// containing
// the input
// data parsed
// into segments
// - (includes
// only data -
// LLLL, LLZZ
// and trancode
// not included)
private int numberOfSegments;
private int[] segmentLengths;
private int bytesProcessed = 0;
private int bytesCopied = 0;
private boolean inConversation;
private boolean rebuildData; // set to true if the InputMessageImpl.data
// needs to be rebuilt because the data or
// conversational state changed
private boolean tranRemoved = false;
byte[] aBlankTrancodeByteArray = null; // a byte array containing 8 blanks
// for use as trancode for
// interactions that do not require
// a trancode (e.g. ACK, NAK,
// RESUMETPIPE)
byte[] anEmptyTrancodeByteArray = {}; // an empty byte array for use as
// trancode for interactions that do
// not require a trancode (e.g. ACK,
// NAK, RESUMETPIPE)
/**
*
*/
public InputMessageImpl(TmInteraction myTMInteraction)
throws ImsConnectApiException {
logger = Logger.getLogger("com.ibm.ims.connect");
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("--> InputMessage(TmInteraction)");
myTmInteractionImpl = (TmInteractionImpl) myTMInteraction;
this.reset();
this.setImsConnectCodepage(myTmInteractionImpl.getImsConnectCodepage());
try {
aBlankTrancodeByteArray = new String(" ")
.getBytes(imsConnectCodepage);
} catch (UnsupportedEncodingException usee) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0017E, new Object[] {
"input request message", this.imsConnectCodepage });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage constructor: ["
+ e.getMessage() + "]+");
throw e;
}
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("<-- InputMessage(TmInteraction)");
}
public void reset() throws ImsConnectApiException {
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("--> InputMessage.reset()");
setLlll(0);
this.setImsConnectCodepage(DEFAULT_CODEPAGE_EBCDIC_US);
this.setIrmLen(InputMessageProperties.IRM_LEN_DEFAULT);
this.setIrmArch(DEFAULT_ARCH_LEVEL);
this.setIrmF0(InputMessageProperties.IRM_F0_NOFLAG);
this.setIrmId(InputMessageProperties.IRM_ID_DEFAULT);
// ------------------Added for Sync
// callout------------------------------////
this.setIrmNakReasonCode(IRM_NAK_REASONCODE_DEFAULT);
// ---------------------End------------------------------------
this.setIrmResWord(InputMessageProperties.IRM_RES_HALFWORD_DEFAULT);
this.setIrmF5(InputMessageProperties.IRM_F5_NOFLAG);
this.setIrmTimer(InputMessageProperties.IRM_TIME_QS);
this.setIrmSocType(InputMessageProperties.IRM_SOCT_PER);
this.setIrmEncodingSchema(InputMessageProperties.IRM_ES_NOFLAG);
this.setIrmClientId(InputMessageProperties.IRM_CLIENTID_DEFAULT);
this.setIrmF1(InputMessageProperties.IRM_F1_NOFLAG);
this.setIrmF2(InputMessageProperties.IRM_F2_CMODE1);
this.setIrmF3(InputMessageProperties.IRM_F3_SYNC_NONE);
this.setIrmF4(InputMessageProperties.IRM_F4_DATA);
this.setIrmTrancode(InputMessageProperties.IRM_TRANCODE_DEFAULT);
this.setIrmDestId(InputMessageProperties.IRM_IMSDESTID_DEFAULT);
this.setIrmLTerm(InputMessageProperties.IRM_LTERM_DEFAULT);
this.setIrmRacfUserId(InputMessageProperties.IRM_RACF_USERID_DEFAULT);
this
.setIrmRacfGroupName(InputMessageProperties.IRM_RACF_GRNAME_DEFAULT);
this.setIrmRacfPassword(InputMessageProperties.IRM_RACF_PW_DEFAULT);
this.setIrmRacfApplName(InputMessageProperties.IRM_APPL_NM_DEFAULT);
this.setIrmRerouteName(InputMessageProperties.IRM_REROUT_NM_DEFAULT);
this.setIrmTagAdapter(InputMessageProperties.IRM_TAG_ADAPT_DEFAULT);
this.setIrmTagMap(InputMessageProperties.IRM_TAG_MAP_DEFAULT);
// ------------------Added for Sync
// callout------------------------------////
this.setIrmInputModName(ApiProperties.DEFAULT_INPUT_MOD_NAME);
this.setIrmCorelatorToken(ApiProperties.CORRELATOR_TOKEN_DEFAULT);
// -------------------End-----------------------------------------
setInputMessageDataSegmentsIncludeLlzzAndTrancode(false);
setData(null);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL)) {
logger.finer(" InputMessage.llll reset to 0");
logger
.finer(" InputMessage IRM properties reset to default values");
logger
.finer(" InputMessage.inputMessageDataSegmentsIncludeLlzzAndTrancode reset to false");
logger.finer(" InputMessage.data reset to null");
}
this.setConnectionPropertiesAlreadySet(false);
this.setTmInteractionPropertiesAlreadySet(false);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("<-- InputMessage.reset()");
}
public void copyInputMessage(InputMessageImpl anInputMessage)
throws UnsupportedEncodingException {
setLlll(anInputMessage.getLlll());
this.imsConnectCodepage = anInputMessage.getImsConnectCodepage();
this.irmLen = anInputMessage.getIrmLen();
this.irmArch = anInputMessage.getIrmArch();
this.irmF0 = anInputMessage.getIrmF0();
this.irmId = anInputMessage.getIrmId();
// ------------------Added for Sync
// callout------------------------------////
this.irmNakReasonCode = anInputMessage.getIrmNakReasonCode();
// ------------------End------------------------------////
this.irmResWord = anInputMessage.getIrmResWord();
this.irmF5 = anInputMessage.getIrmF5();
this.irmTimer = anInputMessage.getIrmTimer();
this.irmSocType = anInputMessage.getIrmSocType();
this.irmEncodingSchema = anInputMessage.getIrmEncodingSchema();
this.irmClientId = anInputMessage.getIrmClientId();
this.irmF1 = anInputMessage.getIrmF1();
this.irmF2 = anInputMessage.getIrmF2();
this.irmF3 = anInputMessage.getIrmF3();
this.irmF4 = anInputMessage.getIrmF4();
this.irmTrancode = anInputMessage.getIrmTrancode();
this.irmDestId = anInputMessage.getIrmDestId();
this.irmLTerm = anInputMessage.getIrmLTerm();
this.irmRacfUserId = anInputMessage.getIrmRacfUserId();
this.irmRacfGroupName = anInputMessage.getIrmRacfGroupName();
this.irmRacfPassword = anInputMessage.getIrmRacfPassword();
this.irmRacfApplName = anInputMessage.getIrmRacfApplName();
this.irmRerouteName = anInputMessage.getIrmRerouteName();
this.irmTagAdapter = anInputMessage.getIrmTagAdapter();
this.irmTagMap = anInputMessage.getIrmTagMap();
this.irmInputModname = anInputMessage.getIrmInputModName();
this.irmCorelatorToken = anInputMessage.getIrmCorelatorToken();
this.setInputMessageDataSegmentsIncludeLlzzAndTrancode(anInputMessage
.isInputMessageDataSegmentsIncludeLlzzAndTrancode());
this.setData(anInputMessage.getData());
}
public void setConnectionProperties(ConnectionImpl aConnImpl)
throws ImsConnectApiException {
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger
.finer("--> InputMessage.setConnectionProperties(ConnectionImpl)");
this.setIrmSocType(aConnImpl.getSocketType());
if (aConnImpl.updateIrmClientId) {
this.setIrmClientId(aConnImpl.getClientId());
aConnImpl.updateIrmClientId = false;
}
this.connectionPropertiesAlreadySet = true;
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger
.finer("<-- InputMessage.setConnectionProperties(ConnectionImpl)");
}
public void setTmInteractionProperties() throws ImsConnectApiException {
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("--> InputMessage.setTMInteractionProperties()");
String interTypeDesc = ((this.myTmInteractionImpl
.getInteractionTypeDescription()).trim());
String iconUserMessageExitId = this.myTmInteractionImpl
.getImsConnectUserMessageExitIdentifier();
this.setImsConnectCodepage(this.myTmInteractionImpl
.getImsConnectCodepage());
/*
* System.out.println("CodePage = " +
* this.myTmInteractionImpl.getImsConnectCodepage() +
* this.getImsConnectCodepage());
*/
this
.setIrmArch((byte) (this.myTmInteractionImpl
.getArchitectureLevel()));
this.setIrmF0(this.myTmInteractionImpl.getXmlMessageType());
if (this.myTmInteractionImpl.updateIrmId) {
// if (interTypeDesc.equals(INTERACTION_TYPE_DESC_TYPE2_COMMAND)) {
// this.setIrmId("*HWSCS1*");
// } else {
this.setIrmId(iconUserMessageExitId);
// }
this.myTmInteractionImpl.updateIrmId = false;
}
/*
* System.out.println("this.getIrmId()=" + this.getIrmId().toString());
*
* byte[] A = this.getIrmId(); for (int s = 0; s < A.length; s++)
* System.out.print(A[s] + " "); System.out.println("");
*/
if (iconUserMessageExitId.equals("*SAMPLE*"))
this.myTmInteractionImpl
.setResponseIncludesLlll(ApiProperties.RESPONSE_DOES_NOT_INCLUDE_LLLL);
else if ((iconUserMessageExitId.equals("*SAMPL1*"))
|| (iconUserMessageExitId.equals("*HWSCSL*"))
|| (iconUserMessageExitId.equals("*HWSCS1*")))
this.myTmInteractionImpl
.setResponseIncludesLlll(ApiProperties.RESPONSE_DOES_INCLUDE_LLLL);
// ------------------Added for Sync
// callout------------------------------////
this.setIrmNakReasonCode(this.myTmInteractionImpl.getNakReasonCode());
// --------------------End-------------------------------------
this.setIrmResWord(InputMessageProperties.IRM_RES_HALFWORD_DEFAULT);
// Clear out irmF5 prior to OR'ing in new values using setIRM_F5 calls
this.irmF5 = (byte) 0x00;
this.setIrmF5(this.myTmInteractionImpl.getInputMessageOptions()); // irm_F5
// value
// logged
// later
// Commented out by shali from Dave Cameron's suggestion
// setIrmF5(this.myTmInteractionImpl.getResumeTpipeProcessing());
// if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL))
// logger.finer(" InputMessage.irmF5 set to [" + this.getIrmF5() +
// "]"); // irmF5 modified later on so print out value then
this.setIrmTimer(this.myTmInteractionImpl.getImsConnectTimeoutIndex());
this.setIrmEncodingSchema(this.myTmInteractionImpl
.getImsConnectUnicodeEncodingSchema());
// Clear out irmF1 prior to OR'ing in new values using setIRM_F1 calls
this.irmF1 = (byte) 0x00; // irm_Fx values logged after irm_F5 has been
// set
this
.setIrmF1(this.myTmInteractionImpl
.isOtmaTransactionExpiration() ? InputMessageProperties.IRM_F1_TRANEXP
: 0x00);
this.setIrmF1(this.myTmInteractionImpl.getImsConnectUnicodeUsage());
this.setIrmF1(this.myTmInteractionImpl.isReturnMfsModname() ? InputMessageProperties.IRM_F1_MFSREQ
: 0x00);
this.setIrmF1(this.myTmInteractionImpl.isCM0AckNoWaitCanBeUsed() ? InputMessageProperties.IRM_F1_CM0ANW
: 0x00);
this.setIrmF1(this.myTmInteractionImpl.isReturnClientId() ? InputMessageProperties.IRM_F1_CIDREQ
: 0x00);
this.setIrmF2(this.myTmInteractionImpl.getCommitMode());
//Adding AutoGeneration of client ID by IMS connect incase of duplicate clientId set
if (this.myTmInteractionImpl.isGenerateClientIdWhenDuplicate())
this.irmF2 |= (byte) 0x01;
// Clear out irmF3 prior to OR'ing in new values using setIRM_F3 calls
if (this.myTmInteractionImpl.isCancelClientId())
this.irmF3 = (byte) 0x80; // initialize irmF3 to cancelClientId since we
else
this.irmF3 = (byte) 0x00;
// always want to use it (as long as we are
// not doing a Cancel Timer interaction) and
// do not expose it
this.setIrmF3(this.myTmInteractionImpl.getSyncLevel());
if (this.myTmInteractionImpl.isPurgeUndeliverableOutput())
this.setIrmF3((byte) 0x04);
if (this.myTmInteractionImpl.isRerouteUndeliverableOutput())
this.setIrmF3((byte) 0x08);
if (this.myTmInteractionImpl.isCm0IgnorePurge())
this.setIrmF3((byte) 0x20);
if (this.myTmInteractionImpl
.isReturnDFS2082AfterCM0SendRecvNoResponse())
this.setIrmF3((byte) 0x40);
if (interTypeDesc.equals(INTERACTION_TYPE_DESC_ACK)) {
if (this.myTmInteractionImpl.updateIrmInteractionType) {
this.setIrmF4(InputMessageProperties.IRM_F4_ACK);
this.myTmInteractionImpl.updateIrmInteractionType = false;
}
} else if (interTypeDesc.equals(INTERACTION_TYPE_DESC_CANCELTIMER)) {
if (this.myTmInteractionImpl.updateIrmInteractionType) {
this.setIrmF4(InputMessageProperties.IRM_F4_CANTIMER);
this.myTmInteractionImpl.updateIrmInteractionType = false;
}
this.irmF3 &= (byte) 0x7F; // suppress cancelClientId flag when
// executing a cancel timer interaction
} else if (interTypeDesc.equals(INTERACTION_TYPE_DESC_ENDCONVERSATION)) {
if (this.myTmInteractionImpl.updateIrmInteractionType) {
this.setIrmF4(InputMessageProperties.IRM_F4_DEALLOC);
this.myTmInteractionImpl.updateIrmInteractionType = false;
}
} else if (interTypeDesc.equals(INTERACTION_TYPE_DESC_RECEIVE)) {
// setIRM_F4(InputMessageProperties.IRM_F4_RECEIVE); // not required
// since INTERACTION_TYPE_DESC_RECEIVE drives a TCP/IP receive, w/o
// sending anything to Connect
} else if (interTypeDesc.equals(INTERACTION_TYPE_DESC_SENDONLYACK)) {
if (this.myTmInteractionImpl.updateIrmInteractionType) {
this.setIrmF4(InputMessageProperties.IRM_F4_SENDOACK);
this.myTmInteractionImpl.updateIrmInteractionType = false;
}
} else if (interTypeDesc.equals(INTERACTION_TYPE_DESC_NAK)) {
if (this.myTmInteractionImpl.updateIrmInteractionType) {
this.setIrmF4(InputMessageProperties.IRM_F4_NACK);
this.myTmInteractionImpl.updateIrmInteractionType = false;
}
// ------------------Added for Sync
// callout------------------------------////
if (myTmInteractionImpl.getCalloutRequestNakProcessing().equals(
NAK_DISCARD_SYNC_CALLOUT_REQUEST_CONTINUE_RESUMETPIPE)) {
this.setIrmF3((byte) 0x08);
} else if (myTmInteractionImpl.getCalloutRequestNakProcessing()
.equals(NAK_REQUEUE_SYNC_CALLOUT_REQUEST_END_RESUMETPIPE)) {
this.setIrmF0((byte) 0x20);
}
if (myTmInteractionImpl.getCalloutRequestNakProcessing().equals(
NAK_DISCARD_SYNC_CALLOUT_REQUEST_END_RESUMETPIPE)
|| myTmInteractionImpl
.getCalloutRequestNakProcessing()
.equals(
NAK_DISCARD_SYNC_CALLOUT_REQUEST_CONTINUE_RESUMETPIPE)) {
if (myTmInteractionImpl.getNakReasonCode() != 0x00)
this.setIrmF0((byte) 0x10);
}
// --------------End-------------------------------------
} else if (interTypeDesc.equals(INTERACTION_TYPE_DESC_RESUMETPIPE)) {
if (this.myTmInteractionImpl.updateIrmInteractionType) {
this.setIrmF4(InputMessageProperties.IRM_F4_RESUMET);
this.myTmInteractionImpl.updateIrmInteractionType = false;
}
// Changed by shali by Dave's suggestion
this.setIrmF5(this.myTmInteractionImpl.getResumeTpipeProcessing());
// ------------------Added for Sync
// callout------------------------------////
this.setIrmF0(this.myTmInteractionImpl
.getResumeTpipeRetrievalType());
// --------------End------------------------------------------------
} else if (interTypeDesc.equals(INTERACTION_TYPE_DESC_SENDONLY)) {
if (this.myTmInteractionImpl.updateIrmInteractionType) {
this.setIrmF4(InputMessageProperties.IRM_F4_SENDONLY);
this.myTmInteractionImpl.updateIrmInteractionType = false;
}
} else if (interTypeDesc
.equals(INTERACTION_TYPE_DESC_SENDONLYXCFORDEREDDELIVERY)) {
if (this.myTmInteractionImpl.updateIrmInteractionType) {
this.setIrmF4(InputMessageProperties.IRM_F4_SENDONLY);
this.myTmInteractionImpl.updateIrmInteractionType = false;
}
this.setIrmF3((byte) 0x10);
} else if (interTypeDesc.equals(INTERACTION_TYPE_DESC_SENDRECV)
|| interTypeDesc.equals(INTERACTION_TYPE_DESC_TYPE2_COMMAND)) {
if (this.myTmInteractionImpl.updateIrmInteractionType) {
this.setIrmF4(InputMessageProperties.IRM_F4_SENDRECV);
this.myTmInteractionImpl.updateIrmInteractionType = false;
}
}
// ------------------Added for Sync
// callout------------------------------////
else if (interTypeDesc
.equals(INTERACTION_TYPE_DESC_SENDONLY_CALLOUT_RESPONSE)) {
this.irmF0 = (byte) 0x00;
this.setIrmArch((byte) 0x03);
if (this.myTmInteractionImpl.updateIrmInteractionType) {
this.setIrmF4(InputMessageProperties.IRM_F4_SENDONLY_CALLOUT);
this.myTmInteractionImpl.updateIrmInteractionType = false;
}
if (myTmInteractionImpl.getCalloutResponseMessageType().equals(
CALLOUT_ERROR_MESSAGE)) {
if(myTmInteractionImpl.getNakReasonCode()!=IRM_NAK_REASONCODE_DEFAULT)
this.setIrmF0((byte) 0x10);
else
this.setIrmF0((byte) 0x20);
}
}
//Added to support SendOnlyAck response for Sync callout.
else if (interTypeDesc
.equals(INTERACTION_TYPE_DESC_SENDONLYACK_CALLOUT_RESPONSE)) {
this.irmF0 = (byte) 0x00;
this.setIrmArch((byte) 0x03);
if (this.myTmInteractionImpl.updateIrmInteractionType) {
this.setIrmF4(InputMessageProperties.IRM_F4_SENDONLYACK_CALLOUT);
this.myTmInteractionImpl.updateIrmInteractionType = false;
}
if (myTmInteractionImpl.getCalloutResponseMessageType().equals(
CALLOUT_ERROR_MESSAGE)) {
if(myTmInteractionImpl.getNakReasonCode()!=IRM_NAK_REASONCODE_DEFAULT)
this.setIrmF0((byte) 0x10);
else
this.setIrmF0((byte) 0x20);
}
}
// ---------------------End---------------------------------------
else {
String validInteractionTypeDescription = "";
try {
validInteractionTypeDescription = ImsConnectErrorMessage
.getString(ImsConnectErrorMessage.VALID_PROPERTY_VALUE_INTERACTIONTYPEDESCRIPTION);
} catch (Exception e) {
}
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0030E, new Object[] {
"interactionTypeDescription", interTypeDesc,
validInteractionTypeDescription });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0030E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in InputMessage.setTMInteractionProperties(): ["
+ e.getMessage() + "]+");
throw e;
}
if (this.myTmInteractionImpl.updateIrmTrancode) {
if (interTypeDesc.equals(INTERACTION_TYPE_DESC_RESUMETPIPE)
|| interTypeDesc
.equals(INTERACTION_TYPE_DESC_SENDONLY_CALLOUT_RESPONSE)
||interTypeDesc
.equals(INTERACTION_TYPE_DESC_SENDONLYACK_CALLOUT_RESPONSE)
|| interTypeDesc
.equals(INTERACTION_TYPE_DESC_TYPE2_COMMAND)) {
this.setIrmTrancode(ApiProperties.EIGHT_BLANKS);
} else {
this.setIrmTrancode(this.myTmInteractionImpl.getTrancode());
}
this.myTmInteractionImpl.updateIrmTrancode = false;
}
if (this.myTmInteractionImpl.updateIrmDestId) {
this.setIrmDestId(this.myTmInteractionImpl.getImsDatastoreName());
this.myTmInteractionImpl.updateIrmDestId = false;
}
if (this.myTmInteractionImpl.updateIrmLTerm) {
this.setIrmLTerm(this.myTmInteractionImpl.getLtermOverrideName());
this.myTmInteractionImpl.updateIrmLTerm = false;
}
if (this.myTmInteractionImpl.updateIrmRacfUserId) {
this.setIrmRacfUserId(this.myTmInteractionImpl.getRacfUserId());
this.myTmInteractionImpl.updateIrmRacfUserId = false;
}
if (this.myTmInteractionImpl.updateIrmRacfGroupName) {
this.setIrmRacfGroupName(this.myTmInteractionImpl
.getRacfGroupName());
this.myTmInteractionImpl.updateIrmRacfGroupName = false;
}
if (this.myTmInteractionImpl.updateIrmRacfPassword) {
this.setIrmRacfPassword(this.myTmInteractionImpl.getRacfPassword());
this.myTmInteractionImpl.updateIrmRacfPassword = false;
}
if (this.myTmInteractionImpl.updateIrmRacfApplName) {
this.setIrmRacfApplName(this.myTmInteractionImpl.getRacfApplName());
this.myTmInteractionImpl.updateIrmRacfApplName = false;
}
if (this.myTmInteractionImpl.updateIrmRerouteName) {
if (!interTypeDesc.equals(INTERACTION_TYPE_DESC_RESUMETPIPE)) {
this.setIrmRerouteName(this.myTmInteractionImpl
.getRerouteName());
// this.setIrmRerouteName(new
// String(this.myTmInteractionImpl.getRerouteName()));
} else {
this.setIrmRerouteName(this.myTmInteractionImpl
.getResumeTpipeAlternateClientId());
// this.setIrmRerouteName(new
// String(this.myTmInteractionImpl.getResumeTpipeAlternateClientId()));
}
this.myTmInteractionImpl.updateIrmRerouteName = false;
}
// setIrmTagAdapter(this.myTmInteractionImpl.getXmlAdapterName());
if (this.myTmInteractionImpl.updateIrmTagAdapter) {
this.setIrmTagAdapter(InputMessageProperties.IRM_TAG_ADAPT_DEFAULT);
this.myTmInteractionImpl.updateIrmTagAdapter = false;
}
// setIrmTagMap(this.myTmInteractionImpl.getXmlConverterName());
if (this.myTmInteractionImpl.updateIrmTagMap) {
this.setIrmTagMap(InputMessageProperties.IRM_TAG_MAP_DEFAULT);
this.myTmInteractionImpl.updateIrmTagMap = false;
}
// ------------------Added for Sync callout------------------------------////
if (this.myTmInteractionImpl.updateIrmInputModName) {
this.setIrmInputModName(this.myTmInteractionImpl.getInputModName());
this.myTmInteractionImpl.updateIrmInputModName = false;
}
this
.setIrmCorelatorToken(this.myTmInteractionImpl
.getCorrelatorToken());
// ---------------------End------------------------------------
this
.setInputMessageDataSegmentsIncludeLlzzAndTrancode(this.myTmInteractionImpl
.isInputMessageDataSegmentsIncludeLlzzAndTrancode());
if (interTypeDesc.equals(INTERACTION_TYPE_DESC_TYPE2_COMMAND)) {
this.setTrancodeInData(ApiProperties.EIGHT_BLANKS);
} else {
if (this.myTmInteractionImpl.updateIrmTrancodeInData) {
this.setTrancodeInData(this.myTmInteractionImpl.getTrancode());
this.myTmInteractionImpl.updateIrmTrancodeInData = false;
}
}
this.setInConversation(this.myTmInteractionImpl.testInConversation());
this.setTmInteractionPropertiesAlreadySet(true);
this.rebuildData |= this.myTmInteractionImpl.isRebuildMessage();
try {
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL)) {
if (this.getImsConnectCodepage() != null)
logger
.finer(" InputMessage.imsConnectCodepage set to ["
+ this.getImsConnectCodepage() + "]");
if (this.getIrmId() != null)
logger.finer(" InputMessage.irmId set to ["
+ new String(this.getIrmId(), this
.getImsConnectCodepage()) + "]");
logger
.finer(" InputMessage.TmInteractionImpl.responseIncludesLlll internally set to ["
+ this.myTmInteractionImpl
.isResponseIncludesLlll() + "]");
logger.finer(" InputMessage.irmNakReasonCode set to ["
+ this.getIrmNakReasonCode() + "]");
logger.finer(" InputMessage.irmResWord set to ["
+ this.getIrmResWord() + "]");
logger.finer(" InputMessage.irmTimer set to ["
+ this.getIrmTimer() + "]");
logger.finer(" InputMessage.irmEncodingSchema set to ["
+ this.getIrmEncodingSchema() + "]");
logger.finer(" InputMessage.irmF0 set to ["
+ this.getIrmF0() + "]");
logger.finer(" InputMessage.irmF1 set to ["
+ this.getIrmF1() + "]");
logger.finer(" InputMessage.irmF2 set to ["
+ this.getIrmF2() + "]");
logger.finer(" InputMessage.irmF3 set to ["
+ this.getIrmF3() + "]");
byte[] tmpByteArray = new byte[1];
String outStr = null;
tmpByteArray[0] = (byte) this.getIrmF4();
if (tmpByteArray != null)
outStr = new String(tmpByteArray, this.imsConnectCodepage);
logger.finer(" InputMessage.irmF4 set to [" + outStr + "]");
// logger.finer(" InputMessage.irmF4 set to [" +
// this.getIrmF4()
// + "]");
logger.finer(" InputMessage.irmF5 set to ["
+ this.getIrmF5() + "]");
if (this.getIrmTrancode() != null)
logger.finer(" InputMessage.irmTrancode set to ["
+ new String(this.getIrmTrancode(), this
.getImsConnectCodepage()) + "]");
if (this.getIrmDestId() != null)
logger.finer(" InputMessage.irmDestId set to ["
+ new String(this.getIrmDestId(), this
.getImsConnectCodepage()) + "]");
if (this.getIrmLTerm() != null)
logger.finer(" InputMessage.irmLTerm set to ["
+ new String(this.getIrmLTerm(), this
.getImsConnectCodepage()) + "]");
if (this.getIrmRacfUserId() != null)
logger.finer(" InputMessage.irmRacfUserId set to ["
+ new String(this.getIrmRacfUserId(), this
.getImsConnectCodepage()) + "]");
if (this.getIrmRacfGroupName() != null)
logger.finer(" InputMessage.irmRacfGroupName set to ["
+ new String(this.getIrmRacfGroupName(), this
.getImsConnectCodepage()) + "]");
if (this.getIrmRacfPassword() != null)
logger.finer(" InputMessage.irmRacfPassword set to ["
+ new String("********") + "]");
if (this.getIrmRacfApplName() != null)
logger.finer(" InputMessage.irmRacfApplName set to ["
+ new String(this.getIrmRacfApplName(), this
.getImsConnectCodepage()) + "]");
if (this.getIrmRerouteName() != null)
logger.finer(" InputMessage.irmRerouteName set to ["
+ new String(this.getIrmRerouteName(), this
.getImsConnectCodepage()) + "]");
if (this.getIrmTagAdapter() != null)
logger.finer(" InputMessage.irmTagAdapter set to ["
+ new String(this.getIrmTagAdapter(), this
.getImsConnectCodepage()) + "]");
if (this.getIrmTagMap() != null)
logger.finer(" InputMessage.irmTagMap set to ["
+ new String(this.getIrmTagMap(), this
.getImsConnectCodepage()) + "]");
if (this.getIrmInputModName() != null)
logger.finer(" InputMessage.irmInputModName set to ["
+ new String(this.getIrmInputModName(), this
.getImsConnectCodepage()) + "]");
if (this.getIrmCorelatorToken() != null)
logger.finer(" InputMessage.irmCorelatorToken set to ["
+ bytesToHexString(this.getIrmCorelatorToken()) + "] in Hex");
/*logger.finer(" InputMessage.irmCorelatorToken set to ["
+ new String(this.getIrmCorelatorToken(), this
.getImsConnectCodepage()) + "]");
*/
logger
.finer(" InputMessage.inputMessageDataSegmentsIncludeLlzzAndTrancode set to ["
+ this
.isInputMessageDataSegmentsIncludeLlzzAndTrancode()
+ "]");
if (this.getTrancodeInData() != null)
logger.finer(" InputMessage.trancodeInData set to ["
+ new String(this.getTrancodeInData(), this
.getImsConnectCodepage()) + "]");
logger.finer(" InputMessage.inConversation set to ["
+ this.myTmInteractionImpl.testInConversation() + "]");
logger.finer(" InputMessage.rebuildData set to ["
+ this.rebuildData + "]");
}
} catch (UnsupportedEncodingException e) {
}
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("<-- InputMessage.setTMInteractionProperties()");
}
public void setType2CommandInteractionProperties()
throws ImsConnectApiException {
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger
.finer("--> InputMessage.setType2CommandInteractionProperties()");
this.setIrmTrancode(ApiProperties.EIGHT_BLANKS);
this.setIrmRacfApplName(ApiProperties.EIGHT_BLANKS);
setTrancodeInData("");
this.setInConversation(false);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL)) {
logger.finer(" InputMessage.irmTrancode set to ["
+ ApiProperties.EIGHT_BLANKS + "]");
logger.finer(" InputMessage.irmRacfApplName set to ["
+ ApiProperties.EIGHT_BLANKS + "]");
logger.finer(" InputMessage.trancodeInData set to []");
logger.finer(" InputMessage.inConversation set to [false]");
}
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger
.finer("<-- InputMessage.setType2CommandInteractionProperties()");
}
/**
* ok the input message message data byte array including LLZZ for each
* segment and trancode for 1st segment if applicable
*
* @return Return the input message data in a byte array
* @throws ImsConnectApiException
* if error occurred building the data bytearray
*/
public void buildDataByteArray() // throws ImsConnectApiException
{
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("--> InputMessage.buildDataByteArray()");
if (this.rebuildData) {
if ((this.myTmInteractionImpl.getInteractionTypeDescription()
.equals(ApiProperties.INTERACTION_TYPE_DESC_SENDRECV))
|| (this.myTmInteractionImpl
.getInteractionTypeDescription()
.equals(ApiProperties.INTERACTION_TYPE_DESC_SENDONLY))
|| (this.myTmInteractionImpl
.getInteractionTypeDescription()
.equals(ApiProperties.INTERACTION_TYPE_DESC_SENDONLY_CALLOUT_RESPONSE))
|| (this.myTmInteractionImpl
.getInteractionTypeDescription()
.equals(ApiProperties.INTERACTION_TYPE_DESC_SENDONLYACK_CALLOUT_RESPONSE))
|| (this.myTmInteractionImpl
.getInteractionTypeDescription()
.equals(ApiProperties.INTERACTION_TYPE_DESC_SENDONLYACK))
|| (this.myTmInteractionImpl
.getInteractionTypeDescription()
.equals(ApiProperties.INTERACTION_TYPE_DESC_TYPE2_COMMAND))
|| (this.myTmInteractionImpl
.getInteractionTypeDescription()
.equals(ApiProperties.INTERACTION_TYPE_DESC_SENDONLYXCFORDEREDDELIVERY))) {
i = 0;
bytesCopied = 0;
segmentLengths = new int[numberOfSegments];
messageLength = 0;
// if inputMessageDataSegmentsIncludeLlzzAndTrancode == true,
// copy segments in inputData Vector to this.data (1D bytearray
// return value)
// else add LLZZ to each segment in inputData Vector before
// copying to this.data
if (this.inputMessageDataSegmentsIncludeLlzzAndTrancode == true) {
while (i < numberOfSegments) {
segmentLengths[i] = (inputData.get(i)).length;
messageLength += segmentLengths[i++];
}
this.data = new byte[messageLength];
i = 0;
while (i < numberOfSegments) {
System.arraycopy(inputData.get(i), 0, this.data,
bytesCopied, segmentLengths[i]);
bytesCopied += segmentLengths[i++];
}
} else // inputMessageDataSegmentsIncludeLlzzAndTrancode ==
// false, so add LLZZ and trancode to this.data (1D
// bytearray return value)
{
this.trancodeInDataLength = (myTmInteractionImpl
.testInConversation() ? 0 : trancodeInData.length);
while (i < numberOfSegments) {
segmentLengths[i] = 4 + (inputData.get(i)).length;
if ((i == 0)
&& (!myTmInteractionImpl.isInConversation())) {
segmentLengths[i] += trancodeInDataLength;
}
messageLength += segmentLengths[i++];
}
this.data = new byte[messageLength];
i = 0;
while (i < numberOfSegments) {
byte[] llzzBytes = this
.createLlzzByteArrayFromLlValue(segmentLengths[i]);
System.arraycopy(llzzBytes, 0, this.data, bytesCopied,
4);
bytesCopied += 4;
if (i == 0) {
System.arraycopy(trancodeInData, 0, this.data,
bytesCopied, this.trancodeInDataLength);
bytesCopied += this.trancodeInDataLength;
System.arraycopy(inputData.get(i), 0, this.data,
bytesCopied, segmentLengths[i] - 4
- this.trancodeInDataLength);
bytesCopied += segmentLengths[i++] - 4
- this.trancodeInDataLength;
} else {
System.arraycopy(inputData.get(i), 0, this.data,
bytesCopied, segmentLengths[i] - 4);
bytesCopied += segmentLengths[i++] - 4;
}
}
}
this.rebuildData = false;
} else {
this.data = null;
this.rebuildData = true;
}
}
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("<-- InputMessage.buildDataByteArray()");
}
/**
* Build the input message. The input message is in the form of LLLLIRMData
*
* @return Return the input messages in a byte array
* @throws ImsConnectApiException
* if error occurred building the input message
*/
public byte[] buildInputMessageByteArray(boolean aSuppressLogging)
throws ImsConnectApiException {
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger
.finer("--> InputMessage.buildInputMessageByteArray(boolean)");
if ((myTmInteractionImpl.testInConversation())
&& (myTmInteractionImpl.getCommitMode() == ApiProperties.COMMIT_MODE_0)) {
myTmInteractionImpl.setCommitMode(ApiProperties.COMMIT_MODE_1);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL)) {
logger
.finest(" TmInteractionImpl.execute() - Commit mode changed internally from CM0 to CM1 for conversational interaction");
logger
.finest(" Conversational interactions are only supported with Commit Mode 1");
}
} else if (((myTmInteractionImpl.getInteractionTypeDescription() == INTERACTION_TYPE_DESC_RESUMETPIPE)
|| (myTmInteractionImpl.getInteractionTypeDescription() == INTERACTION_TYPE_DESC_SENDONLY)
|| (myTmInteractionImpl.getInteractionTypeDescription() == INTERACTION_TYPE_DESC_SENDONLY_CALLOUT_RESPONSE)
|| (myTmInteractionImpl.getInteractionTypeDescription() == INTERACTION_TYPE_DESC_SENDONLYACK_CALLOUT_RESPONSE)
|| (myTmInteractionImpl.getInteractionTypeDescription() == INTERACTION_TYPE_DESC_SENDONLYACK)
|| (myTmInteractionImpl.getInteractionTypeDescription() == INTERACTION_TYPE_DESC_SENDONLYXCFORDEREDDELIVERY))
&& (myTmInteractionImpl.getCommitMode() == ApiProperties.COMMIT_MODE_1)) {
myTmInteractionImpl.setCommitMode(ApiProperties.COMMIT_MODE_0);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL)) {
logger
.finest(" TmInteractionImpl.execute() - Commit mode changed internally from CM1 to CM0 for interaction type "
+ myTmInteractionImpl
.getInteractionTypeDescription());
logger
.finest(" Interaction type "
+ myTmInteractionImpl
.getInteractionTypeDescription()
+ " is only supported with Commit Mode 0");
}
}
if ((myTmInteractionImpl.getCommitMode() == ApiProperties.COMMIT_MODE_0)
&& (myTmInteractionImpl.getSyncLevel() == ApiProperties.SYNC_LEVEL_NONE)) {
myTmInteractionImpl.setSyncLevel(ApiProperties.SYNC_LEVEL_CONFIRM);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL)) {
logger
.finest(" TmInteractionImpl.execute() - Sync Level changed internally from SYNC_LEVEL_NONE to SYNC_LEVEL_CONFIRM");
logger
.finest(" Commit Mode 0 is only supported with Sync Level CONFIRM");
}
}
if ((myTmInteractionImpl.rebuildMessage) || (this.rebuildData)) {
this.setConnectionProperties((ConnectionImpl) this.myTmInteractionImpl.getMyConnection());
this.setTmInteractionProperties();
if (this.getMyTmInteractionImpl().getInteractionTypeDescription()
.equals(ApiProperties.INTERACTION_TYPE_DESC_TYPE2_COMMAND))
this.setType2CommandInteractionProperties();
boolean addDataToMessageBuilt = true;
this.buildDataByteArray();
// Set flag to not include data in message to be returned if there
// is no data
if (this.data == null)
addDataToMessageBuilt = false;
// Determine the IRM and message length based on architecture level
/*
* switch (this.IRM_ARCH) { case IRM_ARCH0: irmLen =
* InputMessageProperties.IRM_LEN_ARCH0; break; case IRM_ARCH1:
* irmLen = InputMessageProperties.IRM_LEN_ARCH1; break; case
* IRM_ARCH2: irmLen = InputMessageProperties.IRM_LEN_ARCH2; break;
* default: irmLen = InputMessageProperties.IRM_LEN_ARCH0; break; }
*/
// Changed for Sync callout support
// irmLen = InputMessageProperties.IRM_LEN_ARCH2; // hard-code
// architecture level 2 for now
irmLen = InputMessageProperties.IRM_LEN_ARCH3; // hard-code
// architecture
// level 3 for now
// length of llll + IRM length + length of data if applicable +
// length of end-of-message segment
this.setLlll(4 + irmLen
+ (addDataToMessageBuilt ? this.data.length : 0)
+ InputMessageProperties.MESSAGE_END.length); // length of
// LLLL +
// length of
// MESSAGE_END
// Build the message
this.message = new byte[(int) this.llll];
// ByteArrayOutputStream bout = new ByteArrayOutputStream();
// DataOutputStream dout = new DataOutputStream(bout);
ByteBuffer buf = ByteBuffer.wrap(this.message);
if ((myTmInteractionImpl.testInConversation() == true)
|| (myTmInteractionImpl.getInteractionTypeDescription()
.equals(ApiProperties.INTERACTION_TYPE_DESC_RESUMETPIPE))
|| (myTmInteractionImpl.getInteractionTypeDescription()
.equals(ApiProperties.INTERACTION_TYPE_DESC_ACK))
|| (myTmInteractionImpl.getInteractionTypeDescription()
.equals(ApiProperties.INTERACTION_TYPE_DESC_TYPE2_COMMAND))
|| (myTmInteractionImpl.getInteractionTypeDescription()
.equals(ApiProperties.INTERACTION_TYPE_DESC_NAK))
|| // NAK does not require trancode either
(myTmInteractionImpl.getInteractionTypeDescription()
.equals(ApiProperties.INTERACTION_TYPE_DESC_CANCELTIMER))
|| (myTmInteractionImpl.getInteractionTypeDescription()
.equals(ApiProperties.INTERACTION_TYPE_DESC_ENDCONVERSATION))) {
trancodeInDataToBeUsed = anEmptyTrancodeByteArray;
} else {
trancodeInDataToBeUsed = this.trancodeInData;
}
try {
// 4 bytes LLLL denotes the length of the input message
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL)
&& !aSuppressLogging)
logger
.finest(" InputMessage.buildInputMessageByteArray(boolean) - writing LLLL ["
+ this.getLlll()
+ "] to \"dout\" DataOutStream");
buf.putInt(this.llll);
// IRM Header
buf.putShort((short) irmLen);
buf.put(this.irmArch);
buf.put(this.irmF0);
buf.put(this.irmId);
buf.putShort(this.irmNakReasonCode);
buf.putShort(this.irmResWord);
buf.put((byte) this.irmF5);
buf.put((byte) this.irmTimer);
buf.put(this.irmSocType);
buf.put(this.irmEncodingSchema);
buf.put(this.irmClientId);
buf.put(this.irmF1);
buf.put(this.irmF2);
buf.put(this.irmF3);
buf.put((byte) this.irmF4);
buf.put(irmTrancode);
buf.put(this.irmDestId);
buf.put(this.irmLTerm);
buf.put(this.irmRacfUserId);
buf.put(this.irmRacfGroupName);
buf.put(this.irmRacfPassword);
buf.put(this.irmRacfApplName);
if (this.irmArch >= InputMessageProperties.IRM_ARCH1)
buf.put(this.irmRerouteName);
if (this.irmArch >= InputMessageProperties.IRM_ARCH2) {
buf.put(this.irmTagAdapter);
buf.put(this.irmTagMap);
}
if (this.irmArch >= InputMessageProperties.IRM_ARCH3) {
buf.put(this.irmInputModname);
buf.put(this.irmCorelatorToken);
}
} catch (Exception e1) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0001E,
new Object[] { ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.MSG_BLD_ERR,
new Object[] { ImsConnectErrorMessage
.getExceptionMessage(e1) }) });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0001E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.buildInputMessageByteArray(boolean): ["
+ e.getMessage() + "]+");
throw e;
}
try {
// Input Data
if (addDataToMessageBuilt == true || this.data != null)
buf.put(this.data);
// 4 bytes End of message indicator
buf.put(InputMessageProperties.MESSAGE_END, 0, 4);
// this.message = buf.array();
this.myTmInteractionImpl.rebuildMessage = false;
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL)
&& !aSuppressLogging) {
boolean obfuscatePassword = true;
String bufferToSend = myTmInteractionImpl
.formatBufferForTracing(message, obfuscatePassword);
String[] bufferToSendStringArray = myTmInteractionImpl
.stringToStringArray(bufferToSend);
String[] interpretedIrm = myTmInteractionImpl
.interpretIrm(message);
logger.finest(" InputMessage.buildInputMessageByteArray(boolean) - Buffer to be sent:");
int sendBufStringArrayLen = bufferToSendStringArray.length;
for (int i = 0; i < sendBufStringArrayLen; i++) {
logger.finest(bufferToSendStringArray[i]);
}
for (int i = 0; i < interpretedIrm.length; i++) {
logger.finest(interpretedIrm[i]);
}
}
} catch (UnsupportedEncodingException usee) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0017E, new Object[] {
"input request message",
this.imsConnectCodepage });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.buildInputMessageByteArray(boolean): ["
+ e.getMessage() + "]+");
throw e;
} catch (Exception e1) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0001E,
new Object[] { ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.MSG_BLD_ERR,
new Object[] { ImsConnectErrorMessage
.getExceptionMessage(e1) }) });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0001E, errMsg);
if (logger.getLevel().intValue() <= ApiProperties.TRACE_LEVEL_EXCEPTION
.intValue())
logger
.severe(" Exception caught in InputMessage.buildInputMessageByteArray(boolean): ["
+ e.getMessage() + "]+");
throw e;
}
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger
.finer("<-- InputMessage.buildInputMessageByteArray(boolean)");
}
return message;
}
/**
* Pad the string with the desired character to the specified length
* converting the codepage of each character in the new padded string
*
* @param str
* Input string to be written to outputstream
* @param c
* Character to pad string with
* @param len
* Full length (padded) of data to be written to outputstream
* @param codepage
* Codepage to use when converting string to bytes to be written
* to output stream
*
* @throws UnsupportedEncodingException
*/
public String padAndConvertString(String str, char c, int len,
String codepage) throws ImsConnectApiException {
aCodepage = codepage;
try {
StringBuffer buf = new StringBuffer(str);
if (str.length() < len) {
for (int i = str.length(); i < len; i++)
buf.append(c);
}
return new String(buf.toString().getBytes(codepage));
} catch (UnsupportedEncodingException usee) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0017E, new Object[] {
"input request message", this.aCodepage });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.padAndConvertString(String, char, int, String): ["
+ e.getMessage() + "]+");
throw e;
}
}
/**
* Pad the string with the desired character to the specified length without
* converting the codepage of the characters of the new string
*
* @param str
* Input string to be written to outputstream
* @param c
* Character to pad string with
* @param len
* Full length (padded) of data to be written to outputstream
*/
public String padString(String str, char c, int len) {
int strLen = 0;
StringBuilder buf;
if (str != null)
strLen = str.length();
if (strLen < len) {
if (str != null) {
buf = new StringBuilder(len);
buf.append(str);
} else
buf = new StringBuilder();
for (int i = strLen; i < len; i++)
buf.append(c);
return buf.toString();
} else
return str;
}
/**
* @return the numberOfSegments
*/
public int getNumberOfSegments() {
return this.numberOfSegments;
}
/*
* Returns the data in this InputMessage object as a byte array. The
* returned byte array contains the input data without LLLL or LLZZ and
* trancode bytes.
*/
public byte[] getDataAsByteArray() {
int i = 0;
int dataLen = 0;
int copyPos = this.isInputMessageDataSegmentsIncludeLlzzAndTrancode() ? 4 + (((TmInteractionImpl) this.myTmInteractionImpl)
.testInConversation() ? 0 : this.myTmInteractionImpl
.getTrancode().length())
: 0; // skip LLZZ and trancode if present
// int copyPos = 4 +
// (((TmInteractionImpl)this.myTmInteractionImpl).testInConversation() ?
// 0 : this.myTmInteractionImpl.getTrancode().length()); // skip LLZZ
// and trancode if present
int savedCopyPos = copyPos;
int bytesCopied = 0;
int bytesToCopy = 0;
int numberOfSegments = inputData.size();
short[] segmentLengths = new short[numberOfSegments]; // lengths of
// segments of
// inData
// including
// LLZZ and
// trancode if
// present
while (i < numberOfSegments) // calculate total length of inputData
// inputDataLen
{
segmentLengths[i] = (short) ((byte[]) inputData.get(i)).length;
// segmentLengths[i] -= copyPos;
// copyPos = this.isInputMessageDataSegmentsIncludeLlzzAndTrancode()
// ? 4 : 0;
dataLen += segmentLengths[i++] - copyPos;
copyPos = 4;
}
// allocate new byte array, inputDataByteArray, of length inputDataLen
byte[] inputDataByteArray = new byte[dataLen];
i = 0;
copyPos = savedCopyPos;
while (i < numberOfSegments)// arrayCopy loop from inputData to
// inputDataByteArray
{
bytesToCopy = segmentLengths[i] - copyPos;
System.arraycopy(inputData.get(i++), copyPos, inputDataByteArray,
bytesCopied, bytesToCopy);
bytesCopied += bytesToCopy;
// bytesCopied += segmentLengths[i++];
copyPos = 4;
}
return inputDataByteArray;
}
/**
* @param string
*/
public byte[][] getDataAsArrayOfByteArrays() {
int i = 0;
int dataLen = 0;
int copyPos = this.isInputMessageDataSegmentsIncludeLlzzAndTrancode() ? 4 + (((TmInteractionImpl) this.myTmInteractionImpl)
.testInConversation() ? 0 : this.myTmInteractionImpl
.getTrancode().length())
: 0; // skip LLZZ and trancode if present
// int copyPos = 4 +
// (((TmInteractionImpl)this.myTmInteractionImpl).testInConversation() ?
// 0 : this.myTmInteractionImpl.getTrancode().length()); // skip LLZZ
// and trancode if present
int numberOfSegments = inputData.size();
byte[][] returnArrayOfByteArrays = new byte[numberOfSegments][];
// copyPos = this.isInputMessageDataSegmentsIncludeLlzzAndTrancode() ? 4
// + (this.isInConversation() ? 0 :
// this.myTmInteractionImpl.getTrancode().length()) : 0;
while (i < numberOfSegments) // copy segments to returnStringArray
{
dataLen = ((byte[]) (inputData.get(i))).length;
returnArrayOfByteArrays[i] = new byte[dataLen - copyPos];
System.arraycopy((byte[]) inputData.get(i), copyPos,
returnArrayOfByteArrays[i], 0, dataLen - copyPos);
// copyPos = this.isInputMessageDataSegmentsIncludeLlzzAndTrancode()
// ? 4 : 0;
copyPos = 4;
i++;
}
return returnArrayOfByteArrays;
}
/**
* @param string
*/
public String getDataAsString() throws ImsConnectApiException {
try {
return new String(this.getDataAsByteArray(),
this.imsConnectCodepage);
// return new String(this.getDataAsByteArray());
} catch (UnsupportedEncodingException usee) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0017E, new Object[] {
"input request message", this.imsConnectCodepage });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.getDataAsString(): ["
+ e.getMessage() + "]+");
throw e;
}
}
public String[] getDataAsArrayOfStrings() throws ImsConnectApiException {
int i = 0;
int numberOfSegments = inputData.size();
String[] returnStringArray = new String[numberOfSegments];
byte[][] tmpArrayOfByteArrays = this.getDataAsArrayOfByteArrays();
try {
while (i < numberOfSegments) // copy segments to returnStringArray
{
returnStringArray[i] = new String(tmpArrayOfByteArrays[i],
this.imsConnectCodepage);
// returnStringArray[i] = new String(tmpArrayOfByteArrays[i]);
i++;
}
} catch (UnsupportedEncodingException usee) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0017E, new Object[] {
"input request message", this.imsConnectCodepage });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.getDataAsArrayOfStrings(): ["
+ e.getMessage() + "]+");
throw e;
}
return returnStringArray;
}
// Methods which retrieve data at the segment level
/**
* Gets the segment, at the segment location specified by aSegmentNumber, as
* a byte array without the LLLL, but including the LLZZ along with the
* trancode field if applicable.
*
* @param aSegmentNumber
* 0-based segment index
* @return a byte array containing the segment data without the LLLL, but
* with the LLZZ field and trancode field if applicable.
*/
public byte[] getSegmentAsByteArray(int aSegmentNumber) {
return (byte[]) inputData.get(aSegmentNumber);
}
/**
* @param string
*/
public String getSegmentAsString(int aSegmentNumber) // throws
// ImsConnectApiException
{
// try
{
// return new String((byte[])inputData.elementAt(aSegmentNumber),
// imsConnectCodepage);
return new String((byte[]) inputData.get(aSegmentNumber));
}
/*
* catch (UnsupportedEncodingException usee) { String errMsg =
* ImsConnectErrorMessage.getString( ImsConnectErrorMessage.HWS0017E,
* new Object[] {"input request message segment", this.dataCodepage});
* ImsConnectApiException e = new ImsConnectApiException(
* ImsConnectErrorMessage.HWS0017E, errMsg);
*
* if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
* logger.severe
* (" Exception caught in InputMessage.getSegmentAsString(): [" +
* e.toString() + "]+");
*
* throw e; }
*/}
// Methods which add a single data segment to inputData
/**
* @param byte[] aSegmentData
*/
public void appendSegment(byte[] aSegmentData) {
// append byte[] aSegmentData to end of inputData
if (this.isInputMessageDataSegmentsIncludeLlzzAndTrancode()) {
bytesProcessed = 0;
int dataLen = aSegmentData.length;
int ll;
int numberOfSegments = 0;
byte[] inputSegment;
while (bytesProcessed < dataLen) {
ll = getLlValueFromLlzzBytes(aSegmentData, bytesProcessed);
inputSegment = new byte[ll];
System.arraycopy(aSegmentData, bytesProcessed, inputSegment, 0,
ll);
inputData.add(inputSegment);
numberOfSegments++;
bytesProcessed += (ll);
}
} else
inputData.add(aSegmentData);
this.setNumberOfSegments(this.inputData.size());
this.rebuildData = true;
}
/**
* @param string
*/
public void appendSegment(String aSegmentData)
throws ImsConnectApiException {
byte[] inputSegment;
imsConnectCodepage = myTmInteractionImpl.getImsConnectCodepage();
if (this.myTmInteractionImpl
.isInputMessageDataSegmentsIncludeLlzzAndTrancode()) {
String errMsg = ImsConnectErrorMessage
.getString(ImsConnectErrorMessage.HWS0035E);
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0035E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.appendSegment(String). Exception caught was: "
+ e.getMessage());
throw e;
} else {
// append String aSegmentData to end of inputData
try {
inputSegment = aSegmentData.getBytes(imsConnectCodepage);
} catch (UnsupportedEncodingException usee) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0017E, new Object[] {
"input request message segment",
this.imsConnectCodepage });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.appendSegment(String): ["
+ e.getMessage() + "]+");
throw e;
}
this.appendSegment(inputSegment);
}
}
/**
* @param string
*/
public void insertSegment(byte[] aSegmentData, int aLocation)
// throws UnsupportedEncodingException
{
if (this.isInputMessageDataSegmentsIncludeLlzzAndTrancode()) // insert
// aSegmentData
// without
// modification
{
bytesProcessed = 0;
int dataLen = aSegmentData.length;
short ll;
byte[] inputSegment;
while (bytesProcessed < dataLen) {
ll = (short) getLlValueFromLlzzBytes(aSegmentData,
bytesProcessed);
inputSegment = new byte[ll];
System.arraycopy(aSegmentData, bytesProcessed, inputSegment, 0,
ll);
// insert aSegmentData at location aLocation of inputData
this.inputData.add(aLocation++, aSegmentData); // insert
// segment
// to
// inputData
// Vector
// at
// aLocation
bytesProcessed += (ll);
}
} else // input data segment does not include LLZZ, so it must be a
// single segment
{
this.inputData.add(aLocation++, aSegmentData); // insert
// segment
// to
// inputData
// Vector
// at
// aLocation
}
this.setNumberOfSegments(this.inputData.size());
this.rebuildData = true;
}
/**
* @param string
*/
public void insertSegment(String aSegmentData, int aLocation)
throws ImsConnectApiException {
imsConnectCodepage = myTmInteractionImpl.getImsConnectCodepage();
if (this.myTmInteractionImpl
.isInputMessageDataSegmentsIncludeLlzzAndTrancode()) {
String errMsg = ImsConnectErrorMessage
.getString(ImsConnectErrorMessage.HWS0035E);
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0035E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.insertSegment(String, int). Exception caught was: "
+ e.getMessage());
throw e;
} else {
// append String aSegmentData to end of inputData
try {
insertSegment(aSegmentData.getBytes(imsConnectCodepage),
aLocation);
} catch (UnsupportedEncodingException usee) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0017E, new Object[] {
"input request message segment",
this.imsConnectCodepage });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.insertSegment(String, int): ["
+ usee.getMessage() + "]+");
throw e;
}
}
}
// Methods which replace the contents of a single data segment in inputData
public void changeSegment(byte[] aSegmentData, int aLocation) {
bytesProcessed = 0;
int dataLen = aSegmentData.length;
int ll;
int numberOfSegments = 0;
byte[] inputSegment;
// delete segment at aLocation and then insert aSegmentData at aLocation
// of inputData
this.inputData.remove(aLocation);
if (this.isInputMessageDataSegmentsIncludeLlzzAndTrancode()) {
while (bytesProcessed < dataLen) {
ll = getLlValueFromLlzzBytes(aSegmentData, bytesProcessed);
inputSegment = new byte[ll];
System.arraycopy(aSegmentData, bytesProcessed, inputSegment, 0,
ll);
this.inputData.add(aLocation, inputSegment);
numberOfSegments++;
bytesProcessed += (ll);
}
} else
inputData.add(aSegmentData);
this.rebuildData = true;
}
/**
* @param string
*/
public void changeSegment(String aSegmentData, int aLocation)
throws ImsConnectApiException {
imsConnectCodepage = myTmInteractionImpl.getImsConnectCodepage();
if (this.myTmInteractionImpl
.isInputMessageDataSegmentsIncludeLlzzAndTrancode()) {
String errMsg = ImsConnectErrorMessage
.getString(ImsConnectErrorMessage.HWS0035E);
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0035E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.changeSegment(String, int). Exception caught was: "
+ e.getMessage());
throw e;
} else {
// insert aSegmentData at location aLocation of inputData
try {
changeSegment(aSegmentData.getBytes(imsConnectCodepage),
aLocation);
// changeSegment(aSegmentData.getBytes(), aLocation);
} catch (UnsupportedEncodingException usee) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0017E, new Object[] {
"input request message segment",
this.imsConnectCodepage });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.changeSegment(String, int): ["
+ e.getMessage() + "]+");
throw e;
}
}
}
// Methods which set the data segments in inputData (clears out and replaces
// whatever segments are currently in inputData)
/**
* Writes data including LLLL, LLZZ and trancode in this.inputData Vector.
* Caution, this method should not be called before the properties that it
* uses, TmInteraction inputMessageDataSegmentsIncludeLlzzAndTrancode and
* trancode properties and the anInputDataByteArray input parameter have
* been set or initialized as needed.
*
* @param bytearray
* containing input data with or without LLZZ and trancode
* depending on the application
*/
public void setInputMessageData(byte[] anInputDataByteArray)
throws ImsConnectApiException {
bytesProcessed = 0;
int dataLen = 0;
int ll;
byte[] tmpInputSegment;
if (anInputDataByteArray != null) {
dataLen = anInputDataByteArray.length;
/*
* if (isTmInteractionPropertiesAlreadySet() == false) {
* this.setTmInteractionProperties();
* if(this.myTmInteractionImpl.getInteractionTypeDescription
* ().equalsIgnoreCase
* (ApiProperties.INTERACTION_TYPE_DESC_TYPE2_COMMAND)) {
* this.setType2CommandInteractionProperties(); }
* this.tmInteractionPropertiesAlreadySet = true; }
*/
this
.setInputMessageDataSegmentsIncludeLlzzAndTrancode(this.myTmInteractionImpl
.isInputMessageDataSegmentsIncludeLlzzAndTrancode());
this.inputData.clear(); // clear out Vector of bytearrays
if (!this.isInputMessageDataSegmentsIncludeLlzzAndTrancode()) // input
// data
// does
// not
// include
// LLZZ
// so
// it
// must
// be
// a
// single-segment
{ // input message
this.inputData.add(anInputDataByteArray); // add segment
// to
// inputData
// Vector
numberOfSegments = 1;
} else // can be single or multi-segment input message since
// inputMessageDataSegmentsIncludeLlzzAndTrancode is true
{
// verify LLZZ are correct based on length of bytearray element
// (LL can be less than length of bytearray but not more than)
// and trancode is correct based on trancodeInData value (which
// must be reset whenever TmInteraction.trancode changes) and
// the conversational state and/or the type of interaction
numberOfSegments = 0;
while (bytesProcessed < dataLen) // dataLen includes LLZZ and
// trancode lengths since
// inputMessageDataSegmentsIncludeLlzzAndTrancode
// is true
{
ll = getLlValueFromLlzzBytes(anInputDataByteArray,
bytesProcessed); // get LL of segment
if ((ll < 4) || (ll > 32767)) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0036E,
new Object[] { ll });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0036E, errMsg);
if (logger
.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessageImpl.setInputMessageData(byte[]). Exception caught was: "
+ e.getMessage());
throw e;
}
tmpInputSegment = new byte[ll];
System.arraycopy(anInputDataByteArray, bytesProcessed,
tmpInputSegment, 0, ll); // copy data for each
// segment from input
// byte array to temp
// segment byte array
this.inputData.add(tmpInputSegment); // add each segment to
// inputData Vector
numberOfSegments++;
bytesProcessed += (ll);
}
}
this.rebuildData = true;
}
}
/**
* @param string
*/
public void setInputMessageData(byte[][] anInputData2DByteArray)
throws ImsConnectApiException {
int i = 0;
int numberOfSegments = anInputData2DByteArray.length;
int numberOfSegmentsProcessed = 0;
int inputData2DByteArraySegmentLen = 0;
/*
* if (isTmInteractionPropertiesAlreadySet() == false) {
* this.setTmInteractionProperties();
* if(this.myTmInteractionImpl.getInteractionTypeDescription
* ().equalsIgnoreCase
* (ApiProperties.INTERACTION_TYPE_DESC_TYPE2_COMMAND)) {
* this.setType2CommandInteractionProperties(); }
* this.tmInteractionPropertiesAlreadySet = true; }
*/
this
.setInputMessageDataSegmentsIncludeLlzzAndTrancode(this.myTmInteractionImpl
.isInputMessageDataSegmentsIncludeLlzzAndTrancode());
this.inputData.clear();
while (i < numberOfSegments) {
inputData2DByteArraySegmentLen = anInputData2DByteArray[i].length;
if (inputData2DByteArraySegmentLen > 0) // If there is data in
// anInputData2DByteArray[i]
// to be processed
{
short segLen = (short) anInputData2DByteArray[i].length;
if ((segLen < 4) || (segLen > 32767)) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0036E,
new Object[] { segLen });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0036E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessageImpl.setInputMessageData(byte[][]). Exception caught was: "
+ e.getMessage());
throw e;
}
this.inputData.add(anInputData2DByteArray[i]);
numberOfSegmentsProcessed++;
}
i++;
}
this.setNumberOfSegments(numberOfSegmentsProcessed);
this.rebuildData = true;
}
/**
* @param string
*/
public void setInputMessageData(String anInputDataString)
throws ImsConnectApiException {
imsConnectCodepage = myTmInteractionImpl.getImsConnectCodepage();
try {
if (this.myTmInteractionImpl
.isInputMessageDataSegmentsIncludeLlzzAndTrancode()) {
String errMsg = ImsConnectErrorMessage
.getString(ImsConnectErrorMessage.HWS0035E);
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0035E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.setInputMessageData(String). Exception caught was: "
+ e.getMessage());
throw e;
} else {
this.setInputMessageData(anInputDataString
.getBytes(imsConnectCodepage));
}
} catch (UnsupportedEncodingException usee) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0017E, new Object[] {
"input request message", this.imsConnectCodepage });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.setInputMessageData(String): ["
+ e.getMessage() + "]+");
throw e;
}
}
public void setInputMessageData(String[] anInputDataStringArray)
throws ImsConnectApiException {
int i = 0;
int numberOfSegments = anInputDataStringArray.length;
byte[][] inData2DByteArray = new byte[numberOfSegments][];
try {
if (this.myTmInteractionImpl
.isInputMessageDataSegmentsIncludeLlzzAndTrancode()) {
String errMsg = ImsConnectErrorMessage
.getString(ImsConnectErrorMessage.HWS0035E);
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0035E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.setInputMessageData(String[]). Exception caught was: "
+ e.getMessage());
throw e;
} else {
while (i < numberOfSegments) {
inData2DByteArray[i] = (anInputDataStringArray[i]
.getBytes(imsConnectCodepage));
i++;
}
this.setInputMessageData(inData2DByteArray);
}
} catch (UnsupportedEncodingException usee) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0017E, new Object[] {
"input request message", this.imsConnectCodepage });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.setInputMessageData(String[]): ["
+ e.getMessage() + "]+");
throw e;
}
}
// Property Getters and Setters
/**
* @return
*/
public byte[] getData() {
return data;
}
/**
* @param bs
*/
public void setData(byte[] aByteArray) {
if ((aByteArray != null)
&& (this.myTmInteractionImpl.isInConversation())
&& (!this.isTranRemoved())
&& ((this.myTmInteractionImpl.getInteractionTypeDescription() == "SENDRECV")
|| (this.myTmInteractionImpl
.getInteractionTypeDescription() == "SENDONLY")
|| (this.myTmInteractionImpl
.getInteractionTypeDescription() == "SENDONLYACK") || (this.myTmInteractionImpl
.getInteractionTypeDescription() == "SENDONLYXCFORDDLV"))) {
int trancodeLen = 0;
if (trancodeInData != null)
trancodeLen = trancodeInData.length;
int tmpByteArrayLen = aByteArray.length - trancodeLen;
byte[] tmpByteArray = new byte[tmpByteArrayLen];
System.arraycopy(this
.createLlzzByteArrayFromLlValue(tmpByteArrayLen), 0,
tmpByteArray, 0, 4);
System.arraycopy(aByteArray, 4 + trancodeLen, tmpByteArray, 4,
tmpByteArrayLen - 4);
data = tmpByteArray;
this.setTranRemoved(true);
} else
data = aByteArray;
this.setTmInteractionPropertiesAlreadySet(false);
}
/**
* @return Returns the imsConnectCodepage.
*/
public String getImsConnectCodepage() {
return imsConnectCodepage;
}
/**
* @param anImsConnectCodepage
*/
protected void setImsConnectCodepage(String anImsConnectCodepage) {
if ((this.imsConnectCodepage == anImsConnectCodepage)
|| (anImsConnectCodepage == null))
return;
else {
this.imsConnectCodepage = anImsConnectCodepage;
this.rebuildData = true;
}
}
/**
* @return the inConversation
*/
protected boolean isInConversation() {
return this.inConversation;
}
/**
* @param inConversation
* the inConversation to set
*/
protected void setInConversation(boolean inConversation) {
this.inConversation = inConversation;
}
/**
* @return the inputData
*/
/*
* private Vector getInputData() { return this.inputData; }
*
* /**
*
* @param inputData the inputData to set
*/
/*
* private void setInputData(Vector inputData) { this.inputData = inputData;
* }
*
* /**
*
* @return the inputMessageDataSegmentsIncludeLlzzAndTrancode property value
*/
public boolean isInputMessageDataSegmentsIncludeLlzzAndTrancode() {
return this.inputMessageDataSegmentsIncludeLlzzAndTrancode;
}
/**
* @param b
*/
public void setInputMessageDataSegmentsIncludeLlzzAndTrancode(
boolean anInputMessageDataSegmentsIncludeLlzzAndTrancode) {
this.inputMessageDataSegmentsIncludeLlzzAndTrancode = anInputMessageDataSegmentsIncludeLlzzAndTrancode;
}
/**
* @return the irmArch
*/
private byte getIrmArch() {
return this.irmArch;
}
/**
* @param b
*/
private void setIrmArch(byte b) {
irmArch = b;
}
/**
* @return the irmClientId
*/
private byte[] getIrmClientId() {
return this.irmClientId;
}
/**
* @param aClientId
*/
private void setIrmClientId(String aClientId) throws ImsConnectApiException {
try {
String anIrmClientId = padString(aClientId, ' ',
InputMessageProperties.IRM_CLIENTID_LEN);
this.irmClientId = anIrmClientId.getBytes(this.imsConnectCodepage);
} catch (UnsupportedEncodingException usee) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0017E, new Object[] {
"input request message", this.imsConnectCodepage });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.setIrmClientId(String): ["
+ e.getMessage() + "]+");
throw e;
}
}
/**
* @return the irmDestId
*/
private byte[] getIrmDestId() {
return this.irmDestId;
}
/**
* @param aDestId
*/
private void setIrmDestId(String aDestId) throws ImsConnectApiException {
try {
String anIrmDestId = padString(aDestId, ' ',
InputMessageProperties.IRM_IMSDESTID_MAX_LEN);
this.irmDestId = anIrmDestId.getBytes(this.imsConnectCodepage);
} catch (UnsupportedEncodingException usee) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0017E, new Object[] {
"input request message", this.imsConnectCodepage });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.setIrmDestId(String): ["
+ e.getMessage() + "]+");
throw e;
}
}
/**
* @return the irmEncodingSchema
*/
private byte getIrmEncodingSchema() {
return this.irmEncodingSchema;
}
/**
* @param b
*/
private void setIrmEncodingSchema(byte b) {
irmEncodingSchema = b;
}
/**
* @return the irmF0
*/
private byte getIrmF0() {
return this.irmF0;
}
/**
* @param b
*/
private void setIrmF0(byte b) {
irmF0 |= b;
}
/**
* @return the irmF1
*/
private byte getIrmF1() {
return this.irmF1;
}
/**
* @param b
*/
private void setIrmF1(byte aByte) {
irmF1 |= aByte;
}
/**
* @return the irmF2
*/
private byte getIrmF2() {
return this.irmF2;
}
/**
* @param b
*/
private void setIrmF2(byte b) {
irmF2 = b;
}
/**
* @return the irmF3
*/
private byte getIrmF3() {
return this.irmF3;
}
/**
* @param b
*/
private void setIrmF3(byte b) {
irmF3 |= b;
}
/**
* @return the irmF4
*/
private char getIrmF4() {
return this.irmF4;
}
/**
* @param c
*/
private void setIrmF4(char c) throws ImsConnectApiException {
try {
// irmF4 = c;
irmF4 = (char) ((new StringBuffer().append(c)).toString())
.getBytes(imsConnectCodepage)[0];
} catch (UnsupportedEncodingException usee) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0017E, new Object[] {
"input request message", this.imsConnectCodepage });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.setIrmF4(char): ["
+ e.getMessage() + "]+");
throw e;
} catch (Exception e) {
}
}
/**
* @return the irmF5
*/
private int getIrmF5() {
return this.irmF5;
}
/**
* @param b
*/
private void setIrmF5(int anInt) {
irmF5 |= anInt;
}
/**
* @return the irmId
*/
private byte[] getIrmId() {
return this.irmId;
}
/**
* @param anIrmId
*/
private void setIrmId(String anIrmIdString) throws ImsConnectApiException {
try {
String anIrmId = null;
anIrmId = padString(anIrmIdString, ' ',
InputMessageProperties.IRM_ID_LEN);
this.irmId = anIrmId.getBytes(this.imsConnectCodepage);
} catch (UnsupportedEncodingException usee) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0017E, new Object[] {
"input request message", this.imsConnectCodepage });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.setIrmId(String): ["
+ e.getMessage() + "]+");
throw e;
}
}
/**
* @return the irmLen
*/
private int getIrmLen() {
return this.irmLen;
}
/**
* @param irmLen
* the irmLen to set
*/
private void setIrmLen(int anIrmLen) {
this.irmLen = anIrmLen;
}
/**
* @return the irmLTerm
*/
private byte[] getIrmLTerm() {
return this.irmLTerm;
}
/**
* @param anIrmLTerm
*/
private void setIrmLTerm(String anIrmLTerm) throws ImsConnectApiException {
try {
String anIrmLTermName = padString(anIrmLTerm, ' ',
InputMessageProperties.IRM_LTERM_MAX_LEN);
this.irmLTerm = anIrmLTermName.getBytes(this.imsConnectCodepage);
} catch (UnsupportedEncodingException usee) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0017E, new Object[] {
"input request message", this.imsConnectCodepage });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.setIrmId(String): ["
+ e.getMessage() + "]+");
throw e;
}
}
/**
* @return the irmRacfApplName
*/
private byte[] getIrmRacfApplName() {
return this.irmRacfApplName;
}
/**
* @param anIrmRacfApplName
*/
private void setIrmRacfApplName(String anIrmRacfApplName)
throws ImsConnectApiException {
try {
String aIrmRacfApplName = padString(anIrmRacfApplName, ' ',
InputMessageProperties.IRM_APPL_NM_MAX_LEN);
this.irmRacfApplName = aIrmRacfApplName
.getBytes(this.imsConnectCodepage);
} catch (UnsupportedEncodingException usee) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0017E, new Object[] {
"input request message", this.imsConnectCodepage });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.setIrmRacfApplName(String): ["
+ e.getMessage() + "]+");
throw e;
}
}
/**
* @return the irmRacfGroupName
*/
private byte[] getIrmRacfGroupName() {
return this.irmRacfGroupName;
}
/**
* @param aRacfGroupName
*/
private void setIrmRacfGroupName(String aRacfGroupName)
throws ImsConnectApiException {
try {
String anIrmRacfGroupName = padString(aRacfGroupName, ' ',
InputMessageProperties.IRM_RACF_GRNAME_MAX_LEN);
this.irmRacfGroupName = anIrmRacfGroupName
.getBytes(this.imsConnectCodepage);
} catch (UnsupportedEncodingException usee) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0017E, new Object[] {
"input request message", this.imsConnectCodepage });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.setIrmRacfGroupName(String): ["
+ e.getMessage() + "]+");
throw e;
}
}
/**
* @return the irmRacfPassword
*/
private byte[] getIrmRacfPassword() {
return this.irmRacfPassword;
}
/**
* @param anIrmRacfPassword
*/
private void setIrmRacfPassword(String anIrmRacfPassword)
throws ImsConnectApiException {
try {
String anIrmRacfPasswordString = padString(anIrmRacfPassword, ' ',
InputMessageProperties.IRM_RACF_PW_MAX_LEN);
this.irmRacfPassword = anIrmRacfPasswordString
.getBytes(this.imsConnectCodepage);
} catch (UnsupportedEncodingException usee) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0017E, new Object[] {
"input request message", this.imsConnectCodepage });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.setIrmRacfPassword(String): ["
+ e.getMessage() + "]+");
throw e;
}
}
/**
* @return the irmRacfUserId
*/
private byte[] getIrmRacfUserId() {
return this.irmRacfUserId;
}
/**
* @param anIrmRacfUserId
*/
private void setIrmRacfUserId(String anIrmRacfUserId)
throws ImsConnectApiException {
try {
String anIrmRacfUserIdString = padString(anIrmRacfUserId, ' ',
InputMessageProperties.IRM_RACF_USERID_MAX_LEN);
this.irmRacfUserId = anIrmRacfUserIdString
.getBytes(this.imsConnectCodepage);
} catch (UnsupportedEncodingException usee) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0017E, new Object[] {
"input request message", this.imsConnectCodepage });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.setIrmRacfUserId(String): ["
+ e.getMessage() + "]+");
throw e;
}
}
/**
* @return the irmRerouteName
*/
private byte[] getIrmRerouteName() {
return this.irmRerouteName;
}
/**
* @param anIrmRerouteName
*/
private void setIrmRerouteName(String anIrmRerouteName)
throws ImsConnectApiException {
try {
String anIrmRerouteNameString = padString(anIrmRerouteName, ' ',
InputMessageProperties.IRM_REROUT_NM_MAX_LEN);
this.irmRerouteName = anIrmRerouteNameString
.getBytes(this.imsConnectCodepage);
} catch (UnsupportedEncodingException usee) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0017E, new Object[] {
"input request message", this.imsConnectCodepage });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.setIrmRerouteName(String): ["
+ e.getMessage() + "]+");
throw e;
}
}
/**
* @return the irmResWord
*/
private short getIrmResWord() {
return this.irmResWord;
}
/**
* @param bs
*/
private void setIrmResWord(short i) {
irmResWord = i;
}
/**
* @return the irmSocType
*/
private byte getIrmSocType() {
return this.irmSocType;
}
/**
* @param b
*/
private void setIrmSocType(byte b) {
irmSocType = b;
}
/**
* @return the irmTagAdapter
*/
private byte[] getIrmTagAdapter() {
return this.irmTagAdapter;
}
/**
* @param anIrmTagAdapter
*/
private void setIrmTagAdapter(String anIrmTagAdapter)
throws ImsConnectApiException {
try {
String anIrmTagAdapterString = padString(anIrmTagAdapter, ' ',
InputMessageProperties.IRM_TAG_ADAPT_MAX_LEN);
this.irmTagAdapter = anIrmTagAdapterString
.getBytes(this.imsConnectCodepage);
} catch (UnsupportedEncodingException usee) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0017E, new Object[] {
"input request message", this.imsConnectCodepage });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.setIrmTagAdapter(String): ["
+ e.getMessage() + "]+");
throw e;
}
}
/**
* @return the irmTagMap
*/
private byte[] getIrmTagMap() {
return this.irmTagMap;
}
/**
* @param anIrmTagMap
*/
private void setIrmTagMap(String anIrmTagMap) throws ImsConnectApiException {
try {
String anIrmTagMapString = padString(anIrmTagMap, ' ',
InputMessageProperties.IRM_TAG_MAP_MAX_LEN);
this.irmTagMap = anIrmTagMapString
.getBytes(this.imsConnectCodepage);
} catch (UnsupportedEncodingException usee) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0017E, new Object[] {
"input request message", this.imsConnectCodepage });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.setIrmTagMap(String): ["
+ e.getMessage() + "]+");
throw e;
}
}
/**
* @return the irmTimer
*/
private int getIrmTimer() {
return this.irmTimer;
}
/**
* @param b
*/
protected void setIrmTimer(int anInt) {
irmTimer = anInt;
}
/**
* @return the irmTrancode
*/
private byte[] getIrmTrancode() {
return this.irmTrancode;
}
/**
* @param anIrmTrancode
*/
private void setIrmTrancode(String anIrmTrancode)
throws ImsConnectApiException {
try {
int irmTrancodeLen = anIrmTrancode.length();
String anIrmTrancodeString;
if (irmTrancodeLen < 8)
anIrmTrancodeString = padString(anIrmTrancode, ' ',
InputMessageProperties.IRM_TRANCODE_MAX_LEN);
else if (irmTrancodeLen > 8)
anIrmTrancodeString = anIrmTrancode.substring(0, 8);
else
anIrmTrancodeString = anIrmTrancode;
this.irmTrancode = anIrmTrancodeString
.getBytes(this.imsConnectCodepage);
} catch (Exception e1) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0017E, new Object[] {
"input request message", this.imsConnectCodepage });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.setIrmTrancode(String): ["
+ e1.toString() + "]+");
throw e;
}
}
/**
* @return
*/
public int getLlll() {
return llll;
}
/**
* @param string
*/
private void setLlll(int anLlll) {
this.llll = anLlll;
}
/**
* @return the message
*/
public byte[] getMessage() throws ImsConnectApiException {
boolean suppressLogging = true;
return this.buildInputMessageByteArray(suppressLogging);
}
/**
* @param message
* the message to set
*/
/*
* private void setMessage(byte[] message) { this.message = message; }
*
* /**
*
* @return Returns myTmInteractionImpl.
*/
private TmInteraction getMyTmInteractionImpl() {
return myTmInteractionImpl;
}
/**
* @param myTMInteraction
* The myTmInteractionImpl to set.
*/
protected void setMyTmInteractionImpl(TmInteractionImpl myTMInteractionImpl) {
this.myTmInteractionImpl = myTMInteractionImpl;
}
/**
* @return the connectionPropertiesAlreadySet
*/
protected boolean isConnectionPropertiesAlreadySet() {
return this.connectionPropertiesAlreadySet;
}
/**
* @param connectionPropertiesAlreadySet
* the connectionPropertiesAlreadySet to set
*/
protected void setConnectionPropertiesAlreadySet(
boolean connectionPropertiesAlreadySet) {
this.connectionPropertiesAlreadySet = connectionPropertiesAlreadySet;
}
/**
* @return the tmInteractionPropertiesAlreadySet
*/
protected boolean isTmInteractionPropertiesAlreadySet() {
return this.tmInteractionPropertiesAlreadySet;
}
/**
* @param tmInteractionPropertiesAlreadySet
* the tmInteractionPropertiesAlreadySet to set
*/
protected void setTmInteractionPropertiesAlreadySet(
boolean tmInteractionPropertiesAlreadySet) {
this.tmInteractionPropertiesAlreadySet = tmInteractionPropertiesAlreadySet;
}
/**
* @return Returns the trancodeInData.
*/
@SuppressWarnings("unused")
private byte[] getTrancodeInData() {
return this.trancodeInData;
}
/**
* @param aTrancodeInData
* The trancodeInData to set.
*/
protected void setTrancodeInData(String aTrancodeInData)
throws ImsConnectApiException {
try {
// Don't check for length since trancode in data segment can be
// longer than 8 bytes
this.trancodeInData = aTrancodeInData.getBytes(imsConnectCodepage);
} catch (UnsupportedEncodingException usee) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0017E, new Object[] {
"input request message", this.imsConnectCodepage });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.setIrmTrancodeInData(String): ["
+ e.getMessage() + "]+");
throw e;
}
}
/**
* @param numberOfSegments
* the numberOfSegments to set
*/
private void setNumberOfSegments(int numberOfSegments) {
this.numberOfSegments = numberOfSegments;
}
/**
* @return the logger
*/
/*
* private Logger getLogger() { return this.logger; }
*
* /**
*
* @param logger the logger to set
*/
/*
* private void setLogger(Logger logger) { this.logger = logger; }
*
* /**
*
* @return the tranRemoved
*/
protected boolean isTranRemoved() {
return this.tranRemoved;
}
/**
* @param tranRemoved
* the tranRemoved to set
*/
protected void setTranRemoved(boolean tranRemoved) {
this.tranRemoved = tranRemoved;
}
public String toString() {
String str = "";
int dataBeginPos = 4; // for LLLL
if (this.irmArch == InputMessageProperties.IRM_ARCH0)
dataBeginPos += InputMessageProperties.IRM_LEN_ARCH0;
else if (this.irmArch == InputMessageProperties.IRM_ARCH1)
dataBeginPos += InputMessageProperties.IRM_LEN_ARCH1;
else if (this.irmArch == InputMessageProperties.IRM_ARCH2)
dataBeginPos += InputMessageProperties.IRM_LEN_ARCH2;
if (!this.inputMessageDataSegmentsIncludeLlzzAndTrancode) // add len for
// LLZZTrancode
dataBeginPos += (4 + this.irmTrancode.length);
try {
boolean logBuffer = false;
byte[] bytes = this.buildInputMessageByteArray(logBuffer);
// str = ImsConnectTrace.toHexString(bytes, dataBeginPos,
// bytes.length - 4, this.irmCodepage,
// this.dataCodepage, ImsConnectTrace.STYLE_INPUT_FORMAT);
boolean obfuscatePassword = true;
str = myTmInteractionImpl.formatBufferForTracing(bytes,
obfuscatePassword);
} catch (Exception e1) {
}
return str;
}
/**
* @param byte[] aSegmentData (data for a particular segment)
* @param int llPos (position of first byte of LL within aSegmentData)
*/
private int getLlValueFromLlzzBytes(byte[] aSegmentData, int llPos) {
int lowOrderLL;
int highOrderLL;
lowOrderLL = (new Integer(aSegmentData[llPos + 1])).intValue();
lowOrderLL += (lowOrderLL < 0 ? 256 : 0); // add 256 if highest order
// bit is set on
highOrderLL = (new Integer(aSegmentData[llPos])).intValue();
highOrderLL += (highOrderLL < 0 ? 256 : 0); // add 256 if highest order
// bit is set on
highOrderLL *= 256;
return lowOrderLL + highOrderLL;
}
/**
* @param int anLlValue (the LL value to be converted to a 4-byte LLZZ
* bytearray (ZZ will be set to 0000)
*/
private byte[] createLlzzByteArrayFromLlValue(int anLlValue) {
byte[] llzzByteArray = new byte[4];
if (this.myTmInteractionImpl.getInteractionTypeDescription().equals(
ApiProperties.INTERACTION_TYPE_DESC_SENDONLY_CALLOUT_RESPONSE)
||this.myTmInteractionImpl.getInteractionTypeDescription().equals(
ApiProperties.INTERACTION_TYPE_DESC_SENDONLYACK_CALLOUT_RESPONSE)) {
llzzByteArray[0] = (byte) ((anLlValue >> 24) & 0xff);
llzzByteArray[1] = (byte) ((anLlValue >> 16) & 0xff);
llzzByteArray[2] = (byte) ((anLlValue >> 8) & 0xff);
llzzByteArray[3] = (byte) (anLlValue & 0xff);
} else {
llzzByteArray[0] = (byte) ((anLlValue >> 8) & 0xff);
llzzByteArray[1] = (byte) (anLlValue & 0xff);
llzzByteArray[2] = 0;
llzzByteArray[3] = 0;
}
return llzzByteArray;
}
/**
* @param byte[] aSegmentData (data for a particular segment)
*/
@SuppressWarnings("unused")
private int getLlllValueFromBytes(byte[] aSegmentData) {
return (aSegmentData[0] << 24) + ((aSegmentData[1] & 0xFF) << 16)
+ ((aSegmentData[2] & 0xFF) << 8) + (aSegmentData[3] & 0xFF);
/*
* int llll;
*
* llll = (new Integer(aSegmentData[3])).intValue(); llll += ((new
* Integer(aSegmentData[2])).intValue())*16; llll += ((new
* Integer(aSegmentData[1])).intValue())*256; llll += ((new
* Integer(aSegmentData[0])).intValue())*65536;
*
* return llll;
*/
}
/**
* @param int anLlllValue (the LLLL value to be converted to a 4-byte LLLL
* bytearray
*/
@SuppressWarnings("unused")
private byte[] createLlllByteArrayFromLlllValue(int anLlllValue) {
return new byte[] { (byte) (anLlllValue >>> 24),
(byte) (anLlllValue >>> 16), (byte) (anLlllValue >>> 8),
(byte) anLlllValue };
/*
* byte[] llllByteArray = new byte[4];
*
* llllByteArray[0] = (byte)((anLlllValue >> 24) & 0xff);
* llllByteArray[1] = (byte)((anLlllValue >> 16) & 0xff);
* llllByteArray[2] = (byte)((anLlllValue >> 8) & 0xff);
* llllByteArray[3] = (byte)(anLlllValue & 0xff);
*
* return llllByteArray;
*/
}
// ------------------Added for Sync
// callout------------------------------////
// sets the value of the corelator to be set to the IRM header
private void setIrmCorelatorToken(byte[] acorelatorToken) {
this.irmCorelatorToken = acorelatorToken;
}
// Gets the value of the corelator that is st to the IRM header
private byte[] getIrmCorelatorToken() {
return this.irmCorelatorToken;
}
// sets the value of the corelator to be set to the IRM header
private void setIrmInputModName(String anInputModName)
throws ImsConnectApiException {
try {
String anIrmInputModNameString = padString(anInputModName, ' ',
InputMessageProperties.IRM_INPUT_MOD_NAME_MAX_LEN);
this.irmInputModname = anIrmInputModNameString
.getBytes(this.imsConnectCodepage);
} catch (UnsupportedEncodingException usee) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0017E, new Object[] {
"input request message", this.imsConnectCodepage });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.setIrmInputModName(String): ["
+ e.getMessage() + "]+");
throw e;
}
}
// Gets the value of the corelator that is st to the IRM header
private byte[] getIrmInputModName() {
return this.irmInputModname;
}
// sets the value of the corelator to be set to the IRM header
private void setIrmNakReasonCode(short aNakReasonCode)
throws ImsConnectApiException {
if ((aNakReasonCode >= InputMessageProperties.IRM_NAK_REASONCODE_LOW && aNakReasonCode <= InputMessageProperties.IRM_NAK_REASONCODE_HIGH)
|| (aNakReasonCode == 0)) {
this.irmNakReasonCode = aNakReasonCode;
} else {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0041E, new Object[] {
aNakReasonCode,
InputMessageProperties.IRM_NAK_REASONCODE_LOW,
InputMessageProperties.IRM_NAK_REASONCODE_HIGH });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0041E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in InputMessage.setIrmNakReasonCode(short): ["
+ e.getMessage() + "]+");
throw e;
}
}
// Gets the value of the corelator that is st to the IRM header
private short getIrmNakReasonCode() {
return this.irmNakReasonCode;
}
// --------------------End-----------------------------------------------////
public static String bytesToHexString(byte[] bytes) {
if (bytes == null || bytes.length == 0)
return "";
StringBuilder sb = new StringBuilder(bytes.length * 2);
Formatter formatter = new Formatter(sb);
for (byte b : bytes) {
formatter.format("%02x", b);
}
// insert a space every 4 bytes
int length = sb.length();
int i = 0;
//int j = 0;
//while( i < length) {
//if (i%8 == 0){
// sb.insert(i+j, ' ');
// j++;
//}
// i++;
//}
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy