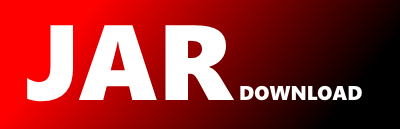
com.ibm.ims.connect.ApiLoggingConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of IMSConnectAPI Show documentation
Show all versions of IMSConnectAPI Show documentation
API that allows Java applications to interface with IMS Connect
The newest version!
/*
* File: ApiLoggingConfiguration.java
* ==========================================================================
* Licensed Material - Property of IBM
*
* IBM Confidential
*
* OCO Source Materials
*
* 5655-T62
*
* (C) Copyright IBM Corp. 2009,2014 All Rights Reserved.
*
* The source code for this program is not published or
* otherwise divested of its trade secrets, irrespective
* of what has been deposited with the U.S. Copyright
* Office.
*
* US Government Users Restricted Rights - Use, duplication or
* disclosure restricted by GSA ADP Schedule Contract with
* IBM Corp.
* ===========================================================================
*/
package com.ibm.ims.connect;
import java.util.logging.FileHandler;
//import java.util.logging.Formatter;
import java.util.logging.Handler;
import java.util.logging.Level;
import java.util.logging.LogRecord;
import java.util.logging.Logger;
import java.util.logging.SimpleFormatter;
import java.util.Date;
import java.io.IOException;
import java.text.DateFormat;
//import java.text.MessageFormat;
import java.text.SimpleDateFormat;
/**
* A helper class to configure tracing for IMS Connect API for Java applications.
*
*/
public class ApiLoggingConfiguration
{
@SuppressWarnings("unused")
private static final String copyright =
"Licensed Material - Property of IBM "
+ "5655-TDA"
+ "(C) Copyright IBM Corp. 2009,2015 All Rights Reserved. "
+ "US Government Users Restricted Rights - Use, duplication or "
+ "disclosure restricted by GSA ADP Schedule Contract with IBM Corp. ";
private Logger logger;
private Handler loggerFileHandler;
private Level loggerLevel;
private String loggerTraceFileName;
private SimpleFormatter mySimpleFormatter;
private boolean loggerAppendMode = ApiProperties.DISABLE_LOGGER_APPEND_MODE;
private int loggerFileLimit = ApiProperties.DEFAULT_LOGGER_FILE_LIMIT;
private int loggerFileCount = ApiProperties.DEFAULT_LOGGER_FILE_COUNT;
private static final String sdf = "EEE, MMM dd yyyy, HH.mm.ss.SSS z";
// See SimpleDateFormatter class Javadoc for other formatting options
private static final DateFormat df = new SimpleDateFormat(sdf);
private static final String lBracket = "[";
private static final String rBracket = "] ";
private static final String blank = " ";
private static final String newLine = System.getProperty("line.separator");
private static final String version = "3.1.0.8";
private static final String build = "2015_03_31_2029" +
" ";
private int i;
/**
* Logs trace information for diagnosing problems with the IMS Connect API. You can specify the trace level
* for this logger.
* The valid values, for which the following constants are defined in ApiProperties
, are:
*
* TRACE_LEVEL_NONE
- API tracing disabled
* TRACE_ERROR_EXCEPTION
- Trace errors and exceptions only
* TRACE_ENTRY_EXIT
- Trace API errors and exceptions plus method entry and exit
* TRACE_INTERNAL
- Full internal API tracing enabled. Trace API errors and exceptions,
* method entry and exit, and internal trace statements
* DEFAULT_TRACE_LEVEL
- same as TRACE_LEVEL_NONE
*
* If a value for this property is not supplied by the client, the default value (TRACE_LEVEL_NONE
) will be used.
*
* @param aTraceFileName file to store trace output
* @param aLevel tracing level. Use the constants defined in ApiProperties
to specify this parameter.
* @return a java.util.logging.Logger
instance.
* @throws IMSConnectAPIException
* @see ApiProperties#DEFAULT_TRACE_LEVEL
* @see ApiProperties#TRACE_LEVEL_ENTRY_EXIT
* @see ApiProperties#TRACE_LEVEL_EXCEPTION
* @see ApiProperties#TRACE_LEVEL_INTERNAL
* @see ApiProperties#TRACE_LEVEL_NONE
*/
public Logger configureApiLogging(String aTraceFileName, Level aLevel) throws ImsConnectApiException
{
loggerTraceFileName = aTraceFileName;
loggerLevel = aLevel;
try
{
mySimpleFormatter = new SimpleFormatter()
{
public String format(LogRecord record)
{
long threadId = Thread.currentThread().getId();
StringBuffer sb = new StringBuffer();
sb.append(df.format(new Date(record.getMillis()))).append(blank);
sb.append(lBracket).append("Thread # : "+threadId).append(rBracket);
sb.append(lBracket).append(record.getLevel().getName()).append(rBracket);
sb.append(formatMessage(record)).append(newLine);
return sb.toString();
}
};
setLoggerFileHandler();
logger.setLevel(aLevel);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL))
{
logger.finest(" configureApiLogging() - Trace log now open for IMS Connect API version [" + version + "], build [" + build + "]");
// System.out.println("Tracing output will be logged to " + aTraceFileName); // Write name and location of trace output file to console if trace level is internal
}
}
catch (ImsConnectApiException e)
{
// re-throw exception
throw e;
}
return logger;
}
private void setLoggerFileHandler()
throws ImsConnectApiException
{
try
{
logger = Logger.getLogger("com.ibm.ims.connect");
Handler[] handlers = logger.getHandlers();
for (i=0; i < handlers.length; i++)
{
if (handlers[i].getClass() == FileHandler.class)
break;
}
if (i < handlers.length)
logger.removeHandler(handlers[i]);
loggerFileHandler = new FileHandler(loggerTraceFileName,this.getLoggerFileLimit(),this.getLoggerFileCount(),this.isLoggerAppendModeEnabled());
loggerFileHandler.setFormatter(mySimpleFormatter);
loggerFileHandler.setLevel(loggerLevel);
loggerFileHandler.setEncoding(ApiProperties.DEFAULT_CODEPAGE_FOR_LOGGING);
logger.addHandler(loggerFileHandler);
}
catch (IOException e)
{
// throw exception
String fileTypeString = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.TRACE_FILE);
String errMsg = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0002E,
new Object[] { fileTypeString, loggerTraceFileName, e });
ImsConnectApiException newExc = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0002E, errMsg);
throw newExc;
}
}
/**
* Gets the current API build number.
* @return the build
*/
public static String getBuild()
{
return build;
}
/**
* Gets the current API version number
* @return the version
*/
public static String getVersion()
{
return version;
}
/**
* Gets the current API logging level.
* @return the loggerLevel
*/
public Level getLoggerLevel()
{
return this.loggerLevel;
}
/**
* Gets the current trace file name.
* @return the loggerTraceFileName
*/
public String getLoggerTraceFileName()
{
return this.loggerTraceFileName;
}
/**
* Sets the tracing level for the logger.
* The valid values, for which the following constants are defined in ApiProperties
, are:
*
* TRACE_LEVEL_NONE
- API tracing disabled
* TRACE_ERROR_EXCEPTION
- Trace errors and exceptions only
* TRACE_ENTRY_EXIT
- Trace API errors and exceptions plus method entry and exit
* TRACE_INTERNAL
- Full internal API tracing enabled. Trace API errors and exceptions,
* method entry and exit, and internal trace statements
* DEFAULT_TRACE_LEVEL
- same as TRACE_LEVEL_NONE
*
* If a value for this property is not supplied by the client, the default value (TRACE_LEVEL_NONE
) will be used.
* @param aLevel tracing level. Use the constants defined in ApiProperties
to specify this parameter.
* @throws ImsConnectApiException
* @see ApiProperties#DEFAULT_TRACE_LEVEL
* @see ApiProperties#TRACE_LEVEL_ENTRY_EXIT
* @see ApiProperties#TRACE_LEVEL_EXCEPTION
* @see ApiProperties#TRACE_LEVEL_INTERNAL
* @see ApiProperties#TRACE_LEVEL_NONE
*/
public void setLoggerLevel(Level aLevel) throws ImsConnectApiException
{
loggerLevel = aLevel;
try
{
setLoggerFileHandler();
}
catch (ImsConnectApiException e)
{
// re-throw exception
throw e;
}
logger.setLevel(aLevel);
}
/**
* Sets the file to store trace output.
* @param aTraceFileName the name of the file to store trace output.
* @throws ImsConnectApiException
*/
public void setLoggerTraceFileName(String aTraceFileName) throws ImsConnectApiException
{
loggerTraceFileName = aTraceFileName;
try
{
setLoggerFileHandler();
}
catch (ImsConnectApiException e)
{
// re-throw exception
throw e;
}
}
/**
*Sets the append mode of the Connect API logging file handler to true or false
** @param
* aBooleanAppendValue value. Use the constants defined in ApiProperties
* to specify this parameter.
* @throws ImsConnectApiException
* @see ApiProperties#ENABLE_LOGGER_APPEND_MODE
* @see ApiProperties#DISABLE_LOGGER_APPEND_MODE
*/
public void setLoggerToAppendMode(boolean aBooleanAppendValue)
{
this.loggerAppendMode = aBooleanAppendValue;
}
/**
* Gets the API logging file handler's append mode.
* @return Handler
*/
public boolean isLoggerAppendModeEnabled()
{
return this.loggerAppendMode;
}
/**
*Sets the maximum number of bytes that can be written to an API log file through the Connect API logging file handler.
** @param
* aLimitValue value. Use the constants defined in ApiProperties
* to specify this parameter.
* @throws ImsConnectApiException
* @see ApiProperties#LOGGER_FILE_NO_LIMIT
*/
public void setLoggerFileLimit(int aLimitValue)
{
this.loggerFileLimit = aLimitValue;
}
/**
* Gets the current setting for the maximum number of bytes that can be written to an API log file.
* @return Handler
*/
public int getLoggerFileLimit()
{
return this.loggerFileLimit;
}
/**
*Sets the number of log files that the API logger will create before overwriting the oldest log file.
** @param
* aCountValue value. Use the constants defined in ApiProperties
* to specify this parameter.
* @throws ImsConnectApiException
* @see ApiProperties#DEFAULT_LOGGER_FILE_COUNT
*/
public void setLoggerFileCount(int aCountValue)
{
this.loggerFileCount = aCountValue;
}
/**
* Gets the number of log files that the API logger will create before overwriting the oldest log file.
* @return Handler
*/
public int getLoggerFileCount()
{
return this.loggerFileCount;
}
/**
* Gets the API logging file handler.
* @return Handler
*/
public Handler getLoggerFileHandler()
{
return loggerFileHandler;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy