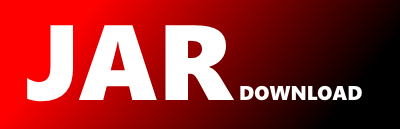
com.ibm.ims.connect.ApiProperties Maven / Gradle / Ivy
Show all versions of IMSConnectAPI Show documentation
/*
* File: ApiProperties.java
* ==========================================================================
* Licensed Material - Property of IBM
*
* IBM Confidential
*
* OCO Source Materials
*
* 5655-TDA
*
* (C) Copyright IBM Corp. 2009,2014 All Rights Reserved.
*
* The source code for this program is not published or
* otherwise divested of its trade secrets, irrespective
* of what has been deposited with the U.S. Copyright
* Office.
*
* US Government Users Restricted Rights - Use, duplication or
* disclosure restricted by GSA ADP Schedule Contract with
* IBM Corp.
* ===========================================================================
*/
package com.ibm.ims.connect;
import java.io.InputStream;
import java.net.URL;
import java.util.logging.Level;
/**
* Constants for setting IMS Connect API connection and interaction properties.
*/
public interface ApiProperties {
public static final String copyright = "Licensed Material - Property of IBM "
+ "5655-TDA"
+ "(C) Copyright IBM Corp. 2009, 2013 All Rights Reserved. "
+ "US Government Users Restricted Rights - Use, duplication or "
+ "disclosure restricted by GSA ADP Schedule Contract with IBM Corp. ";
/**
* A string of eight blank characters.
*/
public final static String EIGHT_BLANKS = " ";
/**
* An EBCDIC blank character with byte value of 0x40.
*/
public final static byte BLANK_EBCDIC = (byte) 0x40;
/**
* An ASCII blank character with byte value of 0x20.
*/
public final static byte BLANK_ASCII = (byte) 0x20;
/**
* A string of forty binary zero characters.
*/
public final static byte[] FORTY_BINARY_ZEROES = { 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00 };
/**
* API client application is an IMS Connect RYO (roll-your-own) application,
* that is an application that interfaces directly to IMS Connect without
* going through IMS TM Resource Adapter or IMS SOAP Gateway.
*/
public final static int CLIENT_TYPE_ICON_RYO = 1;
/**
* Constant with the default value for the TmInteraction
* clientType
property which maps to
* {@link ApiProperties#CLIENT_TYPE_ICON_RYO}.
*/
public final static int DEFAULT_CLIENT_TYPE = CLIENT_TYPE_ICON_RYO;
// Connection properties
/**
* Constant for Connection socketType
property with byte value
* of 0x00.
*
* @see Connection#setSocketType(byte)
* @see Connection#getSocketType()
*/
public final static byte SOCKET_TYPE_TRANSACTION = (byte) 0x00;
/**
* Constant for Connection socketType
property with byte value
* of 0x10.
*
* @see Connection#setSocketType(byte)
* @see Connection#getSocketType()
*/
public final static byte SOCKET_TYPE_PERSISTENT = (byte) 0x10;
/**
* Constant with the default value for the Connection
* socketType
property which maps to
* {@link ApiProperties#SOCKET_TYPE_PERSISTENT}.
*
* @see Connection#setSocketType(byte)
* @see Connection#getSocketType()
*/
public final static byte DEFAULT_SOCKET_TYPE = SOCKET_TYPE_PERSISTENT;
/**
* Constant with the default value for the Connection hostName
* property which maps to "HOSTNAME".
*
* @see Connection#setHostName(String)
* @see Connection#getHostName()
*/
public final static String DEFAULT_HOSTNAME = "HOSTNAME";
/**
* Constant with integer value of 8, indicating the maximum length of a
* valid hostName value.
*/
public final static int HOSTNAME_MAX_LEN = 8;
/**
* Constant with the default value for Connection portNumber
* which maps to 9999.
*
* @see Connection#setPortNumber(int)
* @see Connection#getPortNumber()
*/
public final static int DEFAULT_PORTNUMBER = 9999;
/**
* Constant with the default value for Connection clientId
* property which maps to " " (eight blank characters. A blank
* clientId value triggers to IMS Connect to generate a guaranteed unique
* clientId value to be used for that Connection.
*
* @see Connection#setClientId(String)
* @see Connection#getClientId()
*/
public final static String DEFAULT_CLIENTID = EIGHT_BLANKS;
/**
* Constant with integer value of 8, indicating the maximum length of a
* valid clientId value.
*/
public final static int CLIENTID_MAX_LEN = 8;
/**
* Constant for TmInteraction
* inputMessageDataSegmentsIncludeLlzzAndTrancode
property with
* value of true
.
*/
public final static boolean INPUT_MESSAGE_DATA_SEGMENTS_DO_INCLUDE_LLZZ_AND_TRANCODE = true;
/**
* Constant for TmInteraction
* inputMessageDataSegmentsIncludeLlzzAndTrancode
property with
* value of false
.
*/
public final static boolean INPUT_MESSAGE_DATA_SEGMENTS_DO_NOT_INCLUDE_LLZZ_AND_TRANCODE = false;
/**
* Constant with the default value for TmInteraction
* inputMessageDataSegmentsIncludeLlzzAndTrancode
which maps to
* {@link ApiProperties#INPUT_MESSAGE_DATA_SEGMENTS_DO_NOT_INCLUDE_LLZZ_AND_TRANCODE}
* .
*/
public final static boolean DEFAULT_INPUT_MESSAGE_DATA_SEGMENTS_INCLUDE_LLZZ_AND_TRANCODE = INPUT_MESSAGE_DATA_SEGMENTS_DO_NOT_INCLUDE_LLZZ_AND_TRANCODE;
// Contants that can be used to set imsConnectTimeout, interactionTimeout,
// and/or socketConnectTimeout
/**
* Constant for setting the imsConnectTimeout
,
* interactionTimeout
, and socketConnectTimeout
* property. The constant value is decimal -1. For the imsConnectTimeout
* property, this constant sets an IRM_TIMER value of X'FF'. For the
* interactionTimeout property, the property value is converted internally
* to 0 and used to set the socket SO_TIMEOUT value. For the
* socketConnectTimeout property, this constant causes the API to connect
* without specifying a connection timeout value. The API follows the TCP/IP
* default timeout behavior for connection attempts.
*/
public final static int TIMEOUT_WAIT_FOREVER = -1; // (for
// imsConnectTimeout,
// corresponds to
// IRM_TIMER value of
// X'FF' )
// (for interactionTimeout, converted internally to 0 and used to set
// SO_TIMEOUT value for socket)
// (for socketConnectTimeout, causes API to connect without specifying
// connection timeout value - TCP/IP will use its own default timeout
// behavior for connection attempts)
public final static int TIMEOUT_1_MILLISECOND = 1; // (converted to 10 for
// imsConnectTimeout)
public final static int TIMEOUT_2_MILLISECONDS = 2; // (converted to 10 for
// imsConnectTimeout)
public final static int TIMEOUT_5_MILLISECONDS = 5; // (converted to 10 for
// imsConnectTimeout)
public final static int TIMEOUT_10_MILLISECONDS = 10; // (minimum
// imsConnectTimeout
// value supported
// by IMS Connect)
public final static int TIMEOUT_20_MILLISECONDS = 20;
public final static int TIMEOUT_50_MILLISECONDS = 50;
public final static int TIMEOUT_100_MILLISECONDS = 100;
public final static int TIMEOUT_200_MILLISECONDS = 200;
public final static int TIMEOUT_500_MILLISECONDS = 500;
public final static int TIMEOUT_1_SECOND = 1000;
public final static int TIMEOUT_2_SECONDS = 2000;
public final static int TIMEOUT_5_SECONDS = 5000;
public final static int TIMEOUT_10_SECONDS = 10000;
public final static int TIMEOUT_15_SECONDS = 15000;
public final static int TIMEOUT_30_SECONDS = 30000;
public final static int TIMEOUT_45_SECONDS = 45000;
public final static int TIMEOUT_1_MINUTE = 60000;
public final static int TIMEOUT_2_MINUTES = 120000;
public final static int TIMEOUT_5_MINUTES = 300000;
public final static int TIMEOUT_10_MINUTES = 600000;
public final static int TIMEOUT_15_MINUTES = 900000;
public final static int TIMEOUT_30_MINUTES = 1800000;
public final static int TIMEOUT_45_MINUTES = 2700000;
public final static int TIMEOUT_1_HOUR = 3600000; // (maximum
// imsConnectTimeout
// value supported by
// IMS Connect)
public final static int TIMEOUT_2_HOURS = 7200000; // (not usable for
// imsConnectTimeout)
public final static int TIMEOUT_4_HOURS = 14400000; // (not usable for
// imsConnectTimeout)
public final static int TIMEOUT_8_HOURS = 28800000; // (not usable for
// imsConnectTimeout)
public final static int TIMEOUT_12_HOURS = 43200000; // (not usable for
// imsConnectTimeout)
public final static int TIMEOUT_1_DAY = 86400000; // (not usable for
// imsConnectTimeout)
public final static int TIMEOUT_2_DAYS = 172800000; // (not usable for
// imsConnectTimeout)
public final static int TIMEOUT_5_DAYS = 432000000; // (not usable for
// imsConnectTimeout)
public final static int TIMEOUT_1_WEEK = 604800000; // (not usable for
// imsConnectTimeout)
public final static int TIMEOUT_2_WEEKS = 1209600000; // (not usable for
// imsConnectTimeout)
// Timeout properties used specifically for imsConnectTimeout (timeout value passed to IMS Connect to override IMS Connects TIMEOUT value)
/**
* Constant for imsConnectTimeout
property with a decimal value
* of -23.
*
* @see TmInteraction#setImsConnectTimeout(int)
* @see TmInteraction#getImsConnectTimeout()
*/
public final static int IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_NOWAIT_VALUE = -23; // (corresponds to IRM_TIMER value in message of X'E9'
/**
* Constant for imsConnectTimeout
property with decimal value
* of 0.
*
* @see TmInteraction#setImsConnectTimeout(int)
* @see TmInteraction#getImsConnectTimeout()
*/
public final static int IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_DEFAULT_VALUE = 0;
/**
* Constant with the default value for imsConnectTimeout
which
* maps to
* {@link ApiProperties#IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_DEFAULT_VALUE}.
*
* @see TmInteraction#setImsConnectTimeout(int)
* @see TmInteraction#getImsConnectTimeout()
*/
public final static int DEFAULT_IMS_CONNECT_TIMEOUT = IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_DEFAULT_VALUE;
public final static int DEFAULT_CONVERTED_IMS_CONNECT_TIMEOUT = IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_DEFAULT_VALUE; // internal use only
public final static int DEFAULT_IMS_CONNECT_TIMEOUT_INDEX = IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_DEFAULT_VALUE; // internal use only
// Timeout properties used specifically for interactionTimeout (time that
// API will wait to receive a response from IMS Connect)
/**
* Constant with the default value for interactionTimeout
. Prevents
* interactions from timing out.
*
* @see Connection#setInteractionTimeout(int)
* @see Connection#getInteractionTimeout()
*/
public final static int DEFAULT_INTERACTION_TIMEOUT = -1;
/**
* Constant for interactionTimeout
property with decimal value
* of 2147483647. The maximum timeout value is 2147483647 milliseconds or
* approximately 3.5 weeks.
*
* @see Connection#setInteractionTimeout(int)
* @see Connection#getInteractionTimeout()
*/
public final static int INTERACTION_TIMEOUT_MAX = 2147483647; // (maximum value (approx. 3.5 weeks) other than WAIT_FOREVER)
// Timeout properties used for socketConnectTimeout (time that API will wait for a socket.connect() request to complete)
/**
* Constant for socketConnectTimeout
property with decimal
* value of -1 {@link ApiProperties#TIMEOUT_WAIT_FOREVER}.
*
* @see Connection#setSocketConnectTimeout(int)
* @see Connection#getSocketConnectTimeout()
*/
public final static int SOCKET_CONNECT_TIMEOUT_USE_TCPIP_DEFAULTS = TIMEOUT_WAIT_FOREVER; // API issues connect() call without specifying a timeout
/**
* Constant with the default value for socketConnectTimeout
* which maps to timeout parameter on socket.connect() call unless
* TIMEOUT_WAIT_FOREVER is specified. If TIMEOUT_WAIT_FOREVER (or the
* default value, DEFAULT_SOCKET_CONNECT_TIMEOUT) is specified,
* socket.connect() will be called without a timeout parameter causing the
* TCP/IP to use its own default timeout behavior (attempting to connect 5
* times on Windows (which will take approximately 3 minutes) before
* returning an error message
* {@link ApiProperties#SOCKET_CONNECT_TIMEOUT_USE_TCPIP_DEFAULTS}.
*
* @see Connection#setSocketConnectTimeout(int)
* @see Connection#getSocketConnectTimeout()
*/
public final static int DEFAULT_SOCKET_CONNECT_TIMEOUT = SOCKET_CONNECT_TIMEOUT_USE_TCPIP_DEFAULTS;
/**
* Constant for otmaTransactionExpiration
property with boolean
* value of true
. A value of true will cause IMS Connect and
* OTMA to use the imsConnectTimeout value for the current interaction as
* the transaction expiration timeout value for the current interaction
* instead of the transaction expiration timeout value configured in OTMA.
*
* @see ApiProperties#DO_NOT_EXPIRE_OTMA_TRANSACTION_ON_IMS_CONNECT_TIMEOUT
* @see TmInteraction#isOtmaTransactionExpiration()
* @see TmInteraction#setOtmaTransactionExpiration(boolean)
*/
public final static boolean DO_EXPIRE_OTMA_TRANSACTION_ON_IMS_CONNECT_TIMEOUT = true;
/**
* Constant for otmaTransactionExpiration
property with boolean
* value of false
. A value of false will cause IMS Connect and
* OTMA to use the configured value for the transaction expiration timeout
* value in OTMA instead of the imsConnectTimeout value for the current
* interaction. This is the default value for the
* otmaTransactionExpiration
property.
*
* @see ApiProperties#DO_EXPIRE_OTMA_TRANSACTION_ON_IMS_CONNECT_TIMEOUT
* @see TmInteraction#isOtmaTransactionExpiration()
* @see TmInteraction#setOtmaTransactionExpiration(boolean)
*/
public final static boolean DO_NOT_EXPIRE_OTMA_TRANSACTION_ON_IMS_CONNECT_TIMEOUT = false;
/**
* Constant with the default value for
* otmaTransactionExpiration
property which maps to boolean
* value of false
.
*
* @see ApiProperties#DO_EXPIRE_OTMA_TRANSACTION_ON_IMS_CONNECT_TIMEOUT
* @see ApiProperties#DO_NOT_EXPIRE_OTMA_TRANSACTION_ON_IMS_CONNECT_TIMEOUT
* @see TmInteraction#isOtmaTransactionExpiration()
* @see TmInteraction#setOtmaTransactionExpiration(boolean)
*/
public final static boolean DEFAULT_OTMA_TRANSACTION_EXPIRATION = DO_NOT_EXPIRE_OTMA_TRANSACTION_ON_IMS_CONNECT_TIMEOUT;
/**
* Constant for useCM0AckNoWait
property with boolean value of
* true
. A value of true will cause the API to override the
* IRM_TIMER value on an ACK response to receipt of a CM0 (Commit Mode 0 -
* Commit then Send) response with a value of -23 (0xE9 - IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_NOWAIT_VALUE) and then
* send the ACK without issuing a subsequent receive call to receive the
* timeout response to the ACK message.
*
* @see ApiProperties#DO_NOT_USE_CM0_ACK_NOWAIT
* @see ApiProperties#IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_NOWAIT_VALUE
* @see TmInteraction#isUseCM0AckNoWait()
* @see TmInteraction#setUseCM0AckNoWait(boolean)
*/
public final static boolean DO_USE_CM0_ACK_NOWAIT = true;
/**
* Constant for useCM0AckNoWait
property with boolean value of
* false
. A value of false will cause the API to NOT override
* the IRM_TIMER value on an ACK response to receipt of a CM0 (Commit Mode 0
* - Commit then Send) response and to send the ACK and then issue a
* subsequent receive call to receive the timeout response to the ACK
* message.
*
* @see ApiProperties#DO_USE_CM0_ACK_NOWAIT
* @see ApiProperties#IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_NOWAIT_VALUE
* @see TmInteraction#isUseCM0AckNoWait()
* @see TmInteraction#setUseCM0AckNoWait(boolean)
*/
public final static boolean DO_NOT_USE_CM0_ACK_NOWAIT = false;
/**
* Constant with the default value for useCM0AckNoWait
property
* which maps to boolean value of true
.
*
* @see ApiProperties#DO_USE_CM0_ACK_NOWAIT
* @see ApiProperties#DO_NOT_USE_CM0_ACK_NOWAIT
* @see TmInteraction#isUseCM0AckNoWait()
* @see TmInteraction#setUseCM0AckNoWait(boolean)
*/
public final static boolean DEFAULT_USE_CM0_ACK_NOWAIT = DO_NOT_USE_CM0_ACK_NOWAIT;
/**
* Constant with the value for
* returnDFS2082AfterCM0SendRecvNoResponse
property which maps
* to boolean value of true
.
*
* @see ApiProperties#DO_NOT_RETURN_DFS2082_AFTER_CM0_SENDRECV_NO_RESPONSE
* @see ApiProperties#DEFAULT_RETURN_DFS2082_AFTER_CM0_SENDRECV_NO_RESPONSE
* @see TmInteraction#isReturnDFS2082AfterCM0SendRecvNoResponse()
* @see TmInteraction#setReturnDFS2082AfterCM0SendRecvNoResponse(boolean)
*/
public final static boolean DO_RETURN_DFS2082_AFTER_CM0_SENDRECV_NO_RESPONSE = true;
/**
* Constant with the value for
* returnDFS2082AfterCM0SendRecvNoResponse
property which maps
* to boolean value of false
.
*
* @see ApiProperties#DO_RETURN_DFS2082_AFTER_CM0_SENDRECV_NO_RESPONSE
* @see ApiProperties#DEFAULT_RETURN_DFS2082_AFTER_CM0_SENDRECV_NO_RESPONSE
* @see TmInteraction#isReturnDFS2082AfterCM0SendRecvNoResponse()
* @see TmInteraction#setReturnDFS2082AfterCM0SendRecvNoResponse(boolean)
*/
public final static boolean DO_NOT_RETURN_DFS2082_AFTER_CM0_SENDRECV_NO_RESPONSE = false;
/**
* Constant with the default value for
* returnDFS2082AfterCM0SendRecvNoResponse
property which maps
* to boolean value of false
.
*
* @see ApiProperties#DO_RETURN_DFS2082_AFTER_CM0_SENDRECV_NO_RESPONSE
* @see ApiProperties#DO_NOT_RETURN_DFS2082_AFTER_CM0_SENDRECV_NO_RESPONSE
* @see TmInteraction#isReturnDFS2082AfterCM0SendRecvNoResponse()
* @see TmInteraction#setReturnDFS2082AfterCM0SendRecvNoResponse(boolean)
*/
public final static boolean DEFAULT_RETURN_DFS2082_AFTER_CM0_SENDRECV_NO_RESPONSE = DO_NOT_RETURN_DFS2082_AFTER_CM0_SENDRECV_NO_RESPONSE;
// Target IMS properties
/**
* Constant with the default value for TmInteraction
* imsDatastoreName
which maps to String
value
* "MYDSTRNM".
*
* @see TmInteraction#setImsDatastoreName(String)
* @see TmInteraction#getImsDatastoreName()
*/
public final static String DEFAULT_IMS_DATASTORE_NAME = "MYDSTRNM";
/**
* Constant with value 8, indicating the maximum number of characters of a
* valid TmInteraction imsDatastoreName
field.?
*/
public final static int MAX_LEN_IMS_DATASTORE_NAME = 8;
/**
* Constant with the default value for TmInteraction trancode
* which maps to String
value "TRANCODE".
*
* @see TmInteraction#setTrancode(String)
* @see TmInteraction#getTrancode()
*/
public final static String DEFAULT_TRANCODE = "TRANCODE";
/**
* Constant with value 8, indicating the maximum number of characters of a
* valid TmInteraction trancode
field.??
*/
public final static int MAX_LEN_TRANCODE = 8;
/**
* Constant for TmInteraction
* imsConnectUserMessageExitIdentifier
property with
* String
value of "*SAMPLE*".
*
* @see TmInteraction#setImsConnectUserMessageExitIdentifier(String)
* @see TmInteraction#getImsConnectUserMessageExitIdentifier()
*/
public final static String IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSSMPL0 = "*SAMPLE*";
/**
* Constant for TmInteraction
* imsConnectUserMessageExitIdentifier
property with
* String
value of "*SAMPL1*".
*
* @see TmInteraction#setImsConnectUserMessageExitIdentifier(String)
* @see TmInteraction#getImsConnectUserMessageExitIdentifier()
*/
public final static String IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSSMPL1 = "*SAMPL1*";
/**
* Constant for TmInteraction
* imsConnectUserMessageExitIdentifier
property with
* String
value of "*HWSCSL*".
*
* @see TmInteraction#setImsConnectUserMessageExitIdentifier(String)
* @see TmInteraction#getImsConnectUserMessageExitIdentifier()
*/
public final static String IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSCSLO0 = "*HWSCSL*";
/**
* Constant for TmInteraction
* imsConnectUserMessageExitIdentifier
property with
* String
value of "*HWSCS1*".
*
* @see TmInteraction#setImsConnectUserMessageExitIdentifier(String)
* @see TmInteraction#getImsConnectUserMessageExitIdentifier()
*/
public final static String IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSCSLO1 = "*HWSCS1*";
/**
* Constant with the default value for TmInteraction
* imsConnectUserMessageExitIdentifier
which maps to
* {@link ApiProperties#IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSSMPL1}
* .
*
* @see TmInteraction#setImsConnectUserMessageExitIdentifier(String)
* @see TmInteraction#getImsConnectUserMessageExitIdentifier()
*/
public final static String DEFAULT_IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER = IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSSMPL1;
/**
* Constant with the default value for TmInteraction
* imsConnectUserMessageExitIdentifier
which maps to
* {@link ApiProperties#IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSSMPL1}
* .
*
* @see TmInteraction#setImsConnectUserMessageExitIdentifier(String)
* @see TmInteraction#getImsConnectUserMessageExitIdentifier()
*/
public final static String DEFAULT_IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_TYPE2CMD = IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSCSLO1;
public final static String IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSDPWR1 = "*HWSDP1*";
// TmInteraction properties
/*
* Constant for TmInteraction architectureLevel
.
*/
public final static byte ARCH_LEVEL_MIN_VALUE = (byte) 0x00;
/*
* Constant for TmInteraction architectureLevel
.
*/
public final static byte ARCH_LEVEL_MAX_VALUE = (byte) 0x03;
public final static String ARCH_LEVEL_NAME[] = { "ARCH_LEVEL_BASE",
"ARCH_LEVEL_REROUTE", "ARCH_LEVEL_ATM" };
/*
* Constant for TmInteraction architectureLevel
. Used
* internally only.
*/
public final static byte ARCH_LEVEL_ATM = (byte) 0x02;
public final static byte ARCH_LEVEL_SYNC_CALLOUT = (byte) 0x03;
/*
* Constant for TmInteraction architectureLevel
. The default
* value maps to {@link ApiProperties#ARCH_LEVEL_ATM}. Used internally only.
*/
public final static byte DEFAULT_ARCH_LEVEL = ARCH_LEVEL_SYNC_CALLOUT;
public final static short PROTOCOL_LEVEL_BASE = (short) 0x00;
public final static short PROTOCOL_LEVEL_ = (short) 0x01; // not used
public final static short PROTOCOL_LEVEL_CM0_ACK_NOWAIT_SUPPORT = (short) 0x02;
/**
* Constant for TmInteraction ackNakProvider
property with byte
* value of 0x00, indicating that the API will send an ACK on behalf of the
* client whenever IMS Connect returns an ACK_NAK_NEEDED response .
*
* @see TmInteraction#setAckNakProvider(byte)
* @see TmInteraction#getAckNakProvider()
*/
public final static byte API_INTERNAL_ACK = 0x00;
/**
* Constant for TmInteraction ackNakProvider
property with byte
* value of 0x01, indicating that the client application must submit an
* acknowledgement interaction when IMS Connect returns ACK_NAK_NEEDED.
*
* @see TmInteraction#setAckNakProvider(byte)
* @see TmInteraction#getAckNakProvider()
*/
public final static byte CLIENT_ACK_NAK = 0x01;
/**
* Constant with the default value for TmInteraction
* ackNakProvider
which maps to
* {@link ApiProperties#API_INTERNAL_ACK}.
*
* @see TmInteraction#setAckNakProvider(byte)
* @see TmInteraction#getAckNakProvider()
*/
public final static byte DEFAULT_ACK_NAK_PROVIDER = API_INTERNAL_ACK;
/**
* Constant for TmInteraction inputMessageOptions
property with
* byte value of 0x00.
*/
public final static int INPUT_MESSAGE_OPTIONS_NO_OPTIONS = 0x00;
/**
* Constant for TmInteraction inputMessageOptions
property with
* byte value of 0x40.
*
* @see TmInteraction#setInputMessageOptions(int)
* @see TmInteraction#getInputMessageOptions()
*/
public final static int INPUT_MESSAGE_OPTIONS_EBCDIC = 0x40;
/**
* Constant with the default value for TmInteraction
* inputMessageOptions
which maps to
* {@link ApiProperties#INPUT_MESSAGE_OPTIONS_NO_OPTIONS}.
*
* @see TmInteraction#setInputMessageOptions(int)
* @see TmInteraction#getInputMessageOptions()
*/
public final static int DEFAULT_INPUT_MESSAGE_OPTIONS = INPUT_MESSAGE_OPTIONS_NO_OPTIONS;
/**
* Constant for TmInteraction resumeTpipeProcessing
property
* with byte value of 0x01.
*
* @see TmInteraction#setResumeTpipeProcessing(int)
* @see TmInteraction#getResumeTpipeProcessing()
*/
public final static int RESUME_TPIPE_SINGLE_NOWAIT = 0x01;
/**
* Constant for TmInteraction resumeTpipeProcessing
property
* with byte value of 0x02.
*
* @see TmInteraction#setResumeTpipeProcessing(int)
* @see TmInteraction#getResumeTpipeProcessing()
*/
public final static int RESUME_TPIPE_AUTO = 0x02;
/**
* Constant for TmInteraction resumeTpipeProcessing
property
* with byte value of 0x04.
*
* @see TmInteraction#setResumeTpipeProcessing(int)
* @see TmInteraction#getResumeTpipeProcessing()
*/
public final static int RESUME_TPIPE_NOAUTO = 0x04;
/**
* Constant for TmInteraction resumeTpipeProcessing
property
* with byte value of 0x10.
*
* @see TmInteraction#setResumeTpipeProcessing(int)
* @see TmInteraction#getResumeTpipeProcessing()
*/
public final static int RESUME_TPIPE_SINGLE_WAIT = 0x10;
/**
* Constant with the default value for TmInteraction
* resumeTpipeProcessing
which maps to
* {@link ApiProperties#RESUME_TPIPE_SINGLE_WAIT}.
*
* @see TmInteraction#setResumeTpipeProcessing(int)
* @see TmInteraction#getResumeTpipeProcessing()
*/
public final static int DEFAULT_RESUME_TPIPE_PROCESSING = RESUME_TPIPE_SINGLE_WAIT;
/**
* Constant for TmInteraction returnMfsModname
property with
* value of true
.
*
* @see TmInteraction#setReturnMfsModname(boolean)
* @see TmInteraction#isReturnMfsModname()
*/
public final static boolean DO_RETURN_MFS_MODNAME = true;
/**
* Constant for TmInteraction returnMfsModname
property with
* value of false
.
*
* @see TmInteraction#setReturnMfsModname(boolean)
* @see TmInteraction#isReturnMfsModname()
*/
public final static boolean DO_NOT_RETURN_MFS_MODNAME = false;
/**
* Constant with the default value for TmInteraction
* returnMfsModname
which maps to
* {@link ApiProperties#DO_NOT_RETURN_MFS_MODNAME}.
*
* @see TmInteraction#setReturnMfsModname(boolean)
* @see TmInteraction#isReturnMfsModname()
*/
public final static boolean DEFAULT_RETURN_MFS_MODNAME = DO_NOT_RETURN_MFS_MODNAME;
/**
* Constant for TmInteraction commitMode
field, with byte value
* 0x40, indicating commit-then-send mode.
*
* @see TmInteraction#setCommitMode(byte)
* @see TmInteraction#getCommitMode()
*/
public final static byte COMMIT_MODE_0 = (byte) 0x40;
/**
* Constant for TmInteraction commitMode
field, with byte value
* 0x20, indicating send-then-commit mode.
*
* @see TmInteraction#setCommitMode(byte)
* @see TmInteraction#getCommitMode()
*/
public final static byte COMMIT_MODE_1 = (byte) 0x20;
/**
* Constant with the default value for TmInteraction commitMode
* which maps to {@link ApiProperties#COMMIT_MODE_0}.
*
* @see TmInteraction#setCommitMode(byte)
* @see TmInteraction#getCommitMode()
*/
public final static byte DEFAULT_COMMIT_MODE = COMMIT_MODE_0;
/**
* Constant for TmInteraction cm0IgnorePurge field, with boolean value
* true
, indicating that IMS should ignore DL/I PURG calls
* until all segments of a multi-segment output message have been inserted
* to the TP PCB. This property is useful only if the transaction is run
* with Commit Mode 0, and the IMS application issues a DL/I PURG call for
* each segment of a multi-segment output message that it inserts to TP PCB.
*
* From the perspective of the client, multi-segment output is returned as a
* single, multiple-segment message rather than as multiple, single-segment
* messages. This behavior matches the behavior of the same application run
* with Commit Mode 1 and is useful when switching from an existing
* application using CM1 to CM0.
*
* @see TmInteraction#setCm0IgnorePurge(boolean)
* @see TmInteraction#isCm0IgnorePurge()
*/
public final static boolean CM0_DO_IGNORE_PURGE = true;
/**
* Constant for TmInteraction cm0IgnorePurge field, with boolean value
* false
, indicating that IMS should accept all DL/I PURG calls
* after a segment is inserted to the TP PCB. This is the default behavior
* for a transaction that is running under Commit Mode 0. This property is
* useful only if the transaction is run with Commit Mode 0 and the IMS
* application issues a DL/I PURG call for each segment of a multi-segment
* output message that it inserts to the TP PCB.
*
* From the perspective of the client, multi-segment output is returned as
* multiple, single-segment messages rather than as a single,
* multiple-segment message, as would be the case for a application that is
* running with Commit Mode 1.
*
* @see TmInteraction#setCm0IgnorePurge(boolean)
* @see TmInteraction#isCm0IgnorePurge()
*/
public final static boolean CM0_DO_NOT_IGNORE_PURGE = false;
/**
* Constant with the default value for TmInteraction
* cm0IgnorePurge
which maps to
* {@link ApiProperties#CM0_DO_NOT_IGNORE_PURGE}.
*
* @see TmInteraction#setCm0IgnorePurge(boolean)
* @see TmInteraction#isCm0IgnorePurge()
*/
public final static boolean DEFAULT_CM0_IGNORE_PURGE = CM0_DO_NOT_IGNORE_PURGE;
/**
* Constant for TmInteraction cancelClientId field, with boolean value
* true
, indicating that if there is another connection with the same client ID
* that connection should be closed and the requesting connection should be granted.
*
* @see TmInteraction#setCancelClientId(boolean)
* @see TmInteraction#isCancelClientId()
*/
public final static boolean DO_CANCEL_CLIENTID = true;
/**
* Constant for TmInteraction cancelClientId field, with boolean value
* false
, indicating that if there is another connection
* with the same client ID that connection should be kept live
* and the current request denied.
*
* @see TmInteraction#setCancelClientId(boolean)
* @see TmInteraction#isCancelClientId()
*/
public final static boolean DO_NOT_CANCEL_CLIENTID = false;
/**
* Constant with the default value for TmInteraction cancelClientId
* which maps to {@link ApiProperties#DO_CANCEL_CLIENTID}.
*
* @see TmInteraction#setCancelClientId(boolean)
* @see TmInteraction#isCancelClientId()
*/
public final static boolean DEFAULT_CANCEL_CLIENTID = DO_CANCEL_CLIENTID;
/**
* Constant for TmInteraction imsConnectGenerateClientId field, with boolean value
* true
, indicating to IMS Connect to generate the clientId for the connection.
* back to the client.
* @see TmInteraction#setImsConnectGenerateClientId(boolean)
* @see TmInteraction#isImsConnectGenerateClientId()
*/
public final static boolean DO_GENERATE_CLIENTID = true;
/**
* Constant for TmInteraction imsConnectGenerateClientId field, with boolean value
* false
, indicating to IMS Connect not to generate the client Id for the connection.
*
* @see TmInteraction#setImsConnectGenerateClientId(boolean)
* @see TmInteraction#isImsConnectGenerateClientId()
*/
public final static boolean DO_NOT_GENERATE_CLIENTID = false;
/**
* Constant with the default value for TmInteraction imsConnectGenerateClientId
,
* which maps to {@link ApiProperties#DO_NOT_GENERATE_CLIENTID}.
*
* @see TmInteraction#setImsConnectGenerateClientId(boolean)
* @see TmInteraction#isImsConnectGenerateClientId()
*/
public final static boolean DEFAULT_IMS_CONNECT_GENERATE_CLIENTID = DO_NOT_GENERATE_CLIENTID;
/**
* Constant for TmInteraction returnClientId field, with boolean value
* true
, indicating to return the clientId as part of the output message
* back to the client.
* @see TmInteraction#setReturnClientId(boolean)
* @see TmInteraction#isReturnClientId()
*/
public final static boolean DO_RETURN_CLIENTID = true;
/**
* Constant for TmInteraction returnClientId field, with boolean value
* false
, indicating to IMS Connect not to return the client ID of the connection in output.
*
* @see TmInteraction#setReturnClientId(boolean)
* @see TmInteraction#isreturnClientId()
*/
public final static boolean DO_NOT_RETURN_CLIENTID = false;
/**
* Constant with the default value for TmInteraction returnClientId
,
* which maps to {@link ApiProperties#DO_NOT_RETURN_CLIENTID}.
*
* @see TmInteraction#setReturnClientId(boolean)
* @see TmInteraction#isReturnClientId()
*/
public final static boolean DEFAULT_RETURN_CLIENTID = DO_NOT_RETURN_CLIENTID;
/**
* Constant for TmInteraction syncLevel
with byte value 0x00.
*
* @see TmInteraction#setSyncLevel(byte)
* @see TmInteraction#getSyncLevel()
*/
public final static byte SYNC_LEVEL_NONE = (byte) 0x00;
/**
* Constant for TmInteraction syncLevel
with byte value 0x01.
*
* @see TmInteraction#setSyncLevel(byte)
* @see TmInteraction#getSyncLevel()
*/
public final static byte SYNC_LEVEL_CONFIRM = (byte) 0x01;
/**
* Constant for TmInteraction syncLevel
with byte value 0x02.
*
* @see TmInteraction#setSyncLevel(byte)
* @see TmInteraction#getSyncLevel()
*/
public final static byte SYNC_LEVEL_SYNCPT = (byte) 0x02;
/**
* Constant with the default value for TmInteraction syncLevel
* which maps to {@link ApiProperties#SYNC_LEVEL_CONFIRM}.
*
* @see TmInteraction#setSyncLevel(byte)
* @see TmInteraction#getSyncLevel()
*/
public final static byte DEFAULT_SYNC_LEVEL = SYNC_LEVEL_CONFIRM;
/**
* Constant for TmInteraction interactionTypeDescription
with
* String
value "ACK".
*
* @see TmInteraction#setInteractionTypeDescription(String)
* @see TmInteraction#getInteractionTypeDescription()
*/
public final static String INTERACTION_TYPE_DESC_ACK = "ACK"; // maps to IRM_F4 = 'A';
/**
* Constant for TmInteraction interactionTypeDescription
with
* String
value "CANCELTIMER".
*
* @see TmInteraction#setInteractionTypeDescription(String)
* @see TmInteraction#getInteractionTypeDescription()
*/
public final static String INTERACTION_TYPE_DESC_CANCELTIMER = "CANCELTIMER"; // maps to IRM_F4 = 'C';
/**
* Constant for TmInteraction interactionTypeDescription
with
* String
value "ENDCONVERSATION".
*
* @see TmInteraction#setInteractionTypeDescription(String)
* @see TmInteraction#getInteractionTypeDescription()
*/
public final static String INTERACTION_TYPE_DESC_ENDCONVERSATION = "ENDCONVERSATION"; // maps to IRM_F4 = 'D'
/**
* Constant for TmInteraction interactionTypeDescription
with
* String
value "RECEIVE".
*
* @see TmInteraction#setInteractionTypeDescription(String)
* @see TmInteraction#getInteractionTypeDescription()
*/
public final static String INTERACTION_TYPE_DESC_RECEIVE = "RECEIVE"; // "maps" to TCP?IP receive - no IRM involved
/**
* Constant for TmInteraction interactionTypeDescription
with
* String
value "SENDONLYACK".
*
* @see TmInteraction#setInteractionTypeDescription(String)
* @see TmInteraction#getInteractionTypeDescription()
*/
public final static String INTERACTION_TYPE_DESC_SENDONLYACK = "SENDONLYACK"; // maps to IRM_F4 = 'K'
/**
* Constant for TmInteraction interactionTypeDescription
with
* String
value "NAK".
*
* @see TmInteraction#setInteractionTypeDescription(String)
* @see TmInteraction#getInteractionTypeDescription()
*/
public final static String INTERACTION_TYPE_DESC_NAK = "NAK"; // maps to IRM_F4 = 'N'
/**
* Constant for TmInteraction interactionTypeDescription
with
* String
value "SENDONLYXCFORDDLV".
*
* @see TmInteraction#setInteractionTypeDescription(String)
* @see TmInteraction#getInteractionTypeDescription()
*/
public final static String INTERACTION_TYPE_DESC_SENDONLYXCFORDEREDDELIVERY = "SENDONLYXCFORDDLV"; // maps to IRM_F4 ='S' + IMR_F3 = 0x10
/**
* Constant for TmInteraction interactionTypeDescription
with
* String
value "RESUMETPIPE".
*
* @see TmInteraction#setInteractionTypeDescription(String)
* @see TmInteraction#getInteractionTypeDescription()
*/
public final static String INTERACTION_TYPE_DESC_RESUMETPIPE = "RESUMETPIPE"; // maps to IRM_F4 = 'R'
/**
* Constant for TmInteraction interactionTypeDescription
with
* String
value "SENDONLY".
*
* @see TmInteraction#setInteractionTypeDescription(String)
* @see TmInteraction#getInteractionTypeDescription()
*/
public final static String INTERACTION_TYPE_DESC_SENDONLY = "SENDONLY"; // maps to IRM_F4 = 'S'
/**
* Constant for TmInteraction interactionTypeDescription
with
* String
value "SENDRECV".
*
* @see TmInteraction#setInteractionTypeDescription(String)
* @see TmInteraction#getInteractionTypeDescription()
*/
public final static String INTERACTION_TYPE_DESC_SENDRECV = "SENDRECV"; // maps to IRM_F4 = ' ' (single blank character)
/**
* Constant for TmInteraction interactionTypeDescription
with
* String
value "SENDONLYCALLOUTRESPONSE".
*
* @see TmInteraction#setInteractionTypeDescription(String)
*/
public final static String INTERACTION_TYPE_DESC_SENDONLY_CALLOUT_RESPONSE = "SENDONLYCALLOUTRESPONSE"; // maps
/**
* Constant for TmInteraction interactionTypeDescription
with
* String
value "SENDONLYACKCALLOUTRESPONSE".
*
* @see TmInteraction#setInteractionTypeDescription(String)
*/
public final static String INTERACTION_TYPE_DESC_SENDONLYACK_CALLOUT_RESPONSE = "SENDONLYACKCALLOUTRESPONSE"; // maps to IRM_F4= 'L'
/**
* Constant for TmInteraction interactionTypeDescription
with
* String
value "TYPE2CMD".
*
* @see TmInteraction#setInteractionTypeDescription(String)
* @see TmInteraction#getInteractionTypeDescription()
*/
public final static String INTERACTION_TYPE_DESC_TYPE2_COMMAND = "TYPE2CMD"; // maps to IRM_F4 = ' ' (single blank character for sendrecv interaction)
/**
* Constant with the default value for TmInteraction
* interactionTypeDescription
which maps to
* {@link ApiProperties#INTERACTION_TYPE_DESC_SENDRECV}.
*
* @see TmInteraction#setInteractionTypeDescription(String)
* @see TmInteraction#getInteractionTypeDescription()
*/
public final static String DEFAULT_INTERACTION_TYPE_DESC = INTERACTION_TYPE_DESC_SENDRECV;
/**
* Constant with the default value for TmInteraction
* ltermOverrideName
which maps to a String
of
* eight blank characters.
*
* @see TmInteraction#setLtermOverrideName(String)
* @see TmInteraction#getLtermOverrideName()
*/
public final static String DEFAULT_LTERM_OVERRIDE_NAME = EIGHT_BLANKS;
/**
* Constant with value 8, indicating the maximum number of characters of a
* valid TmInteraction ltermOverrideName
field.??
*/
public final static int MAX_LEN_LTERM_OVERRIDE_NAME = 8;
/**
* Constant with value 8, indicating the maximum number of characters of a
* valid TmInteraction imsConnectUserMessageExitIdentifier
* field.??
*/
public final static int MAX_LEN_IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER = 8;
// IMS Connect XML properties - provided in this release for possible future support
public final static int XML_ADAPTER_TYPE_MIN_VALUE = 0;
public final static int XML_ADAPTER_TYPE_NONE = 0;
public final static int XML_ADAPTER_TYPE_COBOL = 1; // support this only
public final static int XML_ADAPTER_TYPE_RYO = 2;
public final static int XML_ADAPTER_TYPE_MFS = 3;
public final static int DEFAULT_XML_ADAPTER_TYPE = XML_ADAPTER_TYPE_COBOL;
public final static int XML_ADAPTER_TYPE_MAX_VALUE = 1;
public final static String XML_ADAPTER_TYPE_NAME[] = { "ADAPTER_TYPE_NONE",
"ADAPTER_TYPE_COBOL", "ADAPTER_TYPE_RYO", "ADAPTER_TYPE_MFS",
"ADAPTER_TYPE_UNKNOWN" };
public final static String DEFAULT_XML_ADAPTER_NAME = "HWSXMLA0";
public final static int XML_ADAPTER_NAME_MAX_LEN = 8;
public final static byte XML_MESSAGE_TYPE_NONE = (byte) 0x00;
public final static byte DEFAULT_XML_MESSAGE_TYPE = XML_MESSAGE_TYPE_NONE;
public final static String DEFAULT_XML_CONVERTER_NAME = "HWSXCNV0";
public final static int XML_CONVERTER_NAME_MAX_LEN = 8;
// Message routing properties
/**
* Constant for TmInteraction purgeUndeliverableOutput
with
* value true
.
*
* @see TmInteraction#setPurgeUndeliverableOutput(boolean)
* @see TmInteraction#isPurgeUndeliverableOutput()
*/
public final static boolean PURGE_UNDELIVERABLE_OUTPUT = true;
/**
* Constant for TmInteraction purgeUndeliverableOutput
field
* with value false
.
*
* @see TmInteraction#setPurgeUndeliverableOutput(boolean)
* @see TmInteraction#isPurgeUndeliverableOutput()
*/
public final static boolean DO_NOT_PURGE_UNDELIVERABLE_OUTPUT = false;
/**
* Constant with the default value for TmInteraction
* purgeUndeliverableOutput
which maps to
* {@link ApiProperties#DO_NOT_PURGE_UNDELIVERABLE_OUTPUT}.
*
* @see TmInteraction#setPurgeUndeliverableOutput(boolean)
* @see TmInteraction#isPurgeUndeliverableOutput()
*/
public final static boolean DEFAULT_PURGE_UNDELIVERABLE_OUTPUT = DO_NOT_PURGE_UNDELIVERABLE_OUTPUT;
/**
* Constant for TmInteraction rerouteName
with value of eight
* blank characters.
*
* @see TmInteraction#setRerouteName(String)
* @see TmInteraction#getRerouteName()
*/
public final static String REROUTE_NAME_NONE = EIGHT_BLANKS;
/**
* Constant for TmInteraction rerouteName
with value of
* "HWS$DLQ".
*
* @see TmInteraction#setRerouteName(String)
* @see TmInteraction#getRerouteName()
*/
public final static String REROUTE_NAME_IMS_CONNECT_DEAD_LETTER_QUEUE = "HWS$DLQ";
/**
* Constant with the default value for TmInteraction
* rerouteName
field which maps to a String
of 8
* blank characters.
*
* @see TmInteraction#setRerouteName(String)
* @see TmInteraction#getRerouteName()
*/
public final static String DEFAULT_REROUTE_NAME = REROUTE_NAME_NONE;
/**
* Constant with value 8, indicating the maximum number of characters of a
* valid TmInteraction rerouteName
field.??
*/
public final static int MAX_LEN_REROUTE_NAME = 8;
/**
* Constant for TmInteraction rerouteUndeliverableOutput
with
* value true
.
*
* @see TmInteraction#setRerouteUndeliverableOutput(boolean)
* @see TmInteraction#isRerouteUndeliverableOutput()
*/
public final static boolean REROUTE_UNDELIVERABLE_OUTPUT = true;
/**
* Constant for TmInteraction rerouteUndeliverableOutput
with
* value false
.
*
* @see TmInteraction#setRerouteUndeliverableOutput(boolean)
* @see TmInteraction#isRerouteUndeliverableOutput()
*/
public final static boolean DO_NOT_REROUTE_UNDELIVERABLE_OUTPUT = false;
/**
* Constant with the default value for TmInteraction
* rerouteUndeliverableOutput
which maps to
* {@link ApiProperties#DO_NOT_REROUTE_UNDELIVERABLE_OUTPUT}.
*
* @see TmInteraction#setRerouteUndeliverableOutput(boolean)
* @see TmInteraction#isRerouteUndeliverableOutput()
*/
public final static boolean DEFAULT_REROUTE_UNDELIVERABLE_OUTPUT = DO_NOT_REROUTE_UNDELIVERABLE_OUTPUT;
/**
* Constant with the default value for TmInteraction
* resumeTpipeAlternateClientId
which maps to a
* String
of eight blank characters.
*
* @see TmInteraction#setResumeTpipeAlternateClientId(String)
* @see TmInteraction#getResumeTpipeAlternateClientId()
*/
public final static String DEFAULT_RESUMETPIPE_ALTERNATE_CLIENTID = EIGHT_BLANKS;
/**
* Constant with value 8, indicating the maximum number of characters of a
* valid TmInteraction resumeTpipeAlternateClientId
field.??
*/
public final static int MAX_LEN_RESUMETPIPE_ALTERNATE_CLIENTID = 8;
// Security properties
/**
* Constant with the default value for the TmInteraction
* racfUserId
property which maps to "RACFUID".
*
* @see TmInteraction#setRacfUserId(String)
* @see TmInteraction#getRacfUserId()
*/
public final static String DEFAULT_RACF_USERID = "RACFUID";
/**
* Constant with value 8, indicating the maximum number of characters of a
* valid TmInteraction racfUserId
field.??
*/
public final static int MAX_LEN_RACF_USERID = 8;
/**
* Constant with the default value for the TmInteraction
* racfGroupName
field which maps to "RACFGRUP".
*
* @see TmInteraction#setRacfGroupName(String)
* @see TmInteraction#getRacfGroupName()
*/
public final static String DEFAULT_RACF_GROUP_NAME = "RACFGRUP";
/**
* Constant with value 8, indicating the maximum number of characters of a
* valid TmInteraction racfGroupName
field.??
*/
public final static int MAX_LEN_RACF_GROUP_NAME = 8;
/**
* Constant with the default value for the TmInteraction
* racfPassword
field which maps to "RACFPSWD".
*
* @see TmInteraction#setRacfPassword(String)
* @see TmInteraction#getRacfPassword()
*/
public final static String DEFAULT_RACF_PASSWORD = "RACFPSWD";
/**
* Constant with value 8, indicating the maximum number of characters of a
* valid TmInteraction racfPassword
field.??
*/
public final static int MAX_LEN_RACF_PASSWORD = 8;
/**
* Constant with the default value for the TmInteraction
* racfApplName
field which maps to "RACFAPNM".
*
* @see TmInteraction#setRacfApplName(String)
* @see TmInteraction#getRacfApplName()
*/
public final static String DEFAULT_RACF_APPL_NAME = "RACFAPNM";
/**
* Constant with value 8, indicating the maximum number of characters of a
* valid TmInteraction racfApplName
field.
*/
public final static int MAX_LEN_RACF_APPL_NAME = 8;
/**
* Constant for Connection useSslConnection
with value
* true
.
*
* @see Connection#isUseSslConnection()
* @see Connection#setUseSslConnection(boolean)
*/
public static final boolean USE_SSL_CONNECTION = true;
/**
* Constant for Connection useSslConnection
with value
* false
.
*
* @see Connection#isUseSslConnection()
* @see Connection#setUseSslConnection(boolean)
*/
public static final boolean DO_NOT_USE_SSL_CONNECTION = false;
/**
* Constant with the default value for the Connection
* useSslConnection
field which maps to
* {@link ApiProperties#DO_NOT_USE_SSL_CONNECTION}.
*
* @see Connection#isUseSslConnection()
* @see Connection#setUseSslConnection(boolean)
*/
public static final boolean DEFAULT_USE_SSL_CONNECTION = DO_NOT_USE_SSL_CONNECTION;
/**
* Constant with the default value for the Connection
* sslKeystoreInputStream
field which maps to null.
*
* @see Connection#setSslKeystoreInputStream(InputStream)
* @see Connection#getSslKeystoreInputStream()
*/
public static final InputStream DEFAULT_SSL_KEYSTORE_INPUT_STREAM = null;
/**
* Constant with the default value for the Connection
* sslKeystoreUrl
field which maps to null.
*
* @see Connection#setSslKeystoreUrl(java.net.URL)
* @see Connection#getSslKeystoreUrl()
*/
public static final URL DEFAULT_SSL_KEYSTORE_URL = null;
/**
* Constant with the default value for the Connection
* sslKeystoreName
field which maps to
* "c:\\MySSLKeystores\\myKeystore.ks".
*
* @see Connection#setSslKeystoreName(String)
* @see Connection#getSslKeystoreName()
*/
//public static final String DEFAULT_SSL_KEYSTORE_NAME = "c:\\MySSLKeystores\\myKeystore.ks";
public static final String DEFAULT_SSL_KEYSTORE_NAME = System.getProperty("java.home") +System.getProperty("file.separator")+ "lib" + System.getProperty("file.separator") + "security" + System.getProperty("file.separator") +"cacerts";
/**
* Constant with the default value for the Connection
* sslKeystorePassword
field which maps to "keystrPw".
*
* @see Connection#setSslKeystorePassword(String)
* @see Connection#getSslKeystorePassword()
*/
public static final String DEFAULT_SSL_KEYSTORE_PASSWORD = "keystrPw";
/**
* Constant with the default value for the Connection
* sslTruststoreInputStream
field which maps to null.
*
* @see Connection#setSslTruststoreInputStream(InputStream)
* @see Connection#getSslTruststoreInputStream()
*/
public static final InputStream DEFAULT_SSL_TRUSTSTORE_INPUT_STREAM = null;
/**
* Constant with the default value for the Connection
* sslTruststoreUrl
field which maps to null.
*
* @see Connection#setSslTruststoreUrl(URL)
* @see Connection#getSslTruststoreUrl()
*/
public static final URL DEFAULT_SSL_TRUSTSTORE_URL = null;
/**
* Constant with the default value for the Connection
* sslTruststoreName
field which maps to
* "c:\\MySSLTruststores\\myTruststore.ks".
*
* @see Connection#setSslTruststoreName(String)
* @see Connection#getSslTruststoreName()
*/
//public static final String DEFAULT_SSL_TRUSTSTORE_NAME = "c:\\MySSLTruststores\\myTruststore.ks";
public static final String DEFAULT_SSL_TRUSTSTORE_NAME = System.getProperty("java.home") +System.getProperty("file.separator")+ "lib" + System.getProperty("file.separator") + "security" + System.getProperty("file.separator") +"cacerts";
/**
* Constant with the default value for the Connection
* sslTruststorePassword
field which maps to "trststPw".
*
* @see Connection#setSslTruststorePassword(String)
* @see Connection#getSslTruststorePassword()
*/
public static final String DEFAULT_SSL_TRUSTSTORE_PASSWORD = "trststPw";
/**
* Constant for the Connection sslEncryptionType
property with
* byte value of 0x00.
*
* @see Connection#setSslEncryptionType(byte)
* @see Connection#getSslEncryptionType()
*/
public static final byte SSL_ENCRYPTIONTYPE_NONE = (byte) 0x00;
/**
* Constant for the Connection sslEncryptionType
property with
* byte value of 0x01.
*
* @see Connection#setSslEncryptionType(byte)
* @see Connection#getSslEncryptionType()
*/
public static final byte SSL_ENCRYPTIONTYPE_WEAK = (byte) 0x01;
/**
* Constant for the Connection sslEncryptionType
property with
* byte value of 0x02.
*
* @see Connection#setSslEncryptionType(byte)
* @see Connection#getSslEncryptionType()
*/
public static final byte SSL_ENCRYPTIONTYPE_STRONG = (byte) 0x02;
/**
* Constant with the default value for the Connection
* sslEncryptionType
field which maps to
* {@link ApiProperties#SSL_ENCRYPTIONTYPE_WEAK}.
*
* @see Connection#setSslEncryptionType(byte)
* @see Connection#getSslEncryptionType()
*/
public static final byte DEFAULT_SSL_ENCRYPTIONTYPE = SSL_ENCRYPTIONTYPE_WEAK;
/**
* Constant with value 256, indicating the maximum number of characters for
* the SSLKeyStorePassword
and
* SSLTrustStorePassword
property. Currently the maximum is 256
* characters to accomodate possible future use of passphrases.
*/
public static final int MAX_SSLSTOREPASSWORD_LEN = 256;
/* STORE_TYPE and CERT_TYPE values are used internally only */
public static final String SSL_STORE_TYPE_JKS = "JKS";
public static final String SSL_STORE_TYPE_JCERACFKS = "JCERACFKS";
public static final String SSL_STORE_TYPE_JCE4758RACFKS = "JCE4758RACFKS";
public static final String IBM_SSL_CERT_TYPE = "IbmX509";
public static final String SUN_SSL_CERT_TYPE = "SunX509";
// Message encoding properties
public final static String DEFAULT_CODEPAGE_EBCDIC_US = "CP037";
public final static String DEFAULT_CODEPAGE_ASCII_US = "CP1252";
public final static String DEFAULT_CODEPAGE_UTF8 = "UTF-8";
public final static String DEFAULT_CODEPAGE_FOR_LOGGING =DEFAULT_CODEPAGE_UTF8 ;
/**
* Constant with the default value for TmInteraction
* imsConnectCodepage
property which maps to "CP037" for EBCDIC
* code page 037 for Australia, Brazil, Canada, New Zealand, Portugal, South
* Africa, and United States.
*
* @see TmInteraction#setImsConnectCodepage(String)
* @see TmInteraction#getImsConnectCodepage()
*/
public final static String DEFAULT_IMS_CONNECT_CODEPAGE = DEFAULT_CODEPAGE_EBCDIC_US;
/**
* Constant for TmInteraction unicodeEncodingSchema
with byte
* value 0x00.
*/
public final static byte IMS_CONNECT_UNICODE_ENCODING_SCHEMA_NONE = (byte) 0x00;
/**
* Constant for TmInteraction unicodeEncodingSchema
field. The
* default value maps to
* {@link ApiProperties#IMS_CONNECT_UNICODE_ENCODING_SCHEMA_NONE}.
*/
public final static byte DEFAULT_IMS_CONNECT_UNICODE_ENCODING_SCHEMA = IMS_CONNECT_UNICODE_ENCODING_SCHEMA_NONE;
/**
* Constant for TmInteraction imsConnectUnicodeUsage
with value
* 0x00.
*/
public final static byte IMS_CONNECT_UNICODE_USAGE_NONE = (byte) 0x00;
/**
* Constant for TmInteraction imsConnectUnicodeUsage
with byte
* value 0x10.
*/
public final static byte IMS_CONNECT_UNICODE_USAGE_TRANCODE = (byte) 0x10;
/**
* Constant for TmInteraction imsConnectUnicodeUsage
with byte
* value 0x20.
*/
public final static byte IMS_CONNECT_UNICODE_USAGE_DATA = (byte) 0x20;
/**
* Constant with the default value for TmInteraction
* imsConnectUnicodeUsage
which maps to
* {@link ApiProperties#IMS_CONNECT_UNICODE_USAGE_NONE}.
*/
public final static byte DEFAULT_IMS_CONNECT_UNICODE_USAGE = IMS_CONNECT_UNICODE_USAGE_NONE;
// Tracing properties
/**
* Constant containing the default Windows value for TmInteraction
* traceFileName
field which maps to ".\\trace.log".
*/
public final static String DEFAULT_TRACE_FILE_NAME_WINDOWS = ".\\trace.log";
/**
* Constant containing the default Unix value for TmInteraction
* traceFileName
field which maps to
* "/usr/lpp/ims/iconapi/trace.log".
*/
public final static String DEFAULT_TRACE_FILE_NAME_UNIX = "/usr/lpp/ims/iconapi/trace.log";
/**
* Constant containing the default value for TmInteraction
* traceFileName
field which maps to
* {@link ApiProperties#DEFAULT_TRACE_FILE_NAME_WINDOWS}.
*/
public final static String DEFAULT_TRACE_FILE_NAME = DEFAULT_TRACE_FILE_NAME_WINDOWS;
public final static String DEFAULT_TRACE_FILE_NAME_USS = DEFAULT_TRACE_FILE_NAME_UNIX; // z/OS
// Unix
// System
/**
* Constant for the traceLevel
property with value of
* java.util.logging.Level.OFF
.
*/
public final static Level TRACE_LEVEL_NONE = Level.OFF; // intValue =
// Integer.MAX_VALUE
/**
* Constant for the traceLevel
property with value of
* java.util.logging.Level.SEVERE
.
*/
public final static Level TRACE_LEVEL_EXCEPTION = Level.SEVERE; // intValue = 1000
/**
* Constant for the traceLevel
property with value of
* java.util.logging.Level.FINER
.
*/
public final static Level TRACE_LEVEL_ENTRY_EXIT = Level.FINER; // intValue = 400
/**
* Constant for the traceLevel
property with value of
* java.util.logging.Level.FINEST
.
*/
public final static Level TRACE_LEVEL_INTERNAL = Level.FINEST; // intValue = 300
/**
* Constant containing the default value for traceLevel
which
* maps to {@link ApiProperties#TRACE_LEVEL_NONE}.
*/
public final static Level DEFAULT_TRACE_LEVEL = TRACE_LEVEL_NONE;
// Response Properties
/**
* Constant for TmInteraction responseIncludesLlll
with value
* of true
.
*/
public final static boolean RESPONSE_DOES_INCLUDE_LLLL = true; // HWSSMPL1/HWSDPWR1 style response message
/**
* Constant for TmInteraction responseIncludesLlll
with value
* of false
.
*/
public final static boolean RESPONSE_DOES_NOT_INCLUDE_LLLL = false; // HWSSMPL0 style response message
/**
* Constant with the default value for the TmInteraction
* responseIncludesLlll
property which maps to
* {@link ApiProperties#RESPONSE_DOES_INCLUDE_LLLL}.
*/
public final static boolean DEFAULT_RESPONSE_INCLUDES_LLLL = RESPONSE_DOES_INCLUDE_LLLL;
/**
* Response property with value of true
indicating that there
* is additional asynchronous output available to be retrieved from OTMA.
*
* @see TmInteraction#isAsyncOutputAvailable()
* @see ApiProperties#NO_ASYNC_OUTPUT_AVAILABLE
*/
public final static boolean ASYNC_OUTPUT_AVAILABLE = true;
/**
* Constant for TmInteraction asyncOutputAvailable with the value
* false
.
*
* @see TmInteraction#isAsyncOutputAvailable()
* @see ApiProperties#ASYNC_OUTPUT_AVAILABLE
*/
public final static boolean NO_ASYNC_OUTPUT_AVAILABLE = false;
/**
* Constant for TmInteraction inConversation
with value
* true
.
*
* @see ApiProperties#NOT_IN_CONVERSATION
* @see TmInteraction#isInConversation()
*/
public final static boolean IN_CONVERSATION = true;
/**
* Constant for TmInteraction inConversation
with value
* false
.
*
* @see ApiProperties#IN_CONVERSATION
* @see TmInteraction#isInConversation()
*/
public final static boolean NOT_IN_CONVERSATION = false;
/**
* TmInteraction property constant with value of true
. This
* constant is a response property indicating that IMS Connect requires an
* ACK (positive) or NAK (negative) response acknowledgement interaction as
* the next interaction from the client application (or an ACK from the API
* on behalf of the client application, although clients will never actually
* see an ACK_NAK_NEEDED if ACK_NAK_PROVIDER is set to API_INTERNAL_ACK
* since, in this case, the API will have already sent in an ACK before the
* client application gets control back).
*
* @see ApiProperties#NO_ACK_NAK_NEEDED
* @see TmInteraction#isAckNakNeeded()
*/
public final static boolean ACK_NAK_NEEDED = true;
/**
* Constant for TmInteraction ackNakNeeded
with value
* false
.
*
* @see ApiProperties#ACK_NAK_NEEDED
* @see TmInteraction#isAckNakNeeded()
*/
public final static boolean NO_ACK_NAK_NEEDED = false;
/**
* Constant for TmInteraction imsConnectReturnCode
with int
* value 0.
*
* @see TmInteraction#getImsConnectReturnCode()
*/
public final static int IMS_CONNECT_RETURN_CODE_SUCCESS = 0;
/**
* Constant for TmInteraction imsConnectReasonCode
with int
* value 0.
*
* @see TmInteraction#getImsConnectReasonCode()
*/
public final static int IMS_CONNECT_REASON_CODE_SUCCESS = 0;
/**
* Constant for TmInteraction otmaSenseCode
with int value 0.
*
* @see TmInteraction#getOtmaSenseCode()
*/
public final static int OTMA_SENSE_CODE_SUCCESS = (int) 0x00;
/**
* Constant for the TmInteraction CalloutRequestNakProcessing
* property with the value "DISCARDREQUESTCONTINUERESUMETPIPE"
.
* The byte
value for this constant is 08.
*
* @see TmInteraction#setCalloutRequestNakProcessing(String)
* @since Enterprise Suite 2.1
*/
public final static String NAK_DISCARD_SYNC_CALLOUT_REQUEST_CONTINUE_RESUMETPIPE = "DISCARDREQUESTCONTINUERESUMETPIPE";
public final static byte NAK_DISCARD_SYNC_CALLOUT_REQUEST_CONTINUE_RESUMETPIPE_VALUE = 0x08;
/**
* Constant for the TmInteraction CalloutRequestNakProcessing
* property with the value "DISCARDREQUESTENDRESUMETPIPE"
. The
* byte
value for this constant is 00.
*
* @see TmInteraction#setCalloutRequestNakProcessing(String)
* @since Enterprise Suite 2.1
*/
public final static String NAK_DISCARD_SYNC_CALLOUT_REQUEST_END_RESUMETPIPE = "DISCARDREQUESTENDRESUMETPIPE";
public final static byte NAK_DISCARD_SYNC_CALLOUT_REQUEST_END_RESUMETPIPE_VALUE = 0x00;
/**
* Constant for the TmInteraction CalloutRequestNakProcessing
* property with the value "REQUEUEREQUESTENDRESUMETPIPE"
. The
* byte
value for this constant is 20.
*
* @see TmInteraction#setCalloutRequestNakProcessing(String)
* @since Enterprise Suite 2.1
*/
public final static String NAK_REQUEUE_SYNC_CALLOUT_REQUEST_END_RESUMETPIPE = "REQUEUEREQUESTENDRESUMETPIPE";
public final static byte NAK_REQUEUE_SYNC_CALLOUT_REQUEST_END_RESUMETPIPE_VALUE = 0x20;
/**
* Constant for the TmInteraction property
* CalloutRequestNakProcessing
with the value
* "DISCARDREQUESTENDRESUMETPIPE"
.
*
* @see TmInteraction#setCalloutRequestNakProcessing(String)
* @since Enterprise Suite 2.1
*/
public final static String DEFAULT_CALLOUT_REQUEST_NAK_PROCESSING = NAK_DISCARD_SYNC_CALLOUT_REQUEST_END_RESUMETPIPE;
/**
* Constant for the TmInteraction CalloutRequestNakProcessing
* property with the value "DISCARDREQUESTENDRESUMETPIPE"
.
*
* @see ApiProperties#NAK_DISCARD_SYNC_CALLOUT_REQUEST_END_RESUMETPIPE_VALUE
* @see TmInteraction#setCalloutRequestNakProcessing(String)
* @since Enterprise Suite 2.1
*/
public final static byte DEFAULT_CALLOUT_REQUEST_NAK_PROCESSING_VALUE = NAK_DISCARD_SYNC_CALLOUT_REQUEST_END_RESUMETPIPE_VALUE;
// calloutResponseMessageType property values
/**
* Constant for the TmInteraction calloutResponseMessageType
* property with the value "CALLOUTRESPONSEMESSAGE"
.
*
* @see TmInteraction#setCalloutResponseMessageType(String)
* @since Enterprise Suite 2.1
*/
public final static String CALLOUT_RESPONSE_MESSAGE = "CALLOUTRESPONSEMESSAGE";
/**
* Constant for the TmInteraction calloutResponseMessageType
* property with the value "CALLOUTERRORMESSAGE"
.
*
* @see TmInteraction#setCalloutResponseMessageType(String)
* @since Enterprise Suite 2.1
*/
public final static String CALLOUT_ERROR_MESSAGE = "CALLOUTERRORMESSAGE";
/**
* Constant for the TmInteraction calloutResponseMessageType
* property with the value "CALLOUTRESPONSEMESSAGE"
.
*
* @see TmInteraction#setCalloutResponseMessageType(String)
* @since Enterprise Suite 2.1
*/
public final static String DEFAULT_CALLOUT_RESPONSE_MESSAGE_TYPE = CALLOUT_RESPONSE_MESSAGE;
// resumeTPipeRetrievalType
/**
* Constant for the TmInteraction resumeTPipeRetrievalType
* property with the byte
value 0x80
.
*
* @see TmInteraction#setResumeTpipeRetrievalType(byte)
* @since Enterprise Suite 2.1
*/
public final static byte RETRIEVE_SYNC_MESSAGE_ONLY = (byte) 0x80;
/**
* Constant for the TmInteraction resumeTPipeRetrievalType
* property with the byte
value 0x40
.
*
* @see TmInteraction#setResumeTpipeRetrievalType(byte)
* @since Enterprise Suite 2.1
*/
public final static byte RETRIEVE_SYNC_OR_ASYNC_MESSAGE = (byte) 0x40;
/**
* Constant for the TmInteraction resumeTPipeRetrievalType
* property with the byte
value 0x00
.
*
* @see TmInteraction#setResumeTpipeRetrievalType(byte)
* @since Enterprise Suite 2.1
*/
public final static byte RETRIEVE_ASYNC_MESSAGE = (byte) 0x00;
public final static byte RETRIEVE_ASYNC_MESSAGE_ONLY = (byte) 0x00;
/**
* Constant for the TmInteraction resumeTPipeRetrievalType
* property with the byte
value 0x00
.
*
* @see TmInteraction#setResumeTpipeRetrievalType(byte)
* @since Enterprise Suite 2.1
*/
public final static byte DEFAULT_RESUME_TPIPE_RETRIEVAL_TYPE = RETRIEVE_ASYNC_MESSAGE_ONLY;
// Correlator Token
/**
* Constant for the TmInteraction correlatorToken
property with
* the byte[]
value Empty byte array
.
*
* @see TmInteraction#setCorrelatorToken
* @since Enterprise Suite 2.1
*/
public final static byte[] CORRELATOR_TOKEN_DEFAULT = new byte[ApiProperties.IRM_CT_LEN];
/**
* The maximum length of the correlator token.
*
* @since Enterprise Suite 2.1
*/
public final static short IRM_CT_LEN = 0x28;// 40 bytes len
/**
* The size of LL field of correlator token.
*
* @since Enterprise Suite 2.2
*/
public final static int IRM_CT_LEN_SIZE = 2;
/**
* The reserved byte value in the correlatorToken.
*
* @since Enterprise Suite 2.1
*/
public final static short IRM_CT_RESV1 = 0;
/**
* The reserved byte size in the correlatorToken.
*
* @since Enterprise Suite 2.1
*/
public final static int IRM_CT_RESV1_LEN = 2;
/**
* The default IMSID in the correlatorToken.
*
* @since Enterprise Suite 2.1
*/
public final static String IRM_CT_IMSID_DEFAULT = " ";
/**
* The length of the IMSID in the correlatorToken.
*
* @since Enterprise Suite 2.1
*/
public final static int IRM_CT_IMSID_LEN = 4;
/**
* The default MEMTK value in the correlatorToken.
*
* @since Enterprise Suite 2.1
*/
public final static String IRM_CT_MEMTK_DEFAULT = EIGHT_BLANKS;
/**
* The MEMTK length in the correlatorToken.
*
* @since Enterprise Suite 2.1
*/
public final static int IRM_CT_MEMTK_LEN = 8;
/**
* The default AWETK value in the correlatorToken.
*
* @since Enterprise Suite 2.1
*/
public final static String IRM_CT_AWETK_DEFAULT = EIGHT_BLANKS;
/**
* The AWETK length in the correlatorToken.
*
* @since Enterprise Suite 2.1
*/
public final static int IRM_CT_AWETK_LEN = 8;
/**
* The default TPIPE name in the correlatorToken.
*
* @since Enterprise Suite 2.1
*/
public final static String IRM_CT_TPIPE_DEFAULT = EIGHT_BLANKS;
/**
* The TPIPE length in the correlatorToken.
*
* @since Enterprise Suite 2.1
*/
public final static int IRM_CT_TPIPE_LEN = 8;
/**
* The default USERID in the correlatorToken.
*
* @since Enterprise Suite 2.1
*/
public final static String IRM_CT_USERID_DEFAULT = EIGHT_BLANKS;
/**
* The maximum length of the USERID in the correlatorToken.
*
* @since Enterprise Suite 2.1
*/
public final static int IRM_CT_USERID_MAX_LEN = 8;
/**
* The default reason code for NAK error responses.
*
* @since Enterprise Suite 2.1
*/
public static final short IRM_NAK_REASONCODE_DEFAULT = 0;
// Input Mod Name
/**
* The default value for the input mod name.
*
* @since Enterprise Suite 2.1
*/
public final static String DEFAULT_INPUT_MOD_NAME = EIGHT_BLANKS;
/**
* The maximum length of the input mod name.
*
* @since Enterprise Suite 2.1
*/
public final static int MAX_LEN_INPUT_MOD_NAME = 8;
/**
* Constant for ApiLoggingConfiguration
* loggerAppendMode
property with
* value of true
.
*/
public final static boolean ENABLE_LOGGER_APPEND_MODE = true;
/**
* Constant for ApiLoggingConfiguration
* loggerAppendMode
property with
* value of false
.
*/
public final static boolean DISABLE_LOGGER_APPEND_MODE = false;
/**
* Constant with the default value for ApiLoggingConfiguration
* loggerAppendMode
which maps to
* {@link ApiProperties#DISABLE_LOGGER_APPEND_MODE}
* .
*/
public final static boolean DEFAULT_LOGGER_APPEND_MODE = DISABLE_LOGGER_APPEND_MODE;
/**
* Constant for ApiLoggingConfiguration
* loggerFileLimit
property with
* value of 0
.
*/
public final static int LOGGER_FILE_NO_LIMIT =0;
/**
* Constant with the default value for ApiLoggingConfiguration
* loggerFileLimit
which maps to
* {@link ApiProperties#LOGGER_FILE_NO_LIMIT}
* .
*/
public final static int DEFAULT_LOGGER_FILE_LIMIT= LOGGER_FILE_NO_LIMIT;
/**
* Constant for ApiLoggingConfiguration
* loggerFileCount
property with
* value of 1
.
*/
public final static int DEFAULT_LOGGER_FILE_COUNT =1;
}