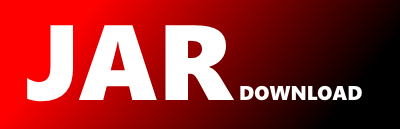
com.ibm.ims.connect.Connection Maven / Gradle / Ivy
Show all versions of IMSConnectAPI Show documentation
/*
* File: Connection.java
* ==========================================================================
* Licensed Material - Property of IBM
*
* IBM Confidential
*
* OCO Source Materials
*
* 5655-TDA
*
* (C) Copyright IBM Corp. 2009,2013 All Rights Reserved.
*
* The source code for this program is not published or
* otherwise divested of its trade secrets, irrespective
* of what has been deposited with the U.S. Copyright
* Office.
*
* US Government Users Restricted Rights - Use, duplication or
* disclosure restricted by GSA ADP Schedule Contract with
* IBM Corp.
* ===========================================================================
*/
package com.ibm.ims.connect;
import java.net.*;
import java.io.*;
/**
* A TCP/IP socket connection for communicating with IMS Connect.
*
*/
public interface Connection extends ApiProperties
{
public static final String copyright =
"Licensed Material - Property of IBM "
+ "5655-TDA"
+ "(C) Copyright IBM Corp. 2009, 2013 All Rights Reserved. "
+ "US Government Users Restricted Rights - Use, duplication or "
+ "disclosure restricted by GSA ADP Schedule Contract with IBM Corp. ";
/**
* Opens a new TCP/IP socket connection that will be associated
* with this Connection
object. Depending on the value of the useSsl
* connection property that was set, calling this method creates a non-SSL connection
* if useSsl is false
or an SSL connection if useSsl is true
.
* @throws ImsConnectApiException, ImsConnectCommunicationException, SocketException
*/
public void connect() throws ImsConnectApiException, ImsConnectCommunicationException, SocketException;
/**
* Closes the TCP/IP socket connection and destroys the Connection
object.
*
* The disconnect
method internally closes a socket and then sets that socket instance to null
* if the socket is not null. If the socket is already null, the disconnect
method just returns
* control to the client application. Calling the disconnect
method is optional since the socket
* will be reused if it is not disconnected by the client application, and will automatically be destroyed
* when the client application ends.
* @throws ImsConnectApiException
*
*/
public void disconnect() throws ImsConnectApiException;
/**
* Instantiates a new TmInteraction
instance that encapsulates the properties and methods to
* set up, execute, and retrieve results from an interaction with IMS Connect.
* Calling the createInteraction() method sets the connection field of the new interaction to
* this Connection
instance.
* @throws ImsConnectApiException
*/
public TmInteraction createInteraction() throws ImsConnectApiException;
/**
* Creates a new TmInteraction
instance that encapsulates the properties and methods to set up,
* execute, and retrieve results from an interaction with IMS Connect. The generated TmInteraction
* instance will be configured with interaction properties taken from the TmInteractionAttributes
* instance passed in as input to this method.
* @param aTMInteractionAttributes a TmInteractionAttributes
instance that stores the
* properties that are used to perform an interaction with IMS Connect.
* @return a new TmInteraction
instance
* @throws ImsConnectApiException
*/
public TmInteraction createInteraction(TmInteractionAttributes aTMInteractionAttributes)
throws ImsConnectApiException;
/**
* Sets the connection properties of this Connection
instance with values read in
* from a connection properties file.
* @param aConnectionAttributesTextFileName a text file containing the connection properties
* @throws Exception
*/
public void loadConnectionAttributesFromFile(String aConnectionAttributesTextFileName)
throws Exception;
/**
* Sets the connection properties of this Connection
instance with the property values from a
* ConnectionAttributes
instance.
* @param aConnectionAttributes a ConnectionAttributes
instance with
* connection properties.
*/
public void loadConnectionAttributesFromObject(ConnectionAttributes aConnectionAttributes);
/**
* Reads any available data received on the socket associated with this Connection
instance.
* This is a blocking call. The client application will not regain control until after data has been received
* or the receive call has been interrupted by an InteractionTimeout exception.
* The receive
method is intended primarily to be used internally by the API during execute,
* but can be used directly by client applications as long as the IMS Connect protocol for sending
* and receiving data is followed.
* @return a byte array containing the data returned by IMS Connect
* @throws ImsConnectApiException
*/
public byte[] receive() throws Exception;
/**
* Writes data to be sent to IMS Connect to the socket associated with this Connection
instance.
* The send
method is intended primarily to be used internally by the API during execute,
* but can be used directly by client applications as long as the IMS Connect protocol for sending and
* receiving data is followed.
* @param outBytes a byte array containing the data to be sent to IMS Connect.
* @throws ImsConnectApiException
*/
public void send(byte[] outBytes) throws ImsConnectApiException;
// Getter and setter methods
/**
* Indicates if there is a connected socket associated with this connection.
* This method only indicates that the socket is connected to the TCP/IP network at
* the API (client application) end. It does not give an indication if the partner end socket
* connection to IMS Connect is still connected. In most cases, you will only know if a socket has
* been disconnected at the IMS Connect end if you get a connection failure during the receive
* call performed by the API during the execute
method.
* @return true
if this connection is connected, otherwise false
.
*/
public boolean isConnected();
/**
* Gets the clientId property value. Note that this method will only return clientId property values
* that have been set using the Connection.setClientId(String) method of this Connection or the
* ConnectionFactory from which this Connection Interface was obtained. If the clientId was generated
* by the IMS Connect user message exit (as is the case when the clientId value is all blanks when
* connect() is called for that Connection,) that generated clientId value will not be returned (the
* original value of all blanks will be returned) by default unless the returnClientId property is
* set to true in the TmInteraction.
* @return the clientId property value as a String
.
* @see Connection#setClientId(String)
*/
public String getClientId();
/**
* Sets the clientId field of this Connection
object, which is used by IMS Connect and IMS
* to identify IMS Connect clients. The clientId is also used for the names of asynchronous message queues
* (OTMA Tpipes) and other internal data structures in IMS Connect and OTMA.
*
The clientId value is a string of 1 to 8 uppercase alphanumeric (A through Z, 0 to 9) or special
* (@, #, $) characters, left justified, and padded with blanks.
*
This value can be created by the client application or assigned by IMS Connect. If, on the first
* input message for a transaction request, the clientId property value is all blanks, the clientID will
* be assigned by IMS Connect. Because IMS Connect is not able to return the generated clientId value to
* the client application in the response message, the clientId value in the Connection (all blanks) will
* be different than the clientId value used for this connection in IMS Connect (the generated clientId
* value.)
*
The clientID value specified in the Connection object will only be used by IMS Connect during
* processing of the first request message received on that connection. After that time, the clientID value
* specified by the client during subsequent interactions on that connection will be ignored by IMS Connect.
* If a Connection is disconnected, and then reconnected, the connection will be treated as a new connection
* and the clientId at the time of the first input message for a transaction request on the new connection
* will be used as described above.
*
If setClientId is called after a connection has been established (in other words, after the connect()
* method has been called either by the client application or by the API interally,) an exception will be
* thrown by the API indicating that an attempt was made to change the clientId of an already-connected
* connection which is not allowed.
* @param aClientId the clientID to be used for this interaction.
* @see ApiProperties#DEFAULT_CLIENTID
* @throws ImsConnectApiException
*/
public void setClientId(String aClientId) throws ImsConnectApiException;
/**
* Gets the hostName property value.
* @return the hostName property value as a String
.
* @see Connection#setHostName(String)
*/
public String getHostName();
/**
* Sets the value of the hostName field of this Connection
. The hostName property is a String
* variable whose value is the hostname or IP address of the target IMS Connect for this connection.
*
The input String
must be either:
*
* - a 1 to 8 character
String
set to the correct hostname of the target IMS Connect, or
* - a
String
containing the IP V4 or IP V6 address of the target IMS Connect
* (e.g. "nnn.nnn.nnn.nnn" or "nnn.nnn.nnn.nnn.nnn.nnn").
*
* If a value for this property is not supplied by the client, the default value ("HOSTNAME") will be used.
* @param aHostName the hostName or IP address to be used for this interaction.
* @see ApiProperties#DEFAULT_HOSTNAME
* @throws ImsConnectApiException
*/
public void setHostName(String aHostName) throws ImsConnectApiException;
/**
* Gets the interactionTimeout property value.
* @return the interactionTimeout property value as an int
in milliseconds.
*/
public int getInteractionTimeout();
/**
* Sets the interactionTimeout field of this Connection
instance and the
* SO_TIMEOUT value of the underlying socket.
*
The interaction timeout value is in milliseconds. A non-zero value for
* the interaction timeout sets the SO_TIMEOUT value to that number of milliseconds. A timeout
* value of zero disables the SO_TIMEOUT function. If disabled, a socket will block forever
* if a read is executed on that socket and nothing is ever available to be read on that
* socket by the IMS Connect API on behalf of the client application.
*
* @param anInteractionTimeout the interaction timeout value in milliseconds. You can
* specify this parameter by using the constants defined in ApiProperties or by providing
* a valid non-negative integer value.
* @see ApiProperties#TIMEOUT_1_MILLISECOND
* @see ApiProperties#TIMEOUT_2_MILLISECONDS
* @see ApiProperties#TIMEOUT_5_MILLISECONDS
* @see ApiProperties#TIMEOUT_10_MILLISECONDS
* @see ApiProperties#TIMEOUT_20_MILLISECONDS
* @see ApiProperties#TIMEOUT_50_MILLISECONDS
* @see ApiProperties#TIMEOUT_100_MILLISECONDS
* @see ApiProperties#TIMEOUT_200_MILLISECONDS
* @see ApiProperties#TIMEOUT_500_MILLISECONDS
* @see ApiProperties#TIMEOUT_1_SECOND
* @see ApiProperties#TIMEOUT_2_SECONDS
* @see ApiProperties#TIMEOUT_5_SECONDS
* @see ApiProperties#TIMEOUT_10_SECONDS
* @see ApiProperties#TIMEOUT_30_SECONDS
* @see ApiProperties#TIMEOUT_45_SECONDS
* @see ApiProperties#TIMEOUT_1_MINUTE
* @see ApiProperties#TIMEOUT_2_MINUTES
* @see ApiProperties#TIMEOUT_5_MINUTES
* @see ApiProperties#TIMEOUT_10_MINUTES
* @see ApiProperties#TIMEOUT_30_MINUTES
* @see ApiProperties#TIMEOUT_45_MINUTES
* @see ApiProperties#TIMEOUT_1_HOUR
* @see ApiProperties#TIMEOUT_2_HOURS
* @see ApiProperties#TIMEOUT_4_HOURS
* @see ApiProperties#TIMEOUT_8_HOURS
* @see ApiProperties#TIMEOUT_12_HOURS
* @see ApiProperties#TIMEOUT_1_DAY
* @see ApiProperties#TIMEOUT_2_DAYS
* @see ApiProperties#TIMEOUT_5_DAYS
* @see ApiProperties#TIMEOUT_1_WEEK
* @see ApiProperties#TIMEOUT_2_WEEKS
* @see ApiProperties#INTERACTION_TIMEOUT_MAX
* @see ApiProperties#TIMEOUT_WAIT_FOREVER
* @throws ImsConnectCommunicationException
*/
public void setInteractionTimeout(int anInteractionTimeout) throws ImsConnectApiException;
/**
* Gets the portNumber property value.
* @return the portNumber value.
* @see Connection#setPortNumber(int)
*/
public int getPortNumber();
/**
* Sets the value of the portNumber field of this Connection object. The portNumber property specifies
* a TCP/IP port where the target IMS Connect is listening for requests for
* new connections from clients.
*
If a value for this property is not supplied by the client, the default value (9999) will be used.
* @param aPortNumber a valid port number on which the target IMS Connect is listening for incoming
* requests from IMS Connnect clients.
* @see ApiProperties#DEFAULT_PORTNUMBER
* @throws ImsConnectApiException
*/
public void setPortNumber(int aPortNumber) throws ImsConnectApiException;
/**
* Gets the socketConnectTimeout property value.
* @return the socketConnectTimeout
*/
public int getSocketConnectTimeout();
/**
* Sets the socketConnectTimeout field of this Connection
.
*
The socketConnectTimeout determines the amount of time that the IMS Connect API will wait for
* a socket.connect() call to complete. Only one value can be specified per socket.
*
The valid input values, for some of which, constants are defined in ApiProperties, are:
*
* - -1 - API will wait forever (or until the connect request times out due to exceeding the maximum
* number of connection retry attempts defined in the TCP/IP properties.)
*
- > 0 - API will wait either until the maximum number of connection retry attempts defined in the
* TCP/IP properties has been attempted or until the socketConnectTimeout period has elapsed before
* throwing a java.net.SocketTimeoutException.
*
* Specifying a value less than -1 will result in an ImsConnectCommunicationException being thrown
* back to the client application
* @param aSocketConnectTimeout the socketConnectTimeout to set in milliseconds
* @see ApiProperties#DEFAULT_SOCKET_CONNECT_TIMEOUT
* @see ApiProperties#TIMEOUT_1_MILLISECOND
* @see ApiProperties#TIMEOUT_2_MILLISECONDS
* @see ApiProperties#TIMEOUT_5_MILLISECONDS
* @see ApiProperties#TIMEOUT_10_MILLISECONDS
* @see ApiProperties#TIMEOUT_20_MILLISECONDS
* @see ApiProperties#TIMEOUT_50_MILLISECONDS
* @see ApiProperties#TIMEOUT_100_MILLISECONDS
* @see ApiProperties#TIMEOUT_200_MILLISECONDS
* @see ApiProperties#TIMEOUT_500_MILLISECONDS
* @see ApiProperties#TIMEOUT_1_SECOND
* @see ApiProperties#TIMEOUT_2_SECONDS
* @see ApiProperties#TIMEOUT_5_SECONDS
* @see ApiProperties#TIMEOUT_10_SECONDS
* @see ApiProperties#TIMEOUT_30_SECONDS
* @see ApiProperties#TIMEOUT_45_SECONDS
* @see ApiProperties#TIMEOUT_1_MINUTE
* @see ApiProperties#TIMEOUT_2_MINUTES
* @see ApiProperties#TIMEOUT_5_MINUTES
* @see ApiProperties#TIMEOUT_10_MINUTES
* @see ApiProperties#TIMEOUT_30_MINUTES
* @see ApiProperties#TIMEOUT_45_MINUTES
* @see ApiProperties#TIMEOUT_1_HOUR
* @see ApiProperties#TIMEOUT_2_HOURS
* @see ApiProperties#TIMEOUT_4_HOURS
* @see ApiProperties#TIMEOUT_8_HOURS
* @see ApiProperties#TIMEOUT_12_HOURS
* @see ApiProperties#TIMEOUT_1_DAY
* @see ApiProperties#TIMEOUT_2_DAYS
* @see ApiProperties#TIMEOUT_5_DAYS
* @see ApiProperties#TIMEOUT_1_WEEK
* @see ApiProperties#TIMEOUT_2_WEEKS
* @see ApiProperties#TIMEOUT_WAIT_FOREVER
* @see ApiProperties#SOCKET_CONNECT_TIMEOUT_USE_TCPIP_DEFAULTS
* @throws ImsConnectCommunicationException
*/
public void setSocketConnectTimeout(int aSocketConnectTimeout) throws ImsConnectApiException;
/**
* Gets the socketType property value.
* @return the socketType value.
* @see ApiProperties#SOCKET_TYPE_PERSISTENT
* @see ApiProperties#SOCKET_TYPE_TRANSACTION
*/
public byte getSocketType();
/**
* Sets the socketType field of this Connection
.
*
The type of socket connection of a message is set by the client in the initial input message and
* maintained in the message until the interaction has completed. Only one value can be specified per
* interaction.
*
The valid input values, for which the following constants are defined in ApiProperties, are:
*
* - SOCKET_TYPE_TRANSACTION = 0x00. A transaction socket can only be disconnected by IMS Connect.
* Transaction sockets are used for one transaction (including and required ACKs or NAKs) for non-conversational
* transactions or for one complete conversation (until the conversation is ended by the client or host application)
* for conversational transactions. Once the non-conversational IMS transaction interaction or IMS conversation
* has completed, the transaction socket is automatically closed by both IMS Connect and the API. The
* transaction socket also closes automatically after IMS itself has terminated or after an error has occured.
*
- SOCKET_TYPE_PERSISTENT = 0x10. A persistent socket is left connected by IMS Connect until a fatal error
* has occured on that connection while IMS Connect is processing an interaction, or until a disconnect request
* has been received by IMS Connect. Any open persistent connections will be closed automatically by the API
* when the client application ends.
*
- DEFAULT_SOCKET_TYPE (same as SOCKET_TYPE_PERSISTENT)
*
* If a value for this property is not supplied by the client, the default value (SOCKET_TYPE_PERSISTENT)
* will be used.
* @param aSocketType the socket type to be used for this interaction. Use the constants defined
* in ApiProperties to specify this parameter.
* @see ApiProperties#DEFAULT_SOCKET_TYPE
* @see ApiProperties#SOCKET_TYPE_PERSISTENT
* @see ApiProperties#SOCKET_TYPE_TRANSACTION
* @throws ImsConnectApiException
*/
public void setSocketType(byte aSocketType) throws ImsConnectApiException;
/**
* Returns the SSL encryption type property.
* @return the sslEncryptionType.
*/
public byte getSslEncryptionType();
/**
* Sets the sslEncryptionType field of this Connection
. The sslEncryptionType property is a byte
* value used to set the encryption type for the underlying connection when that connnection is an SSL
* connection.
*
The valid values, for which the following constants are defined in ApiProperties
, are:
*
* - SSL_ENCRYPTIONTYPE_STRONG = 0x02
*
- SSL_ENCRYPTIONTYPE_WEAK = 0x01
*
- SSL_ENCRYPTYPE_NONE = 0x00
*
- DEFAULT_SSL_ENCRYPTIONTYPE (same as SSL_ENCRYPTIONTYPE_WEAK)
*
* If a value for this property is not supplied by the client, the default value (SSL_ENCRYPTIONTYPE_WEAK)
* will be used.
*
SSL_ENCRYPTIONTYPE_STRONG and SSL_ENCRYPTIONTYPE_WEAK reflect the strength of the cipher used which
* is related to the key length. All those ciphers that can be used for export are in the
* SSL_ENCRYPTIONTYPE_WEAK category and the rest go into the strong category. The IMS Connect API will
* negotiate with IMS Connect to use a strong (greater than 64-bit encryption key) or weak (64-bit
* or smaller encryption key) cipher specification accordingly if either of these two settings are specified.
*
When SSL_ENCRYPTIONTYPE_NONE is specified, the IMS Connect API will negotiate with IMS Connect to
* use a cipher spec whose name contains the string "NULL". Null encryption will allow for
* authentication to take place during the SSL handshaking process. Once the handshaking process for a
* socket has completed including authentication as required, all messages will flow in the clear (without
* encryption) over that socket.
* @param anSslEncryptionType the sslEncryptionType to be used for this interaction. Use the constants defined
* in ApiProperties to specify this parameter.
* @see ApiProperties#DEFAULT_SSL_ENCRYPTIONTYPE
* @see ApiProperties#SSL_ENCRYPTIONTYPE_NONE
* @see ApiProperties#SSL_ENCRYPTIONTYPE_STRONG
* @see ApiProperties#SSL_ENCRYPTIONTYPE_WEAK
* @throws ImsConnectApiException
*/
public void setSslEncryptionType(byte anSslEncryptionType) throws ImsConnectApiException;
/**
* Returns the SSL keystore InputStream
instance.
* @return the sslKeystoreInputStream
*/
public InputStream getSslKeystoreInputStream();
/**
* Sets the sslKeystoreInputStream field of this Connection
.
*
An SSL keystore is a password-protected database intended to contain private key
* material, such as private keys and their associated private key certificates. The private key
* in your keystore is used for encrypting/signing outgoing messages that can then only be
* decrytped using your public key which you have distributed to your partners. For your
* convenience, the IMS Connect API allows both private keys (and their associated private key
* certificates) as well as trusted certificates (usually stored in your truststore) to be stored
* in the same keystore, in which case the sslTruststoreInputStream, sslTruststoreUrl and/or
* sslTruststoreName property can either be null or can point to the keystore InputStream, URL or
* file, respectively. Likewise, private keys or certificates could be stored in the truststore
* along with your trusted certificates, in which case the sslKeystoreInputStream, sslKeystoreUrl
* and/or sslKeystoreName properties could either be null or could point to the same or a different
* keystore or truststore InputStream, URL or file, respectively.
*
The sslKeystoreInputStream property can be used to specify an InputStream that wraps a JKS
* keystore file. When TmInteraction.execute() is called, if a value is specified for the
* sslKeystoreName, the sslKeystoreUrl and the sslKeystoreInputStream, the sslKeystoreInputStream
* value takes precedence and will be used by the Connect API to load the keystore. If the
* sslKeystoreInputStream value is null, the sslKeystoreUrl value, if non-null, will be used. Only
* when both the sslKeystoreInputStream and sslKeystoreUrl values are null, will the sslKeystoreName
* be used. If a value for this property is not set by the client application, the default value
* (null) will be used for this property.
*
It is important to note that an InputStream, because it does not support the mark or reset
* features, can only be used once. In fact, due to this restriction, the API internally closes an
* InputStream after it has loaded the keystore from that InputStream.
* @param anSslKeystoreInputStream a java.io.InputStream
to be used to set the
* sslKeystoreInputStream value for this interaction
* @see ApiProperties#DEFAULT_SSL_KEYSTORE_INPUT_STREAM
* @see Connection#setSslKeystoreName(String anSslKeystoreName)
*/
public void setSslKeystoreInputStream(InputStream anSslKeystoreInputStream);
/**
* Returns the SSL keystore URL.
* @return the sslKeystoreUrl
*/
public URL getSslKeystoreUrl();
/**
* Sets the sslKeystoreUrl field of this Connection
.
*
An SSL keystore is a password-protected database intended to contain private key
* material, such as private keys and their associated private key certificates. The private key
* in your keystore is used for encrypting/signing outgoing messages that can then only be
* decrytped using your public key which you have distributed to your partners. For your
* convenience, the IMS Connect API allows both private keys (and their associated private key
* certificates) as well as trusted certificates (usually stored in your truststore) to be stored
* in the same keystore in which case the sslTruststoreInputStream, sslTruststoreUrl and/or
* sslTruststoreName property can either be null or can point to the keystore InputStream, URL or
* file, respectively. Likewise, private keys or certificates could be stored in the truststore
* along with your trusted certificates, in which case the sslKeystoreInputStream, sslKeystoreUrl
* and/or sslKeystoreName properties could either be null or could point to the same or a different
* keystore or truststore InputStream, URL or file, respectively.
*
The sslKeystoreUrl property can be used to specify a URL that wraps a JKS keystore file. When
* TmInteraction.execute() is called, if a value is specified for the sslKeystoreName, the
* sslKeystoreUrl and the sslKeystoreInputStream, the sslKeystoreInputStream value takes precedence
* and will be used by the Connect API to load the keystore. If the sslKeystoreInputStream value is
* null, the sslKeystoreUrl value, if non-null, will be used. Only when both the
* sslKeystoreInputStream and sslKeystoreUrl values are null, will the sslKeystoreName be used. If
* a value for this property is not set by the client application, the default value (null) will be
* used for this property.
* @param anSslKeystoreUrl a java.net.URL
to be used to set the sslKeystoreUrl value for
* this interaction
* @see ApiProperties#DEFAULT_SSL_KEYSTORE_URL
* @see Connection#setSslKeystoreName(String anSslKeystoreName)
*/
public void setSslKeystoreUrl(URL anSslKeystoreUrl);
/**
* Returns the SSL keystore name as a String
.
* @return the sslKeystoreName.
*/
public String getSslKeystoreName();
/**
* Sets the sslKeystoreName field of this Connection
.
*
An SSL keystore is a password-protected database intended to contain private key
* material, such as private keys and their associated private key certificates. The private key
* in your keystore is used for encrypting/signing outgoing messages that can then only be
* decrytped using your public key which you have distributed to your partners. For your
* convenience, the IMS Connect API allows both private keys (and their associated private key
* certificates) as well as trusted certificates (usually stored in your truststore) to be stored
* in the same keystore in which case the sslTruststoreInputStream, sslTruststoreUrl and/or
* sslTruststoreName property can either be null or can point to the keystore InputStream, URL or
* file, respectively. Likewise, private keys or certificates could be stored in the truststore
* along with your trusted certificates, in which case the sslKeystoreInputStream, sslKeystoreUrl
* and/or sslKeystoreName properties could either be null or could point to the same or a different
* keystore or truststore InputStream, URL or file, respectively.
*
The sslKeystoreName property can be used to specify either a JKS keystore or, when running on
* z/OS, a RACF keyring. For non-z/OS platforms, specify the fully-qualified path name of your JKS
* keystore file. For z/OS, you can specify either the name of your JKS keystore file or a special
* string that provides the information needed to access your RACF keyring.
*
An example of a fully-qualified path name of your JKS keystore file is "c:\keystore\MyKeystore.ks".
*
A RACF keyring is specified as: "keystore_type:keyring_name:racfid". The keystore_type must be
* either JCERACFKS when software encryption is used for SSL or JCE4758RACFKS if hardware encryption
* is used. Replace keyring_name with the name of the RACF keyring that you are using as your
* keystore and racfid with a RACF ID that is authorized to access the specified keyring. Examples of
* RACF keyring specifications are "JCERACFKS:myKeyring:kruser01" or "JCE4758RACFKS:myKeyring:kruser01".
*
When running on z/OS, if the sslKeystoreName matches the above RACF keyring format, the IMS
* Connect API will use the specified RACF keyring as its keystore. If the keystore type specified is
* anything other than JCERACFKS or JCE4758RACFKS, the IMS Connect API attempts to interpret the
* sslKeystoreName specified as the name and location of a JKS keystore file.
*
When TmInteraction.execute() is called, if a value is specified for the sslKeystoreName, the
* sslKeystoreUrl and the sslKeystoreInputStream, the sslKeystoreInputStream value takes precedence
* and will be used by the Connect API to load the keystore. If the sslKeystoreInputStream value is
* null, the sslKeystoreUrl value, if non-null, will be used. Only when both the
* sslKeystoreInputStream and sslKeystoreUrl values are null, will the sslKeystoreName be used. If
* a value for this property is not supplied by the client, nor are values supplied for the
* sslKeystoreInputStream or sslKeystoreUrl, the default value from the default value from the
* java_home\lib\security\cacerts file will be used.
*
Note that the JKS keystore file can have other file extensions; it does not have to use the
* ".ks" file name extension.
* @param aSslKeystoreName a java.lang.String
containing the name of the JKS keystore
* file to be used for this interaction
* @see ApiProperties#DEFAULT_SSL_KEYSTORE_NAME
* @throws ImsConnectApiException
*/
public void setSslKeystoreName(String aSslKeystoreName) throws ImsConnectApiException;
/**
* Returns the SSL keystore password as a String
.
* @return the sslKeystorePassword.
*/
public String getSslKeystorePassword();
/**
* Sets the sslKeystorePassword field of this Connection object. The sslKeystorePassword specifies the password
* sslKeystorePassword specifies the password for the JKS keystore pointed to by
* sslKeystoreInputStream, sslKeystoreUrl or sslKeystoreName.
*
If a value for this property is not supplied by the client, the default value
* ("keystrPw") will be used.
* @param aSslKeystorePassword a String
specifying the SSL keystore
* password to be used for this interaction
* @see ApiProperties#DEFAULT_SSL_KEYSTORE_PASSWORD
* @throws ImsConnectApiException
*/
public void setSslKeystorePassword(String aSslKeystorePassword) throws ImsConnectApiException;
/**
* Returns the SSL truststore InputStream
instance.
* @return the sslTruststoreInputStream
*/
public InputStream getSslTruststoreInputStream();
/**
* Sets the sslTruststoreInputStream field of this Connection
.
*
An SSL truststore is a password-protected database intended to contain certificates of
* trusted certificate authorities. These certificates are used to validate the authenticity of a
* public key certificate during the handshaking process. For your convenience, the IMS Connect
* API allows both private key material usually stored in a keystore as well as the trusted
* certificates usually stored in a truststore to be stored in the same truststore, in which case
* the sslKeystoreInputStream, sslKeytstoreUrl and/or sslKeystoreName properties can either be null
* or can point to the truststore InputStream, URL or file, respectively. Likewise, both private
* key material and trusted certificates could be stored in the same keystore, in which case the
* sslTruststoreInputStream, sslTruststoreUrl and/or sslTruststoreName properties could either be
* null or could point to the same or a different keystore or truststore InputStream, URL or file,
* respectively.
*
The sslTruststoreInputStream property can be used to specify an InputStream that wraps a JKS
* keystore file which will be used as a keystore which contains trusted certificates. When
* TmInteraction.execute() is called, if a value is specified for the sslTruststoreName, the
* sslTruststoreUrl and the sslTruststoreInputStream, the sslTruststoreInputStream value takes
* precedence and will be used by the Connect API to load the truststore. If the
* sslTruststoreInputStream value is null, the sslTruststoreUrl value, if non-null, will be used.
* Only when both the sslTruststoreInputStream and sslTruststoreUrl values are null, will the
* sslTruststoreName be used. If a value for this property is not supplied by the client, the
* default value (null) will be used for this property.
*
It is important to note that an InputStream, because it does not support the mark or reset
* features, can only be used once. In fact, due to this restriction, the API internally closes an
* InputStream after it has loaded the truststore from that InputStream.
* @param anSslTruststoreInputStream an java.io.InputStream
specifying the SSL truststore
* to be used for this interaction.
* @see ApiProperties#DEFAULT_SSL_TRUSTSTORE_INPUT_STREAM
* @see Connection#setSslKeystoreName(String anSslKeystoreName)
*/
public void setSslTruststoreInputStream(InputStream anSslTruststoreInputStream);
/**
* Returns the SSL truststore URL
.
* @return the sslTruststoreUrl
*/
public URL getSslTruststoreUrl();
/**
* Sets the sslTruststoreUrl field of this Connection
.
*
An SSL truststore is a password-protected database intended to contain certificates of
* trusted certificate authorities. These certificates are used to validate the authenticity of a
* public key certificate during the handshaking process. For your convenience, the IMS Connect
* API allows both private key material usually stored in a keystore as well as the trusted
* certificates usually stored in a truststore to be stored in the same truststore, in which case
* the sslKeystoreInputStream, sslKeytstoreUrl and/or sslKeystoreName properties can either be null
* or can point to the truststore InputStream, URL or file, respectively. Likewise, both private
* key material and trusted certificates could be stored in the same keystore, in which case the
* sslTruststoreInputStream, sslTruststoreUrl and/or sslTruststoreName properties could either be
* null or could point to the same or a different keystore or truststore InputStream, URL or file,
* respectively.
*
The sslTruststoreUrl property can be used to specify a URL that wraps a JKS keystore file.
* When TmInteraction.execute() is called, if a value is specified for the sslTruststoreName, the
* sslTruststoreUrl and the sslTruststoreInputStream, the sslTruststoreInputStream value takes
* precedence and will be used by the Connect API to load the truststore. If the
* sslTruststoreInputStream value is null, the sslTruststoreUrl value, if non-null, will be used.
* Only when both the sslTruststoreInputStream and sslTruststoreUrl values are null, will the
* sslTruststoreName be used. If a value for this property is not supplied by the client, the
* default value (null) will be used for this property.
* @param anSslTruststoreUrl an java.net.URL
specifying the SSL truststore to be used for
* used for this interaction.
* @see ApiProperties#DEFAULT_SSL_TRUSTSTORE_URL
* @see Connection#setSslTruststoreName(String anSslTruststoreName)
*/
public void setSslTruststoreUrl(URL anSslTruststoreUrl);
/**
* Returns the SSL truststore name as a String
.
* @return the sslTruststoreName.
*/
public String getSslTruststoreName();
/**
* Sets the sslTruststoreName field of this Connection object.
*
An SSL truststore is a password-protected database intended to contain certificates of
* trusted certificate authorities. These certificates are used to validate the authenticity of a
* public key certificate during the handshaking process. For your convenience, the IMS Connect
* API allows both private key material in a keystore as well as the trusted certificates usually
* stored in a truststore to be stored in the same truststore, in which case the sslKeystoreInputStream,
* sslKeytstoreUrl and/or sslKeystoreName properties can either be null or can point to the
* truststore InputStream, URL or file, respectively. Likewise, both private key material and
* trusted certificates could be stored in the same keystore, in which case the
* sslTruststoreInputStream, sslTruststoreUrl and/or sslTruststoreName properties could either be
* null or could point to the same or a different keystore or truststore InputStream, URL or file,
* respectively.
*
The same format is used for the values of the sslKeystoreName and sslTruststoreName properties.
* See the description of {@link ConnectionFactory#setSslKeystoreName(String)} for a discussion of
* this format. The sslTruststoreName property can either be empty (all blanks) or can point to the
* keystore file.
*
The sslTruststoreName property can be used to point to a JKS trusted keystore file.
* When TmInteraction.execute() is called, if a value is specified for the sslTruststoreName, the
* sslTruststoreUrl and the sslTruststoreInputStream, the sslTruststoreInputStream value takes
* precedence and will be used by the Connect API to load the truststore. If the
* sslTruststoreInputStream value is null, the sslTruststoreUrl value, if non-null, will be used.
* Only when both the sslTruststoreInputStream and sslTruststoreUrl values are null, will the
* sslTruststoreName be used.
*
If a value for this property is not supplied by the client, nor are values supplied for the
* sslKeystoreInputStream or sslKeystoreUrl, the default value from the
* java_home\lib\security\cacerts file will be used.
*
Note that the JKS truststore file can have other file extensions; it does not have to use
* the ".ks" file name extension.
* @param anSslTrustStoreName a java.lang.String
containing the name of the JKS trust
* store file to be used for this interaction
* @see ApiProperties#DEFAULT_SSL_TRUSTSTORE_NAME
* @see Connection#setSslKeystoreName(String anSslKeystoreName)
* @throws ImsConnectApiException
*/
//Old keystore default location: c:\\MySSLKeystores\\myKeystore.ks
public void setSslTruststoreName(String anSslTruststoreName) throws ImsConnectApiException;
/**
* Returns the SSL trust store password as a String
.
* @return the sslTruststorePassword.
*/
public String getSslTruststorePassword();
/**
* Sets the sslTruststorePassword field of this Connection object which specifies the
* password for the JKS truststore pointed to by sslTruststoreName. This value is ignored if
* sslTruststoreName is null or blanks or if sslTruststoreName points to a RACF keyring. The
* sslTruststorePassword value is the password for the truststore file specified in the
* truststore pointed to by the sslTruststoreName value.
*
If a value for this property is not supplied by the client, the default value
* of "sslTruststorePassword" will be used.
* @param aSslTruststorePassword the JKS truststore password to be used for this interaction.
* @see ApiProperties#DEFAULT_SSL_TRUSTSTORE_PASSWORD
* @throws ImsConnectApiException
*/
public void setSslTruststorePassword(String aSslTruststorePassword) throws ImsConnectApiException;
/**
* Obtains the list of locally supported cipher suites at the specified encryption
* level, which can be used during the SSL handshaking process while initializing
* the context for this SSL socket connection. The list obtained is then used to
* set the enabled cipher suites for this SSL socket before returning to the caller.
* @return list of supported optional cipher suites for the current sslSocket.
*/
public String[] getSupportedCipherSuites();
/**
* Gets the useSslConnection property value.
* If this method returns true
, the underlying connection is an SSL connection
* or will be when connected.
* @return true
if the connection uses SSL. Otherwise, false
.
*/
public boolean isUseSslConnection();
/**
* Configures this Connection
instance to connect to IMS Connect using an SSL connection.
* A true
value sets the connection as an SSL connection, while a false
* value indicates that the connection will be a non-SSL TCP/IP connection.
* If a value for this property is not supplied by the client, the default value of false
will
* be used.
* @param aUseSslConnection
* Set the input to true
to set the connection to be an SSL connection.
* Set the input to false
to set the connection to be an non-SSL connection.
* @see ApiProperties#DEFAULT_USE_SSL_CONNECTION
* @throws ImsConnectApiException
*/
public void setUseSslConnection(boolean aUseSslConnection) throws ImsConnectApiException;
}