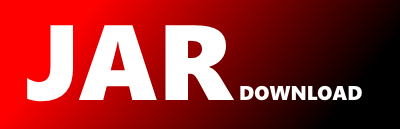
com.ibm.ims.connect.ConnectionAttributes Maven / Gradle / Ivy
Show all versions of IMSConnectAPI Show documentation
/*
* File: ConnectionAttributes.java
* ==========================================================================
* Licensed Material - Property of IBM
*
* IBM Confidential
*
* OCO Source Materials
*
* 5655-TDA
*
* (C) Copyright IBM Corp. 2009 All Rights Reserved.
*
* The source code for this program is not published or
* otherwise divested of its trade secrets, irrespective
* of what has been deposited with the U.S. Copyright
* Office.
*
* US Government Users Restricted Rights - Use, duplication or
* disclosure restricted by GSA ADP Schedule Contract with
* IBM Corp.
* ===========================================================================
*/
package com.ibm.ims.connect;
import java.io.InputStream;
import java.net.URL;
/**
* This class represents the configuration of a connection.
*
*
*/
public class ConnectionAttributes
{
public static final String copyright =
"Licensed Material - Property of IBM "
+ "5655-TDA"
+ "(C) Copyright IBM Corp. 2009, 2013 All Rights Reserved. "
+ "US Government Users Restricted Rights - Use, duplication or "
+ "disclosure restricted by GSA ADP Schedule Contract with IBM Corp. ";
/*** Connection properties ***/
/**
* hostName is a String variable whose value is the hostname (or IP address???)
* of the target IMS Connect for this connection. The default value is
* "myHostNm". Values must be a 1 to 8 character String set to the correct
* hostname of the target IMS Connect (or a string containing the IP address
* of the target IMS Connect (e.g., nnn.nnn.nnn.nnn or nnnn.nnnn.nnnn.nnnn.nnnn.nnnn
* or some permutations of these)
*/
private String hostName = ApiProperties.DEFAULT_HOSTNAME;
/**
* portNumber is an int variable whose value is the port number of the
* target IMS Connect for this connection. The default value is
* 9999. Values must be a valid port number set to the port number
* on which the target IMS Connect is listening.
*/
private int portNumber = ApiProperties.DEFAULT_PORTNUMBER;
/**
* socketType is an int variable whose value is used to determine whether a
* socket connection is a transaction socket which can only be disconnected
* by IMS Connect (either after a single non-conversational IMS transaction
* interaction has completed, after an IMS conversation (most likely
* including multiple iterations of the conversation) has completed, or
* after IMS itself has terminated or after an error has occured) or a
* persistent socket (left connected by IMS Connect until a fatal error
* has occured on that connection while IMS Connect is processing an
* interaction or until a disconnect request has been received by IMS
* Connect.)
* valid values for socketType:
* SOCKET_TYPE_TRANSACTION
* SOCKET_TYPE_PERSISTENT
* DEFAULT_SOCKET_TYPE (SOCKET_TYPE_PERSISTENT)
*/
private byte socketType = ApiProperties.DEFAULT_SOCKET_TYPE;
/**
* clientID is a String variable whose value is used to identify a connection.
* This value can either be created by the client application or, if not
* specified (that is, the string is set to 1 to 8 blanks,) or if
* setGenerateClientID is set to "true," the clientID will be assigned by IMS
* Connect.
*/
private String clientId = ApiProperties.DEFAULT_CLIENTID;
/*** SSL properties ***/
/**
* sslEncryptionType is the Encryption Type for the SSL connection. It can have a value of
* "Strong" for strong encryption, i.e encryption with ciphers that have large key
* sizes or it can have a value of "Weak" for weak encryption with ciphers that have
* small key sizes, or "none" for no encryption.
*/
private byte sslEncryptionType = ApiProperties.DEFAULT_SSL_ENCRYPTIONTYPE;
/**
* sslKeystoreInputStream is an InputStream which wraps a keystore file which
* contains keys required during an SSL handshake. It usually holds public
* keys or certificates but it can also be used to store private keys for the
* client. If a value is specified for both the sslKeystoreName and the
* sslKeystoreInputStream, the sslKeystoreInputStream value will be used by
* the Connect API to load the keystore.
*/
private InputStream sslKeystoreInputStream = ApiProperties.DEFAULT_SSL_KEYSTORE_INPUT_STREAM;
/**
* The sslKeystoreUrl property can be used to specify an URL that points to a JKS keystore file
* which will override the sslKeystoreName property and be used to load the SSL keystore used by the
* API. When TmInteraction.execute() is called, if a value is specified for the sslKeystoreName,
* the sslKeystoreUrl and the sslKeystoreInputStream, the sslKeystoreInputStream value takes precedence
* and will be used by the Connect API to load the keystore. If the sslKeystoreInputStream value is
* null, the sslKeystoreUrl value, if non-null, will be used. Only when both the sslKeystoreInputStream
* and sslKeystoreUrl values are null, will the sslKeystoreName be used. If a value for this property is
* not set by the client application, the default value (null) will be used for this property.
*/
private java.net.URL sslKeystoreURL = ApiProperties.DEFAULT_SSL_KEYSTORE_URL;
/**
* sslKeystoreName is the filename (along with the fullpath) of a keyStore
* which contains keys required during the SSL handshake. It usually holds
* private keys or certificates but it can also be used to store private keys
* for the client.
*
*/
private String sslKeystoreName = ApiProperties.DEFAULT_SSL_KEYSTORE_NAME;
/**
* sslKeystorePassword is the password for the keyStore file which contains keys.
*/
private String sslKeystorePassword = ApiProperties.DEFAULT_SSL_KEYSTORE_PASSWORD;
/**
* sslTruststoreInputStream is an InputStream which wraps a truststore file which
* contains keys required during an SSL handshake. It usually holds public
* keys or certificates but it can also be used to store private keys for the
* client. If a value is specified for both the sslTruststoreName and the
* sslTruststoreInputStream, the sslTruststoreInputStream value will be used by
* the Connect API to load the truststore.
*/
private InputStream sslTruststoreInputStream = ApiProperties.DEFAULT_SSL_TRUSTSTORE_INPUT_STREAM;
/**
* The sslTruststoreUrl property can be used to specify an URL that points to a JKS keystore
* file which will override the sslTruststoreName property and be used to load the SSL truststore
* used by the API.
*/
private URL sslTruststoreURL = ApiProperties.DEFAULT_SSL_TRUSTSTORE_URL;
/**
* sslTruststoreName is the filename (along with the fullpath) of a keyStore which
* contains keys required during the SSL handshake. It usually holds private keys
* for the client but it can also be used to store private keys or certificates.
*/
private String sslTruststoreName = ApiProperties.DEFAULT_SSL_TRUSTSTORE_NAME;
/**
* sslTruststorePassword is the password for the keyStore file which contains
* keys for trusted entities.
*/
private String sslTruststorePassword = ApiProperties.DEFAULT_SSL_TRUSTSTORE_PASSWORD;
/**
* useSslConnection is a Boolean variable whose value indictes whether or not this
* connection is an SSL connection. A true value indicates that this connection is
* or will be an SSL connection while a false value indicates that SSL is not being
* or will not be used.
*/
private boolean useSslConnection = ApiProperties.DEFAULT_USE_SSL_CONNECTION;
/*** Timeout properties ***/
/*
* The value of interactionTimeout will be set automatically during an TmInteraction.execute()
* call to the value of the interactionTimeout property of that TmInteraction instance. This
* value will be used to control the amount of time that the API will wait for a response from
* IMS Connect for interactions such as a resumeTpipe or receive interaction. Note that a
* receive interaction is invoked internally as part of a sendreceive interaction.
*/
private int interactionTimeout = ApiProperties.DEFAULT_INTERACTION_TIMEOUT;
/*
* The value of socketConnectTimeout will be used internally by the API during a
* Connection.connect() call set the time that TCP/IP should wait for a socket connect request
* to complete successfully. However it should be noted that this value is used by TCP/IP in
* conjunction with the TCP/IP maximum socket connect retries value (which may or may not be
* user-customizable depending on runtime platform) to determine the amount of time that
* TCP/IP will actually wait for a socket connect request to complete successfully. Whichever
* event occurs first, the socket connect timeout or the maximum socket connect retries
* exceeded will determine when the connect attemp will be aborted by TCP/IP and an error
* returned to the calling program.
*/
private int socketConnectTimeout = ApiProperties.DEFAULT_SOCKET_CONNECT_TIMEOUT;
/** Default constructor
*
*/
public ConnectionAttributes()
{
// Instantiates ConnectionAttributes using the default values specified in the field declarations
}
public ConnectionAttributes(String aConnectionAttributesFileName)
throws Exception
{
loadConnectionAttributesFromFile(aConnectionAttributesFileName);
}
/**
* Populates ConnectionAttributes properties with values read in from a connection
* attributes file
*
*/
public void loadConnectionAttributesFromFile(String aConnectionAttributesFileName)
throws Exception
{
PropertiesFileLoader myPropertiesFileLoader = new PropertiesFileLoader();
myPropertiesFileLoader.loadPropertiesFile(this, aConnectionAttributesFileName);
}
// Getter and setter methods
/**
* Gets the clientId property value.
* @return the clientId property value as a String
.
* @see Connection#setClientId(String)
*/
public String getClientId()
{
return this.clientId;
}
/**
* Sets the clientId field of this Connection
object, which is used by IMS Connect and IMS
* to identify IMS Connect clients. The clientId is also used for the names of asynchronous message queues
* (OTMA Tpipes) and other internal data structures in IMS Connect and OTMA.
* The clientId value is a string of 1 to 8 uppercase alphanumeric (A through Z, 0 to 9) or special
* (@, #, $) characters, left justified, and padded with blanks.
*
This value can be created by the client application or assigned
* by IMS Connect. If, on the first input message of a transaction, this string is not supplied by the client
* or if the value supplied is all blanks, or if setGenerateClientID is set to true, the clientID will be
* assigned by IMS Connect.
*
The clientID value will only be used by the API and IMS Connect
* while creating a connection. After the connection has been created, the clientID value specified by the
* client for subsequent interactions on that connection including re-use of the connection by other clients
* will be ignored by IMS Connect. The API will pass the specified clientID in the input message but IMS
* Connect will return the correct clientID (the original clientID for that connection) in the response message.
* @param aClientId the clientID to be used for this interaction.
* @see ApiProperties#DEFAULT_CLIENTID
* @see Connection#getClientId()
*/
public void setClientId(String aClientId)
{
this.clientId = aClientId;
}
/**
* Gets the hostName property value.
* @return the hostName property value as a String
.
* @see Connection#setHostName(String)
*/
public String getHostName()
{
return this.hostName;
}
/**
* Sets the value of the hostName field of this Connection
. The hostName property is a String
* variable whose value is the hostname or IP address of the target IMS Connect for this connection.
*
The input String
must be either:
*
* - a 1 to 8 character
String
set to the correct hostname of the target IMS Connect, or
* - a
String
containing the IP V4 or IP V6 address of the target IMS Connect
* (e.g. "nnn.nnn.nnn.nnn" or "nnn.nnn.nnn.nnn.nnn.nnn").
*
* If a value for this property is not supplied by the client, the default value ("HOSTNAME") will be used.
* @param aHostName the hostName or IP address to be used for this interaction.
* @see ApiProperties#DEFAULT_HOSTNAME
* @see Connection#getHostName()
*/
public void setHostName(String aHostName)
{
this.hostName = aHostName;
}
/**
* Gets the interactionTimeout property value.
* @return the interactionTimeout property value as an int
in milliseconds.
* @see Connection#setInteractionTimeout(int)
*/
public int getInteractionTimeout()
{
return this.interactionTimeout;
}
/**
* Sets the interactionTimeout field of this Connection
instance and the
* SO_TIMEOUT value of the underlying socket.
*
The interaction timeout value is in milliseconds. A non-zero value for
* the interaction timeout sets the SO_TIMEOUT value to that number of milliseconds. A timeout
* value of zero disables the SO_TIMEOUT function. If disabled, a socket will block forever
* if a read is executed on that socket and nothing is ever available to be read on that
* socket by the IMS Connect API on behalf of the client application.
*
* @param anInteractionTimeout the interaction timeout value in milliseconds. You can
* specify this parameter by using the constants defined in ApiProperties or by providing
* a valid non-negative integer value.
* @see ApiProperties#TIMEOUT_1_MILLISECOND
* @see ApiProperties#TIMEOUT_2_MILLISECONDS
* @see ApiProperties#TIMEOUT_5_MILLISECONDS
* @see ApiProperties#TIMEOUT_10_MILLISECONDS
* @see ApiProperties#TIMEOUT_20_MILLISECONDS
* @see ApiProperties#TIMEOUT_50_MILLISECONDS
* @see ApiProperties#TIMEOUT_100_MILLISECONDS
* @see ApiProperties#TIMEOUT_200_MILLISECONDS
* @see ApiProperties#TIMEOUT_500_MILLISECONDS
* @see ApiProperties#TIMEOUT_1_SECOND
* @see ApiProperties#TIMEOUT_2_SECONDS
* @see ApiProperties#TIMEOUT_5_SECONDS
* @see ApiProperties#TIMEOUT_10_SECONDS
* @see ApiProperties#TIMEOUT_30_SECONDS
* @see ApiProperties#TIMEOUT_45_SECONDS
* @see ApiProperties#TIMEOUT_1_MINUTE
* @see ApiProperties#TIMEOUT_2_MINUTES
* @see ApiProperties#TIMEOUT_5_MINUTES
* @see ApiProperties#TIMEOUT_10_MINUTES
* @see ApiProperties#TIMEOUT_30_MINUTES
* @see ApiProperties#TIMEOUT_45_MINUTES
* @see ApiProperties#TIMEOUT_1_HOUR
* @see ApiProperties#TIMEOUT_2_HOURS
* @see ApiProperties#TIMEOUT_4_HOURS
* @see ApiProperties#TIMEOUT_8_HOURS
* @see ApiProperties#TIMEOUT_12_HOURS
* @see ApiProperties#TIMEOUT_1_DAY
* @see ApiProperties#TIMEOUT_2_DAYS
* @see ApiProperties#TIMEOUT_5_DAYS
* @see ApiProperties#TIMEOUT_1_WEEK
* @see ApiProperties#TIMEOUT_2_WEEKS
* @see ApiProperties#INTERACTION_TIMEOUT_MAX
* @see ApiProperties#TIMEOUT_WAIT_FOREVER
* @see Connection#getInteractionTimeout()
*/
public void setInteractionTimeout(int anInteractionTimeout)
{
this.interactionTimeout = anInteractionTimeout;
}
/**
* Gets the portNumber property value.
* @return the portNumber value.
* @see Connection#setPortNumber(int)
*/
public int getPortNumber()
{
return this.portNumber;
}
/**
* Sets the value of the portNumber field of this Connection object. The portNumber property specifies
* a TCP/IP port where the target IMS Connect is listening for requests for
* new connections from clients.
*
If a value for this property is not supplied by the client, the default value (9999) will be used.
* @param aPortNumber a valid port number on which the target IMS Connect is listening for incoming
* requests from IMS Connnect clients.
* @see ApiProperties#DEFAULT_PORTNUMBER
* @see Connection#getPortNumber()
*/
public void setPortNumber(int aPortNumber)
{
this.portNumber = aPortNumber;
}
/**
* Gets the socketConnectTimeout property value.
* @return the socketConnectTimeout property value as an int
in milliseconds.
* @see Connection#setSocketConnectTimeout(int)
*/
public int getSocketConnectTimeout()
{
return this.socketConnectTimeout;
}
/**
* Sets the socketConnectTimeout field of this Connection
.
*
The socketConnectTimeout determines the amount of time that the IMS Connect API will wait for
* a socket.connect() call to complete. Only one value can be specified per socket. The value
*
The valid input values, for some of which, constants are defined in ApiProperties, are:
*
* - -1 - API will wait forever (or until the connect request times out due to exceeding the maximum
* number of connection retry attempts defined in the TCP/IP properties.)
*
- > 0 - API will wait either until the maximum number of connection retry attempts defined in the
* TCP/IP properties has been attempted or until the socketConnectTimeout period has elapsed before
* throwing a java.net.SocketTimeoutException.
*
* Specifying a value less than zero will result in an ImsConnectCommunicationException being thrown
* back to the client application. It should be noted that the actual timeout may be affected by the
* maximum number of connect retries allowed by TCP/IP. If the maximum number of connect retries is
* exceeded before the timeout pops, an exception will be thrown by TCP/IP.
* @param aSocketConnectTimeout the socketConnectTimeout value to set in milliseconds
* @see ApiProperties#DEFAULT_SOCKET_CONNECT_TIMEOUT
* @see ApiProperties#TIMEOUT_1_MILLISECOND
* @see ApiProperties#TIMEOUT_2_MILLISECONDS
* @see ApiProperties#TIMEOUT_5_MILLISECONDS
* @see ApiProperties#TIMEOUT_10_MILLISECONDS
* @see ApiProperties#TIMEOUT_20_MILLISECONDS
* @see ApiProperties#TIMEOUT_50_MILLISECONDS
* @see ApiProperties#TIMEOUT_100_MILLISECONDS
* @see ApiProperties#TIMEOUT_200_MILLISECONDS
* @see ApiProperties#TIMEOUT_500_MILLISECONDS
* @see ApiProperties#TIMEOUT_1_SECOND
* @see ApiProperties#TIMEOUT_2_SECONDS
* @see ApiProperties#TIMEOUT_5_SECONDS
* @see ApiProperties#TIMEOUT_10_SECONDS
* @see ApiProperties#TIMEOUT_30_SECONDS
* @see ApiProperties#TIMEOUT_45_SECONDS
* @see ApiProperties#TIMEOUT_1_MINUTE
* @see ApiProperties#TIMEOUT_2_MINUTES
* @see ApiProperties#TIMEOUT_5_MINUTES
* @see ApiProperties#TIMEOUT_10_MINUTES
* @see ApiProperties#TIMEOUT_30_MINUTES
* @see ApiProperties#TIMEOUT_45_MINUTES
* @see ApiProperties#TIMEOUT_1_HOUR
* @see ApiProperties#TIMEOUT_2_HOURS
* @see ApiProperties#TIMEOUT_4_HOURS
* @see ApiProperties#TIMEOUT_8_HOURS
* @see ApiProperties#TIMEOUT_12_HOURS
* @see ApiProperties#TIMEOUT_1_DAY
* @see ApiProperties#TIMEOUT_2_DAYS
* @see ApiProperties#TIMEOUT_5_DAYS
* @see ApiProperties#TIMEOUT_1_WEEK
* @see ApiProperties#TIMEOUT_2_WEEKS
* @see ApiProperties#TIMEOUT_WAIT_FOREVER
* @see ApiProperties#SOCKET_CONNECT_TIMEOUT_USE_TCPIP_DEFAULTS
* @see Connection#getSocketConnectTimeout()
*/
public void setSocketConnectTimeout(int aSocketConnectTimeout)
{
this.socketConnectTimeout = aSocketConnectTimeout;
}
/**
* Gets the socketType property value.
* @return the socketType value.
* @see ApiProperties#SOCKET_TYPE_PERSISTENT
* @see ApiProperties#SOCKET_TYPE_TRANSACTION
* @see Connection#setSocketType(byte)
*/
public byte getSocketType()
{
return this.socketType;
}
/**
* Sets the socketType field of this Connection
.
*
The type of socket connection of a message is set by the client in the initial input message and
* maintained in the message until the interaction has completed. Only one value can be specified per
* interaction.
*
The valid input values, for which the following constants are defined in ApiProperties, are:
*
* - SOCKET_TYPE_TRANSACTION = 0x00. A transaction socket can only be disconnected by IMS Connect.
* Transaction sockets are used for one transaction (including and required ACKs or NAKs) for non-conversational
* transactions or for one complete conversation (until the conversation is ended by the client or host application)
* for conversational transactions. Once the non-conversational IMS transaction interaction or IMS conversation
* has completed, the transaction socket is automatically closed by both IMS Connect and the API. The
* transaction socket also closes automatically after IMS itself has terminated or after an error has occured.
*
- SOCKET_TYPE_PERSISTENT = 0x10. A persistent socket is left connected by IMS Connect until a fatal error
* has occurred on that connection while IMS Connect is processing an interaction, or until a disconnect request
* has been received by IMS Connect. Any open persistent connections will be closed automatically by the API
* when the client application ends.
*
- DEFAULT_SOCKET_TYPE (same as SOCKET_TYPE_PERSISTENT)
*
* If a value for this property is not supplied by the client, the default value (SOCKET_TYPE_PERSISTENT)
* will be used.
* @param aSocketType the socket type to be used for this interaction. Use the constants defined
* in ApiProperties to specify this parameter.
* @see ApiProperties#DEFAULT_SOCKET_TYPE
* @see ApiProperties#SOCKET_TYPE_PERSISTENT
* @see ApiProperties#SOCKET_TYPE_TRANSACTION
* @see Connection#getSocketType()
*/
public void setSocketType(byte aSocketType)
{
this.socketType = aSocketType;
}
/**
* Returns the SSL encryption type property.
* @return the sslEncryptionType.
* @see Connection#setSslEncryptionType(byte)
*/
public byte getSslEncryptionType()
{
return this.sslEncryptionType;
}
/**
* Sets the sslEncryptionType field of this Connection
. The sslEncryptionType property is a byte
* value used to set the encryption type for the underlying connection when that connnection is an SSL
* connection.
*
The valid values, for which the following constants are defined in ApiProperties
, are:
*
* - SSL_ENCRYPTIONTYPE_STRONG = 0x02
*
- SSL_ENCRYPTIONTYPE_WEAK = 0x01
*
- SSL_ENCRYPTYPE_NONE = 0x00
*
- DEFAULT_SSL_ENCRYPTIONTYPE (same as SSL_ENCRYPTIONTYPE_WEAK)
*
* If a value for this property is not supplied by the client, the default value (SSL_ENCRYPTIONTYPE_WEAK)
* will be used.
*
SSL_ENCRYPTIONTYPE_STRONG and SSL_ENCRYPTIONTYPE_WEAK reflect the strength of the cipher used which
* is related to the key length. All those ciphers that can be used for export are in the
* SSL_ENCRYPTIONTYPE_WEAK category and the rest go into the strong category. The IMS Connect API will
* negotiate with IMS Connect to use a strong (greater than 64-bit encryption key) or weak (64-bit
* or smaller encryption key) cipher specification accordingly if either of these two settings are specified.
*
When SSL_ENCRYPTIONTYPE_NONE is specified, the IMS Connect API will negotiate with IMS Connect to
* use a cipher spec whose name contains the string "NULL". Null encryption will allow for
* authentication to take place during the SSL handshaking process. Once the handshaking process for a
* socket has completed including authentication as required, all messages will flow in the clear (without
* encryption) over that socket.
* @param anSslEncryptionType the sslEncryptionType to be used for this interaction. Use the constants defined
* in ApiProperties to specify this parameter.
* @see Connection#getSslEncryptionType()
* @see ApiProperties#DEFAULT_SSL_ENCRYPTIONTYPE
* @see ApiProperties#SSL_ENCRYPTIONTYPE_NONE
* @see ApiProperties#SSL_ENCRYPTIONTYPE_STRONG
* @see ApiProperties#SSL_ENCRYPTIONTYPE_WEAK
* @see Connection#getSslEncryptionType()
*/
public void setSslEncryptionType(byte anSslEncryptionType)
{
this.sslEncryptionType = anSslEncryptionType;
}
/**
* Returns the SSL keystore InputStream
instance.
* @return the sslKeystoreInputStream
* @see Connection#setSslKeystoreInputStream(InputStream)
*/
public InputStream getSslKeystoreInputStream(){
return this.sslKeystoreInputStream;
}
/**
* Sets the sslKeystoreInputStream field of this Connection
.
*
An SSL keystore is a password-protected database that contains private key material, such as private keys
* and their associated private key certificates. The private key in your keystore is used for encrypting
* or signing outgoing messages that can be decrypted using only the public key that you distribute.
*
The IMS Connect API allows private keys, their associated private key certificates, and trusted certificates
* (usually stored in your truststore) to be stored in the same keystore. The sslTruststoreInputStream,
* sslTruststoreUrl, or sslTruststoreName property can either be null
or point to the keystore
* InputStream, URL, or file, respectively.
*
Likewise, you can store private keys or certificates in the truststore along with your
* trusted certificates, and the sslKeystoreInputStream, sslKeystoreUrl, or sslKeystoreName properties
* can either be null or point to the same or a different keystore or truststore InputStream, URL, or file,
* respectively.
*
Use the sslKeystoreInputStream property to specify an InputStream
instance that wraps a JKS keystore
* file.
*
When TmInteraction.execute
is called:
*
* - If a non-null value is specified for sslKeystoreInputStream, its value takes precedence over values specified
* for sslKeystoreUrl and sslKeystoreName.
*
- If the sslKeystoreInputStream value is null, the sslKeystoreUrl value, if non-null, will be used.
*
- If both the sslKeystoreInputStream and sslKeystoreUrl values are null, the sslKeystoreName be used.
*
* If a value for this property is not set by the client application, the default value (null
) will be used.
*
Note that an InputStream
does not support the mark or reset features and can only be used once.
* Due to this restriction, the API internally closes an InputStream
after loading the keystore from that InputStream.
* @param sslKeystoreInputStream a java.io.InputStream
instance specifying the SSL keystore to use for this interaction.
* @see ApiProperties#DEFAULT_SSL_KEYSTORE_INPUT_STREAM
* @see Connection#getSslKeystoreInputStream()
* @see Connection#setSslKeystoreUrl(URL)
* @see Connection#setSslKeystoreName(String)
*/
public void setSslKeystoreInputStream(InputStream sslKeystoreInputStream){
this.sslKeystoreInputStream = sslKeystoreInputStream;
}
/**
* Returns the SSL keystore URL.
* @return the sslKeystoreUrl
* @see Connection#setSslKeystoreUrl(URL)
*/
public URL getSslKeystoreUrl() {
return this.sslKeystoreURL;
}
/**
* Sets the sslKeystoreUrl field of this Connection
.
*
An SSL keystore is a password-protected database that contains private key material, such as private keys
* and their associated private key certificates. The private key in your keystore is used for encrypting
* or signing outgoing messages that can be decrypted using only the public key that you distribute.
*
The IMS Connect API allows private keys, their associated private key certificates, and trusted certificates
* (usually stored in your truststore) to be stored in the same keystore. The sslTruststoreInputStream,
* sslTruststoreUrl, or sslTruststoreName property can either be null
or point to the keystore
* InputStream, URL, or file, respectively.
*
Likewise, you can store private keys or certificates in the truststore along with your
* trusted certificates, and the sslKeystoreInputStream, sslKeystoreUrl, or sslKeystoreName properties
* can either be null or point to the same or a different keystore or truststore InputStream, URL, or file,
* respectively.
*
Use the sslKeystoreUrl property to specify a URL that wraps a JKS keystore file.
* When TmInteraction.execute
is called:
*
* - If a non-null value is specified for sslKeystoreInputStream, its value takes precedence over values specified
* for sslKeystoreUrl and sslKeystoreName.
*
- If the sslKeystoreInputStream value is null, the sslKeystoreUrl value, if non-null, will be used.
*
- If both the sslKeystoreInputStream and sslKeystoreUrl values are null, the sslKeystoreName be used.
*
* If a value for this property is not set by the client application, the default value (null
) will be used.
* @param anSslKeystoreUrl a java.net.URL
specifying the sslKeystoreUrl value for
* this interaction
* @see ApiProperties#DEFAULT_SSL_KEYSTORE_URL
* @see Connection#getSslKeystoreUrl()
* @see Connection#setSslKeystoreInputStream(InputStream)
* @see Connection#setSslKeystoreName(String)
*/
public void setSslKeystoreUrl(URL anSslKeystoreUrl) {
this.sslKeystoreURL = anSslKeystoreUrl;
}
/**
* Returns the SSL keystore name as a String
.
* @return the sslKeystoreName.
* @see Connection#setSslKeystoreName(String)
*/
public String getSslKeystoreName()
{
return this.sslKeystoreName;
}
/**
* Sets the sslKeystoreName field of this Connection
.
*
An SSL keystore is a password-protected database that contains private key material, such as private keys
* and their associated private key certificates. The private key in your keystore is used for encrypting
* or signing outgoing messages that can be decrypted using only the public key that you distribute.
*
The IMS Connect API allows private keys, their associated private key certificates, and trusted certificates
* (usually stored in your truststore) to be stored in the same keystore. The sslTruststoreInputStream,
* sslTruststoreUrl, or sslTruststoreName property can either be null
or point to the keystore
* InputStream, URL, or file, respectively.
*
Likewise, you can store private keys or certificates in the truststore along with your
* trusted certificates, and the sslKeystoreInputStream, sslKeystoreUrl, or sslKeystoreName properties
* can either be null or point to the same or a different keystore or truststore InputStream, URL, or file,
* respectively.
*
The sslKeystoreName property can be used to specify either a JKS keystore or a RACF keyring when
* running on z/OS. For non-z/OS platforms, specify the fully-qualified path name of your JKS keystore file.
* For z/OS, you can specify either the name of your JKS keystore file or a special string that provides the
* information needed to access your RACF keyring.
*
An example of a fully-qualified path name of your JKS keystore file is "c:\keystore\MyKeystore.ks".
*
A RACF keyring is specified as: "keystore_type:keyring_name:racfid". The keystore_type must be either
* JCERACFKS when software encryption is used for SSL or JCE4758RACFKS if hardware encryption is used.
* Replace keyring_name with the name of the RACF keyring that you are using as your keystore and racfid
* with a RACF ID that is authorized to access the specified keyring. Examples of RACF keyring specifications
* are "JCERACFKS:myKeyring:kruser01" or "JCE4758RACFKS:myKeyring:kruser01".
*
When running in z/OS, if the sslKeystoreName matches the above RACF keyring format, the IMS Connect
* API will use the specified RACF keyring as its keystore. If the keystore type specified is anything other
* than JCERACFKS or JCE4758RACFKS, the IMS Connect API attempts to interpret the sslKeystoreName specified as
* the name and location of a JKS keystore file.
*
Note that the JKS keystore file can have other file extensions; it does not have to have to use
* the '.ks' file name extension.
*
When TmInteraction.execute
is called:
*
* - If a non-null value is specified for sslKeystoreInputStream, its value takes precedence over values specified
* for sslKeystoreUrl and sslKeystoreName.
*
- If the sslKeystoreInputStream value is null, the sslKeystoreUrl value, if non-null, will be used.
*
- If both the sslKeystoreInputStream and sslKeystoreUrl values are null, the sslKeystoreName be used.
*
* If a value for this property is not supplied by the client, and values are not supplied for the
* sslKeystoreInputStream or sslKeystoreUrl, the default value {@link ApiProperties#DEFAULT_SSL_KEYSTORE_NAME} will be used.
* @param aSslKeystoreName a String
specifying the SSL keystore to be used for this interaction.
* @see ApiProperties#DEFAULT_SSL_KEYSTORE_NAME
* @see Connection#getSslKeystoreName()
* @see Connection#setSslKeystoreInputStream(InputStream)
* @see Connection#setSslKeystoreUrl(URL)
*/
public void setSslKeystoreName(String anSslKeystoreName)
{
this.sslKeystoreName = anSslKeystoreName;
}
/**
* Returns the SSL keystore password as a String
.
* @return the sslKeystorePassword.
* @see Connection#setSslKeystorePassword(String)
*/
public String getSslKeystorePassword()
{
return this.sslKeystorePassword;
}
/**
* Sets the sslKeystorePassword field of this Connection object. The sslKeystorePassword specifies the password
* for the JKS keystore pointed to by sslKeystoreName. This value is ignored if sslKeystoreName is null or blanks or
* if sslKeystoreName points to a RACF keyring.
*
If a value for this property is not supplied by the client, the default value ("sslKeystorePassword")
* will be used.
* @param anSslKeystorePassword a String
specifying the SSL truststore to be used for this interaction.
* @see ApiProperties#DEFAULT_SSL_KEYSTORE_PASSWORD
* @see Connection#getSslKeystorePassword()
*/
public void setSslKeystorePassword(String anSslKeystorePassword)
{
this.sslKeystorePassword = anSslKeystorePassword;
}
/**
* Returns the SSL truststore InputStream
instance.
* @return the sslTruststoreInputStream
* @see Connection#setSslTruststoreInputStream(InputStream)
*/
public InputStream getSslTruststoreInputStream()
{
return this.sslTruststoreInputStream;
}
/**
* Sets the sslTruststoreInputStream property of this Connection
.
*
An SSL keystore is a password-protected database that contains private key material, such as private keys
* and their associated private key certificates. The private key in your keystore is used for encrypting
* or signing outgoing messages that can be decrypted using only the public key that you distribute.
*
The IMS Connect API allows private keys, their associated private key certificates, and trusted certificates
* (usually stored in your truststore) to be stored in the same keystore. The sslTruststoreInputStream,
* sslTruststoreUrl, or sslTruststoreName property can either be null
or point to the keystore
* InputStream, URL, or file, respectively.
*
Likewise, you can store private keys or certificates in the truststore along with your
* trusted certificates, and the sslKeystoreInputStream, sslKeystoreUrl, or sslKeystoreName properties
* can either be null or point to the same or a different keystore or truststore InputStream, URL, or file,
* respectively.
*
Use the sslTruststoreInputStream property to specify an InputStream
instance that wraps
* a JKS keystore file specifying a keystore that contains trusted certificates.
* When TmInteraction.execute
is called:
*
* - If a non-null value is specified for sslTruststoreInputStream, its value takes precedence over values specified
* for sslTruststoreUrl and sslTruststoreName.
*
- If the sslTruststoreInputStream value is null, the sslTruststoreUrl value, if non-null, will be used.
*
- If both the sslTruststoreInputStream and sslTruststoreUrl values are null, the sslTruststoreName be used.
*
* If a value for this property is not supplied by the client, the default value (null
) will be used.
*
Note that an InputStream
does not support the mark or reset features and can only be used once.
* Due to this restriction, the API internally closes an InputStream
after loading the truststore from that InputStream.
* @param sslTruststoreInputStream a java.io.InputStream
instance specifying the SSL
* truststore to be used for this interaction.
* @see ApiProperties#DEFAULT_SSL_TRUSTSTORE_INPUT_STREAM
* @see Connection#getSslTruststoreInputStream()
* @see Connection#setSslTruststoreUrl(URL)
* @see Connection#setSslTruststoreName(String)
*/
public void setSslTruststoreInputStream(InputStream sslTruststoreInputStream)
{
this.sslTruststoreInputStream = sslTruststoreInputStream;
}
/**
* Returns the SSL truststore URL
.
* @return the sslTruststoreUrl
* @see Connection#setSslTruststoreURL
*/
public URL getSslTruststoreUrl() {
return this.sslTruststoreURL;
}
/**
* Sets the sslTruststoreUrl field of this Connection
.
*
An SSL keystore is a password-protected database that contains private key material, such as private keys
* and their associated private key certificates. The private key in your keystore is used for encrypting
* or signing outgoing messages that can be decrypted using only the public key that you distribute.
*
The IMS Connect API allows private keys, their associated private key certificates, and trusted certificates
* (usually stored in your truststore) to be stored in the same keystore. The sslTruststoreInputStream,
* sslTruststoreUrl, or sslTruststoreName property can either be null
or point to the keystore
* InputStream, URL, or file, respectively.
*
Likewise, you can store private keys or certificates in the truststore along with your
* trusted certificates, and the sslKeystoreInputStream, sslKeystoreUrl, or sslKeystoreName properties
* can either be null or point to the same or a different keystore or truststore InputStream, URL, or file,
* respectively.
*
Use the sslTruststoreUrl property to specify a URL that wraps a JKS keystore file.
* When TmInteraction.execute
is called:
*
* - If a non-null value is specified for sslTruststoreInputStream, its value takes precedence over values specified
* for sslTruststoreUrl and sslTruststoreName.
*
- If the sslTruststoreInputStream value is null, the sslTruststoreUrl value, if non-null, will be used.
*
- If both the sslTruststoreInputStream and sslTruststoreUrl values are null, the sslTruststoreName be used.
*
* If a value for this property is not supplied by the client, the default value (null
) will be used.
* @param anSslTruststoreUrl java.net.URL
instance specifying the SSL truststore for this interaction.
* @see ApiProperties#DEFAULT_SSL_TRUSTSTORE_URL
* @see Connection#getSslTruststoreURL
* @see Connection#setSslTruststoreInputStream(InputStream)
* @see Connection#setSslTruststoreName(String)
*/
public void setSslTruststoreUrl(URL anSslTruststoreUrl) {
this.sslTruststoreURL = anSslTruststoreUrl;
}
/**
* Returns the SSL truststore name as a String
.
* @return the sslTruststoreName.
* @see Connection#setSslTruststoreName(String)
*/
public String getSslTruststoreName()
{
return this.sslTruststoreName;
}
/**
* Sets the sslTruststoreName field of this Connection object.
*
An SSL keystore is a password-protected database that contains private key material, such as private keys
* and their associated private key certificates. The private key in your keystore is used for encrypting
* or signing outgoing messages that can be decrypted using only the public key that you distribute.
*
The IMS Connect API allows private keys, their associated private key certificates, and trusted certificates
* (usually stored in your truststore) to be stored in the same keystore. The sslTruststoreInputStream,
* sslTruststoreUrl, or sslTruststoreName property can either be null
or point to the keystore
* InputStream, URL, or file, respectively.
*
Likewise, you can store private keys or certificates in the truststore along with your
* trusted certificates, and the sslKeystoreInputStream, sslKeystoreUrl, or sslKeystoreName properties
* can either be null or point to the same or a different keystore or truststore InputStream, URL, or file,
* respectively.
*
Use the sslTruststoreName field to specify either a JKS keystore or a RACF keyring when running on z/OS. For non-z/OS platforms,
* specify the fully-qualified path name of your JKS truststore file. For z/OS, specify the JKS name or the RACF keyring used for the truststore.
*
The same format is used for the values of the sslKeystoreName and sslTruststoreName properties.
* See the description of {@link Connection#setSslKeystoreName(String)} for a discussion of this format.
* The sslTruststoreName property can either be empty (all blanks) or can point to the keystore file.
*
When TmInteraction.execute
is called:
*
* - If a non-null value is specified for sslTruststoreInputStream, its value takes precedence over values specified
* for sslTruststoreUrl and sslTruststoreName.
*
- If the sslTruststoreInputStream value is null, the sslTruststoreUrl value, if non-null, will be used.
*
- If both the sslTruststoreInputStream and sslTruststoreUrl values are null, the sslTruststoreName be used.
*
* If a value for this property is not supplied by the client, the default value {@link ApiProperties#DEFAULT_SSL_TRUSTSTORE_NAME}
* will be used.
*
Note that the JKS truststore file can have other file extensions; it does not have to have to use the '.ks'
* file name extension.
* @param anSslTruststoreName the sslTrustStoreName to be used for this interaction.
* @see Connection#getSslTruststoreName()
* @see Connection#setSslTruststoreInputStream(InputStream)
* @see Connection#setSslTruststoreUrl(URL)
*/
public void setSslTruststoreName(String anSslTruststoreName)
{
this.sslTruststoreName = anSslTruststoreName;
}
/**
* Returns the SSL trust store password as a String
.
* @return the sslTruststorePassword.
* @see Connection#setSslTruststorePassword(String)
*/
public String getSslTruststorePassword()
{
return this.sslTruststorePassword;
}
/**
* Sets the sslTruststorePassword field of this Connection object which specifies the
* password for the JKS truststore pointed to by sslTruststoreName. This value is ignored if
* sslTruststoreName is null or blanks or if sslTruststoreName points to a RACF keyring. The
* sslTruststorePassword value is the password for the truststore file specified in the
* truststore pointed to by the sslTruststoreName value.
*
If a value for this property is not supplied by the client, the default value
* of "sslTruststorePassword" will be used.
* @param anSslTruststorePassword the JKS truststore password to be used for this interaction.
* @see ApiProperties#DEFAULT_SSL_TRUSTSTORE_PASSWORD
* @see Connection#getSslTruststorePassword()
*/
public void setSslTruststorePassword(String anSslTruststorePassword)
{
this.sslTruststorePassword = anSslTruststorePassword;
}
/**
* Gets the useSslConnection property value.
* If this method returns true
, the underlying connection is an SSL connection
* or will be when connected.
* @return true
if the connection uses SSL. Otherwise, false
.
* @see Connection#setUseSslConnection(boolean)
*/
public boolean isUseSslConnection()
{
return this.useSslConnection;
}
/**
* Configures this Connection
instance to connect to IMS Connect using an SSL connection.
* A true
value sets the connection as an SSL connection, while a false
* value indicates that the connection will be a non-SSL TCP/IP connection.
* If a value for this property is not supplied by the client, the default value of false
will
* be used.
* @param aUseSslConnection
* Set the input to true
to set the connection to be an SSL connection.
* Set the input to false
to set the connection to be an non-SSL connection.
* @see ApiProperties#DEFAULT_USE_SSL_CONNECTION
* @see Connection#isUseSslConnection()
*/
public void setUseSslConnection(boolean aUseSslConnection)
{
this.useSslConnection = aUseSslConnection;
}
}