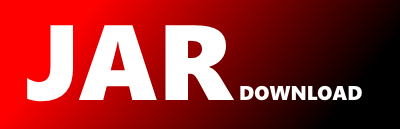
com.ibm.ims.connect.Hdr Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of IMSConnectAPI Show documentation
Show all versions of IMSConnectAPI Show documentation
API that allows Java applications to interface with IMS Connect
The newest version!
/**
* File: Hdr.java
* ==========================================================================
* Licensed Material - Property of IBM
*
* IBM Confidential
*
* OCO Source Materials
*
* 5655-TDA
*
* (C) Copyright IBM Corp. 2011, 2013 All Rights Reserved.
*
* The source code for this program is not published or
* otherwise divested of its trade secrets, irrespective
* of what has been deposited with the U.S. Copyright
* Office.
*
* US Government Users Restricted Rights - Use, duplication or
* disclosure restricted by GSA ADP Schedule Contract with
* IBM Corp.
* ===========================================================================
*/
package com.ibm.ims.connect;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.UnsupportedEncodingException;
import java.util.InvalidPropertiesFormatException;
import java.util.logging.*;
import java.util.Properties;
import java.util.Vector;
import org.xml.sax.Attributes;
/**
* Represents a hdr
element of a type-2 command response message. This element contains
* formatting information for the output data. One hdr
element is returned for each column
* in the response message data. The number and type of columns varies depending on the type-2 command that
* was issued.
*
*@since Enterprise Suite 2.1
*/
public interface Hdr
{
/**
* Gets the formatting attributes as XML.
* @return the elementText
*/
public String getHdrAttributesAsXmlString();
/**
* Gets the formatting attributes as a Properties object. You must specify the code page to use to render the attributes.
* @return the elementText
*/
public Properties getHdrElementAttributesAsPropertiesObject(String anEncoding)
throws ImsConnectApiException;
/**
* Gets any plain text encapsulated by the element.
* @return the elementText
*/
public String getElementText();
/**
* Gets the element data as a URI string.
* @return the uri
*/
public String getUri();
/**
* Gets the response message formatting attributes as an Attributes object.
* @return the attributes
*/
public Attributes getAttributes();
/**
* Gets the text from the slbl
element of the response message. This element contains
* the short label for the column.
* @return the slbl
*/
public String getSlbl();
/**
* Gets the text from the llbl
element of the response message. This element contains
* the long label for the column.
* @return the llbl
*/
public String getLlbl();
/**
* Gets the text from the scope
element of the response message. This element describes
* whether the column data is global (GBL) or local (LCL). Global information applies to other
* rows with the same resource name in different IMSPlex members, while local information applies to only one
* IMSPlex member.
* @return the scope
*/
public String getScope();
/**
* Gets the text from the sort
element of the response message. This element indicates whether the column data
* should be presented in ascending order (A), in descending order (D), or unsorted (N).
* @return the sort
*/
public String getSort();
/**
* Gets the text from the key
element of the response message. This element indicates the sort priority of
* the data in the column. You can use this value to determine the correct sort order for all of the columns in the response.
* The key values are:
*
* - 0 - This field is not sorted.
* - 1 - This field has the highest sort priority.
* - 2 - This field has the second highest sort priority.
* - n - This field has the nth highest sort priority.
*
* @return the key
*/
public String getKey();
/**
* Gets the text from the scroll
element of the response message. This element indicates if the column
* data is scrollable. If the element contains YES, the column can be scrolled off the screen if needed. If the element
* contains NO, the column data must be locked onto the screen. Typically, a column must be locked if it contains
* an identifier for other data columns in the response.
* @return the scroll
*/
public String getScroll();
/**
* Gets the text from the len
element of the response message. This element indicates the maximum length
* of the data returned in this column. You can use this information to determine how much space to allocate for
* the response data in a graphical display.
* @return the len
*/
public String getLen();
/**
* Gets the text from the dtype
element of the response message. This element indicates the original
* data type of the field. This value can be either CHAR for character data or INT for integer data.
* @return the dtype
*/
public String getDtype();
/**
* Gets the text from the skipb
element of the response message. This element indicates if the column
* should be omitted from a graphical display if none of the responding command processing clients returned any data for the column.
* If the element value is no
, the column should always be displayed.
* If the element value is yes
, the column can be omitted if no client returned any relevant data.
* @return the skipb
*/
public String getSkipb();
/**
* Gets the text from the align
element of the response message. This element contains the recommended
* visual alignment for the data in the column: right
, left
, or center
.
* @return the align
*/
public String getAlign();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy