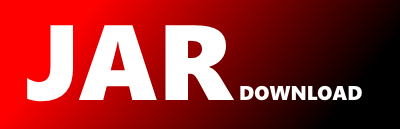
com.ibm.ims.connect.ImsConnectErrorMessage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of IMSConnectAPI Show documentation
Show all versions of IMSConnectAPI Show documentation
API that allows Java applications to interface with IMS Connect
The newest version!
/*
* File: ImsConnectErrorMessage.java
* ==========================================================================
* Licensed Material - Property of IBM
*
* IBM Confidential
*
* OCO Source Materials
*
* 5655-TDA
*
* (C) Copyright IBM Corp. 2009, 2013 All Rights Reserved.
*
* The source code for this program is not published or
* otherwise divested of its trade secrets, irrespective
* of what has been deposited with the U.S. Copyright
* Office.
*
* US Government Users Restricted Rights - Use, duplication or
* disclosure restricted by GSA ADP Schedule Contract with
* IBM Corp.
* ===========================================================================
*/
package com.ibm.ims.connect;
import java.util.*;
import java.text.MessageFormat;
/**
* This class defines a list of key constants which is used to identify the
* error messages. It loads a resource bundles according to the system locale
* and uses the key constant to load the corresponding error message from the
* resource bundles.
*/
public class ImsConnectErrorMessage
{
public static final String copyright =
"Licensed Material - Property of IBM "
+ "5655-TDA"
+ "(C) Copyright IBM Corp. 2009, 2013 All Rights Reserved. "
+ "US Government Users Restricted Rights - Use, duplication or "
+ "disclosure restricted by GSA ADP Schedule Contract with IBM Corp. ";
// public final static String HWS0000E = "HWS0000E";
public final static String HWS0001E = "HWS0001E";
public final static String HWS0002E = "HWS0002E";
public final static String HWS0003E = "HWS0003E";
public final static String HWS0004E = "HWS0004E";
public final static String HWS0005E = "HWS0005E";
public final static String HWS0006E = "HWS0006E";
public final static String HWS0007E = "HWS0007E";
public final static String HWS0008E = "HWS0008E";
public final static String HWS0009E = "HWS0009E";
public final static String HWS0010E = "HWS0010E";
public final static String HWS0011E = "HWS0011E";
public final static String HWS0012E = "HWS0012E";
public final static String HWS0015E = "HWS0015E";
public final static String HWS0016E = "HWS0016E";
public final static String HWS0017E = "HWS0017E";
public final static String HWS0018E = "HWS0018E";
public final static String HWS0019E = "HWS0019E";
public final static String HWS0020E = "HWS0020E";
public final static String HWS0025E = "HWS0025E";
public final static String HWS0026E = "HWS0026E";
public final static String HWS0027E = "HWS0027E";
public final static String HWS0028E = "HWS0028E";
public final static String HWS0029E = "HWS0029E";
public final static String HWS0030E = "HWS0030E";
public final static String HWS0031E = "HWS0031E";
public final static String HWS0032E = "HWS0032E";
public final static String HWS0033E = "HWS0033E";
public final static String HWS0034E = "HWS0034E";
public final static String HWS0035E = "HWS0035E";
public final static String HWS0036E = "HWS0036E";
public final static String HWS0037E = "HWS0037E";
public final static String HWS0038E = "HWS0038E";
public final static String HWS0039E = "HWS0039E";
public final static String HWS0040E = "HWS0040E";
public final static String HWS0041E = "HWS0041E";
public final static String HWS0042E = "HWS0042E";
public final static String HWS0043E = "HWS0043E";
public final static String HWS0044E = "HWS0044E";
public final static String HWS0045E = "HWS0045E";
// Detailed API internal error messages
public final static String NO_CONN = "NO_CONN";
public final static String MSG_BLD_ERR = "MSG_BLD_ERR";
public final static String CODEPAGE_ERR = "CODEPAGE_ERR";
public final static String INVALID_SSLSESSION = "INVALID_SSLSESSION";
public final static String TRACE_FILE = "TRACE_FILE";
public final static String CONNECTION_PROPERTIES_FILE = "CONNECTION_PROPERTIES_FILE";
public final static String TMINTERACTION_PROPERTIES_FILE = "TMINTERACTION_PROPERTIES_FILE";
public final static String UNKNOWN_TYPE_FILE = "UNKNOWN_TYPE_FILE";
public final static String FILE_LINE_LIMIT = "FILE_LINE_LIMIT";
// Detailed IMS Connect Reason code
public static final String HWS_RESCODE_4 = "HWS_RESCODE_4"; // Input data exceeds buffer size
public static final String HWS_RESCODE_5 = "HWS_RESCODE_5"; // Negative length value
public static final String HWS_RESCODE_6 = "HWS_RESCODE_6"; // IRM length invalid
public static final String HWS_RESCODE_7 = "HWS_RESCODE_7"; // Total message length invalid
public static final String HWS_RESCODE_8 = "HWS_RESCODE_8"; // OTMA NAK with no sense code or RC
public static final String HWS_RESCODE_9 = "HWS_RESCODE_9"; // Contents of buffer invalid
public static final String HWS_RESCODE_10 = "HWS_RESCODE_10"; // Output data exceeds buffer size
public static final String HWS_RESCODE_11 = "HWS_RESCODE_11"; // Invalid unicode definition
public static final String HWS_RESCODE_12 = "HWS_RESCODE_12"; // Invalid message, no data
public static final String HWS_RESCODE_13 = "HWS_RESCODE_13"; //
public static final String HWS_RESCODE_14 = "HWS_RESCODE_14"; //
public static final String HWS_RESCODE_15 = "HWS_RESCODE_15"; //
public static final String HWS_RESCODE_16 = "HWS_RESCODE_16"; // Do not know who client is
public static final String HWS_RESCODE_17 = "HWS_RESCODE_17"; //
public static final String HWS_RESCODE_20 = "HWS_RESCODE_20"; // OTMA segment length error
public static final String HWS_RESCODE_24 = "HWS_RESCODE_24"; // FIC missing
public static final String HWS_RESCODE_28 = "HWS_RESCODE_28"; // LIC missing
public static final String HWS_RESCODE_32 = "HWS_RESCODE_32"; // Sequence number error
public static final String HWS_RESCODE_34 = "HWS_RESCODE_34"; // Unable to locate context token.
public static final String HWS_RESCODE_36 = "HWS_RESCODE_36"; // Protocol error
public static final String HWS_RESCODE_40 = "HWS_RESCODE_40"; // Security violation
public static final String HWS_RESCODE_44 = "HWS_RESCODE_44"; // Message incomplete
public static final String HWS_RESCODE_48 = "HWS_RESCODE_48"; // Incorrect message length
public static final String HWS_RESCODE_51 = "HWS_RESCODE_51"; //NOSECHDR
public static final String HWS_RESCODE_52 = "HWS_RESCODE_52"; //INVSECHL
public static final String HWS_RESCODE_53 = "HWS_RESCODE_53"; //SECFNOPW
public static final String HWS_RESCODE_54 = "HWS_RESCODE_54"; //SECFNUID
public static final String HWS_RESCODE_55 = "HWS_RESCODE_55"; //SECFNPUI
public static final String HWS_RESCODE_56 = "HWS_RESCODE_56"; //DUPECLNT
public static final String HWS_RESCODE_57 = "HWS_RESCODE_57"; //INVLDTOK
public static final String HWS_RESCODE_58 = "HWS_RESCODE_58"; //INVLDSTA
public static final String HWS_RESCODE_59 = "HWS_RESCODE_59"; //CANTIMER
public static final String HWS_RESCODE_70 = "HWS_RESCODE_70"; //NFNDCOMP
public static final String HWS_RESCODE_71 = "HWS_RESCODE_71"; //NFNDFUNC
public static final String HWS_RESCODE_72 = "HWS_RESCODE_72"; //NFNDDST
public static final String HWS_RESCODE_73 = "HWS_RESCODE_73"; //DSCLOSE
public static final String HWS_RESCODE_74 = "HWS_RESCODE_74"; //STP_CLSE
public static final String HWS_RESCODE_75 = "HWS_RESCODE_75"; //DSCERR
public static final String HWS_RESCODE_76 = "HWS_RESCODE_76"; //STOPCMD
public static final String HWS_RESCODE_77 = "HWS_RESCODE_77"; //COMMERR
public static final String HWS_RESCODE_78 = "HWS_RESCODE_78"; //SECFAIL
public static final String HWS_RESCODE_79 = "HWS_RESCODE_79"; //PROTOERR
public static final String HWS_RESCODE_80 = "HWS_RESCODE_80"; //NOTACTV
public static final String HWS_RESCODE_93 = "HWS_RESCODE_93"; //INVLDCM1
public static final String HWS_RESCODE_94 = "HWS_RESCODE_94"; //REQUEST
public static final String HWS_RESCODE_95 = "HWS_RESCODE_95"; //CONVER
public static final String HWS_RESCODE_96 = "HWS_RESCODE_96"; //REQ_CON
public static final String HWS_RESCODE_97 = "HWS_RESCODE_97"; //DEAL_CTD
public static final String HWS_RESCODE_98 = "HWS_RESCODE_98"; //DEAL_ABT
public static final String HWS_RESCODE_99 = "HWS_RESCODE_99"; //DEFAULT
// OTMA reason codes associated with OTMA sense code X'1A' (decimal 26)
public static final String OTMA_RESCODE_03 = "OTMA_RESCODE_03"; // RACF RACROUTE VERIFY call failed for incorrect input user ID
public static final String OTMA_RESCODE_21 = "OTMA_RESCODE_21"; // The message segment length or ZZ field cannot be changed by DFSNPRT0 exit
public static final String OTMA_RESCODE_22 = "OTMA_RESCODE_22"; // Invalid security option specified in the message prefix
public static final String OTMA_RESCODE_23 = "OTMA_RESCODE_23"; // Invalid command from an OTMA client. See DFS1285E
public static final String OTMA_RESCODE_24 = "OTMA_RESCODE_24"; // Transaction currently not available for use. See DFS3470E
public static final String OTMA_RESCODE_25 = "OTMA_RESCODE_25"; // SMB transaction/LTERM is stopped. See DFS065
public static final String OTMA_RESCODE_26 = "OTMA_RESCODE_26"; // Invalid CPIC transaction. See DFS1286E
public static final String OTMA_RESCODE_27 = "OTMA_RESCODE_27"; // Invalid remote destination (RCNT). See DFS1287E
public static final String OTMA_RESCODE_28 = "OTMA_RESCODE_28"; // Invalid CNT name specified. See DFS1288E
public static final String OTMA_RESCODE_29 = "OTMA_RESCODE_29"; // SMB not found. See DFS064
public static final String OTMA_RESCODE_30 = "OTMA_RESCODE_30"; // Invalid security. See DFS1292E
public static final String OTMA_RESCODE_31 = "OTMA_RESCODE_31"; // System error requested
public static final String OTMA_RESCODE_32 = "OTMA_RESCODE_32"; // System error message
public static final String OTMA_RESCODE_33 = "OTMA_RESCODE_33"; // User error message
public static final String OTMA_RESCODE_34 = "OTMA_RESCODE_34"; // Single-segment message. See DFS1290E
public static final String OTMA_RESCODE_35 = "OTMA_RESCODE_35"; // All messages discarded. See DFS249
public static final String OTMA_RESCODE_36 = "OTMA_RESCODE_36"; // Null segment sent. See DFS249.
// One cause for this error maybe the length specified on the MSGLEN key parameter of the IXCMSGO macro does not
// match the length of the OTMA data. No extra or null data can be padded at the end of the application data section
public static final String OTMA_RESCODE_37 = "OTMA_RESCODE_37"; // Queue overflow as unsuccessful insert
public static final String OTMA_RESCODE_38 = "OTMA_RESCODE_38"; // Commit mode 0 not allowed for IMS conversational or Fast Path
// transaction. See DFS1291E
public static final String OTMA_RESCODE_39 = "OTMA_RESCODE_39"; // IMS conversation is stopped, similar to an /EXIT command
public static final String OTMA_RESCODE_40 = "OTMA_RESCODE_40"; // DFSNPRT0 requested that a message be rerouted to a remote system,
// but failed. See DFS064
public static final String OTMA_RESCODE_41 = "OTMA_RESCODE_41"; // DFSNPRT0 requested that a message be rerouted to a remote system,
// but failed. See DFS070
public static final String OTMA_RESCODE_50 = "OTMA_RESCODE_50"; // The length specified in the application data section or the segment
// length for multi-segment input data exceeds the length specified on
// the MSGLEN key parameter of the XCF IXCMSGO macro
public static final String RACF_RETCODE_4 = "RACF_RETCODE_04"; // The user profile is not defined to RACF.
public static final String RACF_RETCODE_8 = "RACF_RETCODE_08"; // The password or password phrase is not authorized.
public static final String RACF_RETCODE_12 = "RACF_RETCODE_12"; // The password or password phrase has expired.
public static final String RACF_RETCODE_16 = "RACF_RETCODE_16"; // At least one of the following conditions has occurred:
// The new password or password phrase is not valid.
// A password or password phrase change is disallowed at this time
// because the minimum password-change interval has not passed.
public static final String RACF_RETCODE_20 = "RACF_RETCODE_20"; // The user is not defined to the group.
public static final String RACF_RETCODE_24 = "RACF_RETCODE_24"; // RACROUTE REQUEST=VERIFY was failed by the installation exit routine.
public static final String RACF_RETCODE_28 = "RACF_RETCODE_28"; // The user's access has been revoked.
public static final String RACF_RETCODE_36 = "RACF_RETCODE_36"; // The user's access to the specified group has been revoked.
public static final String RACF_RETCODE_40 = "RACF_RETCODE_40"; // OIDCARD parameter is required but not supplied.
public static final String RACF_RETCODE_44 = "RACF_RETCODE_44"; // OIDCARD parameter is not valid for specified user.
public static final String RACF_RETCODE_48 = "RACF_RETCODE_48"; // The user is not authorized to the port of entry in the TERMINAL,
// JESINPUT, or CONSOLE class.
public static final String RACF_RETCODE_52 = "RACF_RETCODE_52"; // The user is not authorized to use the application.
public static final String RACF_RETCODE_56 = "RACF_RETCODE_56"; // SECLABEL checking failed.
public static final String RACF_RETCODE_68 = "RACF_RETCODE_68"; // A default token is used as input token.
public static final String RACF_RETCODE_72 = "RACF_RETCODE_72"; // Indicates that an unprivileged user issued a RACROUTE REQUEST=VERIFY
// in a tranquil state (MLQUIET).
public static final String RACF_RETCODE_76 = "RACF_RETCODE_76"; // NODES checking failed.
public static final String RACF_RETCODE_80 = "RACF_RETCODE_80"; // Indicates that a surrogate submit attempt failed.
public static final String RACF_RETCODE_84 = "RACF_RETCODE_84"; // Indicates that a JESJOBS check failed.
public static final String RACF_RETCODE_92 = "RACF_RETCODE_92"; // Indicates that an error occurred while retrieving data from the RACF
// database.
public static final String RACF_RETCODE_100 = "RACF_RETCODE_100"; // Indicates that the CHECK subparameter of the RELEASE keyword was
// specified on the execute form of the RACROUTE REQUEST=VERIFY macro;
// Detailed Cobol Adapter Reason code
public static final String CBLADP_RETCODE_8 = "CBLADP_RETCODE_8_INVFUNC"; // Invalid function code
public static final String CBLADP_RETCODE_10 = "CBLADP_RETCODE_10"; // XML Adapter received an invalid function code from IMS Connect???
// RCs from 100-199 are outbound errors (inbound from Connect's perspective - descriptions use in/outbound from Connect's perspective)
public static final String CBLADP_RETCODE_108 = "CBLADP_RETCODE_108_IXCNVFND"; // Input XML Converter driver (not???) found
public static final String CBLADP_RETCODE_110 = "CBLADP_RETCODE_110"; // Specified XML Converter Driver not found or load failure???
public static final String CBLADP_RETCODE_112 = "CBLADP_RETCODE_112_NOLODIXCNV"; // Failed to load inbound XML Converter
public static final String CBLADP_RETCODE_115 = "CBLADP_RETCODE_115"; // LE failed to load XML Converter Driver to PreInit table???
public static final String CBLADP_RETCODE_116 = "CBLADP_RETCODE_116_NOLODIXCNVMDSVC"; // Failed to load inbound XML Converter Metadata Service
public static final String CBLADP_RETCODE_118 = "CBLADP_RETCODE_118_IPREINITFULL"; // Inbound preInit table full. Failed to delete entry from table
public static final String CBLADP_RETCODE_120 = "CBLADP_RETCODE_120_NOINVKIXCNVMDSVC"; // Failed to invoke inbound XML Converter Metadata Service
public static final String CBLADP_RETCODE_124 = "CBLADP_RETCODE_124_NOGETISTRG"; // Failed to obtain inbound storage
public static final String CBLADP_RETCODE_125 = "CBLADP_RETCODE_125_NOCNV105HMSG2ICDPG"; // Failed to convert HWSB0105E message to inbound code page
public static final String CBLADP_RETCODE_128 = "CBLADP_RETCODE_128_NOINVIXCNV"; // Failed to invoke XML Converter on inbound message
public static final String CBLADP_RETCODE_129 = "CBLADP_RETCODE_129_NOCNV110HMSG2ICDPG"; // Failed to convert HWSB0110E message to inbound code page
public static final String CBLADP_RETCODE_131 = "CBLADP_RETCODE_131"; // Failed to obtain storage for storing converted message???
public static final String CBLADP_RETCODE_132 = "CBLADP_RETCODE_132_IXCNVERR"; // Inbound XML Converter error
public static final String CBLADP_RETCODE_133 = "CBLADP_RETCODE_133_NOCNVXCNVERRMSG2ICDPG"; // Failed to convert XML Converter error message to inbound code page
public static final String CBLADP_RETCODE_136 = "CBLADP_RETCODE_136_CNVINMSGGT32K"; // Converted inbound message is greater than max supported size of 32,767 bytes
public static final String CBLADP_RETCODE_137 = "CBLADP_RETCODE_137_NOCNV115HMSG2ICDPG"; // Failed to convert HWSB0115E message to inbound code page
public static final String CBLADP_RETCODE_141 = "CBLADP_RETCODE_141_NOCNVOOC2ICDPG"; // Failed to convert output open and close tags to inbound code page
// RCs from 200-299 are inbound errors (outbound from Connect's perspective - descriptions use in/outbound from Connect's perspective)
public static final String CBLADP_RETCODE_208 = "CBLADP_RETCODE_208_OXCNVNFND"; // Output XML Converter not found
public static final String CBLADP_RETCODE_210 = "CBLADP_RETCODE_210"; // Specified XML Converter Driver not found or load failure???
public static final String CBLADP_RETCODE_212 = "CBLADP_RETCODE_212_NOLODOXCNV"; // Failed to load output XML Converter Metadata Service.
public static final String CBLADP_RETCODE_215 = "CBLADP_RETCODE_215"; // LE failed to load XML Converter Driver to PreInit table???
public static final String CBLADP_RETCODE_218 = "CBLADP_RETCODE_218_OPREINITFULL"; // Outbound preInit table full. Failed to delete entry from table
public static final String CBLADP_RETCODE_220 = "CBLADP_RETCODE_220_NOINVKOXCNVMDSVC"; // Failed to invoke output XML Converter Metadata Service
public static final String CBLADP_RETCODE_224 = "CBLADP_RETCODE_224_NOGETOSTRG"; // Failed to obtain outbound storage
public static final String CBLADP_RETCODE_225 = "CBLADP_RETCODE_225_NOCNV205HMSG2OCDPG"; // Failed to convert HWSB0205E message to outbound code page
public static final String CBLADP_RETCODE_228 = "CBLADP_RETCODE_228_NOINVOXCNV"; // Failed to invoke XML Converter on outbound message
public static final String CBLADP_RETCODE_229 = "CBLADP_RETCODE_229_NOCNV210HMSG2OCDPG"; // Failed to convert HWSB0210E message to outbound code page
public static final String CBLADP_RETCODE_231 = "CBLADP_RETCODE_231"; // Failed to obtain storage for converted message???
public static final String CBLADP_RETCODE_232 = "CBLADP_RETCODE_232_OXCNVERR"; // Outbound XML Converter error
public static final String CBLADP_RETCODE_233 = "CBLADP_RETCODE_233_NOCNVXCNVERRMSG2OCDPG"; // Failed to convert XML Converter error message to outbound code page
public static final String CBLADP_RETCODE_236 = "CBLADP_RETCODE_236"; // LE failed to invoke the XML Converter Driver???
public static final String CBLADP_RETCODE_237 = "CBLADP_RETCODE_237_NOCNVDFSMSG2OCDPG"; // Failed to convert IMS DFS message to outbound code page
public static final String CBLADP_RETCODE_241 = "CBLADP_RETCODE_241_NOCNVOOC2OCDPG"; // Failed to convert output open and close tags to the outbound code page
// Detailed valid property value descriptions
// generic property types
public static final String VALID_PROPERTY_VALUE_STRING = "VALID_PROPERTY_VALUE_STRING";
public static final String VALID_PROPERTY_VALUE_BOOLEAN = "VALID_PROPERTY_VALUE_BOOLEAN";
public static final String VALID_PROPERTY_VALUE_INTEGER = "VALID_PROPERTY_VALUE_INTEGER";
public static final String VALID_PROPERTY_VALUE_BYTE = "VALID_PROPERTY_VALUE_BYTE";
// Connection property types
public static final String VALID_PROPERTY_VALUE_HOSTNAME = "VALID_PROPERTY_VALUE_HOSTNAME";
public static final String VALID_PROPERTY_VALUE_PORTNUMBER = "VALID_PROPERTY_VALUE_PORTNUMBER";
public static final String VALID_PROPERTY_VALUE_SOCKETTYPE = "VALID_PROPERTY_VALUE_SOCKETTYPE";
public static final String VALID_PROPERTY_VALUE_SSLENCRYPTIONTYPE = "VALID_PROPERTY_VALUE_SSLENCRYPTIONTYPE";
//TmInteraction property types
public static final String VALID_PROPERTY_VALUE_ARCHLEVEL = "VALID_PROPERTY_VALUE_ARCHLEVEL";
public static final String VALID_PROPERTY_VALUE_ACKNAKPROVIDER = "VALID_PROPERTY_VALUE_ACKNAKPROVIDER";
public static final String VALID_PROPERTY_VALUE_COMMITMODE = "VALID_PROPERTY_VALUE_COMMITMODE";
public static final String VALID_PROPERTY_VALUE_IMSCONNECTTIMEOUT = "VALID_PROPERTY_VALUE_IMSCONNECTTIMEOUT";
public static final String VALID_PROPERTY_VALUE_IMSCONNECTUNICODEENCODINGSCHEMA = "VALID_PROPERTY_VALUE_IMSCONNECTUNICODEENCODINGSCHEMA";
public static final String VALID_PROPERTY_VALUE_IMSCONNECTUNICODEUSAGE = "VALID_PROPERTY_VALUE_IMSCONNECTUNICODEUSAGE";
public static final String VALID_PROPERTY_VALUE_INPUTMESSAGEOPTIONS = "VALID_PROPERTY_VALUE_INPUTMESSAGEOPTIONS";
public static final String VALID_PROPERTY_VALUE_INTERACTIONTYPEDESCRIPTION = "VALID_PROPERTY_VALUE_INTERACTIONTYPEDESCRIPTION";
//------------------Added for Sync callout------------------------------////
public static final String VALID_PROPERTY_VALUE_CALLOUTREQUESTNAKPROCESSING = "VALID_PROPERTY_VALUE_CALLOUTREQUESTNAKPROCESSING";
public static final String VALID_PROPERTY_VALUE_RESPONSEMESSAGETYPE = "VALID_PROPERTY_VALUE_RESPONSEMESSAGETYPE";
public static final String VALID_PROPERTY_VALUE_RESUMETPIPERETRIEVALTYPE = "VALID_PROPERTY_VALUE_RESUMETPIPERETRIEVALTYPE";
//--------------End--------------------------------------------------------/////
public static final String VALID_PROPERTY_VALUE_RESUMETPIPEPROCESSING = "VALID_PROPERTY_VALUE_RESUMETPIPEPROCESSING";
public static final String VALID_PROPERTY_VALUE_SYNCLEVEL = "VALID_PROPERTY_VALUE_SYNCLEVEL";
// Resource Bundle
static private ResourceBundle res = null;
public static final String getExceptionMessage(Exception e)
{
String expMsg = "";
String errClassName = e.getClass().getName();
int index = errClassName.lastIndexOf('.');
if (index > 0)
{
errClassName = errClassName.substring(index + 1);
if (e.getMessage() != null)
expMsg = errClassName + ": " + e.getMessage();
else
expMsg = errClassName;
}
else
{
expMsg = e.toString();
}
return expMsg;
}
/**
* Load and Return the Resource Bundle for IMS Connector.
*
* @return java.util.ResourceBundle The Resource Bundle
*/
private static ResourceBundle getResourceBundle()
{
if (res == null)
{
res = ResourceBundle.getBundle(
"com.ibm.ims.connect.ImsConnectErrorMessageResourceBundle",
Locale.getDefault());
}
return res;
}
/**
* Gets an IMS Connector resource message.
*
* @return java.lang.String formatted IMS Connector resource message
* @param key
* java.lang.String The identification key of the resource
* message
*/
public static final String getString(String key)
throws MissingResourceException
{
return getResourceBundle().getString(key);
}
/**
* Gets an IMS resource message.
*
* @return java.lang.String formatted IMS Connector resource message
* @param key
* java.lang.String The identification key of the resource
* message
* @param arg
* java.lang.Object The message inserts of the resource message
*/
public static final String getString(String key, Object arg[])
throws MissingResourceException
{
String basemsgfmt = (String) getResourceBundle().getString(key);
MessageFormat msgfmt = new MessageFormat(basemsgfmt);
return msgfmt.format(arg);
}
protected static Object getExceptionMessage(Throwable e) {
String expMsg = "";
String errClassName = e.getClass().getName();
int index = errClassName.lastIndexOf('.');
if (index > 0)
{
errClassName = errClassName.substring(index + 1);
if (e.getMessage() != null)
expMsg = errClassName + ": " + e.getMessage();
else
expMsg = errClassName;
}
else
{
expMsg = e.toString();
}
return expMsg;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy