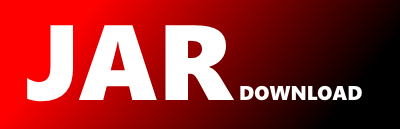
com.ibm.ims.connect.InputMessage Maven / Gradle / Ivy
Show all versions of IMSConnectAPI Show documentation
/*
* File: InputMessage.java
* ==========================================================================
* Licensed Material - Property of IBM
*
* IBM Confidential
*
* OCO Source Materials
*
* 5655-TDA
*
* (C) Copyright IBM Corp. 2009, 2013 All Rights Reserved.
*
* The source code for this program is not published or
* otherwise divested of its trade secrets, irrespective
* of what has been deposited with the U.S. Copyright
* Office.
*
* US Government Users Restricted Rights - Use, duplication or
* disclosure restricted by GSA ADP Schedule Contract with
* IBM Corp.
* ===========================================================================
*/
package com.ibm.ims.connect;
import java.io.*;
/**
* Contains the data in the input message to be sent to IMS Connect.
* The class provides setter and getter methods for the data in each segment
* in the input message to be sent to IMS Connect to invoke the IMS transaction.
*
* Note that if the inputMessageDataSegmentsIncludeLlzz interaction property value
* is true
, the byte arrays and Strings passed in as input parameters
* to the append, change, insert, and set methods should include values for the
* LLZZ and trancode fields. If the inputMessageDataSegmentsIncludeLlzz value is
* false
, the byte arrays and String input parameters should contain
* data only.
* @see InputMessage#setInputMessageDataSegmentsIncludeLlzzAndTrancode(boolean)
* @see TmInteraction#getInputMessage()
*/
public interface InputMessage extends ApiProperties
{
public static final String copyright =
"Licensed Material - Property of IBM "
+ "5655-TDA"
+ "(C) Copyright IBM Corp. 2009, 2013 All Rights Reserved. "
+ "US Government Users Restricted Rights - Use, duplication or "
+ "disclosure restricted by GSA ADP Schedule Contract with IBM Corp. ";
// Customer populates InputMessage with byte[] byte[][], String, or String[]
// representing complete input message data or, for populating individual
// segments, with byte [] or String representing each segment. A String is
// always interpreted as a single segment while a String array is interpreted
// as multiple segments. Both a byte array and an array of byte arrays can
// contain either a single segment or multiple segment. If a byte array
// contains the data for the segments of a multi-segment message, each segment
// in the data must be preceded by LLZZ (or LLZZ and trancode for the first
// segment of a transaction request.) Single-segment data can be stored in an
// array of byte arrays (a two-dimensional byte array,) as the one and only
// element of that array of byte arrays.
//
// Methods are provided for getting the data from an InputMessage object as
// either a one- or two-dimensional byte array, as a String or as an array of
// Strings.
//
// InputMessage will internally store byte array elements as received (or as
// converted from String array elements) if the input data is provided in a
// 2-dimensional array of byte arrays or as an array of Strings. If the data
// is provided in a single byte array or in a single String, it will be stored
// after conversion to a 2-dimensional byte array. If the user sets
// dataIncludesLlzzAndTrancode to true, the input data will be split up into
// separate byte array elements based on the supplied LLZZ's in the message.
// Getters will return data as stored (w/ or w/o LLZZ and trancode depending
// on whether or not customer supplied input data w/ or w/o LLZZ and trancode.)
//
// The API will internally add LLZZ's to input message (along w/ LLLL and IRM)
// if LLZZ's are not provided with the input data before sending the input
// message to Connect.
/**
* Gets the data from the input message as an array of byte arrays with each byte array containing a
* segment of the input data without the LLLL, LLZZ, or trancode fields.
* @return an array of byte arrays with each byte array containing a segment without LLLL,
* LLZZ or trancode.
*/
public byte[][] getDataAsArrayOfByteArrays();
/**
* Gets the data from the input message as a byte array containing all of the segments
* of input data concatenated without the LLLL, LLZZ, or trancode fields.
* @return a byte array containing all of the data segments concatenated without the LLLL, LLZZ or trancode.
*/
public byte[] getDataAsByteArray();
/**
* Gets the data from the input message as a String
with all of the segments
* of input data concatenated without the LLLL, LLZZ, or trancode fields.
* @return a String
with all of the segments
* of input data concatenated without the LLLL, LLZZ, or trancode fields.
* @throws ImsConnectApiException
*/
public String getDataAsString() throws ImsConnectApiException;
/**
* Gets the data from the input message as a String
array with each String
containing
* a segment of the input data without the LLLL, LLZZ, or trancode fields.
* @return a String
array with each String
containing
* a segment of the input data without the LLLL, LLZZ, or trancode fields.
* @throws ImsConnectApiException
*/
public String[] getDataAsArrayOfStrings() throws ImsConnectApiException;
/**
* Gets the segment, at the segment location specified by aSegmentNumber, as a
* byte array without the LLLL, but including the LLZZ along with the trancode
* field if applicable.
* @param aSegmentNumber 0-based segment index
* @return a byte array containing the segment data without the LLLL, but with the
* LLZZ field and trancode field if applicable.
*/
public byte[] getSegmentAsByteArray(int aSegmentNumber);
/**
* Gets the segment, at the segment location specified by aSegmentNumber, as a
* String
without the LLLL, LLZZ or trancode fields.
* @param aSegmentNumber 0-based segment index
* @return a String
containing the segment data without the LLLL, LLZZ or trancode fields.
* @throws ImsConnectApiException
*/
public String getSegmentAsString(int aSegmentNumber) throws ImsConnectApiException;
/**
* Gets the LLLL field value in this input message.
* @return the LLLL field value as an int.
*/
public int getLlll();
/**
* Gets the number of segments in this input message.
* @return the number of segments.
*/
public int getNumberOfSegments();
/**
* Gets the input message as a byte array.
* @return a byte array containing the input message.
* @throws ImsConnectApiException
*/
public byte[] getMessage() throws ImsConnectApiException; // retrieves message byte array built by getBytes()
/**
* Appends the data in the aSegmentData byte array as a new segment in the input message.
*
* Note that if the inputMessageDataSegmentsIncludeLlzz interaction property value
* is true
, the byte array passed in as the input parameter to the append method should
* include values for the LLZZ and trancode fields. If the inputMessageDataSegmentsIncludeLlzz value is
* false
, the byte array input parameter should contain data only.
* @param aSegmentData a byte array that contains the data to be appended.
*/
public void appendSegment(byte[] aSegmentData);
/**
* Appends the data in the aSegmentData String
as a new segment in the input message.
*
* Note that if the inputMessageDataSegmentsIncludeLlzz interaction property value
* is true
, the String
passed in as input parameter to the append method should
* include values for the LLZZ and trancode fields. If the inputMessageDataSegmentsIncludeLlzz value is
* false
, the String
input parameter should contain data only.
* @param aSegmentData a String
that contains the data to be appended.
* @throws ImsConnectApiException
*/
public void appendSegment(String aSegmentData) throws ImsConnectApiException;
/**
* Inserts the data in the aSegmentData byte array as a new segment, at the location specified by aLocation,
* in the input message.
*
* Note that if the inputMessageDataSegmentsIncludeLlzz interaction property value
* is true
, the byte array passed in as the input parameter to the insert method should
* include values for the LLZZ and trancode fields. If the inputMessageDataSegmentsIncludeLlzz value is
* false
, the byte array input parameter should contain data only.
* @param aSegmentData a new segment to be inserted in the input message
* @param aLocation 0-based index of the insertion point for the new segment.
*/
public void insertSegment(byte[] aSegmentData, int aLocation) throws UnsupportedEncodingException;
/**
* Inserts the data in the aSegmentData String
as a new segment, at the location specified by aLocation,
* in the input message.
*
* Note that if the inputMessageDataSegmentsIncludeLlzz interaction property value
* is true
, the String
passed in as the input parameter to the insert method should
* include values for the LLZZ and trancode fields. If the inputMessageDataSegmentsIncludeLlzz value is
* false
, the String
input parameter should contain data only.
* @param aSegmentData a new segment to be inserted in the input message
* @param aLocation 0-based index of the insertion point for the new segment.
* @throws ImsConnectApiException
*/
public void insertSegment(String aSegmentData, int aLocation) throws Exception;
/**
* Sets (or replaces) the data in the anInputDataByteArray byte array parameter as the data in the input message.
*
* If the inputMessageDataSegmentsIncludeLlzz interaction property value
* is true
, the byte array passed in as the input parameter to the set method should
* include values for the LLZZ and trancode fields. If the inputMessageDataSegmentsIncludeLlzz value is
* false
, the byte array input parameter should contain data only.
*
Do not invoke this method before setting or initializing the API properties for the input message to the
* values required by your client application program.
*
* @param anInputDataByteArray a byte array containing data.
*/
/* Must add caution note in API doc about invoking this method before the properties that it uses (TmInteraction,
inputMessageDataSegmentsIncludeLlzzAndTrancode and trancode properties along with the anInputDataByteArray
input parameter) have been set or initialized as needed*/
public void setInputMessageData(byte[] anInputDataByteArray) throws ImsConnectApiException;
/**
* Sets (or replaces) the data in the anInputData2DByteArray two-dimensional byte array parameter as the
* data in the input message.
*
* Note that if the inputMessageDataSegmentsIncludeLlzz interaction property value
* is true
, the array of byte arrays passed in as the input parameter to the set method should
* include values for the LLZZ and trancode fields. If the inputMessageDataSegmentsIncludeLlzz value is
* false
, the array of byte arrays input parameter should contain data only.
*
>>>> Must add caution note in API doc about invoking this method before the properties that it uses (TmInteraction,
*
Do not invoke this method before setting or initializing the API properties for the input message to the
*values required by your client application program.
*
* @param anInputData2DByteArray a two-dimensional byte array containing data.
*/
public void setInputMessageData(byte[][] anInputData2DByteArray) throws ImsConnectApiException;
/**
* Sets (or replaces) the data in the anInputDataString String parameter as the data in the input message.
*
Note that if the inputMessageDataSegmentsIncludeLlzz interaction property value
* is true
, the String
passed in as the input parameter to the set method should
* include values for the LLZZ and trancode fields. If the inputMessageDataSegmentsIncludeLlzz value is
* false
, the String
input parameter should contain data only.
*
Imaginary Buffer Line
*
Do not invoke this method before setting or initializing the API properties for the input message to the
*values required by your client application program.
*
* @param anInputDataString a String
containing data.
* @throws ImsConnectApiException
*/
public void setInputMessageData(String anInputDataString) throws ImsConnectApiException;
/**
* Sets (or replaces) the data in the anInputDataStringArray String
array parameter as the
* data in the input message.
*
Note that if the inputMessageDataSegmentsIncludeLlzz interaction property value
* is true
, the String
array passed in as the input parameter to the set method should
* include values for the LLZZ and trancode fields. If the inputMessageDataSegmentsIncludeLlzz value is
* false
, the String
array input parameter should contain data only.
*
Imaginary Buffer Line
*
Do not invoke this method before setting or initializing the API properties for the input message to the
*values required by your client application program.
*
* @param anInputDataStringArray a String
array containing data.
* @throws ImsConnectApiException
*/
public void setInputMessageData(String[] anInputDataStringArray) throws ImsConnectApiException;
/**
* Replaces the data in the input message, at the segment at specified by aLocation, with the
* contents of the aSegmentData byte array.
*
* Note that if the inputMessageDataSegmentsIncludeLlzz interaction property value
* is true
, the byte array passed in as the input parameter to the change method should
* include values for the LLZZ and trancode fields. If the inputMessageDataSegmentsIncludeLlzz value is
* false
, the byte array input parameter should contain data only.
* @param aSegmentData a byte array that contains the data to be substituted in.
* @param aLocation 0-based index of the segment to be changed.
*/
public void changeSegment(byte[] aSegmentData, int aLocation);
/**
* Replaces the data in the input message, at the segment at specified by aLocation, with the
* contents of the aSegmentData String
.
*
* Note that if the inputMessageDataSegmentsIncludeLlzz interaction property value
* is true
, the String
passed in as the input parameter to the change method should
* include values for the LLZZ and trancode fields. If the inputMessageDataSegmentsIncludeLlzz value is
* false
, the String
input parameter should contain data only.
* @param aSegmentData a String
that contains the data to be substituted in.
* @param aLocation 0-based index of the segment to be changed.
* @throws ImsConnectApiException
*/
public void changeSegment(String aSegmentData, int aLocation) throws ImsConnectApiException;
/**
* Gets the inputMessageDataSegmentsIncludeLlzzAndTrancode property value in this input message.
* @return the inputMessageDataSegmentsIncludeLlzzAndTrancode property value as a boolean, true
if this input message has LLZZ and trancode fields. Otherwise, false
.
*/
public boolean isInputMessageDataSegmentsIncludeLlzzAndTrancode();
/**
* Sets the inputMessageDataSegmentsIncludeLlzzAndTrancode property value in this input message.
* @param anInputMessageDataSegmentsIncludeLlzzAndTrancode a boolean true
if this input message has LLZZ and trancode fields. Otherwise, false
.
*/
public void setInputMessageDataSegmentsIncludeLlzzAndTrancode(boolean anInputMessageDataSegmentsIncludeLlzzAndTrancode);
public String padString(String str, char c, int len);
}