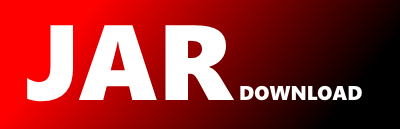
com.ibm.ims.connect.OutputMessage Maven / Gradle / Ivy
Show all versions of IMSConnectAPI Show documentation
/*
* File: OutputMessage.java
* ==========================================================================
* Licensed Material - Property of IBM
*
* IBM Confidential
*
* OCO Source Materials
*
* 5655-TDA
*
* (C) Copyright IBM Corp. 2009, 2014 All Rights Reserved.
*
* The source code for this program is not published or
* otherwise divested of its trade secrets, irrespective
* of what has been deposited with the U.S. Copyright
* Office.
*
* US Government Users Restricted Rights - Use, duplication or
* disclosure restricted by GSA ADP Schedule Contract with
* IBM Corp.
* ===========================================================================
*/
package com.ibm.ims.connect;
/**
* Contains the data in the output message received from IMS Connect.
* Use the getter methods in this class to retrieve the response message contents
* including the LLLL value of the response message, the LL and ZZ values, and the data
* for each segment in the response message.
*/
public interface OutputMessage extends ApiProperties
{
public static final String copyright =
"Licensed Material - Property of IBM "
+ "5655-T62"
+ "(C) Copyright IBM Corp. 2009,2015 All Rights Reserved. "
+ "US Government Users Restricted Rights - Use, duplication or "
+ "disclosure restricted by GSA ADP Schedule Contract with IBM Corp. ";
// API populates 1-dimensional data byte[] in outputMessage with response byte[]
// array as received from IMS Connect.
// Methods are provided for getting the data from the output message as either
// a 1- or 2-dimensional byte array, as an array of Strings or as a single
// String after conversion as required from the 1-dimensional array in which it
// is stored and removal of LLZZ as needed.
/**
* Gets the length of the response message, which includes the length of the LLLL field,
* the length of LLZZ field, and the length of the trancode field if present.
* @return the length of the response message as an integer.
*/
public int getMessageLength();
/**
* Gets the output message as a byte array.
* @return a byte array containing the output message.
*/
public byte[] getResponseMessage();
/**
* Gets the LLLL from the output message response as a byte[].
* @return the LLLL from the output message as a byte[].
*/
public byte[] getLlll();
/**
* Gets the LLLL from the output message response as a String of hexidecimal digits.
* @return the LLLL from the output message as a hex String or a string of four 00's
* if the output message does not include an LLLL.
*/
public String getLlllAsHexString();
/**
* Gets the LLLL value from the output message response as an integer.
* @return the LLLL from the output message as an integer or the
* value 0 if there is no LLLL in the output message
*/
public int getLlllValue();
/**
* Gets the response data from the output message as an array of byte arrays, with each byte array
* containing a segment without the LLLL, LLZZ, or trancode fields.
* @return the response data from the output message as an array of byte arrays.
*/
public byte[][] getDataAsArrayOfByteArrays();
/**
* Gets the response data from the output message as a byte array, with all of the segments concatenated
* without the LLLL, LLZZ, or trancode fields.
* @return the response data from the output message as a byte array.
*/
public byte[] getDataAsByteArray();
/**
* Gets the response data from the output message as a String
with all of the segments
* concatenated without the LLLL, LLZZ or trancode fields.
* @return the response data from the output message as a String
.
* @throws ImsConnectApiException
*/
public String getDataAsString() throws ImsConnectApiException;
/**
* Gets the response data from the output message as a String
array, with each individual
* String
containing a segment without the LLLL, LLZZ, or trancode fields.
* @return the response data from the output message as a String
array.
* @throws ImsConnectApiException
*/
public String[] getDataAsArrayOfStrings() throws ImsConnectApiException;
/**
* Gets the LL bytes from the segment at the location specified by aSegmentNumber
* in the output message as a byte array.
* @param aSegmentNumber segment offset
* @return the LL bytes from the specified segment as a byte array
*/
public byte[] getSegmentLl(int aSegmentNumber);
/**
* Gets the LL bytes from the segment at the location specified by aSegmentNumber
* in the output message in hex as a String.
* @param aSegmentNumber segment offset
* @return the LL bytes from the specified segment in hex as a String
*/
public String getSegmentLlAsHexString(int aSegmentNumber);
/**
* Gets the LL value from the segment at the location specified by aSegmentNumber
* in the output message as a short value.
* @param aSegmentNumber segment offset
* @return the LL value from the specified segment as a short
*/
public short getSegmentLlValue(int aSegmentNumber);
/**
* Gets the ZZ value from the segment at the location specified by aSegmentNumber
* in the output message as a byte array.
* @param aSegmentNumber segment offset
* @return the ZZ bytes from the specified segment as a byte array
*/
public byte[] getSegmentZz(int aSegmentNumber);
/**
* Gets the ZZ bytes from the segment at the location specified by aSegmentNumber
* in the output message in hex as a String.
* @param aSegmentNumber segment offset
* @return the ZZ bytes from the specified segment in hex as a String
*/
public String getSegmentZzAsHexString(int aSegmentNumber);
/**
* Gets the segment at the location specified by aSegmentNumber in the output message
* as a byte array without the LLLL, LLZZ, or trancode fields.
* @param aSegmentNumber segment offset
* @return the segment of the response data in the output message as a byte array
*/
public byte[] getSegmentDataAsByteArray(int aSegmentNumber);
/**
* Gets the segment at the location specified by aSegmentNumber in the output message as
* a String
without the LLLL, LLZZ, or trancode fields.
* @param aSegmentNumber segment offset
* @return the segment of the response data in the output message as a String
* @throws ImsConnectApiException
*/
public String getSegmentDataAsString(int aSegmentNumber) throws ImsConnectApiException;
/**
* Gets the number of segments in this output message as an int value
* @return the number of segments in this output message as an int
* @throws ImsConnectApiException
*/
public int getNumberOfSegments();
/**
* Gets a boolean
value indicating whether each response message does or
* does not include LLLL
* @return a boolean
value containing whether the response messages
* returned to the IMS Connect API by IMS Connect start with LLLL (true -
* response messages processed using HWSSMPL1 in IMS Connect do start with
* LLLL) or do not start with LLLL (false - response messages processed
* using HWSSMPL0 do not start with LLLL)
*/
public boolean isResponseIncludesLlll();
/**
* Gets the ackNakNeeded property value.
* This property indicates whether or not this response from IMS must be acknowledged or negatively
* acknowledged prior to any other activity on this connection.
*
If ackNakProvider was set to API_INTERNAL_ACK (the default value) in the original request message,
* this property will always be set to false in the corresponding response message since the acknowledgement
* of the response, if required, will have been processed internally by the IMS Connect API.
*
When ackNakProvider is set to CLIENT_ACK_NAK in the original request message, this property will
* be set according to the IMS Connect protocol. Whenever this property is set to true in the response, the
* client must send in an ACK or NAK interaction as the next interaction on that connection or IMS Connect
* will respond with a protocol violation.
*
* Use the constants defined in ApiProperties
to determine the ackNakNeeded property value.
* For example,
*
* if(myTmInteraction.isAckNakNeeded() == ApiProperties.ACK_NAK_NEEDED)
* {
* // submit an ACK or NAK interaction as required as the next
* // interaction on this connection
* }
*
* @return a boolean
value indicating whether or not the response from IMS must be acknowledged
* or negatively acknowledged prior to any other activity on this connection
* @see ApiProperties#ACK_NAK_NEEDED
* @see ApiProperties#NO_ACK_NAK_NEEDED
*/
public boolean isAckNakNeeded();
/**
* Gets the asyncOutputAvailable property value.
* This property specifies whether or not there are any more asynchronous output messages queued in on
* the asynchronous hold queue for this client's tpipe in IMS and available for retrieval by this or another
* client.
*
The value of this property is only valid in a non-shared queues environment. In a shared queues
* environment, the client must issue a ResumeTpipe interaction in order to know if there is asynchronous
* output available (in which case, output will be returned if there is any available).
*
For more information about asynchronous output support, see Asynchronous Output Support.
*
* Use the constants defined in ApiProperties
to determine the asyncOutputAvailable property value.
* For example,
*
* if(myTmInteraction.isAsyncOutputAvailable() == ApiProperties.ASYNC_OUTPUT_AVAILABLE)
* {
* // retrieve additional asynchronous output or save a flag that you can use
* // to retrieve the asynchronous output at a later time
* }
*
* @return a boolean
value indicating whether or not there are any more asynchronous
* output messages queued on the asynchronous hold queue for this client's tpipe in IMS and
* available for retrieval by this or another client
* @see ApiProperties#ASYNC_OUTPUT_AVAILABLE
* @see ApiProperties#NO_ASYNC_OUTPUT_AVAILABLE
*/
public boolean isAsyncOutputAvailable();
/**
* Gets the inCoversation property value.
* This response property specifies whether or not this interaction is part of a currently active IMS
* conversation. *
* Use the constants defined in ApiProperties
to determine the InCoversation property value.
* For example,
*
* if(myTmInteraction.isInConversation() == ApiProperties.IN_CONVERSATION)
* {
* // client application needs to continue the conversation or send in
* // an interaction to end the conversation. API will not include the
* // trancode in an input message for an interaction if the
* // inConversation property value is true.
* }
*
* @return a boolean
value indicating whether or not this interaction is part of a
* currently active IMS conversation
* @see ApiProperties#IN_CONVERSATION
* @see ApiProperties#NOT_IN_CONVERSATION
*/
public boolean isInConversation();
/**
* Gets the imsConnectReasonCode property value.
* This response property specifies the IMS Connect or IMS Connect user message exit reason code if
* IMS Connect or the IMS Connect user message exit detected an error during processing of the current
* interaction.
* @return an int
value containing the reason code
*/
public int getImsConnectReasonCode();
/**
* Gets the ImsConnectReturnCode property value.
* This response property specifies the IMS Connect or IMS Connect user message exit return code if
* IMS Connect or the IMS Connect user message exit detected an error during processing of the current
* interaction.
* @return an int
value containing the return code
*/
public int getImsConnectReturnCode();
/**
* Gets the clientId name property value. The clientId name property will have a meaningful value only if the user
* requests that the clientld to be returned. In this case, IMS Connect returns an GENCID segment at the beginning
* of the response message, if the interaction is successful. If the interaction fails, the clientId is not returned.
* In case when a clientId is not returned, the value will be set to an empty string.
* @return the clientID value to the client from IMS Connect.
*/
public String getReturnedClientId();
/**
* Gets the mfsModname name property value. The mfsModname property will have a meaningful value only if the user
* requests that the MFS modname be returned. In this case, IMS Connect returns an RMM segment at the beginning
* of the response message, if the interaction is successful. If the interaction fails, the modname is not returned.
* @return the mfsModname value returned by the IMS application through IMS Connect
*/
public String getMfsModname();
/**
* Gets the OTMA reason code. An OTMA reason code may be returned whenever OTMA returns a
* sense code value of X'1A' (decimal 26.)
* @return an int
value containing the otmaReasonCode
* @see TmInteraction#getOtmaSenseCode()
*/
public byte getOtmaReasonCode();
/**
* Gets the OTMA sense code property value. For information about OTMA sense codes, see
* the IMS Messages and Codes documentation, under the topic
* "OTMA sense codes for NAK messages".
* @return int value representing the OTMA sense code
*/
public int getOtmaSenseCode();
/**
* Gets the RACF return code property value.
* @return int value representing the RACF return code
*/
public int getRacfReturnCode();
/**
* Gets the RACF return code string property value.
* @return String value representing the RACF return code meaning
*/
public String getRacfReturnCodeString();
/**
* Gets a Type-2 command response returned by IMS.
* @return the type2CommandResponse object from this OutputMessage
*/
public Type2CmdResponse getType2CommandResponse() throws ImsConnectApiException;
}