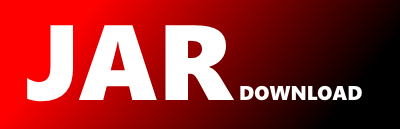
com.ibm.ims.connect.TmInteraction Maven / Gradle / Ivy
Show all versions of IMSConnectAPI Show documentation
/*
* File: TmInteraction.java
* ==========================================================================
* Licensed Material - Property of IBM
*
* IBM Confidential
*
* OCO Source Materials
*
* 5655-TDA
*
* (C) Copyright IBM Corp. 2009, 2014 All Rights Reserved.
*
* The source code for this program is not published or
* otherwise divested of its trade secrets, irrespective
* of what has been deposited with the U.S. Copyright
* Office.
*
* US Government Users Restricted Rights - Use, duplication or
* disclosure restricted by GSA ADP Schedule Contract with
* IBM Corp.
* ===========================================================================
*/
package com.ibm.ims.connect;
import com.ibm.ims.connect.impl.TmInteractionImpl.CorrelatorToken;
/**
* Configures interaction properties and executes an interaction with IMS Connect in an IMS transaction.
*
* The following code sample shows how to obtain a TMInteraction
instance
* from a valid com.ibm.ims.connect.Connection
instance (myConn), load
* the initial interaction properties settings from a TMInteraction attributes file,
* use the TMInteraction
methods to customize the interaction, and execute
* the interaction using the configured settings.
*
*
*
* // a previously configured connection object
* Connection myConn;
* ...
*
* // create a new TMInteraction instance
* TMInteraction myTMInteraction = myConn.createInteraction();
*
* // load properties from file
* myTMInteraction.loadTMInteractionAttributesFromFile("TMIntAttributes.txt");
*
* // customize the TMInteraction property settings
* myTMInteraction.setImsDatastoreName("IMS1");
*
* myTMInteraction.setTrancode("IVTNO ");
*
* // create an input byte array and set thetransaction input data
* byte[] indata;
*
* indata = (new String("DISPLAY LAST1 ").getBytes(myTMInteraction.getImsConnectDataCodepage()));
*
* // get InputMessage instance from myTMInteraction
* InputMessage inMsg = myTMInteraction.getInputMessage();
*
* // the setInputMessageSegmentsIncludeLlzzAndTrancode(boolean) method specifies if the input message will include
* // values for LLZZ (and trancode, if applicable).
* inMsg.setInputMessageSegmentsIncludeLlzzAndTrancode(false);
*
* // populate input message data with indata byte array
* inMsg.setInputMessageData(indata);
*
* // execute the transaction
* myTMInteraction.execute();
*
* // process the output
* if ((myTMInteraction.getImsConnectReturnCode() == ApiProperties.IMS_CONNECT_RETURN_CODE_SUCCESS) &&
* (myTMInteraction.getImsConnectReasonCode() == ApiProperties.IMS_CONNECT_REASON_CODE_SUCCESS))
* {
* System.out.println("\nInteraction was successful");
*
* // get output from myTMInteraction
* OutputMessage outMsg = myTMInteraction.getOutputMessage();
*
* // get data from outMsg as a string
* String outData = outMsg.getDataAsString();
* } else
* {
* System.out.println("\nInteraction returned a non-zero return code and/or reason code");
* }
*
*
*/
public interface TmInteraction extends ApiProperties
{
public static final String copyright =
"Licensed Material - Property of IBM "
+ "5655-TDA"
+ "(C) Copyright IBM Corp. 2009, 2013 All Rights Reserved. "
+ "US Government Users Restricted Rights - Use, duplication or "
+ "disclosure restricted by GSA ADP Schedule Contract with IBM Corp. ";
/**
* Executes a complete interaction with IMS Connect in an IMS transaction or executes an IMS command.
* Use the execute method to execute a single interaction with IMS Connect. Based on the interaction type
* specified, you can use this method to send a request to execute an IMS transaction, receive the output of an
* IMS transaction, or both send the request and receive the output. The execute method handles:
*
* - Creating a socket connection to be used for the interaction,
*
- Setting up the IMS Connect header for the interaction,
*
- Submitting the interaction request,
*
- Receiving the interaction response, and finally
*
- Closing the socket.
*
* The execute method builds an IRM header based on the interaction properties specified in the
* dynamically loaded interaction properties file, or set programmatically by the user. The method sends
* the input data byte array for an IMS transaction and returns the output data (transaction response)
* in the form of an output data array, and updates the interaction status of the TmInteraction
* instance.
*
* This version of execute
is used to execute an interaction using the InputMessage
* object which must be configured beforehand in the parent TmInteraction
instance.
* The response will be used to populate the OutputMessage
object which is also a member of
* the parent TmInteraction
instance.
* @throws Exception
* @see InputMessage
* @see OutputMessage
*/
public void execute() throws Exception;
/**
* Reads in interaction attributes from a interaction attributes files and configures this
* TmInteraction
instance.
* @throws Exception
* @param anInteractionAttributesFileName text file containing the interaction attributes
*/
public void loadTmInteractionAttributesFromFile(String anInteractionAttributesFileName)
throws Exception;
// Getter methods for read-only output properties
/**
* Gets the InputMessage
instance to store the input data to send to IMS Connect.
* @return the InputMessage
instance
* @throws ImsConnectApiException
* @see InputMessage
*/
public InputMessage getInputMessage() throws ImsConnectApiException;
/**
* Get the OutputMessage
instance which stores the output data received from IMS Connect.
* @return the OutputMessage
instance
* @throws ImsConnectApiException
* @see OutputMessage
*/
public OutputMessage getOutputMessage() throws ImsConnectApiException;
/**
* Gets the clientType property value.
*
* @return an int
value containing the clientType property value
*/
// Response properties
/**
* Gets the ImsConnectReturnCode property value.
* This response property specifies the IMS Connect or IMS Connect user message exit return code if
* IMS Connect or the IMS Connect user message exit detected an error during processing of the current
* interaction.
*
See Return and reason codes" for a description of the IMS Connect return codes.
* @return an int
value containing the return code
*/
public int getImsConnectReturnCode();
/**
* Gets the imsConnectReasonCode property value.
*
This response property specifies the IMS Connect or IMS Connect user message exit reason code if
* IMS Connect or the IMS Connect user message exit detected an error during processing of the current
* interaction.
*
See Return and reason codes for a description of the IMS Connect reason codes.
* @return an int
value containing the reason code
*/
public int getImsConnectReasonCode();
/**
* Gets the OTMA reason code. An OTMA reason code may be returned whenever OTMA returns a
* sense code value of X'1A' (decimal 26.)
* @return an int
value containing the otmaReasonCode
* @see TmInteraction#getOtmaSenseCode()
*/
public int getOtmaReasonCode();
/**
* Gets the OTMA sense code property value. For information about OTMA sense codes, see
* the IMS Messages and Codes documentation, under the topic
* "OTMA sense codes for NAK messages".
* @return int value representing the OTMA sense code
*/
public int getOtmaSenseCode();
/**
* Gets the RACF return code property value.
* @return int value representing the RACF return code
*/
public int getRacfReturnCode();
/**
* Gets a short descriptive String associated with the RACF return code.
* @return String value representing the RACF return code meaning
*/
public String getRacfReturnCodeString();
/**
* Gets the ackNakNeeded property value.
*
This property indicates whether or not this response from IMS must be acknowledged or negatively
* acknowledged prior to any other activity on this connection.
*
If ackNakProvider was set to API_INTERNAL_ACK (the default value) in the original request message,
* this property will always be set to false in the corresponding response message since the acknowledgement
* of the response, if required, will have been processed internally by the IMS Connect API. The internal ACK
* function is only supported for RESUMETPIPE_SINGLE and RESUMETPIPE_SINGLE_NOWAIT values for {@link #setResumeTpipeProcessing(int)}
*
When ackNakProvider is set to CLIENT_ACK_NAK in the original request message, this property will
* be set according to the IMS Connect protocol. Whenever this property is set to true in the response, the
* client must send in an ACK or NAK interaction as the next interaction on that connection or IMS Connect
* will respond with a protocol violation.
*
* Use the constants defined in ApiProperties
to determine the ackNakNeeded property value.
* For example,
*
* if(myTmInteraction.isAckNakNeeded() == ApiProperties.ACK_NAK_NEEDED)
* {
* // submit an ACK or NAK interaction as required as the next
* // interaction on this connection
* }
*
* @return a boolean
value indicating whether or not the response from IMS must be acknowledged
* or negatively acknowledged prior to any other activity on this connection
* @see ApiProperties#ACK_NAK_NEEDED
* @see ApiProperties#NO_ACK_NAK_NEEDED
*/
public boolean isAckNakNeeded();
/**
* Gets the asyncOutputAvailable property value.
* This property specifies whether or not there are any more asynchronous output messages queued in on
* the asynchronous hold queue for this client's tpipe in IMS and available for retrieval by this or another
* client.
* The value of this property is only valid in a non-shared queues environment. In a shared queues
* environment, the client must issue a ResumeTpipe interaction in order to know if there is asynchronous
* output available (in which case, output will be returned if there is any available).
*
* Use the constants defined in ApiProperties
to determine the asyncOutputAvailable property value.
* For example,
*
* if(myTmInteraction.isAsyncOutputAvailable() == ApiProperties.ASYNC_OUTPUT_AVAILABLE)
* {
* // retrieve additional asynchronous output or save a flag that you can use
* // to retrieve the asynchronous output at a later time
* }
*
* For more information, see Asynchronous output support
* in IMS Version 12 Communications and Connections.
* @return a boolean
value indicating whether or not there are any more asynchronous
* output messages queued on the asynchronous hold queue for this client's tpipe in IMS and
* available for retrieval by this or another client
* @see ApiProperties#ASYNC_OUTPUT_AVAILABLE
* @see ApiProperties#NO_ASYNC_OUTPUT_AVAILABLE
*/
public boolean isAsyncOutputAvailable();
/**
*
Gets the inCoversation property value.
* This response property specifies whether or not this interaction uses IMS Connect conversational transaction
* support.
* Use the constants defined in ApiProperties
to determine the InCoversation property value.
* For example,
*
* if(myTmInteraction.isInConversation() == ApiProperties.IN_CONVERSATION)
* {
* // client application needs to continue the conversation or send in
* // an interaction to end the conversation. API will not include the
* // trancode in an input message for an interaction if the
* // inConversation property value is true.
* }
*
* For more information, see
* IMS Connect conversational support in IMS Version 12 Communications and Connections.
* @return a boolean
value indicating whether or not this interaction is part of a
* currently active IMS conversation
* @see ApiProperties#IN_CONVERSATION
* @see ApiProperties#NOT_IN_CONVERSATION
*/
public boolean isInConversation();
/**
* Gets the mfsModname name property value. The mfsModname property value is set internally
* when the API client application receives an output message with a request mod message (RMM).
* This property value is the name of the requested MFS MOD.
* If the interaction fails, the modname is not returned.
* @return the mfsModname value returned by the IMS application through IMS Connect
*/
public String getMfsModname();
// Getter and setter methods for user-settable properties
// Interaction properties
/**
* Gets a boolean
value indicating whether each response message does or
* does not include LLLL
* @return a boolean
value containing whether the response messages
* returned to the IMS Connect API by IMS Connect start with LLLL (true -
* response messages processed using HWSSMPL1 in IMS Connect do start with
* LLLL) or do not start with LLLL (false - response messages processed
* using HWSSMPL0 do not start with LLLL)
* @see TmInteraction#setResponseIncludesLlll(boolean)
*/
public boolean isResponseIncludesLlll();
/**
* Sets the responseIncludesLlll property value to be used for this interaction. This property is used to tell
* the API whether each response message does or does not include LLLL. This property is most useful in client
* applications which use a non-IBM supplied user message exit which has a user message exit identifier other
* than *SAMPLE*
or *SAMPL1*
, in which case, the API needs to be told whether the
* user-written user message exit prepends an LLLL value to each response message. If the
* imsConnectUserMessageExitIdentifier property is set to *SAMPL1*
, the IBM-supplied HWSSMPL1 user
* message exit will be used and response messages processed using the HWSSMPL1 t in IMS Connect do start with
* an LLLL value so true
is returned by this method. On the other hand, if the
* imsConnectUserMessageExitIdentifier property is set to *SAMPLE*
, the HWSSMPL0 user message exit
* will be used and response messages processed using the HWSSMPL0 user message exit in IMS Connect do not start
* include an LLLL value so false
is returned by this method.
* @param aResponseIncludesLlll
* a boolean
value indicating whether the response messages
* returned to the IMS Connect API by IMS Connect do or do not start with
* an LLLL value.
* @see TmInteraction#isResponseIncludesLlll()
*/
public void setResponseIncludesLlll(boolean aResponseIncludesLlll);
/**
* Gets a byte
value representing whether the client application code or the
* internal API code will provide the acknowledgment messages to IMS Connect when required
* after receiving output from IMS Connect.
* @return a byte
value containing whether the IMS Connect API (0) or the
* client application (1) will ACK or NAK replies from IMS Connect whenever an ACK
* or NAK response is required
* @see TmInteraction#setAckNakProvider(byte)
*/
public byte getAckNakProvider();
/**
* Sets the ackNakProvider property value which specifies whether the IMS Connect API or the client
* application will ACK or NAK replies from IMS Connect whenever an ACK or NAK response is required.
* The valid values, for which the following constants are defined in ApiProperties
, are:
*
* API_INTERNAL_ACK
- Indicates that the API will submit ACK messages internally (API never
* NAKs output as it has no criteria for determining when expected output should be NAKed rather than ACKed). If
* API_INTERNAL_ACK is used, the client must not submit ACK or NAK interactions since the acknowledgement
* response will already have been sent back to IMS Connect before the client application receives
* back control after an execute()
method call has completed. The internal ACK function is
* only available when link {@link #setResumeTpipeProcessing(int)} is set to RESUMETPIPE_SINGLE_WAIT or RESUMETPIPE_SINGLE_NOWAIT.
* CLIENT_ACK_NAK
- Indicates that the client application will submit an ACK or NAK response explicitly as
* a separate interaction after a execute()
method call for a send-receive, receive, or ResumeTpipe
* interaction.
* DEFAULT_ACK_NAK_PROVIDER
- same as API_INTERNAL_ACK
*
* If a value for this property is not supplied by the client, the default value (API_INTERNAL_ACK
)
* will be used.
* Note that if API_INTERNAL_ACK is used, the IMS Connect API will internally issue a receive
* interaction after sending the ACK message, but will return the return and reason codes of the send-receive,
* receive, or ResumeTpipe interaction which triggered the response that the ACK is acknowledging. The
* exception to this is if the ACK fails, in which case an exception containing the non-zero IMS Connect
* return code and reason code for the ACK will be thrown to the client application.
*
API_INTERNAL_ACK is intended for primarily for use in transactions that return a single response message.
* When used with send-receive interactions for transactions which return multiple output messages, the
* first output message will be returned to the client application and all subsequent messages will be
* internally NAKed by the API causing them to be returned to the OTMA asynchronous output hold queue.
* While this does work, it results in inefficient use of network resources and is therefore not recommended.
* The preferred approach for transactions which return multiple response messages is to use CLIENT_ACK_NAK so
* that the subsequent messages can be received and processed by the client application without being NAKed.
* @param
* anAckNakProvider value for this interaction. Use the constants defined in ApiProperties
* to specify this parameter.
* @throws ImsConnectApiException
* @see ApiProperties#CLIENT_ACK_NAK
* @see ApiProperties#API_INTERNAL_ACK
* @see TmInteraction#getAckNakProvider()
*/
public void setAckNakProvider(byte anAckNakProvider) throws ImsConnectApiException;
// Interaction properties
/**
* Gets the cm0IgnorePurge property value.
* @return a boolean indicating if the IMS application should ignore DL/I purge calls
* for multi-segment commit mode 0 response messages prior to insertion of the last
* segment of the message.
*
* @see TmInteraction#setCm0IgnorePurge(boolean)
*/
public boolean isCm0IgnorePurge();
/**
* Sets the cm0IgnorePurge property value for this interaction. Use this property to indicate
* if the IMS application should ignore DL/I purge calls for multi-segment commit mode 0 response
* messages prior to insertion of the last segment of the message.
*
The valid values, for which the following constants are defined in ApiProperties
, are:
*
* CM0_DO_IGNORE_PURGE
- Indicate that IMS should ignore DL/I PURG calls until all segments
* of a multi-segment output message have been inserted to the TP PCB
* CM0_DO_NOT_IGNORE_PURGE
- Indicate that IMS should accept all DL/I PURG calls after a
* segment is inserted to the TP PCB
* DEFAULT_CM0_IGNORE_PURGE
- same as CM0_DO_NOT_IGNORE_PURGE
*
* If a value for this property is not supplied by the client, the default value
* (CM0_DO_NOT_IGNORE_PURGE
) will be used.
* @param acm0IgnorePurge the cm0IgnorePurge value to use for this interaction.
* Use the constants defined in ApiProperties
to specify this parameter.
* @see ApiProperties#DEFAULT_CM0_IGNORE_PURGE
* @see ApiProperties#CM0_DO_IGNORE_PURGE
* @see ApiProperties#CM0_DO_NOT_IGNORE_PURGE
* @see TmInteraction#isCm0IgnorePurge()
* @see Output message to client in IMS V11 Communications and Connections
*/
public void setCm0IgnorePurge(boolean aCm0IgnorePurge);
/**
* Gets the cancelClientId property value.
* @return a boolean indicating whether an existing connection with the same clientID will be canceled or retained for the interaction.
* client ID should be canceled or not.
*
* @see TmInteraction#setCancelClientId(boolean)
*/
public boolean isCancelClientId();
/**
* Sets the cancelClientId property value for this interaction. Use this property to indicate whether
* an existing connection with the same clientID should be canceled or retained for the interaction.
* The valid values, for which the following constants are defined in ApiProperties
, are:
*
* DO_CANCEL_CLIENTID
- Indicates that if there is another connection with the same client ID
* that connection should be closed and the requesting connection should be granted.
* DO_NOT_CANCEL_CLIENTID
- Indicates that that if there is another connection
* with the same client ID that connection should be kept live
* and the current request denied.
* DEFAULT_CANCEL_CLIENTID
- same as DO_CANCEL_CLIENTID
*
*
* If a value for this property is not supplied by the client, the default value
* (DO_CANCEL_CLIENTID
) will be used.
* @param aCancelClientID the cancelClientID value to use for this interaction.
* Use the constants defined in ApiProperties
to specify this parameter.
* @return a boolean indicating if the IMS application should ignore DL/I purge calls
* for multi-segment commit mode 0 response messages prior to insertion of the last
* segment of the message.
* @see TmInteraction#isCancelClientId()
*/
public void setCancelClientId(boolean aCancelClientID);
/**
* Specifies to IMS Connect whether to generate a unique client ID for the connection if a duplicate client ID already exists.
* To enable this property the cancelClientId property should be set to false,else the cancel clientId gets the precedence.
* The valid values, for which the following constants are defined in ApiProperties
, are:
*
* DO_GENERATE_CLIENTID
- Indicates to IMS Connect to generate the client ID for the connection.
* DO_NOT_GENERATE_CLIENTID
- Indicates to IMS Connect not to generate the client ID for the connection.
*
* DEFAULT_IMS_CONNECT_GENERATE_CLIENTID
- Same as DO_NOT_GENERATE_CLIENTID
.
*
*
* If a value for this property is not supplied by the client, the default value
* (DO_NOT_GENERATE_CLIENTID
) is used.
* @param aGenerateValue the imsConnectGenerateClientID value to use for this interaction.
* Use the constants defined in ApiProperties
to specify this parameter.
* @see ApiProperties#DEFAULT_IMS_CONNECT_GENERATE_CLIENTID
* @see ApiProperties#DO_GENERATE_CLIENTID
* @see ApiProperties#DO_NOT_GENERATE_CLIENTID
* @see TmInteraction#isImsConnectGenerateClientId()
*/
public void setGenerateClientIdWhenDuplicate(boolean aGenerateClientIdValue);
/**
* Gets the imsConnectGenerateClientId property value.
* @return a boolean indicating if a client ID will be generated by IMS Connect for a given duplicate client ID.
* @see TmInteraction#setGenerateClientIdWhenDuplicate(boolean)
*/
public boolean isGenerateClientIdWhenDuplicate();
/**
* Identifies if IMS Connect would generate a unique client ID for the connection when no client ID is
* specified by the client, or a duplicate client ID already exists for the connection.
* The valid values, for which the following constants are defined in ApiProperties
, are:
*
* DO_RETURN_CLIENTID
- Indicates to IMS Connect to return the client ID for the connection.
* DO_NOT_RETURN_CLIENTID
- Indicates to IMS Connect not to return the client ID for the connection.
* DEFAULT_RETURN_CLIENTID
- Same as DO_NOT_RETURN_CLIENTID
*
*
* If a value for this property is not supplied by the client, the default value
* (DO_NOT_RETURN_CLIENTID
) is used.
* @param aReturnClientIdValue the returnClientID value to use for this interaction.
* Use the constants defined in ApiProperties
to specify this parameter.
* @see ApiProperties#DEFAULT_RETURN_CLIENTID
* @see ApiProperties#DO_RETURN_CLIENTID
* @see ApiProperties#DO_NOT_RETURN_CLIENTID
* @see TmInteraction#isReturnClientId()
*/
public void setReturnClientId(boolean aReturnClientIdValue);
/**
* Gets the returnClientId property value.
* @return a boolean indicating if a client Id will be returned with the output
* client ID should be canceled or not.
*
* @see TmInteraction#setReturnClientId(boolean)
*/
public boolean isReturnClientId();
/**
* Gets the commitMode property value.
* @return an byte
value containing the commitMode property value
* @see ApiProperties#DEFAULT_COMMIT_MODE
* @see ApiProperties#COMMIT_MODE_0
* @see ApiProperties#COMMIT_MODE_1
* @see TmInteraction#setCommitMode(byte)
*/
public byte getCommitMode();
/**
* Sets the commitMode property value to be used for this interaction. Only one value can be specified
* per interaction. The valid values, for which the following constants are defined in ApiProperties
, are:
*
* COMMIT_MODE_0
- Commit-then-send (CM0 maps to IRM_F2 value of 0X40)
* COMMIT_MODE_1
- Send-then-commit (CM1 maps to IRM_F2 value of 0X20)
* DEFAULT_COMMIT_MODE
- same as COMMIT_MODE_0
*
* COMMIT_MODE_0 is the default used if client does not provide a value.
* A value for commit mode is required by IMS Connect and OTMA. However, the value provided for commitMode
* will be over-ridden internally if the type of interaction is only supported with a specific commit mode.
* For example, resumeTpipe and send-only interactions are only supported for Commit Mode 0 while Two-Phase
* Commit (2PC), though not supported in the initial release of the IMS Connect API, always uses Commit Mode 1.
* If the commitMode property value is over-ridden internally by the API and if the traceLevel is set to
* TRACE_LEVEL_INTERNAL, an informational trace message will be written to the trace output file.
* If a value for this property is not supplied by the client and cannot be determined based on the
* messageFunction value, the default value (COMMIT_MODE_0
) will be used. The commit mode value is passed to
* IMS Connect in the OMHDRSYN field of the OTMA header.
* @param aCommitMode byte value representing the commit mode. Use the constants defined in ApiProperties to specify this parameter.
* @throws ImsConnectApiException
* @see ApiProperties#COMMIT_MODE_0
* @see ApiProperties#COMMIT_MODE_1
* @see TmInteraction#getCommitMode()
*/
public void setCommitMode(byte aCommitMode) throws ImsConnectApiException;
/**
* Gets the IMS Connect User Message Exit Identifier that specifies which User
* Message Exit is to be used by IMS Connect for this interaction.
* @return a String
value containing the imsConnectUserMessageExit property value
* @see ApiProperties#IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSSMPL0
* @see ApiProperties#IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSSMPL1
* @see TmInteraction#setImsConnectUserMessageExitIdentifier(String)
*/
public String getImsConnectUserMessageExitIdentifier();
/**
* Sets the imsConnectUserMessageExitIdentifier property value which is used to determine which IMS Connect
* user message exit is to be used for this interaction after the complete input or output message has been
* received by IMS Connect. Only one value can be specified per interaction.
* The valid values, for which the following constants are defined in ApiProperties
, are:
*
* IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSSMPL0
- Use the HWSSMPL0 user message
* exit (identifier is *SAMPLE*)
* IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSSMPL1
- Use the HWSSMPL1 user message
* exit (identifier is *SAMPL1*)
* IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSCSLO1
- Use the HWSCSLO1 user message exit. Use
* this exit with the Type-2 command interaction type only.
* DEFAULT_IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER
- same as IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSSMPL1
* *USRMOD*
- Set this property to the name of a user exit routine module to request that IMS Connect load that module
* for this interaction. The specified module must follow the same input and output message format as the HWSSMPL0 and HWSSMPL1
* user message exits. You must also request the response LLLL by using setResponseIncludesLlll(true).
*
*
* If a value for this property is not supplied by the client, the default value (IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSSMPL1
) will be used.
* For more details on the use of this property, see the topic
* "IMS Connect exit routines"
* in the V13 IMS Communications and Connections documentation.
* @param anIConUserMessageExitIdentifier
* a String value containing the IMS Connect user message exit identifier to be used for this interaction.
* Use the constants defined in ApiProperties to specify this parameter.
* @throws ImsConnectApiException
* @see ApiProperties#IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSSMPL0
* @see ApiProperties#IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSSMPL1
* @see ApiProperties#IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSCSLO1
* @see TmInteraction#getImsConnectUserMessageExitIdentifier()
*/
public void setImsConnectUserMessageExitIdentifier(String anIConUserMessageExitIdentifier) throws ImsConnectApiException;
/**
* Gets imsDatastoreName property value, which identifies the target IMS datastore for this interaction.
* @return a String
value containing the imsDatastoreName property value
* @see TmInteraction#setImsDatastoreName(String)
*/
public String getImsDatastoreName();
/**
* Sets the imsDatastoreName property value.
* The imsDatastoreName property specifies the target IMS datastore name (a string of 1 to 8 uppercase
* alphanumeric (A through Z, 0 to 9) or special (@, #, $) characters identifying the IMS destination
* ID as defined in a DATASTORE statement in the IMS Connect configuration member. The client or the IMS
* Connect user message exit must provide a valid value for this property. Neither IMS Connect nor the IMS
* Connect user message exit validates the imsDatastoreName value. Instead, it is copied directly into the
* IRM_IMSDESTID field. The unvalidated imsDatastoreName value will also be copied by the IMS Connect user
* message exit into the OMUSR_DESTID field in the OTMA header. If a datastore by that name cannot be found,
* IMS Connect will return a datastore not found error (Return Code 4, Reason Code NFNDDST) to the client.
* If the datastore is found but is currently closed, IMS Connect will return a datastore closed error
* (Return Code 12, Reason Code DSCLOSE) to the client.
* @param aDataStoreName
* the IMS datastore name to be used for this interaction.
* @throws ImsConnectApiException
* @see TmInteraction#getImsDatastoreName()
*/
public void setImsDatastoreName(String aDataStoreName) throws ImsConnectApiException;
/**
* Gets a boolean value representing whether the data segments in the input messages include
* an LLZZ (plus trancode if applicable) prefix.
* @return a boolean
value containing the inputMessageDataSegmentsIncludeLlzzAndTrancode
* property value
* @see ApiProperties#INPUT_MESSAGE_DATA_SEGMENTS_DO_NOT_INCLUDE_LLZZ_AND_TRANCODE
* @see ApiProperties#INPUT_MESSAGE_DATA_SEGMENTS_DO_INCLUDE_LLZZ_AND_TRANCODE
* @see TmInteraction#setInputMessageDataSegmentsIncludeLlzzAndTrancode(boolean)
*/
public boolean isInputMessageDataSegmentsIncludeLlzzAndTrancode();
/**
* Sets the inputMessageDataSegmentsIncludeLlzzAndTrancode property value to be used for this interaction.
* This property is used to tell the API whether the client application code has already included
* an LLZZ value and a trancode value in the message data provided to the InputMessage object.
* When the inputMessageDataSegmentsIncludeLlzzAndTrancode property is set to true
, the API
* will interpret the first four bytes of the provided input message data as an LLZZ value and
* will not insert a trancode into the input message data byte array to be sent to IMS
* Connect (on the assumption that the trancode is in the provided input message data.)
*
If this property is set to false
, the API uses the trancode value specified with
* {@link TmInteraction#setTrancode(String)}, or the default value ("TRANCODE") if the value has not been set.
* @param anInputMessageDataSegmentsIncludeLlzzAndTrancode
* a boolean value representing whether or not the data segments
* in the input messages include an LLZZ prefix and a trancode if required
* @see TmInteraction#isInputMessageDataSegmentsIncludeLlzzAndTrancode()
*/
public void setInputMessageDataSegmentsIncludeLlzzAndTrancode(boolean anInputMessageDataSegmentsIncludeLlzzAndTrancode);
/**
* Gets the message type.
* @return an int
value containing the inputMessageOptions property value
* @see ApiProperties#INPUT_MESSAGE_OPTIONS_NO_OPTIONS
* @see ApiProperties#INPUT_MESSAGE_OPTIONS_EBCDIC
* @see TmInteraction#setInputMessageOptions(int)
*/
public int getInputMessageOptions();
/**
* Sets the InputMessageOptions property value which specifies the characteristics of the input message
* byte array supplied by the client. Multiple values, if specified, are logically OR'd together.
* The valid values, for which the following constants are defined in ApiProperties
, are:
*
* INPUT_MESSAGE_OPTIONS_NO_OPTIONS
- Hex value of 0X00. No input message options specified.
* INPUT_MESSAGE_OPTIONS_EBCDIC
- Hex value of 0X40. Input message is in EBCDIC.
* No translation from ASCII to EBCDIC required in the IMS Connect user message exit.
* DEFAULT_INPUT_MESSAGE_OPTIONS
- Uses INPUT_MESSAGE_OPTIONS_NO_OPTIONS
.
*
* If a value for this property is not supplied by the client,
* the default value (INPUT_MESSAGE_OPTIONS_NO_OPTIONS
) will be used.
* @param inputMessageOptions The inputMessageOptions to set. Use the constants defined in ApiProperties to specify this parameter.
* @throws ImsConnectApiException
* @see ApiProperties#INPUT_MESSAGE_OPTIONS_NO_OPTIONS
* @see ApiProperties#INPUT_MESSAGE_OPTIONS_EBCDIC
* @see TmInteraction#getInputMessageOptions()
*/
public void setInputMessageOptions(int inputMessageOptions) throws ImsConnectApiException;
/**
* Gets the type of interaction with IMS Connect to be performed.
* @return a String
value containing the InteractionTypeDescription property value
* @see ApiProperties#INTERACTION_TYPE_DESC_ACK
* @see ApiProperties#INTERACTION_TYPE_DESC_CANCELTIMER
* @see ApiProperties#INTERACTION_TYPE_DESC_ENDCONVERSATION
* @see ApiProperties#INTERACTION_TYPE_DESC_NAK
* @see ApiProperties#INTERACTION_TYPE_DESC_RECEIVE
* @see ApiProperties#INTERACTION_TYPE_DESC_RESUMETPIPE
* @see ApiProperties#INTERACTION_TYPE_DESC_SENDONLY
* @see ApiProperties#INTERACTION_TYPE_DESC_SENDONLYACK
* @see ApiProperties#INTERACTION_TYPE_DESC_SENDRECV
* @see TmInteraction#setInteractionTypeDescription(String)
*/
public String getInteractionTypeDescription();
/**
* Sets the interactionTypeDescription property value which specifies the function to be performed in
* IMS Connect and/or OTMA in this interaction. Only one function can be specified at a time.
* The valid values, for which the following constants are defined in ApiProperties
, are:
*
* INTERACTION_TYPE_DESC_ACK
- Positive acknowledgment (IRM_F4 = 'A').
* Must be sent to IMS Connect with no application data segment in the message.
* Used to accept the IMS response to an IMS transaction request message in which the sync level was set to
* CONFIRM.
* INTERACTION_TYPE_DESC_CANCELTIMER
- Cancel wait for response from IMS (IRM_F4 = 'C').
* Must be sent to IMS Connect with no application data segment in the message. Used to cancel the current
* CONN state (wait for response from IMS) on another connection which is using the same client ID as this
* connection and is connected to the same port on this IMS Connect. Then IMS Connect will return that other
* connection to RECV state and send a canceltimer complete message to the client and disconnect this connection.
* INTERACTION_TYPE_DESC_END_CONVERSATION
- End conversation associated with this convID and/or
* connection (IRM_F4 = 'D')
* INTERACTION_TYPE_DESC_NAK
- Negative acknowledgment (IRM_F4 = 'N'). Must be sent to
* IMS Connect with no application data segment in the message. Used to reject the IMS response to an IMS
* transaction request message in which the sync level was set to CONFIRM.
* INTERACTION_TYPE_DESC_RECEIVE
- Receive-only request to receive a single response message
* from IMS. This corresponds to an internal API interaction type that causes the API to do only a receive on
* the TCP/IP socket for the current interaction. It does not involve sending any type of request to IMS Connect,
* so there is no IRM created and therefore, no flag setting associated with this interaction type.
* INTERACTION_TYPE_DESC_RESUMETPIPE
- Request asynchronous output (IRM_F4 = 'R').
* Must be sent to IMS Connect with no application data segment in the message. Used to request asynchronous
* output data from the OTMA Tpipe whose name is the same as the client ID for this connection. The
* RESUMETPIPE must execute on a transaction socket or persistent socket using commit mode zero.
* INTERACTION_TYPE_DESC_SENDONLY
- Send-only IMS transaction request with no response from
* IMS expected to be returned (IRM_F4 = 'S'). Used to send a transaction request for a non-response mode
* transaction to IMS Connect and IMS. The application data segment must contain the transaction code and input
* data required for that transaction. The DFS2082 message that would normally be returned to the client if the
* host application terminates without issuing an insert to the IOPCB is suppressed for SENDONLY requests.
* SENDONLY requests must execute as commit mode zero. Any response resulting from a SENDONLY function will be
* queued on an asynchronous hold queue associated with the tpipe used by the client application and can be retrieved
* later by submitting a RESUMETPIPE messageFunction.
* INTERACTION_TYPE_DESC_SENDONLYACK
- Send-only with acknowledgment IMS transaction
* request (IRM_F4 = 'K'). Used to send a transaction request for a non-response mode transaction to IMS
* Connect and IMS. The application data segment must contain the transaction code and input data required
* for that transaction. The DFS2082 message that would normally be returned to the client if the host
* application terminates without issuing an insert to the IOPCB is suppressed for SENDONLY requests.
* SENDONLY requests must execute as commit mode zero. The INTERACTION_TYPE_SENDONLYACK
function differs
* from the INTERACTION_TYPE_SENDONLY
function in that the client application receives an ACK response message
* from OTMA for each input message successfully enqueued by IMS. All output generated by the
* INTERACTION_TYPE_SENDONLYACK
interaction is sent to the asynchronous hold queue.
* INTERACTION_TYPE_DESC_SENDONLYXCFORDEREDDELIVERY
- Send-only with XCF ordered delivery IMS
* transaction request (IRM_F4 = 'S', IRM_F3 = 0x10). Used to send a transaction request for a non-response mode
* transaction to IMS Connect and IMS. The application data segment must contain the transaction code and input
* data required for that transaction. The DFS2082 message that would normally be returned to the client if the host
* application terminates without issuing an insert to the IOPCB is suppressed for SENDONLY requests. SENDONLY
* requests must execute as commit mode zero. The INTERACTION_TYPE_DESC_SENDONLYXCFORDEREDDELIVERY
* function is similar to the INTERACTION_TYPE_SENDONLY
function except that the client application is
* guaranteed that all input messages are delivered by XCF to IMS OTMA in the order in which they are received by XCF.
* Note that the ordered delivery of input messages does not imply that the response messages will be returned to the
* client application in that order. This is because the order of delivery of response messages is dependent not only
* on the order that the input messages are received, but also on the time that it takes to process each message which
* will vary due to a number of factors including the transaction execution time which varies from one transaction to
* another. All output generated by the INTERACTION_TYPE_DESC_SENDONLYXCFORDEREDDELIVERY
interaction is
* sent to the asynchronous hold queue.
* INTERACTION_TYPE_DESC_SENDONLY_CALLOUT_RESPONSE
- Send-only IMS synchronous callout response message.
* Used to send a normal or error response to an IMS callout request. The callout correlator token in the original
* callout request message (retrieved with {@link TmInteraction#getCorrelatorToken()}) must be set with
* {@link TmInteraction#setCorrelatorToken(byte[])} for the response to associate the response message with the original request.
* Use {@link #setCalloutResponseMessageType(String)} to specify a normal (CALLOUT_RESPONSE_MESSAGE) or error
* (CALLOUT_ERROR_MESSAGE) response. For a normal response, set the callout response message data in the
* {@link InputMessage} object for the interaction. For an error response, you can include a return code with
* {@link TmInteraction#setNakReasonCode(short)}.
* INTERACTION_TYPE_DESC_SENDONLYACK_CALLOUT_RESPONSE
- Send-only IMS synchronous callout response message with
* acknowledgment. You can use this interaction type to return a response message or an error response.
* This interaction type is the same as INTERACTION_TYPE_DESC_SENDONLY_CALLOUT_RESPONSE
, but
* IMS sends an ACK or NAK response to the client application after it receives the response message. You can use this
* interaction type for applications where you must know whether the IMS application successfully received
* the callout response message. After the {@link #execute()} method is called for the interaction,
* the API issues an internal call to retrieve the ACK or NAK response from IMS. The return and reason code
* associated with the ACK or NAK reply can be retrieved with the {@link #getImsConnectReturnCode()} and
* {@link #getImsConnectReasonCode()} methods. If the return code and reason code are 0, the response was
* a positive ACK indicating that the message was received successfully.
* INTERACTION_TYPE_DESC_SENDRECV
- Send-Receive IMS transaction request with a response from
* IMS expected to be returned IRM_F4 = ' ' (single blank character)). A blank character (0X40 for EBCDIC
* messages, 0X20 for ASCII messages) is used to send SENDRECV requests for response-mode IMS transaction
* requests to IMS Connect and IMS. The application data segment must contain the transaction code and input
* data required for that transaction for IMS non-conversational transactions or for the first iteration of
* an IMS conversational transaction. The application data segment for subsequent iterations of an IMS
* conversational transaction does not contain a transaction code but rather contains the input data only.
* This is the default value.
* INTERACTION_TYPE_DESC_TYPE2_COMMAND
- Send a type-2 command to an IMS Connect with a registered
* CSL OM (Common Service Layer Operations Manager) and one or more command processing clients.
* DEFAULT_INTERACTION_DESC_TYPE
- same as INTERACTION_TYPE_DESC_SENDRECV
*
* If a value for this property is not supplied by the client, the default value (INTERACTION_TYPE_DESC_SENDRECV
) will be used.
* @param anInterTypeDesc
* type of interaction to be executed. Use the constants defined in ApiProperties to specify this parameter.
* @throws ImsConnectApiException
* @see ApiProperties#INTERACTION_TYPE_DESC_ACK
* @see ApiProperties#INTERACTION_TYPE_DESC_CANCELTIMER
* @see ApiProperties#INTERACTION_TYPE_DESC_ENDCONVERSATION
* @see ApiProperties#INTERACTION_TYPE_DESC_NAK
* @see ApiProperties#INTERACTION_TYPE_DESC_RECEIVE
* @see ApiProperties#INTERACTION_TYPE_DESC_RESUMETPIPE
* @see ApiProperties#INTERACTION_TYPE_DESC_SENDONLY
* @see ApiProperties#INTERACTION_TYPE_DESC_SENDONLYACK
* @see ApiProperties#INTERACTION_TYPE_DESC_SENDONLYXCFORDEREDDELIVERY
* @see ApiProperties#INTERACTION_TYPE_DESC_SENDONLY_CALLOUT_RESPONSE
* @see ApiProperties#INTERACTION_TYPE_DESC_SENDONLYACK_CALLOUT_RESPONSE
* @see ApiProperties#INTERACTION_TYPE_DESC_TYPE2_COMMAND
* @see ApiProperties#INTERACTION_TYPE_DESC_SENDRECV
* @see TmInteraction#getInteractionTypeDescription()
*/
public void setInteractionTypeDescription(String anInterTypeDesc) throws ImsConnectApiException;
/**
* Gets the LTERM override name.
* @return a String
value containing the ltermOverrideName property value
* @see TmInteraction#setLtermOverrideName(String)
*/
public String getLtermOverrideName();
/**
* Sets the ltermOverrideName property value to a valid MVS-style name which is a string of 1 to 8 uppercase alphanumeric
* (A through Z, 0 to 9) or special (@, #, $) characters. This field can be set to a valid name or to blanks.
* The ltermOverrideName is copied by the IMS Connect user message exit to the OMHDRLTM field in the OTMA header.
* The specified IMS Lterm override name can also be ignored and replaced by a value dynamically or statically
* determined in the IMS Connect user message exit. Neither IMS Connect nor the IMS Connect user message exit
* validates the ltermOverrideName value.
* If there is a non-blank, non-null Lterm override name value after the message is processed in the IMS
* Connect user exit, OTMA will place that value in the IOPCB LTERM field. If instead, the Lterm override name
* value is all blanks or null, OTMA will place the IMS Connect-defined Tpipe name in the IOPCB LTERM field.
* The TPIPE name is set to the CLIENT ID for commit mode 0 interactions and set to the PORT number for commit
* mode 1 interactions.
*
If you use the LTERM value in the IOPCB to make logic decisions, you must be careful to observe the
* naming conventions of the IOPCB LTERM name.
*
Note that the default value for ltermOverrideName is a string of eight blank characters, and the
* ltermOverrideName property must be changed to a valid LTerm override name before use.
* @param anLtermOverrideName the ltermOverrideName to set.
* @throws ImsConnectApiException
* @see TmInteraction#getLtermOverrideName()
*/
public void setLtermOverrideName(String anLtermOverrideName) throws ImsConnectApiException;
/**
* Gets the otmaTransactionExpiration property value.
* @return a boolean
value indicating whether IMS Connect will pass a transaction expiration
* time to OTMA for this interaction. If this value is true, OTMA halts processing of the
* transaction for this interaction if the transaction has not yet completed or IMS Connect
* has not yet received the transaction response when the IMS Connect timeout expires.
* @see TmInteraction#setOtmaTransactionExpiration(boolean)
*/
public boolean isOtmaTransactionExpiration();
/**
* Sets the otmaTransactionExpiration property that controls the transaction expiration
* time that OTMA uses for the current interaction.
*
If the otmaTransactionExpiration property is turned on (set to true
,) IMS Connect will
* pass a transaction expiration time that matches the expiration time specified for its TIMEOUT value for
* that interaction. OTMA then uses that value as its transaction expiration time, overriding the
* transaction expiration set for that transaction in IMS.
*
If the otmaTransactionExpiration property is turned off (set to false
,) IMS Connect will
* not pass a transaction expiration time. In this case, OTMA either uses the transaction expiration time
* that was set in IMS, or disables transaction expiration if no transaction expiration time was specified
* in IMS.
*
The valid values, for which the following constants are defined in ApiProperties
, are:
*
* DO_NOT_EXPIRE_OTMA_TRANSACTION_ON_IMS_CONNECT_TIMEOUT
: false
* DO_EXPIRE_OTMA_TRANSACTION_ON_IMS_CONNECT_TIMEOUT
: true
* DEFAULT_OTMA_TRANSACTION_EXPIRATION
:
* Uses DO_NOT_EXPIRE_OTMA_TRANSACTION_ON_IMS_CONNECT_TIMEOUT
*
(false)
*
* If a value for this property is not supplied by the client, the default value (SET_OTMA_TRANSACTION_EXPIRATION_OFF
) will be used.
* For more details on the use of this property, see the topic
* "IMS Connect transaction expiration support"
* in IMS Version 12 Communications and Connections.
* @param otmaTransactionExpiration
* the otmaTransactionExpiration to set. Use the constants defined in ApiProperties to specify this parameter.
* @see ApiProperties#DO_EXPIRE_OTMA_TRANSACTION_ON_IMS_CONNECT_TIMEOUT
* @see ApiProperties#DO_NOT_EXPIRE_OTMA_TRANSACTION_ON_IMS_CONNECT_TIMEOUT
* @see ApiProperties#DEFAULT_OTMA_TRANSACTION_EXPIRATION
* @see TmInteraction#isOtmaTransactionExpiration()
*/
public void setOtmaTransactionExpiration(boolean otmaTransactionExpiration);
/**
* Gets a boolean
value representing whether or not the target IMS Connect supports
* returning the IMS Connect protocol level in the CSM. This value is determined internally by the API
* based on information in the previous response from IMS Connect received by the API. If
* isProtocolLevelAvailable() returns a value of true when preparing to issue an ACK of a CM0
* SendReceive response, the getProtocolLevel() method can be used to determine if the target IMS
* Connect supports CM0 ACK NO WAIT.
* @return the protocolLevelAvailable boolean value
* @see ApiProperties#PROTOCOL_LEVEL_BASE (CM0_ACK_NOWAIT not supported)
* @see ApiProperties#PROTOCOL_LEVEL_CM0_ACK_NOWAIT_SUPPORT
* @see TmInteraction#getProtocolLevel()
*/
public boolean isProtocolLevelAvailable();
/**
* Gets a byte
value representing the protocol level of the target IMS Connect which
* indicates whether or not the target IMS Connect supports CM0 ACK NO WAIT. This value is determined
* internally by the API based on information in the previous response from IMS Connect received by the
* API. If getProtocolLevel() returns a value of ApiProperties#PROTOCOL_LEVEL_CM0_ACK_NOWAIT_SUPPORT
* when preparing to issue an ACK of a CM0 SendReceive response, the API will be able to use its CM0
* ACK NO WAIT processing when sending in an ACK to a CM0 SendReceive response. Otherwise, the API
* will not use its CM0 ACK NO WAIT processing when sending in an ACK to a CM0 SendReceive response.
* @return the protocolLevelAvailable byte value
* @see ApiProperties#PROTOCOL_LEVEL_BASE (CM0_ACK_NOWAIT not supported)
* @see ApiProperties#PROTOCOL_LEVEL_CM0_ACK_NOWAIT_SUPPORT
* @see TmInteraction#isProtocolLevelAvailable()
*/
public byte getProtocolLevel();
/**
* Gets a boolean
value representing whether or not the API should wait for a timeout response
* after an ACK of a CM0 response if the target IMS Connect supports CM0 ACK No Wait.
* @return a boolean
value containing the useCM0AckNoWait property value
* @see ApiProperties#DO_USE_CM0_ACK_NOWAIT
* @see ApiProperties#DO_NOT_USE_CM0_ACK_NOWAIT
* @see ApiProperties#DEFAULT_USE_CM0_ACK_NOWAIT
* @see TmInteraction#setUseCM0AckNoWait(boolean)
* @return the useCM0AckNoWait value
*/
public boolean isUseCM0AckNoWait();
/**
* Sets the useCM0AckNoWait property that controls whether or not user wants the API to wait for a timeout
* response after an ACK of a CM0 (Commit Mode 0) response. This property is used to shield the API user
* from the need to understand the details for using IMS Connect's CM0 ACK (and NAK) No Wait protocol.
*
If the useCM0AckNoWait property is turned on (set to true
) and IMS Connect has indicated
* in a CSM for the response that you are acknowledging that it supports CM0 ACK No Wait, the API will set
* the appropriate values in the IRM to tell IMS Connect that it is executing a CM0 ACK No Wait and therefore
* will not be waiting for a timeout response after sending in the ACK.
*
If the useCM0AcNakNoWait property is turned off (set to false
) the API will not override the
* the "normal" IRM values with the values needed for a CM0 Ack No Wait message and will do a receive for the
* timeout response from IMS Connect after sending in the ACK.
*
The valid values, for which the following constants are defined in ApiProperties
, are:
*
* DO_USE_CM0_ACK_NOWAIT
(true)
* DO_NOT_USE_CM0_ACK_NOWAIT
(false)
* DEFAULT_USE_CM0_ACK_NOWAIT
- same as DO_NOTUSE_CM0_ACK_NOWAIT
(true)
*
* If a value for this property is not supplied by the client, the default value (DO_USE_CM0_ACK_NOWAIT
)
* will be used.
* For more details on the use of this property, see the
* "IMS Connect protocol level"
* topic in IBM Knowledge Center.
* @see ApiProperties#DO_USE_CM0_ACK_NOWAIT
* @see ApiProperties#DO_NOT_USE_CM0_ACK_NOWAIT
* @see ApiProperties#DEFAULT_USE_CM0_ACK_NOWAIT
* @see TmInteraction#isUseCM0AckNoWait()
* @param aUseCM0AckNoWait value to set
*/
public void setUseCM0AckNoWait(boolean aUseCM0AckNoWait);
/**
* Gets a boolean
value representing whether or not the API should request that IMS Connect
* return a response message containing a DFS2082 instead of a timeout error response when a CM0 SendReceive
* interaction times out in IMS Connect.
* @return a boolean
value containing the returnDFS2082AfterCM0SendRecvNoResponse property value
* @see ApiProperties#DO_RETURN_DFS2082_AFTER_CM0_SENDRECV_NO_RESPONSE
* @see ApiProperties#DO_NOT_RETURN_DFS2082_AFTER_CM0_SENDRECV_NO_RESPONSE
* @see ApiProperties#DEFAULT_RETURN_DFS2082_AFTER_CM0_SENDRECV_NO_RESPONSE
* @see TmInteraction#setReturnDFS2082AfterCM0SendRecvNoResponse(boolean)
*/
public boolean isReturnDFS2082AfterCM0SendRecvNoResponse();
/**
* Sets the returnDFS2082AfterCM0SendRecvNoResponse property value with a boolean
value
* representing whether or not the API should request that IMS Connect return a response message
* containing a DFS2082 instead of a timeout error response when a CM0 SendReceive interaction
* times out in IMS Connect.
* @param aReturnDFS2082AfterCM0SendRecvNoResponse the returnDFS2082AfterCM0SendRecvNoResponse to set
* @see ApiProperties#DO_RETURN_DFS2082_AFTER_CM0_SENDRECV_NO_RESPONSE
* @see ApiProperties#DO_NOT_RETURN_DFS2082_AFTER_CM0_SENDRECV_NO_RESPONSE
* @see ApiProperties#DEFAULT_RETURN_DFS2082_AFTER_CM0_SENDRECV_NO_RESPONSE
* @see TmInteraction#isReturnDFS2082AfterCM0SendRecvNoResponse()
*/
public void setReturnDFS2082AfterCM0SendRecvNoResponse(boolean aReturnDFS2082AfterCM0SendRecvNoResponse);
/**
* Gets a boolean
value representing whether or not IMS Connect should pass back to the client
* the MOD name returned by the IMS application.
* @return a boolean
value containing the returnMfsModname property value
* @see ApiProperties#DO_RETURN_MFS_MODNAME
* @see ApiProperties#DO_NOT_RETURN_MFS_MODNAME
* @see TmInteraction#setReturnMfsModname(boolean)
*/
public boolean isReturnMfsModname();
/**
* Sets the returnMfsModname property value which specifies if the MFS MOD name is to be returned to the
* client by OTMA and IMS Connect. This value determines if an RMM (Request Mod Message) segment is built by IMS
* Connect and returned to the client as part of the response message. Only one value can be specified per interaction.
* The valid values, for which the following constants are defined in ApiProperties
, are:
*
* DO_NOT_RETURN_MFS_MODNAME
- Boolean value false. User requests no MFS MOD name to be
* returned (maps to IRM_F1 value of 0X00).
* DO_RETURN_MFS_MODNAME
- Boolean value true. User requests MFS MOD name to be returned
* (maps to IRM_F1 value of 0X80)
* DEFAULT_RETURN_MFS_MODNAME
- same as DO_NOT_RETURN_MFS_MODNAME
*
* If a value for this property is not supplied by the client, the default value (DO_NOT_RETURN_MFS_MODNAME
) will be used.
* @param aRreturnMfsModname to set. Use the constants defined in ApiProperties to specify this parameter.
* @see ApiProperties#DO_NOT_RETURN_MFS_MODNAME
* @see ApiProperties#DO_RETURN_MFS_MODNAME
* @see TmInteraction#isReturnMfsModname()
*/
public void setReturnMfsModname(boolean aRreturnMfsModname);
/**
* Gets the sync level.
* @return a byte
value containing the syncLevel property value
* @see ApiProperties#SYNC_LEVEL_CONFIRM
* @see ApiProperties#SYNC_LEVEL_NONE
* @see TmInteraction#setSyncLevel(byte)
*/
public byte getSyncLevel();
/**
* Sets the syncLevel property value which specifies the synch level to be used for this interaction.
* Only one value can be specified.
* The valid values, for which the following constants are defined in ApiProperties
, are:
*
* SYNC_LEVEL_NONE
- Maps to IRM_F3 byte value of 0X00. If a transaction is specified with
* SYNC_LEVEL_NONE, no acknowledgement is required from the client.
* SYNC_LEVEL_CONFIRM
- Maps to IRM_F3 byte value of 0X01. If a transaction is specified with
* SYNC_LEVEL_CONFIRM, the client is required to send an acknowledgement to signal to IMS Connect whether or not
* the output message was successfully (ACK) or unsuccessfully (NAK) processed by the client.
* DEFAULT_SYNC_LEVEL
- SYNC_LEVEL_CONFIRM
*
* If a value for this property is not supplied by the client, the default value (SYNC_LEVEL_CONFIRM
) will be used.
* A value for synch level is required by IMS Connect and OTMA. However, the value provided for synch level
* will be over-ridden internally if the commit mode or type of the interaction is only supported with a
* specific synch level. For example, for Commit Mode 0 interactions, syncLevel must be set to CONFIRM. For
* Commit Mode 1 interactions, synch level can be set to NONE or CONFIRM. If a value for synch level is not
* supplied by the client and cannot be determined based on the messageFunction and commitMode values, the
* default value (SYNC_LEVEL_CONFIRM
) will be used. If the syncLevel property value is over-ridden
* internally by the API and if the traceLevel is set to TRACE_LEVEL_INTERNAL, an informational trace
* message will be written to the trace output file.
*
For more details on the synch level property, see the topic
* "Commit mode and synch level definitions" in the V11 Communications and Connections guide documentation.
* @param aLevel
* a byte value representing the syncLevel. Use the constants defined in ApiProperties to specify this parameter.
* @throws ImsConnectApiException
* @see ApiProperties#SYNC_LEVEL_CONFIRM
* @see ApiProperties#SYNC_LEVEL_NONE
* @see TmInteraction#getSyncLevel()
*/
public void setSyncLevel(byte aLevel) throws ImsConnectApiException;
/**
* Gets the IMS transaction code (trancode).
* @return a String
value containing the trancode property value
* @see TmInteraction#setTrancode(String)
*/
public String getTrancode();
/**
* Sets the trancode property value. The trancode property specifies the IMS transaction code which is a
* string of uppercase alphanumeric (A through Z, 0 to 9) or special (@, #, $) characters. The trancode
* property identifies the IMS application (transaction) to be executed. This trancode property is used by
* the API to set the trancode in the data in situations where the client application has provided an input
* data byte array that is not prefixed with LLZZ and the trancode. The first 8 characters of the trancode
* property are also used internally by IMS Connect and OTMA to identify the trancode of the target IMS
* application.
*
If the trancode property value is in EBCDIC and contains invalid characters (i.e. characters
* other than those listed above), an invalid trancode error will be returned to the client from OTMA.
* If the trancode property value is in ASCII and contains invalid characters, an invalid trancode error
* will be returned to the client by IMS Connect. If the trancode value is valid but cannot be found by IMS,
* a trancode not found error will be returned to the client from IMS. The trancode value is placed in the
* IRM_TRNCOD field in the IRM.
*
The trancode must be formatted in the same format required by IMS Connect and the IMS
* transaction. For example, if the trancode is always followed by 5 blanks before the LLZZ for the first
* data segment starts, the trancode field must be set to a value that includes those 5 extra bytes. In the
* case of the IMS Sample/IVP IVTNO program, the trancode would be set to one of the valid trancodes such as
* IVTNO followed by 5 bytes of spaces.
*
Note that the DEFAULT_TRANCODE has the String value "TRANCODE", and the trancode property must be
* changed to a trancode that is defined in the target IMS system before use.
* @param aTrancode
* the trancode to be used for this interaction.
* @throws ImsConnectApiException
* @see TmInteraction#getTrancode()
*/
public void setTrancode(String aTrancode) throws ImsConnectApiException;
// Message encoding properties
/**
* Gets the IMS Connect codepage property value for messages sent to and received from IMS
* Connect. Used for conversion between the client codepage and the IMS Connect codepage
* for this interaction.
* @return a String
value containing the imsConnectCodepage property value
* @see TmInteraction#setImsConnectCodepage(String)
*/
public String getImsConnectCodepage();
/**
* Sets the imsConnectCodepage property value which specifies the codepage for messages
* from IMS Connect.
*
This property is used internally by the API to determine what codepage is used for
* conversion of messages passed back and forth between the client and IMS Connect.
* Examples of valid values are:
*
* "037"
- EBCDIC code page 037 for Australia, Brazil, Canada, New Zealand,
* Portugal, South Africa and USA
* "1047"
- EBCDIC code page 1047 for Open Systems (MVS C compiler)
* "1252"
- ASCII code page 1252 for Windows Latin-1 (Western Europe) (SAP
* Codepage 1100, ISO-8859-1) (English, French, German, Danish, Norwegian, Finnish, Swedish,
* Italian, Spanish, Icelandic and Dutch)
*
* The DEFAULT_IMS_CONNECT_CODEPAGE has the value "037"
.
* @param aCodepage
* codepage to be used for conversion from the client codepage to the IMS Connect
* codepage for input request messages and vice versa for the output response
* messages for this interaction - used internally by the API
* @see TmInteraction#getImsConnectCodepage()
*/
public void setImsConnectCodepage(String aCodepage); //throws ImsConnectApiException;
// Timeout properties
/**
* Gets the imsConnectTimeout property value.
* @return an int
value containing the IMS Connect timeout property value
* @see TmInteraction#setImsConnectTimeout(int)
*/
public int getImsConnectTimeout();
/**
* Sets the imsConnectTimeout property value.
* The imsConnectTimeout property specifies the time that IMS Connect will
* wait to receive a response from IMS to be returned to the client. This property is
* specified by the client on any input and updated by IMS Connect in the reply to the
* client with the actual IRM_TIMER value used for that interaction. The default value is
* IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_DEFAULT_VALUE
(timeout determined by IMS Connect based on its defaults or configured TIMEOUT value).
* The following request types use the imsConnectTimeout to set the IMS Connect response timeout:
*
* - RESUME TPIPE
*
- ACK or NAK
*
- IMS transaction or IMS Command (both include data)
*
*
* For more details on the use of this property, see the topic
*
* "Timeout intervals on input messages"
* in the V11 IMS Communications and Connections guide documentation.
*
* The valid values are:
*
* IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_DEFAULT_VALUE
- Use the IMS Connect TIMEOUT value as follows:
*
* - Use 0.25 second wait for ResumeTpipe non-single ACK/NAK calls
*
- Use 2 seconds for all RESUME_TPIPE calls
*
- Use IMS Connect's configured TIMEOUT value (or the default value if a TIMEOUT value
* has not been configured) for IMS transactions or IMS commands
*
* IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_NOWAIT_VALUE
- Use the IMS Connect-defined NOWAIT value as follows:
*
* - 2 second delay for:
*
* - RESUME_TPIPE request
*
- conversational iteration (with or without trancode)
*
- ACK or NAK associated with a conversational transaction
*
- non-conversational transaction
*
*
* - 0.25 second delay for:
*
* - ACK or NAK associated with a non-conversational Commit Mode 1, Sync level confirm transaction
*
- ACK or NAK associated with a RESUMETPIPE_AUTO or RESUMETPIPE_NOAUTO
*
- ACK or NAK associated with non-conversational Commit Mode 0, Sync Level Confirm transaction
*
*
* - 0 second delay for:
*
* - SENDONLY
*
- ACK or NAK associated with RESUMETPIPE_SINGLE_WAIT or RESUMETPIPE_SINGLE_NOWAIT
*
*
*
* TIMEOUT_WAIT_FOREVER
- For the imsConnectTimeout property, this constant sets an IRM_TIMER value of X'FF'.
* - Specific time value between 10 milliseconds and 60 minutes (see following table for actual values used):
*
*
* Value specified
* Resulting IRM_TIMER value
*
*
* 0x01 - 0x19
* 10 - 250 milliseconds in 10 milliseconds increments
*
*
* 0x1A - 0x27
* 300 - 950 milliseconds in 50 milliseconds increments
*
*
* 0x28 - 0x63
* 1 - 30 seconds in 1 second increments
*
*
* 0x64 - 0x9E
* 1 - 60 minutes in 1 minute increments
*
*
* DEFAULT_IMS_CONNECT_TIMEOUT
- same as IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_DEFAULT_VALUE
*
* @param anImsConnectTimeout
* the imsConnectTimeout value to be used for this interaction.
* Use the constants defined in ApiProperties
to specify this parameter.
* @throws ImsConnectApiException
* @see ApiProperties#DEFAULT_IMS_CONNECT_TIMEOUT
* @see ApiProperties#TIMEOUT_10_MILLISECONDS
* @see ApiProperties#TIMEOUT_20_MILLISECONDS
* @see ApiProperties#TIMEOUT_50_MILLISECONDS
* @see ApiProperties#TIMEOUT_100_MILLISECONDS
* @see ApiProperties#TIMEOUT_500_MILLISECONDS
* @see ApiProperties#TIMEOUT_1_SECOND
* @see ApiProperties#TIMEOUT_2_SECONDS
* @see ApiProperties#TIMEOUT_5_SECONDS
* @see ApiProperties#TIMEOUT_10_SECONDS
* @see ApiProperties#TIMEOUT_30_SECONDS
* @see ApiProperties#TIMEOUT_45_SECONDS
* @see ApiProperties#TIMEOUT_1_MINUTE
* @see ApiProperties#TIMEOUT_2_MINUTES
* @see ApiProperties#TIMEOUT_5_MINUTES
* @see ApiProperties#TIMEOUT_15_MINUTES
* @see ApiProperties#TIMEOUT_10_MINUTES
* @see ApiProperties#TIMEOUT_30_MINUTES
* @see ApiProperties#TIMEOUT_45_MINUTES
* @see ApiProperties#TIMEOUT_1_HOUR
* @see ApiProperties#IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_DEFAULT_VALUE
* @see ApiProperties#IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_NOWAIT_VALUE
* @see ApiProperties#TIMEOUT_WAIT_FOREVER
* @see TmInteraction#getImsConnectTimeout()
*/
public void setImsConnectTimeout(int anImsConnectTimeout) throws ImsConnectApiException;
/**
* Gets the interaction timeout.
* @return an int
value containing the interactionTimeout property value
* @see TmInteraction#setInteractionTimeout(int)
*/
public int getInteractionTimeout();
/**
*
* Sets the interactionTimeout property value.
* The interactionTimeout property specifies the time that the IMS Connect API will
* wait to receive a response from IMS Connect to be returned to the client. This property
* is specified by the client on any input.
* Note that the interactionTimeout value should always be set to a larger value than
* the imsConnectTimeout value. Otherwise, the interactionTimeout will mask the
* imsConnectTimeout meaning that the interactionTimeout would be triggered in situations
* where the problem should have caused the imsConnectTimeout to be triggered.
* Constants are provided in ApiProperties
for specifying the interactionTimeout value.
* The constant INTERACTION_TIMEOUT_MAX
represents 2,147,483,647 msec or approximately 3.5 weeks.
* If a value for this property is not supplied by the client, the default value
* (INTERACTION_TIMEOUT_WAIT_FOREVER
) will be used.
* @param interactionTimeout
* the interactionTimeout to be used for this interaction.
* Use the constants defined in ApiProperties
to specify this parameter.
* @throws ImsConnectApiException
* @see ApiProperties#DEFAULT_INTERACTION_TIMEOUT
* @see ApiProperties#TIMEOUT_1_MILLISECOND
* @see ApiProperties#TIMEOUT_2_MILLISECONDS
* @see ApiProperties#TIMEOUT_5_MILLISECONDS
* @see ApiProperties#TIMEOUT_10_MILLISECONDS
* @see ApiProperties#TIMEOUT_20_MILLISECONDS
* @see ApiProperties#TIMEOUT_50_MILLISECONDS
* @see ApiProperties#TIMEOUT_100_MILLISECONDS
* @see ApiProperties#TIMEOUT_200_MILLISECONDS
* @see ApiProperties#TIMEOUT_500_MILLISECONDS
* @see ApiProperties#TIMEOUT_1_SECOND
* @see ApiProperties#TIMEOUT_2_SECONDS
* @see ApiProperties#TIMEOUT_5_SECONDS
* @see ApiProperties#TIMEOUT_10_SECONDS
* @see ApiProperties#TIMEOUT_30_SECONDS
* @see ApiProperties#TIMEOUT_45_SECONDS
* @see ApiProperties#TIMEOUT_1_MINUTE
* @see ApiProperties#TIMEOUT_2_MINUTES
* @see ApiProperties#TIMEOUT_5_MINUTES
* @see ApiProperties#TIMEOUT_10_MINUTES
* @see ApiProperties#TIMEOUT_30_MINUTES
* @see ApiProperties#TIMEOUT_45_MINUTES
* @see ApiProperties#TIMEOUT_1_HOUR
* @see ApiProperties#TIMEOUT_2_HOURS
* @see ApiProperties#TIMEOUT_4_HOURS
* @see ApiProperties#TIMEOUT_8_HOURS
* @see ApiProperties#TIMEOUT_12_HOURS
* @see ApiProperties#TIMEOUT_1_DAY
* @see ApiProperties#TIMEOUT_2_DAYS
* @see ApiProperties#TIMEOUT_5_DAYS
* @see ApiProperties#TIMEOUT_1_WEEK
* @see ApiProperties#TIMEOUT_2_WEEKS
* @see ApiProperties#INTERACTION_TIMEOUT_MAX
* @see ApiProperties#TIMEOUT_WAIT_FOREVER
* @see TmInteraction#getInteractionTimeout()
*/
public void setInteractionTimeout(int interactionTimeout) throws ImsConnectApiException;
// Message routing properties
/**
* Gets a boolean value representing whether undeliverable output will be purged.
* @return a boolean
value containing the purgeUndeliverableOutput property value
* @see TmInteraction#setPurgeUndeliverableOutput(boolean)
*/
public boolean isPurgeUndeliverableOutput();
/**
* Sets the purgeUndeliverableOutput property value. The valid values, for which the following constants are defined in ApiProperties
, are:
*
* PURGE_UNDELIVERABLE_OUTPUT
- Boolean value true. Discard undeliverable primary and/or
* secondary commit mode 0 output (maps to IRM_F3_PURGE value of 0X04). Tells IMS Connect and OTMA to discard
* undeliverable primary and/or secondary commit mode 0 output resulting from a transaction.
* DO_NOT_PURGE_UNDELIVERABLE_OUTPUT
- Boolean value false. Requeue undeliverable primary
* and/or secondary commit mode 0 output (no bits set in IRM_F3). Tells IMS Connect and OTMA to requeue
* undeliverable primary and/or secondary commit mode 0 output resulting from a transaction. Causes undelivered
* output indicator to not be returned to IMS Connect from the user message exit.
* DEFAULT_PURGE_UNDELIVERABLE_OUTPUT
- same as DO_NOT_PURGE_UNDELIVERABLE_OUTPUT
*
* If a value for this property is not supplied by the client, the default value (DO_NOT_PURGE_UNDELIVERABLE_OUTPUT
) will be used.
* If you specify true values for both purgeUndeliverableOutput and rerouteUndeliveredOutput at the same time,
* undeliverable primary and/or secondary commit mode 0 output resulting from a transaction is neither purged
* nor rerouted. In this case, OTMA stores the output onto the asynchronous message hold queue of the inputting
* Tpipe (clientID Tpipe) and issues a DFS2407W warning message.
* For more details on the purge undeliverable property, see the topic
* "Purging undeliverable commit-then-send output" in the V13 Communications and Connections guide documentation.
* @param aPurgeUndeliverableOutput the rerouteUndeliverableOutput to set. Use the constants defined in ApiProperties to specify this parameter.
* @throws ImsConnectApiException
* @see ApiProperties#PURGE_UNDELIVERABLE_OUTPUT
* @see ApiProperties#DO_NOT_PURGE_UNDELIVERABLE_OUTPUT
* @see TmInteraction#isPurgeUndeliverableOutput()
*/
public void setPurgeUndeliverableOutput (boolean aPurgeUndeliverableOutput) throws ImsConnectApiException;
/**
* Gets a boolean value representing whether undeliverable output will be rerouted to a
* different asynchronous hold queue.
* @return a boolean
value containing the rerouteUndeliverableOutput
* property value
* @see TmInteraction#setRerouteUndeliverableOutput(boolean)
*/
public boolean isRerouteUndeliverableOutput();
/**
* Sets the rerouteUndeliverableOutput property value.
* The valid values, for which the following constants are defined in ApiProperties
, are:
*
* REROUTE_UNDELIVERABLE_OUTPUT
- Boolean value true. Save undeliverable primary and/or
* secondary commit mode 0 output on the OTMA asynchronous hold queue corresponding to the configured
* reroute name (maps to IRM_F3_REROUT value of 0X08).
* DO_NOT_REROUTE_UNDELIVERABLE_OUTPUT
- Boolean value false. Save undeliverable
* primary and/or secondary commit mode 0 output on the OTMA asynchronous message hold queue of the
* inputting Tpipe (clientID Tpipe) (no bits set in IRM_F3). Causes the reroute undelivered output indicator
* to not be returned to IMS Connect from the user message exit and, if the purgeUndeliverableOutput value is
* also false, causes the undelivered output to be stored on the OTMA asynchronous message hold queue of the
* inputting Tpipe (clientID Tpipe).
* DEFAULT_REROUTE_UNDELIVERABLE_OUTPUT
- same as DO_NOT_REROUTE_UNDELIVERABLE_OUTPUT
*
* If a value for this property is not supplied by the client, the default value (DO_NOT_REROUTE_UNDELIVERABLE_OUTPUT
) will be used.
* If the rerouteUndeliverableOutput property is set to true, providing a value for the rerouteName property is
* optional. The reroute function applies only to Send-Recv, Send-Only and Send-Only-with-Acknowledgement
* interactions. If you specify both purgeUndeliverableOutput and rerouteUndeliverableOutput at the same time,
* undeliverable primary and/or secondary commit mode 0 output resulting from a transaction is neither purged
* nor rerouted. In this case, OTMA stores the output onto the asynchronous message hold queue of the inputting
* Tpipe (clientID Tpipe) and issues a DFS2407W warning message.
* For more details on rerouting output, see the topic
* "Rerouting commit-then-send output" in the V11 Communications and Connections guide documentation.
* @param aRerouteUndeliverableOutput the rerouteUndeliverableOutput to set.
* Use the constants defined in ApiProperties to specify this parameter.
* @throws ImsConnectApiException
* @see ApiProperties#REROUTE_UNDELIVERABLE_OUTPUT
* @see ApiProperties#DO_NOT_REROUTE_UNDELIVERABLE_OUTPUT
* @see TmInteraction#isRerouteUndeliverableOutput()
*/
public void setRerouteUndeliverableOutput(boolean aRerouteUndeliverableOutput) throws ImsConnectApiException;
/**
* Gets the reroute name property value.
* @return a String
value containing the rerouteName property
* @see TmInteraction#setRerouteName(String)
*/
public String getRerouteName();
/**
* Sets the rerouteName property value which specifies the optional name of a reroute destination
* (an OTMA Tpipe asynchronous hold queue). The rerouteName property is a string of 1 to 8 uppercase
* alphanumeric (A through Z, 0 to 9) or special (@, #, $) characters.
* The value of the rerouteName property is validated in IMS Connect by converting all invalid characters
* to blanks and then truncating the resulting rerouteName value at the leftmost blank character. As a result,
* OTMA will always be passed a valid rerouteName to use (though not the intended rerouteName if the rerouteName
* value specified is prefixed with or contains imbedded invalid characters or blanks).
*
A value of all blanks in the resulting rerouteName value will cause the default reroute name defined in
* IMS Connect, which is always validated during IMS Connect initialization, to be used as the reroute name
* by IMS Connect. If the validation results in a non-blank rerouteName value, that value will be used as the
* destination Tpipe name for all undeliverable Commit Mode 0 output in a SENDRECV, SENDONLY or SENDONLYACK
* function interaction. Unless the IMS Connect user message exit or another user exit has been modified to
* do otherwise, the rerouteName value is copied to the OMUSR_REROUT_NM field in the OTMA header by the IMS
* Connect user message exit.
*
The rerouteName and resumeTpipe_AlternateClientId values are mapped to the same offset in the IRM as
* well as the OTMA header. As a result, they are mutually exclusive; that is, both cannot be used in the same request
* message. The IMS Connect API will determine which value to use based on the function specified in the request.
* If the function specified is a RESUMETPIPE, the rerouteName value will be ignored and the
* resumeTpipeAlternateClientId value will be used by the API. Otherwise, the resumeTpipeAlternateClientId
* value will be ignored and the rerouteName value will be used by the API.
*
If the reroute function is to be used successfully, the following steps must be completed:
*
* - The architectureLevel property must be set to 0X01 (Reroute support included) or higher. This is done
* internally by the API since the API always sets the architectureLevel property value to 0x02.
*
- The purgeUndeliveredOutput property must be set to false and the rerouteUndeliveredOutput
* property must be set to true.
*
- A valid value (or blanks if you have configured your application to use IMS Connect reroute name value)
* must be provided for the rerouteName property.
*
* Note that DEFAULT_REROUTE_NAME has the
String
value of eight blank characters and the rerouteName property has to
* be set during runtime to the name of the reroute queue on which you want OTMA to place undeliverable
* response messages.
* For more details on rerouting output, see the topic
* "Rerouting commit-then-send output" in the V11 Communications and Connections guide documentation.
* @param rerouteName
* the rerouteName to be used for this interaction.
* @throws ImsConnectApiException
* @see ApiProperties#REROUTE_NAME_IMS_CONNECT_DEAD_LETTER_QUEUE
* @see ApiProperties#REROUTE_NAME_NONE
* @see TmInteraction#getRerouteName()
*/
public void setRerouteName(String rerouteName) throws ImsConnectApiException;
/**
* Gets the name of an alternate clientId (whose value is the name of an asynchronous
* hold queue) from which output will be retrieved for a resumeTpipe interaction.
* @return a String
value containing the resumeTpipeAlternateClientId
* property value
* @see TmInteraction#setResumeTpipeAlternateClientId(String)
*/
public String getResumeTpipeAlternateClientId();
/**
* Sets the resumeTpipeAlternateClientId property value which specifies the optional name of a reroute
* destination (an OTMA Tpipe asynchronous hold queue). The resumeTpipeAlternateClientId property is a
* string of 1 to 8 uppercase alphanumeric (A through Z, 0 to 9) or special (@, #, $) characters.
*
The value of the resumeTpipeAlternateClientId property is validated in IMS Connect by converting
* all invalid characters to blanks and then truncating the resulting resumeTpipeAlternateClientId
* value at the leftmost blank character. As a result, OTMA will always be passed a valid alternate client
* ID to use (though not the intended rerouteName if the rtAltClientID value specified is prefixed with
* or contains imbedded invalid characters or blanks).
*
A value of all blanks in the resulting resumeTpipeAlternateClientId value will cause the
* RESUMETPIPE interaction to be processed as a normal RESUMETPIPE function in which the output is
* retrieved from the Tpipe associated with the clientID of the connection on which the RESUMETPIPE
* request is received. If the validation results in a non-blank resumeTpipeAlternateClientId value,
* that value will be used as the as name of the Tpipe from which one or more asynchronous output messages
* are retrieved for RESUMETPIPE requests. Unless the IMS Connect user message exit or another user exit has
* been modified to do otherwise, the validated resumeTpipeAlternateClientId value is copied to the
* OMUSR_REROUT_NM field in the OTMA header by the IMS Connect user message exit.
* @param aResumeTpipeAlternateClientId
* the alternate client ID to set.
* @throws ImsConnectApiException
* @see TmInteraction#getResumeTpipeAlternateClientId()
*/
public void setResumeTpipeAlternateClientId(String aResumeTpipeAlternateClientId) throws ImsConnectApiException;
/**
* Gets an int
value representing the type of resumeTpipe processing
* (single-no wait, single-wait, auto, or no-auto) to be performed for a resumeTpipe
* interaction.
* @return an int
value containing the resumeTpipeProcessing property value
* @see TmInteraction#setResumeTpipeProcessing(int)
*/
public int getResumeTpipeProcessing();
/**
*
* Sets the resumeTpipeProcessing property value which specifies the type of processing to be used for
* a resumeTpipe request. All of the SINGLE and AUTO values are mutually exclusive. The appropriate value
* must be specified by the client on input and will be maintained in the message until the interaction
* has completed. All RESUMETIPE interactions are used to retrieve output response messages from an OTMA
* asynchronous message hold queue. The hold queue from which messages are retrieved is one of the following:
*
*
* - If a resumeTpipeAlternateClientID value has not been specified for this interaction, the hold queue is
* the Tpipe with the name of the clientID value for the current RESUMETPIPE interaction.
* - If a resumeTpipeAlternateClientID value has been specified, the hold queue is the Tpipe with the name
* matches the resumeTpipeAlternateClientID value for the current RESUMETPIPE interaction.
*
* Only one value can be specified per interaction.
* The valid values, for which the following constants are defined in {@link ApiProperties}, are:
*
* RESUMETPIPE_SINGLE_NOWAIT
- Retrieves at most one response message per RESUMETPIPE_SINGLE_NOWAIT request.
* If no message is present when the current RESUMETPIPE_SINGLE_NOWAIT interaction is received by OTMA from IMS
* Connect, IMS Connect will return a timeout error to the client as soon as the IMS Connect response timer
* (set according to the imsConnectResponseTimeout value in use for that interaction) has expired.
* If there was no response message already available when OTMA received the RESUMETPIPE_SINGLE_NOWAIT request,
* any output received by OTMA after time (but before a subsequent RESUMETPIPE request has been received by OTMA)
* will be moved immediately to its asynchronous message hold queue by OTMA. Set the imsConnectResponseTimeout
* to a smaller value to reduce the amount of time wasted waiting for the IMS Connect response timer to expire
* when there is no message available to be retrieved at the time the RESUMETPIPE request is processed by OTMA.
*
* RESUMETPIPE_AUTO
- Retrieves all available messages, one at a time. It is recommended that
* the RESUMETPIPE_AUTO option be used only if the client is a dedicated output client and the ackNakProvider property is set to CLIENT_ACK_NAK. Set the imsConnectResponseTimeout to a larger value
* to increase the probability of retrieving all expected response messages in a single RESUMETIPE interaction.
* Each ACK sent by the client resets the IMS Connect response timer controlled by the
* imsConnectResponseTimeout value. The IMS Connect response timer value used by the RESUMETPIPE_AUTO
* interaction applies only to the first receive state. OTMA will continue to return output messages that
* it gets from IMS after the time the RESUMETPIPE_AUTO request is received by OTMA until both of the
* following conditions are true:
* - no more output is available, and
*
- the IMS Connect response timer has expired in IMS Connect
*
* RESUMETPIPE_NOAUTO
- Retrieves all messages available at the time the RESUMETPIPE_NOAUTO
* request is received by OTMA, one at a time. It is recommended that the RESUMETPIPE_NOAUTO option be used only if the client is a dedicated
* output client and the ackNakProvider property is set to CLIENT_ACK_NAK. Set the imsConnectResponseTimeout to a smaller value in order to reduce the amount of wait
* time after the last message has been returned. Each ACK sent by the client resets the
* imsConnectResponseTimeout value. The IMS Connect response timer value used by the RESUMETPIPE_NOAUTO
* interaction applies only to the first receive state. A RESUMETPIPE_NOAUTO interaction differs from a
* RESUMETPIPE_AUTO interaction in that, for _NOAUTO, OTMA will only return output messages that are already
* available on the hold queue at the time the RESUMETPIPE_NOAUTO request is received by OTMA. Any output
* received after the request is received by OTMA will not be returned to the client until a subsequent
* RESUMETPIPE call is made.
*
* RESUMETPIPE_SINGLE_WAIT
- Retrieves at most one response message per
* RESUMETPIPE_SINGLE_WAIT request. If no message is present when the current RESUMETPIPE_SINGLE_WAIT
* interaction is received by IMS Connect, IMS Connect will continue to wait for a response message to be
* returned by OTMA or for the IMS Connect response timer (set according to the imsConnectResponseTimeout
* value for this interaction) to time out. If IMS Connect receives a response message before the IMS Connect
* response timer times out, it will return that response to the client and wait for an ACK or NAK from the
* client. If the IMS Connect response timer times out before IMS Connect receives a response message, IMS
* Connect will return a timeout error to the client. Any output received by IMS Connect from OTMA after the
* IMS Connect response timer has expired but before a subsequent RESUMETPIPE request has been received by IMS
* Connect for the target Tpipe will be considered a late response message and will be NAK'ed by IMS Connect
* and returned to its asynchronous hold queue by OTMA. Set the imsConnectResponseTimeout to a larger value to
* increase the probability of retrieving all expected response messages in a single RESUMETPIPE interaction.
*
* DEFAULT_RESUME_TPIPE_PROCESSING
- same as RESUMETPIPE_SINGLE_WAIT
*
* If a value for this property is not supplied by the client, the default value (RESUMETPIPE_SINGLE_WAIT
) will be used.
* @param resumeTpipeProcessing
* the resumeTpipeProcessing to set. Use the constants defined in ApiProperties to specify this parameter.
* @throws ImsConnectApiException
* @see ApiProperties#RESUME_TPIPE_SINGLE_NOWAIT
* @see ApiProperties#RESUME_TPIPE_NOAUTO
* @see ApiProperties#RESUME_TPIPE_SINGLE_WAIT
* @see ApiProperties#RESUME_TPIPE_AUTO
* @see TmInteraction#getResumeTpipeProcessing()
*/
public void setResumeTpipeProcessing(int resumeTpipeProcessing) throws ImsConnectApiException;
// Security properties
/**
* Gets the RACF APPL name.
* @return a String
value containing the RACF APPL name property value
* @see TmInteraction#setRacfApplName(String)
*/
public String getRacfApplName();
/**
* Sets the racfApplName property value. The racfApplName property is a string of 1 to 8 uppercase
* alphanumeric (A through Z, 0 to 9) or special (@, #, $) characters and is intended to match a RACF
* APPL name defined to RACF in a PTKTDATA definition. If the racfApplName value specified is fewer than 8
* characters, the racfApplName value is right-padded with blanks to 8 characters by the IMS Connect API
* before being copied to the IRM_APPL_NM field. If the racfApplName value is 8 0X00s or 8 blanks after
* padding with blanks, the IMS Connect user message exit will use the default value from the IMS Connect
* configuration member. If there is no value specified in the IMS Connect configuration, 8 blanks will be
* copied to the IRM_APPL_NM field. If the racfApplName value is not 8 0X00s or 8 blanks after padding, it
* is passed back to IMS Connect by the IMS Connect user message exit without first checking the validity.
* IMS Connect will then use this racfApplName value in the RACROUTE VERIFY call where it is validated
* before it is used.
*
Note that the DEFAULT_RACF_APPL_NAME is a String of eight blank characters, and the racfApplName
* property must be changed during runtime to the appropriate RACF or SAF APPLID used by the target IMS
* Connect or IMS if the client wishes to submit a request containing a passticket rather than a password.
* @param aRacfApplName
* the RACF application name to be used for this interaction
* @throws ImsConnectApiException
* @see TmInteraction#getRacfApplName()
*/
public void setRacfApplName(String aRacfApplName) throws ImsConnectApiException;
/**
* Gets the RACF Group name.
* @return a String
value containing the RACF group name property value
* @see TmInteraction#setRacfGroupName(String)
*/
public String getRacfGroupName();
/**
* Sets the racfGroupName property value. The racfGroupName property specifies the RACF group name which is a
* string of 1 to 8 uppercase alphanumeric (A through Z, 0 to 9) or special (@, #, $) characters. Providing a
* value for the racfGroupName is optional. If the racfGroupName value specified is fewer than 8 characters,
* it will be right-padded with blanks by the IMS Connect API. If RACF is on, IMS Connect will validate the
* racfGroupName value by checking for invalid characters. IMS Connect will move all valid characters of
* racfGroupName starting with the leftmost character up to but NOT including the first invalid character, into
* a buffer which has been initialized to all blanks. If RACF is off, IMS Connect does not perform any
* validation and the 8-character, unvalidated racfGroupName value is used directly by the IMS Connect user
* message exit unless the IMS Connect user message exit has been modified to do otherwise. The RACF group
* name value is used by IMS Connect during racroute verify calls for user authentication and by IMS OTMA for
* user authorization. RACF will accept either the correct group name for the specified RACF user ID or, if
* the group name is to be ignored in racroute verify calls, 0 to 8 blanks for this value.
*
Note that the DEFAULT_RACF_GROUP_NAME has the String value "RACFGRUP", and the racfGroupName property
* must be changed during runtime to the name of appropriate RACF or SAF group under which the interaction
* will be executed in IMS Connect.
* @param aRacfGroupName
* the RACF group name to be used for this interaction.
* @throws ImsConnectApiException
* @see TmInteraction#getRacfGroupName()
*/
public void setRacfGroupName(String aRacfGroupName) throws ImsConnectApiException;
/**
* Gets the RACF password.
* @return a String
value containing the RACF password property value
* @see TmInteraction#setRacfPassword(String)
*/
public String getRacfPassword();
/**
* Sets the racfPassword property value. The racfPassword property specifies the RACF passticket
* or password which is a string of 1 to 8 uppercase alphanumeric (A through Z, 0 to 9) or special (@, #, $,.,<,+,|,&,!,*,-,%,_,>,?,:,=)
* characters. If the racfPassword value specified is fewer than 8 characters, it will be right-padded with
* blanks by the IMS Connect API. For non-trusted users, the client or one of the IMS Connect user exits
* must provide a valid value for the racfPassword property if IMS Connect is configured to check SAF
* authentication of clients. For trusted users or if IMS Connect is configured to NOT check SAF
* authentication of clients, the client authentication is bypassed and therefore, the racfPassword
* value is ignored by IMS Connect. The racfPassword value is not used by OTMA or IMS even if OTMA is
* configured to check SAF authorization of clients. If IMS Connect is configured to check SAF
* authentication of clients (i.e., RACF is on or yes in IMS Connect), IMS Connect checks for invalid
* characters in the racfPassword value. It copies all valid characters in racfPassword that it encounters
* in sequence, starting with the leftmost character and ending at 8 characters or before the first
* invalid character, into a buffer which has been initialized to all blanks. If the leftmost character
* is invalid, the buffer will contain 8 blanks. This buffer containing the validated racfPassword value
* is used by the IMS Connect user message exit and also passed back to IMS Connect regardless of whether
* or not it is used by IMS Connect, OTMA or IMS. If RACF is on and the racfPassword value is all blanks
* or resolves to all blanks, IMS Connect will return an error. If RACF is off, IMS Connect does not
* perform any validation.
*
Note that the DEFAULT_RACF_PASSWORD has the String value "RACFPSWD", and the racfPassword property
* must be changed during runtime to the RACF or SAF password of the RACF or SAF userID under which the
* interaction will be executed in IMS Connect.
* @param aRacfPassword
* the RACF password to be used for this interaction.
* @throws ImsConnectApiException
* @see TmInteraction#getRacfPassword()
*/
public void setRacfPassword(String aRacfPassword) throws ImsConnectApiException;
/**
* Gets the RACF userID.
* @return a String
value containing the RACF userID property value
* @see TmInteraction#setRacfUserId(String)
*/
public String getRacfUserId();
/**
* Sets the racfUserId property value. The racfUserId property specifies the RACF user ID which is a
* string of 1 to 8 uppercase alphanumeric (A through Z, 0 to 9) or special (@, #, $) characters. The
* client or one of the IMS Connect or IMS user exits must provide a valid value for the racfUserId
* property if IMS Connect is configured to check SAF authentication of clients or OTMA is configured
* to check SAF authorization of clients. If the racfUserId value specified is fewer than 8 characters,
* it will be right-padded with blanks by the IMS Connect API. If RACF is on, IMS Connect checks for
* invalid characters in the racfUserId value and moves all valid characters of racfUserId starting
* with the leftmost character up to but NOT including the first invalid character, into a buffer
* which has been pre-initialized to all blanks. If the leftmost character is invalid, IMS Connect
* will use 8 blank characters as the racfUserId value. If RACF is on and the racfUserId value is all
* blanks or resolves to all blanks, IMS Connect will return an error. If RACF is off, IMS Connect
* does not perform any validation.
*
Note that the DEFAULT_RACF_USERID has the String value "RACFUID", and the racfUserId property must
* be changed during runtime to the name of appropriate RACF or SAF userID under which the interaction
* will be executed in IMS Connect.
* @param aRacfUserId
* the RACF userId to be used for this interaction.
* @throws ImsConnectApiException
* @see TmInteraction#getRacfUserId()
*/
public void setRacfUserId(String aRacfUserId) throws ImsConnectApiException;
/**
* Sets the calloutRequestNakProcessing property value. Set this property to send a negative
* acknowledgement (NAK) in response to a synchronous callout message from
* a RESUME TPIPE request. A NAK message frees the tpipe queue and tells OTMA
* what to do with the synchronous callout request message and whether to maintain or end the
* current RESUME TPIPE call.
*
*
The IMS Connect client can specify one of the following options for a NAK response:
*
* - NAK_DISCARD_SYNC_CALLOUT_REQUEST_END_RESUMETPIPE: Discard the rejected synchronous callout request
* message and terminate the RESUME TPIPE connection. This is the default option if the client application does
* not use this method to set a value for the property.
* - NAK_DISCARD_SYNC_CALLOUT_REQUEST_CONTINUE_RESUMETPIPE: Discard the rejected synchronous callout
* request message, but maintain the RESUME TPIPE connection to continue retrieving other synchronous
* callout request messages.
* - NAK_REQUEUE_SYNC_CALLOUT_REQUEST_END_RESUMETPIPE: Keep the rejected synchronous callout request message
* on the tpipe queue, but terminate the RESUME TPIPE connection.
*
* @param aCalloutNakProcessing
the calloutNakProcessing value needed by the client application
* @throws ImsConnectApiException
* @see TmInteraction#getCalloutRequestNakProcessing()
* @see ApiProperties#NAK_DISCARD_SYNC_CALLOUT_REQUEST_CONTINUE_RESUMETPIPE
* @see ApiProperties#NAK_DISCARD_SYNC_CALLOUT_REQUEST_CONTINUE_RESUMETPIPE_VALUE
* @see ApiProperties#NAK_DISCARD_SYNC_CALLOUT_REQUEST_END_RESUMETPIPE
* @see ApiProperties#NAK_DISCARD_SYNC_CALLOUT_REQUEST_END_RESUMETPIPE_VALUE
* @see ApiProperties#NAK_REQUEUE_SYNC_CALLOUT_REQUEST_END_RESUMETPIPE
* @see ApiProperties#NAK_REQUEUE_SYNC_CALLOUT_REQUEST_END_RESUMETPIPE_VALUE
* @since Enterprise Suite 2.1
*/
public void setCalloutRequestNakProcessing(String aCalloutNakProcessing) throws ImsConnectApiException;
/**
* Gets the calloutRequestNakProcessing type.
* @return a String
value containing the calloutRequestNakProcessing property value
* @see TmInteraction#setCalloutRequestNakProcessing(String)
* @since Enterprise Suite 2.1
*/
public String getCalloutRequestNakProcessing();
/**
* Sets the calloutResponseMessageType property value. A client application returns
* callout response messages using the send-only protocol. The send-only message contains
* no transaction code and can contain either the response data or error information for
* the IMS application program. To distingush between an error and a response data message
* the API uses the calloutResponseMessageType property. Use this method to set
* the property before sending the callout response message. The valid values are:
*
* - CALLOUT_ERROR_MESSAGE: The response message contains an error code.
*
- CALLOUT_RESPONSE_MESSAGE: The response message contains the expected response data.
*
* The DEFAULT_CALLOUT_RESPONSE_MESSAGE_TYPE is CALLOUT_RESPONSE_MESSAGE.
* @param aCalloutResponseMsgType
* the CalloutResponseMsgType value needed by the client application
* @throws ImsConnectApiException
* @see TmInteraction#getCalloutResponseMessageType()
* @see ApiProperties#CALLOUT_ERROR_MESSAGE
* @see ApiProperties#CALLOUT_RESPONSE_MESSAGE
* @since Enterprise Suite 2.1
*/
public void setCalloutResponseMessageType(String aCalloutResponseMsgType) throws ImsConnectApiException;
/**
* Gets the calloutResponseMessageType property value.
* @return a String
value containing the calloutResponseMessageType property value.
* @see TmInteraction#setCalloutResponseMessageType(String)
* @since Enterprise Suite 2.1
*/
public String getCalloutResponseMessageType();
/**
* Sets the resumeTpipeRetrievalType property value. Synchronous callout request messages are retrieved
* by issuing a RESUME TPIPE call. You can code the RESUME TPIPE call to retrieve only synchronous callout
* messages, only asynchronous callout messages, or both types of messages.
*
The valid values for the resumeTpipeRetrievalType property are:
*
* - RETRIEVE_SYNC_CALLOUT_ONLY
*
- RETRIEVE_SYNC_CALLOUT_OR_ASYNC_OUTPUT
*
- RETRIEVE_ASYNC_OUTPUT (This is equivalent to the DEFAULT_RESUME_TPIPE_RETRIEVAL_TYPE property value.)
*
* @throws ImsConnectApiException
* @see TmInteraction#getCalloutResponseMessageType()
* @see ApiProperties#RETRIEVE_SYNC_MESSAGE_ONLY
* @see ApiProperties#RETRIEVE_SYNC_OR_ASYNC_MESSAGE
* @see ApiProperties#RETRIEVE_ASYNC_MESSAGE_ONLY
* @since Enterprise Suite 2.1
*/
public void setResumeTpipeRetrievalType(byte aRetrievalType) throws ImsConnectApiException;
/**
* Gets the resumeTpipeRetrievalType property value.
* @return
* a byte
value containing the ResumeTpipeRetrievalType property value
* @see TmInteraction#setResumeTpipeRetrievalType(byte)
* @since Enterprise Suite 2.1
*/
public byte getResumeTpipeRetrievalType();
/**
* Sets the nakReasoncode property value. If an error occurs after the client application has
* already returned an ACK message to IMS Connect after retrieving a synchronous callout request message,
* the client application can return an error response to IMS instead of the expected
* data. The nakReasonCode property allows the client application to include an error
* reason code with the error response message. This reason code value is not interpreted by
* IMS and is passed directly to your IMS application.
* The IRM_NAK_REASONCODE_DEFAULT property value is 0X00.
*
Values between 0X7D1 and 0XBB8 are valid for the reason code.
* @param nakReasonCode
* the nakReasonCode value needed by the client application
* @see TmInteraction#getNakReasonCode()
* @since Enterprise Suite 2.1
*/
public void setNakReasonCode(short nakReasonCode);
/**
* Gets the nakReasonCode value associated with this TmInteraction object.
* @return a byte
byte value of the nakReasonCode property.
* @see TmInteraction#setNakReasonCode(short)
* @since Enterprise Suite 2.1
*/
public short getNakReasonCode();
/**
* Gets the correlatorToken value for this TmInteraction object.
* @return the byte[]
value of the correlatorToken property
* @see TmInteraction#setCorrelatorToken(byte[])
* @since Enterprise Suite 2.1
*/
public byte[] getCorrelatorToken();
/**
* Sets the correlatorToken property value. Inbound and outbound synchronous callout messages contain a segment
* for the correlator token. The correlator token is used to match a response message with the waiting
* IMS application program that issued the callout requests. Client
* application programs must store the token when reading callout request messages
* and return it with callout response messages. The API internally sets this property value using the
* setter method when a request is received. Your client application must set the property value to the value of
* the received token before sending the response message.
*
The CORRELATOR_TOKEN_DEFAULT property value is an empty byte array.
* @param aCorrelatorToken
* @see TmInteraction#getCorrelatorToken()
* @throws ImsConnectApiException
* @since Enterprise Suite 2.1
*/
public void setCorrelatorToken(byte[] aCorrelatorToken) throws ImsConnectApiException;
/**
*
Provides access to several inner class methods that retrieve particular fields from the correlator token:
*
* public short getCorrelatorTokenDetails().getCorrelatorLength()
- Gets the length of the IRM.
* public String getCorrelatorTokenDetails().getCorrelatorICALUserID()
- Gets the 8-character user ID under which the ICAL call was executed.
* public String getCorrelatorTokenDetails().getCorrelatorIMSID()
- Gets the 4-character IMS ID.
* public String getCorrelatorTokenDetails().getCorrelatorOTMATMember()
- Gets the 8-character OTMA tmember name.
* public String getCorrelatorTokenDetails().getCorrelatorOTMATPipeName()
- Gets the 8-character OTMA tpipe name.
* public String getCorrelatorTokenDetails().getCorrelatorOTMAMessageToken()
- Gets the 8-character OTMA Message Token.
*
* @since Enterprise Suite 2.2
*/
public CorrelatorToken getCorrelatorTokenDetails();
}