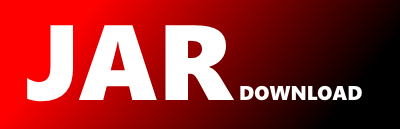
com.ibm.ims.connect.impl.CmdrsphdrImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of IMSConnectAPI Show documentation
Show all versions of IMSConnectAPI Show documentation
API that allows Java applications to interface with IMS Connect
The newest version!
/**
* File: CmdrsphdrImpl.java
* ==========================================================================
* Licensed Material - Property of IBM
*
* IBM Confidential
*
* OCO Source Materials
*
* 5655-TDA
*
* (C) Copyright IBM Corp. 2011, 2013 All Rights Reserved.
*
* The source code for this program is not published or
* otherwise divested of its trade secrets, irrespective
* of what has been deposited with the U.S. Copyright
* Office.
*
* US Government Users Restricted Rights - Use, duplication or
* disclosure restricted by GSA ADP Schedule Contract with
* IBM Corp.
* ===========================================================================
*/
package com.ibm.ims.connect.impl;
import com.ibm.ims.connect.Cmdrsphdr;
import com.ibm.ims.connect.Hdr;
import java.util.ArrayList;
import java.util.Vector;
/**
* @author kevin
*
*/
public class CmdrsphdrImpl implements Cmdrsphdr
{
private String elementText = null;
private ArrayList hdrArray = new ArrayList();
/**
* @param anElementText the elementText to use for this cmdrsphdr
*/
public CmdrsphdrImpl(String anElementText)
{
this.setElementText(anElementText);
}
/**
* @return the cmdrsphdrElementText
*/
public String getElementText()
{
return this.elementText;
}
/**
* @param anElementText the elementText to set
*/
public void setElementText(String anElementText)
{
this.elementText = anElementText;
}
/**
* @return the hdrArray
*/
public ArrayList getHdrArray()
{
return this.hdrArray;
}
/**
* @param aHdrArray the hdrArray to set
*/
public void setHdrArray(ArrayList aHdrArray)
{
this.hdrArray = aHdrArray;
}
/**
* @return the hdr element at anIndex
*/
public Hdr getHdrArrayElement(int anIndex)
{
return this.hdrArray.get(anIndex);
}
/**
* @param aHdrArrayElement the hdr to set
*/
public void setHdrArrayElement(Hdr aHdrArrayElement, int anIndex)
{
this.hdrArray.set(anIndex, aHdrArrayElement);
}
/**
* @param aHdrArrayElement the hdrArray element to append to this hdrArray
*/
protected void addHdrArrayElement(Hdr aHdrArrayElement)
{
this.hdrArray.add(aHdrArrayElement);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy