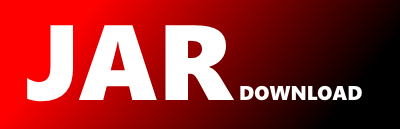
com.ibm.ims.connect.impl.ImsoutImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of IMSConnectAPI Show documentation
Show all versions of IMSConnectAPI Show documentation
API that allows Java applications to interface with IMS Connect
The newest version!
/**
* File: ImsoutImpl.java
* ==========================================================================
* Licensed Material - Property of IBM
*
* IBM Confidential
*
* OCO Source Materials
*
* 5655-TDA
*
* (C) Copyright IBM Corp. 2011, 2013 All Rights Reserved.
*
* The source code for this program is not published or
* otherwise divested of its trade secrets, irrespective
* of what has been deposited with the U.S. Copyright
* Office.
*
* US Government Users Restricted Rights - Use, duplication or
* disclosure restricted by GSA ADP Schedule Contract with
* IBM Corp.
* ===========================================================================
*/
package com.ibm.ims.connect.impl;
import com.ibm.ims.connect.Cmd;
import com.ibm.ims.connect.Cmdclients;
import com.ibm.ims.connect.Cmderr;
import com.ibm.ims.connect.Cmdrspdata;
import com.ibm.ims.connect.Cmdrsphdr;
import com.ibm.ims.connect.Cmdsecerr;
import com.ibm.ims.connect.Ctl;
import com.ibm.ims.connect.Imsout;
import com.ibm.ims.connect.Msgdata;
/**
* @author kevin
*
*/
public class ImsoutImpl implements Imsout
{
private String elementText = null;
private Ctl ctl = null;
private Cmdclients cmdclients = null;
private String cmdsyntax = null;
private String cmddtd = null;
private String cmdtext = null;
private Cmderr cmderr = null;
private Cmdsecerr cmdsecerr = null;
private Cmd cmd = new CmdImpl("");
private Cmdrsphdr cmdrsphdr = null;
private Cmdrspdata cmdrspdata = null;
private Msgdata msgdata = null;
private Object[] imsoutObject =
{
ctl,
cmdclients,
cmdsyntax,
cmddtd,
cmdtext,
cmderr,
cmdsecerr,
cmd,
cmdrsphdr,
cmdrspdata,
msgdata
};
/* response message structure
imsout
ctl
omname?
omvsn?
xmlvsn?
statime
stotime
staseq
stoseq
rqsttkn1?
rqsttkn2?
rc
rsn
rsnmsg?
rsntxt?
uom?
cmdclients?
mbr+
typ
styp
(vsn, jobname) | (rc, rsn, rsntxt)) | msg
vsn?
jobname?
rc?
sn?
rsntxt?
msg?
cmdsyntax?
cmddtd?
cmdtext?
cmderr?
mbr*
(typ, styp, ((vsn, jobname) | (rc, rsn, rsntxt))) | msg
typ?
styp?
vsn?
jobname?
rc?
rsn?
rsntxt?
msg?
cmdsecerr?
exit
rc
userdata
saf
rc
racfrc
racfrsn
cmd
master?
userid?
verb
kwd
input
cmdrsphdr (we used to ignore in API processing since the response is not formatted for display in the API - added for internal user requirement)
hdr* (hdr element is saved as a string which must be parsed by the getter methods)
slbl
llbl
scope
sort
key
scroll
len
dtype
skipb?
align
cmdrspdata (used for IMS and IMS Connect Type2 commands - submitted through OM)
rsp*
msgdata? (used for IMS Type1 commands submitted as Type2 commands through OM)
mbr (only one instance for any type 1 command???)
typ
styp
(vsn, jobname) | (rc, rsn, rsntxt)) | msg
vsn?
jobname?
rc?
sn?
rsntxt?
msg?
Key:
* = 0 or more occurrences
+ = 1 or more occurrences
? = 0 or 1 occurrences
*/
/**
* @return the elementText
*/
public String getElementText()
{
return this.elementText;
}
/**
* @param anElementText the elementText to set
*/
public void setElementText(String anElementText)
{
this.elementText = anElementText;
}
/**
* @return the ctl
*/
public Ctl getCtl()
{
return this.ctl;
}
/**
* @param ctl the ctl to set
*/
public void setCtl(Ctl ctl)
{
this.ctl = ctl;
}
/**
* @return the cmdclients
*/
public Cmdclients getCmdclients()
{
return this.cmdclients;
}
/**
* @param cmdclients the cmdclients to set
*/
public void setCmdclients(Cmdclients cmdclients)
{
this.cmdclients = cmdclients;
}
/**
* @return the cmdsyntax
*/
public String getCmdsyntax()
{
return this.cmdsyntax;
}
/**
* @param cmdsyntax the cmdsyntax to set
*/
public void setCmdsyntax(String cmdsyntax)
{
this.cmdsyntax = cmdsyntax;
}
/**
* @return the cmddtd
*/
public String getCmddtd()
{
return this.cmddtd;
}
/**
* @param cmddtd the cmddtd to set
*/
public void setCmddtd(String cmddtd)
{
this.cmddtd = cmddtd;
}
/**
* @return the cmdtext
*/
public String getCmdtext()
{
return this.cmdtext;
}
/**
* @param cmdtext the cmdtext to set
*/
public void setCmdtext(String cmdtext)
{
this.cmdtext = cmdtext;
}
/**
* @return the cmderr
*/
public Cmderr getCmderr()
{
return this.cmderr;
}
/**
* @param cmderr the cmderr to set
*/
public void setCmderr(Cmderr cmderr)
{
this.cmderr = cmderr;
}
/**
* @return the cmdsecerr
*/
public Cmdsecerr getCmdsecerr()
{
return this.cmdsecerr;
}
/**
* @param cmdsecerr the cmdsecerr to set
*/
public void setCmdsecerr(Cmdsecerr cmdsecerr)
{
this.cmdsecerr = cmdsecerr;
}
/**
* @return the cmd
*/
public Cmd getCmd()
{
return this.cmd;
}
/**
* add a property key and value to the cmd element
*/
public void addCmdProperty(String aKey, String aValue)
{
// this.getCmd().put(aKey, aValue);
}
/**
* @param cmd the cmd to set
*/
public void setCmd(Cmd cmd)
{
this.cmd = cmd;
}
/**
* @return the cmdrsphdr
*/
public Cmdrsphdr getCmdrsphdr()
{
return this.cmdrsphdr;
}
/**
* @param cmdrsphdr the cmdrsphdr to set
*/
public void setCmdrsphdr(Cmdrsphdr cmdrsphdr)
{
this.cmdrsphdr = cmdrsphdr;
}
/**
* @return the cmdrspdata
*/
public Cmdrspdata getCmdrspdata()
{
return this.cmdrspdata;
}
/**
* @param cmdrspdata the cmdrspdata to set
*/
public void setCmdrspdata(Cmdrspdata cmdrspdata)
{
this.cmdrspdata = cmdrspdata;
}
/**
* @return the msgdata
*/
public Msgdata getMsgdata()
{
return this.msgdata;
}
/**
* @param msgdata the msgdata to set
*/
public void setMsgdata(Msgdata msgdata)
{
this.msgdata = msgdata;
}
/**
* @param mbr the mbr to set
*/
public boolean isMbrComplete()
{
// if(!this.mbr.equals("") && !this.typ.equals("") && !this.styp.equals("") &&
// ((!this.vsn.equals("") && !this.jobname.equals("")) |
// (!this.rc.equals("") && !this.rsn.equals(""))) || !this.msg.equals("") )
// return true;
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy