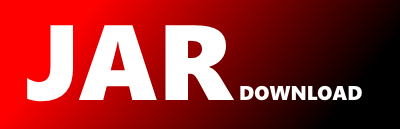
com.ibm.ims.connect.impl.MbrImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of IMSConnectAPI Show documentation
Show all versions of IMSConnectAPI Show documentation
API that allows Java applications to interface with IMS Connect
The newest version!
/**
* File: MbrImpl.java
* ==========================================================================
* Licensed Material - Property of IBM
*
* IBM Confidential
*
* OCO Source Materials
*
* 5655-TDA
*
* (C) Copyright IBM Corp. 2011, 2013 All Rights Reserved.
*
* The source code for this program is not published or
* otherwise divested of its trade secrets, irrespective
* of what has been deposited with the U.S. Copyright
* Office.
*
* US Government Users Restricted Rights - Use, duplication or
* disclosure restricted by GSA ADP Schedule Contract with
* IBM Corp.
* ===========================================================================
*/
package com.ibm.ims.connect.impl;
import com.ibm.ims.connect.Mbr;
import java.util.Vector;
/**
* @author kevin
*
*/
public class MbrImpl implements Mbr
{
private String elementText = null;
private boolean typPresent = false;
private boolean stypPresent = false;
private boolean vsnPresent = false;
private boolean jobnamePresent = false;
private boolean rcPresent = false;
private boolean rsnPresent = false;
private boolean rsntxtPresent = false;
private boolean msgPresent = false;
private String typ = null;
private String styp = null;
private String vsn = null;
private String jobname = null;
private String rc = null;
private String rsn = null;
private String rsntxt = null;
private String[] msg = null;
public MbrImpl(String aMbrElementText)
{
this.setElementText(aMbrElementText);
}
/**
* @return the typPresent
*/
public boolean isTypPresent()
{
return this.typPresent;
}
/**
* @param typPresent the typPresent to set
*/
public void setTypPresent(boolean typPresent)
{
this.typPresent = typPresent;
}
/**
* @return the stypPresent
*/
public boolean isStypPresent()
{
return this.stypPresent;
}
/**
* @param stypPresent the stypPresent to set
*/
public void setStypPresent(boolean stypPresent)
{
this.stypPresent = stypPresent;
}
/**
* @return the vsnPresent
*/
public boolean isVsnPresent()
{
return this.vsnPresent;
}
/**
* @param vsnPresent the vsnPresent to set
*/
public void setVsnPresent(boolean vsnPresent)
{
this.vsnPresent = vsnPresent;
}
/**
* @return the jobnamePresent
*/
public boolean isJobnamePresent()
{
return this.jobnamePresent;
}
/**
* @param jobnamePresent the jobnamePresent to set
*/
public void setJobnamePresent(boolean jobnamePresent)
{
this.jobnamePresent = jobnamePresent;
}
/**
* @return the rcPresent
*/
public boolean isRcPresent()
{
return this.rcPresent;
}
/**
* @param rcPresent the rcPresent to set
*/
public void setRcPresent(boolean rcPresent)
{
this.rcPresent = rcPresent;
}
/**
* @return the rsnPresent
*/
public boolean isRsnPresent()
{
return this.rsnPresent;
}
/**
* @param rsnPresent the rsnPresent to set
*/
public void setRsnPresent(boolean rsnPresent)
{
this.rsnPresent = rsnPresent;
}
/**
* @return the rsntxtPresent
*/
public boolean isRsntxtPresent()
{
return this.rsntxtPresent;
}
/**
* @param rsntxtPresent the rsntxtPresent to set
*/
public void setRsntxtPresent(boolean rsntxtPresent)
{
this.rsntxtPresent = rsntxtPresent;
}
/**
* @return the msgPresent
*/
public boolean isMsgPresent()
{
return this.msgPresent;
}
/**
* @param msgPresent the msgPresent to set
*/
public void setMsgPresent(boolean msgPresent)
{
this.msgPresent = msgPresent;
}
/**
* @return the jobname
*/
public String getJobname()
{
return this.jobname;
}
/**
* @param aJobname the jobname to set
*/
public void setJobname(String aJobname)
{
this.jobname = aJobname;
this.setJobnamePresent(true);
}
/**
* @return the elementText
*/
public String getElementText()
{
return this.elementText;
}
/**
* @param anElementText the elementText to set
*/
public void setElementText(String anElementText)
{
this.elementText = anElementText;
}
/**
* @return the msg
*/
public String[] getMsg()
{
return this.msg;
}
/**
* @return the last element of the msg String Vector
*/
public String getLastMsgElement()
{
return this.msg[this.msg.length - 1];
}
/**
* @return the msg element at position anElementNumber
*/
public String getMsg(int anElementNumber)
{
return this.msg[anElementNumber];
}
/**
* @param aMsg the msg to set
*/
public void setMsg(String[] aMsg)
{
this.msg = aMsg;
this.setMsgPresent(true);
}
/**
* @param aNewMsgElement a new Msg element to be appended to the end of the current msg String Vector
*/
public void addMsgElement(String aNewMsgElement)
{
String[] newMsg = new String[msg.length + 1];
if(msg.length > 0)
System.arraycopy(msg, 0, newMsg, 0, msg.length);
newMsg[msg.length] = aNewMsgElement;
this.msg = newMsg;
this.setMsgPresent(true);
}
/**
* @param anElementNumber the position of the element in the msg Vector to be changed
* @param aNewMsgElement the new msg element to be placed at position anElementNumber of the msg
*/
public void setMsgElement(int anElementNumber, String aNewMsgElement)
{
this.msg[anElementNumber] = aNewMsgElement;
this.setMsgPresent(true);
}
/**
* @return the rc
*/
public String getRc()
{
return this.rc;
}
/**
* @param aRc the rc to set
*/
public void setRc(String aRc)
{
this.rc = aRc;
this.setRcPresent(true);
}
/**
* @return the rsn
*/
public String getRsn()
{
return this.rsn;
}
/**
* @param aRsn the rsn to set
*/
public void setRsn(String aRsn)
{
this.rsn = aRsn;
this.setRsnPresent(true);
}
/**
* @return the rsntxt
*/
public String getRsntxt()
{
return this.rsntxt;
}
/**
* @param aRsntxt the rsntxt to set
*/
public void setRsntxt(String aRsntxt)
{
this.rsntxt = aRsntxt;
this.setRsntxtPresent(true);
}
/**
* @return the styp
*/
public String getStyp()
{
return this.styp;
}
/**
* @param styp the styp to set
*/
public void setStyp(String styp)
{
this.styp = styp;
this.setStypPresent(true);
}
/**
* @return the typ
*/
public String getTyp()
{
return this.typ;
}
/**
* @param typ the typ to set
*/
public void setTyp(String typ)
{
this.typ = typ;
this.setTypPresent(true);
}
/**
* @return the vsn
*/
public String getVsn()
{
return this.vsn;
}
/**
* @param vsn the vsn to set
*/
public void setVsn(String vsn)
{
this.vsn = vsn;
this.setVsnPresent(true);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy