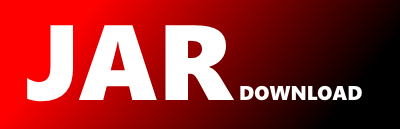
com.ibm.ims.connect.impl.OutputMessageImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of IMSConnectAPI Show documentation
Show all versions of IMSConnectAPI Show documentation
API that allows Java applications to interface with IMS Connect
The newest version!
/*
* File: OutputMessageImpl.java
* ==========================================================================
* Licensed Material - Property of IBM
*
* IBM Confidential
*
* OCO Source Materials
*
* 5655-TDA
*
* (C) Copyright IBM Corp. 2009, 2014 All Rights Reserved.
*
* The source code for this program is not published or
* otherwise divested of its trade secrets, irrespective
* of what has been deposited with the U.S. Copyright
* Office.
*
* US Government Users Restricted Rights - Use, duplication or
* disclosure restricted by GSA ADP Schedule Contract with
* IBM Corp.
* ===========================================================================
*/
package com.ibm.ims.connect.impl;
import java.io.UnsupportedEncodingException;
import java.util.ArrayList;
import java.util.logging.Level;
import java.util.logging.Logger;
import com.ibm.ims.connect.ApiProperties;
import com.ibm.ims.connect.ImsConnectApiException;
import com.ibm.ims.connect.ImsConnectErrorMessage;
import com.ibm.ims.connect.ImsConnectExecutionException;
import com.ibm.ims.connect.OutputMessage;
import com.ibm.ims.connect.OutputMessageProperties;
import com.ibm.ims.connect.TmInteraction;
import com.ibm.ims.connect.Type2CmdResponse;
import com.ibm.ims.connect.XmlParser;
/**
* The class represents the output message received from IMS Connect.
*
* @author hfung
*
* To change the template for this generated type comment go to
* Window>Preferences>Java>Code Generation>Code and Comments
*/
public class OutputMessageImpl implements OutputMessage {
@SuppressWarnings("unused")
private static final String copyright = "Licensed Material - Property of IBM "
+ "5655-TDA"
+ "(C) Copyright IBM Corp. 2009,2013 All Rights Reserved. "
+ "US Government Users Restricted Rights - Use, duplication or "
+ "disclosure restricted by GSA ADP Schedule Contract with IBM Corp. ";
private TmInteractionImpl myTmInteractionImpl;
private Type2CmdResponse myType2CommandResponse = null;
private XmlParser myXmlParser = new XmlParser();
// private SAXParser mySAXParser;
// private ResponseProcessor myResponseProcessor = new ResponseProcessor();
// ------------------Added for Sync
// callout------------------------------////
private int[] parsedOutLlllValIntArray; // array of LL values as an array of
// shorts (each LL value as a short)
// for all of the segments in the
// output message
private byte[][] parsedOutLlllByteArray;
// -------------------End-----------------------------
private byte[][] parsedOutLlByteArray; // array of LL bytes (each LL as a
// byte array) for all of the
// segments in the output message
private short[] parsedOutLlValShortArray; // array of LL values as an array
// of shorts (each LL value as a
// short) for all of the
// segments in the output
// message
private byte[][] parsedOutZzByteArray; // array of ZZ bytes (each ZZ as a
// byte array) for all of the
// segments in the output message
private byte[][] parsedOutDataByteArray; // array of data bytes (each
// segment of data as a byte
// array) for all of the
// segments in the output
// message
private byte[] llll;
private byte[] ll;
// private short llVal = 0;
private byte[] zz;
private int numberOfSegments;
private byte[] responseMessage = null; // response message as received from
// IMS Connect including segment
// data and LLZZs for each segment
// plus optional LLLL
private int messageLength = 0; // length of responseMessage
@SuppressWarnings("static-access")
private int msgType = OutputMessageProperties.MSGTYPE_INVALID;
private Logger logger;
private byte csmFlag1 = OutputMessageProperties.CSM_FLG1_NOFLAG;
private byte csmFlag2 = OutputMessageProperties.CSM_FLG2_NOFLAG;
private String csmStr;
private int rsmReturnCode;
private int rsmReasonCode;
private int rsmOtmaSenseCode;
private byte rsmOtmaReasonCode;
private int rsmRacfReturnCode;
private String racfReturnCodeString;
private boolean responseIncludesLlll;
private boolean ackNakNeeded;
private boolean asyncOutputAvailable;
private boolean inConversation;
private byte protocolLevel;
private boolean protocolLevelAvailable;
private String mfsModname;
private String clientId;
private boolean responsePropertiesUpdatedAfterResponse = false;
// private boolean asyncOutputAvailablePropertyUpdated = false;
// private boolean inConversationPropertyUpdated = false;
private String myImsConnectCodepage = DEFAULT_CODEPAGE_EBCDIC_US;
private boolean includeLlzzInSegments;
private boolean messageIsSingleSegment;
private int rmmOffset = 0;
private int gencidOffset = 0;
private int corOffset = 0;
private boolean isSyncCalloutReq = false;
OutputMessageImpl(TmInteraction myTMInteraction) {
logger = Logger.getLogger("com.ibm.ims.connect");
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
// if
// (logger.getLevel().intValue()<=ApiProperties.TRACE_LEVEL_ENTRY_EXIT.intValue())
logger.finer("--> OutputMessage(TmInteraction) constructor");
myTmInteractionImpl = (TmInteractionImpl) myTMInteraction;
this.myImsConnectCodepage = myTmInteractionImpl.getImsConnectCodepage();
this.setIncludeLlzzInSegments(myTMInteraction
.isInputMessageDataSegmentsIncludeLlzzAndTrancode());
// this.setUse2DimensionalByteArray(myTMInteraction.isUse2DimensionalByteArray());
this.setMessageIsSingleSegment(((TmInteractionImpl) myTMInteraction)
.getNumberOfSegmentsFromOutput() > 2 ? false : true);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
// if
// (logger.getLevel().intValue()<=ApiProperties.TRACE_LEVEL_ENTRY_EXIT.intValue())
logger.finer("<-- OutputMessage(TmInteraction)");
}
public void reset(TmInteraction aTMInteraction) {
this.responseMessage = null;
this.messageLength = 0;
this.msgType = OutputMessageProperties.MSGTYPE_INVALID;
this.csmFlag1 = OutputMessageProperties.CSM_FLG1_NOFLAG;
this.csmStr = null;
this.rsmReturnCode = 0;
this.rsmReasonCode = 0;
this.setIncludeLlzzInSegments(aTMInteraction
.isInputMessageDataSegmentsIncludeLlzzAndTrancode());
// this.setUse2DimensionalByteArray(aTMInteraction.isUse2DimensionalByteArray());
this.rsmOtmaSenseCode = 0;
this.rsmOtmaReasonCode = 0;
this.rsmRacfReturnCode = 0;
this.racfReturnCodeString = "";
this.responseIncludesLlll = true;
this.ackNakNeeded = false;
this.asyncOutputAvailable = false;
this.inConversation = false;
this.isSyncCalloutReq = false;
this.protocolLevel = 0;
this.protocolLevelAvailable = false;
this.mfsModname = " ";
this.clientId = "";
this.responsePropertiesUpdatedAfterResponse = false;
if (this.myType2CommandResponse != null)
myType2CommandResponse = new Type2CmdResponse(
this.myTmInteractionImpl.getImsConnectCodepage());
}
public void copyOutputMessage(OutputMessageImpl anOutputMessage) {
this.responseMessage = anOutputMessage.getResponseMessage();
this.messageLength = anOutputMessage.getMessageLength();
this.msgType = anOutputMessage.getMsgType();
this.numberOfSegments = anOutputMessage.getNumberOfSegments();
this.parsedOutLlllByteArray = anOutputMessage
.getParsedOutLlllByteArray();
this.parsedOutLlllValIntArray = anOutputMessage
.getParsedOutLlllValIntArray();
this.parsedOutLlByteArray = anOutputMessage.getParsedOutLlByteArray();
this.parsedOutLlValShortArray = anOutputMessage
.getParsedOutLlValShortArray();
this.parsedOutZzByteArray = anOutputMessage.getParsedOutZzByteArray();
this.parsedOutDataByteArray = anOutputMessage
.getParsedOutDataByteArray();
this.setImsConnectReturnCode(anOutputMessage.getImsConnectReturnCode());
this.setImsConnectReasonCode(anOutputMessage.getImsConnectReasonCode());
this.setOtmaSenseCode(anOutputMessage.getOtmaSenseCode());
this.setOtmaReasonCode(anOutputMessage.getOtmaReasonCode());
this.myImsConnectCodepage = anOutputMessage.getMyImsConnectCodepage();
this.csmFlag1 = anOutputMessage.getCsmFlag1();
this.csmStr = anOutputMessage.getCsmStr();
this.includeLlzzInSegments = anOutputMessage.isIncludeLlzzInSegments();
}
public void parse(boolean aResponseIncludesLlll) throws Exception {
this.msgType = 0xFF; // ???
String checkStr = null;
String checkRsmStr = "";
String checkCsmStr = null;
// String checkTknStr =null;
String checkT2CmdStr = null;
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("--> OutputMessage.parse(boolean)");
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL)) {
logger.finest(" OutputMessage.parse(boolean) - message length: ["
+ responseMessage.length + "]");
}
// This parse method will check for:
// COR (The correlation token is the first structure in a synchronous
// callout request and can be followed by the optional RMM structure, followed by optional GENCID, and
// the application data)
// 2 bytes COR_Len
// 2 bytes COR_Rsvd
// 8 bytes COR_Id (*CORTKN*)
// 40 bytes Corelator token
// 52 bytes total w/0 LLLL
// (56 bytes total w/ LLLL)
//
// RMM (optional message that contains MODNAME + data - not applicable
// to IMS command output)
// 2 bytes length
// 2 bytes zz flag
// 8 bytes RMM ID (*REQMOD*)
// 8 bytes MOD
// 20 bytes total w/o LLLL
// (24 bytes total w/ LLLL)
//
// GENCID: (optional message that contains CLIENID + data - not applicable
// to IMS command output)
// 2 bytes length
// 2 bytes zz flag
// 8 bytes GEN CLIENT ID (*GENCID*)
// 8 bytes Client ID name
// 20 bytes total w/o LLLL
// (24 bytes total w/ LLLL)
//
// CSM : (last structure in an output message resulting from a
// successfully processed IMS tran or IMS command input message)
// 2 byte length
// 1 byte CSM flag
// 1 byte reserved
// 8 bytes CSM ID (*CSMOKY*)
// 12 bytes total w/o LLLL
// (16 bytes total w/ LLLL)
//
// RSM : (only structure in an output message resulting from an
// unsuccessfully processed intput message - also applicable to IMS
// command output)
// 2 byte length
// 1 byte RSM flag 1 (Z1)
// 1 byte RSM flag 2 (Z2 - OTMA reason code if retcode=16 or RACF return
// code if retcode=8 and rescode=40)
// 8 bytes RSM ID (*REQSTS*)
// 4 bytes retcode
// 4 bytes rescode (OTMA reason code if retcode=16)
// 20 bytes total w/o LLLL
// (24 bytes total w/ LLLL)
//
// Structure of output message from IMS tran containing RMM + Data + CSM
// (not applicable to IMS command output)
// [LLLL]*|[RMM]*|[LLZZ|Data]#|[CSM]
// Structure of output message from IMS tran containing RMM + GENCID + Data + CSM
// (not applicable to IMS command output)
// [LLLL]*|[RMM]*|[GENCID]*|[LLZZ|Data]#|[CSM]
// Structure of output message from IMS tran containing GENCID + Data + CSM
// (not applicable to IMS command output)
// [LLLL]*|[GENCID]*|[LLZZ|Data]#|[CSM]
//
// Structure of output message from IMS tran or IMS command containing
// only Data + CSM (LLLL optional)
// [LLLL]*|[LLZZ|Data]#|[CSM] // one LLZZData for each segment in the
// message)
//
// Structure of a synchronous callout request message sent by IMS
// Connect to a client application
// [LLLL]*|[LLZZ COR]*|[RMM]*|[LLLLData]#|[CSM]
//
// Structure of a synchronous callout request message sent by IMS with RMM and CLIENTID
// Connect to a client application
// [LLLL]*|[LLZZ COR]*|[RMM]*|[GENCID]*|[LLLLData]#|[CSM]
//
// Structure of a synchronous callout request message sent by IMS without RMM
// Connect to a client application
// [LLLL]*|[LLZZ COR]*|[LLLLData]#|[CSM]
//
// Structure of a Type-2 command response message sent by IMS Connect to
// a client application
// [LLLL]|[XML message] (ends with <\imsout>)
//
// Structure of error message (not applicable to IMS command output)
// [LLLL]*|[RSM]
// * - zero or one (e.g., zero or one LLLL depending on the processing
// in user message exit)
// # - one LLZZData (or LLLLData for synchronous callout) for each
// segment in the message
try {
// If the message length is smaller than the minimum length of a
// valid message,
// the message is bad.
if (this.responseMessage.length < OutputMessageProperties.LEN_MSG_MIN) {
String errMsg = ImsConnectErrorMessage
.getString(ImsConnectErrorMessage.HWS0019E);
ImsConnectExecutionException e = new ImsConnectExecutionException(
ImsConnectErrorMessage.HWS0019E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in OutputMessage.parse(boolean). Exception was: "
+ e.toString());
throw e;
}
checkStr = new String(this.responseMessage, this.myImsConnectCodepage);
//Checking for Type2Command message
if (this.myTmInteractionImpl.getInteractionTypeDescription()
.equals(ApiProperties.INTERACTION_TYPE_DESC_TYPE2_COMMAND))
{
checkT2CmdStr = (checkStr.toUpperCase()
.endsWith(OutputMessageProperties.T2C_ID)) ? OutputMessageProperties.T2C_ID
: null;
if (checkT2CmdStr == (null)) {
//Checking if its an RSM that is received
checkRsmStr = checkStr.substring((aResponseIncludesLlll ? 8
: 4), (aResponseIncludesLlll ? 16 : 12));
}
}
//Checking if its a CSM that is received
else {
checkCsmStr = (checkStr
.endsWith(OutputMessageProperties.CSM_ID_CSMOKY)) ? OutputMessageProperties.CSM_ID_CSMOKY
: null;
if (checkCsmStr == (null)) {
//Checking if its and RSM
checkRsmStr = checkStr.substring((aResponseIncludesLlll ? 8
: 4), (aResponseIncludesLlll ? 16 : 12));
}
}
// By now in the code we know if we have got an RSM, CSM or a type2 message.
//Now we have to determine if the message came with LLLL or not
if (aResponseIncludesLlll == false)
{
llll = null;
// If its and RSM
if (this.responseMessage.length == OutputMessageProperties.LEN_RSM)
// w/o
// LLLL
{
// Check for RSM ID to make sure
if (checkRsmStr.equals(OutputMessageProperties.RSM_ID_REQSTS)) {
// It's RSM message
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL))
logger.finest(" OutputMessage.parse(boolean) - message includes RSM (*REQSTS*)");
this.msgType = OutputMessageProperties.MSGTYPE_RSM_REQSTS;
} else if (checkRsmStr.equals(OutputMessageProperties.RSM_ID_CBLADP)) {
// It's RSM message
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL))
logger.finest(" OutputMessage.parse(boolean) - message includes RSM (*XADPST*)");
this.msgType = OutputMessageProperties.MSGTYPE_RSM_CBLADP; // check w/ Himakar about what this is
} else if (checkCsmStr.equals(OutputMessageProperties.CSM_ID_CSMOKY)) {
// It's CSM message
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL))
logger.finest(" OutputMessage.parse(boolean) - message includes CSM (*CSMOKY*)");
this.msgType = OutputMessageProperties.MSGTYPE_DATA;
}
else { // Bad message - does not contain any valid RSM or
// CSM ID,
String errMsg = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0018E);
ImsConnectExecutionException e = new ImsConnectExecutionException(
ImsConnectErrorMessage.HWS0018E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception thrown in OutputMessage.parse(boolean). Exception was: "
+ e.toString());
throw e;
}
} else // this.responseMessage.length != OutputMessageProperties.LEN_RSM (w/o LLLL)
{
// Check for CSM ID
if (checkCsmStr.equals(OutputMessageProperties.CSM_ID_CSMOKY)) {
// It's response message with CSM
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL))
logger.finest(" OutputMessage.parse(boolean) - message includes CSM (*CSMOKY*)");
this.msgType = OutputMessageProperties.MSGTYPE_DATA;
}
else { // Bad message - does not contain any valid RSM or
// CSM ID,
String errMsg = ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0018E);
ImsConnectExecutionException e = new ImsConnectExecutionException(
ImsConnectErrorMessage.HWS0018E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger.severe(" Exception thrown in OutputMessage.parse(boolean). Exception was: "
+ e.toString());
throw e;
}
}
}
else // aResponseIncludesLlll = true
{
llll = new byte[4];
System.arraycopy(this.responseMessage, 0, llll, 0, 4);
if ((checkCsmStr != null)
&& checkCsmStr.equals(OutputMessageProperties.CSM_ID_CSMOKY)) {
// It's response message with CSM
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL))
logger.finest(" OutputMessage.parse(boolean) - message includes CSM (*CSMOKY*)");
this.msgType = OutputMessageProperties.MSGTYPE_DATA;
} else if ((checkT2CmdStr != null)
&& (checkT2CmdStr.equals(OutputMessageProperties.T2C_ID))) {
// It's Type-2 command XML response message
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL))
logger.finest(" OutputMessage.parse(boolean) - message includes XML Type-2 command response");
this.msgType = OutputMessageProperties.MSGTYPE_T2CMD_XML_RESPONSE;
} else if ((checkRsmStr != null)
&& (this.responseMessage.length == OutputMessageProperties.LEN_RSM + 4)) // RSM
// w/LLLL
{
// Check for RSM ID to make sure
if (checkRsmStr .equals(OutputMessageProperties.RSM_ID_REQSTS)) {
// It's RSM message
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL))
// if
// (logger.getLevel().intValue()<=ApiProperties.TRACE_LEVEL_INTERNAL.intValue())
logger .finest(" OutputMessage.parse(boolean) - message includes RSM (*REQSTS*)");
this.msgType = OutputMessageProperties.MSGTYPE_RSM_REQSTS;
} else if (checkRsmStr
.equals(OutputMessageProperties.RSM_ID_CBLADP)) {
// It's RSM message
if (logger.getLevel() == Level.INFO) // himakar Callout
// changes
logger.finest(" OutputMessage.parse(boolean) - message includes RSM (*XADPST*)");
this.msgType = OutputMessageProperties.MSGTYPE_RSM_CBLADP; // check w/ Himakar about what this is
}
} else { // Bad message - does not contain any valid RSM or CSM
// ID,
String errMsg = ImsConnectErrorMessage
.getString(ImsConnectErrorMessage.HWS0018E);
ImsConnectExecutionException e = new ImsConnectExecutionException(
ImsConnectErrorMessage.HWS0018E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in OutputMessage.parse(boolean). Exception was: "
+ e.toString());
throw e;
}
}
// By Now we know what kind of message we have retried.
//Now will be parsing the code to break down the message
if (checkStr != null) {
// himakar 03/05/07 Callout changes reset the CSM response indicator
this.csmFlag1 = OutputMessageProperties.CSM_FLG1_NOFLAG;
// Retrieve the Return code and Reason Code
// for JDK1.4
java.nio.ByteBuffer buf = java.nio.ByteBuffer.wrap(this
.getResponseMessage());
if ((this.msgType == OutputMessageProperties.MSGTYPE_RSM_REQSTS)
|| (this.msgType == OutputMessageProperties.MSGTYPE_RSM_CBLADP)) {
if (aResponseIncludesLlll == false) {
this.rsmReturnCode = buf
.getInt(OutputMessageProperties.POS_RSM_RETCOD);
if (this.rsmReturnCode == 16) {
this
.setOtmaSenseCode(buf
.getInt(OutputMessageProperties.POS_RSM_RSNCOD));
this
.setOtmaReasonCode((byte) this.responseMessage[OutputMessageProperties.POS_CSM_FLG_Z2]);
} else if ((this.rsmReturnCode == 8)
&& ((byte) buf
.getInt(OutputMessageProperties.POS_RSM_RSNCOD) == 40)) {
this
.setImsConnectReasonCode(buf
.getInt(OutputMessageProperties.POS_RSM_RSNCOD));
this
.setRacfReturnCode((byte) this.responseMessage[OutputMessageProperties.POS_CSM_FLG_Z2]);
} else
this
.setImsConnectReasonCode(buf
.getInt(OutputMessageProperties.POS_RSM_RSNCOD));
} else {
this
.setImsConnectReturnCode(buf
.getInt(OutputMessageProperties.POS_RSM_RETCOD + 4));
if (this.rsmReturnCode == 16) {
this
.setOtmaSenseCode(buf
.getInt(OutputMessageProperties.POS_RSM_RSNCOD + 4));
this
.setOtmaReasonCode((byte) this.responseMessage[OutputMessageProperties.POS_CSM_FLG_Z2 + 4]);
} else if ((this.rsmReturnCode == 8)
&& ((byte) buf
.getInt(OutputMessageProperties.POS_RSM_RSNCOD + 4) == 40)) {
this
.setImsConnectReasonCode(buf
.getInt(OutputMessageProperties.POS_RSM_RSNCOD + 4));
this
.setRacfReturnCode((byte) this.responseMessage[OutputMessageProperties.POS_CSM_FLG_Z2 + 4]);
} else
this
.setImsConnectReasonCode(buf
.getInt(OutputMessageProperties.POS_RSM_RSNCOD + 4));
}
this.mfsModname = new String(" ");
}
else if (this.msgType == OutputMessageProperties.MSGTYPE_DATA) {
// Retrieve CSM response indicator byte
this.csmStr = OutputMessageProperties.CSM_ID_CSMOKY;
this.csmFlag1 = this.responseMessage[this.responseMessage.length
- OutputMessageProperties.LEN_CSM
+ OutputMessageProperties.POS_CSM_FLG_Z1];
this.csmFlag2 = this.responseMessage[this.responseMessage.length
- OutputMessageProperties.LEN_CSM
+ OutputMessageProperties.POS_CSM_FLG_Z2];
checkCsmStr = null;
checkCsmStr = checkStr.substring((aResponseIncludesLlll ? 8
: 4), (aResponseIncludesLlll ? 16 : 12));
// ------------------Added for Synccallout------------------------------////
// Check for COR
if (checkCsmStr.equals(OutputMessageProperties.COR_ID_CORTKN))
{
//Dynamically taking the correlator token Len
//this.setCorrelatorLength((short) (((aCorrelatorToken[i++] & 0xFF) << 8) | (aCorrelatorToken[i++] & 0xFF)));
//int correlatorLen =(short) (((this.responseMessage[aResponseIncludesLlll ? 16 : 12] & 0xFF) << 8) | (this.responseMessage[(aResponseIncludesLlll ? 16 : 12)+1] & 0xFF));
//System.out.println("Correlator token len = "+correlatorLen);
//byte[] correlatorTokenByteArray = new byte[correlatorLen];
byte[] correlatorTokenByteArray = new byte[IRM_CT_LEN];
// copying the corelator token;
System.arraycopy(this.responseMessage,
aResponseIncludesLlll ? 16 : 12,
correlatorTokenByteArray, 0,
OutputMessageProperties.LEN_COR);
myTmInteractionImpl.setCorrelatorToken(correlatorTokenByteArray);
corOffset = 52;
this.isSyncCalloutReq = true;
// Checking if RMM
checkCsmStr = null;
int tempStPOS = OutputMessageProperties.LEN_COR_SEGMENT+4;
int tempEndPOS = OutputMessageProperties.LEN_COR_SEGMENT+4+OutputMessageProperties.LEN_RMM_ID_REQMOD;
checkCsmStr = checkStr.substring(
aResponseIncludesLlll ? (4+tempStPOS) : (tempStPOS),
aResponseIncludesLlll ? (4+tempEndPOS) : tempEndPOS);
if (checkCsmStr
.equals(OutputMessageProperties.RMM_ID_REQMOD)) {
byte[] mfsModnameByteArray = new byte[8];
tempStPOS = tempEndPOS;
System.arraycopy(this.responseMessage,
aResponseIncludesLlll ? (tempStPOS+4) : (tempStPOS),
mfsModnameByteArray, 0,
OutputMessageProperties.LEN_RMM);
this.mfsModname = new String(mfsModnameByteArray,
myImsConnectCodepage);
rmmOffset = 20;
// To Check if there is a CLIENTID after RMM changing the ckeckCsmStr so that it can be checked outsid ethe RMM loop
tempStPOS = tempEndPOS + OutputMessageProperties.LEN_LLZZ+ OutputMessageProperties.LEN_RMM ;
tempEndPOS = tempStPOS + OutputMessageProperties.LEN_CLIENTID_ID_GENCID;
checkCsmStr = checkStr.substring(
aResponseIncludesLlll ? (4+tempStPOS) : (tempStPOS),
aResponseIncludesLlll ? (4+tempEndPOS) : tempEndPOS);
} else {
this.mfsModname = new String(" ");
rmmOffset = 0;
}
//Checking if there is a clientID after the CORTKN or RMM
if (checkCsmStr.equals(OutputMessageProperties.CLIENTID_ID_GENCID))
{
byte[] clientIdByteArray = new byte[8];
tempStPOS = tempEndPOS;
System.arraycopy(this.responseMessage,
aResponseIncludesLlll ? (tempStPOS+4) : (tempStPOS),
clientIdByteArray, 0,
OutputMessageProperties.LEN_GENCID);
this.clientId = new String(clientIdByteArray,
myImsConnectCodepage);
gencidOffset = 20;
}else {
this.clientId = new String("");
gencidOffset = 0;
}
}
else
{
// ------------------End----------------------------
// Check for RMM
int tempStPOS = 12;
int tempEndPOS =0;
if (checkCsmStr
.equals(OutputMessageProperties.RMM_ID_REQMOD)) {
byte[] mfsModnameByteArray = new byte[8];
System.arraycopy(this.responseMessage,
aResponseIncludesLlll ? (tempStPOS+4) : (tempStPOS),
mfsModnameByteArray, 0,
OutputMessageProperties.LEN_RMM);
this.mfsModname = new String(mfsModnameByteArray,
myImsConnectCodepage);
rmmOffset = 20;
//if RMM is there we need to check if there is a ClientID following it
//For that we should change the checkCsmStr so that it can be checked in the outer loop.
// To Check if there is a CLIENTID after RMM changing the ckeckCsmStr so that it can be checked outsid ethe RMM loop
tempStPOS = tempStPOS+ OutputMessageProperties.LEN_RMM_ID_REQMOD +OutputMessageProperties.LEN_LLZZ;
tempEndPOS = tempStPOS + OutputMessageProperties.LEN_CLIENTID_ID_GENCID;
checkCsmStr = checkStr.substring(
aResponseIncludesLlll ? (4+tempStPOS) : (tempStPOS),
aResponseIncludesLlll ? (4+tempEndPOS) : tempEndPOS);
} else {
this.mfsModname = new String(" ");
rmmOffset = 0;
}
//Checking if there is a clientID after the RMM
if (checkCsmStr.equals(OutputMessageProperties.CLIENTID_ID_GENCID))
{
if(tempStPOS!= 12)
tempStPOS = tempEndPOS;
byte[] clientIdByteArray = new byte[8];
System.arraycopy(this.responseMessage,
aResponseIncludesLlll ? (tempStPOS+4) : (tempStPOS),
clientIdByteArray, 0,
OutputMessageProperties.LEN_GENCID);
this.clientId = new String(clientIdByteArray,
myImsConnectCodepage);
gencidOffset = 20;
}else {
this.clientId = new String("");
gencidOffset = 0;
}
}// End of Else for inbound conditions
//Setting MFS Modname and ClientId
myTmInteractionImpl.setMfsModname(this.mfsModname);
if(!this.clientId.equals(""))
myTmInteractionImpl.getMyConnection().setClientId(this.clientId);
} else if (this.msgType == OutputMessageProperties.MSGTYPE_T2CMD_XML_RESPONSE)
{
checkCsmStr = null;
checkCsmStr = checkStr.substring((aResponseIncludesLlll ? 8
: 4), (aResponseIncludesLlll ? 16 : 12));
//Checking if there is a clientID after the CORTKN or RMM
if (checkCsmStr.equals(OutputMessageProperties.CLIENTID_ID_GENCID))
{
byte[] clientIdByteArray = new byte[8];
System.arraycopy(this.responseMessage,
aResponseIncludesLlll ? 16 : 12,
clientIdByteArray, 0,
OutputMessageProperties.LEN_GENCID);
this.clientId = new String(clientIdByteArray,
myImsConnectCodepage);
gencidOffset = 20;
}else {
this.clientId = new String("");
gencidOffset = 0;
}
if(!this.clientId.equals(""))
myTmInteractionImpl.getMyConnection().setClientId(this.clientId);
} else {
// Bad message - does not contain any valid RSM or CSM ID,
String errMsg = ImsConnectErrorMessage
.getString(ImsConnectErrorMessage.HWS0018E);
ImsConnectExecutionException e = new ImsConnectExecutionException(
ImsConnectErrorMessage.HWS0018E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in OutputMessage.parse(boolean). Exception was: "
+ e.toString());
throw e;
}
}
// save LLLL and numberOfSegments values and LL, ZZ and data for
// each segment
parseOutMsgByteArray();
}
/*
* catch (Exception e2) { if (e2 instanceof ImsConnectApiException)
* throw e2; else { if
* (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
* logger.severe
* (" OutputMessage.parse(boolean) encountered an exception: " +
* e2.getClass() + e2.getMessage() + " - cause was " + (e2.getCause() ==
* null ? "not identified" : e2.getCause().toString())); //
* logger.severe
* (" OutputMessage.parse(boolean) - unsupportedEncodingException");
* String errMsg =
* ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0017E, new
* Object[] { e2 });
*
* ImsConnectApiException e = new
* ImsConnectApiException(ImsConnectErrorMessage.HWS0017E, errMsg);
*
* throw e; } }
*/
finally {
/*
* if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL)) { int
* msgTyp = this.getMsgType(); String msgTypStr = null;
*
* switch (msgTyp) { case 0: msgTypStr = "MSGTYPE_DATA"; break;
*
* case 1: msgTypStr = "MSGTYPE_DFS"; break;
*
* case 2: msgTypStr = "MSGTYPE_RSM_REQSTS"; break;
*
* case 3: msgTypStr = "MSGTYPE_RSM_CBLADP"; break;
*
* case -1:
*
* default: msgTypStr = "MSGTYPE_INVALID"; }
* logger.finest(" OutputMessage.parse(boolean) - msgType: " +
* msgTypStr); }
*/if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("<-- OutputMessage.parse(boolean)");
}
}
/*
* non-javadoc, internal use only method
*
* Save LLLL and numberOfSegments values and LL, ZZ and data for each
* segment For Type-2 commands, this methods produces a byte array
* containing the complete response message with all the header elements.
* Must be further parsed in two steps; first, to get the header elements
* and second, to get the actual data elements out of the element of
* the response.
*/
public void parseOutMsgByteArray() throws ImsConnectApiException,
ImsConnectExecutionException {
int i = 0;
ArrayList segmentLengths = new ArrayList();
ArrayList segmentLls = new ArrayList();
ArrayList segmentZzs = new ArrayList();
ArrayList segmentData = new ArrayList();
byte[] inputSegment;
int dataLen = 0;
int startCpySrcPos = 0;
setMessageLength();
dataLen = getMessageLength();
if (this.myTmInteractionImpl.getInteractionTypeDescription().equals(
ApiProperties.INTERACTION_TYPE_DESC_TYPE2_COMMAND)) {
startCpySrcPos = 0; // no LLZZ so don't skip over LLLL
} else {
if (this.myTmInteractionImpl.isResponseIncludesLlll())
startCpySrcPos = 4; // skip over LLLL
startCpySrcPos += rmmOffset;
startCpySrcPos += gencidOffset;
}
// ------------------Added for Sync
// callout------------------------------////
if (this.isSyncCalloutReq) // sync callout request
{
int l0Nibble;
int l1Nibble;
int l2Nibble;
int l3Nibble;
startCpySrcPos += corOffset;
// For Data with LLLL
while (startCpySrcPos < dataLen) // calculate number of segments and
// assemble arrays of LL's, ZZ's
// and segment data
{
if (startCpySrcPos == dataLen - OutputMessageProperties.LEN_CSM) // no data,just CSM
l0Nibble = OutputMessageProperties.LEN_CSM;
else {
// Getting the LLLL value of the segment which is 4 bytes
// for Sync callout Data messages
l0Nibble = this.responseMessage[startCpySrcPos] & 0xff;
l0Nibble <<= 24; // set top 8 bits
l0Nibble = l0Nibble & 0xff000000;
l1Nibble = this.responseMessage[startCpySrcPos + 1] & 0xff;
l1Nibble <<= 16; // set upper middle 8 bits
l1Nibble = l1Nibble & 0x00ff0000;
l2Nibble = this.responseMessage[startCpySrcPos + 2] & 0xff;
l2Nibble <<= 8; // set lower middle 8 bits
l2Nibble = l2Nibble & 0x0000ff00;
l3Nibble = this.responseMessage[startCpySrcPos + 3] & 0xff;
l3Nibble = l3Nibble & 0x000000ff;
l0Nibble = (l3Nibble | l2Nibble | l1Nibble | l0Nibble) & 0x7fffffff; // set
// bottom 8 bits
}
segmentLengths.add(l0Nibble);
l0Nibble -= 4; // l0Nibble includes 4 bytes of LLLL, so data
// length is 4 less
this.ll = new byte[4];
System.arraycopy(this.responseMessage, startCpySrcPos, this.ll,
0, 4);
segmentLls.add(ll);
inputSegment = new byte[l0Nibble];
System.arraycopy(this.responseMessage, startCpySrcPos + 4,
inputSegment, 0, l0Nibble);
segmentData.add(inputSegment);
startCpySrcPos += 4 + l0Nibble;
}
numberOfSegments = segmentData.size();
this.parsedOutLlllByteArray = new byte[numberOfSegments][];
this.parsedOutLlllValIntArray = new int[numberOfSegments];
// this.parsedOutZzByteArray = new byte[numberOfSegments][];
this.parsedOutDataByteArray = new byte[numberOfSegments][];
while (i < numberOfSegments) {
this.parsedOutLlllByteArray[i] = new byte[4];
System.arraycopy(segmentLls.get(i), 0,
this.parsedOutLlllByteArray[i], 0, 4);
if (i == numberOfSegments - 1)
parsedOutLlllValIntArray[i] = OutputMessageProperties.LEN_CSM;
else
parsedOutLlllValIntArray[i] = (((Integer) (segmentLengths
.get(i))).intValue());
this.parsedOutDataByteArray[i] = new byte[this.parsedOutLlllValIntArray[i] - 4];
System.arraycopy(segmentData.get(i), 0,
this.parsedOutDataByteArray[i], 0,
this.parsedOutLlllValIntArray[i] - 4);
i++;
}
} else if (this.myTmInteractionImpl.getInteractionTypeDescription()
.equals(ApiProperties.INTERACTION_TYPE_DESC_TYPE2_COMMAND)) {
int l0Nibble;
int l1Nibble;
int l2Nibble;
int l3Nibble;
// Getting the LLLL value of the segment which is 4 bytes for Sync
// callout Data messages
l0Nibble = this.responseMessage[startCpySrcPos] & 0xff;
l0Nibble <<= 24; // set top 8 bits
l0Nibble = l0Nibble & 0xff000000;
l1Nibble = this.responseMessage[startCpySrcPos + 1] & 0xff;
l1Nibble <<= 16; // set upper middle 8 bits
l1Nibble = l1Nibble & 0x00ff0000;
l2Nibble = this.responseMessage[startCpySrcPos + 2] & 0xff;
l2Nibble <<= 8; // set lower middle 8 bits
l2Nibble = l2Nibble & 0x0000ff00;
l3Nibble = this.responseMessage[startCpySrcPos + 3] & 0xff;
l3Nibble = l3Nibble & 0x000000ff;
l0Nibble = (l3Nibble | l2Nibble | l1Nibble | l0Nibble) & 0x7fffffff; // set
// bottom
// 8
// bits
segmentLengths.add(l0Nibble);
inputSegment = new byte[l0Nibble - 4];
System.arraycopy(this.responseMessage, startCpySrcPos + 4,
inputSegment, 0, l0Nibble - 4);
segmentData.add(inputSegment);
startCpySrcPos += l0Nibble - 4;
numberOfSegments = segmentData.size();
this.parsedOutLlllByteArray = new byte[1][];
this.parsedOutLlllValIntArray = new int[1];
// this.parsedOutZzByteArray = new byte[1][];
this.parsedOutDataByteArray = new byte[1][];
this.parsedOutLlllByteArray[0] = new byte[] { 0, 0, 0, 0 };
parsedOutLlllValIntArray[0] = (((Integer) (segmentLengths.get(0)))
.intValue());
this.parsedOutDataByteArray[0] = new byte[this.parsedOutLlllValIntArray[0] - 4];
System.arraycopy(segmentData.get(0), 0,
this.parsedOutDataByteArray[0], 0,
this.parsedOutLlllValIntArray[0] - 4);
myType2CommandResponse.setType2CmdrspdataStale(true);
} else {
short l0Nibble;
short l1Nibble;
while (startCpySrcPos < dataLen) // calculate number of segments and
// assemble arrays of LL's, ZZ's
// and segment data
{
l0Nibble = (short) (this.responseMessage[startCpySrcPos] & 0xff);
l0Nibble <<= 8; // set top 8 bits
l0Nibble = (short) (l0Nibble & 0xff00);
l1Nibble = (short) (this.responseMessage[startCpySrcPos + 1] & 0xff);
l1Nibble &= 0x00ff; // remove propagated sign bits
l1Nibble = (short) (l1Nibble & 0x00ff);
l0Nibble |= l1Nibble; // set bottom 8 bits
segmentLengths.add(l0Nibble);
l0Nibble -= 4; // LL includes LLZZ bytes, so data length is 4
// less
this.ll = new byte[2];
// this.ll[0] = this.responseMessage[startCpySrcPos];
// this.ll[1] = this.responseMessage[startCpySrcPos + 1];
System.arraycopy(this.responseMessage, startCpySrcPos, this.ll,
0, 2);
segmentLls.add(ll);
this.zz = new byte[2];
// this.zz[0] = this.responseMessage[startCpySrcPos + 2];
// this.zz[1] = this.responseMessage[startCpySrcPos + 3];
System.arraycopy(this.responseMessage, startCpySrcPos + 2,
this.zz, 0, 2);
segmentZzs.add(zz);
inputSegment = new byte[l0Nibble];
System.arraycopy(this.responseMessage, startCpySrcPos + 4,
inputSegment, 0, l0Nibble);
segmentData.add(inputSegment);
startCpySrcPos += 4 + l0Nibble;
}
numberOfSegments = segmentData.size();
this.parsedOutLlByteArray = new byte[numberOfSegments][];
this.parsedOutLlValShortArray = new short[numberOfSegments];
this.parsedOutZzByteArray = new byte[numberOfSegments][];
this.parsedOutDataByteArray = new byte[numberOfSegments][];
while (i < numberOfSegments) {
this.parsedOutLlByteArray[i] = new byte[2];
System.arraycopy(segmentLls.get(i), 0,
this.parsedOutLlByteArray[i], 0, 2);
parsedOutLlValShortArray[i] = (((Short) (segmentLengths.get(i)))
.shortValue());
this.parsedOutZzByteArray[i] = new byte[2];
System.arraycopy(segmentZzs.get(i), 0,
this.parsedOutZzByteArray[i], 0, 2);
this.parsedOutDataByteArray[i] = new byte[this.parsedOutLlValShortArray[i] - 4];
System.arraycopy(segmentData.get(i), 0,
this.parsedOutDataByteArray[i], 0,
this.parsedOutLlValShortArray[i] - 4);
i++;
}
}
}
void processType2CmdRsp(byte[] aParsedOutDataByteArray)
throws ImsConnectApiException {
myXmlParser.parseXml(this.myType2CommandResponse,
aParsedOutDataByteArray, myImsConnectCodepage);
/*
* if (this.type2CommandResponse.isResponseContainsMsgdataElement()) {
* Mbr[] messageMemberElements =
* this.type2CommandResponse.getAllMsgdataMbrElements();
*
* if((messageMemberElements != null) && (messageMemberElements.length
* != 0)) { for(int i = 0; i < messageMemberElements.length; i++) {
* Properties rspProps =
* (myResponseProcessor.processProperties(messageMemberElements[i],
* myImsConnectCodepage));
* this.type2CommandResponse.addArrayOfMbrElementsArrayElement
* (rspProps); } } }
*/
myType2CommandResponse.setType2CmdrspdataStale(false);
}
/*
* Returns an array of bytes containing the output data with or without LLLL
* and LLZZ depending on the settings of responseIncludesLlll and
* includeLlzzInSegments. If the response was multi-segment, then the
* includeLlzzInSegments setting is ignored and LLZZ is included in the
* returned byte array.
*/
public byte[] getDataBytes() {
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("--> OutputMessage.getDataBytes()");
byte[] dataBytes;
this.setMessageIsSingleSegment(this.getNumberOfSegments() > 1 ? false
: true);
if (this.responseMessage != null && csmStr != null) {
if (csmStr.equalsIgnoreCase(OutputMessageProperties.CSM_ID_CSMOKY)) {
messageLength = this.responseMessage.length;
if (messageLength > 0) {
int dataBytesLen = messageLength
- ((!this.includeLlzzInSegments && messageIsSingleSegment) ? this.myTmInteractionImpl
.getNumberOfSegmentsFromOutput() * 4
: 0);
dataBytes = new byte[dataBytesLen];
if (this.myTmInteractionImpl
.getIncludeLlllInOutputMessages()) {
// Copy LLLL into 1st 4 bytes of dataBytes (starting at
// 0)
System.arraycopy(this.responseMessage, 0, dataBytes, 0,
4);
if (!includeLlzzInSegments && messageIsSingleSegment) {
// Copy data (starting at 8 since ICON always
// returns LLZZ) into dataBytes (starting at 4)
// Up to
System.arraycopy(this.responseMessage, 8,
dataBytes, 4, messageLength - 4 - 12); // copy
// message
// itself
System.arraycopy(this.responseMessage,
messageLength - 8, dataBytes,
dataBytesLen - 8, 8); // copy CSM segment
} else {
// Copy LLZZ and data into dataBytes (starting at 4)
System.arraycopy(this.responseMessage, 4,
dataBytes, 4, dataBytesLen - 4);
}
} else // Response does not include LLLL so no copying or
// skipping of copying LLLL required
{
if (!includeLlzzInSegments && messageIsSingleSegment) {
// Copy data (starting at 4 since no LLLL but ICON
// always returns LLZZ so it must be skipped) into
// dataBytes (starting at 0)
System.arraycopy(this.responseMessage, 4,
dataBytes, 0, messageLength - 4 - 12);
System.arraycopy(this.responseMessage,
messageLength - 8, dataBytes,
dataBytesLen - 8, 8);
} else // Response does not include LLLL and does
// include LLZZ so no skipping over LLLL or LLZZ
{
// Copy LLZZ + data into dataBytes (starting at 0)
System.arraycopy(this.responseMessage, 0,
dataBytes, 0, messageLength);
}
}
return dataBytes;
}
}
}
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("<-- OutputMessage.getDataBytes()");
return null;
}
/*
* Returns the data in this OutputMessage object as a byte array. The
* returned byte array contains the response message after removal as
* required from the message received from IMS Connect of the LLLL, if
* applicable, and LLZZ bytes.
*/
public byte[] getDataAsByteArray() {
int i = 0;
int llVal;
byte[] returnByteArray = null;
int returnByteArrayLen = 0;
int copyPos = 0;
while (i < numberOfSegments) // count up the number of bytes of data
{
returnByteArrayLen += parsedOutDataByteArray[i++].length;
}
returnByteArray = new byte[returnByteArrayLen];
i = 0;
while (i < numberOfSegments) {
if (this.isSyncCalloutReq)
llVal = (new Integer(parsedOutLlllValIntArray[i])).intValue() - 4;
else
llVal = (new Short(parsedOutLlValShortArray[i])).shortValue() - 4;
System.arraycopy(parsedOutDataByteArray[i++], 0, returnByteArray,
copyPos, llVal);
copyPos += llVal;
}
return returnByteArray;
}
/*
* Returns the data in this OutputMessage object as a 2-dimensional byte
* array. The returned array of byte arrays contains the response message
* received from IMS Connect after it has been split up into a separate byte
* array for each segment in the response message.
*/
public byte[][] getDataAsArrayOfByteArrays() {
return parsedOutDataByteArray;
}
/*
* Returns the data in this OutputMessage object as a String. The returned
* String contains the data portion of the response message received from
* IMS Connect after each byte in the data of the response message has been
* converted to its character representation. Unprintable characters will be
* left as unprintable characters in the returned String.
*/
public String getDataAsString() throws ImsConnectApiException {
StringBuffer strBuf = new StringBuffer("");
int i = 0;
try {
while (i < this.numberOfSegments) {
strBuf.append(new String(this.parsedOutDataByteArray[i++],
this.myImsConnectCodepage));
// strBuf.append(new String(this.parsedOutDataByteArray[i++]));
}
return strBuf.toString();
} catch (UnsupportedEncodingException usee) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0017E, new Object[] {
"output response message",
this.myImsConnectCodepage });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in OutputMessage.getDataAsString(). Exception was: "
+ e.toString());
throw e;
}
}
/*
* Returns the data in this OutputMessage object as a String array. The
* returned array of Strings contains the response message received from IMS
* Connect after it has been split up into a separate Strings for each
* segment in the response message.
*/
public String[] getDataAsArrayOfStrings() throws ImsConnectApiException {
int i = 0;
String[] msgAsStringArray = new String[this.numberOfSegments];
try {
while (i < this.numberOfSegments) {
msgAsStringArray[i] = new String(
this.parsedOutDataByteArray[i],
this.myImsConnectCodepage);
// msgAsStringArray[i] = new
// String(this.parsedOutDataByteArray[i]);
i++;
}
} catch (UnsupportedEncodingException usee) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0017E, new Object[] {
"output response message",
this.myImsConnectCodepage });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0017E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in OutputMessage.getDataAsArrayOfStrings(). Exception was: "
+ e.toString());
throw e;
}
return msgAsStringArray;
}
/*
* Returns the data in the specified segment of this OutputMessage object as
* a byte array. The returned byte array contains the response message after
* removal as required from the message received from IMS Connect of the
* LLLL and/or LLZZ bytes.
*/
public byte[] getSegmentDataAsByteArray(int aSegmentNumber) {
return this.parsedOutDataByteArray[aSegmentNumber];
}
/*
* Returns the data in the specified segment of this OutputMessage object as
* a byte array. The returned byte array contains the response message after
* removal as required from the message received from IMS Connect of the
* LLLL and/or LLZZ bytes.
*/
public String getSegmentDataAsString(int aSegmentNumber) // throws
// ImsConnectApiException
{
// try
{
// return new String(getSegmentDataAsByteArray(aSegmentNumber),
// myImsConnectCodepage);
return new String(getSegmentDataAsByteArray(aSegmentNumber));
}
/*
* catch (UnsupportedEncodingException usee) { String errMsg =
* ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0017E, new
* Object[] {"output response message segment", this.dataCodepage});
* ImsConnectApiException e = new ImsConnectApiException(
* ImsConnectErrorMessage.HWS0017E, errMsg);
*
* if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
* logger.severe(
* " Exception caught in OutputMessage.getDataAsString(). Exception was: "
* + e.toString());
*
* throw e; }
*/}
/**
* Gets the LLLL from the output message response as a byte array.
*
* @return the LLLL from the output message as a byte array
*/
public byte[] getLlll() {
return this.llll;
}
/**
* Gets the LLLL from the output message response as a String of hexidecimal
* digits.
*
* @return the LLLL from the output message as a hex String or a string of
* four 00's if the output message does not include an LLLL.
*/
public String getLlllAsHexString() {
byte[] llllByteArray = this.getLlll();
if (llllByteArray != null) {
int l0 = (int) ((llllByteArray[0] & 0xF0) >> 4);
int l1 = (int) (llllByteArray[0] & 0x0F);
int l2 = (int) ((llllByteArray[1] & 0xF0) >> 4);
int l3 = (int) (llllByteArray[1] & 0x0F);
int l4 = (int) ((llllByteArray[2] & 0xF0) >> 4);
int l5 = (int) (llllByteArray[2] & 0x0F);
int l6 = (int) ((llllByteArray[3] & 0xF0) >> 4);
int l7 = (int) (llllByteArray[3] & 0x0F);
StringBuffer strbuf = new StringBuffer("");
strbuf.append(Integer.toString(l0, 16)); // append high-order nibble
// of 1st byte
strbuf.append(Integer.toString(l1, 16) + " "); // append low-order
// nibble of 1st
// byte
strbuf.append(Integer.toString(l2, 16)); // append high-order nibble
// of 2nd byte
strbuf.append(Integer.toString(l3, 16) + " "); // append low-order
// nibble of 2nd
// byte
strbuf.append(Integer.toString(l4, 16)); // append high-order nibble
// of 1st byte
strbuf.append(Integer.toString(l5, 16) + " "); // append low-order
// nibble of 1st
// byte
strbuf.append(Integer.toString(l6, 16)); // append high-order nibble
// of 2nd byte
strbuf.append(Integer.toString(l7, 16)); // append low-order nibble
// of 2nd byte
return new String(strbuf);
} else
return "00 00 00 00";
}
/**
* Gets the LLLL from the output message response as an integer.
*
* @return the LLLL from the output message as an integer or the value 0 if
* there is no LLLL in the output message
*/
public int getLlllValue() {
byte[] llllByteArray = this.getLlll();
if (llllByteArray != null) {
int l0 = (int) ((llllByteArray[0] & 0xF0) >> 4);
int l1 = (int) (llllByteArray[0] & 0x0F);
int l2 = (int) ((llllByteArray[1] & 0xF0) >> 4);
int l3 = (int) (llllByteArray[1] & 0x0F);
int l4 = (int) ((llllByteArray[2] & 0xF0) >> 4);
int l5 = (int) (llllByteArray[2] & 0x0F);
int l6 = (int) ((llllByteArray[3] & 0xF0) >> 4);
int l7 = (int) (llllByteArray[3] & 0x0F);
int llllValue = l0 << 28 | l1 << 24 | l2 << 20 | l3 << 16
| l4 << 12 | l5 << 8 | l6 << 4 | l7;
return llllValue;
} else
return 0;
}
/**
* Gets the LL bytes from the segment at the location specified by
* aSegmentNumber in the output message as a byte array.
*
* @param aSegmentNumber
* segment offset
* @return the LL bytes from the specified segment as a byte array
*/
public byte[] getSegmentLl(int aSegmentNumber) {
return this.parsedOutLlByteArray[aSegmentNumber];
}
/**
* Gets the LL bytes from the segment at the location specified by
* aSegmentNumber in the output message in hex as a String.
*
* @param aSegmentNumber
* segment offset
* @return the LL bytes from the specified segment in hex as a String
*/
public String getSegmentLlAsHexString(int aSegmentNumber) {
byte[] segmentLlByteArray = this.parsedOutLlByteArray[aSegmentNumber];
int l0 = (int) ((segmentLlByteArray[0] & 0xF0) >> 4);
int l1 = (int) (segmentLlByteArray[0] & 0x0F);
int l2 = (int) ((segmentLlByteArray[1] & 0xF0) >> 4);
int l3 = (int) (segmentLlByteArray[1] & 0x0F);
StringBuffer strbuf = new StringBuffer("");
strbuf.append(Integer.toString(l0, 16)); // append high-order nibble of
// 1st byte
strbuf.append(Integer.toString(l1, 16) + " "); // append low-order
// nibble of 1st byte
strbuf.append(Integer.toString(l2, 16)); // append high-order nibble of
// 2nd byte
strbuf.append(Integer.toString(l3, 16)); // append low-order nibble of
// 2nd byte
return new String(strbuf);
}
/**
* Gets the LL value from the segment at the location specified by
* aSegmentNumber in the output message as a short value.
*
* @param aSegmentNumber
* segment offset
* @returnthe LL value from the specified segment as a short
*/
public short getSegmentLlValue(int aSegmentNumber) {
return this.parsedOutLlValShortArray[aSegmentNumber];
}
// ------------------Added for Sync
// callout------------------------------////
/**
* Gets the LLLL bytes from the segment at the location specified by
* aSegmentNumber in the output message as a byte array. used for sync
* callout requests
*
* @param aSegmentNumber
* segment offset
* @return the LL bytes from the specified segment as a byte array
*/
public byte[] getSegmentLlll(int aSegmentNumber) {
return this.parsedOutLlllByteArray[aSegmentNumber];
}
/**
* Gets the LLLL bytes from the segment at the location specified by
* aSegmentNumber in the output message in hex as a String. *for sync
* callout request
*
* @param aSegmentNumber
* segment offset
* @return the LL bytes from the specified segment in hex as a String
*/
public String getSegmentLlllAsHexString(int aSegmentNumber) {
byte[] segmentLlllByteArray = this.parsedOutLlllByteArray[aSegmentNumber];
int l0 = (int) ((segmentLlllByteArray[0] & 0xF0) >> 4);
int l1 = (int) (segmentLlllByteArray[0] & 0x0F);
int l2 = (int) ((segmentLlllByteArray[1] & 0xF0) >> 4);
int l3 = (int) (segmentLlllByteArray[1] & 0x0F);
int l4 = (int) ((segmentLlllByteArray[2] & 0xF0) >> 4);
int l5 = (int) (segmentLlllByteArray[2] & 0x0F);
int l6 = (int) ((segmentLlllByteArray[3] & 0xF0) >> 4);
int l7 = (int) (segmentLlllByteArray[3] & 0x0F);
StringBuffer strbuf = new StringBuffer("");
strbuf.append(Integer.toString(l0, 16)); // append high-order nibble of
// 1st byte
strbuf.append(Integer.toString(l1, 16) + " "); // append low-order
// nibble of 1st byte
strbuf.append(Integer.toString(l2, 16)); // append high-order nibble of
// 2nd byte
strbuf.append(Integer.toString(l3, 16) + " "); // append low-order
// nibble of 2nd byte
strbuf.append(Integer.toString(l4, 16)); // append high-order nibble of
// 1st byte
strbuf.append(Integer.toString(l5, 16) + " "); // append low-order
// nibble of 1st byte
strbuf.append(Integer.toString(l6, 16)); // append high-order nibble of
// 2nd byte
strbuf.append(Integer.toString(l7, 16)); // append low-order nibble of
// 2nd byte
return new String(strbuf);
}
/**
* Gets the LLLL value from the segment at the location specified by
* aSegmentNumber in the output message as a short value. for sync callout
* request
*
* @param aSegmentNumber
* segment offset
* @returnthe LL value from the specified segment as a short
*/
public int getSegmentLlllValue(int aSegmentNumber) {
return this.parsedOutLlllValIntArray[aSegmentNumber];
}
// -----------------End--------------------------------------
/**
* Gets the ZZ value from the segment at the location specified by
* aSegmentNumber in the output message as a byte array.
*
* @param aSegmentNumber
* segment offset
* @return the ZZ bytes from the specified segment as a byte array
*/
public byte[] getSegmentZz(int aSegmentNumber) {
return this.parsedOutZzByteArray[aSegmentNumber];
}
/**
* Gets the ZZ bytes from the segment at the location specified by
* aSegmentNumber in the output message in hex as a String.
*
* @param aSegmentNumber
* segment offset
* @return the ZZ bytes from the specified segment in hex as a String
*/
public String getSegmentZzAsHexString(int aSegmentNumber) {
byte[] segmentZzByteArray = this.parsedOutZzByteArray[aSegmentNumber];
int z0 = (int) ((segmentZzByteArray[0] & 0xF0) >> 4);
int z1 = (int) (segmentZzByteArray[0] & 0x0F);
int z2 = (int) ((segmentZzByteArray[1] & 0xF0) >> 4);
int z3 = (int) (segmentZzByteArray[1] & 0x0F);
StringBuffer strbuf = new StringBuffer("");
strbuf.append(Integer.toString(z0, 16)); // append high-order nibble of
// 1st byte
strbuf.append(Integer.toString(z1, 16) + " "); // append low-order
// nibble of 1st byte
strbuf.append(Integer.toString(z2, 16)); // append high-order nibble of
// 2nd byte
strbuf.append(Integer.toString(z3, 16)); // append low-order nibble of
// 2nd byte
return new String(strbuf);
}
/**
* Gets the number of segments in this output message as an int value
*
* @return the number of segments in this output message as an
* int
*/
public int getNumberOfSegments() {
return numberOfSegments;
}
/*
* (non-Javadoc) Convert an LL value in a byte array to a short value
*/
/*
* private short getShortFrom2Bytes(byte[] aSegmentData, int llPos) { byte
* highOrderShortNibble; byte lowOrderShortNibble; short ll;
*
* highOrderShortNibble = aSegmentData[llPos]; lowOrderShortNibble =
* aSegmentData[llPos + 1];
*
* ll = highOrderShortNibble; // set top 8 bits ll <<= 8; ll |=
* lowOrderShortNibble; // set bottom 8 bits ll &= 0x7fff; //remove
* propagated sign bits
*
* return ll; }
*
* static final byte[] HEX_CHAR_TABLE = { (byte)'0', (byte)'1', (byte)'2',
* (byte)'3', (byte)'4', (byte)'5', (byte)'6', (byte)'7', (byte)'8',
* (byte)'9', (byte)'A', (byte)'B', (byte)'C', (byte)'D', (byte)'E',
* (byte)'F' };
*
* /* (non-Javadoc) Convert a byte array to a hex string
*/
/*
* private String getHexString(byte[] anIntByteArray) throws
* UnsupportedEncodingException { byte[] hex = new byte[2 *
* anIntByteArray.length]; int index = 0;
*
* for (byte b : anIntByteArray) { int v = b & 0xFF; hex[index++] =
* HEX_CHAR_TABLE[v >>> 4]; hex[index++] = HEX_CHAR_TABLE[v & 0xF]; } return
* new String(hex, "ASCII"); }
*/
/*
* (non-Javadoc)
*
* @see java.lang.Object#toString()
*/
public String toString() {
// int dataposBegin = -1;
// int dataposEnd = -1;
boolean obfuscatePassword = false; // no password in response messages
// so no need to obfuscate
// String codepage = DEFAULT_CODEPAGE_EBCDIC_US;
// return ImsConnectTrace.toHexString(this.getResponseMessage(),
// dataposBegin,
// dataposEnd, this.irmCodepage, codepage,
// ImsConnectTrace.STYLE_OUTPUT_FORMAT);
return myTmInteractionImpl.formatBufferForTracing(this
.getResponseMessage(), obfuscatePassword);
}
// Getter methods
/**
* @return Returns the csmFlag1.
*/
protected byte getCsmFlag1() {
return this.csmFlag1;
}
/**
* @return the csmFlag2
*/
public byte getCsmFlag2() {
return this.csmFlag2;
}
/**
* @return Returns the csmStr.
*/
protected String getCsmStr() {
return this.csmStr;
}
/**
* @return Returns the myImsConnectCodepage.
*/
protected String getMyImsConnectCodepage()
{
return myImsConnectCodepage;
}
/**
* @return the messageIsSingleSegment
*/
protected boolean isMessageIsSingleSegment() {
return messageIsSingleSegment;
}
/**
* @return the messageLength
*/
public int getMessageLength() {
return messageLength;
}
/**
* @return the msgType
*/
protected int getMsgType() {
return msgType;
}
/**
* @return the parsedOutDataByteArray
*/
protected byte[][] getParsedOutDataByteArray() {
return this.parsedOutDataByteArray;
}
/**
* @return the parsedOutLlByteArray
*/
protected byte[][] getParsedOutLlByteArray() {
return this.parsedOutLlByteArray;
}
/**
* @return the parsedOutLlValShortArray
*/
protected short[] getParsedOutLlValShortArray() {
return this.parsedOutLlValShortArray;
}
// ------------------Added for Sync
// callout------------------------------////
/**
* @return the parsedOutLlllByteArray
*/
protected byte[][] getParsedOutLlllByteArray() {
return this.parsedOutLlllByteArray;
}
/**
* @return the parsedOutLlllValIntArray
*/
protected int[] getParsedOutLlllValIntArray() {
return this.parsedOutLlllValIntArray;
}
// ------------End--------------------------------------------------
/**
* @return the parsedOutZzByteArray
*/
protected byte[][] getParsedOutZzByteArray() {
return this.parsedOutZzByteArray;
}
/**
* @return the responseMessage
*/
public byte[] getResponseMessage() {
return this.responseMessage;
}
public String getRSMErrorMsg() {
String hwsrescode = "HWS_RESCODE_";
String rsmErrMsg = ImsConnectErrorMessage.getString(hwsrescode
+ String.valueOf(this.rsmReasonCode));
return rsmErrMsg;
}
/**
* @return Returns the rsmReturnCode.
*/
public int getImsConnectReturnCode() {
return this.rsmReturnCode;
}
/**
* @return Returns the rsmReasonCode.
*/
public int getImsConnectReasonCode() {
return this.rsmReasonCode;
}
/**
* Get mfsModname name property value
*
* @return the mfsModname value returned by the IMS application through IMS
* Connect
*/
public String getMfsModname() {
return this.mfsModname;
}
/**
* Get mfsModname name property value
*
* @return the mfsModname value returned by the IMS application through IMS
* Connect
*/
public String getReturnedClientId() {
return this.clientId;
}
/**
* @return Returns the rsmOtmaSenseCode.
*/
public int getOtmaSenseCode() {
return this.rsmOtmaSenseCode;
}
/**
* @return Returns the rsmOtmaReasonCode.
*/
public byte getOtmaReasonCode() {
return this.rsmOtmaReasonCode;
}
/**
* @return the rsmRacfReturnCode
*/
public int getRacfReturnCode() {
return this.rsmRacfReturnCode;
}
/*
* (non-Javadoc)
*
* @see com.ibm.ims.connect.OutputMessage#getRacfReturnCodeString()
*/
public String getRacfReturnCodeString() {
return racfReturnCodeString;
}
/**
* Get responseIncludesLlll property value
*
* @return a boolean value representing whether the messages passed between
* the IMS Connect API and IMS Connect start with LLLL (true -
* messages targeted for HWSSMPL1 do start with LLLL) or do not
* start with LLLL (messages targeted for HWSSMPL0 do start with
* LLLL)
*/
public boolean isResponseIncludesLlll() {
return this.responseIncludesLlll;
}
/**
* @return Returns the ackNakNeeded property value - used internally only
*/
public boolean isAckNakNeeded() {
if (this.isResponsePropertiesUpdatedAfterResponse())
return ackNakNeeded;
else {
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL))
logger
.finest(" isAckNakNeeded returned the default value, false, since no response has been yet from IMS Connect\n");
return false;
}
}
/**
* @return the responsePropertiesUpdatedAfterResponse
*/
protected boolean isResponsePropertiesUpdatedAfterResponse() {
return this.responsePropertiesUpdatedAfterResponse;
}
/**
* @return Returns the asyncOutputAvailable property value - used internally
* only
*/
public boolean isAsyncOutputAvailable() {
if (this.isAsyncOutputAvailablePropertyUpdated())
return asyncOutputAvailable;
else {
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL))
logger
.finest(" isAsyncOutputAvailable returned the default value, false, since no response has been yet from IMS Connect\n");
return false;
}
}
/**
* @return the responsePropertiesUpdatedAfterResponse
*/
protected boolean isAsyncOutputAvailablePropertyUpdated() {
return this.responsePropertiesUpdatedAfterResponse;
}
/**
* @return Returns the inConversation property value - used internally only
*/
public boolean isInConversation() {
if (this.isResponsePropertiesUpdatedAfterResponse())
return inConversation;
else {
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL))
logger
.finest(" isInConversation returned the default value, false, since no response has been received yet from IMS Connect\n");
return false;
}
}
/**
* @return the responsePropertiesUpdatedAfterResponse
*/
/*
* protected boolean isInConversationPropertyUpdated() { return
* this.inConversationPropertyUpdated; }
*/
/**
* @return the protocolLevel
*/
public byte getProtocolLevel() {
return this.protocolLevel;
}
/**
* @return the protocolLevelAvailable
*/
public boolean isProtocolLevelAvailable() {
return this.protocolLevelAvailable;
}
/**
* @return the segmentIncludesLlzz
*/
protected boolean isIncludeLlzzInSegments() {
return this.includeLlzzInSegments;
}
// Setter methods
/**
* @param csmFlag1
* the csmFlag1 to set
*/
protected void setCsmFlag1(byte csmFlag) {
this.csmFlag1 = csmFlag;
}
/**
* @param csmFlag2
* the csmFlag2 to set
*/
public void setCsmFlag2(byte csmFlag2) {
this.csmFlag2 = csmFlag2;
}
/**
* @param aCsmStr
* The csmStr to set.
*/
protected void setCsmStr(String aCsmStr) {
this.csmStr = aCsmStr;
}
/**
* @param string
*/
protected void setMyImsConnectCodepage(String string) {
if (string == null)
return;
myImsConnectCodepage = string;
}
protected void setMessageLength() throws ImsConnectExecutionException {
int l0Nibble;
int l1Nibble;
int l2Nibble;
int l3Nibble;
if (myTmInteractionImpl.isResponseIncludesLlll()) {
byte[] messageAsByteArray = this.responseMessage;
l0Nibble = messageAsByteArray[0] & 0xff;
l0Nibble <<= 24; // set top 8 bits
l0Nibble = l0Nibble & 0xff000000;
l1Nibble = messageAsByteArray[1] & 0xff;
l1Nibble <<= 16; // set upper middle 8 bits
l1Nibble = l1Nibble & 0x00ff0000;
l2Nibble = messageAsByteArray[2] & 0xff;
l2Nibble <<= 8; // set lower middle 8 bits
l2Nibble = l2Nibble & 0x0000ff00;
l3Nibble = messageAsByteArray[3] & 0xff;
l3Nibble = l3Nibble & 0x000000ff;
this.messageLength = (l3Nibble | l2Nibble | l1Nibble | l0Nibble) & 0x7fffffff; // set
// bottom
// 8
// bits
if (this.messageLength != this.responseMessage.length) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0020E, new Object[] {
this.responseMessage.length, messageLength });
ImsConnectExecutionException e = new ImsConnectExecutionException(
ImsConnectErrorMessage.HWS0020E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in OutputMessage.setMessageLength(). Exception was: "
+ e.toString());
throw e;
}
} else
this.messageLength = this.responseMessage.length;
}
/**
* @param messageLength
* The messageLength to set.
*/
protected void setMessageLength(int aMessageLength) {
this.messageLength = aMessageLength;
}
/**
* @param message
* The message to set.
*/
protected void setResponseMessage(byte[] outMessage) {
this.responseMessage = outMessage;
}
/**
* @param messageIsSingleSegment
* the messageIsSingleSegment to set
*/
protected void setMessageIsSingleSegment(boolean messageIsSingleSegment) {
this.messageIsSingleSegment = messageIsSingleSegment;
}
/**
* @param msgType
* the msgType to set
*/
protected void setMsgType(int msgType) {
this.msgType = msgType;
}
/**
* @param numberOfSegments
* the numberOfSegments to set
*/
protected void setNumberOfSegments(int numberOfSegments) {
this.numberOfSegments = numberOfSegments;
}
/**
* @param anRsmReasonCode
* The rsmReasonCode to set.
*/
protected void setImsConnectReasonCode(int anRsmReasonCode) {
this.rsmReasonCode = anRsmReasonCode;
}
/**
* @param rsmReturnCode
* The rsmReturnCode to set.
*/
protected void setImsConnectReturnCode(int anRsmReturnCode) {
this.rsmReturnCode = anRsmReturnCode;
}
/**
* @param anRsmOtmaSenseCode
* The rsmOtmaSenseCode to set.
*/
protected void setOtmaSenseCode(int anRsmOtmaSenseCode) {
this.rsmOtmaSenseCode = anRsmOtmaSenseCode;
}
/**
* @param rsmOtmaReasonCode
* The rsmOtmaReasonCode to set.
*/
protected void setOtmaReasonCode(byte anRsmOtmaReasonCode) {
this.rsmOtmaReasonCode = anRsmOtmaReasonCode;
}
/**
* @param rsmRacfReturnCode
* the rsmRacfReturnCode to set
*/
public void setRacfReturnCode(int rsmRacfReturnCode) {
this.rsmRacfReturnCode = rsmRacfReturnCode;
}
/**
* @param aRacfReturnCodeString
* the racfReturnCodeString to set
*/
public void setRacfReturnCodeString(String aRacfReturnCodeString) {
this.racfReturnCodeString = aRacfReturnCodeString;
}
/**
* @param ackNakNeeded
* the ackNakNeeded to set
*/
protected void setAckNakNeeded(boolean ackNakNeeded) {
this.ackNakNeeded = ackNakNeeded;
}
/**
* @param aResponsePropertiesUpdatedAfterResponse
* the responsePropertiesUpdatedAfterResponse to set
*/
protected void setResponsePropertiesUpdatedAfterResponse(
boolean aResponsePropertiesUpdatedAfterResponse) {
this.responsePropertiesUpdatedAfterResponse = aResponsePropertiesUpdatedAfterResponse;
}
/**
* @param asyncOutputAvailable
* the asyncOutputAvailable to set
*/
protected void setAsyncOutputAvailable(boolean asyncOutputAvailable) {
this.asyncOutputAvailable = asyncOutputAvailable;
}
/**
* @param asyncOutputAvailablePropertyUpdated
* the asyncOutputAvailablePropertyUpdated to set
*/
/*
* protected void setAsyncOutputAvailablePropertyUpdated( boolean
* asyncOutputAvailablePropertyUpdated) {
* this.asyncOutputAvailablePropertyUpdated =
* asyncOutputAvailablePropertyUpdated; }
*/
/**
* @param inConversation
* the inConversation to set
*/
protected void setInConversation(boolean inConversation) {
this.inConversation = inConversation;
}
/**
* @param inConversationPropertyUpdated
* the inConversationPropertyUpdated to set
*/
/*
* protected void setInConversationPropertyUpdated( boolean
* inConversationPropertyUpdated) { this.inConversationPropertyUpdated =
* inConversationPropertyUpdated; }
*/
/**
* @param protocolLevel
* the protocolLevel to set
*/
public void setProtocolLevel(byte protocolLevel) {
this.protocolLevel = protocolLevel;
}
/**
* @param protocolLevelAvailable
* the protocolLevelAvailable to set
*/
public void setProtocolLevelAvailable(boolean protocolLevelAvailable) {
this.protocolLevelAvailable = protocolLevelAvailable;
}
/**
* @param aSegmentsIncludeLlzz
* the includeLlzzInSegments to set
*/
protected void setIncludeLlzzInSegments(boolean aSegmentsIncludeLlzz) {
this.includeLlzzInSegments = aSegmentsIncludeLlzz;
}
/**
* @return the myType2CommandResponse
*/
public Type2CmdResponse getType2CommandResponse()
throws ImsConnectApiException {
if (this.myType2CommandResponse == null)
this.myType2CommandResponse = new Type2CmdResponse(
myImsConnectCodepage);
else if (this.myType2CommandResponse.isType2CmdRspStale())
this.processType2CmdRsp(this.parsedOutDataByteArray[0]);
return this.myType2CommandResponse;
}
/**
* @param myType2CommandResponse
* the myType2CommandResponse to set
*/
@SuppressWarnings( { "unused", "unused" })
private void setMyType2CommandResponse(Type2CmdResponse type2CommandResponse) {
this.myType2CommandResponse = type2CommandResponse;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy