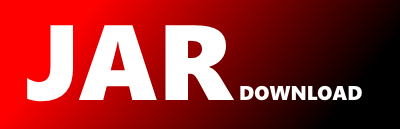
com.ibm.ims.connect.impl.TmInteractionImpl Maven / Gradle / Ivy
Show all versions of IMSConnectAPI Show documentation
/*
* File: TmInteractionImpl.java
* ==========================================================================
* Licensed Material - Property of IBM
*
* IBM Confidential
*
* OCO Source Materials
*
* 5655-TDA
*
* (C) Copyright IBM Corp. 2009,2015 All Rights Reserved.
*
* The source code for this program is not published or
* otherwise divested of its trade secrets, irrespective
* of what has been deposited with the U.S. Copyright
* Office.
*
* US Government Users Restricted Rights - Use, duplication or
* disclosure restricted by GSA ADP Schedule Contract with
* IBM Corp.
* ===========================================================================
*/
package com.ibm.ims.connect.impl;
import java.io.UnsupportedEncodingException;
import java.net.SocketException;
import java.util.logging.Logger;
import com.ibm.ims.connect.ApiProperties;
import com.ibm.ims.connect.Connection;
import com.ibm.ims.connect.ImsConnectApiException;
import com.ibm.ims.connect.ImsConnectErrorMessage;
import com.ibm.ims.connect.ImsConnectExecutionException;
import com.ibm.ims.connect.ImsOtmaExecutionException;
import com.ibm.ims.connect.InputMessage;
import com.ibm.ims.connect.OutputMessage;
import com.ibm.ims.connect.OutputMessageProperties;
import com.ibm.ims.connect.PropertiesFileLoader;
import com.ibm.ims.connect.TmInteraction;
import com.ibm.ims.connect.TmInteractionAttributes;
/**
* This class represents an interaction with IMS Connect to run an IMS
* transaction or invoke an IMS command. TmInteraction is the main API class
* used to execute an interaction with IMS Connect or through IMS Connect with
* IMS.
*
* @author hfung
*/
public final class TmInteractionImpl implements TmInteraction, Cloneable {
@SuppressWarnings("unused")
private static final String copyright = "Licensed Material - Property of IBM "
+ "5655-TDA"
+ "(C) Copyright IBM Corp. 2009,2013 All Rights Reserved. "
+ "US Government Users Restricted Rights - Use, duplication or "
+ "disclosure restricted by GSA ADP Schedule Contract with IBM Corp. ";
private TmInteractionAttributes interAttr = null;
private ConnectionImpl myConnection = null;
private InputMessageImpl internalAckInputMessage;
private InputMessage inputMsg = (InputMessage) new InputMessageImpl(this);
private OutputMessage outputMsg = (OutputMessage) new OutputMessageImpl(
this);
// --------Adding for Kevin Hite - NEED TO BE
// REMOVED-----------------------------------------//
// public ResponseTimeStatistics rts = null;
// --------END OF CODE - NEED TO BE
// REMOVED-----------------------------------------//
// private boolean generateClientID = DEFAULT_USE_CONNECTION_MANAGER;
// Introducing following Booleans for Performance improvements
protected boolean updateIrmDestId = true;
protected boolean updateIrmId = true;
protected boolean updateIrmLTerm = true;
protected boolean updateIrmRacfApplName = true;
protected boolean updateIrmRacfGroupName = true;
protected boolean updateIrmRacfPassword = true;
protected boolean updateIrmRacfUserId = true;
protected boolean updateIrmRerouteName = true;
protected boolean updateIrmTagAdapter = false;
protected boolean updateIrmTagMap = true;
protected boolean updateIrmTrancode = true;
protected boolean updateIrmTrancodeInData = true;
protected boolean updateIrmInputModName = true;
protected boolean updateIrmInteractionType = true;
protected boolean copyInputMessageForInternalAck = true;
// Input properties
private byte architectureLevel = DEFAULT_ARCH_LEVEL; // Used internally only
private byte ackNakProvider = DEFAULT_ACK_NAK_PROVIDER;
private int clientType = DEFAULT_CLIENT_TYPE; // may be used internally only
// when API used for more
// than ICON RYO
private byte commitMode = DEFAULT_COMMIT_MODE;
private boolean cm0IgnorePurge = DEFAULT_CM0_IGNORE_PURGE;
private boolean cancelClientId = DEFAULT_CANCEL_CLIENTID;
private boolean returnClientId = DEFAULT_RETURN_CLIENTID;
private boolean generateClientIdWhenDuplicate = DEFAULT_IMS_CONNECT_GENERATE_CLIENTID;
private boolean inputMessageDataSegmentsIncludeLlzzAndTrancode = DEFAULT_INPUT_MESSAGE_DATA_SEGMENTS_INCLUDE_LLZZ_AND_TRANCODE;
// private boolean savedInputMessageDataSegmentsIncludeLlzzAndTrancode =
// inputMessageDataSegmentsIncludeLlzzAndTrancode;
private String imsConnectUserMessageExitIdentifier = DEFAULT_IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER;
private int inputMessageOptions = DEFAULT_INPUT_MESSAGE_OPTIONS;
private String interactionTypeDescription = DEFAULT_INTERACTION_TYPE_DESC;
private String ltermOverrideName = DEFAULT_LTERM_OVERRIDE_NAME;
private boolean returnMfsModname = DEFAULT_RETURN_MFS_MODNAME;
private byte syncLevel = DEFAULT_SYNC_LEVEL;
// IMS Connect XML properties
private String xmlAdapterName = DEFAULT_XML_ADAPTER_NAME;
private String xmlConverterName = DEFAULT_XML_CONVERTER_NAME;
// private byte xmlAdapterType = DEFAULT_XML_ADAPTER_TYPE;
private byte xmlMessageType = DEFAULT_XML_MESSAGE_TYPE;
// Message encoding properties
private String imsConnectCodepage = DEFAULT_IMS_CONNECT_CODEPAGE; // ?????
private byte imsConnectUnicodeEncodingSchema = DEFAULT_IMS_CONNECT_UNICODE_ENCODING_SCHEMA;
private byte imsConnectUnicodeUsage = DEFAULT_IMS_CONNECT_UNICODE_USAGE;
// ResumeTpipe and Message routing properties
private boolean purgeUndeliverableOutput = DEFAULT_PURGE_UNDELIVERABLE_OUTPUT;
private boolean rerouteUndeliverableOutput = DEFAULT_REROUTE_UNDELIVERABLE_OUTPUT;
private String rerouteName = DEFAULT_REROUTE_NAME;
private String resumeTpipeAlternateClientId = DEFAULT_RESUMETPIPE_ALTERNATE_CLIENTID;
private int resumeTpipeProcessing = DEFAULT_RESUME_TPIPE_PROCESSING;
// Callout properties
private String calloutRequestNakProcessing = ApiProperties.DEFAULT_CALLOUT_REQUEST_NAK_PROCESSING;
private String calloutResponseMessageType = ApiProperties.DEFAULT_CALLOUT_RESPONSE_MESSAGE_TYPE;
private byte resumeTPipeRetrievalType = ApiProperties.DEFAULT_RESUME_TPIPE_RETRIEVAL_TYPE;
private short nakReasonCode = ApiProperties.IRM_NAK_REASONCODE_DEFAULT;
private String inputModName = DEFAULT_INPUT_MOD_NAME;
private byte[] correlatorTkn = CORRELATOR_TOKEN_DEFAULT;
// Security properties
private String racfApplName = DEFAULT_RACF_APPL_NAME;
private String racfGroupName = DEFAULT_RACF_GROUP_NAME;
private String racfPassword = DEFAULT_RACF_PASSWORD;
private String racfUserId = DEFAULT_RACF_USERID;
// Target IMS properties
private String imsDatastoreName = DEFAULT_IMS_DATASTORE_NAME;
private String trancode = DEFAULT_TRANCODE;
// Timeout properties
private int interactionTimeout = DEFAULT_INTERACTION_TIMEOUT;
private int imsConnectTimeout = DEFAULT_IMS_CONNECT_TIMEOUT;
private int imsConnectConvertedTimeout = DEFAULT_CONVERTED_IMS_CONNECT_TIMEOUT;
private int imsConnectTimeoutIndex = DEFAULT_IMS_CONNECT_TIMEOUT_INDEX;
private boolean otmaTransactionExpiration = DEFAULT_OTMA_TRANSACTION_EXPIRATION;
private boolean useCM0AckNoWait = DEFAULT_USE_CM0_ACK_NOWAIT;
private boolean cM0AckNoWaitCanBeUsed = false; // true after a message has
// been received containing
// protocol level available
// and protocol level 2 or
// greater
private boolean returnDFS2082AfterCM0SendRecvNoResponse = false;
// Tracing properties
// private String traceFileName = DEFAULT_TRACE_FILE_NAME;
// private Level traceLevel = DEFAULT_TRACE_LEVEL;
// Response Properties
private boolean ackNakNeeded;
private boolean asyncOutputAvailable;
private boolean inConversation;
private boolean protocolLevelAvailable; // set in this.processCsmFlag()
private byte protocolLevel; // set in this.processCsmFlag()
private int imsConnectReturnCode;
private int imsConnectReasonCode;
private String mfsModname;
private int otmaSenseCode;
private int otmaReasonCode;
private int racfReturnCode;
private String racfReturnCodeString;
private boolean responseIncludesLlll;
// Other properties
byte[] inputMsgBytes = null; // byte array returned from getBytes and sent
// to IMS Connect (includes LLLL + IRM +
// LLZZ + trancode + data as required)
byte[] outputMsgBytes = null; // raw bytes received from IMS Connect
byte[] anEmptyByteArray = {}; // an empty byte array for use as input data
// for interactions that do not take input
// data (e.g. ACK, NAK, RESUMETPIPE)
private Logger logger;
private byte csmFlag1;
private byte csmFlag2;
private SavedResponseValues mySavedResponseValues = null;
private boolean recvAfterResumeTpipe = false;
private boolean use2DimensionalByteArray;
private int cursor;
private int numberOfSegments;
private boolean includeLlllInOutputMessages = true;
private boolean includeLlzzInOutputMessages = true;
private boolean ackNakNeededPropertyUpdated = false;
private boolean responsePropertiesUpdatedAfterResponse = false;
// private boolean inConversationPropertyUpdated = false;
protected boolean rebuildMessage = true;
private String allValidInteractionTypeDescriptionsString = " ACK CANCELTIMER ENDCONVERSATION RECEIVE SENDONLYACK NAK SENDONLYXCFORDDLV RESUMETPIPE SENDONLY SENDRECV SENDONLYCALLOUTRESPONSE SENDONLYACKCALLOUTRESPONSE TYPE2CMD";
// ------------------Added for Sync
// callout------------------------------////
private String allValidCalloutRequestNakProcessingsString = " DISCARDREQUESTCONTINUERESUMETPIPE DISCARDREQUESTENDRESUMETPIPE REQUEUEREQUESTENDRESUMETPIPE";
private String allValidCalloutResponseMessageTypesString = " CALLOUTRESPONSEMESSAGE CALLOUTERRORMESSAGE";
private class SavedResponseValues {
String tmpIntTypeDesc;
int tmpImsConnectTimeout;
InputMessageImpl tmpInputMsg;
OutputMessageImpl tmpOutputMsg;
byte[] tmpOutputMsgBytes;
int tmpImsConnectReturnCode;
int tmpImsConnectReasonCode;
int tmpOtmaSenseCode;
boolean tmpAsyncOutputAvailable;
boolean tmpInConversation;
byte tmpProtocolLevel;
boolean tmpUseCM0AckNoWait;
boolean tmpResponsePropertiesSetAfterResponse;
SavedResponseValues(TmInteractionImpl aTMInteractionImpl)
throws ImsConnectApiException, UnsupportedEncodingException,
CloneNotSupportedException {
setTmpIntTypeDesc(aTMInteractionImpl
.getInteractionTypeDescription());
tmpImsConnectTimeout = ((TmInteractionImpl) aTMInteractionImpl)
.getImsConnectTimeout();
InputMessageImpl myTmpInMsg = new InputMessageImpl(
aTMInteractionImpl);
myTmpInMsg.copyInputMessage((InputMessageImpl) aTMInteractionImpl
.getInputMessage());
setTmpInputMsg(myTmpInMsg);
OutputMessageImpl myTmpOutMsg = new OutputMessageImpl(
aTMInteractionImpl);
myTmpOutMsg
.copyOutputMessage((OutputMessageImpl) aTMInteractionImpl
.getOutputMessage());
setTmpOutputMsg(myTmpOutMsg);
setTmpOutputMsgBytes(aTMInteractionImpl.outputMsgBytes);
setTmpImsConnectReturnCode(aTMInteractionImpl
.getImsConnectReturnCode());
setTmpImsConnectReasonCode(aTMInteractionImpl
.getImsConnectReasonCode());
setTmpOtmaSenseCode(aTMInteractionImpl.getOtmaSenseCode());
setTmpAsyncOutputAvailable(aTMInteractionImpl
.isAsyncOutputAvailable());
setTmpInConversation(aTMInteractionImpl.inConversation);
setTmpProtocolLevel(aTMInteractionImpl.protocolLevel);
setTmpUseCM0AckNoWait(aTMInteractionImpl.useCM0AckNoWait);
setTmpResponsePropertiesSetAfterResponse(aTMInteractionImpl.responsePropertiesUpdatedAfterResponse);
}
/**
* @return the tmpImsConnectTimeout
*/
protected int getTmpImsConnectTimeout() {
return this.tmpImsConnectTimeout;
}
/**
* @param tmpImsConnectTimeout
* the tmpImsConnectTimeout to set
*/
protected void setTmpImsConnectTimeout(int tmpImsConnectTimeout) {
this.tmpImsConnectTimeout = tmpImsConnectTimeout;
}
/**
* @return the tmpImsConnectReasonCode
*/
protected int getTmpImsConnectReasonCode() {
return tmpImsConnectReasonCode;
}
/**
* @return the tmpImsConnectReturnCode
*/
protected int getTmpImsConnectReturnCode() {
return tmpImsConnectReturnCode;
}
/**
* @return the tmpIntTypeDesc
*/
protected String getTmpIntTypeDesc() {
return tmpIntTypeDesc;
}
/**
* @return the tmpOtmaSenseCode
*/
protected int getTmpOtmaSenseCode() {
return tmpOtmaSenseCode;
}
/**
* @return the tmpInputMsg
*/
protected InputMessageImpl getTmpInputMsg() {
return tmpInputMsg;
}
/**
* @return the tmpOutputMsg
*/
protected OutputMessageImpl getTmpOutputMsg() {
return tmpOutputMsg;
}
/**
* @return the tmpAsyncOutputAvailable
*/
protected boolean isTmpAsyncOutputAvailable() {
return tmpAsyncOutputAvailable;
}
/**
* @return the tmpInConversation
*/
protected boolean isTmpInConversation() {
return tmpInConversation;
}
/**
* @return the tmpProtocolLevel
*/
public byte getTmpProtocolLevel() {
return this.tmpProtocolLevel;
}
/**
* @return the tmpUseCM0AckNoWait
*/
public boolean isTmpUseCM0AckNoWait() {
return this.tmpUseCM0AckNoWait;
}
/**
* @return the tmpResponsePropertiesSetAfterResponse
*/
protected boolean isTmpResponsePropertiesSetAfterResponse() {
return this.tmpResponsePropertiesSetAfterResponse;
}
/**
* @@return the tmpOutputMsgBytes
*/
protected byte[] getTmpOutputMsgBytes() {
return this.tmpOutputMsgBytes;
}
/**
* @param aTmpProtocolLevel
* the tmpProtocolLevel to set
*/
public void setTmpProtocolLevel(byte aTmpProtocolLevel) {
this.tmpProtocolLevel = aTmpProtocolLevel;
}
/**
* @param aTmpUseCM0AckNoWait
* the tmpUseCM0AckNoWait to set
*/
public void setTmpUseCM0AckNoWait(boolean aTmpUseCM0AckNoWait) {
this.tmpUseCM0AckNoWait = aTmpUseCM0AckNoWait;
}
/**
* @param aTmpAsyncOutputAvailable
* the tmpAsyncOutputAvailable to set
*/
protected void setTmpAsyncOutputAvailable(
boolean aTmpAsyncOutputAvailable) {
this.tmpAsyncOutputAvailable = aTmpAsyncOutputAvailable;
}
/**
* @param aTmpImsConnectReasonCode
* the tmpImsConnectReasonCode to set
*/
protected void setTmpImsConnectReasonCode(int aTmpImsConnectReasonCode) {
this.tmpImsConnectReasonCode = aTmpImsConnectReasonCode;
}
/**
* @param aTmpImsConnectReturnCode
* the tmpImsConnectReturnCode to set
*/
protected void setTmpImsConnectReturnCode(int aTmpImsConnectReturnCode) {
this.tmpImsConnectReturnCode = aTmpImsConnectReturnCode;
}
/**
* @param aTmpInConversation
* the tmpInConversation to set
*/
protected void setTmpInConversation(boolean aTmpInConversation) {
this.tmpInConversation = aTmpInConversation;
}
/**
* @param aTmpResponsePropertiesSetAfterResponse
* the tmpResponsePropertiesSetAfterResponse to set
*/
protected void setTmpResponsePropertiesSetAfterResponse(
boolean aTmpInConversationPropertiesUpdated) {
this.tmpResponsePropertiesSetAfterResponse = aTmpInConversationPropertiesUpdated;
}
/**
* @param aTmpIntTypeDesc
* the tmpIntTypeDesc to set
*/
protected void setTmpIntTypeDesc(String aTmpIntTypeDesc) {
this.tmpIntTypeDesc = aTmpIntTypeDesc;
}
/**
* @param aTmpOtmaSenseCode
* the tmpOtmaSenseCode to set
*/
protected void setTmpOtmaSenseCode(int aTmpOtmaSenseCode) {
this.tmpOtmaSenseCode = aTmpOtmaSenseCode;
}
/**
* @param aTmpInputMsg
* the tmpInputMsg to set
*/
protected void setTmpInputMsg(InputMessageImpl aTmpInputMsg) {
this.tmpInputMsg = aTmpInputMsg;
}
/**
* @param tmpOutputMsg
* the tmpOutputMsg to set
*/
protected void setTmpOutputMsg(OutputMessageImpl aTmpOutputMsg) {
this.tmpOutputMsg = aTmpOutputMsg;
}
/**
* @param tmpOutputMsgBytes
* the tmpOutputMsgBytes to set
*/
protected void setTmpOutputMsgBytes(byte[] aTmpOutputMsgBytes) {
this.tmpOutputMsgBytes = aTmpOutputMsgBytes;
}
}
/*
* private ApiLoggingConfiguration loggingConfig = new
* ApiLoggingConfiguration();
*/
/**
* This class represents an interaction with IMS Connect invoked through the
* IMS Connect API to run an IMS transaction or execute an IMS command.
*
* @author hfung
*/
public TmInteractionImpl() throws ImsConnectApiException {
super();
logger = Logger.getLogger("com.ibm.ims.connect");
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("<-> TmInteractionImpl()");
}
/**
* This class represents an interaction with IMS Connect invoked through the
* IMS Connect API to run an IMS transaction or execute an IMS command.
*
* @author hfung
*/
public TmInteractionImpl(TmInteractionAttributes tmInterAttr)
throws ImsConnectApiException {
logger = Logger.getLogger("com.ibm.ims.connect");
new TmInteractionImpl();
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("--> TmInteractionImpl(TmInteractionAttributes)");
interAttr = tmInterAttr;
// xmlAdapterType = tmInterAttr.getXmlAdapterType();
ackNakProvider = tmInterAttr.getAckNakProvider();
commitMode = tmInterAttr.getCommitMode();
syncLevel = tmInterAttr.getSyncLevel();
interactionTypeDescription = tmInterAttr
.getInteractionTypeDescription();
imsDatastoreName = tmInterAttr.getImsDatastoreName();
racfUserId = tmInterAttr.getRacfUserId();
racfPassword = tmInterAttr.getRacfPassword();
racfGroupName = tmInterAttr.getRacfGroupName();
racfApplName = tmInterAttr.getRacfApplName();
ltermOverrideName = tmInterAttr.getLtermOverrideName();
// xmlAdapterName = tmInterAttr.getXmlAdapterName();
imsConnectUserMessageExitIdentifier = tmInterAttr
.getImsConnectUserMessageExitIdentifier();
inputMessageDataSegmentsIncludeLlzzAndTrancode = tmInterAttr
.isInputMessageDataSegmentsIncludeLlzzAndTrancode();
responseIncludesLlll = tmInterAttr.isResponseIncludesLlll();
trancode = tmInterAttr.getTrancode();
// clientType = tmInterAttr.getClientType();
purgeUndeliverableOutput = tmInterAttr.isPurgeUndeliverableOutput();
rerouteUndeliverableOutput = tmInterAttr.isRerouteUndeliverableOutput();
rerouteName = tmInterAttr.getRerouteName();
resumeTpipeAlternateClientId = tmInterAttr
.getResumeTpipeAlternateClientId(); // himakar 2/26/07 Callout
imsConnectTimeout = tmInterAttr.getImsConnectTimeout();
// imsConnectConvertedTimeout =
// tmInterAttr.getImsConnectConvertedTimeout();
// imsConnectTimeoutIndex = tmInterAttr.getImsConnectTimeoutIndex();
otmaTransactionExpiration = tmInterAttr.isOtmaTransactionExpiration();
imsConnectCodepage = tmInterAttr.getImsConnectCodepage();
resumeTpipeProcessing =tmInterAttr.getResumeTpipeProcessing();
interactionTimeout = tmInterAttr.getInteractionTimeout();
useCM0AckNoWait = tmInterAttr.isCm0IgnorePurge();
cancelClientId = tmInterAttr.isCancelClientId();
returnClientId = tmInterAttr.isReturnClientId();
generateClientIdWhenDuplicate = tmInterAttr.isGenerateClientIdWhenDuplicate();
calloutRequestNakProcessing = tmInterAttr.getCalloutRequestNakProcessing();
calloutResponseMessageType = tmInterAttr.getCalloutResponseMessageType();
resumeTPipeRetrievalType = tmInterAttr.getResumeTpipeRetrievalType();
nakReasonCode = tmInterAttr.getNakReasonCode();
returnMfsModname = tmInterAttr.isReturnMfsModname();
correlatorTkn = tmInterAttr.getCorrelatorToken();
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("<-- TmInteractionImpl(TmInteractionAttributes)");
}
/**
* The execute method is used to execute a complete interaction with IMS
* Connect which runs an IMS transaction or executes an IMS command. This
* method encompasses the connect() and send()/receive()/ sendReceive()
* functions in a single call. The execute method uses the parent
* TmInteraction's InputMessage object which must be configured prior to
* invoking the execute method. The response will be used to populate the
* OutputMessage object which is also a member of the parent TmInteraction
* object.
*/
public void execute() throws ImsConnectApiException,
ImsConnectExecutionException, SocketException, Exception {
boolean suppressLogging = false;
byte[] inputData = null;
// build of input message moved to just before each send call so that it
// never needs to be rebuilt
// if(this.interactionTypeDescription != INTERACTION_TYPE_DESC_RECEIVE)
// inputData =
// ((InputMessageImpl)(this.inputMsg)).buildInputMessageByteArray(suppressLogging);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("--> TmInteractionImpl.execute(byte[])");
/*
* if(this.isInConversation() && (this.interactionTypeDescription !=
* INTERACTION_TYPE_DESC_ACK)) { //
* ((InputMessageImpl)(this.inputMsg)).setInputMessageData(anInputData);
* }
*/
// System.out.println("code page =" + this.getImsConnectCodepage());
((OutputMessageImpl) (this.outputMsg)).reset(this);
// System.out.println("code page =" + this.getImsConnectCodepage());
myConnection.setInteractionTimeout(this.interactionTimeout);
if (!this.myConnection.isConnected())
this.myConnection.connect();
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL))
logger.finest(" TmInteractionImpl.execute(byte[]) - processing "
+ this.interactionTypeDescription + " interaction");
if ((this.getInteractionTypeDescription() == INTERACTION_TYPE_DESC_RESUMETPIPE)
&& (this.getAckNakProvider() == ApiProperties.API_INTERNAL_ACK)
&& ((this.getResumeTpipeProcessing() == ApiProperties.RESUME_TPIPE_AUTO) || (this
.getResumeTpipeProcessing() == ApiProperties.RESUME_TPIPE_NOAUTO))) {
String errMsg = ImsConnectErrorMessage
.getString(ImsConnectErrorMessage.HWS0015E);
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0015E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in TmInteraction.execute(byte[]). Exception caught was: "
+ e.toString());
throw e;
} else if ((this.inConversation)
&& ((this.interactionTypeDescription == INTERACTION_TYPE_DESC_RESUMETPIPE)
|| (this.interactionTypeDescription == INTERACTION_TYPE_DESC_SENDONLY)
|| (this.interactionTypeDescription == INTERACTION_TYPE_DESC_SENDONLYACK)
|| (this.interactionTypeDescription == INTERACTION_TYPE_DESC_SENDONLYXCFORDEREDDELIVERY))) {
String errMsg = ImsConnectErrorMessage
.getString(ImsConnectErrorMessage.HWS0016E);
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0016E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in TmInteraction.execute(byte[]). Exception caught was: "
+ e.toString());
throw e;
}
if ((this.ackNakNeeded && (ackNakProvider == CLIENT_ACK_NAK))
&& ((this.interactionTypeDescription != INTERACTION_TYPE_DESC_ACK) && (this.interactionTypeDescription != INTERACTION_TYPE_DESC_NAK))) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0045E,
new Object[] { this.interactionTypeDescription });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0045E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in TmInteraction.execute(byte[]). Exception caught was: "
+ e.toString());
throw e;
}
if (((this.interactionTypeDescription == INTERACTION_TYPE_DESC_SENDRECV)
||this.interactionTypeDescription == INTERACTION_TYPE_DESC_RESUMETPIPE)
|| (this.interactionTypeDescription == INTERACTION_TYPE_DESC_SENDONLYACK_CALLOUT_RESPONSE)
|| (this.interactionTypeDescription == INTERACTION_TYPE_DESC_TYPE2_COMMAND)
|| (this.interactionTypeDescription == INTERACTION_TYPE_DESC_NAK)
|| (this.interactionTypeDescription == INTERACTION_TYPE_DESC_SENDONLYACK)
|| (this.interactionTypeDescription == INTERACTION_TYPE_DESC_CANCELTIMER))
{
if (this.interactionTypeDescription == INTERACTION_TYPE_DESC_TYPE2_COMMAND)
{
if (this.imsConnectUserMessageExitIdentifier != IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSCSLO1
&& this.imsConnectUserMessageExitIdentifier != IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSCSLO0)
this.imsConnectUserMessageExitIdentifier = DEFAULT_IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_TYPE2CMD;
this.outputMsg.getType2CommandResponse().reset(
imsConnectCodepage);
}
inputData = ((InputMessageImpl) (this.inputMsg))
.buildInputMessageByteArray(suppressLogging);
this.myConnection.send(inputData);
this.outputMsgBytes = this.myConnection.receive();
((OutputMessageImpl) (this.outputMsg))
.setResponseMessage(outputMsgBytes);
this.processOutputMessage();
} else if (((this.interactionTypeDescription == INTERACTION_TYPE_DESC_ACK) && (this.inConversation))
|| (this.interactionTypeDescription == INTERACTION_TYPE_DESC_SENDONLY)
|| (this.interactionTypeDescription == INTERACTION_TYPE_DESC_SENDONLY_CALLOUT_RESPONSE)
|| (this.interactionTypeDescription == INTERACTION_TYPE_DESC_SENDONLYXCFORDEREDDELIVERY)
|| (this.interactionTypeDescription == INTERACTION_TYPE_DESC_ENDCONVERSATION)) {
inputData = ((InputMessageImpl) (this.inputMsg))
.buildInputMessageByteArray(suppressLogging);
this.myConnection.send(inputData);
// zero out response since there was no response to this interaction
this.imsConnectReturnCode = 0;
this.imsConnectReasonCode = 0;
this.otmaSenseCode = 0;
this.setAckNakNeeded(false);
if (this.interactionTypeDescription == INTERACTION_TYPE_DESC_ENDCONVERSATION)
this.setInConversation(false); // we have to set inConversation
// state to false since there is
// no response to an
// ENDCONVERSATION interaction
// this.setAsyncOutputAvailable(false); // retain previous
// asyncOutputAvailable state
} else if (this.interactionTypeDescription == INTERACTION_TYPE_DESC_ACK) {
if (this.cM0AckNoWaitCanBeUsed) {
this
.setImsConnectTimeout(ApiProperties.IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_NOWAIT_VALUE);
inputData = ((InputMessageImpl) (this.inputMsg))
.buildInputMessageByteArray(suppressLogging);
this.myConnection.send(inputData);
// zero out response since there was no response to this
// interaction
this.imsConnectReturnCode = 0;
this.imsConnectReasonCode = 0;
this.otmaSenseCode = 0;
this.setAckNakNeeded(false);
if (this.interactionTypeDescription == INTERACTION_TYPE_DESC_ENDCONVERSATION)
this.setInConversation(false); // we have to set
// inConversation state to
// false since there is no
// response to an
// ENDCONVERSATION
// interaction
// this.setAsyncOutputAvailable(false); // retain previous
// asyncOutputAvailable state
} else {
if ((this.useCM0AckNoWait)
&& (!(this.protocolLevelAvailable) || (this.protocolLevel != 0x02))
&& (logger
.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL))) {
logger
.finest(" The useCm0AckNoWait value of true was ignored because CM0 ACK nowait is "
+ "not supported in the target IMS Connect. The API will send in the ACK "
+ "message using the configured imsConnectTimeout value and wait that "
+ "length of time for the timeout response to be returned by IMS Connect.");
}
inputData = ((InputMessageImpl) (this.inputMsg))
.buildInputMessageByteArray(suppressLogging);
this.myConnection.send(inputData);
outputMsgBytes = this.myConnection.receive();
((OutputMessageImpl) (this.outputMsg))
.setResponseMessage(outputMsgBytes);
this.processOutputMessage();
}
} else if (this.interactionTypeDescription
.equals(INTERACTION_TYPE_DESC_RECEIVE)) {
outputMsgBytes = this.myConnection.receive();
((OutputMessageImpl) (this.outputMsg))
.setResponseMessage(outputMsgBytes);
this.processOutputMessage();
}
if (((this.myConnection.getSocketType() == ApiProperties.SOCKET_TYPE_TRANSACTION)
&& (!this.inConversation) && (!this.ackNakNeeded))
|| (this.getInteractionTypeDescription() == INTERACTION_TYPE_DESC_CANCELTIMER))
this.myConnection.disconnect();
// process output received from IMS Connect
if ((this.imsConnectReturnCode == 0)
|| // successful interaction return code
((this.commitMode == ApiProperties.COMMIT_MODE_1) && // successful
// CM1 deallocate commit return and reason code
((this.imsConnectReturnCode == 4) && (this.imsConnectReasonCode == 97)))
|| (((this.imsConnectReturnCode == 32) || // timeout return code
(this.imsConnectReturnCode == 36) || // timeout return
// code
(this.imsConnectReturnCode == 40)) && // timeout return code
((this.getInteractionTypeDescription() == INTERACTION_TYPE_DESC_ACK)
|| // ACK or
(this.getInteractionTypeDescription() == INTERACTION_TYPE_DESC_NAK) || // NAK
// or
(this.recvAfterResumeTpipe == true)))) // receive after
// resumeTpipe
{
if (this.ackNakNeeded && (ackNakProvider == API_INTERNAL_ACK)) {
mySavedResponseValues = new SavedResponseValues(this);
this.sendInternalAck();
this
.restoreResponseValuesAfterInternalAck(mySavedResponseValues);
if ((this.myConnection.getSocketType() == ApiProperties.SOCKET_TYPE_TRANSACTION)
&& (!this.inConversation))
this.myConnection.disconnect();
}
// if ((this.commitMode == ApiProperties.COMMIT_MODE_1) && // successful
// // CM1 deallocate commit return and reason code
// ((this.imsConnectReturnCode == 4) && (this.imsConnectReasonCode == 97))&&
// (this.myConnection.getSocketType() == ApiProperties.SOCKET_TYPE_TRANSACTION)) {
// this.myConnection.disconnect();
// }
} else if ((this.imsConnectReturnCode == 8)
&& (this.imsConnectReasonCode == 40)) {
StringBuffer racfRetCodeBuf = new StringBuffer("RACF_RETCODE_");
racfRetCodeBuf.append(racfReturnCode);
String key = racfRetCodeBuf.toString();
try {
this.setRacfReturnCodeString(ImsConnectErrorMessage.getString(
(String) ImsConnectErrorMessage.class.getField(key)
.get(null), new Object[] {/*
* racfUserId,
* racfGroupName
*/}));
((OutputMessageImpl) this.outputMsg)
.setRacfReturnCodeString(this.getRacfReturnCodeString());
} catch (Exception e) {
// Should not occur in production environments since we would
// only
// be dealing with known exceptions
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in TmInteraction.execute(byte[]) - unknown RACF return code. Exception caught was: "
+ e.toString());
// don't rethrow exception since we'll just use null for the
// reason code meaning string;
racfReturnCodeString = "";
((OutputMessageImpl) this.outputMsg)
.setRacfReturnCodeString("");
}
this.myConnection.disconnect(); // disconnect from our end because
// IMS Connect has disconnected
// (always disconnects for RC8)
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0043E, new Object[] {
new Integer(this.imsConnectReturnCode),
new Integer(this.imsConnectReasonCode),
new Integer(this.racfReturnCode),
this.racfReturnCodeString });
ImsConnectExecutionException e1 = new ImsConnectExecutionException(
ImsConnectErrorMessage.HWS0043E, errMsg,
this.imsConnectReturnCode, this.imsConnectReasonCode,
this.racfReturnCode, racfReturnCodeString);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.execute(byte[]). Exception thrown was: \n"
+ e1.toString());
throw e1;
} else if ((this.imsConnectReturnCode == 8)
&& (this.imsConnectReasonCode == 59)
&& (this.getInteractionTypeDescription() == INTERACTION_TYPE_DESC_CANCELTIMER)) {
this.myConnection.disconnect();
} else if (this.imsConnectReturnCode == 12) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0004E, new Object[] {
new Integer(imsConnectReturnCode),
new Integer(otmaSenseCode) });
ImsOtmaExecutionException e2 = new ImsOtmaExecutionException(
ImsConnectErrorMessage.HWS0004E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in TmInteraction.execute(byte[]). Exception caught was: "
+ e2.toString());
this.myConnection.disconnect();
throw e2;
} else if (this.imsConnectReturnCode == 16) {
ImsOtmaExecutionException e3;
if (this.otmaSenseCode != 26) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0004E, new Object[] {
new Integer(imsConnectReturnCode),
new Integer(otmaSenseCode) });
e3 = new ImsOtmaExecutionException(
ImsConnectErrorMessage.HWS0004E, errMsg);
} else {
StringBuffer rsBuf = new StringBuffer("OTMA_RESCODE_");
rsBuf.append(otmaReasonCode);
String key = rsBuf.toString();
String rsValue = "";
try {
rsValue = ImsConnectErrorMessage
.getString((String) ImsConnectErrorMessage.class
.getField(key).get(null));
} catch (Exception e3a) {
rsValue = ""; // ensure that rsValue is null string since
// the OTMA reason code returned is not
// recognized by the API
}
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0005E, new Object[] {
new Integer(imsConnectReturnCode),
new Integer(otmaSenseCode),
new Integer(otmaReasonCode), rsValue });
e3 = new ImsOtmaExecutionException(
ImsConnectErrorMessage.HWS0005E, errMsg);
}
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in TmInteraction.execute(byte[]). Exception caught was: "
+ e3.toString());
this.myConnection.disconnect();
throw e3;
} else if (((this.imsConnectReturnCode == 32) || (this.imsConnectReturnCode == 40))
&& (this.imsConnectReasonCode != 0)) {
int convertedImsConnectReasonCode = this.imsConnectReasonCode;
String convertedImsConnectReasonCodeString = "";
if ((this.imsConnectReasonCode > 0)
&& (this.imsConnectReasonCode <= 25)) // (dec 25 = 0x19 ->
// 10 - 250
// milliseconds)
{
convertedImsConnectReasonCode = this.imsConnectReasonCode * 10;
convertedImsConnectReasonCodeString = Integer
.toString(convertedImsConnectReasonCode)
+ " milliseconds";
} else if ((this.imsConnectReasonCode > 25)
&& (this.imsConnectReasonCode <= 39)) // (dec 39 = 0x27 ->
// 300 - 950
// milliseconds)
{
convertedImsConnectReasonCode = ((this.imsConnectReasonCode - 25) * 50) + 250;
convertedImsConnectReasonCodeString = Integer
.toString(convertedImsConnectReasonCode)
+ " milliseconds";
} else if ((this.imsConnectReasonCode > 39)
&& (this.imsConnectReasonCode <= 99)) // (dec 99 = 0x63 -> 1
// - 60 seconds)
{
convertedImsConnectReasonCode = ((this.imsConnectReasonCode - 40) * 1000) + 1000;
convertedImsConnectReasonCodeString = Integer
.toString(convertedImsConnectReasonCode / 1000)
+ " seconds";
} else if ((this.imsConnectReasonCode > 99)
&& (this.imsConnectReasonCode <= 158)) // (dec 158 = 0x9E ->
// 2 - 60 minutes)
{
convertedImsConnectReasonCode = ((this.imsConnectReasonCode - 99) * 60000) + 60000;
convertedImsConnectReasonCodeString = Integer
.toString(convertedImsConnectReasonCode / 60000)
+ " minutes";
}
ImsConnectExecutionException e4;
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0011E, new Object[] {
new Integer(this.imsConnectReturnCode),
new Integer(this.imsConnectReasonCode),
convertedImsConnectReasonCodeString });
e4 = new ImsConnectExecutionException(
ImsConnectErrorMessage.HWS0011E, errMsg);
e4.setReturnCode(this.imsConnectReturnCode);
e4.setReasonCode(this.imsConnectReasonCode);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.execute(byte[]). Exception thrown was: "
+ e4.toString());
throw e4;
} else if ((this.imsConnectReturnCode == 36)
|| ((this.imsConnectReturnCode == 32) || (this.imsConnectReturnCode == 40))
&& (this.imsConnectReasonCode == 0)) {
ImsConnectExecutionException e5;
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0012E,
new Object[] { new Integer(this.imsConnectReturnCode) });
e5 = new ImsConnectExecutionException(
ImsConnectErrorMessage.HWS0012E, errMsg);
e5.setReturnCode(this.imsConnectReturnCode);
e5.setReasonCode(0);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.execute(byte[]). Exception thrown was: "
+ e5.toString());
throw e5;
} else {
StringBuffer rsBuf = new StringBuffer("HWS_RESCODE_");
rsBuf.append(imsConnectReasonCode);
String key = rsBuf.toString();
String rsValue = null;
try {
if (imsConnectReasonCode == 14)
rsValue = ImsConnectErrorMessage
.getString((String) ImsConnectErrorMessage.class
.getField(key).get(null),
new Object[] { xmlAdapterName });
else if (imsConnectReasonCode == 40)
rsValue = ImsConnectErrorMessage.getString(
(String) ImsConnectErrorMessage.class.getField(key)
.get(null), new Object[] { racfUserId,
racfGroupName });
else if (imsConnectReasonCode == 56)
rsValue = ImsConnectErrorMessage.getString(
(String) ImsConnectErrorMessage.class.getField(key)
.get(null), new Object[] { myConnection
.getClientId() });
else if ((imsConnectReasonCode == 72)
|| (imsConnectReasonCode == 74))
rsValue = ImsConnectErrorMessage.getString(
(String) ImsConnectErrorMessage.class.getField(key)
.get(null),
new Object[] { imsDatastoreName });
else
rsValue = ImsConnectErrorMessage
.getString((String) ImsConnectErrorMessage.class
.getField(key).get(null));
} catch (Exception e6) {
// Should not occur in production environments since we would
// only
// be dealing with known exceptions
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in TmInteraction.execute(byte[]) - unknown IMS Connect reason code. Exception caught was: "
+ e6.toString());
// don't rethrow exception since we'll just use null for the
// reason code meaning string;
rsValue = "";
}
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0003E, new Object[] {
new Integer(this.imsConnectReturnCode),
new Integer(this.imsConnectReasonCode), rsValue });
ImsConnectExecutionException e6a = new ImsConnectExecutionException(
ImsConnectErrorMessage.HWS0003E, errMsg);
e6a.setReturnCode(this.imsConnectReturnCode);
e6a.setReasonCode(this.imsConnectReasonCode);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.execute(byte[]). Exception thrown was: "
+ e6a.toString());
this.myConnection.disconnect();
throw e6a;
}
// no output to return, so exit
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("<-- TmInteraction.execute()");
}
/**
* Send internal ACK after receive
*
*/
public void sendInternalAck() throws Exception {
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("--> TmInteractionImpl.sendInternalAck()");
byte[] internalAckInputMsgBytes = null;
byte[] internalAckResponseMsg = null;
if (internalAckInputMessage == null
|| this.copyInputMessageForInternalAck == true) {
internalAckInputMessage = null;
internalAckInputMessage = new InputMessageImpl(this);
internalAckInputMessage
.copyInputMessage((InputMessageImpl) this.inputMsg);
internalAckInputMessage.setData(anEmptyByteArray);
internalAckInputMessage.setTrancodeInData("");
}
// String saveInteractionTypeDescription =
// this.getInteractionTypeDescription();
this
.setInteractionTypeDescription(ApiProperties.INTERACTION_TYPE_DESC_ACK);
// ((InputMessageImpl)(this.inputMsg)).setIrmTimer((byte)ApiProperties.IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_NOWAIT_VALUE);
// this.setImsConnectTimeout((byte)ApiProperties.IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_NOWAIT_VALUE);
if (this.cM0AckNoWaitCanBeUsed) {
this
.setImsConnectTimeout(ApiProperties.IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_NOWAIT_VALUE);
} else {
// this.setImsConnectTimeout((byte)ApiProperties.IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_DEFAULT_VALUE);
this.setImsConnectTimeout(ApiProperties.TIMEOUT_10_MILLISECONDS);
}
boolean aSuppressLogging = false;
internalAckInputMsgBytes = internalAckInputMessage
.buildInputMessageByteArray(aSuppressLogging);
this.myConnection.send(internalAckInputMsgBytes);
// this.setInteractionTypeDescription(saveInteractionTypeDescription);
// if (!this.inConversation) // ||
// ((this.getInteractionTypeDescription() ==
// INTERACTION_TYPE_DESC_RESUMETPIPE) && // no response to ACK returned
// by Connect if in conversation
// ((this.getResumeTpipeProcessing() == ApiProperties.RESUME_TPIPE_AUTO)
// ||
// (this.getResumeTpipeProcessing() ==
// ApiProperties.RESUME_TPIPE_NOAUTO))))
if ((!this.inConversation) && // no response to ACK returned by Connect
// if in conversation
(!this.cM0AckNoWaitCanBeUsed)) // no response if ACK NoWait was
// used
{
internalAckResponseMsg = this.myConnection.receive();
if ((this.useCM0AckNoWait) && (!this.cM0AckNoWaitCanBeUsed)
&& (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL))) {
logger
.finest(" The useCm0AckNoWait value of true was ignored because CM0 ACK nowait is "
+ "not supported in the target IMS Connect. The API will send in the ACK "
+ "message using the configured imsConnectTimeout value and wait that "
+ "length of time for the timeout response to be returned by IMS Connect.");
}
/*
* if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL) &&
* (internalAckResponseMsg != null)) { //logger.finest(
* " TmInteractionImpl.sendInternalAck() - internalAckResponseMsg = \n["
* + new String(internalAckResponseMsg, this.imsConnectDataCodepage)
* + "]\n" + //logger.finest(
* " TmInteractionImpl.sendInternalAck() - internalAckResponseMsg = \n["
* + new String(internalAckResponseMsg) + "]\n" + //
* " internalAckResponseMsg.length = [" +
* internalAckResponseMsg.length + "]"); boolean obfuscatePassword =
* false; String bufferReceived =
* this.formatBufferForTracing(internalAckResponseMsg,
* obfuscatePassword);
* logger.finest(" TmInteraction.sendInternalAck() - Buffer received:"
* ); logger.finest(bufferReceived); }
*/
((OutputMessageImpl) (this.outputMsg))
.setResponseMessage(internalAckResponseMsg);
this.outputMsgBytes = internalAckResponseMsg;
this.processOutputMessage();
if (((this.commitMode == ApiProperties.COMMIT_MODE_1) && // CM1
// deallocate commit or CM0 timeout occurred so do nothing
(this.imsConnectReturnCode == 4) && (this.imsConnectReasonCode == 97))
|| (this.imsConnectReturnCode == 32)
|| (this.imsConnectReturnCode == 36)
|| (this.imsConnectReturnCode == 40)) {
// if ((this.imsConnectReturnCode == 4)
// && (this.imsConnectReasonCode == 97)) {
// this.myConnection.disconnect();
// }
} else if (this.imsConnectReturnCode == 0) // no CM1 deallocate or
// CM0 timeout so there is a response message which must be NAK'd
{ // since the API can only return one output message at a time
sendInternalNak(); // NAK response ignored
} else {
StringBuffer rsBuf = new StringBuffer("HWS_RESCODE_");
rsBuf.append(imsConnectReasonCode);
String key = rsBuf.toString();
String rsValue = null;
try {
if (imsConnectReasonCode == 14)
rsValue = ImsConnectErrorMessage.getString(
(String) ImsConnectErrorMessage.class.getField(
key).get(null),
new Object[] { xmlAdapterName });
else if (imsConnectReasonCode == 40)
rsValue = ImsConnectErrorMessage.getString(
(String) ImsConnectErrorMessage.class.getField(
key).get(null), new Object[] {
racfUserId, racfGroupName });
else if ((imsConnectReasonCode == 72)
|| (imsConnectReasonCode == 74))
rsValue = ImsConnectErrorMessage.getString(
(String) ImsConnectErrorMessage.class.getField(
key).get(null),
new Object[] { imsDatastoreName });
else
rsValue = ImsConnectErrorMessage
.getString((String) ImsConnectErrorMessage.class
.getField(key).get(null));
} catch (Exception e) {
// Should not occur in production environments since we
// would only
// be dealing with known exceptions
}
String errMsg = ImsConnectErrorMessage
.getString(ImsConnectErrorMessage.HWS0003E,
new Object[] {
new Integer(this.imsConnectReturnCode),
new Integer(this.imsConnectReasonCode),
rsValue });
ImsConnectExecutionException e = new ImsConnectExecutionException(
ImsConnectErrorMessage.HWS0003E, errMsg);
e.setReturnCode(this.imsConnectReturnCode);
e.setReasonCode(this.imsConnectReasonCode);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.sendInternalAck(). Exception thrown was: "
+ e.toString());
this.myConnection.disconnect();
throw e;
}
} else {
if ((this.cM0AckNoWaitCanBeUsed) && (this.isUseCM0AckNoWait())
&& (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL))) // no
// response
// if
// CM0
// ACK
// NoWait
// was
// used
logger
.finest(" Receive call skipped because CM0 ACK no wait was used\n");
if (this.isInConversation()
&& (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL))) // no
// response
// if
// in
// conversation
logger
.finest(" Receive call skipped because current interaction was within the scope of an IMS Conversation\n");
}
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("<-- TmInteractionImpl.sendInternalAck()");
}
/**
* Send internal NAK to reject an additional response message returned after
* an internal ACK
*
*/
public byte[] sendInternalNak() throws Exception {
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("--> TmInteractionImpl.sendInternalNak()");
byte[] internalNakInputMsgBytes = null;
byte[] internalNakResponseMsg = null;
// String saveInteractionTypeDescription =
// this.getInteractionTypeDescription();
this
.setInteractionTypeDescription(ApiProperties.INTERACTION_TYPE_DESC_NAK);
InputMessageImpl internalNakInputMessage = new InputMessageImpl(this);
internalNakInputMessage
.copyInputMessage((InputMessageImpl) this.inputMsg);
// internalNakInputMessage.copyInputMessage((InputMessageImpl)this.inputMsg);
internalNakInputMessage.setData(anEmptyByteArray);
// internalNakInputMessage.setTrancodeInData("");
boolean aSuppressLogging = true;
internalNakInputMsgBytes = internalNakInputMessage
.buildInputMessageByteArray(aSuppressLogging);
this
.setImsConnectTimeout((byte) ApiProperties.IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_DEFAULT_VALUE);
this.setUseCM0AckNoWait(false);
this.myConnection.send(internalNakInputMsgBytes);
// this.setInteractionTypeDescription(saveInteractionTypeDescription);
internalNakResponseMsg = this.myConnection.receive();
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL)
&& (internalNakResponseMsg != null)) {
// logger.finest(" TmInteractionImpl.sendInternalNak() - internalNakResponseMsg = ["
// + new String(internalNakResponseMsg, this.imsConnectDataCodepage)
// + "]\n" +
// logger.finest(" TmInteractionImpl.sendInternalNak() - internalNakResponseMsg = ["
// + new String(internalNakResponseMsg) + "]\n" +
// " internalNakResponseMsg.length = [" +
// internalNakResponseMsg.length + "]");
boolean obfuscatePassword = false;
String bufferReceived = this.formatBufferForTracing(
internalNakResponseMsg, obfuscatePassword);
logger
.finest(" TmInteraction.sendInternalNak() - Buffer received:");
logger.finest(bufferReceived);
}
((OutputMessageImpl) (this.outputMsg))
.setResponseMessage(internalNakResponseMsg);
this.processOutputMessage();
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("<-- TmInteractionImpl.sendInternalNak()");
if (this.getCommitMode() == ApiProperties.COMMIT_MODE_0)
return null;
else
return internalNakResponseMsg;
}
/**
* Populates TmInteraction response-related properties with from
* aMySavedResponseValues which are the values saved from the original
* interaction that triggered the internal ACK
*
*/
public void restoreResponseValuesAfterInternalAck(
SavedResponseValues aMySavedResponseValues)
throws ImsConnectApiException, UnsupportedEncodingException {
this.setInteractionTypeDescription(aMySavedResponseValues
.getTmpIntTypeDesc());
this.setImsConnectTimeout(aMySavedResponseValues
.getTmpImsConnectTimeout());
((InputMessageImpl) (this.inputMsg))
.copyInputMessage(aMySavedResponseValues.getTmpInputMsg());
((OutputMessageImpl) (this.outputMsg))
.copyOutputMessage(aMySavedResponseValues.getTmpOutputMsg());
this.outputMsgBytes = aMySavedResponseValues.tmpOutputMsgBytes;
this.setImsConnectReturnCode(aMySavedResponseValues
.getTmpImsConnectReturnCode());
this.setImsConnectReasonCode(aMySavedResponseValues
.getTmpImsConnectReasonCode());
this.setOtmaSenseCode(aMySavedResponseValues.getTmpOtmaSenseCode());
this.ackNakNeeded = false;
this.setInConversation(aMySavedResponseValues.isTmpInConversation());
this.setAsyncOutputAvailable(aMySavedResponseValues
.isTmpAsyncOutputAvailable());
this.setProtocolLevel(aMySavedResponseValues.getTmpProtocolLevel());
this.setUseCM0AckNoWait(aMySavedResponseValues.isTmpUseCM0AckNoWait());
this.setResponsePropertiesUpdatedAfterResponse(aMySavedResponseValues
.isTmpResponsePropertiesSetAfterResponse());
}
/**
* Populates MyTMInteractionAttributes properties with values read in from a
* TmInteractionAttributes file
*
*/
public void loadTmInteractionAttributesFromFile(
String anInteractionAttributesFileName) throws Exception {
PropertiesFileLoader myPropertiesFileLoader = new PropertiesFileLoader();
myPropertiesFileLoader.loadPropertiesFile(this,
anInteractionAttributesFileName);
}
/**
* Process the CSM flag in the OutputMessageImpl object.
*/
public void processCsmFlag() {
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("--> TmInteractionImpl.processCsmFlg()");
try {
if (this.outputMsg != null) {
csmFlag1 = ((OutputMessageImpl) (this.outputMsg)).getCsmFlag1();
csmFlag2 = ((OutputMessageImpl) (this.outputMsg)).getCsmFlag2();
// Set protocol level available flag
this
.setProtocolLevelAvailable((csmFlag1 & OutputMessageProperties.CSM_FLG1_PLAVAIL) == OutputMessageProperties.CSM_FLG1_PLAVAIL);
((OutputMessageImpl) this.outputMsg)
.setProtocolLevelAvailable(this
.isProtocolLevelAvailable());
// ((OutputMessageImpl)this.outputMsg).setProtocolLevelAvailablePropertyUpdated(true);
// Set protocol level if available
if (this.isProtocolLevelAvailable()) {
this.setProtocolLevel((byte) (csmFlag2 & 0x03));
((OutputMessageImpl) this.outputMsg).setProtocolLevel(this
.getProtocolLevel());
// ((OutputMessageImpl)this.outputMsg).setProtocolLevelPropertyUpdated(true);
if (this.getCommitMode() == ApiProperties.COMMIT_MODE_0)
this.setCM0AckNoWaitCanBeUsed(this.isUseCM0AckNoWait());
} else {
this.setProtocolLevel((byte) (0x00));
((OutputMessageImpl) this.outputMsg)
.setProtocolLevel(OutputMessageProperties.CSM_FLG2_NOFLAG);
// ((OutputMessageImpl)this.outputMsg).setProtocolLevelPropertyUpdated(true);
this.setCM0AckNoWaitCanBeUsed(false);
}
// Check if the ACK/NAK response needed flag is turned on
this
.setAckNakNeeded((csmFlag1 & OutputMessageProperties.CSM_FLG1_RESP) == OutputMessageProperties.CSM_FLG1_RESP);
((OutputMessageImpl) this.outputMsg).setAckNakNeeded(this
.isAckNakNeeded());
// ((OutputMessageImpl)this.outputMsg).setAckNakNeededPropertyUpdated(true);
// Check if the inConversation flag is turned on
this
.setInConversation((csmFlag1 & OutputMessageProperties.CSM_FLG1_CONV) == OutputMessageProperties.CSM_FLG1_CONV);
((InputMessageImpl) this.inputMsg).setInConversation(this
.testInConversation());
((OutputMessageImpl) this.outputMsg).setInConversation(this
.testInConversation());
// ((OutputMessageImpl)this.outputMsg).setInConversationPropertyUpdated(true);
// Check if the asyncOutputAvailable flag is turned on
this
.setAsyncOutputAvailable((csmFlag1 & OutputMessageProperties.CSM_FLG1_ASYNC) == OutputMessageProperties.CSM_FLG1_ASYNC);
((OutputMessageImpl) this.outputMsg)
.setAsyncOutputAvailable(this.isAsyncOutputAvailable());
// ((OutputMessageImpl)this.outputMsg).setAsyncOutputAvailablePropertyUpdated(true);
this.setResponsePropertiesUpdatedAfterResponse(true);
((OutputMessageImpl) this.outputMsg)
.setResponsePropertiesUpdatedAfterResponse(true);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL)) {
logger.finest(" protocolLevelAvailable = ["
+ this.protocolLevelAvailable + "]");
logger.finest(" ackNakNeeded = ["
+ this.ackNakNeeded + "]");
logger.finest(" inConversation = ["
+ this.inConversation + "]");
logger.finest(" asyncOutputAvailable = ["
+ this.asyncOutputAvailable + "]");
logger.finest(" protocolLevel = ["
+ this.protocolLevel + "]");
}
}
} finally {
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("<-- TmInteractionImpl.processCsmFlg()");
}
}
/**
* Process the output message received from IMS Connect.
*/
public void processOutputMessage() throws Exception {
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("--> TmInteractionImpl.processOutputMessage()");
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL)) {
boolean obfuscatePassword = false;
this.formatAndPrintReceiveBuffer(obfuscatePassword);
}
if ((imsConnectUserMessageExitIdentifier == ApiProperties.IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSSMPL0))
this
.setResponseIncludesLlll(ApiProperties.RESPONSE_DOES_NOT_INCLUDE_LLLL);
else if ((imsConnectUserMessageExitIdentifier == ApiProperties.IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSSMPL1)
|| (imsConnectUserMessageExitIdentifier == ApiProperties.IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSDPWR1)
|| (imsConnectUserMessageExitIdentifier == ApiProperties.IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSCSLO1)
|| (imsConnectUserMessageExitIdentifier == ApiProperties.IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSCSLO0))
this
.setResponseIncludesLlll(ApiProperties.RESPONSE_DOES_INCLUDE_LLLL);
try {
((OutputMessageImpl) (this.outputMsg)).setMyImsConnectCodepage(this
.getImsConnectCodepage());
((OutputMessageImpl) (this.outputMsg))
.setIncludeLlzzInSegments(this.inputMessageDataSegmentsIncludeLlzzAndTrancode);
((OutputMessageImpl) (this.outputMsg)).parse(responseIncludesLlll);
if ((((OutputMessageImpl) this.outputMsg).getMsgType() == OutputMessageProperties.MSGTYPE_DATA)
|| (((OutputMessageImpl) this.outputMsg).getMsgType() == OutputMessageProperties.MSGTYPE_RSM_REQSTS))
this.processCsmFlag();
this.processRtnRsnSnsCodes();
// this.setNumberOfSegments();
} catch (Exception e) {
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in TmInteractionImpl.processOutputMessage(). Exception caught was: "
+ e.toString());
throw e;
} finally {
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("<-- TmInteractionImpl.processOutputMessage()");
}
}
/**
* Process the IMS Connect return and reason codes and OTMA sense code in
* the OutputMessageImpl object.
*/
public void processRtnRsnSnsCodes() {
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("--> TmInteractionImpl.processRtnRsnSnsCodes()");
try {
if (this.outputMsg != null) {
// Set the IMS Connect return and reason codes and OTMA sense
// code
/*
* this .setImsConnectReturnCode(((OutputMessageImpl)
* (this.outputMsg)) .getImsConnectReturnCode()); this
* .setImsConnectReasonCode(((OutputMessageImpl)
* (this.outputMsg)) .getImsConnectReasonCode());
* this.setOtmaSenseCode(((OutputMessageImpl) (this.outputMsg))
* .getOtmaSenseCode());
* this.setOtmaReasonCode(((OutputMessageImpl) (this.outputMsg))
* .getOtmaReasonCode());
* this.setRacfReturnCode(((OutputMessageImpl) (this.outputMsg))
* .getRacfReturnCode());
*/
this.setImsConnectReturnCode(this.outputMsg
.getImsConnectReturnCode());
this.setImsConnectReasonCode(this.outputMsg
.getImsConnectReasonCode());
this.setOtmaSenseCode(this.outputMsg.getOtmaSenseCode());
this.setOtmaReasonCode(this.outputMsg.getOtmaReasonCode());
this.setRacfReturnCode(this.outputMsg.getRacfReturnCode());
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL)) {
logger.finest(" IMS Connect Return Code = ["
+ this.getImsConnectReturnCode() + "]");
logger.finest(" IMS Connect Reason Code = ["
+ this.getImsConnectReasonCode() + "]");
if (this.getImsConnectReturnCode() == 16) {
logger.finest(" IMS OTMA Sense Code = ["
+ this.getOtmaSenseCode() + "]");
logger.finest(" IMS OTMA Reason Code = ["
+ this.getOtmaReasonCode() + "]");
} else if ((this.getImsConnectReturnCode() == 8)
&& (this.getImsConnectReasonCode() == 40)) {
logger.finest(" RACF Return Code = ["
+ this.getRacfReturnCode() + "]");
}
}
}
} finally {
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("<-- TmInteractionImpl.processRtnRsnSnsCodes()");
}
}
// Getter and setter for internal properties
/**
* Gets a byte
value representing whether the client
* application code or the internal API code will provide the acknowledgment
* messages to IMS Connect when required after receiving output from IMS
* Connect.
*
* @return a byte
value containing whether the IMS Connect API
* (0) or the client application (1) will ACK or NAK replies from
* IMS Connect whenever an ACK or NAK response is required
*/
public byte getAckNakProvider() {
return this.ackNakProvider;
}
/**
* Set clientOrInternalAckNak property value
*
* @param a
* byte value representing whether the IMS Connect API (0) or the
* client application (1) will ACK or NAK replies from IMS
* Connect whenever an ACK or NAK response is required
*/
public void setAckNakProvider(byte anAckNakProvider)
throws ImsConnectApiException {
this.ackNakProvider = anAckNakProvider;
if ((anAckNakProvider == ApiProperties.API_INTERNAL_ACK)
|| (anAckNakProvider == ApiProperties.CLIENT_ACK_NAK)) {
this.ackNakProvider = anAckNakProvider;
} else {
String validAckNakProviderString = "";
try {
validAckNakProviderString = ImsConnectErrorMessage
.getString(ImsConnectErrorMessage.VALID_PROPERTY_VALUE_ACKNAKPROVIDER);
} catch (Exception e1) {
// do nothing - this exception should never occur unless the API
// JAR is corrupted
}
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0030E, new Object[] {
"ackNakProvider", String.valueOf(anAckNakProvider),
validAckNakProviderString });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0030E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in Connection.setAckNakProvider(byte). Exception thrown was: "
+ e.toString());
throw e;
}
}
/**
* Get connection used for this interaction
*
* @return myConnection connection
*/
public Connection getMyConnection() {
return this.myConnection;
}
/**
* Get TmInteraction properties
*
* @return TmInteraction properties
*/
public TmInteractionAttributes getInteractionAttributes() {
return interAttr;
}
/**
* @return
*/
public boolean getIncludeLlllInOutputMessages() {
return includeLlllInOutputMessages;
}
/**
* @return
*/
public boolean getIncludeLlzzInOutputMessages() {
return includeLlzzInOutputMessages;
}
/**
* @return
*/
public InputMessage getInputMessage() throws ImsConnectApiException {
return inputMsg;
}
/**
* @return
*/
public OutputMessage getOutputMessage() throws ImsConnectApiException {
return outputMsg;
}
// begin: himakar 02/28/07 Callout changes
/**
* return a boolean indicating if IMS Connect is expecting a message (true)
* or not (false)
*/
public boolean isResponseNeeded() {
boolean result = false;
if (this.outputMsg != null) {
// Check if the ACK/NACK response flag is turned on
result = ((csmFlag1 & OutputMessageProperties.CSM_FLG1_RESP) == OutputMessageProperties.CSM_FLG1_RESP);
}
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL))
if (result)
logger
.finest(" TmInteractionImpl.isResponseNeeded()ACK or NACK response requested");
return result;
}
/**
* Gets the ackNakNeeded property value.
*
* This property indicates whether or not this response from IMS must be
* acknowledged or negatively acknowledged prior to any other activity on
* this connection.
*
* If ackNakProvider was set to API_INTERNAL_ACK (the default value) in the
* original request message, this property will always be set to false in
* the corresponding response message since the acknowledgement of the
* response, if required, will have been processed internally by the IMS
* Connect API.
*
* When ackNakProvider is set to CLIENT_ACK_NAK in the original request
* message, this property will be set according to the IMS Connect protocol.
* Whenever this property is set to true in the response, the client must
* send in an ACK or NAK interaction as the next interaction on that
* connection or IMS Connect will respond with a protocol violation.
*
* Use the constants defined in ApiProperties
to determine the
* ackNakNeeded property value. For example,
*
*
* if (myTmInteraction.isAckNakNeeded() == ApiProperties.ACK_NAK_NEEDED) {
* // submit an ACK or NAK interaction as required as the next
* // interaction on this connection
* }
*
*
* @return a boolean
value indicating whether or not the
* response from IMS must be acknowledged or negatively acknowledged
* prior to any other activity on this connection
* @see ApiProperties#ACK_NAK_NEEDED
* @see ApiProperties#NO_ACK_NAK_NEEDED
*/
public boolean isAckNakNeeded() {
if (this.isAckNakNeededPropertyUpdated())
return ackNakNeeded;
else {
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL))
logger
.finest(" isAckNakNeeded returned the default value, false, since no response has been yet from IMS Connect\n");
return false;
}
}
/**
* @return the ackNakNeededPropertyUpdated
*/
protected boolean isAckNakNeededPropertyUpdated() {
return this.ackNakNeededPropertyUpdated;
}
/**
* @param ackNakNeeded
* The ackNakNeeded to set.
*/
private void setAckNakNeeded(boolean ackNakNeeded) {
this.ackNakNeeded = ackNakNeeded;
this.setAckNakNeededPropertyUpdated(true);
}
/**
* @param ackNakNeededPropertyUpdated
* the ackNakNeededPropertyUpdated to set
*/
protected void setAckNakNeededPropertyUpdated(
boolean anAckNakNeededPropertyUpdated) {
this.ackNakNeededPropertyUpdated = anAckNakNeededPropertyUpdated;
}
/**
* Gets the asyncOutputAvailable property value.
*
* This property specifies whether or not there are any more asynchronous
* output messages queued in on the asynchronous hold queue for this
* client's tpipe in IMS and available for retrieval by this or another
* client.
*
* The value of this property is only valid in a non-shared queues
* environment. In a shared queues environment, the client must issue a
* ResumeTpipe interaction in order to know if there is asynchronous output
* available (in which case, output will be returned if there is any
* available).
*
* Use the constants defined in ApiProperties
to determine the
* asyncOutputAvailable property value. For example,
*
*
* if (myTmInteraction.isAsyncOutputAvailable() == ApiProperties.ASYNC_OUTPUT_AVAILABLE) {
* // retrieve additional asynchronous output or save a flag that you can use
* // to retrieve the asynchronous output at a later time
* }
*
*
* @return a boolean
value indicating whether or not there are
* any more asynchronous output messages queued on the asynchronous
* hold queue for this client's tpipe in IMS and available for
* retrieval by this or another client
* @see ApiProperties#ASYNC_OUTPUT_AVAILABLE
* @see ApiProperties#NO_ASYNC_OUTPUT_AVAILABLE
*/
public boolean isAsyncOutputAvailable() {
if (this.isResponsePropertiesUpdatedAfterResponse())
return asyncOutputAvailable;
else {
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL))
logger
.finest(" isAsyncOutputAvailable returned the default value, false, since no response has been received yet from IMS Connect\n");
return false;
}
}
/**
* @param asyncOutputAvailable
* The asyncOutputAvailable to set.
*/
private void setAsyncOutputAvailable(boolean asyncOutputAvailable) {
this.asyncOutputAvailable = asyncOutputAvailable;
this.setResponsePropertiesUpdatedAfterResponse(true);
}
/**
* Gets a byte
value representing the protocol level of the
* target IMS Connect which indicates whether or not the target IMS Connect
* supports CM0 ACK NO WAIT. This value is determined internally by the API
* based on information in the previous response from IMS Connect received
* by the API. If getProtocolLevel() returns a value of
* ApiProperties#PROTOCOL_LEVEL_CM0_ACK_NOWAIT_SUPPORT when preparing to
* issue an ACK of a CM0 SendReceive response, the API will be able to use
* its CM0 ACK NO WAIT processing when sending in an ACK to a CM0
* SendReceive response. Otherwise, the API will not use its CM0 ACK NO WAIT
* processing when sending in an ACK to a CM0 SendReceive response.
*
* @return the protocolLevelAvailable byte value
* @see ApiProperties#PROTOCOL_LEVEL_BASE (CM0_ACK_NOWAIT not supported)
* @see ApiProperties#PROTOCOL_LEVEL_CM0_ACK_NOWAIT_SUPPORT
* @see TmInteractionImpl#setProtocolLevel()
* @see TmInteractionImpl#isProtocolLevelAvailable()
*/
public byte getProtocolLevel() {
return this.protocolLevel;
}
/**
* @param aProtocolLevel
* the protocolLevel to set
* @see ApiProperties#PROTOCOL_LEVEL_BASE (CM0_ACK_NOWAIT not supported)
* @see ApiProperties#PROTOCOL_LEVEL_CM0_ACK_NOWAIT_SUPPORT
* @see TmInteractionImpl#getProtocolLevel()
* @see TmInteractionImpl#isProtocolLevelAvailable()
*/
public void setProtocolLevel(byte aProtocolLevel) {
this.protocolLevel = aProtocolLevel;
}
/**
* Gets a boolean
value representing whether or not the target
* IMS Connect supports returning the IMS Connect protocol level in the CSM.
* This value is determined internally by the API based on information in
* the previous response from IMS Connect received by the API. If
* isProtocolLevelAvailable() returns a value of true when preparing to
* issue an ACK of a CM0 SendReceive response, the getProtocolLevel() method
* can be used to determine if the target IMS Connect supports CM0 ACK NO
* WAIT.
*
* @return the protocolLevelAvailable boolean value
* @see ApiProperties#PROTOCOL_LEVEL_BASE (CM0_ACK_NOWAIT not supported)
* @see ApiProperties#PROTOCOL_LEVEL_CM0_ACK_NOWAIT_SUPPORT
* @see TmInteraction#setProtocolLevelAvailable(boolean
* protocolLevelAvailable)
* @see TmInteraction#getProtocolLevel()
*/
public boolean isProtocolLevelAvailable() {
return this.protocolLevelAvailable;
}
/**
* @param protocolLevelAvailable
* the protocolLevelAvailable to set
* @see ApiProperties#PROTOCOL_LEVEL_BASE (CM0_ACK_NOWAIT not supported)
* @see ApiProperties#PROTOCOL_LEVEL_CM0_ACK_NOWAIT_SUPPORT
* @see TmInteraction#isProtocolLevelAvailable()
* @see TmInteraction#getProtocolLevel()
*/
public void setProtocolLevelAvailable(boolean protocolLevelAvailable) {
this.protocolLevelAvailable = protocolLevelAvailable;
}
/**
* Gets the inCoversation property value.
*
* This response property specifies whether or not this interaction is part
* of a currently active IMS conversation.
*
* Use the constants defined in ApiProperties
to determine the
* InCoversation property value. For example,
*
*
* if (myTmInteraction.isInConversation() == ApiProperties.IN_CONVERSATION) {
* // client application needs to continue the conversation or send in
* // an interaction to end the conversation. API will not include the
* // trancode in an input message for an interaction if the
* // inConversation property value is true.
* }
*
*
* @return a boolean
value indicating whether or not this
* interaction is part of a currently active IMS conversation
* @see ApiProperties#IN_CONVERSATION
* @see ApiProperties#NOT_IN_CONVERSATION
*/
public boolean isInConversation() {
if (this.responsePropertiesUpdatedAfterResponse)
return inConversation;
else {
if ((logger != null)
&& (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL)))
logger
.finest(" isInConversation returned the default value, false, since no response has been received yet from IMS Connect\n");
return false;
}
}
/**
* Internal method that returns the inCoversation property value simalar to
* the public isInConversation(), method without doing the tracing and
* returning the value of the property rather than false when it has not yet
* been explicitly set.
*
* @return a boolean
value indicating whether or not this
* interaction is part of a currently active IMS conversation
* @see ApiProperties#IN_CONVERSATION
* @see ApiProperties#NOT_IN_CONVERSATION
*/
protected boolean testInConversation() {
return inConversation;
}
/**
* @param anInConversation
* The inConversation to set.
*/
private void setInConversation(boolean anInConversation) {
if (this.inConversation != anInConversation) {
this.inConversation = anInConversation;
this.setRebuildMessage(true);
}
this.setResponsePropertiesUpdatedAfterResponse(true);
}
/**
* @return the rebuildMessage
*/
protected boolean isRebuildMessage() {
return this.rebuildMessage;
}
/**
* @param rebuildMessage
* the rebuildMessage to set
*/
protected void setRebuildMessage(boolean rebuildMessage) {
this.rebuildMessage = rebuildMessage;
}
/**
* Gets a boolean
value indicating whether each response
* message does or does not include LLLL
*
* @return a boolean
value containing whether the response
* messages returned to the IMS Connect API by IMS Connect start
* with LLLL (true - response messages processed using HWSSMPL1 in
* IMS Connect do start with LLLL) or do not start with LLLL (false
* - response messages processed using HWSSMPL0 do not start with
* LLLL)
*/
public boolean isResponseIncludesLlll() {
return this.responseIncludesLlll;
}
// Input properties
/**
* Set responseIncludesLlll property value. Note that the paramater supplied
* to this method is over-ridden by the appropriate value for HWSSMPL0 or
* HWSSMPL1 if the setImsConnectUserMessageExitIdentifier is used to set to
* user message exit identifier to *SAMPLE* or *SAMPL1*, respectively. This
* method should only to be invoked if the
* setImsConnectUserMessageExitIdentifier method is used to set a value
* other than *SAMPLE* or *SAMPL1*.
*
* @param a
* boolean value representing whether the messages returned to
* the IMS Connect API by IMS Connect start with LLLL (true -
* messages sent from HWSSMPL1 start with LLLL) or do not start
* with LLLL (messages sent from HWSSMPL0 do not start with LLLL)
*/
public void setResponseIncludesLlll(boolean aResponsetIncludesLlll) {
this.responseIncludesLlll = aResponsetIncludesLlll;
}
/**
* @return the responsePropertiesUpdatedAfterResponse
*/
protected boolean isResponsePropertiesUpdatedAfterResponse() {
return this.responsePropertiesUpdatedAfterResponse;
}
// Setter methods
/**
* @param ackNakNeededPropertyUpdated
* the ackNakNeededPropertyUpdated to set
*/
protected void setResponsePropertiesUpdatedAfterResponse(
boolean aResponsePropertiesUpdatedAfterResponse) {
this.responsePropertiesUpdatedAfterResponse = aResponsePropertiesUpdatedAfterResponse;
}
/**
* @return the inConversationPropertyUpdated
*/
/*
* protected boolean isInConversationPropertyUpdated() { return
* this.inConversationPropertyUpdated; }
*/
/**
* Gets the imsConnectReasonCode property value.
*
* This response property specifies the IMS Connect or IMS Connect user
* message exit reason code if IMS Connect or the IMS Connect user message
* exit detected an error during processing of the current interaction.
*
* See the V10 IMS Messages and Codes topic
* "Return and reason codes" for a description of
* the IMS Connect reason codes.
*
* @return an int
value containing the reason code
*/
public int getImsConnectReasonCode() {
return imsConnectReasonCode;
}
/**
* @param ackNakNeededPropertyUpdated
* the ackNakNeededPropertyUpdated to set
*/
/*
* protected void setInConversationPropertyUpdated(boolean
* anInConversationPropertyUpdated) { this.inConversationPropertyUpdated =
* anInConversationPropertyUpdated; }
*/
/**
* @param imsConnectReasonCode
* The imsConnectReasonCode to set.
*/
private void setImsConnectReasonCode(int imsConnectReasonCode) {
this.imsConnectReasonCode = imsConnectReasonCode;
}
/**
* Gets the ImsConnectReturnCode property value. This response property
* specifies the IMS Connect or IMS Connect user message exit return code if
* IMS Connect or the IMS Connect user message exit detected an error during
* processing of the current interaction.
*
* See the V10 IMS Messages and Codes topic
* "Return and reason codes" for a description of
* the IMS Connect return codes.
*
* @return an int
value containing the return code
*/
public int getImsConnectReturnCode() {
return imsConnectReturnCode;
}
/**
* @param imsConnectReturnCode
* The imsConnectReturnCode to set.
*/
private void setImsConnectReturnCode(int imsConnectReturnCode) {
this.imsConnectReturnCode = imsConnectReturnCode;
}
/**
* Gets the mfsModname name property value. The mfsModname property will
* have a meaningful value only if the user requests that the MFS modname be
* returned. In this case, IMS Connect returns an RMM segment at the
* beginning of the response message, if the interaction is successful. If
* the interaction fails, the modname is not returned.
*
* @return the mfsModname value returned by the IMS application through IMS
* Connect
*/
public String getMfsModname() {
return this.mfsModname;
}
/**
* @param mfsModname
* The mfsModname to set.
*/
protected void setMfsModname(String mfsModname) {
this.mfsModname = mfsModname;
}
/**
* Gets the OTMA reason code. An OTMA reason code may be returned whenever
* OTMA returns a sense code value of X'1A' (decimal 26.)
*
* @return an int
value containing the otmaReasonCode
* @see TmInteraction#getOtmaSenseCode()
*/
public int getOtmaReasonCode() {
return otmaReasonCode;
}
/**
* @param anOtmaReasonCode
* The otmaReasonCode to set.
*/
private void setOtmaReasonCode(int anOtmaReasonCode) {
this.otmaReasonCode = anOtmaReasonCode;
}
/**
* Gets the OTMA sense code property value. For information about OTMA sense
* codes, see the IMS V10 Messages and Codes documentation, under the topic
* "OTMA sense codes for NAK messages".
*
* @return int value representing the OTMA sense code
*/
public int getOtmaSenseCode() {
return otmaSenseCode;
}
/**
* @param anOtmaSenseCode
* The otmaSenseCode to set.
*/
private void setOtmaSenseCode(int anOtmaSenseCode) {
this.otmaSenseCode = anOtmaSenseCode;
}
/**
* Gets the racfReturnCode property value. This response property specifies
* the RACF return code if RACF detected an error during processing of the
* current interaction.
*
* See the V10 IMS Messages and Codes topic
* "Return and reason codes" for a description of
* the IMS Connect return codes.
*
* @return an int
value containing the RACF return code
*/
public int getRacfReturnCode() {
return racfReturnCode;
}
/**
* @param aRacfReturnCode
* The racfReturnCode to set.
*/
private void setRacfReturnCode(int aRacfReturnCode) {
this.racfReturnCode = aRacfReturnCode;
}
/**
* @return the racfReturnCodeString
*/
public String getRacfReturnCodeString() {
return this.racfReturnCodeString;
}
/**
* @param racfReturnCodeString
* the racfReturnCodeString to set
*/
public void setRacfReturnCodeString(String racfRetCodeString) {
this.racfReturnCodeString = racfRetCodeString;
}
/**
* @param
*/
/*
* public void setIncludeLlllInOutputMessages(boolean
* aIncludeLlllInOutputMessages) { includeLlllInOutputMessages =
* aIncludeLlllInOutputMessages; }
*
* /**
*
* @param
*/
/*
* public void setIncludeLlzzInOutputMessages(boolean
* aIncludeLlzzInOutputMessages) { includeLlzzInOutputMessages =
* aIncludeLlzzInOutputMessages; }
*
* /** Sets the numberOfSegments
*/
protected void setNumberOfSegments() {
int i;
int msgLen = outputMsgBytes.length;
cursor = isResponseIncludesLlll() ? 4 : 0; // skip over LLLL to get
// first LLZZ
// Count the number of segments in the output message - numberOfSegments
// and the number of total bytes in the message - cursor
for (i = 0; cursor < msgLen; i++) {
int low = outputMsgBytes[cursor + 1] & 0xff;
int high = outputMsgBytes[cursor] & 0xff;
cursor += (int) (high << 8 | low);
}
numberOfSegments = i;
}
/**
* @return the numberOfSegments
*/
protected int getNumberOfSegmentsFromOutput() {
return numberOfSegments;
}
/**
* @return the use2DimensionalByteArray
*/
public boolean isUse2DimensionalByteArray() {
return use2DimensionalByteArray;
}
/**
* Set connection used for this interaction
*
* @param myConnection
* connection
*/
public void setConnection(ConnectionImpl conn) {
this.myConnection = conn;
}
/**
* @param inputMsg
* The inputMsg to set.
*/
public void setInputMsg(InputMessageImpl inputMsg) {
if (this.inputMsg != (InputMessage) inputMsg) {
this.inputMsg = (InputMessage) inputMsg;
this.setRebuildMessage(true);
}
}
/**
* @return Returns the savedInputMessageDataSegmentsIncludeLlzzAndTrancode.
*/
/*
* private boolean isSavedInputMessageDataSegmentsIncludeLlzzAndTrancode() {
* return savedInputMessageDataSegmentsIncludeLlzzAndTrancode; }
*
* /**
*
* @param outputMsg The outputMsg to set.
*/
/*
* private void setOutputMsg(OutputMessageImpl outputMsg) { this.outputMsg =
* (OutputMessage) outputMsg; }
*
* /** Set TmInteraction properties
*
* @param spec TmInteraction properties
*/
/*
* private void setInteractionAttributes(TmInteractionAttributes intAttr) {
* interAttr = intAttr; }
*
*
* // Getters and setters for TmInteraction input properties
*
* /** Get the architectureLevel value which represents the highest IMS
* Connect architecture level supported by this version of the IMS Connect
* API
*
* @return an int representing the architecture level of IMS Connect ?????
*/
protected byte getArchitectureLevel() {
return this.architectureLevel;
}
/**
* Set the value of the architectureLevel property which represents the
* highest IMS Connect architecture level supported by this version of the
* IMS Connect API
*
* @param anArchLevel
* an int value representing the highest IMS Connect architecture
* level supported by this version of the IMS Connect API
*/
@SuppressWarnings("unused")
private void setArchitectureLevel(byte anArchLevel)
throws ImsConnectApiException {
if ((ARCH_LEVEL_MIN_VALUE > anArchLevel)
|| (anArchLevel > ARCH_LEVEL_MAX_VALUE)) {
String validArchLevelString = "";
try {
validArchLevelString = ImsConnectErrorMessage
.getString(ImsConnectErrorMessage.VALID_PROPERTY_VALUE_ARCHLEVEL);
} catch (Exception e) {
}
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0030E, new Object[] {
"architectureLevel" + ARCH_LEVEL_NAME[anArchLevel],
Byte.toString(anArchLevel), validArchLevelString });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0030E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setArchitectureLevel(byte). Exception thrown was: "
+ e.toString());
throw e;
}
if (this.architectureLevel != anArchLevel) {
this.architectureLevel = anArchLevel;
this.setRebuildMessage(true);
}
}
/**
* Get clientType property value
*
* @return an int value representing the clientType
*/
protected int getClientType() {
return this.clientType;
}
/**
* Set clientType property value
*
* @param aClientType
* an int value representing the type of client.
*/
@SuppressWarnings("unused")
private void setClientType(int aClientType) {
this.clientType = aClientType;
}
/**
* Get cm0IgnorePurge property value
*
* @return cm0IgnorePurge value
*/
public boolean isCm0IgnorePurge() {
return this.cm0IgnorePurge;
}
/**
* Sets the cm0IgnorePurge property value to be used for this interaction.
* This property is used to tell the IMS application whether or not to
* ignore DL/I purge calls for multi-segment commit mode 0 response messages
* prior to insertion of the last segment of the message.
*
* @param aCm0IgnorePurge
* a boolean value telling the IMS application whether or not to
* ignore DL/I purge calls for individual segments within a
* multi-segment message
*/
public void setCm0IgnorePurge(boolean aCm0IgnorePurge) {
if (this.cm0IgnorePurge != aCm0IgnorePurge) {
this.cm0IgnorePurge = aCm0IgnorePurge;
this.setRebuildMessage(true);
}
}
public void setCancelClientId(boolean aCancelClientId) {
if (this.cancelClientId != aCancelClientId) {
this.cancelClientId = aCancelClientId;
this.setRebuildMessage(true);
}
}
public boolean isCancelClientId() {
return this.cancelClientId;
}
public void setReturnClientId(boolean aReturnClientIdValue) {
if (this.returnClientId != aReturnClientIdValue) {
this.returnClientId = aReturnClientIdValue;
this.setRebuildMessage(true);
}
if(this.returnClientId == true)
this.myConnection.returnedClientID = true;
}
public boolean isReturnClientId() {
return this.returnClientId;
}
public void setGenerateClientIdWhenDuplicate(boolean aGenerateClientIdValue) {
if (this.generateClientIdWhenDuplicate != aGenerateClientIdValue) {
this.generateClientIdWhenDuplicate = aGenerateClientIdValue;
this.setRebuildMessage(true);
}
}
public boolean isGenerateClientIdWhenDuplicate() {
return this.generateClientIdWhenDuplicate;
}
/**
* Get commitMode property value
*
* @return commitMode value
*/
public byte getCommitMode() {
return this.commitMode;
}
/**
* Sets the commitMode property value to be used for this interaction. Only
* one value can be specified per interaction. The valid values, for which
* the following constants are defined in ApiProperties
, are:
*
* COMMIT_MODE_0
- Commit-then-send (CM0 maps to IRM_F2
* value of 0X40)
* COMMIT_MODE_1
- Send-then-commit (CM1 maps to IRM_F2
* value of 0X20)
* DEFAULT_COMMIT_MODE
- same as COMMIT_MODE_0
*
* COMMIT_MODE_0 is the default used if client does not provide a value.
*
* A value for commit mode is required by IMS Connect and OTMA. However, the
* value provided for commitMode will be over-ridden internally if the type
* of interaction is only supported with a specific commit mode. For
* example, resumeTpipe and send-only interactions are only supported for
* Commit Mode 0 while Two-Phase Commit (2PC), though not supported in the
* initial release of the IMS Connect API, always uses Commit Mode 1. If the
* commitMode property value is over-ridden internally by the API and if the
* traceLevel is set to TRACE_LEVEL_INTERNAL, an informational trace message
* will be written to the trace output file.
*
* If a value for this property is not supplied by the client and cannot be
* determined based on the messageFunction value, the default value (
* COMMIT_MODE_0
) will be used. The commit mode value is passed
* to IMS Connect in the OMHDRSYN field of the OTMA header.
*
* For more details on the commit mode property, see the topic
* "Commit mode and synch level definitions" in the V10 Communications
* and Connections guide documentation.
*
* @param an
* byte value representing the commit mode. Use the constants
* defined in ApiProperties to specify this parameter.
* @see ApiProperties#COMMIT_MODE_0
* @see ApiProperties#COMMIT_MODE_1
* @see TmInteraction#getCommitMode()
*/
public void setCommitMode(byte aCommitMode) throws ImsConnectApiException {
if ((aCommitMode == ApiProperties.COMMIT_MODE_0)
|| (aCommitMode == ApiProperties.COMMIT_MODE_1)) {
if (this.commitMode != aCommitMode) {
this.commitMode = aCommitMode;
this.setRebuildMessage(true);
}
} else {
String validCommitModeString = "";
try {
validCommitModeString = ImsConnectErrorMessage
.getString(ImsConnectErrorMessage.VALID_PROPERTY_VALUE_COMMITMODE);
} catch (Exception e1) {
// do nothing - this exception should never occur unless the API
// JAR is corrupted
}
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0030E, new Object[] {
"commitMode", String.valueOf(aCommitMode),
validCommitModeString });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0030E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in Connection.setSocketType(byte). Exception thrown was: "
+ e.toString());
throw e;
}
}
/**
* Gets the IMS Connect codepage property value for messages sent to and
* received from IMS Connect. Used for conversion between the client
* codepage and the IMS Connect codepage for this interaction.
*
* @return a String
value containing the imsConnectCodepage
* property value
*/
public String getImsConnectCodepage() {
return imsConnectCodepage;
}
/**
* Sets the imsConnectCodepage property value which specifies the codepage
* for messages from IMS Connect.
*
* This property is used internally by the API to determine what codepage is
* used for conversion of messages passed back and forth between the client
* and IMS Connect. Examples of valid values are:
*
* "037"
- EBCDIC code page 037 for Australia, Brazil,
* Canada, New Zealand, Portugal, South Africa and USA
* "1047"
- EBCDIC code page 1047 for Open Systems (MVS C
* compiler)
* "1252"
- ASCII code page 1252 for Windows Latin-1
* (Western Europe) (SAP Codepage 1100, ISO-8859-1) (English, French,
* German, Danish, Norwegian, Finnish, Swedish, Italian, Spanish, Icelandic
* and Dutch)
*
* The DEFAULT_IMS_CONNECT_CODEPAGE has the value "037"
.
*
* @param aCodepage
* codepage to be used for conversion from the client codepage to
* the IMS Connect codepage for input request messages and vice
* versa for the output response messages for this interaction -
* used internally by the API
* @see TmInteraction#getImsConnectCodepage()
*/
public void setImsConnectCodepage(String aCodepage) // throws
// ImsConnectApiException
{
// only support UTF-8 in the first release
/*
* if (aCodepage != INBOUND_CODEPAGE_DEFAULT) { String errMsg =
* ImsConnectErrorMessage.getString( ImsConnectErrorMessage.HWS0034E,
* new Object[] {String.valueOf(aCodepage)});
*
* ImsConnectApiException e = new ImsConnectApiException(
* ImsConnectErrorMessage.HWS0034E, errMsg); throw e; }
*/
if (this.imsConnectCodepage != aCodepage) {
this.imsConnectCodepage = aCodepage;
((InputMessageImpl) (this.inputMsg))
.setImsConnectCodepage(aCodepage);
this.rebuildMessage = true;
this.updateIrmDestId = true;
this.updateIrmId = true;
this.updateIrmLTerm = true;
this.updateIrmRacfApplName = true;
this.updateIrmRacfGroupName = true;
this.updateIrmRacfPassword = true;
this.updateIrmRacfUserId = true;
this.updateIrmRerouteName = true;
this.updateIrmTagAdapter = true;
this.updateIrmTagMap = true;
this.updateIrmTrancode = true;
this.updateIrmTrancodeInData = true;
this.updateIrmInputModName = true;
this.updateIrmInteractionType = true;
this.copyInputMessageForInternalAck = true;
}
}
/**
* Get IMS Connect Unicode encoding schema property value for messages to
* and from IMS Connect
*
* @return Returns the imsConnectUnicodeEncodingSchema property value
*/
public byte getImsConnectUnicodeEncodingSchema() {
return imsConnectUnicodeEncodingSchema;
}
/**
* @param imsConnectUnicodeEncodingSchema
* The imsConnectUnicodeEncodingSchema to set.
*/
/*
* public void setImsConnectUnicodeEncodingSchema(byte
* anIConUnicodeEncodingSchema) throws ImsConnectApiException { if
* (((anIConUnicodeEncodingSchema &
* ApiProperties.IMS_CONNECT_UNICODE_ENCODING_SCHEMA_NONE) ==
* ApiProperties.IMS_CONNECT_UNICODE_ENCODING_SCHEMA_NONE)) || //
* ((anIConUnicodeEncodingSchema &
* ApiProperties.IMS_CONNECT_UNICODE_ENCODING_SCHEMA_UTF8) ==
* ApiProperties.IMS_CONNECT_UNICODE_ENCODING_SCHEMA_UTF8) || //
* ((anIConUnicodeEncodingSchema &
* ApiProperties.IMS_CONNECT_UNICODE_ENCODING_SCHEMA_UTF16) ==
* ApiProperties.IMS_CONNECT_UNICODE_ENCODING_SCHEMA_UTF16)) && // same as
* IMS_CONNECT_UNICODE_ENCODING_SCHEMA_UCS2 (anIConUnicodeEncodingSchema &&
* { this.imsConnectUnicodeEncodingSchema = anIConUnicodeEncodingSchema; }
* else { String validImsConnectUnicodeEncodingSchemaString = ""; try {
* validImsConnectUnicodeEncodingSchemaString =
* ImsConnectErrorMessage.getString
* (ImsConnectErrorMessage.VALID_PROPERTY_VALUE_IMSCONNECTUNICODEENCODINGSCHEMA
* ); } catch (Exception e1) { // do nothing - this exception should never
* occur unless the API JAR is corrupted } String errMsg =
* ImsConnectErrorMessage.getString(ImsConnectErrorMessage.HWS0030E, new
* Object[] {"imsConnectUnicodeEncodingSchema",
* String.valueOf(anIConUnicodeEncodingSchema),
* validImsConnectUnicodeEncodingSchemaString});
*
* ImsConnectApiException e = new
* ImsConnectApiException(ImsConnectErrorMessage.HWS0030E, errMsg);
*
* if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
* logger.severe(
* " Exception thrown in Connection.setImsConnectUnicodeEncodingSchema(byte). Exception thrown was: "
* + e.toString());
*
* throw e; } }
*/
/**
* Gets the IMS Connect Unicode usage property value (none, trancode, data
* or trancode and data) for messages sent to and received from IMS Connect
*
* @return a byte
value containing the imsConnectUnicodeUsage
* property value
*/
public byte getImsConnectUnicodeUsage() {
return imsConnectUnicodeUsage;
}
/**
* @param imsConnectUnicodeUsage
* The imsConnectUnicodeUsage to set.
* @see TmInteraction#getImsConnectUnicodeUsage()
*/
public void setImsConnectUnicodeUsage(byte anImsConnectUnicodeUsage)
throws ImsConnectApiException {
if (((anImsConnectUnicodeUsage & ApiProperties.IMS_CONNECT_UNICODE_USAGE_DATA) == ApiProperties.IMS_CONNECT_UNICODE_USAGE_DATA)
|| ((anImsConnectUnicodeUsage & ApiProperties.IMS_CONNECT_UNICODE_USAGE_TRANCODE) == ApiProperties.IMS_CONNECT_UNICODE_USAGE_TRANCODE)) {
this.imsConnectUnicodeUsage = anImsConnectUnicodeUsage;
} else {
String validImsConnectUnicodeUsageString = "";
try {
validImsConnectUnicodeUsageString = ImsConnectErrorMessage
.getString(ImsConnectErrorMessage.VALID_PROPERTY_VALUE_IMSCONNECTUNICODEUSAGE);
} catch (Exception e1) {
// do nothing - this exception should never occur unless the API
// JAR is corrupted
}
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0030E, new Object[] {
"imsConnectUnicodeUsage",
String.valueOf(anImsConnectUnicodeUsage),
validImsConnectUnicodeUsageString });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0030E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in Connection.setImsConnectUnicodeUsage(byte). Exception thrown was: "
+ e.toString());
throw e;
}
}
/**
* Gets the IMS Connect timeout.
*
* @return an int
value containing the IMS Connect timeout
* property value
*/
public int getImsConnectTimeout() {
return imsConnectTimeout;
}
/**
* Sets the imsConnectTimeout property value. The imsConnectTimeout property
* specifies the time that IMS Connect will wait to receive a response from
* IMS to be returned to the client. This property is specified by the
* client on any input and updated by IMS Connect in the reply to the client
* with the actual IRM_TIMER value used for that interaction. The default
* value is IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_DEFAULT_VALUE
* (timeout determined by IMS Connect based on its defaults or configured
* TIMEOUT value).
*
* The following request types use the imsConnectTimeout to set the IMS
* Connect response timeout:
*
* - RESUME TPIPE
*
- ACK or NAK
*
- IMS transaction or IMS Command (both include data)
*
*
* For more details on the use of this property, see the topic "Time-out intervals on input messages" in the V10
* IMS Communications and Connections guide documentation.
*
* The valid values are:
*
* IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_DEFAULT_VALUE
- Use
* the IMS Connect TIMEOUT value as follows:
*
* - Use 0.25 second wait for ResumeTpipe non-single ACK/NAK calls
*
- Use 2 seconds for all RESUME_TPIPE calls
*
- Use IMS Connect's configured TIMEOUT value (or the default value if a
* TIMEOUT value has not been configured) for IMS transactions or IMS
* commands
*
* IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_NOWAIT_VALUE
- Use
* the IMS Connect-defined NOWAIT value as follows:
*
* - 2 second delay for:
*
* - RESUME_TPIPE request
*
- conversational iteration (with or without trancode)
*
- ACK or NAK associated with a conversational transaction
*
- non-conversational transaction
*
*
* - 0.25 second delay for:
*
* - ACK or NAK associated with a non-conversational Commit Mode 1, Sync
* level confirm transaction
*
- ACK or NAK associated with a RESUMETPIPE_AUTO or RESUMETPIPE_NOAUTO
*
- ACK or NAK associated with non-conversational Commit Mode 0, Sync
* Level Confirm transaction
*
*
* - 0 second delay for:
*
* - SENDONLY
*
- ACK or NAK associated with RESUMETPIPE_SINGLE_WAIT or
* RESUMETPIPE_SINGLE_NOWAIT
*
*
*
* IMS_CONNECT_TIMEOUT_WAIT_FOREVER
- Sets the timer to
* wait forever.
* - Specific time value between 10 milliseconds and 60 minutes (see
* following table for actual values used):
*
*
* Value specified
* Resulting IRM_TIMER value
*
*
* between 10 and 250 milliseconds, inclusive
* 0x01 - 0x19 representing timeout values between 10 and 250
* milliseconds inclusive, in 10 milliseconds increments
*
*
* between 300 and 950 milliseconds, inclusive
* 0x1A - 0x27 representing timeout values between 300 and 950
* milliseconds inclusive, in 50 milliseconds increments
*
*
* between 1,000 and 60,000 milliseconds, inclusive
* 0x28 - 0x63 representing timeout values between 1,000 and 60,000
* milliseconds (1 to 60 seconds) inclusive, in 1 second increments
*
*
* between 120,000 and 3,600,000 milliseconds, inclusive
* 0x64 - 0x9E representing timeout values between between 120,000 and
* 3,600,000 milliseconds (2 - 60 minutes) inclusive in 1 minute increments
*
*
* DEFAULT_IMS_CONNECT_TIMEOUT
- same as
* IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_DEFAULT_VALUE
*
*
* @param anImsConnectTimeout
* the imsConnectTimeout value to be used for this interaction.
* Use the constants defined in ApiProperties
to
* specify this parameter.
* @see ApiProperties#DEFAULT_IMS_CONNECT_TIMEOUT
* @see ApiProperties#IMS_CONNECT_TIMEOUT_100_MILLISECONDS
* @see ApiProperties#IMS_CONNECT_TIMEOUT_10_MILLISECONDS
* @see ApiProperties#IMS_CONNECT_TIMEOUT_10_MINUTES
* @see ApiProperties#IMS_CONNECT_TIMEOUT_10_SECONDS
* @see ApiProperties#IMS_CONNECT_TIMEOUT_15_MINUTES
* @see ApiProperties#IMS_CONNECT_TIMEOUT_1_HOUR
* @see ApiProperties#IMS_CONNECT_TIMEOUT_1_MINUTE
* @see ApiProperties#IMS_CONNECT_TIMEOUT_1_SECOND
* @see ApiProperties#IMS_CONNECT_TIMEOUT_20_MILLISECONDS
* @see ApiProperties#IMS_CONNECT_TIMEOUT_2_MINUTES
* @see ApiProperties#IMS_CONNECT_TIMEOUT_2_SECONDS
* @see ApiProperties#IMS_CONNECT_TIMEOUT_30_MINUTES
* @see ApiProperties#IMS_CONNECT_TIMEOUT_30_SECONDS
* @see ApiProperties#IMS_CONNECT_TIMEOUT_45_MINUTES
* @see ApiProperties#IMS_CONNECT_TIMEOUT_45_SECONDS
* @see ApiProperties#IMS_CONNECT_TIMEOUT_500_MILLISECONDS
* @see ApiProperties#IMS_CONNECT_TIMEOUT_50_MILLISECONDS
* @see ApiProperties#IMS_CONNECT_TIMEOUT_5_MINUTES
* @see ApiProperties#IMS_CONNECT_TIMEOUT_5_SECONDS
* @see ApiProperties#IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_DEFAULT_VALUE
* @see ApiProperties#IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_NOWAIT_VALUE
* @see ApiProperties#IMS_CONNECT_TIMEOUT_WAIT_FOREVER
* @see TmInteraction#getImsConnectTimeout()
*/
public void setImsConnectTimeout(int anImsConnectTimeout)
throws ImsConnectApiException {
boolean isValid = true;
if (this.imsConnectTimeout != anImsConnectTimeout) {
if (anImsConnectTimeout < 0) {
// check if it's a wait forever request
if (anImsConnectTimeout == TIMEOUT_WAIT_FOREVER) {
this.imsConnectConvertedTimeout = -1;
this.imsConnectTimeoutIndex = -1;
} else if (anImsConnectTimeout == IMS_CONNECT_TIMEOUT_USE_IMS_CONNECT_NOWAIT_VALUE) {
this.imsConnectConvertedTimeout = -23;
this.imsConnectTimeoutIndex = -23;
} else {
// executionTimeout is less than 0 but not -1 or -23, which
// is not
// supported so set timeout value to default
this.imsConnectConvertedTimeout = 0;
this.imsConnectTimeoutIndex = 0;
isValid = false;
}
} else if (anImsConnectTimeout == 0) {
this.imsConnectConvertedTimeout = 0;
this.imsConnectTimeoutIndex = 0;
} else if (anImsConnectTimeout <= 10) {
this.imsConnectConvertedTimeout = 10;
this.imsConnectTimeoutIndex = 1;
} else if (anImsConnectTimeout <= 250) {
this.imsConnectConvertedTimeout = (int) Math
.round(((double) anImsConnectTimeout) / 10) * 10;
this.imsConnectTimeoutIndex = this.imsConnectConvertedTimeout / 10;
} else if (anImsConnectTimeout <= 1000) {
this.imsConnectConvertedTimeout = (int) Math
.round(((double) anImsConnectTimeout) / 50) * 50;
this.imsConnectTimeoutIndex = ((this.imsConnectConvertedTimeout - 250) / 50) + 25;
} else if (anImsConnectTimeout <= 60000) {
this.imsConnectConvertedTimeout = (int) Math
.round(((double) anImsConnectTimeout) / 1000) * 1000;
this.imsConnectTimeoutIndex = ((this.imsConnectConvertedTimeout - 1000) / 1000) + 40;
} else if (anImsConnectTimeout <= 3600000) {
this.imsConnectConvertedTimeout = (int) Math
.round(((double) anImsConnectTimeout) / 60000) * 60000;
this.imsConnectTimeoutIndex = ((this.imsConnectConvertedTimeout - 60000) / 60000) + 99;
} else {
// executionTimeout is greater than 3600000, which is not
// supported
// set executionTimeout to default
anImsConnectTimeout = 0;
this.imsConnectConvertedTimeout = 0;
this.imsConnectTimeoutIndex = 0;
isValid = false;
}
if (!isValid) {
String validImsConnectTimeoutString = "";
try {
validImsConnectTimeoutString = ImsConnectErrorMessage
.getString(ImsConnectErrorMessage.VALID_PROPERTY_VALUE_IMSCONNECTTIMEOUT);
} catch (Exception e) {
}
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0030E, new Object[] {
"imsConnectTimeout",
new Integer(anImsConnectTimeout),
validImsConnectTimeoutString });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0030E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setImsConnectTimeout(int). Exception thrown is: "
+ e.toString());
throw e;
}
this.imsConnectTimeout = anImsConnectTimeout;
this.setRebuildMessage(true);
}
}
/**
* Get Converted IMS Connect timeout property value
*
* @return an int value representing the converted IMS Connect timeout
*/
public int getImsConnectConvertedTimeout() {
return this.imsConnectConvertedTimeout;
}
/**
* Get IMS Connect timeout index property value
*
* @return IMS Connect timeout index value
*/
public int getImsConnectTimeoutIndex() {
return this.imsConnectTimeoutIndex;
}
/**
* Get imsConnectUserMessageExitIdentifier property value which specifies
* which IMS Connect User Message Exit is to be used for this interaction
*
* @return an int value representing the IMS Connect User Message Exit to be
* used for this interaction
*/
public String getImsConnectUserMessageExitIdentifier() {
return this.imsConnectUserMessageExitIdentifier;
}
/**
* Sets the imsConnectUserMessageExitIdentifier property value which is used
* to determine which IMS Connect user message exit is to be used for this
* interaction after the complete input or output message has been received
* by IMS Connect. Only one value can be specified per interaction. The
* valid values, for which the following constants are defined in
* ApiProperties
, are:
*
* IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSSMPL0
-
* Use HWSSMPL0 user message exit (identifier is *SAMPLE*)
* IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSSMPL1
-
* Use HWSSMPL1 user message exit (identifier is *SAMPL1*)
* DEFAULT_IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER
- same
* as IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSSMPL1
*
* If a value for this property is not supplied by the client, the default
* value (IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSSMPL1
* ) will be used.
*
* For more details on the use of this property, see the topic
* "How IMS Connect Communicates with User Message Exits" in the V10
* IMS Exit Routine Reference documentation.
*
* @param anIConUserMessageExitIdentifier
* a String value containing the IMS Connect user message exit
* identifier to be used for this interaction. Use the constants
* defined in ApiProperties to specify this parameter.
* @see ApiProperties#IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSSMPL0
* @see ApiProperties#IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSSMPL1
* @see TmInteraction#getImsConnectUserMessageExitIdentifier()
*/
public void setImsConnectUserMessageExitIdentifier(
String anImsConnectUserMessageExitIdentifier)
throws ImsConnectApiException {
if (anImsConnectUserMessageExitIdentifier.length() > MAX_LEN_IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER) {
String errMsg = ImsConnectErrorMessage
.getString(
ImsConnectErrorMessage.HWS0026E,
new Object[] {
anImsConnectUserMessageExitIdentifier,
String
.valueOf(MAX_LEN_IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER) });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0026E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setImsConnectUserMessageExitIdentifier(String). Exception thrown was: "
+ e.toString());
throw e;
} else {
if ((anImsConnectUserMessageExitIdentifier.trim().equals(""))) // all
// blanks
// is
// not
// a
// valid
// imsConnectUserMessageExitIdentifier
// value
// !(PropertiesFileLoader.isValidHostStyleName(anImsConnectUserMessageExitIdentifier)))
// // identifier does not have to be a host-style name (*SAMPLE* -
// *'s are not valid host-style name characters)
{
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0029E, new Object[] {
"imsConnectUserMessageExitIdentifier",
anImsConnectUserMessageExitIdentifier });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0029E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setImsConnectUserMessageExitIdentifier(String). Exception thrown was: "
+ e.toString());
throw e;
}
}
if (imsConnectUserMessageExitIdentifier != anImsConnectUserMessageExitIdentifier) {
imsConnectUserMessageExitIdentifier = anImsConnectUserMessageExitIdentifier;
this.setRebuildMessage(true);
this.updateIrmId = true;
}
if (this.imsConnectUserMessageExitIdentifier == ApiProperties.IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSSMPL1 || this.imsConnectUserMessageExitIdentifier == ApiProperties.IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSDPWR1) {
this.responseIncludesLlll = ApiProperties.RESPONSE_DOES_INCLUDE_LLLL;
}
else if (this.imsConnectUserMessageExitIdentifier == ApiProperties.IMS_CONNECT_USER_MESSAGE_EXIT_IDENTIFIER_FOR_HWSSMPL0) {
this.responseIncludesLlll = ApiProperties.RESPONSE_DOES_NOT_INCLUDE_LLLL;
}
}
/**
* Gets IMS Datastore name.
*
* @return a String
value containing the Datastore name
* property value
*/
public String getImsDatastoreName() {
return this.imsDatastoreName;
}
/**
* Sets the imsDatastoreName property value. The imsDatastoreName property
* specifies the target IMS datastore name (a string of 1 to 8 uppercase
* alphanumeric (A through Z, 0 to 9) or special (@, #, $) characters
* identifying the IMS destination ID as defined in a DATASTORE statement in
* the IMS Connect configuration member. The client or the IMS Connect user
* message exit must provide a valid value for this property. Neither IMS
* Connect nor the IMS Connect user message exit validates the
* imsDatastoreName value. Instead, it is copied directly into the
* IRM_IMSDESTID field. The unvalidated imsDatastoreName value will also be
* copied by the IMS Connect user message exit into the OMUSR_DESTID field
* in the OTMA header. If a datastore by that name cannot be found, IMS
* Connect will return a datastore not found error (Return Code 4, Reason
* Code NFNDDST) to the client. If the datastore is found but is currently
* closed, IMS Connect will return a datastore closed error (Return Code 12,
* Reason Code DSCLOSE) to the client.
*
* @param aDataStoreName
* the IMS datastore name to be used for this interaction.
* @see TmInteraction#getImsDatastoreName()
*/
public void setImsDatastoreName(String anImsDatastoreName)
throws ImsConnectApiException {
if ((anImsDatastoreName).trim().equals("")
|| (anImsDatastoreName == null)) // all blanks is a valid
// anImsDatastoreName
{
anImsDatastoreName = EIGHT_BLANKS;
} else if (anImsDatastoreName.length() > MAX_LEN_IMS_DATASTORE_NAME) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0026E, new Object[] {
anImsDatastoreName, "imsDatastoreName",
String.valueOf(MAX_LEN_IMS_DATASTORE_NAME) });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0026E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setImsDatastoreName(String). Exception thrown was: "
+ e.toString());
throw e;
} else if (!(PropertiesFileLoader
.isValidHostStyleName(anImsDatastoreName))) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0029E, new Object[] {
"imsDatastoreName", anImsDatastoreName });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0029E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setImsDatastoreName(String). Exception thrown was: "
+ e.toString());
throw e;
}
if (this.imsDatastoreName != anImsDatastoreName) {
this.imsDatastoreName = anImsDatastoreName;
this.setRebuildMessage(true);
this.updateIrmDestId = true;
}
}
/**
* Get inputMessageDataSegmentsIncludeLlzzAndTrancode property value
*
* @return a boolean value representing whether or not the data segments in
* the input and output messages includes an LLZZ prefix
*/
public boolean isInputMessageDataSegmentsIncludeLlzzAndTrancode() {
return this.inputMessageDataSegmentsIncludeLlzzAndTrancode;
}
/**
* Sets the inputMessageDataSegmentsIncludeLlzzAndTrancode property value to
* be used for this interaction. This property is used to tell the API
* whether the client application code has already included an LLZZ value
* and a trancode value in the message data provided to the InputMessage
* object. When the inputMessageDataSegmentsIncludeLlzzAndTrancode property
* is set to true
, the API will interpret the first four bytes
* of the provided input message data as an LLZZ value and will not
* insert a trancode into the input message data byte array to be sent to
* IMS Connect (on the assumption that the trancode is in the provided input
* message data.)
*
* @param anInputMessageSegmentsIncludeLlzzAndTrancode
* a boolean value representing whether or not the data segments
* in the input messages include an LLZZ prefix and a trancode if
* required
* @see TmInteraction#getInputMessageSegmentsIncludeLlzzAndTrancode()
*/
public void setInputMessageDataSegmentsIncludeLlzzAndTrancode(
boolean anInputMessageDataSegmentsIncludeLlzzAndTrancode) {
if (this.inputMessageDataSegmentsIncludeLlzzAndTrancode != anInputMessageDataSegmentsIncludeLlzzAndTrancode) {
inputMessageDataSegmentsIncludeLlzzAndTrancode = anInputMessageDataSegmentsIncludeLlzzAndTrancode;
this.inputMsg
.setInputMessageDataSegmentsIncludeLlzzAndTrancode(anInputMessageDataSegmentsIncludeLlzzAndTrancode);
this.rebuildMessage = true;
}
}
/**
* Get inputMessageOptions property value
*
* @return Message type
*/
public int getInputMessageOptions() {
return this.inputMessageOptions;
}
/**
* Sets the InputMessageOptions property value which specifies the
* characteristics of the input message byte array supplied by the client.
* Multiple values, if specified, are logically OR'd together. The valid
* values, for which the following constants are defined in
* ApiProperties
, are:
*
* INPUT_MESSAGE_OPTIONS_NO_OPTIONS
- Hex value of 0X00. No
* input message options specified.
* INPUT_MESSAGE_OPTIONS_EBCDIC
- Hex value of 0X40. Input
* message is in EBCDIC. No translation from ASCII to EBCDIC required in the
* IMS Connect user message exit.
* DEFAULT_INPUT_MESSAGE_OPTIONS
- same as
* INPUT_MESSAGE_OPTIONS_NO_OPTIONS
*
*
* If a value for this property is not supplied by the client, the default
* value (INPUT_MESSAGE_OPTIONS_NO_OPTIONS
) will be used.
*
* @param anInputMessageOptions
* The inputMessageOptions to set. Use the constants defined in
* ApiProperties to specify this parameter.
* @see ApiProperties#INPUT_MESSAGE_OPTIONS_NO_OPTIONS
* @see ApiProperties#INPUT_MESSAGE_OPTIONS_EBCDIC
* @see TmInteraction#getInputMessageOptions()
*/
public void setInputMessageOptions(int anInputMessageOptions)
throws ImsConnectApiException {
if ((((anInputMessageOptions & ApiProperties.INPUT_MESSAGE_OPTIONS_NO_OPTIONS) == ApiProperties.INPUT_MESSAGE_OPTIONS_NO_OPTIONS) || ((anInputMessageOptions & ApiProperties.INPUT_MESSAGE_OPTIONS_EBCDIC) == ApiProperties.INPUT_MESSAGE_OPTIONS_EBCDIC))
&& ((anInputMessageOptions & ~ApiProperties.INPUT_MESSAGE_OPTIONS_EBCDIC) == 0)) {
this.inputMessageOptions = anInputMessageOptions;
this.setRebuildMessage(true);
} else {
String validInputMessageOptionsString = "";
try {
validInputMessageOptionsString = ImsConnectErrorMessage
.getString(ImsConnectErrorMessage.VALID_PROPERTY_VALUE_INPUTMESSAGEOPTIONS);
} catch (Exception e1) {
// do nothing - this exception should never occur unless the API
// JAR is corrupted
}
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0030E, new Object[] {
"inputMessageOptions",
String.valueOf(anInputMessageOptions),
validInputMessageOptionsString });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0030E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in Connection.setSocketType(byte). Exception thrown was: "
+ e.toString());
throw e;
}
}
/**
* Gets the interaction timeout.
*
* @return an int
value containing the interactionTimeout
* property value
*/
public int getInteractionTimeout() {
return interactionTimeout;
}
/**
* Sets the interactionTimeout property value. The interactionTimeout
* property specifies the time that the IMS Connect API will wait to receive
* a response from IMS Connect to be returned to the client. This property
* is specified by the client on any input. The interactionTimeout is
* specified by the client using different values than the
* imsConnectTimeout. This is because the imsConnectTimeout must be set
* using the time encoding values used by IMS Connect while the
* interactionTimeout is used internally and does not need to be encoded.
*
* Note that the interactionTimeout value should always be set to a larger
* value than the imsConnectTimeout value. Otherwise, the interactionTimeout
* will mask the imsConnectTimeout meaning that the interactionTimeout would
* be triggered in situations where the problem should have caused the
* imsConnectTimeout to be triggered.
*
* Constants are provided in ApiProperties
for specifying the
* interactionTimeout value. The constant
* INTERACTION_TIMEOUT_MAX
represents 2,147,483,647 msec or
* approximately 3.5 weeks. If a value for this property is not supplied by
* the client, the default value (
* INTERACTION_TIMEOUT_WAIT_FOREVER
) will be used.
*
* @param anInteractionTimeout
* the interactionTimeout to be used for this interaction. Use
* the constants defined in ApiProperties
to specify
* this parameter.
* @see ApiProperties#DEFAULT_INTERACTION_TIMEOUT
* @see ApiProperties#TIMEOUT_100_MILLISECONDS
* @see ApiProperties#TIMEOUT_10_MILLISECONDS
* @see ApiProperties#TIMEOUT_10_MINUTES
* @see ApiProperties#TIMEOUT_10_SECONDS
* @see ApiProperties#TIMEOUT_12_HOURS
* @see ApiProperties#TIMEOUT_1_DAY
* @see ApiProperties#TIMEOUT_1_HOUR
* @see ApiProperties#TIMEOUT_1_MILLISECOND
* @see ApiProperties#TIMEOUT_1_MINUTE
* @see ApiProperties#TIMEOUT_1_SECOND
* @see ApiProperties#TIMEOUT_1_WEEK
* @see ApiProperties#TIMEOUT_200_MILLISECONDS
* @see ApiProperties#TIMEOUT_20_MILLISECONDS
* @see ApiProperties#TIMEOUT_2_DAYS
* @see ApiProperties#TIMEOUT_2_HOURS
* @see ApiProperties#TIMEOUT_2_MILLISECONDS
* @see ApiProperties#TIMEOUT_2_MINUTES
* @see ApiProperties#TIMEOUT_2_SECONDS
* @see ApiProperties#TIMEOUT_2_WEEKS
* @see ApiProperties#TIMEOUT_30_MINUTES
* @see ApiProperties#TIMEOUT_30_SECONDS
* @see ApiProperties#TIMEOUT_45_MINUTES
* @see ApiProperties#TIMEOUT_45_SECONDS
* @see ApiProperties#TIMEOUT_4_HOURS
* @see ApiProperties#TIMEOUT_500_MILLISECONDS
* @see ApiProperties#TIMEOUT_50_MILLISECONDS
* @see ApiProperties#TIMEOUT_5_MINUTES
* @see ApiProperties#TIMEOUT_8_HOURS
* @see ApiProperties#TIMEOUT_5_SECONDS
* @see ApiProperties#TIMEOUT_5_DAYS
* @see ApiProperties#INTERACTION_TIMEOUT_MAX
* @see ApiProperties#INTERACTION_TIMEOUT_WAIT_FOREVER
* @see TmInteraction#getInteractionTimeout()
*/
public void setInteractionTimeout(int anInteractionTimeout)
throws ImsConnectApiException {
if (this.interactionTimeout != anInteractionTimeout) {
if (anInteractionTimeout > 0 || anInteractionTimeout == -1) {
this.interactionTimeout = anInteractionTimeout;
this.setRebuildMessage(true);
} else {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0007E, new Object[] {
"TmInteractionImpl.setInteractionTimeout(int)",
anInteractionTimeout });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0007E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setInteractionTimeout(). Exception thrown was: "
+ e.toString());
throw e;
}
}
}
/**
* Gets the type of interaction with IMS Connect to be performed.
*
* @return a String
value containing the
* InteractionTypeDescription property value
* @see ApiProperties#INTERACTION_TYPE_DESC_ACK
* @see ApiProperties#INTERACTION_TYPE_DESC_CANCELTIMER
* @see ApiProperties#INTERACTION_TYPE_DESC_ENDCONVERSATION
* @see ApiProperties#INTERACTION_TYPE_DESC_NAK
* @see ApiProperties#INTERACTION_TYPE_DESC_RECEIVE
* @see ApiProperties#INTERACTION_TYPE_DESC_RESUMETPIPE
* @see ApiProperties#INTERACTION_TYPE_DESC_SENDONLY
* @see ApiProperties#INTERACTION_TYPE_DESC_SENDONLYACK
* @see ApiProperties#INTERACTION_TYPE_DESC_SENDRECV
*/
public String getInteractionTypeDescription() {
return this.interactionTypeDescription;
}
/**
* Sets the interactionTypeDescription property value which specifies the
* function to be performed in IMS Connect and/or OTMA in this interaction.
* Only one function at a time can be specified in a message. The valid
* values, for which the following constants are defined in
* ApiProperties
, are:
*
* INTERACTION_TYPE_DESC_ACK
- Positive acknowledgment
* (IRM_F4 = 'A'). Must be sent to IMS Connect with no application data
* segment in the message. Used to accept the IMS response to an IMS
* transaction request message in which the sync level was set to CONFIRM.
* INTERACTION_TYPE_DESC_CANCELTIMER
- Cancel wait for
* response from IMS (IRM_F4 = 'C'). Must be sent to IMS Connect with no
* application data segment in the message. Used to cancel the current CONN
* state (wait for response from IMS) on another connection which is using
* the same client ID as this connection and is connected to the same port
* on this IMS Connect. Then IMS Connect will return that other connection
* to RECV state and send a canceltimer complete message to the client and
* disconnect this connection.
* INTERACTION_TYPE_DESC_END_CONVERSATION
- End
* conversation associated with this convID and/or connection (IRM_F4 = 'D')
* INTERACTION_TYPE_DESC_NAK
- Negative acknowledgment
* (IRM_F4 = 'N'). Must be sent to IMS Connect with no application data
* segment in the message. Used to reject the IMS response to an IMS
* transaction request message in which the sync level was set to CONFIRM.
* INTERACTION_TYPE_DESC_RECEIVE
- Receive-only request to
* receive a single response message from IMS. This corresponds to an
* internal API interaction type that causes the API to do only a receive on
* the TCP/IP socket for the current interaction. It does not involve
* sending any type of request to IMS Connect, so there is no IRM created
* and therefore, no flag setting associated with this interaction type.
* INTERACTION_TYPE_DESC_RESUMETPIPE
- Request asynchronous
* output (IRM_F4 = 'R'). Must be sent to IMS Connect with no application
* data segment in the message. Used to request asynchronous output data
* from the OTMA Tpipe whose name is the same as the client ID for this
* connection. The RESUMETPIPE must execute on a transaction socket or
* persistent socket using commit mode zero.
* INTERACTION_TYPE_DESC_SENDONLY
- Send-only IMS
* transaction request with no response from IMS expected to be returned
* (IRM_F4 = 'S'). Used to send a transaction request for a non-response
* mode transaction to IMS Connect and IMS. The application data segment
* must contain the transaction code and input data required for that
* transaction. The DFS2082 message that would normally be returned to the
* client if the host application terminates without issuing an insert to
* the IOPCB is suppressed for SENDONLY requests. SENDONLY requests must
* execute as commit mode zero. Any response resulting from a SENDONLY
* function will be queued on an asynchronous hold queue associated with
* Tpipe used by the client application and can be retrieved later by
* submitting a RESUMETPIPE messageFunction.
* INTERACTION_TYPE_DESC_SENDONLYACK
- Send-only with
* acknowledgement IMS transaction request (IRM_F4 = 'K'). Used to send a
* transaction request for a non-response mode transaction to IMS Connect
* and IMS. The application data segment must contain the transaction code
* and input data required for that transaction. The DFS2082 message that
* would normally be returned to the client if the host application
* terminates without issuing an insert to the IOPCB is suppressed for
* SENDONLY requests. SENDONLY requests must execute as commit mode zero.
* The INTERACTION_TYPE_SENDONLYACK
function differs from the
* INTERACTION_TYPE_SENDONLY
function in that the client
* application receives an ACK response message from OTMA for each input
* message successfully enqueued by IMS. All output generated by the
* INTERACTION_TYPE_SENDONLYACK
interaction is sent to the
* asynchronous hold queue.
* INTERACTION_TYPE_DESC_SENDONLYXCFORDEREDDELIVERY
-
* Send-only with XCF ordered delivery IMS transaction request (IRM_F4 =
* 'S', IMR_F3 = 0x10). Used to send a transaction request for a
* non-response mode transaction to IMS Connect and IMS. The application
* data segment must contain the transaction code and input data required
* for that transaction. The DFS2082 message that would normally be returned
* to the client if the host application terminates without issuing an
* insert to the IOPCB is suppressed for SENDONLY requests. SENDONLY
* requests must execute as commit mode zero. The
* INTERACTION_TYPE_DESC_SENDONLYXCFORDEREDDELIVERY
function is
* similar to the INTERACTION_TYPE_SENDONLY
function except
* that the client application is guaranteed that all input messages are
* delivered by XCF to IMS OTMA in the order in which they are received by
* XCF. Note that the ordered delivery of input messages does not imply that
* the response messages will be returned to the client application in that
* order. This is because the order of delivery of response messages is
* dependent not only on the order that the input messages are received, but
* also on the time that it takes to process each message which will vary
* due to a number of factors including the transaction execution time which
* varies from one transaction to another. All output generated by the
* INTERACTION_TYPE_DESC_SENDONLYXCFORDEREDDELIVERY
interaction
* is sent to the asynchronous hold queue.
* INTERACTION_TYPE_DESC_SENDRECV
- Send-Receive IMS
* transaction request with a response from IMS expected to be returned
* IRM_F4 = ' ' (single blank character)). A blank character (0X40 for
* EBCDIC messages, 0X20 for ASCII messages) is used to send SENDRECV
* requests for response-mode IMS transaction requests to IMS Connect and
* IMS. The application data segment must contain the transaction code and
* input data required for that transaction for IMS non-conversational
* transactions or for the first iteration of an IMS conversational
* transaction. The application data segment for subsequent iterations of an
* IMS conversational transaction does not contain a transaction code but
* rather contains the input data only. This is the default value.
* DEFAULT_INTERACTION_DESC_TYPE
- same as
* INTERACTION_TYPE_DESC_SENDRECV
*
* If a value for this property is not supplied by the client, the default
* value (INTERACTION_TYPE_DESC_SENDRECV
) will be used.
*
* @param anInterTypeDesc
* type of interaction to be executed. Use the constants defined
* in ApiProperties to specify this parameter.
* @see ApiProperties#INTERACTION_TYPE_DESC_ACK
* @see ApiProperties#INTERACTION_TYPE_DESC_CANCELTIMER
* @see ApiProperties#INTERACTION_TYPE_DESC_ENDCONVERSATION
* @see ApiProperties#INTERACTION_TYPE_DESC_NAK
* @see ApiProperties#INTERACTION_TYPE_DESC_RECEIVE
* @see ApiProperties#INTERACTION_TYPE_DESC_RESUMETPIPE
* @see ApiProperties#INTERACTION_TYPE_DESC_SENDONLY
* @see ApiProperties#INTERACTION_TYPE_DESC_SENDONLYACK
* @see ApiProperties#INTERACTION_TYPE_DESC_SENDONLYXCFORDEREDDELIVERY
* @see ApiProperties#INTERACTION_TYPE_DESC_SENDRECV
* @see TmInteraction#getInteractionTypeDescription()
*/
public void setInteractionTypeDescription(String anInterTypeDesc)
throws ImsConnectApiException {
if (allValidInteractionTypeDescriptionsString.indexOf(anInterTypeDesc) == -1) {
String validInteractionTypeDescription = "";
try {
validInteractionTypeDescription = ImsConnectErrorMessage
.getString(com.ibm.ims.connect.ImsConnectErrorMessage.VALID_PROPERTY_VALUE_INTERACTIONTYPEDESCRIPTION);
} catch (Exception e) {
}
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0030E, new Object[] {
"interactionTypeDescription", anInterTypeDesc,
validInteractionTypeDescription });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0030E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setInteractionTypeDescription(): ["
+ e.toString() + "]");
throw e;
}
//if (this.interactionTypeDescription != anInterTypeDesc) {
if (!(this.interactionTypeDescription.equalsIgnoreCase( anInterTypeDesc))) {
this.interactionTypeDescription = anInterTypeDesc;
this.setRebuildMessage(true);
this.updateIrmInteractionType = true;
if (anInterTypeDesc == ApiProperties.INTERACTION_TYPE_DESC_RESUMETPIPE) {
recvAfterResumeTpipe = true;
} else {
recvAfterResumeTpipe = false;
}
}
}
/**
* Gets the LTERM override name.
*
* @return a String
value containing the ltermOverrideName
* property value
*/
public String getLtermOverrideName() {
return this.ltermOverrideName;
}
/**
* Sets the ltermOverrideName property value to a valid MVS-style name which
* is a string of 1 to 8 uppercase alphanumeric (A through Z, 0 to 9) or
* special (@, #, $) characters. This field can be set to a valid name or to
* blanks. The ltermOverrideName is copied by the IMS Connect user message
* exit to the OMHDRLTM field in the OTMA header. The specified IMS Lterm
* override name can also be ignored and replaced by a value dynamically or
* statically determined in the IMS Connect user message exit. Neither IMS
* Connect nor the IMS Connect user message exit validates the
* ltermOverrideName value.
*
* If there is a non-blank, non-null Lterm override name value after the
* message is processed in the IMS Connect user exit, OTMA will place that
* value in the IOPCB LTERM field. If instead, the Lterm override name value
* is all blanks or null, OTMA will place the IMS Connect-defined Tpipe name
* in the IOPCB LTERM field. The TPIPE name is set to the CLIENT ID for
* commit mode 0 interactions and set to the PORT number for commit mode 1
* interactions.
*
* If you use the LTERM value in the IOPCB to make logic decisions, you must
* be careful to observe the naming conventions of the IOPCB LTERM name.
*
* Note that the default value for ltermOverrideName is a string of eight
* blank characters, and the ltermOverrideName property must be changed to a
* valid LTerm override name before use.
*
* @param termOverrideName
* the ltermOverrideName to set.
* @see TmInteraction#getLtermOverrideName()
*/
public void setLtermOverrideName(String anLtermOverrideName)
throws ImsConnectApiException {
if ((anLtermOverrideName).trim().equals("")
|| (anLtermOverrideName == null)) // all blanks is a valid
// ltermOverrideName value
{
anLtermOverrideName = EIGHT_BLANKS;
} else if (anLtermOverrideName.length() > MAX_LEN_LTERM_OVERRIDE_NAME) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0026E, new Object[] {
anLtermOverrideName,
String.valueOf(MAX_LEN_LTERM_OVERRIDE_NAME) });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0026E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setLtermOverrideName(String). Exception thrown was: "
+ e.toString());
throw e;
} else {
if (!(PropertiesFileLoader
.isValidHostStyleName(anLtermOverrideName))) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0029E, new Object[] {
"ltermOverrideName", anLtermOverrideName });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0029E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setLtermOverrideName(String). Exception thrown was: "
+ e.toString());
throw e;
}
}
if (this.ltermOverrideName != anLtermOverrideName) {
this.ltermOverrideName = anLtermOverrideName;
this.setRebuildMessage(true);
this.updateIrmLTerm = true;
}
}
/**
* @return the otmaTransactionExpiration
*/
public boolean isOtmaTransactionExpiration() {
return this.otmaTransactionExpiration;
}
/**
* @param otmaTransactionExpiration
* the otmaTransactionExpiration to set
*/
public void setOtmaTransactionExpiration(boolean otmaTransactionExpiration) {
this.otmaTransactionExpiration = otmaTransactionExpiration;
this.setRebuildMessage(true);
}
/**
* Gets a boolean
value representing whether or not the API
* should wait for a timeout response after an ACK of a CM0 response if the
* target IMS Connect supports CM0 ACK No Wait.
*
* @return a boolean
value containing the useCM0AckNoWait
* property value
* @see ApiProperties#DO_USE_CM0_ACK_NOWAIT
* @see ApiProperties#DO_NOT_USE_CM0_ACK_NOWAIT
* @see ApiProperties#DEFAULT_USE_CM0_ACK_NOWAIT
* @see TmInteraction#setUseCM0AckNoWait()
* @return the useCM0AckNoWait value
*/
public boolean isUseCM0AckNoWait() {
return this.useCM0AckNoWait;
}
/**
* Sets the useCM0AckNoWait property that controls whether or not user wants
* the API to wait for a timeout response after an ACK of a CM0 (Commit Mode
* 0) response. This property is used to shield the API user from the need
* to understand the details for using IMS Connect's CM0 ACK (and NAK) No
* Wait protocol.
*
* If the useCM0AckNoWait property is turned on (set to true
)
* and IMS Connect has indicated in a CSM for the response that you are
* acknowledging that it supports CM0 ACK No Wait, the API will set the
* appropriate values in the IRM to tell IMS Connect that it is executing a
* CM0 ACK No Wait and therefore will not be waiting for a timeout response
* after sending in the ACK.
*
* If the useCM0AcNakNoWait property is turned off (set to
* false
) the API will not override the the "normal" IRM values
* with the values needed for a CM0 Ack No Wait message and will do a
* receive for the timeout response from IMS Connect after sending in the
* ACK.
*
* The valid values, for which the following constants are defined in
* ApiProperties
, are:
*
* DO_USE_CM0_ACK_NOWAIT
(true)
* DO_NOT_USE_CM0_ACK_NOWAIT
(false)
* DEFAULT_USE_CM0_ACK_NOWAIT
- same as
* DO_USE_CM0_ACK_NOWAIT
(true)
*
* If a value for this property is not supplied by the client, the default
* value (DO_USE_CM0_ACK_NOWAIT
) will be used.
*
* For more details on the use of this property, see the topic
* "IMS Connect CM0 Ack No Wait support??????" in the
* V11 IMS Communications and Connections documentation.
*
* @see ApiProperties#DO_USE_CM0_ACK_NOWAIT
* @see ApiProperties#DO_NOT_USE_CM0_ACK_NOWAIT
* @see ApiProperties#DEFAULT_USE_CM0_ACK_NOWAIT
* @see TmInteraction#isUseCM0AckNoWait()
* @param useCM0AckNoWait
* the useCM0AckNoWait to set
*/
public void setUseCM0AckNoWait(boolean aUseCM0AckNoWait) {
this.useCM0AckNoWait = aUseCM0AckNoWait;
this.setRebuildMessage(true);
}
/**
* @return the cM0AckNoWaitCanBeUsed
*/
public boolean isCM0AckNoWaitCanBeUsed() {
return this.cM0AckNoWaitCanBeUsed;
}
/**
* @param aCM0AckNoWaitCanBeUsed
* the cM0AckNoWaitCanBeUsed to set
*/
protected void setCM0AckNoWaitCanBeUsed(boolean aCM0AckNoWaitCanBeUsed) {
this.cM0AckNoWaitCanBeUsed = aCM0AckNoWaitCanBeUsed;
this.setRebuildMessage(true);
}
/**
* Gets a boolean
value representing whether or not the API
* should request that IMS Connect return a response message containing a
* DFS2082 instead of a timeout error response when a CM0 SendReceive
* interaction times out in IMS Connect.
*
* @return a boolean
value containing the
* returnDFS2082AfterCM0SendRecvNoResponse property value
* @see ApiProperties#DO_RETURN_DFS2082_AFTER_CM0_SENDRECV_NO_RESPONSE
* @see ApiProperties#DO_NOT_RETURN_DFS2082_AFTER_CM0_SENDRECV_NO_RESPONSE
* @see ApiProperties#DEFAULT_RETURN_DFS2082_AFTER_CM0_SENDRECV_NO_RESPONSE
* @see TmInteraction#setReturnDFS2082AfterCM0SendRecvNoResponse()
*/
public boolean isReturnDFS2082AfterCM0SendRecvNoResponse() {
return this.returnDFS2082AfterCM0SendRecvNoResponse;
}
/**
* Sets the returnDFS2082AfterCM0SendRecvNoResponse property value with a
* boolean
value representing whether or not the API should
* request that IMS Connect return a response message containing a DFS2082
* instead of a timeout error response when a CM0 SendReceive interaction
* times out in IMS Connect.
*
* @param aReturnDFS2082AfterCM0SendRecvNoResponse
* the returnDFS2082AfterCM0SendRecvNoResponse to set
* @see ApiProperties#DO_RETURN_DFS2082_AFTER_CM0_SENDRECV_NO_RESPONSE
* @see ApiProperties#DO_NOT_RETURN_DFS2082_AFTER_CM0_SENDRECV_NO_RESPONSE
* @see ApiProperties#DEFAULT_RETURN_DFS2082_AFTER_CM0_SENDRECV_NO_RESPONSE
* @see TmInteraction#isReturnDFS2082AfterCM0SendRecvNoResponse()
*/
public void setReturnDFS2082AfterCM0SendRecvNoResponse(
boolean aReturnDFS2082AfterCM0NoResponse) {
this.returnDFS2082AfterCM0SendRecvNoResponse = aReturnDFS2082AfterCM0NoResponse;
this.setRebuildMessage(true);
}
/**
* Sets the purgeUndeliverableOutput property value. The valid values, for
* which the following constants are defined in ApiProperties
,
* are:
*
* PURGE_UNDELIVERABLE_OUTPUT
- Boolean value true. Discard
* undeliverable primary and/or secondary commit mode 0 output (maps to
* IRM_F3_PURGE value of 0X04). Tells IMS Connect and OTMA to discard
* undeliverable primary and/or secondary commit mode 0 output resulting
* from a transaction.
* DO_NOT_PURGE_UNDELIVERABLE_OUTPUT
- Boolean value false.
* Requeue undeliverable primary and/or secondary commit mode 0 output (no
* bits set in IRM_F3). Tells IMS Connect and OTMA to requeue undeliverable
* primary and/or secondary commit mode 0 output resulting from a
* transaction. Causes undelivered output indicator to not be returned to
* IMS Connect from the user message exit.
* DEFAULT_PURGE_UNDELIVERABLE_OUTPUT
- same as
* DO_NOT_PURGE_UNDELIVERABLE_OUTPUT
*
* If a value for this property is not supplied by the client, the default
* value (DO_NOT_PURGE_UNDELIVERABLE_OUTPUT
) will be used. If
* you specify true values for both purgeUndeliverableOutput and
* rerouteUndeliveredOutput at the same time, undeliverable primary and/or
* secondary commit mode 0 output resulting from a transaction is neither
* purged nor rerouted. In this case, OTMA stores the output onto the
* asynchronous message hold queue of the inputting Tpipe (clientID Tpipe)
* and issues a DFS2407W warning message. For more details on the purge
* undeliverable property, see the topic
* "Purging undeliverable commit-then-send output" in
* the V10 Communications and Connections guide documentation.
*
* @param aPurgeUndeliverableOutput
* the rerouteUndeliverableOutput to set. Use the constants
* defined in ApiProperties to specify this parameter.
* @see ApiProperties#PURGE_UNDELIVERABLE_OUTPUT
* @see ApiProperties#DO_NOT_PURGE_UNDELIVERABLE_OUTPUT
* @see TmInteraction#getPurgeUndeliverableOutput()
*/
public void setPurgeUndeliverableOutput(boolean aPurgeUndeliverableOutput)
throws ImsConnectApiException {
if ((this.rerouteUndeliverableOutput) && (aPurgeUndeliverableOutput)) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0027E,
new Object[] { "purgeUndeliverableOutput" });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0027E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setPurgeUndeliverableOutput(boolean). Exception thrown was: "
+ e.toString());
throw e;
} else if (this.purgeUndeliverableOutput != aPurgeUndeliverableOutput) {
this.purgeUndeliverableOutput = aPurgeUndeliverableOutput;
this.setRebuildMessage(true);
}
}
/**
* Gets the RACF APPL name.
*
* @return a String
value containing the RACF APPL name
* property value
*/
public String getRacfApplName() {
return this.racfApplName;
}
/**
* @param savedInputMessageDataSegmentsIncludeLlzzAndTrancode
* The savedInputMessageDataSegmentsIncludeLlzzAndTrancode to
* set.
*/
/*
* private void setSavedInputMessageDataSegmentsIncludeLlzzAndTrancode(
* boolean savedInputMessageSegmentsIncludeLlzzAndTrancode) {
* this.savedInputMessageDataSegmentsIncludeLlzzAndTrancode =
* savedInputMessageSegmentsIncludeLlzzAndTrancode; }
*
*
* /** Sets the racfApplName property value. The racfApplName property is a
* string of 1 to 8 uppercase alphanumeric (A through Z, 0 to 9) or special
* (@, #, $) characters and is intended to match a RACF APPL name defined to
* RACF in a PTKTDATA definition. If the racfApplName value specified is
* fewer than 8 characters, the racfApplName value is right-padded with
* blanks to 8 characters by the IMS Connect API before being copied to the
* IRM_APPL_NM field. If the racfApplName value is 8 0X00s or 8 blanks after
* padding with blanks, the IMS Connect user message exit will use the
* default value from the IMS Connect configuration member. If there is no
* value specified in the IMS Connect configuration, 8 blanks will be copied
* to the IRM_APPL_NM field. If the racfApplName value is not 8 0X00s or 8
* blanks after padding, it is passed back to IMS Connect by the IMS Connect
* user message exit without first checking the validity. IMS Connect will
* then use this racfApplName value in the RACROUTE VERIFY call where it is
* validated before it is used. Note that the DEFAULT_RACF_APPL_NAME is a
* String of eight blank characters, and the racfApplName property must be
* changed during runtime to the appropriate RACF or SAF APPLID used by the
* target IMS Connect or IMS if the client wishes to submit a request
* containing a passticket rather than a password.
*
* @param aRacfApplName the RACF application name to be used for this
* interaction
*
* @see TmInteraction#getRacfApplName()
*/
public void setRacfApplName(String aRacfApplName)
throws ImsConnectApiException {
if ((aRacfApplName).trim().equals("") || (aRacfApplName == null)) // all
// blanks
// is
// a
// valid
// aRacfApplName
{
aRacfApplName = EIGHT_BLANKS;
} else if (aRacfApplName.length() > MAX_LEN_RACF_GROUP_NAME) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0026E, new Object[] {
aRacfApplName, "racfApplName",
String.valueOf(MAX_LEN_RACF_GROUP_NAME) });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0026E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setRacfApplName(String). Exception thrown was: "
+ e.toString());
throw e;
} else if (!(PropertiesFileLoader.isValidHostStyleName(aRacfApplName))) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0029E, new Object[] {
"racfApplName", aRacfApplName });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0029E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setRacfApplName(String). Exception thrown was: "
+ e.toString());
throw e;
}
if (this.racfApplName != aRacfApplName) {
this.racfApplName = aRacfApplName;
this.setRebuildMessage(true);
this.updateIrmRacfApplName = true;
}
}
/**
* Gets the RACF Group name.
*
* @return a String
value containing the RACF group name
* property value
*/
public String getRacfGroupName() {
return this.racfGroupName;
}
/**
* Sets the racfGroupName property value. The racfGroupName property
* specifies the RACF group name which is a string of 1 to 8 uppercase
* alphanumeric (A through Z, 0 to 9) or special (@, #, $) characters.
* Providing a value for the racfGroupName is optional. If the racfGroupName
* value specified is fewer than 8 characters, it will be right-padded with
* blanks by the IMS Connect API. If RACF is on, IMS Connect will validate
* the racfGroupName value by checking for invalid characters. IMS Connect
* will move all valid characters of racfGroupName starting with the
* leftmost character up to but NOT including the first invalid character,
* into a buffer which has been initialized to all blanks. If RACF is off,
* IMS Connect does not perform any validation and the 8-character,
* unvalidated racfGroupName value is used directly by the IMS Connect user
* message exit unless the IMS Connect user message exit has been modified
* to do otherwise. The RACF group name value is used by IMS Connect during
* racroute verify calls for user authentication and by IMS OTMA for user
* authorization. RACF will accept either the correct group name for the
* specified RACF user ID or, if the group name is to be ignored in racroute
* verify calls, 0 to 8 blanks for this value.
*
* Note that the DEFAULT_RACF_GROUP_NAME has the String value "RACFGRUP",
* and the racfGroupName property must be changed during runtime to the name
* of appropriate RACF or SAF group under which the interaction will be
* executed in IMS Connect.
*
* @param aRacfGroupName
* the RACF group name to be used for this interaction.
* @see TmInteraction#getRacfGroupName()
*/
public void setRacfGroupName(String aRacfGroupName)
throws ImsConnectApiException {
if ((aRacfGroupName).trim().equals("") || (aRacfGroupName == null)) // all
// blanks
// is
// a
// valid
// aRacfGroupName
{
aRacfGroupName = EIGHT_BLANKS;
} else if (aRacfGroupName.length() > MAX_LEN_RACF_GROUP_NAME) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0026E, new Object[] {
aRacfGroupName, "racfGroupName",
String.valueOf(MAX_LEN_RACF_GROUP_NAME) });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0026E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setRacfGroupName(String). Exception thrown was: "
+ e.toString());
throw e;
}
else if (!(PropertiesFileLoader.isValidHostStyleName(aRacfGroupName))) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0029E, new Object[] {
"racfGroupName", aRacfGroupName });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0029E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setRacfGroupName(String). Exception thrown was: "
+ e.toString());
throw e;
}
if (this.racfGroupName != aRacfGroupName) {
this.racfGroupName = aRacfGroupName;
this.setRebuildMessage(true);
this.updateIrmRacfGroupName = true;
}
}
/**
* Gets the RACF password.
*
* @return a String
value containing the RACF password property
* value
*/
public String getRacfPassword() {
return this.racfPassword;
}
/**
* Sets the racfPassword property value. The racfPassword property specifies
* the RACF passticket or password which is a string of 1 to 8 uppercase
* alphanumeric (A through Z, 0 to 9) or special (@, #, $,.,<,+,|,&,!,*,-,%,_,>,?,:,=) characters. If
* the racfPassword value specified is fewer than 8 characters, it will be
* right-padded with blanks by the IMS Connect API. For non-trusted users,
* the client or one of the IMS Connect user exits must provide a valid
* value for the racfPassword property if IMS Connect is configured to check
* SAF authentication of clients. For trusted users or if IMS Connect is
* configured to NOT check SAF authentication of clients, the client
* authentication is bypassed and therefore, the racfPassword value is
* ignored by IMS Connect. The racfPassword value is not used by OTMA or IMS
* even if OTMA is configured to check SAF authorization of clients. If IMS
* Connect is configured to check SAF authentication of clients (i.e., RACF
* is on or yes in IMS Connect), IMS Connect checks for invalid characters
* in the racfPassword value. It copies all valid characters in racfPassword
* that it encounters in sequence, starting with the leftmost character and
* ending at 8 characters or before the first invalid character, into a
* buffer which has been initialized to all blanks. If the leftmost
* character is invalid, the buffer will contain 8 blanks. This buffer
* containing the validated racfPassword value is used by the IMS Connect
* user message exit and also passed back to IMS Connect regardless of
* whether or not it is used by IMS Connect, OTMA or IMS.
*
* Note that the DEFAULT_RACF_PASSWORD has the String value "RACFPSWD", and
* the racfPassword property must be changed during runtime to the RACF or
* SAF password of the RACF or SAF userID under which the interaction will
* be executed in IMS Connect.
*
* @param aRacfPassword
* the RACF password to be used for this interaction.
* @see TmInteraction#getRacfPassword()
*/
public void setRacfPassword(String aRacfPassword)
throws ImsConnectApiException {
if ((aRacfPassword).trim().equals("") || (aRacfPassword == null)) // all
// blanks
// is
// a
// valid
// aRacfPassword
{
aRacfPassword = EIGHT_BLANKS;
} else if (aRacfPassword.length() > MAX_LEN_RACF_PASSWORD) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0026E, new Object[] {
aRacfPassword.replaceAll(".", "*"), "racfPassword",
String.valueOf(MAX_LEN_RACF_PASSWORD) });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0026E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setRacfPassword(String). Exception thrown was: "
+ e.toString());
throw e;
} else if (!(this.isValidRacfPassword(aRacfPassword))) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0029E,
new Object[] { "racfPassword",
aRacfPassword.replaceAll(".", "*") });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0029E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setRacfPassword(String). Exception thrown was: "
+ e.toString());
throw e;
}
if (this.racfPassword != aRacfPassword) {
this.racfPassword = aRacfPassword;
this.setRebuildMessage(true);
this.updateIrmRacfPassword = true;
}
}
/**
* Gets the RACF userID.
*
* @return a String
value containing the RACF userID property
* value
*/
public String getRacfUserId() {
return this.racfUserId;
}
// Target IMS properties
/**
* Sets the racfUserId property value. The racfUserId property specifies the
* RACF user ID which is a string of 1 to 8 uppercase alphanumeric (A
* through Z, 0 to 9) or special (@, #, $) characters. The client or one of
* the IMS Connect or IMS user exits must provide a valid value for the
* racfUserId property if IMS Connect is configured to check SAF
* authentication of clients or OTMA is configured to check SAF
* authorization of clients. If the racfUserId value specified is fewer than
* 8 characters, it will be right-padded with blanks by the IMS Connect API.
* If RACF is on, IMS Connect checks for invalid characters in the
* racfUserId value and moves all valid characters of racfUserId starting
* with the leftmost character up to but NOT including the first invalid
* character, into a buffer which has been pre-initialized to all blanks. If
* the leftmost character is invalid, the buffer will contain 8 blanks. This
* buffer containing the validated racfPassword value is used by the IMS
* Connect user message exit and also passed back to IMS Connect regardless
* of whether or not it is used by IMS Connect, OTMA or IMS. If RACF is on
* and the racfPassword value is all blanks or resolves to all blanks, IMS
* Connect will return an error. If RACF is off, IMS Connect does not
* perform any validation.
*
* Note that the DEFAULT_RACF_USERID has the String value "RACFUID", and the
* racfUserId property must be changed during runtime to the name of
* appropriate RACF or SAF userID under which the interaction will be
* executed in IMS Connect.
*
* @param aRacfUserId
* the RACF userId to be used for this interaction.
* @see TmInteraction#getRacfUserId()
*/
public void setRacfUserId(String aRacfUserId) throws ImsConnectApiException {
if ((aRacfUserId).trim().equals("") || (aRacfUserId == null)) // all
// blanks
// is a
// valid
// aRacfUserId
{
aRacfUserId = EIGHT_BLANKS;
} else if (aRacfUserId.length() > MAX_LEN_RACF_USERID) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0026E, new Object[] {
aRacfUserId, "racfUserId",
String.valueOf(MAX_LEN_RACF_USERID) });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0026E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setRacfUserId(String). Exception thrown was: "
+ e.toString());
throw e;
} else if (!(PropertiesFileLoader.isValidHostStyleName(aRacfUserId))) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0029E, new Object[] {
"racfUserId", aRacfUserId });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0029E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setRacfUserId(String). Exception thrown was: "
+ e.toString());
throw e;
}
if (this.racfUserId != aRacfUserId) {
this.racfUserId = aRacfUserId;
this.setRebuildMessage(true);
this.updateIrmRacfUserId = true;
}
}
/**
* Gets a boolean value representing whether undeliverable output will be
* purged.
*
* @return a boolean
value containing the
* purgeUndeliverableOutput property value
*/
public boolean isPurgeUndeliverableOutput() {
return purgeUndeliverableOutput;
}
/**
* Gets the reroute name property value.
*
* @return a String
value containing the rerouteName property
*/
public String getRerouteName() {
return this.rerouteName;
}
/**
* Sets the rerouteName property value which specifies the optional name of
* a reroute destination (an OTMA Tpipe asynchronous hold queue). The
* rerouteName property is a string of 1 to 8 uppercase alphanumeric (A
* through Z, 0 to 9) or special (@, #, $) characters. The value of the
* rerouteName property is validated in IMS Connect by converting all
* invalid characters to blanks and then truncating the resulting
* rerouteName value at the leftmost blank character. As a result, OTMA will
* always be passed a valid rerouteName to use (though not the intended
* rerouteName if the rerouteName value specified is prefixed with or
* contains imbedded invalid characters or blanks).
*
* A value of all blanks in the resulting rerouteName value will cause the
* default reroute name defined in IMS Connect, which is always validated
* during IMS Connect initialization, to be used as the reroute name by IMS
* Connect. If the validation results in a non-blank rerouteName value, that
* value will be used as the destination Tpipe name for all undeliverable
* Commit Mode 0 output in a SENDRECV, SENDONLY or SENDONLYACK function
* interaction. Unless the IMS Connect user message exit or another user
* exit has been modified to do otherwise, the rerouteName value is copied
* to the OMUSR_REROUT_NM field in the OTMA header by the IMS Connect user
* message exit.
*
* The rerouteName and resumeTpipe_AlternateClientId values are mapped to
* the same offset in the IRM as well as the OTMA header. As a result, they
* are mutually exclusive; that is, both cannot be used in the same request
* message. The IMS Connect API will determine which value to use based on
* the function specified in the request. If the function specified is a
* RESUMETPIPE, the rerouteName value will be ignored and the
* resumeTpipeAlternateClientId value will be used by the API. Otherwise,
* the resumeTpipeAlternateClientId value will be ignored and the
* rerouteName value will be used by the API.
*
* If the reroute function is to be used successfully, the following steps
* must be completed:
*
* - The architectureLevel property must be set to 0X01 (Reroute support
* included) or higher. This is done internally by the API since the API
* always sets the architectureLevel property value to 0x02.
*
- The purgeUndeliveredOutput property must be set to false and the
* rerouteUndeliveredOutput property must be set to true.
*
- A valid value (or blanks if you have configured your application to
* use IMS Connect reroute name value) must be provided for the rerouteName
* property. Note that DEFAULT_REROUTE_NAME has the
*
String
value of eight blank characters and the rerouteName
* property has to be set during runtime to the name of the reroute queue on
* which you want OTMA to place undeliverable response messages.
*
* For more details on rerouting output, see the topic
* "Rerouting commit-then-send output" in the V10
* Communications and Connections guide documentation.
*
* @param rerouteName
* the rerouteName to be used for this interaction.
* @see ApiProperties#REROUTE_NAME_IMS_CONNECT_DEAD_LETTER_QUEUE
* @see ApiProperties#REROUTE_NAME_NONE
* @see TmInteraction#getRerouteName()
*/
public void setRerouteName(String aRerouteName)
throws ImsConnectApiException {
if (((aRerouteName).trim().equals("") || aRerouteName == null)) // all
// blanks
// is a
// valid
// rerouteName
// value
{
aRerouteName = EIGHT_BLANKS;
} else if (aRerouteName.length() > MAX_LEN_REROUTE_NAME) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0026E, new Object[] {
aRerouteName, "rerouteName",
String.valueOf(MAX_LEN_REROUTE_NAME) });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0026E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setRerouteName(String). Exception thrown was: "
+ e.toString());
throw e;
} else {
if (!(PropertiesFileLoader.isValidHostStyleName(aRerouteName))) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0029E, new Object[] {
"rerouteName", new String(this.rerouteName) });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0029E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setRerouteName(String). Exception thrown was: "
+ e.toString());
throw e;
}
}
// String newRerouteName = stringPad(aRerouteName, ' ',
// MAX_LEN_REROUTE_NAME);
if (this.rerouteName != aRerouteName) {
this.rerouteName = aRerouteName;
this.setRebuildMessage(true);
this.updateIrmRerouteName = true;
}
}
/**
* Gets a boolean value representing whether undeliverable output will be
* rerouted to a different asynchronous hold queue.
*
* @return a boolean
value containing the
* rerouteUndeliverableOutput property value
*/
public boolean isRerouteUndeliverableOutput() {
return rerouteUndeliverableOutput;
}
/**
* Sets the rerouteUndeliverableOutput property value. The valid values, for
* which the following constants are defined in ApiProperties
,
* are:
*
* REROUTE_UNDELIVERABLE_OUTPUT
- Boolean value true. Save
* undeliverable primary and/or secondary commit mode 0 output on the OTMA
* asynchronous hold queue corresponding to the configured reroute name
* (maps to IRM_F3_REROUT value of 0X08).
* DO_NOT_REROUTE_UNDELIVERABLE_OUTPUT
- Boolean value
* false. Save undeliverable primary and/or secondary commit mode 0 output
* on the OTMA asynchronous message hold queue of the inputting Tpipe
* (clientID Tpipe) (no bits set in IRM_F3). Causes the reroute undelivered
* output indicator to not be returned to IMS Connect from the user message
* exit and, if the purgeUndeliverableOutput value is also false, causes the
* undelivered output to be stored on the OTMA asynchronous message hold
* queue of the inputting Tpipe (clientID Tpipe).
* DEFAULT_REROUTE_UNDELIVERABLE_OUTPUT
- same as
* DO_NOT_REROUTE_UNDELIVERABLE_OUTPUT
*
* If a value for this property is not supplied by the client, the default
* value (DO_NOT_REROUTE_UNDELIVERABLE_OUTPUT
) will be used. If
* the rerouteUndeliverableOutput property is set to true, providing a value
* for the rerouteName property is optional. The reroute function applies
* only to Send-Recv, Send-Only and Send-Only-with-Acknowledgement
* interactions. If you specify both purgeUndeliverableOutput and
* rerouteUndeliverableOutput at the same time, undeliverable primary and/or
* secondary commit mode 0 output resulting from a transaction is neither
* purged nor rerouted. In this case, OTMA stores the output onto the
* asynchronous message hold queue of the inputting Tpipe (clientID Tpipe)
* and issues a DFS2407W warning message.
*
* For more details on rerouting output, see the topic
* "Rerouting commit-then-send output" in the V10
* Communications and Connections guide documentation.
*
* @param aRerouteUndeliverableOutput
* the rerouteUndeliverableOutput to set. Use the constants
* defined in ApiProperties to specify this parameter.
* @see ApiProperties#REROUTE_UNDELIVERABLE_OUTPUT
* @see ApiProperties#DO_NOT_REROUTE_UNDELIVERABLE_OUTPUT
* @see TmInteraction#getRerouteUndeliverableOutput()
*/
public void setRerouteUndeliverableOutput(
boolean aRerouteUndeliverableOutput) throws ImsConnectApiException {
if ((this.purgeUndeliverableOutput) && (aRerouteUndeliverableOutput)){
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0027E,
new Object[] { "rerouteUndeliverableOutput" });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0027E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setRerouteUndeliverableOutput(boolean). Exception thrown was: "
+ e.toString());
throw e;
} else if (this.rerouteUndeliverableOutput != aRerouteUndeliverableOutput) {
this.rerouteUndeliverableOutput = aRerouteUndeliverableOutput;
this.setRebuildMessage(true);
}
}
/**
* Gets the name of an alternate clientId (whose value is the name of an
* asynchronous hold queue) from which output will be retrieved for a
* resumeTpipe interaction.
*
* @return a String
value containing the
* resumeTpipeAlternateClientId property value
*/
public String getResumeTpipeAlternateClientId() {
return resumeTpipeAlternateClientId;
}
/**
* Sets the resumeTpipeAlternateClientId property value which specifies the
* optional name of a reroute destination (an OTMA Tpipe asynchronous hold
* queue). The resumeTpipeAlternateClientId property is a string of 1 to 8
* uppercase alphanumeric (A through Z, 0 to 9) or special (@, #, $)
* characters.
*
* The value of the resumeTpipeAlternateClientId property is validated in
* IMS Connect by converting all invalid characters to blanks and then
* truncating the resulting resumeTpipeAlternateClientId value at the
* leftmost blank character. As a result, OTMA will always be passed a valid
* alternate client ID to use (though not the intended rerouteName if the
* rtAltClientID value specified is prefixed with or contains imbedded
* invalid characters or blanks).
*
* A value of all blanks in the resulting resumeTpipeAlternateClientId value
* will cause the RESUMETPIPE interaction to be processed as a normal
* RESUMETPIPE function in which the output is retrieved from the Tpipe
* associated with the clientID of the connection on which the RESUMETPIPE
* request is received. If the validation results in a non-blank
* resumeTpipeAlternateClientId value, that value will be used as the as
* name of the Tpipe from which one or more asynchronous output messages are
* retrieved for RESUMETPIPE requests. Unless the IMS Connect user message
* exit or another user exit has been modified to do otherwise, the
* validated resumeTpipeAlternateClientId value is copied to the
* OMUSR_REROUT_NM field in the OTMA header by the IMS Connect user message
* exit.
*
* @param altClientID
* the alternate client ID to set.
* @see TmInteraction#getResumeTpipeAlternateClientId()
*/
public void setResumeTpipeAlternateClientId(
String aResumeTpipeAlternateClientId) throws ImsConnectApiException {
if (((aResumeTpipeAlternateClientId).trim().equals("") || aResumeTpipeAlternateClientId == null)) // all
// blanks
// is
// a
// valid
// resumeTpipeAlternateClientId
// value
// (meaning
// you
// want
// to
{ // retrieve async output from your clientID Tpipe rather than some
// other Tpipe
if (this.resumeTpipeAlternateClientId != EIGHT_BLANKS) {
// aResumeTpipeAlternateClientId = EIGHT_BLANKS;
this.resumeTpipeAlternateClientId = EIGHT_BLANKS;
this.setRebuildMessage(true);
}
// this.setRebuildMessage(true);
return;
} else if (aResumeTpipeAlternateClientId.length() > MAX_LEN_RESUMETPIPE_ALTERNATE_CLIENTID) {
String errMsg = ImsConnectErrorMessage
.getString(
ImsConnectErrorMessage.HWS0026E,
new Object[] {
aResumeTpipeAlternateClientId,
"resumeTpipeAlternateClientId",
String
.valueOf(MAX_LEN_RESUMETPIPE_ALTERNATE_CLIENTID) });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0026E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setResumeTpipeAlternateClientId(String). Exception thrown was: "
+ e.toString());
throw e;
} else {
if (!(PropertiesFileLoader
.isValidHostStyleName(aResumeTpipeAlternateClientId.trim()))) {
String errMsg = ImsConnectErrorMessage
.getString(
ImsConnectErrorMessage.HWS0029E,
new Object[] {
"resumeTpipeAlternateClientId",
new String(
this.resumeTpipeAlternateClientId) });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0029E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setResumeTpipeAlternateClientId(String). Exception thrown was: "
+ e.toString());
throw e;
}
}
// String newResumeTpipeAlternateClientId =
// inputMsg.padString(aResumeTpipeAlternateClientId, ' ',
// MAX_LEN_RESUMETPIPE_ALTERNATE_CLIENTID);
if (this.resumeTpipeAlternateClientId != aResumeTpipeAlternateClientId) {
this.resumeTpipeAlternateClientId = aResumeTpipeAlternateClientId;
this.setRebuildMessage(true);
this.updateIrmRerouteName = true;
}
}
/**
* Gets an int
value representing the type of resumeTpipe
* processing (single-no wait, single-wait, auto, or no-auto) to be
* performed for a resumeTpipe interaction.
*
* @return an int
value containing the resumeTpipeProcessing
* property value
*/
public int getResumeTpipeProcessing() {
return resumeTpipeProcessing;
}
/**
* Sets the resumeTpipeProcessing property value which specifies the type of
* processing to be used for a resumeTpipe request. All of the SINGLE and
* AUTO values are mutually exclusive. The appropriate value must be
* specified by the client on input and will be maintained in the message
* until the interaction has completed. All RESUMETIPE interactions are used
* to retrieve output response messages from an OTMA asynchronous message
* hold queue. The hold queue from which messages are retrieved is either:
*
* - the Tpipe whose name matches the clientID value for the current
* RESUMETPIPE interaction if a resumeTpipeAlternateClientID value has not
* been specified for this interaction or,
*
- if a resumeTpipeAlternateClientID value has been specified, the Tpipe
* whose name matches that resumeTpipeAlternateClientID value for the
* current RESUMETPIPE interaction.
*
* Note that the original version of resumeTpipe (retrieve asynchronous
* output from an OTMA hold queue) which did not support any message flow
* options and retrieved output from one or more Tpipes is not supported.
* Only the later version of resumeTpipe which supports message flow options
* and retrieves output from a single Tpipe is supported. Only one value can
* be specified per interaction. The valid values, for which the following
* constants are defined in ApiProperties
, are:
*
* RESUMETPIPE_SINGLE_NOWAIT
- Retrieves at most one
* response message per RESUMETPIPE_SINGLE_NOWAIT request. If no message is
* present when the current RESUMETPIPE_SINGLE_NOWAIT interaction is
* received by OTMA from IMS Connect, IMS Connect will return a timeout
* error to the client as soon as the IMS Connect response timer (set
* according to the imsConnectResponseTimeout value in use for that
* interaction) has expired. If there was no response message already
* available when OTMA received the RESUMETPIPE_SINGLE_NOWAIT request, any
* output received by OTMA after time (but before a subsequent RESUMETPIPE
* request has been received by OTMA) will be moved immediately to its
* asynchronous message hold queue by OTMA. Set the
* imsConnectResponseTimeout to a smaller value to reduce the amount of time
* wasted waiting for the IMS Connect response timer to expire when there is
* no message available to be retrieved at the time the RESUMETPIPE request
* is processed by OTMA.
* RESUMETPIPE_AUTO
- Retrieves all available messages, one
* at a time. It is recommended that the RESUMETPIPE_AUTO option be used
* only if the client is a dedicated output client and the ackNakProvider
* property is set to CLIENT_ACK_NAK. Set the imsConnectResponseTimeout to a
* larger value to increase the probability of retrieving all expected
* response messages in a single RESUMETIPE interaction. Each ACK sent by
* the client resets the IMS Connect response timer controlled by the
* imsConnectResponseTimeout value. The IMS Connect response timer value
* used by the RESUMETPIPE_AUTO interaction applies only to the first
* receive state. OTMA will continue to return output messages that it gets
* from IMS after the time the RESUMETPIPE_AUTO request is received by OTMA
* until both of the following conditions are true:
*
* - no more output is available, and
*
- the IMS Connect response timer has expired in IMS Connect
*
* RESUMETPIPE_NOAUTO
- Retrieves all messages available at
* the time the RESUMETPIPE_NOAUTO request is received by OTMA, one at a
* time. It is recommended that the RESUMETPIPE_NOAUTO option be used only
* if the client is a dedicated output client and the ackNakProvider
* property is set to CLIENT_ACK_NAK. Set the imsConnectResponseTimeout to a
* smaller value in order to reduce the amount of wait time after the last
* message has been returned. Each ACK sent by the client resets the
* imsConnectResponseTimeout value. The IMS Connect response timer value
* used by the RESUMETPIPE_NOAUTO interaction applies only to the first
* receive state. A RESUMETPIPE_NOAUTO interaction differs from a
* RESUMETPIPE_AUTO interaction in that, for _NOAUTO, OTMA will only return
* output messages that are already available on the hold queue at the time
* the RESUMETPIPE_NOAUTO request is received by OTMA. Any output received
* after the request is received by OTMA will not be returned to the client
* until a subsequent RESUMETPIPE call is made.
* RESUMETPIPE_SINGLE_WAIT
- Retrieves at most one response
* message per RESUMETPIPE_SINGLE_WAIT request. If no message is present
* when the current RESUMETPIPE_SINGLE_WAIT interaction is received by IMS
* Connect, IMS Connect will continue to wait for a response message to be
* returned by OTMA or for the IMS Connect response timer (set according to
* the imsConnectResponseTimeout value for this interaction) to time out. If
* IMS Connect receives a response message before the IMS Connect response
* timer times out, it will return that response to the client and wait for
* an ACK or NAK from the client. If the IMS Connect response timer times
* out before IMS Connect receives a response message, IMS Connect will
* return a timeout error to the client. Any output received by IMS Connect
* from OTMA after the IMS Connect response timer has expired but before a
* subsequent RESUMETPIPE request has been received by IMS Connect for the
* target Tpipe will be considered a late response message and will be
* NAK'ed by IMS Connect and returned to its asynchronous hold queue by
* OTMA. Set the imsConnectResponseTimeout to a larger value to increase the
* probability of retrieving all expected response messages in a single
* RESUMETPIPE interaction.
* DEFAULT_RESUME_TPIPE_PROCESSING
- same as
* RESUMETPIPE_SINGLE_WAIT
*
* If a value for this property is not supplied by the client, the default
* value (RESUMETPIPE_SINGLE_WAIT
) will be used. For more
* information about the IMS Connect response timer, which can be controlled
* with the imsConnectResponseTimeout property value, see the IMS V10
* Communications and Connections guide documentation under the topic
* "Time-out intervals on input messages".
*
* @param aResumeTpipeProcessing
* the resumeTpipeProcessing to set. Use the constants defined in
* ApiProperties to specify this parameter.
* @see ApiProperties#RESUME_TPIPE_SINGLE_NOWAIT
* @see ApiProperties#RESUME_TPIPE_NOAUTO
* @see ApiProperties#RESUME_TPIPE_SINGLE_WAIT
* @see ApiProperties#RESUME_TPIPE_AUTO
* @see TmInteraction#getResumeTpipeProcessing()
*/
public void setResumeTpipeProcessing(int aResumeTpipeProcessing)
throws ImsConnectApiException {
if (((aResumeTpipeProcessing & ApiProperties.RESUME_TPIPE_SINGLE_NOWAIT) == ApiProperties.RESUME_TPIPE_SINGLE_NOWAIT)
|| ((aResumeTpipeProcessing & ApiProperties.RESUME_TPIPE_AUTO) == ApiProperties.RESUME_TPIPE_AUTO)
|| ((aResumeTpipeProcessing & ApiProperties.RESUME_TPIPE_NOAUTO) == ApiProperties.RESUME_TPIPE_NOAUTO)
|| ((aResumeTpipeProcessing & ApiProperties.RESUME_TPIPE_SINGLE_WAIT) == ApiProperties.RESUME_TPIPE_SINGLE_WAIT)) {
if (this.resumeTpipeProcessing != aResumeTpipeProcessing) {
this.resumeTpipeProcessing = aResumeTpipeProcessing;
this.setRebuildMessage(true);
}
} else {
String validResumeTpipeProcessingString = "";
try {
validResumeTpipeProcessingString = ImsConnectErrorMessage
.getString(ImsConnectErrorMessage.VALID_PROPERTY_VALUE_RESUMETPIPEPROCESSING);
} catch (Exception e1) {
// do nothing - this exception should never occur unless the API
// JAR is corrupted
}
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0030E, new Object[] {
"resumeTpipeProcessing",
String.valueOf(aResumeTpipeProcessing),
validResumeTpipeProcessingString });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0030E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in Connection.setResumeTpipeProcessing(byte). Exception thrown was: "
+ e.toString());
throw e;
}
}
/**
* Gets a boolean
value representing whether or not IMS Connect
* should pass back to the client the MOD name returned by the IMS
* application.
*
* @return a boolean
value containing the returnMfsModname
* property value
* @ApiProperties#DO_RETURN_MFS_MODNAME
* @ApiProperties#DO_NOT_RETURN_MFS_MODNAME
*/
public boolean isReturnMfsModname() {
return this.returnMfsModname;
}
/**
* Sets the returnMfsModname property value which specifies if the MFS MOD
* name is to be returned to the client by OTMA and IMS Connect. This value
* determines if an RMM (Request Mod Message) segment is built by IMS
* Connect and returned to the client as part of the response message. Only
* one value can be specified per interaction. The valid values, for which
* the following constants are defined in ApiProperties
, are:
*
* DO_NOT_RETURN_MFS_MODNAME
- Boolean value false. User
* requests no MFS MOD name to be returned (maps to IRM_F1 value of 0X00).
* DO_RETURN_MFS_MODNAME
- Boolean value true. User
* requests MFS MOD name to be returned (maps to IRM_F1 value of 0X80)
* DEFAULT_RETURN_MFS_MODNAME
- same as
* DO_NOT_RETURN_MFS_MODNAME
If a value for this property is
* not supplied by the client, the default value (
* DO_NOT_RETURN_MFS_MODNAME
) will be used.
*
* @param the
* aReturnMfsModname to set. Use the constants defined in
* ApiProperties to specify this parameter.
* @see ApiProperties#DO_NOT_RETURN_MFS_MODNAME
* @see ApiProperties#DO_RETURN_MFS_MODNAME
* @see TmInteraction#isReturnMfsModname()
*/
public void setReturnMfsModname(boolean aReturnMfsModname) {
if (this.returnMfsModname != aReturnMfsModname) {
this.returnMfsModname = aReturnMfsModname;
this.setRebuildMessage(true);
}
}
/**
* Gets the sync level.
*
* @return a byte
value containing the syncLevel property value
* @see ApiProperties#SYNC_LEVEL_CONFIRM
* @see ApiProperties#SYNC_LEVEL_NONE
*/
public byte getSyncLevel() {
return this.syncLevel;
}
/**
* Sets the syncLevel property value which specifies the synch level to be
* used for this interaction. Only one value can be specified. The valid
* values, for which the following constants are defined in
* ApiProperties
, are:
*
* SYNC_LEVEL_NONE
- Maps to IRM_F3 byte value of 0X00. If
* a transaction is specified with SYNC_LEVEL_NONE, no acknowledgement is
* required from the client.
* SYNC_LEVEL_CONFIRM
- Maps to IRM_F3 byte value of 0X01.
* If a transaction is specified with SYNC_LEVEL_CONFIRM, the client is
* required to send an acknowledgement to signal to IMS Connect whether or
* not the output message was successfully (ACK) or unsuccessfully (NAK)
* processed by the client.
* DEFAULT_SYNC_LEVEL
- SYNC_LEVEL_CONFIRM
*
* If a value for this property is not supplied by the client, the default
* value (SYNC_LEVEL_CONFIRM
) will be used.
*
* A value for synch level is required by IMS Connect and OTMA. However, the
* value provided for synch level will be over-ridden internally if the
* commit mode or type of the interaction is only supported with a specific
* synch level. For example, for Commit Mode 0 interactions, syncLevel must
* be set to CONFIRM. For Commit Mode 1 interactions, synch level can be set
* to NONE or CONFIRM. If a value for synch level is not supplied by the
* client and cannot be determined based on the messageFunction and
* commitMode values, the default value (SYNC_LEVEL_CONFIRM
)
* will be used. If the syncLevel property value is over-ridden internally
* by the API and if the traceLevel is set to TRACE_LEVEL_INTERNAL, an
* informational trace message will be written to the trace output file.
*
* For more details on the synch level property, see the topic
* "Commit mode and synch level definitions" in the V10 Communications
* and Connections guide documentation.
*
* @param aLevel
* a byte value representing the syncLevel. Use the constants
* defined in ApiProperties to specify this parameter.
* @see ApiProperties#SYNC_LEVEL_CONFIRM
* @see ApiProperties#SYNC_LEVEL_NONE
* @see TmInteraction#getSyncLevel()
*/
public void setSyncLevel(byte aSyncLevel) throws ImsConnectApiException {
// himakar callout changes - now need to support sync level confirm too
// for callout RT processing
// First release support Sync Level None only
if (((aSyncLevel & SYNC_LEVEL_NONE) == SYNC_LEVEL_NONE)
|| (aSyncLevel == SYNC_LEVEL_CONFIRM)) {
if (this.syncLevel != aSyncLevel) {
this.syncLevel = aSyncLevel;
this.setRebuildMessage(true);
}
} else {
String validSyncLevelString = "";
try {
validSyncLevelString = ImsConnectErrorMessage
.getString(ImsConnectErrorMessage.VALID_PROPERTY_VALUE_SYNCLEVEL);
} catch (Exception e) {
}
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0030E, new Object[] {
"syncLevel", String.valueOf(aSyncLevel),
validSyncLevelString });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0030E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setSyncLevel(byte). Exception thrown was: "
+ e.toString());
throw e;
}
}
/**
* Gets the IMS transaction code (trancode.)
*
* @return a String
value containing the trancode property
* value
*/
public String getTrancode() {
return trancode;
}
// Timeout properties
/**
* Sets the trancode property value. The trancode property specifies the IMS
* transaction code which is a string of uppercase alphanumeric (A through
* Z, 0 to 9) or special (@, #, $) characters. The trancode property
* identifies the IMS application (transaction) to be executed. This
* trancode property is used by the API to set the trancode in the data in
* situations where the client application has provided an input data byte
* array that is not prefixed with LLZZ and the trancode. The first 8
* characters ofthe trancode property are also used internally by IMS
* Connect and OTMA to identify the trancode of the target IMS application.
*
* If the trancode property value is in EBCDIC and contains invalid
* characters (i.e. characters other than those listed above), an invalid
* trancode error will be returned to the client from OTMA. If the trancode
* property value is in ASCII and contains invalid characters, an invalid
* trancode error will be returned to the client by IMS Connect. If the
* trancode value is valid but cannot be found by IMS, a trancode not found
* error will be returned to the client from IMS. The trancode value is
* placed in the IRM_TRNCOD field in the IRM.
*
* The trancode must be formatted in the same format required by IMS Connect
* and the IMS transaction. For example, if the trancode is always followed
* by 5 blanks before the LLZZ for the first data segment starts, the
* trancode field must be set to a value that includes those 5 extra bytes.
* In the case of the IMS Sample/IVP IVTNO program, the trancode would be
* set to one of the valid trancodes such as IVTNO followed by 5 bytes of
* spaces.
*
* Note that the DEFAULT_TRANCODE has the String value "TRANCODE", and the
* trancode property must be changed to a trancode that is defined in the
* target IMS system before use.
*
* @param aTrancode
* the trancode to be used for this interaction.
* @see TmInteraction#getTrancode()
*/
public void setTrancode(String aTrancode) throws ImsConnectApiException {
if ((aTrancode == "") || (aTrancode == null)) {
if (this.trancode != "") {
this.trancode = "";
((InputMessageImpl) (this.inputMsg)).setTrancodeInData("");
this.setRebuildMessage(true);
this.updateIrmTrancode = true;
updateIrmTrancodeInData = true;
}
} else {
int len = aTrancode.length();
// remove ""
if (len >= 2) {
if ((aTrancode.charAt(0) == '"')
&& (trancode.charAt(len - 1) == '"')) // if trancode
// enclosed in
// "'s,
// remove them
// trancode with a '"' only at the beginning or the end
// or the end will be treated as a valid trancode and will
// be rejected by Connect or IMS (not sure which)
aTrancode = aTrancode.substring(1, len - 1);
}
if (aTrancode.trim().length() > MAX_LEN_TRANCODE) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0026E, new Object[] {
aTrancode, "trancode",
String.valueOf(MAX_LEN_TRANCODE) });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0026E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setTrancode(String). Exception thrown was: "
+ e.toString());
throw e;
}
int trailingBlankStart = aTrancode.indexOf(' ', 1);
String testTrancode = aTrancode.substring(0,
trailingBlankStart == -1 ? 8 : trailingBlankStart);
if (!PropertiesFileLoader.isValidHostStyleName(testTrancode)) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0029E, new Object[] {
"trancode", aTrancode });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0029E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setTrancode(String). Exception thrown was: "
+ e.toString());
throw e;
}
if (this.trancode != aTrancode) {
this.trancode = aTrancode; // length of trancode cannot be
// greater than 8 non-blank
// characters but
// trancode can be padded with blanks beyond 8 characters, so do
// not
} // truncate trancode at 8 characters. Trailing blanks beyond 8
// characters
// will be truncated in trancode when copied into IRM
((InputMessageImpl) (this.inputMsg)).setTrancodeInData(aTrancode);
this.setRebuildMessage(true);
this.updateIrmTrancode = true;
this.updateIrmTrancodeInData = true;
}
}
/**
* Gets the name of the XML Adapter to be used in the IMS Connect Adapter
* Task Manager
*
* @return a String
value containing the xmlAdapterName
* property value
*/
public String getXmlAdapterName() {
return this.xmlAdapterName;
}
/**
* Set name of XML Adapter to be used in the IMS Connect Adapter Task
* Manager
*
* @param aAtmAdapterName
* the IMS Connect Adapter Task Manager adapter name
*/
public void setXmlAdapterName(String anXmlAdapterName)
throws ImsConnectApiException {
// if (this.atmAdapterName == ADAPTER_TYPE_COBOL) //?????
// return;
if ((anXmlAdapterName.trim().equals("")) || (anXmlAdapterName == null)) {
anXmlAdapterName = EIGHT_BLANKS;
} else if (anXmlAdapterName.length() > XML_ADAPTER_NAME_MAX_LEN) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0029E, new Object[] {
"xmlAdapterName", anXmlAdapterName, });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0029E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setXmlAdapterName(String). Exception thrown was: "
+ e.toString());
throw e;
} else if (!(PropertiesFileLoader
.isValidHostStyleName(anXmlAdapterName))) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0029E, new Object[] {
"xmlAdapterName", new String(anXmlAdapterName) });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0029E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setRerouteName(String). Exception thrown was: "
+ e.toString());
throw e;
}
// String newXmlAdapterName = stringPad(anXmlAdapterName, ' ',
// XML_ADAPTER_NAME_MAX_LEN);
if (this.xmlAdapterName != anXmlAdapterName) {
this.xmlAdapterName = anXmlAdapterName;
this.setRebuildMessage(true);
}
}
/**
* Gets the type of the adapter to be used in the IMS Connect Adapter Task
* Manager
*
* @return a byte
value containing the mlAdapterType property
* value
*/
/*
* private byte getXmlAdapterType() { return this.xmlAdapterType; }
*/
/**
* Set type of adapter to be used in IMS Connect
*
* @param anXmlAdapterType
* an byte representing the type of adapter to be used in IMS
* Connect
*/
/*
* public void setXmlAdapterType(byte anXmlAdapterType) throws
* ImsConnectApiException { // In the first release, only support COBOL
* Adapter and RYO Adapter //if ((anXmlAdapterType != ADAPTER_TYPE_COBOL) &&
* (anXmlAdapterType != ADAPTER_TYPE_RYO)) if ( (XML_ADAPTER_TYPE_MIN_VALUE
* > anXmlAdapterType) || (anXmlAdapterType > XML_ADAPTER_TYPE_MAX_VALUE) )
* { String xmlAdapterTypeNameString; if (anXmlAdapterType == 2 ||
* anXmlAdapterType == 3) xmlAdapterTypeNameString =
* XML_ADAPTER_TYPE_NAME[anXmlAdapterType]; else xmlAdapterTypeNameString =
* "ADAPTER_TYPE_UNKNOWN"; String errMsg = ImsConnectErrorMessage.getString(
* ImsConnectErrorMessage.HWS0022E, new Object[] { anXmlAdapterType,
* xmlAdapterTypeString });
*
* ImsConnectApiException e = new ImsConnectApiException(
* ImsConnectErrorMessage.HWS0022E, errMsg); throw e; } if
* (this.xmlAdapterType != anXmlAdapterType) { this.xmlAdapterType =
* anXmlAdapterType; } /* if (aAdapterType == ADAPTER_TYPE_COBOL)
* this.atmAdapterName = COBOL_ADAPTER_NAME; //?????
*
* this.setRebuildMessage(true);
*/
/*
* }
*/
/**
* Gets the name of the XML Converter to be used in the IMS Connect Adapter
* Task Manager
*
* @return a String
value containing the xmlConverterName
* property value
*/
public String getXmlConverterName() {
return this.xmlConverterName;
}
/**
* @param anXmlConverterName
* The xmlConverterName to set.
*/
public void setXmlConverterName(String anXmlConverterName)
throws ImsConnectApiException {
if ((anXmlConverterName.trim().equals(""))
|| (anXmlConverterName == null)) {
anXmlConverterName = EIGHT_BLANKS;
} else if (anXmlConverterName.length() > XML_CONVERTER_NAME_MAX_LEN) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0029E, new Object[] {
"xmlAdapterName", anXmlConverterName, });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0029E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setXmlAdapterName(String). Exception thrown was: "
+ e.toString());
throw e;
} else if (!(PropertiesFileLoader
.isValidHostStyleName(anXmlConverterName))) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0029E,
new Object[] { "xmlConverterName",
new String(anXmlConverterName) });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0029E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setRerouteName(String). Exception thrown was: "
+ e.toString());
throw e;
}
// String newConverterName = stringPad(anXmlConverterName, ' ',
// XML_CONVERTER_NAME_MAX_LEN);
if (this.xmlConverterName != anXmlConverterName) {
this.xmlConverterName = anXmlConverterName;
this.setRebuildMessage(true);
}
}
/**
* Gets the type of the XML message to be converted in IMS Connect
*
* @return a byte
value containing the xmlMessageType property
* value
*/
public byte getXmlMessageType() {
return this.xmlMessageType;
}
/**
* @param xmlMessageType
* The xmlMessageType to set.
*/
/*
* public void setXmlMessageType(byte xmlMessageType) { // add error
* handling code, i.e., if not valid value, create and throw exception - see
* setCommitMode(byte aCommitMode) this.xmlMessageType = xmlMessageType; }
*/
/**
* @param use2DimensionalByteArray
* the use2DimensionalByteArray to set
*/
/*
* protected void setUse2DimensionalByteArray(boolean
* use2DimensionalByteArray) { this.use2DimensionalByteArray =
* use2DimensionalByteArray; }
*
* /** Pads a String with a specified character.
*
* @param str the input String to be padded.
*
* @param ch the padding character.
*
* @param l the total length of the new String.
*/
/*
* public String stringPad(String str, char ch, int l) { StringBuffer sb =
* null; int len = 0;
*
* if (str == null) sb = new StringBuffer(); else { sb = new
* StringBuffer(str); len = str.length(); }
*
* for (int i = 1; i <= (l - len); i++) sb.append(ch);
*
* return new String(sb); }
*/
/*
* (non-Javadoc)
*
* @see java.lang.Object#toString()
*/
public String toString() {
String str =
// this.generateClientID + "," +
this.architectureLevel
+ ","
+ this.ackNakProvider
+ ","
+
// this.clientType + "," +
this.commitMode
+ ","
+ this.inputMessageDataSegmentsIncludeLlzzAndTrancode
+ ","
+ this.imsDatastoreName
+ ","
+ this.imsConnectUserMessageExitIdentifier
+ ","
+ this.inputMessageOptions
+ ","
+ this.interactionTypeDescription
+ ","
+ this.ltermOverrideName
+ ","
+
// this.mfsModnameOverride + "," +
this.resumeTpipeProcessing
+ ","
+ this.returnMfsModname
+ ","
+
// this.sendOnlyXcfOrderedDelivery + "," +
this.syncLevel
+ ","
+ this.imsDatastoreName
+ ","
+ this.trancode
+ ","
+
// this.xmlAdapterName + "," +
// this.xmlConverterName + "," +
// this.xmlAdapterType + "," +
// this.xmlMessageType + "," +
this.rerouteUndeliverableOutput + "," + this.rerouteName + ","
+ this.resumeTpipeAlternateClientId + "," + this.racfUserId
+ "," + this.racfPassword + "," + this.racfGroupName + ","
+ this.racfApplName + "," + this.interactionTimeout + ","
+ this.imsConnectTimeout + ","
+ this.imsConnectConvertedTimeout + ","
+ this.imsConnectTimeoutIndex + ","
+ this.otmaTransactionExpiration + "," + this.useCM0AckNoWait
+ "," + this.cM0AckNoWaitCanBeUsed + ","
+ this.imsConnectCodepage;// + "," +
// this.imsConnectUnicodeUsage;
return str;
}
/**
* Format a bytearray for output to the console or a trace file
*
* @return an int value representing the Driver Name
*/
protected String formatBufferForTracing(byte[] buffer,
boolean obfuscatePassword) {
StringBuffer strbuf = new StringBuffer(buffer.length * 4
+ (buffer.length / 16) * 6);
strbuf.append(" ");
String passwordObfuscationChar = "*";
byte passwordObfuscationByte = 0x00;
int hiByte;
int lowByte;
int rowByteCount = 0;
int totalByteCount = 0;
int numberOfBytesInRow = 32;
int startOfData = 116;
int positionOfPasswordBegin = 76;
int positionOfPasswordEnd = 83;
String str = "";
byte[] rowBytes;
byte[] irmRowBytes;
byte[] dataRowBytes;
try {
passwordObfuscationByte = (passwordObfuscationChar
.getBytes(imsConnectCodepage))[0];
} catch (UnsupportedEncodingException usee) {
}
for (int i = 0; i < buffer.length; i++) {
if ((i >= positionOfPasswordBegin) && (i <= positionOfPasswordEnd)
&& (obfuscatePassword)) {
hiByte = (int) ((passwordObfuscationByte & 0xF0) >> 4);
lowByte = (int) (passwordObfuscationByte & 0x0F);
} else {
hiByte = (int) ((buffer[i] & 0xF0) >> 4);
lowByte = (int) (buffer[i] & 0x0F);
}
strbuf.append(Integer.toString(hiByte, 16)); // append high-order
// nibble of a byte
strbuf.append(Integer.toString(lowByte, 16)); // append low-order
// nibble of a byte
rowByteCount++;
totalByteCount++;
if (rowByteCount % 4 == 0)
strbuf.append(" "); // add a space after every 4th byte
if (rowByteCount % 16 == 0)
strbuf.append(" "); // add an extra space after every 16th byte
if (rowByteCount == numberOfBytesInRow) // after row has been
// processed, format that
// row's bytes in
// human-readable form
{
try {
int startOfRow = i - numberOfBytesInRow + 1;
rowBytes = new byte[numberOfBytesInRow];
System.arraycopy(buffer, i - numberOfBytesInRow + 1,
rowBytes, 0, numberOfBytesInRow);
if (i < 116) {
if ((positionOfPasswordBegin >= (i - numberOfBytesInRow))
&& (positionOfPasswordEnd <= i)
&& (obfuscatePassword)) {
int startOfPasswordInRowBytes = positionOfPasswordBegin
- (i - numberOfBytesInRow + 1);
// String obfuscatedPasswordString = "********";
byte[] obfuscatedPasswordBytes = new String(
"********")
.getBytes(this.imsConnectCodepage);
str = new String(rowBytes, 0,
startOfPasswordInRowBytes,
imsConnectCodepage)
+ // row up to password
// str = new String(rowBytes, 0,
// startOfPasswordInRowBytes) + // row up to
// password
new String(obfuscatedPasswordBytes, 0, 8,
imsConnectCodepage) + // obfuscated
// password
// new String(obfuscatedPasswordBytes, 0, 8)
// + // obfuscated password
new String(
rowBytes,
startOfPasswordInRowBytes + 8,
rowByteCount
- (startOfPasswordInRowBytes + 8),
imsConnectCodepage); // rest of row
// after
// password
// new String(rowBytes, startOfPasswordInRowBytes +
// 8, rowByteCount - (startOfPasswordInRowBytes +
// 8)); // rest of row after password
} else
str = new String(rowBytes, 0, rowByteCount,
imsConnectCodepage);
// str = new String(rowBytes, 0, rowByteCount);
} else if ((startOfRow) >= 116) {
str = new String(rowBytes, 0, rowByteCount,
imsConnectCodepage);
// str = new String(rowBytes, 0, rowByteCount);
} else {
int startOfDataInRowString = startOfData
- (i - numberOfBytesInRow);
int irmInRowLen = startOfDataInRowString;
int dataInRowLen = numberOfBytesInRow - irmInRowLen;
int dataInRowLen1 = buffer.length - startOfData;
if (dataInRowLen1 < dataInRowLen)
dataInRowLen = dataInRowLen1;
irmRowBytes = new byte[irmInRowLen];
dataRowBytes = new byte[dataInRowLen];
System.arraycopy(buffer, i - numberOfBytesInRow + 1,
irmRowBytes, 0, irmInRowLen);
System.arraycopy(buffer, startOfData + 1, dataRowBytes,
0, dataInRowLen);
str = new String(irmRowBytes, 0, irmInRowLen,
imsConnectCodepage)
+
// str = new String(irmRowBytes, 0, irmInRowLen)
// +
new String(dataRowBytes, 0, dataInRowLen,
imsConnectCodepage);
// new String(dataRowBytes, 0, dataInRowLen);
}
strbuf.append("|");
for (int m = 0; m < rowByteCount; m++) {
if (isPrintableChar(str.charAt(m)))
strbuf.append(str.charAt(m));
else
strbuf.append(".");
}
strbuf.append("|");
} catch (Exception e) {
// System.out.println(e.getMessage());
}
strbuf.append(" : ");
strbuf.append(totalByteCount);
strbuf.append("\n ");
rowByteCount = 0;
}
}
if (rowByteCount != 0) // last row is not a full row of 32 bytes
{
for (int l = rowByteCount; l < 32; l++) {
strbuf.append(" ");
if ((l + 1) % 4 == 0)
strbuf.append(" "); // add an extra space after every 4th
// byte
if ((l + 1) % 16 == 0)
strbuf.append(" "); // add an extra space after every 16th
// byte
}
try {
str = new String(buffer, buffer.length - rowByteCount,
rowByteCount, imsConnectCodepage);
// str = new String(buffer, buffer.length - rowByteCount,
// rowByteCount);
strbuf.append("|");
int m;
for (m = 0; m < rowByteCount; m++) {
if (isPrintableChar(str.charAt(m)))
strbuf.append(str.charAt(m));
else
strbuf.append(".");
}
for (; m < 32; m++)
strbuf.append(" ");
strbuf.append("|");
} catch (Exception e) {
}
strbuf.append(" : ");
strbuf.append(totalByteCount);
}
return new String(strbuf);
}
/**
* Interpret IRM
*
* @return an array of Strings containing the interpreted IRM
*/
protected String[] interpretIrm(byte[] anInputByteArray)
throws UnsupportedEncodingException {
String[] returnStringArray = new String[44];
// String asciiStr = new String(anInputByteArray, 0,
// anInputByteArray.length, DEFAULT_CODEPAGE_ASCII_US);
// System.out.println("asciiStr = [" + asciiStr + "]");
// String ebcdicStr = new String(anInputByteArray, 0,
// anInputByteArray.length, DEFAULT_CODEPAGE_EBCDIC_US);
// System.out.println("ebcdicStr = [" + ebcdicStr + "]");
String inputByteArrayAsString = new String(anInputByteArray, 0,
anInputByteArray.length, this.imsConnectCodepage);
long llll = (long) (((anInputByteArray[0] & 0xFF) << 24)
| // 0-3 (80)
((anInputByteArray[1] & 0xFF) << 16)
| ((anInputByteArray[2] & 0xFF) << 8) | (anInputByteArray[3] & 0xFF));
short irm_ll = (short) (((anInputByteArray[4] & 0xFF) << 8) | // 4-5
// (70)
(anInputByteArray[5] & 0xFF));
byte archLvl = (byte) anInputByteArray[6]; // 6 (02)
byte f0 = (byte) anInputByteArray[7]; // 7 (00 - no XML)
String irmId = new String(anInputByteArray, 8, 8,
this.imsConnectCodepage); // 8-15 (*SAMPLE*)
// String irmId = new String(anInputByteArray,8,8); // 8-15 (*SAMPLE*)
short irmNakReasonCode = (short) (((anInputByteArray[16] & 0xFF) << 8) | // 4-5
// (70)
(anInputByteArray[17] & 0xFF));
short resWrd = (short) (((anInputByteArray[18] & 0xFF) << 8) | // 4-5
// (70)
(anInputByteArray[19] & 0xFF));
byte f5 = (byte) anInputByteArray[20]; // 20 (00 - no option flow of
// messages)
byte irmTimer = (byte) anInputByteArray[21]; // 21 (00 - use ICON
// default TIMEOUT
// value)
byte sockType = (byte) anInputByteArray[22]; // 22 (10 - persistent)
byte encSchema = (byte) anInputByteArray[23]; // 23 (01 - UTF-8)
String clientId = new String(anInputByteArray, 24, 8,
this.imsConnectCodepage); // 24-31 (CLIENT01)
// String clientId = new String(anInputByteArray,24,8); // 24-31
// (CLIENT01)
byte f1 = (byte) anInputByteArray[32]; // 32 (00)
byte f2 = (byte) anInputByteArray[33]; // 33 (20 - CM1)
byte f3 = (byte) anInputByteArray[34]; // 34 (09 - SL CONFIRM + reRoute
// flag)
byte f4 = (byte) anInputByteArray[35]; // 35 (40 - EBCDIC ' ' (blank)
// for send-recv)
String irmTrancode = new String(anInputByteArray, 36, 8,
this.imsConnectCodepage); // 36-43 (ITOC04 )
// String irmTrancode = new String(anInputByteArray,36,8); // 36-43
// (ITOC04 )
String datastoreId = new String(anInputByteArray, 44, 8,
this.imsConnectCodepage); // 44-51 (IMS1 )
// String datastoreId = new String(anInputByteArray,44,8); // 44-51
// (IMS1 )
String ltermOvrd = new String(anInputByteArray, 52, 8,
this.imsConnectCodepage); // 52-59 (LTRMOVRD)
// String ltermOvrd = new String(anInputByteArray,52,8); // 52-59
// (LTRMOVRD)
String racfUserId = new String(anInputByteArray, 60, 8,
this.imsConnectCodepage); // 60-67 (RACFUSID)
// String racfUserId = new String(anInputByteArray,60,8); // 60-67
// (RACFUSID)
String racfGroup = new String(anInputByteArray, 68, 8,
this.imsConnectCodepage); // 68-75 (RACFGRUP)
// String racfGroup = new String(anInputByteArray,68,8); // 68-75
// (RACFGRUP)
// String racfPassword = new
// String(anInputByteArray,76,8,this.imsConnectCodepage); // 76-83
// (RACFPSWD)
String racfPassword = new String("********"); // 76-83 (obfuscated
// RACFPSWD)
String racfApplNm = new String(anInputByteArray, 84, 8,
this.imsConnectCodepage); // 84-91 (RACFAPPL)
// String racfApplNm = new String(anInputByteArray,84,8); // 84-91
// (RACFAPPL)
String rerouteName = new String(anInputByteArray, 92, 8,
this.imsConnectCodepage); // 92-99 (REROUTNM)
// String rerouteName = new String(anInputByteArray,92,8); // 92-99
// (REROUTNM)
String xmlAdapterNm = new String(anInputByteArray, 100, 8,
this.imsConnectCodepage); // 100-107 (HWSXMLA0)
// String xmlAdapterNm = new String(anInputByteArray,100,8); // 100-107
// (HWSXMLA0)
String xmlConvrtrNm = new String(anInputByteArray, 108, 8,
this.imsConnectCodepage); // 108-115 (HWSXCNV0)
// String xmlConvrtrNm = new String(anInputByteArray,108,8); // 108-115
// (HWSXCNV0)
String irmModNm = new String(anInputByteArray, 116, 8,
this.imsConnectCodepage); // 116-123
StringBuffer correlatorTokenStringBuffer = new StringBuffer("");
String correlatorTokenString = "00000000000000000000000000000000000000000000000000000000000000000000000000000000";
if ((f4 == 0x4D) || (f4 == -44)||(f4 == 0x4C) || (f4 == -45)) {
for (int i = 0; i <= IRM_CT_LEN; i++) {
correlatorTokenStringBuffer
.append(convertByteValueTo2CharacterString(anInputByteArray[124 + i]));
}
correlatorTokenString = correlatorTokenStringBuffer.toString();
}
// byte[] correlatorToken = new byte[IRM_CT_LEN];
// for(int i=0;i> 24)))
.toUpperCase()
+ (convertByteValueTo2CharacterString((byte) ((llll & 0x00FF0000) >> 16)))
.toUpperCase()
+ (convertByteValueTo2CharacterString((byte) ((llll & 0x0000FF00) >> 8)))
.toUpperCase()
+ (convertByteValueTo2CharacterString((byte) (llll & 0x000000FF)))
.toUpperCase() + " (decimal " + String.valueOf(llll)
+ ")"; // short
// returnStringArray[1] = "\n IRM length (ll) = 0x" +
// Long.toHexString(new Short(irm_ll).longValue()).toUpperCase() +
// " (decimal " + String.valueOf(irm_ll) + "]"; // short
returnStringArray[1] = "\n IRM length (ll) = 0x"
+ (convertByteValueTo2CharacterString((byte) ((irm_ll & 0xFF00) >> 8)))
.toUpperCase()
+ (convertByteValueTo2CharacterString((byte) (irm_ll & 0x00FF)))
.toUpperCase() + " (decimal " + String.valueOf(irm_ll)
+ ")"; // short
returnStringArray[2] = "\n Archictecture Level = 0x"
+ convertByteValueTo2CharacterString(archLvl); // byte
returnStringArray[3] = "\n Flag 0 = 0x"
+ convertByteValueTo2CharacterString(f0) + " (decimal "
+ (String.valueOf(f0)) + ")";
// ------------------Added for Sync
// callout------------------------------////
// if((f4 == 0x4D) || (f4 == -44)) // 'M' // submitting sync callout
// response
// {
// if(((byte)f0 & (byte)0x20) == (byte)0x20) // sync callout NAK
// response - retain callout message on Tpipe
// returnStringArray[4] =
// " NAK of SENDONLYCALLOUTRESPONSE interaction - keep callout message on Tpipe queue";
// }
// else if((f4 == 0x52) || (f4 == -39)) // 'R' // submitting resumeTpipe
// request
if ((f4 == 0x52) || (f4 == -39)) // 'R' // submitting resumeTpipe
// request
{
if (((byte) f0 & RETRIEVE_SYNC_MESSAGE_ONLY) == RETRIEVE_SYNC_MESSAGE_ONLY) // retrieve
// sync
// callout
// messages
// only
returnStringArray[4] = " resumeTPipeRetrievalType is RETRIEVE_SYNC_MESSAGE_ONLY";
else if (((byte) f0 & RETRIEVE_SYNC_OR_ASYNC_MESSAGE) == RETRIEVE_SYNC_OR_ASYNC_MESSAGE) // retrieve
// sync
// or
// async
// callout
// messages
// or
// async
// output
// messages
returnStringArray[4] = " resumeTPipeRetrievalType is RETRIEVE_SYNC_OR_ASYNC_MESSAGE";
else
// if (((byte)f0 & RETRIEVE_ASYNC_MESSAGE_ONLY) ==
// RETRIEVE_ASYNC_MESSAGE_ONLY) // retrieve async callout or
// non-callout messages
returnStringArray[4] = " resumeTPipeRetrievalType is RETRIEVE_ASYNC_MESSAGE_ONLY";
} else if ((f4 == 0x4E) || (f4 == -43)) // 'N' // submitting NAK
{
if (((byte) f0 & NAK_REQUEUE_SYNC_CALLOUT_REQUEST_END_RESUMETPIPE_VALUE) == NAK_REQUEUE_SYNC_CALLOUT_REQUEST_END_RESUMETPIPE_VALUE)
returnStringArray[4] = " If NAK is NAKing callout request from callout resumetpipe, OTMA will requeue callout request message on Tpipe queue; resumeTpipe will get timeout response";
else if (((byte) f3 & NAK_DISCARD_SYNC_CALLOUT_REQUEST_CONTINUE_RESUMETPIPE_VALUE) == NAK_DISCARD_SYNC_CALLOUT_REQUEST_CONTINUE_RESUMETPIPE_VALUE)
returnStringArray[4] = " If NAK is NAKing callout request from callout resumetpipe, OTMA will discard callout request message; resumeTpipe will continue";
else
returnStringArray[4] = " If NAK is NAKing callout request from callout resumetpipe, OTMA will discard callout request message; resumeTpipe will get timeout response";
} else
returnStringArray[4] = " "
+ ((String.valueOf(f0)).equals("0") ? "No XML transformation"
: String.valueOf(f0).equals("1") ? "XML transformation of trancode and data"
: String.valueOf(f0).equals("2") ? "XML transformation of data only)"
: ""); // byte
// ----------End-----------------------
returnStringArray[5] = "\n IRM identifier = [" + irmId
+ "]"; // String
// returnStringArray[6] = "\n NAK reason code = 0x" +
// Long.toHexString(new
// Short(irmNakReasonCode).longValue()).toUpperCase() + " (decimal " +
// String.valueOf(irmNakReasonCode) + "]"; // short
returnStringArray[6] = "\n NAK reason code = 0x"
+ (convertByteValueTo2CharacterString((byte) ((irmNakReasonCode & 0xFF00) >> 8)))
.toUpperCase()
+ (convertByteValueTo2CharacterString((byte) (irmNakReasonCode & 0x00FF)))
.toUpperCase() + " (decimal "
+ String.valueOf(irmNakReasonCode) + "]"; // short
// returnStringArray[7] = "\n Reserved word = 0x" +
// Long.toHexString(new Short(resWrd).longValue()).toUpperCase() +
// " (decimal " + String.valueOf(resWrd) + "]"; // short
returnStringArray[7] = "\n Reserved word = 0x"
+ (convertByteValueTo2CharacterString((byte) ((resWrd & 0xFF00) >> 8)))
.toUpperCase()
+ (convertByteValueTo2CharacterString((byte) (resWrd & 0x00FF)))
.toUpperCase() + " (decimal " + String.valueOf(resWrd)
+ "]"; // short
returnStringArray[8] = "\n Flag 5 = 0x"
+ convertByteValueTo2CharacterString(f5) + " (decimal "
+ String.valueOf(f5) + "]"; // byte
if ((f5 & (byte) 0x40) == 0x40)
returnStringArray[9] = " EBCDIC translation done by client";
else
returnStringArray[9] = " EBCDIC translation not done by client";
if ((f5 & (byte) 0x10) == 0x10)
returnStringArray[10] = " resumeTpipe option is SINGLE_WAIT";
else if ((f5 & (byte) 0x01) == 0x01)
returnStringArray[10] = " resumeTpipe option is SINGLE_NOWAIT";
else if ((f5 & (byte) 0x02) == 0x02)
returnStringArray[10] = " resumeTpipe option is AUTO";
else if ((f5 & (byte) 0x04) == 0x04)
returnStringArray[10] = " resumeTpipe option is NOAUTO";
else if ((f5 & (byte) 0x00) == 0x00)
returnStringArray[10] = " resumeTpipe option is NOOPTION (same as NOAUTO)";
returnStringArray[11] = "\n IMS Connect timeout = 0x"
+ convertByteValueTo2CharacterString(irmTimer) + " (decimal "
+ String.valueOf(irmTimer) + ")"; // byte
returnStringArray[12] = "\n Socket type = 0x"
+ convertByteValueTo2CharacterString(sockType) + " (decimal "
+ String.valueOf(sockType) + ")"; // byte
if ((sockType & (byte) 0x10) == 0x00)
returnStringArray[13] = " Transaction socket";
else if ((sockType & (byte) 0x10) == 0x10)
returnStringArray[13] = " Persistent socket";
returnStringArray[14] = "\n Unicode encoding schema = 0x"
+ convertByteValueTo2CharacterString(encSchema) + " (decimal "
+ encSchema + ")"; // byte
returnStringArray[15] = "\n clientId = ["
+ clientId + "]\n"; // String
returnStringArray[16] = "\n Flag 1 = 0x"
+ convertByteValueTo2CharacterString(f1) + " (decimal "
+ String.valueOf(f1) + ")"; // byte
if (((byte) f1 & (byte) 0x01) == (byte) 0x01)
returnStringArray[17] = " OTMA Transaction Expiration is true";
else
returnStringArray[17] = " OTMA Transaction Expiration is false";
if (((byte) f1 & (byte) 0x02) == (byte) 0x02)
returnStringArray[18] = "\n CM0 ACK/NAK No Wait requested";
else
returnStringArray[18] = "\n CM0 ACK/NAK No Wait not requested";
if (((byte) f1 & (byte) 0x30) == (byte) 0x00)
returnStringArray[19] = "\n Neither trancode nor data are Unicode";
else if (((byte) f1 & (byte) 0x10) == (byte) 0x10)
returnStringArray[19] = "\n Trancode is Unicode";
else if (((byte) f1 & (byte) 0x20) == (byte) 0x20)
returnStringArray[19] = "\n Data is Unicode";
else if (((byte) f1 & (byte) 0x30) == (byte) 0x30)
returnStringArray[19] = "\n Trancode and data are both Unicode";
if (((byte) f1 & (byte) 0x80) == (byte) 0x80)
returnStringArray[20] = "\n Return MFS modname requested";
else
returnStringArray[20] = "\n Return MFS modname not requested";
if (((byte) f1 & (byte) 0x40) == (byte) 0x40)
returnStringArray[21] = "\n Return ClientId requested";
else
returnStringArray[21] = "\n Return ClientId not requested";
returnStringArray[22] = "\n Flag 2 = 0x"
+ convertByteValueTo2CharacterString(f2) + " (decimal "
+ String.valueOf(f2) + ")"; // byte
if (((byte) f2 & (byte) 0x20) == (byte) 0x20)
returnStringArray[23] = " Commit mode 1";
else if (((byte) f2 & (byte) 0x40) == (byte) 0x40)
returnStringArray[23] = " Commit mode 0";
if (((byte) f2 & (byte) 0x01) == (byte) 0x01)
returnStringArray[24] = "\n Generate clientId when Duplicate requested";
else
returnStringArray[24] = "\n Generate clientId when Duplicate not requested";
returnStringArray[25] = "\n Flag 3 = 0x"
+ convertByteValueTo2CharacterString(f3) + " (decimal "
+ String.valueOf(f3) + ")"; // byte
if (((byte) f3 & (byte) 0x03) == (byte) 0x00)
returnStringArray[26] = " Sync Level is NONE (0)";
else if (((byte) f3 & (byte) 0x03) == (byte) 0x01)
returnStringArray[26] = " Sync Level is CONFIRM (1)";
else if (((byte) f3 & (byte) 0x03) == (byte) 0x02)
returnStringArray[26] = " Sync Level is SYNCPT (2)";
if (((byte) f3 & (byte) 0x04) == (byte) 0x04)
returnStringArray[27] = " Purge undeliverable output is true";
else
returnStringArray[27] = " Purge undeliverable output is false";
if (((byte) f3 & (byte) 0x08) == (byte) 0x08)
returnStringArray[28] = " Reroute undeliverable output is true";
else
returnStringArray[28] = " Reroute undeliverable output is false";
if (((byte) f3 & (byte) 0x40) == (byte) 0x40)
returnStringArray[29] = " Return DFS2082 after CM0 SendRecv timeout with no response is true";
else
returnStringArray[29] = " Return DFS2082 after CM0 SendRecv timeout with no response is false";
// returnStringArray[28] = "\n Flag 4 = 0x" +
// convertByteValueTo2CharacterString(f4) + " (decimal " +
// String.valueOf(f4) + ", chararcter ["+
// inputByteArrayAsString.charAt(35) + "])";
returnStringArray[30] = "\n Flag 4 = 0x"
+ convertByteValueTo2CharacterString(f4) + " (decimal "
+ String.valueOf(f4) + ", chararcter [" + (char) f4 + "])";
if ((f4 == 0x20) || (f4 == 0x40)) // ' '
returnStringArray[31] = " SENDRECV interaction";
else if ((f4 == 0x41) || (f4 == -63)) // 'A'
returnStringArray[31] = " ACK interaction";
else if ((f4 == 0x4E) || (f4 == -43)) // 'N'
returnStringArray[31] = " NAK interaction";
else if ((f4 == 0x44) || (f4 == -60)) // 'D'
returnStringArray[31] = " DEALLOCATE interaction";
else if ((f4 == 0x52) || (f4 == -39)) // 'R'
returnStringArray[31] = " RESUMETPIPE interaction";
else if ((f4 == 0x53) || (f4 == -30)) // 'S'
{
if (((byte) f3 & (byte) 0x10) != (byte) 0x10)
returnStringArray[31] = " SENDONLY interaction";
else
returnStringArray[31] = " SENDONLYXCFORDDEL interaction";
}// ------------------Added for Sync
// callout------------------------------////
else if ((f4 == 0x4D) || (f4 == -44)) // 'M'
{
returnStringArray[31] = " SENDONLYCALLOUTRESPONSE interaction";
}
else if ((f4 == 0x4C) || (f4 == -45)) // 'L'
{
returnStringArray[31] = " SENDONLYACKCALLOUTRESPONSE interaction";
}
// --------------End---------------------------------------////////////////
else if ((f4 == 0x43) || (f4 == -61)) // 'C'
returnStringArray[31] = " CANCELTIMER interaction";
else if ((f4 == 0x4B) || (f4 == -46)) // 'K'
returnStringArray[31] = " SENDONLYACK interaction";
returnStringArray[32] = "\n Trancode = ["
+ irmTrancode + "]"; // String
returnStringArray[33] = "\n Datastore ID = ["
+ datastoreId + "]"; // String
returnStringArray[34] = "\n LTERM override = ["
+ ltermOvrd + "]"; // String
returnStringArray[35] = "\n RACF userId = ["
+ racfUserId + "]"; // String
returnStringArray[36] = "\n RACF group name = ["
+ racfGroup + "]"; // String
returnStringArray[37] = "\n RACF password = ["
+ racfPassword + "]"; // String
returnStringArray[38] = "\n RACF appl name = ["
+ racfApplNm + "]"; // String
if ((f4 == 0x52) || (f4 == -39)) // 'R' for resumeTpipe
returnStringArray[39] = "\n ResumeTpipe alternate clientId = ["
+ resumeTpipeAlternateClientId + "]"; // String
else
returnStringArray[39] = "\n Reroute name = ["
+ rerouteName + "]"; // String
returnStringArray[40] = "\n XML adapter name = ["
+ xmlAdapterNm + "] (not supported in API)"; // String
returnStringArray[41] = "\n XML converter name = ["
+ xmlConvrtrNm + "] (not supported in API)"; // String
returnStringArray[42] = "\n Input MFS MOD name = ["
+ irmModNm + "]"; // String
returnStringArray[43] = "\n Correlator token = ["
+ correlatorTokenString + "]"; // bytes
return returnStringArray;
}
private static boolean isPrintableChar(char ch) {
if (Character.isISOControl(ch))
return false;
return !(Character.isIdentifierIgnorable(ch));
}
private static String convertByteValueTo2CharacterString(byte aByte) {
int b;
StringBuffer hexCharStringBuf = new StringBuffer("");
b = (int) ((aByte & 0xF0) >> 4); // get high-order nibble of a byte and
// shift right 4 bits
hexCharStringBuf.append(Integer.toHexString(b)); // concatenate shifted
// high-order nibble
// of a byte
b = (int) (aByte & 0x0F); // get low-order nibble of a byte
hexCharStringBuf.append(Integer.toHexString(b)); // concatenate
// low-order nibble
// of a byte
return hexCharStringBuf.toString().toUpperCase();
}
/**
* Convert a String containing newline characters) to an array of Strings
*
* @return an array of Strings
*/
protected String[] stringToStringArray(String aMultiLineString) {
int rows = 1;
String tempstr = aMultiLineString.toString();
int bol = 0;
int eol = tempstr.indexOf('\n');
int tempstrlen = tempstr.length() - 1;
while ((eol != -1) && (eol < tempstrlen)) {
bol = eol + 1;
eol = tempstr.indexOf('\n', bol);
rows++;
}
String[] formattedBuffer = new String[rows];
bol = 0;
eol = tempstr.indexOf('\n');
for (int i = 0; i < rows; i++) {
if (eol > -1)
formattedBuffer[i] = new String(tempstr.substring(bol, eol));
else
formattedBuffer[i] = new String(tempstr.substring(bol));
bol = eol + 1;
eol = tempstr.indexOf('\n', bol);
}
return formattedBuffer;
}
// ------------------Added for Sync
// callout------------------------------////
public String getCalloutRequestNakProcessing() {
return this.calloutRequestNakProcessing;
}
public String getCalloutResponseMessageType() {
return this.calloutResponseMessageType;
}
public byte getResumeTpipeRetrievalType() {
return this.resumeTPipeRetrievalType;
}
public void setCalloutRequestNakProcessing(String aCalloutNakProcessing)
throws ImsConnectApiException {
String tempaCalloutNakProcessing = aCalloutNakProcessing.toUpperCase();
if (allValidCalloutRequestNakProcessingsString
.indexOf(tempaCalloutNakProcessing) == -1) {
String validCalloutRequestNakProcessing = "";
try {
validCalloutRequestNakProcessing = ImsConnectErrorMessage
.getString(com.ibm.ims.connect.ImsConnectErrorMessage.VALID_PROPERTY_VALUE_CALLOUTREQUESTNAKPROCESSING);
} catch (Exception e) {
}
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0030E, new Object[] {
"calloutRequestNakProcessing",
aCalloutNakProcessing,
validCalloutRequestNakProcessing });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0030E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setCalloutRequestNakProcessing(): ["
+ e.toString() + "]");
throw e;
}
if (this.calloutRequestNakProcessing != tempaCalloutNakProcessing) {
this.calloutRequestNakProcessing = tempaCalloutNakProcessing;
this.setRebuildMessage(true);
}
}
public void setCalloutResponseMessageType(String aCalloutResponseMsgType)
throws ImsConnectApiException {
String tempaCalloutResponseMsgType = aCalloutResponseMsgType
.toUpperCase();
if (allValidCalloutResponseMessageTypesString
.indexOf(tempaCalloutResponseMsgType) == -1) {
String validCalloutResponseMsgTypes = "";
try {
validCalloutResponseMsgTypes = ImsConnectErrorMessage
.getString(com.ibm.ims.connect.ImsConnectErrorMessage.VALID_PROPERTY_VALUE_RESPONSEMESSAGETYPE);
} catch (Exception e) {
}
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0030E, new Object[] {
"calloutResponseMessageType",
aCalloutResponseMsgType,
validCalloutResponseMsgTypes });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0030E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setCalloutResponseMessageType(): ["
+ e.toString() + "]");
throw e;
}
if (this.calloutResponseMessageType != tempaCalloutResponseMsgType) {
this.calloutResponseMessageType = tempaCalloutResponseMsgType;
this.setRebuildMessage(true);
}
}
public void setResumeTpipeRetrievalType(byte aRetrievalType)
throws ImsConnectApiException {
// if (((aSyncLevel & SYNC_LEVEL_NONE) == SYNC_LEVEL_NONE) ||
// (aSyncLevel == SYNC_LEVEL_CONFIRM))
if ((aRetrievalType == RETRIEVE_SYNC_MESSAGE_ONLY)
|| (aRetrievalType == RETRIEVE_SYNC_OR_ASYNC_MESSAGE)
|| (aRetrievalType == RETRIEVE_ASYNC_MESSAGE)) {
if (this.resumeTPipeRetrievalType != aRetrievalType) {
this.resumeTPipeRetrievalType = aRetrievalType;
this.setRebuildMessage(true);
}
} else {
String validResumeTpipeRetrievalTypeString = "";
try {
validResumeTpipeRetrievalTypeString = ImsConnectErrorMessage
.getString(ImsConnectErrorMessage.VALID_PROPERTY_VALUE_RESUMETPIPERETRIEVALTYPE);
} catch (Exception e) {
}
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0030E, new Object[] {
"ResumeTpipeRetrievalType",
String.valueOf(aRetrievalType),
validResumeTpipeRetrievalTypeString });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0030E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setResumeTpipeRetrievalType(byte). Exception thrown was: "
+ e.toString());
throw e;
}
}
public void setCorrelatorToken(byte[] aCorrelatorToken)
throws ImsConnectApiException {
this.correlatorTkn = aCorrelatorToken;
// Assigning it to the class also
// CorrelatorToken tkn = this.new CorrelatorToken();
// tkn.setCorrelatorTkn(this.correlatorTkn);
this.setRebuildMessage(true);
}
public byte[] getCorrelatorToken() {
return this.correlatorTkn;
}
/**
* @param nakReasonCode
* the nakReasonCode to set
*/
public void setNakReasonCode(short aNakReasonCode) {
this.nakReasonCode = aNakReasonCode;
this.setRebuildMessage(true);
}
/**
* @return the nakReasonCode
*/
public short getNakReasonCode() {
return nakReasonCode;
}
/**
* @param inputModName
* the inputModName to set
* @throws ImsConnectApiException
*/
public void setInputModName(String anInputModName)
throws ImsConnectApiException {
// this.inputModName = inputModName;
if ((anInputModName).trim().equals("") || (anInputModName == null)) // all
// blanks
// is
// a
// valid
// aRacfUserId
{
anInputModName = EIGHT_BLANKS;
} else if (anInputModName.length() > MAX_LEN_INPUT_MOD_NAME) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0026E, new Object[] {
anInputModName, "inputModName",
String.valueOf(MAX_LEN_INPUT_MOD_NAME) });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0026E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setInputModName(String). Exception thrown was: "
+ e.toString());
throw e;
} else if (!(PropertiesFileLoader.isValidHostStyleName(anInputModName))) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0029E, new Object[] {
"inputModName", anInputModName });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0029E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception thrown in TmInteraction.setInputModName(String). Exception thrown was: "
+ e.toString());
throw e;
}
if (this.inputModName != anInputModName) {
this.inputModName = anInputModName;
this.setRebuildMessage(true);
this.updateIrmInputModName = true;
}
}
/**
* @return the inputModName
*/
public String getInputModName() {
return inputModName;
}
/**
* @Format and print out the receive buffer to the trace log
*/
protected void formatAndPrintReceiveBuffer(boolean obfuscatePassword) {
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_INTERNAL)) {
String bufferReceived = this.formatBufferForTracing(outputMsgBytes,
obfuscatePassword);
String[] bufferReceivedStringArray = this
.stringToStringArray(bufferReceived);
int bufferRecvLen = bufferReceivedStringArray.length;
logger.finest(" TmInteraction.execute() - Buffer received:");
for (int i = 0; i < bufferRecvLen; i++) {
logger.finest(bufferReceivedStringArray[i]);
}
}
}
public final class CorrelatorToken {
private short IRM_CT_LEN;
private short IRM_CT_RESV1;
private String IRM_CT_IMSID;
private String IRM_CT_MEMTK;
private String IRM_CT_AWETK;
private String IRM_CT_TPIPE;
private String IRM_CT_USERID;
public short getCorrelatorLength() throws ImsConnectApiException {
try{
byte[]tkn = TmInteractionImpl.this.getCorrelatorToken();
this.IRM_CT_LEN =(short) (((tkn[0] & 0xFF) << 8) | (tkn[1] & 0xFF));
}
catch (Exception e1) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0001E,
new Object[] { ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.MSG_BLD_ERR,
new Object[] { ImsConnectErrorMessage
.getExceptionMessage(e1) }) });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0001E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in getCorrelatorLength(): ["
+ e.toString() + "]+");
throw e;
}
return this.IRM_CT_LEN;
}
@SuppressWarnings("unused")
private short getCorrelatorTokenResv1() throws ImsConnectApiException {
try{
//Getting position from where the value needs to be extracted.
int i =IRM_CT_LEN_SIZE;
//Getting the correlator token
byte[]tkn = TmInteractionImpl.this.getCorrelatorToken();
this.IRM_CT_RESV1 = (short) (((tkn[i] & 0xFF) << 8) | (tkn[i+1] & 0xFF));
}
catch (Exception e1) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0001E,
new Object[] { ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.MSG_BLD_ERR,
new Object[] { ImsConnectErrorMessage
.getExceptionMessage(e1) }) });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0001E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in getCorrelatorTokenResv1(): ["
+ e.toString() + "]+");
throw e;
}
return this.IRM_CT_RESV1;
}
public String getCorrelatorIMSID() throws ImsConnectApiException {
try{
//Getting position from where the value needs to be extracted.
int i =IRM_CT_LEN_SIZE +IRM_CT_RESV1_LEN ;
byte[]tkn = TmInteractionImpl.this.getCorrelatorToken();
this.IRM_CT_IMSID = new String(tkn,i,IRM_CT_IMSID_LEN,TmInteractionImpl.this.imsConnectCodepage);
}
catch (Exception e1) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0001E,
new Object[] { ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.MSG_BLD_ERR,
new Object[] { ImsConnectErrorMessage
.getExceptionMessage(e1) }) });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0001E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in getCorrelatorIMSID(): ["
+ e.toString() + "]+");
throw e;
}
return this.IRM_CT_IMSID;
}
public String getCorrelatorIOTMATMember() throws ImsConnectApiException {
try{
//Getting position from where the value needs to be extracted.
int i =IRM_CT_LEN_SIZE +IRM_CT_RESV1_LEN +IRM_CT_IMSID_LEN;
byte[]tkn = TmInteractionImpl.this.getCorrelatorToken();
this.IRM_CT_MEMTK = new String(tkn,i,IRM_CT_MEMTK_LEN,TmInteractionImpl.this.imsConnectCodepage);
}
catch (Exception e1) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0001E,
new Object[] { ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.MSG_BLD_ERR,
new Object[] { ImsConnectErrorMessage
.getExceptionMessage(e1) }) });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0001E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in getCorrelatorIOTMATMember(): ["
+ e.toString() + "]+");
throw e;
}
return this.IRM_CT_MEMTK;
}
public String getCorrelatorOTMAMessageToken() throws ImsConnectApiException {
try{
//Getting position from where the value needs to be extracted.
int i =IRM_CT_LEN_SIZE +IRM_CT_RESV1_LEN +IRM_CT_IMSID_LEN+IRM_CT_MEMTK_LEN;
byte[]tkn = TmInteractionImpl.this.getCorrelatorToken();
this.IRM_CT_AWETK = new String(tkn,i,IRM_CT_AWETK_LEN,TmInteractionImpl.this.imsConnectCodepage);
}
catch (Exception e1) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0001E,
new Object[] { ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.MSG_BLD_ERR,
new Object[] { ImsConnectErrorMessage
.getExceptionMessage(e1) }) });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0001E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in getCorrelatorOTMAMessageToken: ["
+ e.toString() + "]+");
throw e;
}
return this.IRM_CT_AWETK;
}
public String getCorrelatorOTMATPipeName() throws ImsConnectApiException {
try{
//Getting position from where the value needs to be extracted.
int i =IRM_CT_LEN_SIZE +IRM_CT_RESV1_LEN +IRM_CT_IMSID_LEN+IRM_CT_MEMTK_LEN+IRM_CT_AWETK_LEN;
byte[]tkn = TmInteractionImpl.this.getCorrelatorToken();
this.IRM_CT_TPIPE = new String(tkn,i,IRM_CT_TPIPE_LEN,TmInteractionImpl.this.imsConnectCodepage);
}
catch (Exception e1) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0001E,
new Object[] { ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.MSG_BLD_ERR,
new Object[] { ImsConnectErrorMessage
.getExceptionMessage(e1) }) });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0001E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in getCorrelatorOTMATPipeName(): ["
+ e.toString() + "]+");
throw e;
}
return this.IRM_CT_TPIPE;
}
public String getCorrelatorICALUserId() throws ImsConnectApiException {
try{
//Getting position from where the value needs to be extracted.
int i =IRM_CT_LEN_SIZE +IRM_CT_RESV1_LEN +IRM_CT_IMSID_LEN+IRM_CT_MEMTK_LEN+IRM_CT_AWETK_LEN+IRM_CT_TPIPE_LEN;
byte[]tkn = TmInteractionImpl.this.getCorrelatorToken();
this.IRM_CT_USERID = new String(tkn,i,IRM_CT_USERID_MAX_LEN,TmInteractionImpl.this.imsConnectCodepage);
}
catch (Exception e1) {
String errMsg = ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.HWS0001E,
new Object[] { ImsConnectErrorMessage.getString(
ImsConnectErrorMessage.MSG_BLD_ERR,
new Object[] { ImsConnectErrorMessage
.getExceptionMessage(e1) }) });
ImsConnectApiException e = new ImsConnectApiException(
ImsConnectErrorMessage.HWS0001E, errMsg);
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_EXCEPTION))
logger
.severe(" Exception caught in getCorrelatorICALUserId(): ["
+ e.toString() + "]+");
throw e;
}
return this.IRM_CT_USERID;
}
}
public CorrelatorToken getCorrelatorTokenDetails(){
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("--> TmInteractionImpl.getCorrelatorTokenDetails()...");
CorrelatorToken tkn = new CorrelatorToken();
if (logger.isLoggable(ApiProperties.TRACE_LEVEL_ENTRY_EXIT))
logger.finer("<-- TmInteractionImpl.getCorrelatorTokenDetails()...");
return tkn;
}
/**
* isValidRacfPassword validates the name supplied as an input parameter against the
* standard host naming convention, 1 to 8 alphanumeric characters plus '@', '#', and '$'
* and other newly supported special characters.
* (with no spaces).
*
* #param String aName
*/
private static boolean isValidRacfPassword(String aName)
{
final String validSpecialCharacters = "@#$.<+|&!*-%_>?:=";
if ((aName != null) && (aName.length() > 0))
{
int i = 0;
for ( ; i < aName.length(); i++)
{
char c = aName.charAt(i);
if((!Character.isLetterOrDigit(c)) && (validSpecialCharacters.indexOf(c) == -1))
i = 9;
}
if(i < 9)
return true;
}
return false;
}
}