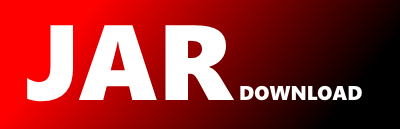
com.ibm.jbatch.container.cdi.BatchProducerBean Maven / Gradle / Ivy
package com.ibm.jbatch.container.cdi;
import java.lang.annotation.Annotation;
import java.lang.reflect.Type;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import java.util.logging.Logger;
import javax.batch.api.BatchProperty;
import javax.batch.runtime.context.JobContext;
import javax.batch.runtime.context.StepContext;
import javax.enterprise.context.Dependent;
import javax.enterprise.context.spi.CreationalContext;
import javax.enterprise.inject.Any;
import javax.enterprise.inject.Default;
import javax.enterprise.inject.Produces;
import javax.enterprise.inject.spi.Bean;
import javax.enterprise.inject.spi.InjectionPoint;
import javax.enterprise.util.AnnotationLiteral;
import com.ibm.jbatch.container.artifact.proxy.ProxyFactory;
import com.ibm.jbatch.container.util.DependencyInjectionUtility;
import com.ibm.jbatch.jsl.model.Property;
public class BatchProducerBean implements Bean {
private final static String sourceClass = BatchProducerBean.class.getName();
private final static Logger logger = Logger.getLogger(sourceClass);
@Produces
@Dependent
@BatchProperty
public String produceProperty(InjectionPoint injectionPoint) {
//Seems like this is a CDI bug where null injection points are getting passed in.
//We should be able to ignore these as a workaround.
if (injectionPoint != null) {
BatchProperty batchPropAnnotation = injectionPoint.getAnnotated().getAnnotation(BatchProperty.class);
// If a name is not supplied the batch property name defaults to
// the field name
String batchPropName = null;
if (batchPropAnnotation.name().equals("")) {
batchPropName = injectionPoint.getMember().getName();
} else {
batchPropName = batchPropAnnotation.name();
}
List propList = ProxyFactory.getInjectionReferences().getProps();
String propValue = DependencyInjectionUtility.getPropertyValue(propList, batchPropName);
return propValue;
}
return null;
}
@Produces
@Dependent
public JobContext getJobContext() {
return ProxyFactory.getInjectionReferences().getJobContext();
}
@Produces
@Dependent
public StepContext getStepContext() {
return ProxyFactory.getInjectionReferences().getStepContext();
}
@Override
public Class> getBeanClass() {
return BatchProducerBean.class;
}
@Override
public Set getInjectionPoints() {
return Collections.emptySet();
}
@Override
public String getName() {
return "batchProducerBean";
}
@Override
public Set getQualifiers() {
Set qualifiers = new HashSet();
qualifiers.add(new AnnotationLiteral() {
@Override
public Class extends Annotation> annotationType() {
return Default.class;
}
});
qualifiers.add(new AnnotationLiteral() {
@Override
public Class extends Annotation> annotationType() {
return Any.class;
}
});
return qualifiers;
}
@Override
public Class extends Annotation> getScope() {
return Dependent.class;
}
@Override
public Set> getStereotypes() {
return Collections.emptySet();
}
@Override
public Set getTypes() {
Set types = new HashSet();
types.add(BatchProducerBean.class);
types.add(Object.class);
return types;
}
@Override
public boolean isAlternative() {
return false;
}
@Override
public boolean isNullable() {
return false;
}
@Override
public BatchProducerBean create(CreationalContext ctx) {
return new BatchProducerBean();
}
@Override
public void destroy(BatchProducerBean instance, CreationalContext ctx) {
ctx.release();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy