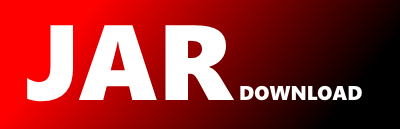
com.mdfromhtml.core.MDfromHTMLDuration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of MDfromHTMLBase Show documentation
Show all versions of MDfromHTMLBase Show documentation
Common utilities and classes used throughout the MDfromHTML project
The newest version!
/**
* (c) Copyright 2020 IBM Corporation
* 1 New Orchard Road,
* Armonk, New York, 10504-1722
* United States
* +1 914 499 1900
* support: Nathaniel Mills [email protected]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package com.mdfromhtml.core;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
/**
* Utility class for durations in time.
*
* @author wnm3
*/
public class MDfromHTMLDuration
implements Serializable, Comparable {
// --------
// statics
// --------
static final public String m_strClassName = MDfromHTMLUtils
.getNameFromClass(MDfromHTMLDuration.class);
private static final long serialVersionUID = 2151322379607630650L;
/**
* An undefined version of this object. An undefinedMDfromHTMLDuration has
* an undefined name.
*
* @see MDfromHTMLUtils#isUndefined(String)
*/
static public MDfromHTMLDuration UNDEFINED_MDfromHTMLDuration = new MDfromHTMLDuration();
/**
* Calculate the number of elapsed days between the start and end of this
* duration. If no end date has been set, then the duration is 0L. If no
* start date has been set, then the duration is the number of days since
* the epoch (midnight 1/1/70 GMT) until the end date. If the start date is
* greater than the end date, then 0L is returned.
*
* @param dateStart
* the start date of the duration
* @param dateEnd
* the end date of the duration
* @return the number of elapsed days between the start and end of this
* duration.
*/
static public long elapsedDays(Date dateStart, Date dateEnd) {
long lElapsedTime = elapsedHours(dateStart, dateEnd);
lElapsedTime /= 24L;
return lElapsedTime;
}
/**
* Calculate the number of elapsed hours between the start and end of this
* duration. If no end date has been set, then the duration is 0L. If no
* start date has been set, then the duration is the number of hours since
* the epoch (midnight 1/1/70 GMT) until the end date. If the start date is
* greater than the end date, then 0L is returned.
*
* @param dateStart
* the start date of the duration
* @param dateEnd
* the end date of the duration
* @return the number of elapsed hours between the start and end of this
* duration.
*/
static public long elapsedHours(Date dateStart, Date dateEnd) {
long lElapsedTime = elapsedMinutes(dateStart, dateEnd);
lElapsedTime /= 60L;
return lElapsedTime;
}
/**
* Calculate the number of elapsed minutes between the start and end of this
* duration. If no end date has been set, then the duration is 0L. If no
* start date has been set, then the duration is the number of minutes since
* the epoch (midnight 1/1/70 GMT) until the end date. If the start date is
* greater than the end date, then 0L is returned.
*
* @param dateStart
* the start date of the duration
* @param dateEnd
* the end date of the duration
* @return the number of elapsed minutes between the start and end of this
* duration.
*/
static public long elapsedMinutes(Date dateStart, Date dateEnd) {
long lElapsedTime = elapsedSeconds(dateStart, dateEnd);
lElapsedTime /= 60L;
return lElapsedTime;
}
/**
* Calculate the number of elapsed seconds between the start and end of this
* duration. If no end date has been set, then the duration is 0L. If no
* start date has been set, then the duration is the number of seconds since
* the epoch (midnight 1/1/70 GMT) until the end date. If the start date is
* greater than the end date, then 0L is returned.
*
* @param dateStart
* the start date of the duration
* @param dateEnd
* the end date of the duration
* @return the number of elapsed seconds between the start and end of this
* duration.
*/
static public long elapsedSeconds(Date dateStart, Date dateEnd) {
long lElapsedTime = elapsedTime(dateStart, dateEnd);
lElapsedTime /= 1000L;
return lElapsedTime;
}
/**
* Calculate the number of elapsed milliseconds between the start and end of
* this duration. If no end date has been set, then the duration is 0L. If
* no start date has been set, then the duration is the number of
* milliseconds since the epoch (midnight 1/1/70 GMT) until the end date. If
* the start date is greater than the end date, then the negative of the
* elapsed time from the end date to the start date is returned.
*
* @param dateStart
* the start date of the duration
* @param dateEnd
* the end date of the duration
* @return the number of elapsed milliseconds between the start and end of
* this duration.
*/
static public long elapsedTime(Date dateStart, Date dateEnd) {
dateStart = MDfromHTMLUtils.undefinedForNull(dateStart);
dateEnd = MDfromHTMLUtils.undefinedForNull(dateEnd);
if (MDfromHTMLDate.isUndefined(dateEnd) == true) {
return 0L;
}
if (MDfromHTMLDate.isUndefined(dateStart) == true) {
return dateEnd.getTime();
}
if (dateStart.compareTo(dateEnd) > 0) {
return 0L - elapsedTime(dateEnd, dateStart);
}
return dateEnd.getTime() - dateStart.getTime();
}
static public String formattedElapsedTime(Date dateStart, Date dateEnd) {
long lElapsedMS = elapsedTime(dateStart, dateEnd);
return formattedElapsedTime(lElapsedMS);
}
static public String formattedElapsedTime(double dElapsedSeconds) {
long lElapsedMS = (long) (dElapsedSeconds * 1000L);
return formattedElapsedTime(lElapsedMS);
}
static public String formattedElapsedTime(long lElapsedMS) {
String strSign = MDfromHTMLConstants.EMPTY_String;
if (lElapsedMS < 0L) {
strSign = "-";
lElapsedMS = -lElapsedMS;
}
long lElapsedMilli = lElapsedMS % 1000;
lElapsedMS /= 1000;
StringBuffer sb = new StringBuffer();
long lDays = lElapsedMS / 86400;
lElapsedMS = lElapsedMS % 86400;
long lHours = lElapsedMS / 3600;
lElapsedMS = lElapsedMS % 3600;
long lMinutes = lElapsedMS / 60;
lElapsedMS = lElapsedMS % 60;
if (MDfromHTMLUtils.isEmpty(strSign) == false) {
sb.append("-");
}
sb.append(lDays);
sb.append("-");
sb.append(MDfromHTMLUtils.padLeftZero((int) lHours, 2));
sb.append(":");
sb.append(MDfromHTMLUtils.padLeftZero((int) lMinutes, 2));
sb.append(":");
sb.append(MDfromHTMLUtils.padLeftZero((int) lElapsedMS, 2));
sb.append(".");
sb.append(MDfromHTMLUtils.padLeftZero((int) lElapsedMilli, 3));
return sb.toString();
}
public static void main(String[] args) {
try {
String strBirthday = MDfromHTMLUtils.prompt(
"Enter your birthday in the form: YYYY/MM/DD-hh:mm:ss.SSS(ZZZ):");
if (strBirthday == null || strBirthday.length() == 0) {
strBirthday = "1957/08/04-07:15:00.000(EDT)";
}
MDfromHTMLDuration dur = new MDfromHTMLDuration("Birthday",
new MDfromHTMLDate(strBirthday), new MDfromHTMLDate());
String strTimeZone = MDfromHTMLUtils.prompt(
"Enter the output timezone (e.g., -0500 for EST or +0000 for GMT):");
System.out.println(dur.toString(strTimeZone));
MDfromHTMLDuration negdur = new MDfromHTMLDuration("Reverse",
dur.getEndDate(), dur.getStartDate());
System.out.println(negdur.toString(strTimeZone));
} catch (Exception aer) {
aer.printStackTrace();
}
System.out.println("Goodbye");
}
/**
* Create anMDfromHTMLDuration from the passed list string.
*
* @param listString
* the list of String fields needed to create
* anMDfromHTMLDuration (name, start date, end date);
* @return a newly createdMDfromHTMLDuration object filled with the content
* of the supplied listString. If the listString is null or empty,
* or does not contain at least the name field, an
* undefinedMDfromHTMLDuration is returned.
* @throws Exception
* if listString is null or empty, or if the name in the list is
* null, empty or undefined.
* @see #isUndefined()
*/
static public MDfromHTMLDuration newInstanceFromListString(
String listString) throws Exception {
if (listString == null || listString.length() == 0) {
throw new Exception("String listString is null or empty.");
}
MDfromHTMLDuration duration = MDfromHTMLDuration.UNDEFINED_MDfromHTMLDuration;
ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy