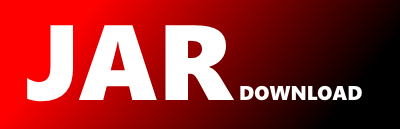
com.mdfromhtml.core.MDfromHTMLUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of MDfromHTMLBase Show documentation
Show all versions of MDfromHTMLBase Show documentation
Common utilities and classes used throughout the MDfromHTML project
The newest version!
/**
* (c) Copyright 2020 IBM Corporation
* 1 New Orchard Road,
* Armonk, New York, 10504-1722
* United States
* +1 914 499 1900
* support: Nathaniel Mills [email protected]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package com.mdfromhtml.core;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.FileWriter;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.InvalidObjectException;
import java.io.OutputStreamWriter;
import java.io.PrintWriter;
import java.io.Serializable;
import java.io.StringWriter;
import java.math.BigInteger;
import java.net.URI;
import java.nio.charset.Charset;
import java.nio.charset.StandardCharsets;
import java.nio.file.DirectoryIteratorException;
import java.nio.file.DirectoryStream;
import java.nio.file.Files;
import java.nio.file.Path;
import java.security.InvalidParameterException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Date;
import java.util.HashSet;
import java.util.List;
import java.util.Properties;
import javax.management.modelmbean.InvalidTargetObjectTypeException;
import com.api.json.JSON;
import com.api.json.JSONArray;
import com.api.json.JSONArtifact;
import com.api.json.JSONObject;
/**
* Utility methods commonly used throughout the MDfromHTML Project
*/
public class MDfromHTMLUtils implements Serializable {
static public final int iDayMilliseconds = 86400000;
static public final int iHourMilliseconds = 3600000;
static public final int iMinuteMilliseconds = 60000;
static private final long serialVersionUID = 8109772978213632637L;
static final public Charset UTF8_CHARSET = Charset.forName("UTF-8");
/**
* Utility method to create a list of the content of an ArrayList by
* converting each object to its toString() representation and appending it
* within a list using the supplied delimiter. If a String is encountered in
* the ArrayList, it will be quoted (e.g., surrounded by double quote
* marks). The list itself is enclosed by an opening ('{') and closing ('}')
* brace.
*
* Note: there is no escaping of the characters in a String object, so if it
* contains a delimiter or a closing brace, you may get unexpected results.
*
* @param list
* the array list of objects to be converted to a list string.
* @return the list comprising an opening brace, then each object in the
* arraylist converted to a string, followed by the closing brace,
* with the caveat that string objects encountered in the arraylist
* are enclosed in a pair of double quotes.
* @see #listStringToArrayList
*/
static public String arrayListToListString(ArrayList> list) {
// WNM3: add support for Int[], Double[], Float[], Long[], String[]
// WNM3: escape the Strings?
if (list == null || list.size() == 0) {
return "{}"; // empty string
}
String strDelimiter = ",";
StringBuffer sb = new StringBuffer();
sb.append("{");
Object item = null;
for (int i = 0; i < list.size(); i++) {
if (i != 0) {
sb.append(strDelimiter);
}
item = list.get(i);
if (item instanceof Integer) {
sb.append(item.toString());
} else if (item instanceof Long) {
sb.append(item.toString());
} else if (item instanceof Double) {
sb.append(item.toString());
} else if (item instanceof Float) {
sb.append(item.toString());
} else if (item instanceof String) {
sb.append("\"");
sb.append(item.toString());
sb.append("\"");
} else {
// WNM3: not sure what to do here... hex of serialized?
sb.append("\"");
sb.append(item.toString());
sb.append("\"");
}
}
sb.append("}");
return sb.toString();
}
/**
* Converts a byte into an array of char's containing the hexadecimal
* digits. For example, 0x2f would return char[] {'2','F'}
*
* @param bIn
* byte to be converted to hexadecimal digits
* @return array of chars containing the hexadecimal digits for the value of
* the input byte.
*/
static public char[] byteToHexChars(byte bIn) {
char[] cOut = new char[2];
int iMasker = bIn & 0x000000ff;
int iMaskerHigh = iMasker & 0x000000f0;
iMaskerHigh = iMaskerHigh >> 4;
int iMaskerLow = iMasker & 0x0000000f;
if (iMaskerHigh > 9) {
iMaskerHigh = iMaskerHigh + 'A' - 10;
} else {
iMaskerHigh = iMaskerHigh + '0';
}
if (iMaskerLow > 9) {
iMaskerLow = iMaskerLow + 'A' - 10;
} else {
iMaskerLow = iMaskerLow + '0';
}
cOut[0] = (char) iMaskerHigh;
cOut[1] = (char) iMaskerLow;
return cOut;
}
/**
* Set up filter if the supplied word contains an @ like in an email
* address, or starts or ends with a number, or contains http
*
* @param word
* value to be tested
* @return true if the word should be filtered
*/
static public boolean checkWordFilter(String word) {
if (word == null) {
return true;
}
word = word.trim();
if (word.length() == 0) {
return true;
}
// check for email pattern
if (word.contains("@")) {
return true;
}
// check for http pattern
if (word.toLowerCase().contains("http")) {
return true;
}
// check for non-words that begin with a number
char wordChar = word.charAt(0);
if (0x0030 <= wordChar && wordChar <= 0x0039) {
return true;
}
// check for non-words that end with a number
wordChar = word.charAt(word.length() - 1);
if (0x0030 <= wordChar && wordChar <= 0x0039) {
return true;
}
return false;
}
/**
* Removes HTML Tags from the supplied text, replacing them with a space
* (0x20)
*
* @param text
* the string to be cleansed
* @return the cleansed string
*/
static public String cleanseHTMLTagsFromText(String text) {
if (text == null || text.trim().length() == 0) {
return "";
}
text = text.toLowerCase();
// remove newlines
text = text.replaceAll("\n", " ");
text = text.replaceAll("\r", " ");
// remove html tags
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\
", " ");
text = text.replaceAll("\\
", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\
", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\ ", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\ ", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\ ", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\
", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\
", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\
", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\ ", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\ ", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\ ", " ");
text = text.replaceAll("\\", " ");
text = text.replaceAll("\\ ", " ");
text = text.replaceAll("\\", " ");
text = removeTag(text, "= 0) {
url = url.substring(0, index);
index = url.indexOf("\r");
}
index = url.indexOf("\n");
while (index >= 0) {
url = url.substring(0, index);
index = url.indexOf("\n");
}
index = url.indexOf("\u00A0");
if (index >= 0) {
url = url.substring(0, index);
}
// strip any trailing period, comma, double quote
while (url.endsWith(".") || url.endsWith(",") || url.endsWith("\"")
|| url.endsWith("!") || url.endsWith("'") || url.endsWith("?")
|| url.endsWith(":") || url.endsWith("]") || url.endsWith(")")
|| url.endsWith("`") || url.endsWith("\\") || url.endsWith("/")
|| url.endsWith("\r") || url.endsWith("\n") || url.endsWith("\t")
|| url.endsWith("\u00A0") || url.endsWith("\u2028")
|| url.endsWith("\u2029") || url.endsWith("\u2019")
|| url.endsWith("\u201A")) {
url = url.substring(0, url.length() - 1);
}
return url;
}
/**
* Strip off non-word characters from the beginning and end of the supplied
* word.
*
* @param word
* the word to be cleansed
* @return array of cleansed word parts: [0] prefix removed from word, [1]
* cleansed word, [2] suffix removed from word
*/
static public String[] cleanWord(String word) {
String[] result = new String[3];
result[0] = "";
result[1] = "";
result[2] = "";
if (word == null) {
return result;
}
word = trimSpaces(word);
if (word.length() == 0) {
result[1] = word;
return result;
}
// clean front
int index = 0;
int len = word.length();
char wordchar = word.charAt(index);
StringBuffer sb = new StringBuffer();
// numbers, letters, some quotes, but not @ (to block emails)
while (wordchar < 0x0030
|| (wordchar > 0x0039 && wordchar < 0x0061 && wordchar != 0x0040)
|| (wordchar > 0x007a && wordchar <= 0x007f) || wordchar == '\u2003'
|| wordchar == '\u2013' || wordchar == '\u2018'
|| wordchar == '\u2019' || wordchar == '\u201C'
|| wordchar == '\u201D' || wordchar == '\u2022'
|| wordchar == '\u2026' || wordchar == '\u2028'
|| wordchar == '\u202A' || wordchar == '\u202C'
|| wordchar == '\u202F') {
sb.append(wordchar);
index++;
if (index == len) {
break;
}
wordchar = word.charAt(index);
}
result[0] = sb.toString();
if (index == len) {
return result;
}
word = word.substring(index);
len = word.length();
index = len - 1;
sb.setLength(0); // clear the accumulator
wordchar = word.charAt(index);
while (wordchar < 0x0030
|| (wordchar > 0x0039 && wordchar < 0x0061 && wordchar != 0x0040)
|| (wordchar > 0x007a && wordchar <= 0x007f) || wordchar == '\u2003'
|| wordchar == '\u2013' || wordchar == '\u2018'
|| wordchar == '\u2019' || wordchar == '\u201C'
|| wordchar == '\u201D' || wordchar == '\u2022'
|| wordchar == '\u2026' || wordchar == '\u2028'
|| wordchar == '\u202A' || wordchar == '\u202C'
|| wordchar == '\u202F') {
sb.append(wordchar);
index--;
if (index == 0) {
break;
}
wordchar = word.charAt(index);
}
result[2] = sb.reverse().toString();
word = word.substring(0, index + 1);
result[1] = word;
return result;
}
/**
* Close a buffered reader opened using {@link #openTextFile(String)}
*
* @param br
*/
static public void closeTextFile(BufferedReader br) {
if (br != null) {
try {
br.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
/**
* Close a buffered writer flushing its content first. Nothing happens if a
* null is passed.
*
* @param bw
* the buffered writer to be flushed and closed.
*/
static public void closeTextFile(BufferedWriter bw) {
if (bw != null) {
try {
bw.flush();
try {
bw.close();
} catch (IOException e) {
e.printStackTrace();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
/**
* Convert a number of milliseconds to a formatted String containing a sign,
* followed by the hours and minutes as in "-0500" or "+0100" which are used
* for TimeZones.
*
* @param iMillisecs
* the number of milliseconds from Greenwich Mean Time.
* @return a string of the form +/-hhmm as in "-0500" or "+0100"
*/
static public String convertMillisecondsToTimeZone(int iMillisecs) {
StringBuffer sb = new StringBuffer();
if (iMillisecs < 0) {
sb.append("-");
iMillisecs *= -1;
} else {
sb.append("+");
}
int iHours = iMillisecs / iHourMilliseconds;
if (iHours < 10) {
sb.append("0");
}
sb.append(iHours);
iMillisecs -= iHours * iHourMilliseconds;
int iMinutes = iMillisecs / iMinuteMilliseconds;
if (iMinutes < 10) {
sb.append("0");
}
sb.append(iMinutes);
return sb.toString();
}
/**
* Converts a timezone of the format +/-hhmm to milliseconds
*
* @param strTimeZone
* timezone offset from Greenwich Mean Time (GMT) for example
* "-0500" is Eastern Standard Time, "-0400" is Eastern Daylight
* Time, "+0000" is Greenwich Mean Time, and "+0100" is the
* offset for Europe/Paris.
*
* @return milliseconds from Greenwich Mean Time
*/
static public int convertTimeZoneToMilliseconds(String strTimeZone) {
int iMillisecs = 0;
if (strTimeZone == null || strTimeZone.length() != 5) {
return iMillisecs;
}
// convert timezone (+/-hhmm)
String strSign = strTimeZone.substring(0, 1);
String strHours = strTimeZone.substring(1, 3);
String strMinutes = strTimeZone.substring(3, 5);
try {
int iHours = new Integer(strHours).intValue();
int iMinutes = new Integer(strMinutes).intValue();
iMillisecs = iMinutes * iMinuteMilliseconds;
iMillisecs = iMillisecs + (iHours * iHourMilliseconds);
if (strSign.startsWith("-") == true) {
iMillisecs *= -1;
}
} catch (NumberFormatException nfe) {
iMillisecs = 0;
}
return iMillisecs;
}
/**
* Captures an exception's stack trace as a string for inclusion in JSON
* objects
*
* @param throwableException
* exception whose stack is to be captured
* @return String representation containing the exception's stack trace
*/
static public String exceptionTraceToString(Throwable throwableException) {
StringWriter sw = new StringWriter();
PrintWriter pw = new PrintWriter(sw);
throwableException.printStackTrace(pw);
String sStackTrace = sw.toString();
return sStackTrace;
}
/**
* Transform the UTF-8 encoded byte array into a String
*
* @param bytes
* @return string built from the supplied UTF-8 bytes
*/
static public String fromUTF8Bytes(byte[] bytes) {
return new String(bytes, UTF8_CHARSET);
}
/**
* @return the key needed for encrypting content
*/
static public char[] getKey() {
String pwKey = System.getenv(MDfromHTMLMasker.ENV_MASKER_KEY);
if (pwKey == null || pwKey.trim().length() == 0) {
pwKey = System.getProperty(MDfromHTMLMasker.ENV_MASKER_KEY);
if (pwKey == null || pwKey.trim().length() == 0) {
return MDfromHTMLConstants.MDfromHTML_DEFAULT_PROPERTY_FILE_KEY;
}
}
return pwKey.toCharArray();
}
/**
*
* @return the fully qualified path to the
* {@link MDfromHTMLConstants#ENV_MDfromHTML_HOME} directory as
* defined by the Environment variable first, or System property
* second as defined by
* {@link MDfromHTMLConstants#ENV_MDfromHTML_HOME}. If that is not
* defined, then the user.home directory will be used. There is no
* trailing {@link File#separator} appended to the path.
* @throws Exception
*/
public static String getMDfromHTMLHomeDirectory() throws Exception {
File retFile = null;
String strPath = System.getenv(MDfromHTMLConstants.ENV_MDfromHTML_HOME);
if (strPath == null) {
strPath = System
.getProperty(MDfromHTMLConstants.ENV_MDfromHTML_HOME);
}
if (strPath == null) {
strPath = System.getProperty("user.home");
if (strPath == null) {
throw new Exception(
"Can not find Environment variable nor System property for "
+ MDfromHTMLConstants.ENV_MDfromHTML_HOME
+ " nor the user.home System property so can not return the MDfromHTML Home Directory.");
}
while (strPath.length() > 2
&& strPath.endsWith(File.separator) == true) {
strPath = strPath.substring(0, strPath.length() - 1);
}
strPath += File.separator + "MDfromHTML";
} else {
while (strPath.length() > 2
&& strPath.endsWith(File.separator) == true) {
strPath = strPath.substring(0, strPath.length() - 1);
}
}
try {
retFile = new File(strPath);
if (retFile.exists() == false || retFile.isFile() == true) {
// try looking in the current directory
strPath = System.getProperty("user.dir");
if (strPath == null) {
throw new Exception(
"Can not find Environment variable nor System property for "
+ MDfromHTMLConstants.ENV_MDfromHTML_HOME
+ " nor the user.home System property so can not return the MDfromHTML Home Directory.");
}
while (strPath.length() > 2
&& strPath.endsWith(File.separator) == true) {
strPath = strPath.substring(0, strPath.length() - 1);
}
strPath += File.separator
+ MDfromHTMLConstants.MDfromHTML_HOME_DIRECTORY_NAME;
retFile = new File(strPath);
if (retFile.exists() == false || retFile.isFile() == true) {
throw new Exception("Can not find directory " + strPath);
} // else fall through with this file
} // else fall through with this file
return retFile.getCanonicalPath();
} catch (Exception fnfe) {
throw new Exception("Can not find directory " + strPath);
}
}
/**
* @return the properties file used to define MDfromHTML interprocess
* communications
* @see #loadMDfromHTMLProperties(String)
* @see MDfromHTMLConstants#MDfromHTML_IPC_PropertiesFileName
*/
static public Properties getMDfromHTMLIPCProps() throws Exception {
Properties ipcProps = MDfromHTMLUtils.loadMDfromHTMLProperties(
MDfromHTMLConstants.MDfromHTML_IPC_PropertiesFileName);
return ipcProps;
}
/**
* @return the properties file used to identify MDfromHTML general control
* parameters
* @throws Exception
*/
static public Properties getMDfromHTMLServicesProps() throws Exception {
return MDfromHTMLUtils.loadMDfromHTMLProperties(
MDfromHTMLConstants.MDfromHTML_SVCS_PropertiesFileName);
}
/**
*
* @return URI for MDfromHTMLwebServices
*/
static public String getMDfromHTMLWebServicesURI() {
StringBuffer sb = new StringBuffer();
sb.append(MDfromHTMLPropertyManager.getProtocol());
sb.append("://");
sb.append(MDfromHTMLPropertyManager.getHostName());
sb.append(":");
sb.append(MDfromHTMLPropertyManager.getPortNumber());
sb.append("/");
sb.append(MDfromHTMLPropertyManager.getServletName());
sb.append("/");
// sb.append(MDfromHTMLPropertyManager.getURLPattern());
// sb.append("/");
sb.append(MDfromHTMLPropertyManager.getVersion());
sb.append("/");
return sb.toString();
}
/**
* Transform a fully qualified Class' name into just the name of the class
* without the leading package. For example,
* "com.mdfromhtml.core.MDfromHTMLDate" would return just "MDfromHTMLDate"
*
* @param inClass
* @return name of the class without leading qualification
*/
static public String getNameFromClass(Class> inClass) {
return inClass.getName().lastIndexOf(".") == -1 ? inClass.getName()
: inClass.getName()
.substring(inClass.getName().lastIndexOf(".") + 1);
}
/**
* @return a 40 byte String random number based on invoking the
* com.ibm.crypto.fips.provider.SecureRandom class.
*/
static public synchronized String getUniqueID() {
byte[] byteID = new byte[20];
if (MDfromHTMLConstants.SEED_SECURE_RANDOM != null) {
MDfromHTMLConstants.SEED_SECURE_RANDOM.nextBytes(byteID);
return hexEncode(byteID);
}
// otherwise, use a less sophisticated generator.
Date date = new Date();
StringBuffer sb = new StringBuffer();
sb.append("X"); // distinguish from s_sr generated.
sb.append(Long.toHexString(date.getTime()));
sb.append(Long.toHexString(MDfromHTMLConstants.SEED_RANDOM.nextLong()));
return sb.toString();
}
/**
* Transform the string of hexadecimal digits into a byte array.
*
* @param strHex
* a String containing pairs of hexadecimal digits
* @return a byte array created by transforming pairs of hexadecimal digits
* into a byte. For example "7F41" would become byte [] { 0x7f,
* 0x41}
* @throws InvalidParameterException
* thrown if the input string is null or empty, or if it does
* not contain an even number of hexadecimal digits, or if it
* contains something other than a hexadecimal digit.
*/
static public byte[] hexDecode(String strHex)
throws InvalidParameterException {
if (strHex == null || strHex.length() == 0) {
throw new InvalidParameterException(
"Null or empty string passed. Must pass string containing pairs of hexadecimal digits.");
}
int iLength = strHex.length();
if (iLength % 2 > 0) {
throw new InvalidParameterException(
"An odd number of bytes was passed in the input string. Must be an even number.");
}
byte[] inBytes = strHex.toUpperCase()
.getBytes(MDfromHTMLConstants.UTF8_CHARSET);
byte[] baRC = new byte[iLength / 2];
int iHighOffset = -1;
int iLowOffset = -1;
for (int i = 0; i < iLength; i += 2) {
iHighOffset = MDfromHTMLConstants.HEX_CHARS
.indexOf((int) inBytes[i]);
if (iHighOffset < 0) {
throw new InvalidParameterException(
"Input string contains non-hexadecimal digit at index " + i
+ ". Must be 0-9 or A-F");
}
iLowOffset = MDfromHTMLConstants.HEX_CHARS
.indexOf((int) inBytes[i + 1]);
if (iLowOffset < 0) {
throw new InvalidParameterException(
"Input string contains non-hexadecimal digit at index " + i
+ ". Must be 0-9 or A-F");
}
baRC[i / 2] = (byte) ((iHighOffset * 16) + iLowOffset);
}
return baRC;
}
/**
* Convert the byte array into a String of hexadecimal digits. For example,
* the bytes[] {0x31,0x0a} would become "310A".
*
* @param bArray
* the array of bytes to be converted.
* @return a String of hexadecimal digits formed by the hexadecimal digit
* for each nibble of the byte.
*/
static public String hexEncode(byte[] bArray) {
StringBuffer sb = new StringBuffer();
// check bad input
if (bArray == null || bArray.length == 0) {
return sb.toString();
}
// else do real work
char[] cHexPair = new char[2];
int iByteCount = 0;
int iArrayLength = bArray.length;
while (iByteCount < iArrayLength) {
cHexPair = byteToHexChars(bArray[iByteCount]);
sb.append(new String(cHexPair));
iByteCount++;
} // end while
return sb.toString();
}
/**
* Determine if the String is empty (equals "").
*
* @param strInput
* the string to be evaluated.
* @return true if the strInput compares to
* {@link MDfromHTMLConstants#EMPTY_String} (""). Returns false if
* strInput is null or not empty.
*/
static public boolean isEmpty(String strInput) {
if (strInput == null) {
return false;
}
return strInput.compareTo(MDfromHTMLConstants.EMPTY_String) == 0;
}
/**
* Determine if the passed BigInteger matches the
* {@link MDfromHTMLConstants#UNDEFINED_BigInteger}.
*
* @param testValue
* value to compare against
* {@link MDfromHTMLConstants#UNDEFINED_BigInteger}.
* @return true if the values are equal, false if they are not. Note, if
* passed value is null, true is returned.
*/
static public boolean isUndefined(BigInteger testValue) {
if (testValue == null) {
return true;
}
return testValue.equals(MDfromHTMLConstants.UNDEFINED_BigInteger);
}
/**
* Determine if the passed Boolean matches the
* {@link MDfromHTMLConstants#UNDEFINED_Boolean}.
*
* @param testValue
* value to compare against
* {@link MDfromHTMLConstants#UNDEFINED_Boolean}.
* @return true if the values are equal, false if they are not.
*/
static public boolean isUndefined(Boolean testValue) {
return testValue == MDfromHTMLConstants.UNDEFINED_Boolean;
}
/**
* Determine if the passed byte matches the
* {@link MDfromHTMLConstants#UNDEFINED_byte}.
*
* @param testValue
* value to compare against
* {@link MDfromHTMLConstants#UNDEFINED_byte}.
* @return true if the values are equal, false if they are not.
*/
static public boolean isUndefined(byte testValue) {
return testValue == MDfromHTMLConstants.UNDEFINED_byte;
}
/**
* Determine if the passed Byte matches the
* {@link MDfromHTMLConstants#UNDEFINED_Byte}.
*
* @param testValue
* value to compare against
* {@link MDfromHTMLConstants#UNDEFINED_Byte}.
* @return true if the values are equal, false if they are not. Note, if
* passed value is null, true is returned.
*/
static public boolean isUndefined(Byte testValue) {
if (testValue == null) {
return true;
}
return testValue.equals(MDfromHTMLConstants.UNDEFINED_Byte);
}
/**
* Determine if the passed char matches the
* {@link MDfromHTMLConstants#UNDEFINED_char}.
*
* @param testValue
* value to compare against
* {@link MDfromHTMLConstants#UNDEFINED_char}.
* @return true if the values are equal, false if they are not.
*/
static public boolean isUndefined(char testValue) {
return testValue == MDfromHTMLConstants.UNDEFINED_char;
}
/**
* Determine if the passed Character matches the
* {@link MDfromHTMLConstants#UNDEFINED_Character}.
*
* @param testValue
* value to compare against
* {@link MDfromHTMLConstants#UNDEFINED_Character}.
* @return true if the values are equal, false if they are not. Note, if
* passed value is null, true is returned.
*/
static public boolean isUndefined(Character testValue) {
if (testValue == null) {
return true;
}
return testValue.equals(MDfromHTMLConstants.UNDEFINED_Character);
}
/**
* Determine if the pass Class matches the
* {@link MDfromHTMLConstants#UNDEFINED_Class}.
*
* @param testValue
* @return whether (true) or not the supplied class is undefined
*/
static public boolean isUndefined(Class> testValue) {
if (testValue == null) {
return true;
}
return false;
}
/**
* Determine if the passed double matches the
* {@link MDfromHTMLConstants#UNDEFINED_double}.
*
* @param testValue
* value to compare against
* {@link MDfromHTMLConstants#UNDEFINED_double} .
* @return true if the values are equal, false if they are not.
*/
static public boolean isUndefined(double testValue) {
return new Double(testValue)
.equals(MDfromHTMLConstants.UNDEFINED_Double);
}
/**
* Determine if the passed Double matches the
* {@link MDfromHTMLConstants#UNDEFINED_Double}.
*
* @param testValue
* value to compare against
* {@link MDfromHTMLConstants#UNDEFINED_Double} .
* @return true if the values are equal, false if they are not. Note, if
* passed value is null, true is returned.
*/
static public boolean isUndefined(Double testValue) {
if (testValue == null) {
return true;
}
return testValue.equals(MDfromHTMLConstants.UNDEFINED_Double);
}
/**
* Determine if the passed float matches the
* {@link MDfromHTMLConstants#UNDEFINED_float}.
*
* @param testValue
* value to compare against
* {@link MDfromHTMLConstants#UNDEFINED_float}.
* @return true if the values are equal, false if they are not.
*/
static public boolean isUndefined(float testValue) {
return new Float(testValue).equals(MDfromHTMLConstants.UNDEFINED_Float);
}
/**
* Determine if the passed Float matches the
* {@link MDfromHTMLConstants#UNDEFINED_Float}.
*
* @param testValue
* value to compare against
* {@link MDfromHTMLConstants#UNDEFINED_Float}.
* @return true if the values are equal, false if they are not. Note, if
* passed value is null, true is returned.
*/
static public boolean isUndefined(Float testValue) {
if (testValue == null) {
return true;
}
return testValue.equals(MDfromHTMLConstants.UNDEFINED_Float);
}
/**
* Determine if the passed int matches the
* {@link MDfromHTMLConstants#UNDEFINED_int} .
*
* @param iTestValue
* value to compare against
* {@link MDfromHTMLConstants#UNDEFINED_int}.
* @return true if the values are equal, false if they are not.
*/
static public boolean isUndefined(int iTestValue) {
return iTestValue == MDfromHTMLConstants.UNDEFINED_int;
}
/**
* Determine if the passed Integer matches the
* {@link MDfromHTMLConstants#UNDEFINED_Integer}.
*
* @param testValue
* value to compare against
* {@link MDfromHTMLConstants#UNDEFINED_Integer}.
* @return true if the values are equal, false if they are not. Note, if
* passed value is null, true is returned.
*/
static public boolean isUndefined(Integer testValue) {
if (testValue == null) {
return true;
}
return testValue.equals(MDfromHTMLConstants.UNDEFINED_Integer);
}
/**
* Determine if the passed long matches the
* {@link MDfromHTMLConstants#UNDEFINED_long}.
*
* @param testValue
* value to compare against
* {@link MDfromHTMLConstants#UNDEFINED_long}.
* @return true if the values are equal, false if they are not.
*/
static public boolean isUndefined(long testValue) {
return testValue == MDfromHTMLConstants.UNDEFINED_long;
}
/**
* Determine if the passed Long matches the
* {@link MDfromHTMLConstants#UNDEFINED_Long}.
*
* @param testValue
* value to compare against
* {@link MDfromHTMLConstants#UNDEFINED_Long}.
* @return true if the values are equal, false if they are not. Note, if
* passed value is null, true is returned.
*/
static public boolean isUndefined(Long testValue) {
if (testValue == null) {
return true;
}
return testValue.equals(MDfromHTMLConstants.UNDEFINED_Long);
}
/**
* Determine if the passed Object matches any of the
* {@link MDfromHTMLConstants} for UNDEFINED_* values.
*
* @param testValue
* value to compare against the appropriate
* {@link MDfromHTMLConstants} for UNDEFINED_* value.
* @return true if the values are equal (ignoring case), or false if they
* are not. Note, if passed value is null, true is returned.
*/
static public boolean isUndefined(Object testValue) {
if (testValue == null) {
return true;
}
Class extends Object> objClass = testValue.getClass();
if (isUndefined(objClass)) {
return true;
}
if (objClass == Double.class) {
return isUndefined((Double) testValue);
} else if (objClass == Integer.class) {
return isUndefined((Integer) testValue);
} else if (objClass == String.class) {
return isUndefined((String) testValue);
} else if (objClass == Byte.class) {
return isUndefined((Byte) testValue);
} else if (objClass == Character.class) {
return isUndefined((Character) testValue);
} else if (objClass == Float.class) {
return isUndefined((Float) testValue);
} else if (objClass == Long.class) {
return isUndefined((Long) testValue);
} else if (objClass == Short.class) {
return isUndefined((Short) testValue);
} else if (objClass == BigInteger.class) {
return isUndefined((BigInteger) testValue);
} else if (objClass == Date.class) {
return MDfromHTMLDate.isUndefined((Date) testValue);
} else if (objClass == MDfromHTMLDate.class) {
return MDfromHTMLDate.isUndefined((MDfromHTMLDate) testValue);
} else if (objClass == JSONObject.class) {
try {
((JSONObject) testValue).serialize();
} catch (IOException e) {
return true;
}
}
return false;
}
/**
* Determine if the passed short matches the
* {@link MDfromHTMLConstants#UNDEFINED_short}.
*
* @param testValue
* value to compare against
* {@link MDfromHTMLConstants#UNDEFINED_short}.
* @return true if the values are equal, false if they are not.
*/
static public boolean isUndefined(short testValue) {
return testValue == MDfromHTMLConstants.UNDEFINED_short;
}
/**
* Determine if the passed Short matches the
* {@link MDfromHTMLConstants#UNDEFINED_Short}.
*
* @param testValue
* value to compare against
* {@link MDfromHTMLConstants#UNDEFINED_Short}.
* @return true if the values are equal, false if they are not. Note, if
* passed value is null, true is returned.
*/
static public boolean isUndefined(Short testValue) {
if (testValue == null) {
return true;
}
return testValue.equals(MDfromHTMLConstants.UNDEFINED_Short);
}
/**
* Determine if the passed String is null, or when trimmed, matches the
* {@link MDfromHTMLConstants#UNDEFINED_String} or is empty or is equal to
* "null" (to support ABLE rules)
*
* @param testValue
* value to compare against
* {@link MDfromHTMLConstants#UNDEFINED_String} .
* @return true if the values are equal (ignoring case), or false if they
* are not. Note, if passed value is null or an empty string, true
* is returned.
*/
static public boolean isUndefined(String testValue) {
if (testValue == null || testValue.trim().length() == 0) {
return true;
}
testValue = testValue.trim();
// WNM3: hack for ABLE support
if ("null".equals(testValue)) {
return true;
}
return testValue.equals(MDfromHTMLConstants.UNDEFINED_String);
}
/**
* Determine if the passed URI is null, or equals the
* {@link MDfromHTMLConstants#UNDEFINED_URI}
*
* @param testValue
* value to compare against
* {@link MDfromHTMLConstants#UNDEFINED_URI}.
* @return true if the values are equal (ignoring case), or false if they
* are not. Note, if passed value is null, true is returned.
*/
static public boolean isUndefined(URI testValue) {
if (testValue == null) {
return true;
}
return (MDfromHTMLConstants.UNDEFINED_URI.equals(testValue));
}
/**
* Tests whether the supplied url is valid
*
* @param url
* the URL to be tested
* @return true if the URL references http or https protocol
*/
static public boolean isValidURL(String url) {
boolean result = false;
// must be http:// at minimum, could be https://
if (url.length() < 7) {
return result;
}
String secure = url.substring(4, 5);
if (secure.equalsIgnoreCase("s")) {
if (url.length() < 8) {
return result;
}
if (url.length() >= 12 && url.substring(5, 12).equals("://http")) {
return result;
}
result = url.substring(5, 8).equals("://");
} else {
if (secure.equals(":") == false) {
return result;
}
// now check for //
if (url.length() >= 11 && url.substring(4, 11).equals("://http")) {
return result;
}
result = url.substring(5, 7).equals("//");
}
return result;
}
/**
* Construct and return a sorted list of files in a directory identified by
* the dir that have extensions matching the ext
*
* @param dir
* the path to the directory containing files to be returned in
* the list
* @param ext
* the file extension (without the leading period) used to filter
* files in the dir
* @return sorted list of files in a directory identified by the dir that
* have extensions matching the ext
* @throws IOException
* if there is difficulty accessing the files in the supplied
* dir
*/
static public List listSourceFiles(Path dir, String ext)
throws IOException {
List result = new ArrayList();
try (DirectoryStream stream = Files.newDirectoryStream(dir,
"*.{" + ext + "}")) {
for (Path entry : stream) {
result.add(entry);
}
} catch (DirectoryIteratorException ex) {
// I/O error encountered during the iteration, the cause is an
// IOException
throw ex.getCause();
}
result.sort(null);
return result;
}
/**
* Transform a list of fields contained in a String bounded with opening
* ('{') and closing ('}') braces, and delimited with one of the delimiters
* (comma, space, tab, pipe). Fields containing strings are expected to be
* enclosed in double quotes ('"').
*
* @param strList
* the list of fields enclosed in braces.
* @return an ArrayList of the fields parsed from the supplied list string.
* Note: if the passed strList is null or empty, an empty ArrayList
* is returned (e.g., its size() is 0).
* @see #arrayListToListString
*/
static public ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy