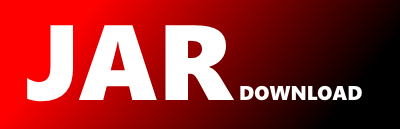
com.ibm.mfp.server.app.external.ApplicationKey Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of adapter-maven-api Show documentation
Show all versions of adapter-maven-api Show documentation
IBM MFP Adapter api for adapter as a maven project. BuildNumber is : 8.0.2024082809
The newest version!
/*
* © Copyright IBM Corp. 2016
* All Rights Reserved. US Government Users Restricted Rights - Use, duplication or disclosure restricted by GSA ADP Schedule Contract with IBM Corp.
*/
package com.ibm.mfp.server.app.external;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
* Mobile application key.
*/
public class ApplicationKey {
@JsonProperty(value = "id")
private String applicationId;
@JsonProperty(value = "clientPlatform")
private String clientPlatform;
@JsonProperty(value = "version")
@JsonInclude(JsonInclude.Include.NON_NULL)
private String version;
public static ApplicationKey parse(String keyStr) {
String[] parts = keyStr.split("\\$");
if (parts.length == 3)
return new ApplicationKey(parts[1], parts[0], parts[2]);
else if (parts.length == 2)
return new ApplicationKey(parts[1], parts[0]);
else
throw new RuntimeException("Failed to parse application key: " + keyStr);
}
// for deserialization
public ApplicationKey() {
}
//web apps do not have version
public ApplicationKey(String clientPlatform, String applicationId) {
this.applicationId = applicationId;
this.clientPlatform = clientPlatform;
}
public ApplicationKey(String clientPlatform, String applicationId, String version) {
this.applicationId = applicationId;
this.clientPlatform = clientPlatform;
this.version = version;
}
/**
* Gets the application ID.
*
* @return the application ID
*/
public String getId() {
return applicationId;
}
/**
* Gets the name of the client platform (ios, android, etc)
*
* @return the name of the client platform
*/
public String getClientPlatform() {
return clientPlatform;
}
/**
* Gets the version of the application.
*
* @return the application version
*/
public String getVersion() {
return version;
}
/**
* Gets a string represntation of the application key.
*
* @return a string represntation of the application key
*/
public String toUniqueString() {
String res = applicationId + "$" + clientPlatform;
if (version != null) res += "$" + version;
return res;
}
/**
* Reserved for internal use only.
*
* @param that the application key to update from
* @return true if this key was updated, false if it remains the same
* @throws Exception if the given key is incompatible with this key
*/
public boolean updateFrom(ApplicationKey that) throws Exception {
boolean res = false;
if (that != null) {
if (clientPlatform != null && !clientPlatform.equals(that.clientPlatform))
throw new Exception("attempt to change client platform");
if (applicationId != null && !applicationId.equals(that.applicationId))
throw new Exception("attempt to change application Id");
if (version != null && !version.equals(that.version)) {
res = true;
version = that.getVersion();
}
}
return res;
}
@Override
public boolean equals(Object obj) {
if (obj == this) return true;
if (obj == null || !(obj instanceof ApplicationKey)) return false;
ApplicationKey that = (ApplicationKey) obj;
return applicationId.equals(that.applicationId) && clientPlatform.equals(that.clientPlatform) &&
(version == null && that.version == null || version != null && version.equals(that.version));
}
}