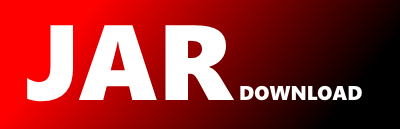
com.ibm.mfp.server.registration.external.model.AuthenticatedUser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of adapter-maven-api Show documentation
Show all versions of adapter-maven-api Show documentation
IBM MFP Adapter api for adapter as a maven project. BuildNumber is : 8.0.2024082809
The newest version!
/*
* © Copyright IBM Corp. 2016
* All Rights Reserved. US Government Users Restricted Rights - Use, duplication or disclosure restricted by GSA ADP Schedule Contract with IBM Corp.
*/
package com.ibm.mfp.server.registration.external.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
/**
* User identity information, including ID and display name, the name of the security check that authenticated the user and the authentication time.
*
* @author artem
* Date: 8/20/15
*/
public class AuthenticatedUser {
@JsonProperty
private String id;
@JsonProperty
private String displayName;
@JsonProperty
private long authenticatedAt;
@JsonProperty
private String authenticatedBy;
@JsonProperty
private Map attributes;
/**
* Reserved for internal use. Security checks should use one of the other constructors.
*/
public AuthenticatedUser() {
}
/**
* Constructs an AuthenticatedUser that has no attributes.
The time of authentication is set to the current time.
* @param id the ID of the user
* @param displayName the display name
* @param securityCheckName the name of the security check that authenticated the user
*/
public AuthenticatedUser(String id, String displayName, String securityCheckName) {
this(id, displayName, securityCheckName, new HashMap());
}
/**
* Constructs an AuthenticatedUser.
The time of authentication is set to the current time.
* @param id the ID of the user
* @param displayName the display name
* @param securityCheckName the name of the security check that authenticated the user
* @param attributes custom attributes of the user, provided as a map of attribute name and value. Can be null, which
* is the same as an empty map.
*/
public AuthenticatedUser(String id, String displayName, String securityCheckName, Map attributes) {
this.id = id;
this.displayName = displayName;
this.authenticatedBy = securityCheckName;
authenticatedAt = System.currentTimeMillis();
this.attributes = (attributes != null ? attributes : new HashMap());
}
/**
* Gets the time when the user was authenticated.
* The time is given in milliseconds since Unix epoch.
* @return the time when the user was authenticated, in milliseconds since Unix epoch
*/
public long getAuthenticatedAt() {
return authenticatedAt;
}
/**
* Gets the name of the security check that authenticated the user.
* @return the name of the security check that authenticated the user
*/
public String getAuthenticatedBy() {
return authenticatedBy;
}
/**
* Gets the user ID assigned by the security check.
* @return the user ID
*/
public String getId() {
return id;
}
/**
* Gets the display name of the user.
* @return the display name
*/
public String getDisplayName() {
return displayName;
}
/**
* Gets the custom attributes of the user, as a map of attribute name and attribute value.
* @return the custom attributes of the user, as a map of attribute name and attribute value
*/
public Map getAttributes() {
return Collections.unmodifiableMap(attributes);
}
@Override
public boolean equals(Object obj) {
if (obj == this) return true;
if (obj == null || !(obj instanceof AuthenticatedUser)) return false;
AuthenticatedUser that = (AuthenticatedUser) obj;
Object[] thisState = {id, displayName, authenticatedAt, authenticatedBy};
Object[] thatState = {that.id, that.displayName, that.authenticatedAt, that.authenticatedBy};
return Arrays.equals(thisState, thatState);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy