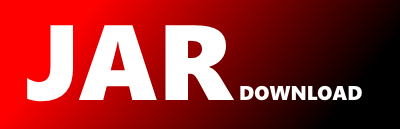
com.ibm.mfp.server.registration.external.model.DeviceData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of adapter-maven-api Show documentation
Show all versions of adapter-maven-api Show documentation
IBM MFP Adapter api for adapter as a maven project. BuildNumber is : 8.0.2024082809
The newest version!
/*
* © Copyright IBM Corp. 2016
* All Rights Reserved. US Government Users Restricted Rights - Use, duplication or disclosure restricted by GSA ADP Schedule Contract with IBM Corp.
*/
package com.ibm.mfp.server.registration.external.model;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.ibm.mfp.server.registration.external.device.management.MFPDeviceStatus;
/**
* This class manages information about the mobile device.
*
* @author artem
* Date: 7/22/15
*/
@JsonIgnoreProperties(ignoreUnknown=true)
public class DeviceData {
private String id;
private String hardware;
private String platform;
private String deviceDisplayName;
private MFPDeviceStatus status;
/**
* Reserved for internal use only.
*/
public DeviceData() {
this.status = MFPDeviceStatus.ACTIVE;
}
/**
* Reserved for internal use only.
* @param id Unique device ID
* @param hardware Device hardware
* @param platform Device platform
*/
public DeviceData(String id, String hardware, String platform) {
this.id = id;
this.hardware = hardware;
this.platform = platform;
this.status = MFPDeviceStatus.ACTIVE;
}
/**
* Reserved for internal use.
* @param that
* @return
* @throws Exception
*/
public boolean updateFrom(DeviceData that) throws Exception {
boolean res = false;
if (that != null) {
if (that.id != null && !that.id.equals(this.id)) {
res = true;
this.id = that.id;
}
if (that.hardware != null && !that.hardware.equals(this.hardware))
throw new Exception("attempt to change device hardware");
if (that.platform != null && !that.platform.equals(this.platform)) {
res = true;
this.platform = that.platform;
}
if (that.status != null && !that.status.equals(this.status)) {
res = true;
this.status = that.status;
}
if (that.deviceDisplayName != null && !that.deviceDisplayName.equals(this.deviceDisplayName)) {
res = true;
this.deviceDisplayName = that.deviceDisplayName;
}
}
return res;
}
@Override
public boolean equals(Object obj) {
if (obj == null || !(obj instanceof DeviceData)) return false;
DeviceData that = (DeviceData) obj;
if(this.deviceDisplayName != null){
if(!this.deviceDisplayName.equals(that.deviceDisplayName)){
return false;
}
}
else {
if(this.deviceDisplayName != that.deviceDisplayName){
return false;
}
}
return this.id.equals(that.id) && this.hardware.equals(that.hardware) && this.platform.equals(that.platform) && this.status.equals(that.status);
}
/**
* Returns the unique ID of the device. The ID is assigned by MobileFirst Server.
*
* @return The unqiue device ID.
*/
public String getId() {
return id;
}
/**
* Returns the hardware of the device.
*
* @return The device hardware.
*/
public String getHardware() {
return hardware;
}
/**
* Returns the name of the operating system of the device.
*
* @return The name of the device operating system.
*/
public String getPlatform() {
return platform;
}
/**
* Returns the display name of the device.
* The initial display name is null. The display name can be set by the client application, or by calling the
* {@link #setDisplayName} method of this class.
*
* @return The device display name.
*/
public String getDisplayName() {
return deviceDisplayName;
}
/**
* Sets the display name of the device.
* Note that adapters must call {@code AdapterSecurityContext.storeClientRegistrationData} to store changes in the registration data, such as the device display name.
*
* @param displayName Device display name to set.
*/
public void setDisplayName(String displayName) {
this.deviceDisplayName = displayName;
}
/**
* Sets the status of the device.
* Note that adapters must call {@link AdapterSecurityContext.storeClientRegistrationData} to store changes in the registration data, such as the device status.
* @param deviceStatus Device status to set.
*/
public void setStatus(MFPDeviceStatus deviceStatus) {
this.status = deviceStatus;
}
/**
* Returns the status of the device.
*
* @return The device status.
*/
public MFPDeviceStatus getStatus() {
return status;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy