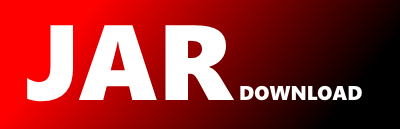
com.ibm.mfp.server.registration.external.model.PersistentAttributes Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of adapter-maven-api Show documentation
Show all versions of adapter-maven-api Show documentation
IBM MFP Adapter api for adapter as a maven project. BuildNumber is : 8.0.2024082809
The newest version!
/*
* © Copyright IBM Corp. 2016
* All Rights Reserved. US Government Users Restricted Rights - Use, duplication or disclosure restricted by GSA ADP Schedule Contract with IBM Corp.
*/
package com.ibm.mfp.server.registration.external.model;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.PropertyAccessor;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Set;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
* Container for custom attributes of a registered client.
* The class constructor is reserved for internal use only. Adapters can call ClientData.getPublicAttributes()
* or ClientData.getProtectedAttributes() to get the public or protected attributes. Adapters can then call put() or delete() to modify
* the attributes. After making changes, call AdapterSecurityContext.storeClientRegistrationData() to store the changes.
*
*
* @author artem on 12/23/15.
*/
public class PersistentAttributes {
private static final Logger logger = Logger.getLogger(PersistentAttributes.class.getName());
private static ObjectMapper objectMapper = new ObjectMapper().configure(JsonParser.Feature.ALLOW_SINGLE_QUOTES, true);;
static {
objectMapper.setVisibility(PropertyAccessor.FIELD, JsonAutoDetect.Visibility.ANY)
.setVisibility(PropertyAccessor.GETTER, JsonAutoDetect.Visibility.NONE)
.setVisibility(PropertyAccessor.SETTER, JsonAutoDetect.Visibility.NONE)
.setVisibility(PropertyAccessor.IS_GETTER, JsonAutoDetect.Visibility.NONE);
objectMapper.disableDefaultTyping();
}
@JsonProperty("attributes")
private HashMap jsonStrValues;
/**
* Reserved for internal use only.
*/
public PersistentAttributes() {
jsonStrValues = new HashMap<>();
}
/**
* Gets the value of an attribute with the given key
* @param key the attribute key
* @param valueType the type of the attribute value
* @param the type of the attribute value
* @return
*/
public ValueType get(String key, Class valueType) {
String jsonStr = jsonStrValues.get(key);
try {
return jsonStr == null ? null : objectMapper.readValue(jsonStr, valueType);
} catch (IOException e) {
logger.log(Level.SEVERE,"Error occurred while trying to get the value of key: " + key);
throw new RuntimeException(e);
}
}
/**
* Gets the value of an attribute with the given key
* @param key the attribute key
* @param the type of the attribute value
* @return the value of the attribute
*/
public ValueType get(String key) {
return (ValueType) get(key, Object.class);
}
/**
* Associates the specified value with the specified attribute key.
* @param key the attribute key
* @param value the attribute value
* @param the type of the value
*/
public void put(String key, ValueType value) {
try {
jsonStrValues.put(key, objectMapper.writeValueAsString(value));
} catch (JsonProcessingException e) {
logger.log(Level.SEVERE,"Error occurred while trying to associate the value: " + value + "with key: " +key);
throw new RuntimeException(e);
}
}
/**
* Deletes the attribute identified by the given key.
* @param key the key of the attribute
*/
public void delete(String key) {
jsonStrValues.remove(key);
}
/**
* Gets the set of attribute keys.
* @return the set of keys
*/
public Set keys() {
return new HashSet<>(jsonStrValues.keySet());
}
@Override
public boolean equals(Object obj) {
if (obj == this) return true;
if (obj == null) return false;
if (obj instanceof PersistentAttributes) {
PersistentAttributes that = (PersistentAttributes) obj;
return this.jsonStrValues.equals(that.jsonStrValues);
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy