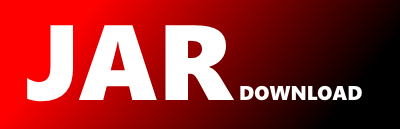
com.ibm.mfp.server.registration.external.model.RegistrationData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of adapter-maven-api Show documentation
Show all versions of adapter-maven-api Show documentation
IBM MFP Adapter api for adapter as a maven project. BuildNumber is : 8.0.2024082809
The newest version!
/*
* © Copyright IBM Corp. 2016
* All Rights Reserved. US Government Users Restricted Rights - Use, duplication or disclosure restricted by GSA ADP Schedule Contract with IBM Corp.
*/
package com.ibm.mfp.server.registration.external.model;
import com.ibm.mfp.server.app.external.ApplicationKey;
import java.util.HashMap;
import java.util.Map;
/**
* Data provided by the mobile client upon registration
*
* @author artem
* Date: 6/29/15
*/
public class RegistrationData {
private ApplicationKey application;
private DeviceData device;
private Map attributes = new HashMap<>();
/**
* Reserved for internal use only. Adapters should use ClientData.getRegistration() to get the registration data.
*/
public RegistrationData() {
}
/**
* Reserved for internal use only. Adapters should use ClientData.getRegistration() to get the registration data.
*
* @param that
*/
public RegistrationData(RegistrationData that) {
this.application = new ApplicationKey(that.application.getClientPlatform(), that.application.getId(), that.application.getVersion());
this.device = that.device == null ? null : new DeviceData(that.device.getId(), that.device.getHardware(), that.device.getPlatform());
}
/**
* Reserved for internal use only. Adapters should use ClientData.getRegistration() to get the registration data.
*
* @param application the application key
* @param clientRegistrationData device data for mobile app or browser data for web app
*/
public RegistrationData(ApplicationKey application, DeviceData clientRegistrationData, Map attributes) {
this.application = application;
this.device = clientRegistrationData;
if (attributes != null) {
this.attributes.putAll(attributes);
}
}
/**
* Reserved for internal use only.
*
* @param that
* @return
* @throws Exception
*/
public boolean updateFrom(RegistrationData that) throws Exception {
boolean reRegisterApp = this.application == null || this.application.updateFrom(that.application);
boolean reRegisterDevice = this.device == null || this.device.updateFrom(that.device);
this.attributes.clear();
if (that.attributes != null) {
this.attributes.putAll(that.attributes);
}
return reRegisterApp || reRegisterDevice;
}
/**
* Reserved for internal use only
* @return the client SDK protocol version. Possible values -1, 1, 2
*/
public int retrieveClientProtocolVersion() {
int version = -1;
try {
version = (Integer) this.attributes.get("sdk_protocol_version");
}catch (Exception e) {
//do nothing.
}
return version;
}
/**
* Gets the application key.
*
* @return the application key
*/
public ApplicationKey getApplication() {
return application;
}
/**
* Gets the data of the device.
*
* @return the data of the device.
*/
public DeviceData getDevice() {
return device;
}
/**
* Gets the attributes map sent by the client during registration.
*
* @return a map
*/
public Map getAttributes() {
return attributes;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy