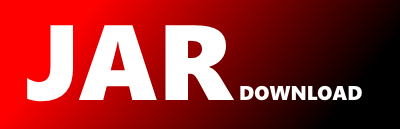
com.ibm.mfp.server.security.external.JSONUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of adapter-maven-api Show documentation
Show all versions of adapter-maven-api Show documentation
IBM MFP Adapter api for adapter as a maven project. BuildNumber is : 8.0.2024082809
The newest version!
/*
* IBM Confidential OCO Source Materials
*
* 5725-I43 Copyright IBM Corp. 2006, 2016
*
* The source code for this program is not published or otherwise
* divested of its trade secrets, irrespective of what has
* been deposited with the U.S. Copyright Office.
*/
package com.ibm.mfp.server.security.external;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.PropertyAccessor;
import com.fasterxml.jackson.core.JsonGenerator;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.databind.*;
import com.fasterxml.jackson.databind.jsontype.TypeSerializer;
import com.fasterxml.jackson.databind.module.SimpleModule;
import org.apache.commons.codec.binary.Base64;
import java.io.IOException;
import java.math.BigInteger;
import java.security.KeyFactory;
import java.security.interfaces.RSAPublicKey;
import java.security.spec.RSAPublicKeySpec;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
* Reusable Jackson ObjectMapper
*
* @author artem
* Date: 9/9/15
*/
public class JSONUtils {
/**
* Includes all the fields except of transient, and ignores all the accessor methods.
* Writes classes for non-concrete objects
*/
public static final ObjectMapper FIELDS_OBJECT_MAPPER;
public static final String RSA_MODULUS_FIELD = "n";
public static final String RSA_EXPONENT_FIELD = "e";
public static final String KEY_TYPE_FIELD = "kty";
public static final String RSA_KEY_TYPE = "RSA";
static {
FIELDS_OBJECT_MAPPER = new ObjectMapper();
FIELDS_OBJECT_MAPPER.configure(JsonParser.Feature.ALLOW_SINGLE_QUOTES, true);
FIELDS_OBJECT_MAPPER.setVisibility(PropertyAccessor.FIELD, JsonAutoDetect.Visibility.ANY)
.setVisibility(PropertyAccessor.GETTER, JsonAutoDetect.Visibility.NONE)
.setVisibility(PropertyAccessor.SETTER, JsonAutoDetect.Visibility.NONE)
.setVisibility(PropertyAccessor.IS_GETTER, JsonAutoDetect.Visibility.NONE);
FIELDS_OBJECT_MAPPER.enableDefaultTyping(ObjectMapper.DefaultTyping.OBJECT_AND_NON_CONCRETE);
SimpleModule module = new SimpleModule();
module.addSerializer(RSAPublicKey.class, new PublicKeySerializer());
module.addDeserializer(RSAPublicKey.class, new PublicKeyDeserializer());
FIELDS_OBJECT_MAPPER.registerModule(module);
}
public static class PublicKeySerializer extends JsonSerializer {
@Override
public void serializeWithType(RSAPublicKey value, JsonGenerator gen, SerializerProvider serializers, TypeSerializer typeSer) throws IOException {
typeSer.writeCustomTypePrefixForObject(value, gen, RSAPublicKey.class.getName());
serialize(value, gen, serializers);
typeSer.writeCustomTypeSuffixForObject(value, gen, RSAPublicKey.class.getName());
}
@Override
public void serialize(RSAPublicKey publicKey, JsonGenerator gen, SerializerProvider serializers) throws IOException {
gen.writeStringField(KEY_TYPE_FIELD, RSA_KEY_TYPE);
String mod = Base64.encodeBase64URLSafeString(publicKey.getModulus().toByteArray());
gen.writeStringField(RSA_MODULUS_FIELD, mod);
String exp = Base64.encodeBase64URLSafeString(publicKey.getPublicExponent().toByteArray());
gen.writeStringField(RSA_EXPONENT_FIELD, exp);
}
}
public static class PublicKeyDeserializer extends JsonDeserializer {
private static final Logger logger = Logger.getLogger(PublicKeyDeserializer.class.getName());
@Override
public RSAPublicKey deserialize(JsonParser p, DeserializationContext ctxt) throws IOException {
JsonNode node = p.getCodec().readTree(p);
String kty = node.get(KEY_TYPE_FIELD).asText();
String modStr = node.get(RSA_MODULUS_FIELD).asText();
BigInteger mod = new BigInteger(1, Base64.decodeBase64(modStr));
String expStr = node.get(RSA_EXPONENT_FIELD).asText();
BigInteger exp = new BigInteger(1, Base64.decodeBase64(expStr));
RSAPublicKeySpec rsaKeyspec = new RSAPublicKeySpec(mod, exp);
try {
return (RSAPublicKey) KeyFactory.getInstance(kty).generatePublic(rsaKeyspec);
} catch (Exception e) {
logger.log(Level.SEVERE, "Error occurred while trying to deserialize publicKey");
throw new RuntimeException(e);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy