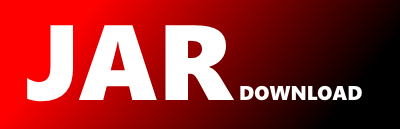
com.ibm.mfp.server.security.external.checks.impl.ExternalizableSecurityCheck Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of adapter-maven-api Show documentation
Show all versions of adapter-maven-api Show documentation
IBM MFP Adapter api for adapter as a maven project. BuildNumber is : 8.0.2024082809
The newest version!
/*
* © Copyright IBM Corp. 2016
* All Rights Reserved. US Government Users Restricted Rights - Use, duplication or disclosure restricted by GSA ADP Schedule Contract with IBM Corp.
*/
package com.ibm.mfp.server.security.external.checks.impl;
import com.ibm.mfp.server.security.external.JSONUtils;
import com.ibm.mfp.server.security.external.checks.*;
import java.io.IOException;
import java.io.ObjectInput;
import java.io.ObjectOutput;
import java.util.*;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
* Convenience base class for security check implementations. Provides the following features:
*
* Automatic externalization as JSON object. Not externalizable fields must be marked transient.
* Configuration property inactivityTimeoutSec
* Implementation of {@link #getExpiresAt()} via state management mechanism that includes
* {@link #initStateDurations(Map)}, {@link #setState(String)}, and {@link #getState()}
* Empty implementation of the {@link #logout()} method
*
*
* @author artem
* Date: 8/17/15
*/
public abstract class ExternalizableSecurityCheck implements SecurityCheck {
private static final Logger logger = Logger.getLogger(ExternalizableSecurityCheck.class.getName());
/**
* Predefined state name that means the security check is expired and its state will not be preserved.
*/
protected static final String STATE_EXPIRED = "expired";
protected transient ExternalizableSecurityCheckConfig config;
protected transient AuthorizationContext authorizationContext;
protected transient RegistrationContext registrationContext;
private transient Map stateDurations;
// runtime state
private String checkName;
private int inactivityTimeoutSec;
private String stateName = STATE_EXPIRED;
private long stateSetAt;
private boolean oldInstance = false;
@Override
public SecurityCheckConfiguration createConfiguration(Properties properties) {
return new ExternalizableSecurityCheckConfig(properties);
}
/**
* Calculates the expiration for the current state set via {@link #setState(String)} based on state durations
* returned by {@link #initStateDurations(Map)}
* If the current state is {@link #STATE_EXPIRED}, this method returns 0
*
* @return the time when the current state will be expired, or 0 if the current state is null
*/
@Override
public long getExpiresAt() {
if (stateName.equals(STATE_EXPIRED)) return 0;
Integer duration = stateDurations.get(stateName);
if (duration == null) {
logger.severe("Unsupported state name '" + stateName + "' for security check '" + checkName + "'. The state is dropped.");
stateName = STATE_EXPIRED;
return 0;
}
return stateSetAt + duration * 1000;
}
@Override
public int getInactivityTimeoutSec() {
return inactivityTimeoutSec;
}
@Override
public void setContext(String name, SecurityCheckConfiguration config, AuthorizationContext authorizationContext, RegistrationContext registrationContext) {
logger.log(Level.FINEST, "Setting context for '" + name + "' security check.");
this.checkName = name;
this.authorizationContext = authorizationContext;
this.registrationContext = registrationContext;
this.config = (ExternalizableSecurityCheckConfig) config;
inactivityTimeoutSec = getConfiguration().inactivityTimeoutSec;
stateDurations = new HashMap<>();
initStateDurations(stateDurations);
if (stateDurations.containsKey(STATE_EXPIRED))
throw new RuntimeException("The state '" + STATE_EXPIRED + "' is predefined and should not be added to the durations map.");
}
@Override
public void logout() {
// do nothing, subclasses may override to implement custom logic
oldInstance= false;
}
public void writeExternal(ObjectOutput out) throws IOException {
String jsonStr = JSONUtils.FIELDS_OBJECT_MAPPER.writeValueAsString(this);
out.writeObject(jsonStr);
}
public void readExternal(ObjectInput in) throws IOException, ClassNotFoundException {
String jsonStr = (String) in.readObject();
JSONUtils.FIELDS_OBJECT_MAPPER.readerForUpdating(this).readValue(jsonStr);
}
protected ExternalizableSecurityCheckConfig getConfiguration() {
return config;
}
protected String getName() {
return checkName;
}
/**
* Set the given state and mark the time.
* If the state is already set to the given value, no change is done.
*
* @param name the name of the state, must be one of those defined by {@link #initStateDurations(Map)}, or {@link #STATE_EXPIRED}
* @see #getExpiresAt()
*/
protected void setState(String name) {
if (!(name.equals(STATE_EXPIRED)) && !stateDurations.containsKey(name)) {
throw new RuntimeException("Unsupported state '" + name + "' for security check '" + checkName + "'.");
}
//if state is expired update the state and the set time
if (!stateName.equals(name) || System.currentTimeMillis() >= getExpiresAt()) {
stateName = name;
stateSetAt = System.currentTimeMillis();
}
}
/**
* Get the current state set via method {@link #setState(String)}
*
* @return the current state name
*/
public String getState() {
// if the state is expired (due to configuration change), drop it
if (!stateName.equals(STATE_EXPIRED) && System.currentTimeMillis() >= getExpiresAt()) {
stateName = STATE_EXPIRED;
}
return stateName;
}
public void resetState(){
logger.severe("Setting state explicitely : oldInstance="+oldInstance);
if(oldInstance){
setState("success");
}else{
oldInstance = true;
}
}
/**
* Put the state names supported by this security check and their durations in seconds into the input map.
* Implementations may use {@link #getConfiguration()} method to access configured values.
* The state {@link #STATE_EXPIRED} is predefined, and should not be put into the map.
*
* @param durations map with the state names as keys and their durations in seconds as values
*/
protected abstract void initStateDurations(Map durations);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy