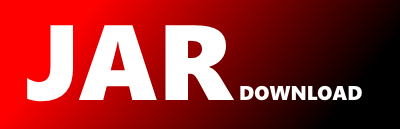
com.ibm.mfp.server.security.external.checks.impl.SecurityCheckConfigurationBase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of adapter-maven-api Show documentation
Show all versions of adapter-maven-api Show documentation
IBM MFP Adapter api for adapter as a maven project. BuildNumber is : 8.0.2024082809
The newest version!
/*
* © Copyright IBM Corp. 2016
* All Rights Reserved. US Government Users Restricted Rights - Use, duplication or disclosure restricted by GSA ADP Schedule Contract with IBM Corp.
*/
package com.ibm.mfp.server.security.external.checks.impl;
import com.ibm.mfp.server.security.external.checks.SecurityCheckConfiguration;
import java.util.HashMap;
import java.util.Map;
import java.util.Properties;
/**
* Convenience base class for implementations of SecurityCheckConfiguration
*
* @author artem on 11/12/15.
*/
public abstract class SecurityCheckConfigurationBase implements SecurityCheckConfiguration {
protected Map errors = new HashMap<>();
protected Map warnings = new HashMap<>();
protected Map info = new HashMap<>();
@Override
public Map getErrors() {
return errors;
}
@Override
public Map getWarnings() {
return warnings;
}
@Override
public Map getInfo() {
return info;
}
/**
* Find property with the given name in Properties.
* If the property exists, it is removed from input Properties to allow tracking unused properties.
* If the property does not exist and the default value is not null, a "missing property" warning is reported, and the default is returned as a result
* If the property does not exist and the default value is null, a "missing property" error is reported.
*
* @param name the property name
* @param properties the properties to get from, modified by the method
* @param defaultValue the value to return if the property does not exist, null means the property is mandatory
* @return property value if the property exists. If the property does not exist - default value if provided, null otherwise
*/
protected String getStringProperty(String name, Properties properties, String defaultValue) {
String val = (String) properties.remove(name);
if (val == null) {
if (defaultValue == null)
addMessage(errors, name, "Missing configuration property");
else {
val = defaultValue;
addMessage(info, name, "Missing configuration property, using default value " + defaultValue);
}
}
return val;
}
/**
* Find property with the given name in Properties and convert it to int.
* If the property exists, it is removed from input Properties to allow tracking unused properties.
* If the value cannot be converted to int, an "invalid value" error is reported
* If the property does not exist and the default value is not null, a "missing property" warning is reported, and the default is returned as a result
* If the property does not exist and the default value is null, a "missing property" error is reported.
*
* @param name the property name
* @param properties the properties to get from, modified by the method
* @param defaultValue the value to return if the property does not exist, null means the property is mandatory
* @return property value if the property exists. If the property does not exist - default value if provided, -1 otherwise
*/
protected int getIntProperty(String name, Properties properties, Integer defaultValue) {
String strVal = getStringProperty(name, properties, defaultValue == null ? null : String.valueOf(defaultValue));
if (strVal != null)
try {
return Integer.parseInt(strVal);
} catch (NumberFormatException e) {
addMessage(errors, name, "Invalid property value: " + strVal);
}
return -1;
}
protected void addMessage(Map map, String property, String msg) {
String messages = map.get(property);
messages = messages == null ? msg : messages + " " + msg;
map.put(property, messages);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy