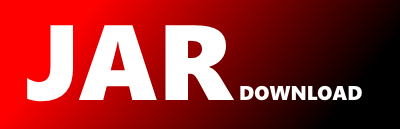
com.ibm.mfp.server.security.external.resource.ClientSearchCriteria Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of adapter-maven-api Show documentation
Show all versions of adapter-maven-api Show documentation
IBM MFP Adapter api for adapter as a maven project. BuildNumber is : 8.0.2024082809
The newest version!
/*
* © Copyright IBM Corp. 2016
* All Rights Reserved. US Government Users Restricted Rights - Use, duplication or disclosure restricted by GSA ADP Schedule Contract with IBM Corp.
*/
package com.ibm.mfp.server.security.external.resource;
import java.util.HashMap;
import java.util.Map;
/**
* A search criteria for finding mobile clients registration data. A search criteria is a combination of filters of the following three types:
*
* - Filter by user ID
* - Filter by application
* - Filter by a public attribute
*
*
* A search criteria can include at most one user filter, at most one application filter and zero or more attribute filters.
* A user filter is added using the method byUser
. A user filter consists of a security check name and a user ID.
* The filter searches for clients associated with the given user ID, authenticated by the given security check
*
* An application filter is added using the method byApplication
. It searches for clients of a given application ID and / or a specific
* application version.
*
* Attribute filters search for public attributes in the client registration data. Attribute filters are added using the method byAttribute
.
* An attribute filter consists of an attribute name and value pair, and it searches for clients with a matching attriute.
*
* Filters can be added to a search criteria by chaining calls to byUser
, byApplication
and byAttribute
. The example below
* constructs a search criteria that combines all three types of filters.
*
*
*
* ClientSearchCriteria criteria = new ClientSearchCriteria()
* .byUser("UserLogin", userName)
* .byApplication("com.ibm.myApp", "1.0")
* .byAttribute(attributeName, attributeValue)
* .maxLength(10);
*
*
*
* A serach criteria has a limit on the number of clients that can be returned in the search results. The default number is 500, and it can be
* changed using the method maxLength
.
*
* @author artem on 12/16/15.
*/
public class ClientSearchCriteria {
private String securityCheckName;
private String userId;
private String appId;
private String appVersion;
private Map attributes = new HashMap<>();
private int maxLength = 500;
/**
* Adds a user ID filter to the search criteria. If the method is called more than once,
* only the last call applies (because a search criteria can have at most one user filter).
*
* @param securityCheckName the security check that authenticated the user, not null
* @param userId the user ID search string, not null
* @return this search criteria instance
*/
public ClientSearchCriteria byUser(String securityCheckName, String userId) {
assert securityCheckName != null && userId != null;
this.securityCheckName = securityCheckName;
this.userId = userId;
return this;
}
/**
* Adds an application filter to the search criteria. An application filter can search by both the application ID and
* the application version, or by one of these parameters.
If the method is called more than once,
* only the last call applies (because a search criteria can have at most one application filter).
*
* @param appId application ID. If the value is null, the filter searches for all clients with the specified version, regardless of their application ID
* @param appVersion application version, null means all versions of the given application ID
* @return this search criteria instance
*/
public ClientSearchCriteria byApplication(String appId, String appVersion) {
this.appId = appId;
this.appVersion = appVersion;
return this;
}
/**
* Adds an attribute filter to the search criteria.
* Can be called multiple times to add multiple attribute filters.
*
* @param name public attribute name, not null
* @param value attribute value, not null
* @return this search criteria instance
*/
public ClientSearchCriteria byAttribute(String name, String value) {
assert name != null && value != null;
attributes.put(name, value);
return this;
}
/**
* Sets the maximum number of clients to be returned in the search results. The default is 500.
*
* @param maxLength maximum result length
* @return this search criteria instance
*/
public ClientSearchCriteria maxLength(int maxLength) {
this.maxLength = maxLength;
return this;
}
/**
* Gets the name of the security check defined in the user filter. Returns null if the search criteria does
* not include a user filter.
* @return the name of the security check defined in the user filter; null if the search criteria does
* not include a user filter
*/
public String getSecurityCheckName() {
return securityCheckName;
}
/**
* Gets the user ID search string defined in the user filter. Returns null if the search criteria does
* not include a user filter.
* @return the user ID search string; null if the search criteria does
* not include a user filter
*/
public String getUserId() {
return userId;
}
/**
* Gets the application ID defined in the application filter. Returns null if the search criteria does not
* include an application filter, or the filter was added with a null application ID.
* @return the application ID; null if the search criteria does not
* include an application filter, or the filter was added with a null application ID
*/
public String getAppId() {
return appId;
}
/**
* Gets the application version defined in the application filter.
Returns null if the search criteria does not
* include an application filter, or the filter was added with a null application version.
* @return the application version; null if the search criteria does not
* include an application filter, or the filter was added with a null application version
*/
public String getAppVersion() {
return appVersion;
}
/**
* Gets the attribute filters (attribute name and value pairs) included in this search criteria.
* @return the attribute filters (attribute name and value pairs) included in this search criteria
*/
public Map getAttributes() {
return attributes;
}
/**
* Gets the max number of clients to be returned in the serach results.
* @return the max number of clients to be returned in the serach results
*/
public int getMaxLength() {
return maxLength;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy