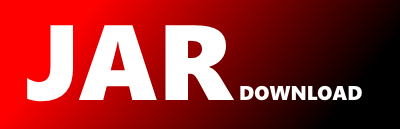
com.ibm.mfp.server.security.external.resource.TokenIntrospectionData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of adapter-maven-api Show documentation
Show all versions of adapter-maven-api Show documentation
IBM MFP Adapter api for adapter as a maven project. BuildNumber is : 8.0.2024082809
The newest version!
/*
* © Copyright IBM Corp. 2016
* All Rights Reserved. US Government Users Restricted Rights - Use, duplication or disclosure restricted by GSA ADP Schedule Contract with IBM Corp.
*/
package com.ibm.mfp.server.security.external.resource;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.ibm.mfp.server.app.external.ApplicationKey;
import com.ibm.mfp.server.registration.external.model.AuthenticatedUser;
import com.ibm.mfp.server.registration.external.model.DeviceData;
import java.util.HashSet;
import java.util.Map;
import java.util.StringTokenizer;
/**
* Following spec:
* https://tools.ietf.org/html/draft-ietf-oauth-introspection-11#section-2.2
* OAuth 2.0 Token Introspection - Introspection Response
* The data includes additional fields that are not included in the spec, such as the application and the device of the client.
*/
public class TokenIntrospectionData {
public static final TokenIntrospectionData INACTIVE_TOKEN = new TokenIntrospectionData();
public static final String ANONYMOUS_USER = "anonymous";
/**
* Is the client authenticated
*/
private boolean active;
/**
* The scope relevant for the introspection data
*/
private String scope;
/**
* Client identifier
*/
@JsonProperty("client_id")
private String clientId;
/**
* The active user
*/
private String username = ANONYMOUS_USER;
/**
* Expiration of the token
*/
@JsonProperty("exp")
private long expiration;
@JsonProperty("mfp-application")
private ApplicationKey application;
@JsonProperty("mfp-device")
private DeviceData device;
@JsonProperty("mfp-user")
private AuthenticatedUser user;
@JsonProperty("mfp-checks")
private Map> checksCustomData;
/**
* Constructor reserved for internal use only.
*/
protected TokenIntrospectionData() {
}
/**
* Constructor reserved for internal use only.
*/
public TokenIntrospectionData(String clientId, String scope, long expiration,
ApplicationKey application, DeviceData device, AuthenticatedUser user,
Map> checksCustomData) {
active = true;
this.clientId = clientId;
this.scope = scope;
this.expiration = expiration;
this.application = application;
this.device = device;
if (user!= null) {
this.username = user.getId();
this.user = user;
}
this.checksCustomData = checksCustomData;
}
/**
* Return true if the token is active. False otherwise.
* @return true if the token is active. False otherwise.
*/
public boolean isActive() {
return active;
}
/**
* Gets the scope associated with this token.
* @return the scope associated with this token
*/
public String getScope() {
return scope;
}
/**
* Gets the client identifier for the client that requested the token.
* @return the client identifier for the client that requested the token
*/
public String getClientId() {
return clientId;
}
/**
* Gets the identifier for the authenticated user associated with this token.
* @return the identifier for the authenticated user
*/
public String getUsername() {
return username;
}
/**
* Gets the expiration time of this token measured in the number of seconds since January 1 1970 UTC.
* @return the expiration time of this token
*/
public long getExpiration() {
return expiration;
}
/**
* Gets the application of the client that requested the token.
* @return the application of the client that requested the token.
*/
public ApplicationKey getApplication() {
return application;
}
/**
* Gets the device of the client that requested the token.
* @return the device of the client that requested the token.
*/
public DeviceData getDevice() {
return device;
}
/**
* Gets the authenticated user associated with this token.
* If none of the security checks has set an active user the method returns null.
* @return the authenticated user associated with this token; null if no active user
*/
public AuthenticatedUser getUser() {
return user;
}
/**
* Gets the custom introspection data provided by the security checks.
* The data is provided as a map between the name of the security check and the custom introspection data it provided.
* The map includes only security checks that provided a non-empty custom introspection data.
* @return custom introspection data provided by the security checks
*/
public Map> getChecksCustomData() {
return checksCustomData;
}
/**
* Checks whether the scope associated with the token covers the given required scope.
* The scope is covered if all scope elements of the required scope are included in the scope associated with this token.
* @param requiredScope the scope that should be covered
* @return true if the given required scope is covered by this token, false otherwise.
*/
public boolean isScopeCovered(String requiredScope) {
HashSet grantedTokens = new HashSet<>();
if (scope != null) {
StringTokenizer tokenizer = new StringTokenizer(scope, " ");
while (tokenizer.hasMoreTokens()) grantedTokens.add(tokenizer.nextToken());
}
StringTokenizer tokenizer = new StringTokenizer(requiredScope, " ");
while(tokenizer.hasMoreTokens())
if (!grantedTokens.contains(tokenizer.nextToken()))
return false;
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy