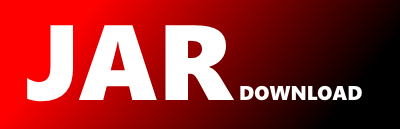
com.ibm.mfp.java.token.validator.TokenIntrospectionData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mfp-java-token-validator Show documentation
Show all versions of mfp-java-token-validator Show documentation
IBM MFP Java token validator is used to validate Oauth tokens against an Authorization server, BuildNumber is : 8.0.2017020112
/*
* IBM Confidential OCO Source Materials
*
* 5725-I43 Copyright IBM Corp. 2006, 2016
*
* The source code for this program is not published or otherwise
* divested of its trade secrets, irrespective of what has
* been deposited with the U.S. Copyright Office.
*/
package com.ibm.mfp.java.token.validator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.ibm.mfp.java.token.validator.data.ApplicationKey;
import com.ibm.mfp.java.token.validator.data.AuthenticatedUser;
import com.ibm.mfp.java.token.validator.data.MobileDeviceData;
import java.util.HashSet;
import java.util.Map;
import java.util.StringTokenizer;
/**
* Following spec:
* https://tools.ietf.org/html/draft-ietf-oauth-introspection-11#section-2.2
* OAuth 2.0 Token Introspection - Introspection Response
*/
public class TokenIntrospectionData {
public static final TokenIntrospectionData INACTIVE_TOKEN = new TokenIntrospectionData();
public static final String ANONYMOUS_USER = "anonymous";
/**
* Is the client authenticated
*/
private boolean active;
/**
* The scope relevant for the introspection data
*/
private String scope;
/**
* Client identifier
*/
@JsonProperty("client_id")
private String clientId;
/**
* The active user
*/
private String username = ANONYMOUS_USER;
/**
* Expiration of the token
*/
@JsonProperty("exp")
private long expiration;
@JsonProperty("mfp-application")
private ApplicationKey application;
@JsonProperty("mfp-device")
private MobileDeviceData device;
@JsonProperty("mfp-user")
private AuthenticatedUser user;
@JsonProperty("mfp-checks")
private Map> checks;
/**
* Create active token data
*
* @param clientId client ID
* @param scope token scope
* @param expiration token expiration
*/
public TokenIntrospectionData(String clientId, String scope, long expiration) {
this.clientId = clientId;
this.scope = scope;
this.expiration = expiration;
active = true;
}
public TokenIntrospectionData() {
}
public boolean isActive() {
return active;
}
public String getScope() {
return scope;
}
public String getClientId() {
return clientId;
}
public String getUsername() {
return username;
}
public long getExpiration() {
return expiration;
}
public ApplicationKey getApplication() {
return application;
}
public MobileDeviceData getDevice() {
return device;
}
public AuthenticatedUser getUser() {
return user;
}
public Map> getChecks() {
return checks;
}
public void setUser(AuthenticatedUser user) {
this.user = user;
if(user != null)
this.username = user.getId();
}
public void setApplication(ApplicationKey application) {
this.application = application;
}
public void setDevice(MobileDeviceData device) {
this.device = device;
}
public void setCustomIntrospectionData(Map> checkIntrospectionData) {
checks = checkIntrospectionData;
}
public boolean isScopeCovered(String requiredScope) {
HashSet grantedTokens = new HashSet<>();
if (scope != null) {
StringTokenizer tokenizer = new StringTokenizer(scope, " ");
while (tokenizer.hasMoreTokens()) grantedTokens.add(tokenizer.nextToken());
}
StringTokenizer tokenizer = new StringTokenizer(requiredScope, " ");
while(tokenizer.hasMoreTokens())
if (!grantedTokens.contains(tokenizer.nextToken()))
return false;
return true;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy