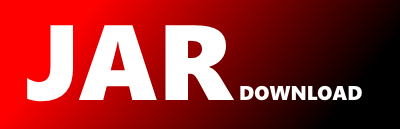
com.ibm.mfp.java.token.validator.TokenIntrospectionData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mfp-java-token-validator Show documentation
Show all versions of mfp-java-token-validator Show documentation
IBM MFP Java token validator is used to validate Oauth tokens against an Authorization server, BuildNumber is : 8.0.2017020112
/*
* © Copyright IBM Corp. 2016
* All Rights Reserved. US Government Users Restricted Rights - Use, duplication or disclosure restricted by GSA ADP Schedule Contract with IBM Corp.
*/
package com.ibm.mfp.java.token.validator;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.HashSet;
import java.util.Map;
import java.util.StringTokenizer;
/**
* Following spec:
* https://tools.ietf.org/html/draft-ietf-oauth-introspection-11#section-2.2
* OAuth 2.0 Token Introspection - Introspection Response
*/
public class TokenIntrospectionData {
public static final TokenIntrospectionData INACTIVE_TOKEN = new TokenIntrospectionData();
/**
* Is the client authenticated
*/
private boolean active;
/**
* The scope relevant for the introspection data
*/
private String scope;
/**
* Expiration of the token
*/
@JsonProperty("exp")
private long expiration;
public void setData(Map data) {
this.data = data;
}
private Map data;
public Map getData() {
return data;
}
/**
* Create active token data
*
* @param scope token scope
* @param expiration token expiration
*/
public TokenIntrospectionData(String scope, long expiration, boolean active) {
this.scope = scope;
this.expiration = expiration;
this.active = active;
}
/**
* Create active token data
*
* @param scope token scope
* @param expiration token expiration
*/
public TokenIntrospectionData(String scope, long expiration) {
this.scope = scope;
this.expiration = expiration;
active = true;
}
public TokenIntrospectionData() {
}
public boolean isActive() {
return active;
}
public String getScope() {
return scope;
}
public long getExpiration() {
return expiration;
}
public boolean isScopeCovered(String requiredScope) {
HashSet grantedTokens = new HashSet<>();
if (scope != null) {
StringTokenizer tokenizer = new StringTokenizer(scope, " ");
while (tokenizer.hasMoreTokens()) grantedTokens.add(tokenizer.nextToken());
}
StringTokenizer tokenizer = new StringTokenizer(requiredScope, " ");
while(tokenizer.hasMoreTokens())
if (!grantedTokens.contains(tokenizer.nextToken()))
return false;
return true;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy