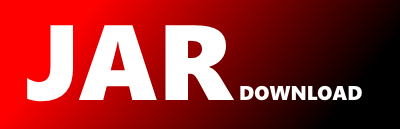
com.ibm.mfp.java.token.validator.TokenValidationCache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mfp-java-token-validator Show documentation
Show all versions of mfp-java-token-validator Show documentation
IBM MFP Java token validator is used to validate Oauth tokens against an Authorization server, BuildNumber is : 8.0.2017020112
/*
* © Copyright IBM Corp. 2016
* All Rights Reserved. US Government Users Restricted Rights - Use, duplication or disclosure restricted by GSA ADP Schedule Contract with IBM Corp.
*/
package com.ibm.mfp.java.token.validator;
import java.util.*;
/**
* A Cache system for TokenIntrospectionData, to be used internally only
* Every time we reach the max, we clean a third of the cache ( Oldest items )
* Created by Ore Poran on 11/25/15.
*/
class TokenValidationCache {
Map cache;
Queue cacheList;
long maxCacheSize = 0;
long cacheSizeCounter = 0;
public TokenValidationCache(long cacheSize) {
this.maxCacheSize = cacheSize;
cache = new HashMap<>();
cacheList = new LinkedList<>();
}
public void set(String authHeader, TokenIntrospectionData data) {
if(cacheSizeCounter >= maxCacheSize) {
removeOldest();
}
if(cacheSizeCounter < maxCacheSize) {
cache.put(authHeader, data);
cacheList.add(authHeader);
cacheSizeCounter++;
}
}
public TokenIntrospectionData get(String authHeader) {
TokenIntrospectionData data = cache.get(authHeader);
if (data != null && data.isActive() && isExpired(data)) {
// Active && Expired or soon to be ?, remove and return inactive token
cache.remove(authHeader);
cacheSizeCounter--;
data = TokenIntrospectionData.INACTIVE_TOKEN;
}
return data;
}
private void removeOldest() {
String removedKey;
try{
removedKey = cacheList.remove();
TokenIntrospectionData removedData = cache.remove(removedKey);
if(removedData != null) cacheSizeCounter--;
} catch(NoSuchElementException e) {
// Do nothing
}
}
private boolean isExpired(TokenIntrospectionData introspectionData) {
long currentTime = System.currentTimeMillis();
return introspectionData.getExpiration() <= currentTime;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy