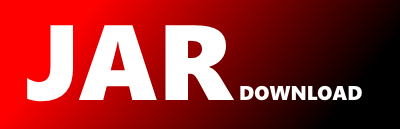
com.ibm.sbt.sample.web.util.Util Maven / Gradle / Ivy
/*
* ��� Copyright IBM Corp. 2013
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.ibm.sbt.sample.web.util;
import java.util.HashMap;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import com.ibm.commons.runtime.Application;
import com.ibm.commons.runtime.Context;
import com.ibm.commons.runtime.util.UrlUtil;
import com.ibm.commons.util.PathUtil;
import com.ibm.commons.util.StringUtil;
import com.ibm.sbt.services.endpoints.EndpointFactory;
/**
* @author Mark Wallace
* @date 16 Jan 2013
*/
public class Util {
public static String[][] JS_LIBS = new String[][] {
// title, id, jsLibPath, jsLibParams, libraryUrl
{ "Dojo Toolkit 1.8.0", "dojo180", "includes/dojo180.jsp", "/library?lib=dojo&ver=1.8.0" },
{ "Dojo Toolkit 1.8.0 CDN", "dojo180cdn", "includes/dojo180cdn.jsp", "/library?lib=dojo&ver=1.8.0" },
{ "Dojo Toolkit 1.8.0 CDN Async", "dojo180cdnasync", "includes/dojo180cdnasync.jsp", "/library?lib=dojo&ver=1.8.0" },
{ "Dojo Toolkit 1.7.0 CDN", "dojo170cdn", "includes/dojo170cdn.jsp", "/library?lib=dojo&ver=1.7.0" },
{ "Dojo Toolkit 1.7.0 CDN Async", "dojo170cdnasync", "includes/dojo170cdnasync.jsp", "/library?lib=dojo&ver=1.7.0" },
{ "Dojo Toolkit 1.4.3", "dojo143", "includes/dojo143.jsp", "/library?lib=dojo&ver=1.4.3" },
{ "jQuery 1.8.2", "jquery182", "includes/jquery182.jsp", "/library?lib=jquery&ver=1.8.2" },
{ "jQuery 1.8.2 ScriptTag", "jquery182ST", "includes/jquery182ScriptTag.jsp", "/library?lib=jquery&ver=1.8.2" },
//{ "jQuery 1.8.2 Debug", "jquery182debug", "includes/jquery182debug.jsp", "/library?lib=jquery&ver=1.8.2" },
{ "jQuery 1.9.1 CDN", "jquery191cdn", "includes/jquery191cdn.jsp", "/library?lib=jquery&ver=1.9.1" },
{ "jQuery 1.9.1 CDN Debug", "jquery191cdndebug", "includes/jquery191cdndebug.jsp", "/library?lib=jquery&ver=1.9.1" }//,
//{ "Dojo Toolkit 1.8.0 SBT Layer", "dojo180Layer", "includes/dojo180Layer.jsp", "/library?lib=dojo&ver=1.8.0&layer=true&noext" }
//{ "Dojo Toolkit 1.8.0 SBT Layer CDN", "dojo180LayerCDN", "includes/dojo180LayerCDN.jsp", "/library?lib=dojo&ver=1.8.0&layer=true&noext" }
};
public static String[][] THEMES = new String[][] {
// title, id
{ "Dojo Claro", "dojo" },
{ "Bootstrap", "bootstrap" },
{ "One UI", "oneui" }
};
public static final String defaultEnvironment = "defaultEnvironment";
public static String[][] ENVIRONMENTS = new String[][] {
// title, id
{ "Default", "defaultEnvironment"},
{ "Connections", "connectionsEnvironment"},
{ "Connections OAuth2.0", "connectionsOA2Environment"},
{ "SmartCloud", "smartcloudEnvironment"},
{ "SmartCloud C1", "smartcloudC1Environment"},
{ "Third Party APIs", "thirdPartyAPIsEnvironment"},
{ "Domino", "dominoEnvironment"},
{ "OpenSocial", "openSocial"}
};
public static String[] BOOTSTRAP_STYLES = {
"/sbt.bootstrap211/bootstrap/css/bootstrap.css",
"/sbt.bootstrap211/bootstrap/css/bootstrap-responsive.css"
};
public static String[] DOJO_STYLES = new String[0];
public static String[] ONE_UI_STYLES = {
// "/sbt.oneui303/css/base/core.css", // this theme doesn't seem to be used in connections.
"/connections/resources/web/_style?include=com.ibm.lconn.core.styles.oneui3/base/package3.css",
"/connections/resources/web/_style?include=com.ibm.lconn.core.styles.oneui3/sprites.css",
"/connections/resources/web/_lconntheme/default.css?version=oneui3&rtl=false",
"/connections/resources/web/_lconnappstyles/default/search.css?version=oneui3&rtl=false"
};
private static Map _styleMap = new HashMap();
static {
_styleMap.put("bootstrap", BOOTSTRAP_STYLES);
_styleMap.put("dojo", DOJO_STYLES);
_styleMap.put("oneui", ONE_UI_STYLES);
}
public static String[] combineStyleArrays(){
int allStylesLength = 0;
for(String[] array : _styleMap.values()){
allStylesLength += array.length;
}
String[] allStyles = new String[allStylesLength];
int offset = 0;
for(String[] array : _styleMap.values()){
System.arraycopy(array, 0, allStyles, offset, array.length);
offset+=array.length;
}
return allStyles;
}
public static String[] ALL_STYLES = combineStyleArrays();
static{
_styleMap.put("all", ALL_STYLES);
};
private static Map _bodyClassMap = new HashMap();
static {
_bodyClassMap.put("bootstrap", "claro");
_bodyClassMap.put("dojo", "claro");
_bodyClassMap.put("oneui", "lotusui30_body lotusui30_fonts lotusui30");
_bodyClassMap.put("all", "lotusui30_body lotusui30_fonts lotusui30");
}
private static String[][] _environments = null;
public static String[][] getJavaScriptLibs(HttpServletRequest request) {
return JS_LIBS;
}
public static String getSelectedLibrary(HttpServletRequest request) {
String id = request.getParameter("jsLibId");
return (StringUtil.isEmpty(id)) ? JS_LIBS[0][1] : id;
}
public static String getLibraryInclude(HttpServletRequest request) {
String id = request.getParameter("jsLibId");
if (StringUtil.isEmpty(id)) {
id = JS_LIBS[0][1];
}
String libraryInclude = null;
for (int i=0; i ";
}
return "";
}
public static String getNavbar(HttpServletRequest request) {
String showSmartCloudNavbar = getProperty("showSmartCloudNavbar");
if ("true".equalsIgnoreCase(showSmartCloudNavbar)) {
String url = getProperty("smartcloud.url");
return "";
}
return "";
}
public static boolean isShowNavbar(HttpServletRequest request) {
String showSmartCloudNavbar = getProperty("showSmartCloudNavbar");
return ("true".equalsIgnoreCase(showSmartCloudNavbar));
}
public static String getProperty(String name) {
String value = Context.get().getProperty(name);
if (value != null) {
return value;
}
return Application.get().getProperty(name);
}
//
// Internals
//
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy