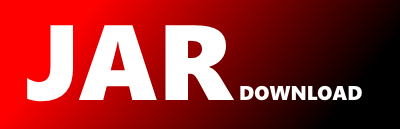
com.ibm.wala.cast.java.translator.JavaCAst2IRTranslator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.ibm.wala.cast.java Show documentation
Show all versions of com.ibm.wala.cast.java Show documentation
T. J. Watson Libraries for Analysis
The newest version!
/*
* Copyright (c) 2002 - 2006 IBM Corporation.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* IBM Corporation - initial API and implementation
*/
/*
* Created on Aug 22, 2005
*/
package com.ibm.wala.cast.java.translator;
import com.ibm.wala.cast.ir.translator.AstTranslator;
import com.ibm.wala.cast.java.loader.JavaSourceLoaderImpl;
import com.ibm.wala.cast.java.ssa.AstJavaInvokeInstruction;
import com.ibm.wala.cast.java.ssa.AstJavaNewEnclosingInstruction;
import com.ibm.wala.cast.java.ssa.EnclosingObjectReference;
import com.ibm.wala.cast.loader.AstMethod.DebuggingInformation;
import com.ibm.wala.cast.tree.CAstControlFlowMap;
import com.ibm.wala.cast.tree.CAstEntity;
import com.ibm.wala.cast.tree.CAstNode;
import com.ibm.wala.cast.tree.CAstQualifier;
import com.ibm.wala.cast.tree.CAstSourcePositionMap.Position;
import com.ibm.wala.cast.tree.CAstType;
import com.ibm.wala.cast.tree.CAstType.Method;
import com.ibm.wala.cast.tree.visit.CAstVisitor;
import com.ibm.wala.cfg.AbstractCFG;
import com.ibm.wala.cfg.IBasicBlock;
import com.ibm.wala.classLoader.CallSiteReference;
import com.ibm.wala.classLoader.IClass;
import com.ibm.wala.classLoader.ModuleEntry;
import com.ibm.wala.classLoader.NewSiteReference;
import com.ibm.wala.ssa.SSAInstruction;
import com.ibm.wala.ssa.SymbolTable;
import com.ibm.wala.types.FieldReference;
import com.ibm.wala.types.TypeName;
import com.ibm.wala.types.TypeReference;
import com.ibm.wala.util.config.SetOfClasses;
import com.ibm.wala.util.debug.Assertions;
import java.util.Collection;
import java.util.Collections;
import java.util.Map;
public class JavaCAst2IRTranslator extends AstTranslator {
private final CAstEntity fSourceEntity;
private final ModuleEntry module;
private final SetOfClasses exclusions;
public JavaCAst2IRTranslator(
ModuleEntry module,
CAstEntity sourceFileEntity,
JavaSourceLoaderImpl loader,
SetOfClasses exclusions) {
super(loader);
fSourceEntity = sourceFileEntity;
this.module = module;
this.exclusions = exclusions;
}
public void translate() {
translate(fSourceEntity, module);
}
public CAstEntity sourceFileEntity() {
return fSourceEntity;
}
public JavaSourceLoaderImpl loader() {
return (JavaSourceLoaderImpl) loader;
}
@Override
protected boolean useDefaultInitValues() {
return true;
}
// Java does not have standalone global variables, and let's not
// adopt the nasty JavaScript practice of creating globals without
// explicit definitions
@Override
protected boolean hasImplicitGlobals() {
return false;
}
@Override
protected TypeReference defaultCatchType() {
return TypeReference.JavaLangThrowable;
}
@Override
protected TypeReference makeType(CAstType type) {
return TypeReference.findOrCreate(
loader.getReference(), TypeName.string2TypeName(type.getName()));
}
// Java globals are disguised as fields (statics), so we should never
// ask this question when parsing Java code
@Override
protected boolean treatGlobalsAsLexicallyScoped() {
Assertions.UNREACHABLE();
return false;
}
@Override
protected void doThrow(WalkContext context, int exception) {
context
.cfg()
.addInstruction(insts.ThrowInstruction(context.cfg().getCurrentInstruction(), exception));
}
@Override
public void doArrayRead(
WalkContext context, int result, int arrayValue, CAstNode arrayRefNode, int[] dimValues) {
TypeReference arrayTypeRef = (TypeReference) arrayRefNode.getChild(1).getValue();
context
.cfg()
.addInstruction(
insts.ArrayLoadInstruction(
context.cfg().getCurrentInstruction(),
result,
arrayValue,
dimValues[0],
arrayTypeRef));
processExceptions(arrayRefNode, context);
}
@Override
public void doArrayWrite(
WalkContext context, int arrayValue, CAstNode arrayRefNode, int[] dimValues, int rval) {
TypeReference arrayTypeRef =
arrayRefNode.getKind() == CAstNode.ARRAY_LITERAL
? ((TypeReference) arrayRefNode.getChild(0).getChild(0).getValue())
.getArrayElementType()
: (TypeReference) arrayRefNode.getChild(1).getValue();
context
.cfg()
.addInstruction(
insts.ArrayStoreInstruction(
context.cfg().getCurrentInstruction(),
arrayValue,
dimValues[0],
rval,
arrayTypeRef));
processExceptions(arrayRefNode, context);
}
@Override
protected void doFieldRead(
WalkContext context, int result, int receiver, CAstNode elt, CAstNode parent) {
// elt is a constant CAstNode whose value is a FieldReference.
FieldReference fieldRef = (FieldReference) elt.getValue();
if (receiver == -1) { // a static field: AstTranslator.getValue() produces
// -1 for null, we hope
context
.cfg()
.addInstruction(
insts.GetInstruction(context.cfg().getCurrentInstruction(), result, fieldRef));
} else {
context
.cfg()
.addInstruction(
insts.GetInstruction(
context.cfg().getCurrentInstruction(), result, receiver, fieldRef));
processExceptions(parent, context);
}
}
@Override
protected void doFieldWrite(
WalkContext context, int receiver, CAstNode elt, CAstNode parent, int rval) {
FieldReference fieldRef = (FieldReference) elt.getValue();
if (receiver == -1) { // a static field: AstTranslator.getValue() produces
// -1 for null, we hope
context
.cfg()
.addInstruction(
insts.PutInstruction(context.cfg().getCurrentInstruction(), rval, fieldRef));
} else {
context
.cfg()
.addInstruction(
insts.PutInstruction(
context.cfg().getCurrentInstruction(), receiver, rval, fieldRef));
processExceptions(parent, context);
}
}
@Override
protected void doMaterializeFunction(
CAstNode n, WalkContext context, int result, int exception, CAstEntity fn) {
// Not possible in Java (no free-standing functions)
Assertions.UNREACHABLE("Real functions in Java??? I don't think so!");
}
@Override
protected void doNewObject(
WalkContext context, CAstNode newNode, int result, Object type, int[] arguments) {
TypeReference typeRef = (TypeReference) type;
NewSiteReference site = NewSiteReference.make(context.cfg().getCurrentInstruction(), typeRef);
if (newNode.getKind() == CAstNode.NEW_ENCLOSING) {
context
.cfg()
.addInstruction(
new AstJavaNewEnclosingInstruction(
context.cfg().getCurrentInstruction(), result, site, arguments[0]));
} else {
context
.cfg()
.addInstruction(
(arguments == null)
? insts.NewInstruction(context.cfg().getCurrentInstruction(), result, site)
: insts.NewInstruction(
context.cfg().getCurrentInstruction(), result, site, arguments));
}
processExceptions(newNode, context);
}
private static void processExceptions(CAstNode n, WalkContext context) {
context.cfg().addPreNode(n, context.getUnwindState());
context.cfg().newBlock(true);
Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy