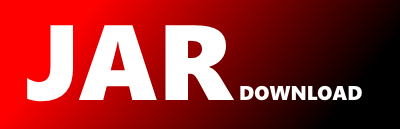
com.ibm.wala.cast.js.vis.JsPaPanel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.ibm.wala.cast.js Show documentation
Show all versions of com.ibm.wala.cast.js Show documentation
T. J. Watson Libraries for Analysis
/*
* Copyright (c) 2013 IBM Corporation.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* IBM Corporation - initial API and implementation
*/
package com.ibm.wala.cast.js.vis;
import com.ibm.wala.analysis.pointers.HeapGraph;
import com.ibm.wala.cast.ipa.callgraph.AstGlobalPointerKey;
import com.ibm.wala.cast.ipa.callgraph.ObjectPropertyCatalogKey;
import com.ibm.wala.core.viz.viewer.PaPanel;
import com.ibm.wala.ipa.callgraph.CGNode;
import com.ibm.wala.ipa.callgraph.CallGraph;
import com.ibm.wala.ipa.callgraph.propagation.InstanceKey;
import com.ibm.wala.ipa.callgraph.propagation.PointerAnalysis;
import com.ibm.wala.ipa.callgraph.propagation.PointerKey;
import com.ibm.wala.util.intset.MutableMapping;
import com.ibm.wala.util.intset.OrdinalSetMapping;
import java.util.ArrayList;
import java.util.List;
/**
* Augments the PaPanel with: 1) global pointer keys at the root level. 2) property catalog key for
* instance keys.
*
* @author yinnonh
*/
public class JsPaPanel extends PaPanel {
private static final long serialVersionUID = 1L;
private final MutableMapping>
instanceKeyIdToObjectPropertyCatalogKey =
MutableMapping.>make();
private final List globalsPointerKeys = new ArrayList<>();
public JsPaPanel(CallGraph cg, PointerAnalysis pa) {
super(cg, pa);
initDataStructures(pa);
}
private void initDataStructures(PointerAnalysis pa) {
HeapGraph heapGraph = pa.getHeapGraph();
OrdinalSetMapping instanceKeyMapping = pa.getInstanceKeyMapping();
for (Object n : heapGraph) {
if (heapGraph.getPredNodeCount(n) == 0) {
if (n instanceof PointerKey) {
if (n instanceof ObjectPropertyCatalogKey) {
ObjectPropertyCatalogKey opck = (ObjectPropertyCatalogKey) n;
InstanceKey instanceKey = opck.getObject();
int instanceKeyId = instanceKeyMapping.getMappedIndex(instanceKey);
mapUsingMutableMapping(instanceKeyIdToObjectPropertyCatalogKey, instanceKeyId, opck);
} else if (n instanceof AstGlobalPointerKey) {
globalsPointerKeys.add((AstGlobalPointerKey) n);
}
} else {
System.err.println("Non Pointer key root: " + n);
}
}
}
}
@Override
protected List getPointerKeysUnderInstanceKey(InstanceKey ik) {
List ret = new ArrayList<>(super.getPointerKeysUnderInstanceKey(ik));
int ikIndex = pa.getInstanceKeyMapping().getMappedIndex(ik);
ret.addAll(nonNullList(instanceKeyIdToObjectPropertyCatalogKey.getMappedObject(ikIndex)));
return ret;
}
private final String cgNodesRoot = "CGNodes";
private final String globalsRoot = "Globals";
@Override
protected List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy