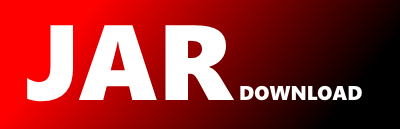
com.ibm.wala.util.graph.impl.SlowSparseNumberedGraph Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.ibm.wala.util Show documentation
Show all versions of com.ibm.wala.util Show documentation
T. J. Watson Libraries for Analysis
/*
* Copyright (c) 2002 - 2006 IBM Corporation.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* IBM Corporation - initial API and implementation
*/
package com.ibm.wala.util.graph.impl;
import com.ibm.wala.util.collections.Iterator2Iterable;
import com.ibm.wala.util.graph.AbstractNumberedGraph;
import com.ibm.wala.util.graph.Graph;
import com.ibm.wala.util.graph.NumberedEdgeManager;
import com.ibm.wala.util.graph.NumberedNodeManager;
import com.ibm.wala.util.intset.BasicNaturalRelation;
import java.io.Serializable;
/** A graph of numbered nodes, expected to have a fairly sparse edge structure. */
public class SlowSparseNumberedGraph extends AbstractNumberedGraph implements Serializable {
private static final long serialVersionUID = 7014361126159594838L;
private final SlowNumberedNodeManager nodeManager = new SlowNumberedNodeManager<>();
private final SparseNumberedEdgeManager edgeManager;
protected SlowSparseNumberedGraph() {
this(0);
}
/**
* If normalOutCount == n, this edge manager will eagerly allocated n words to hold out edges for
* each node. (performance optimization for time)
*
* @param normalOutCount what is the "normal" number of out edges for a node?
*/
public SlowSparseNumberedGraph(int normalOutCount) {
edgeManager =
new SparseNumberedEdgeManager<>(
nodeManager, normalOutCount, BasicNaturalRelation.TWO_LEVEL);
}
/**
* @see com.ibm.wala.util.graph.AbstractGraph#getNodeManager()
*/
@Override
public NumberedNodeManager getNodeManager() {
return nodeManager;
}
/**
* @see com.ibm.wala.util.graph.AbstractGraph#getEdgeManager()
*/
@Override
public NumberedEdgeManager getEdgeManager() {
return edgeManager;
}
/**
* @return a graph with the same nodes and edges as g
*/
public static SlowSparseNumberedGraph duplicate(Graph g) {
SlowSparseNumberedGraph result = make();
copyInto(g, result);
return result;
}
public static void copyInto(Graph g, Graph into) {
if (g == null) {
throw new IllegalArgumentException("g is null");
}
for (T name : g) {
into.addNode(name);
}
for (T n : g) {
for (T succ : Iterator2Iterable.make(g.getSuccNodes(n))) {
into.addEdge(n, succ);
}
}
}
public static SlowSparseNumberedGraph make() {
return new SlowSparseNumberedGraph<>();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy