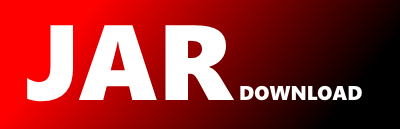
com.ibm.wala.util.graph.traverse.SlowDFSDiscoverTimeIterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.ibm.wala.util Show documentation
Show all versions of com.ibm.wala.util Show documentation
T. J. Watson Libraries for Analysis
The newest version!
/*
* Copyright (c) 2002 - 2006 IBM Corporation.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* IBM Corporation - initial API and implementation
*/
package com.ibm.wala.util.graph.traverse;
import com.ibm.wala.util.collections.HashMapFactory;
import com.ibm.wala.util.collections.NonNullSingletonIterator;
import com.ibm.wala.util.graph.Graph;
import java.util.Iterator;
import java.util.Map;
import org.jspecify.annotations.Nullable;
/**
* This class implements depth-first search over a Graph, return an enumeration of the nodes of the
* graph in order of increasing discover time. This class follows the outNodes of the graph nodes to
* define the graph, but this behavior can be changed by overriding the getConnected method.
*/
public class SlowDFSDiscoverTimeIterator extends GraphDFSDiscoverTimeIterator {
public static final long serialVersionUID = 9439217987188L;
/** An iterator of child nodes for each node being searched A Map: Node -> Iterator */
private final Map> pendingChildren = HashMapFactory.make(25);
/** For use with extreme care by subclasses that know what they're doing. */
protected SlowDFSDiscoverTimeIterator() {}
/**
* Construct a depth-first enumerator starting with a particular node in a directed graph.
*
* @param G the graph whose nodes to enumerate
*/
public SlowDFSDiscoverTimeIterator(Graph G, T N) {
init(G, new NonNullSingletonIterator<>(N));
}
/**
* Construct a depth-first enumerator across the (possibly improper) subset of nodes reachable
* from the nodes in the given enumeration.
*
* @param G the graph whose nodes to enumerate
* @param nodes the set of nodes from which to start searching
*/
public SlowDFSDiscoverTimeIterator(Graph G, Iterator nodes) {
if (nodes == null) {
throw new IllegalArgumentException("null nodes");
}
init(G, nodes);
}
/**
* Constructor SlowDFSDiscoverTimeIterator.
*
* @throws NullPointerException if G is null
*/
public SlowDFSDiscoverTimeIterator(Graph G) throws NullPointerException {
if (G == null) {
throw new IllegalArgumentException("G is null");
}
init(G, G.iterator());
}
@Override
protected @Nullable Iterator extends T> getPendingChildren(Object n) {
return pendingChildren.get(n);
}
/** Method setPendingChildren. */
@Override
protected void setPendingChildren(T v, Iterator extends T> iterator) {
pendingChildren.put(v, iterator);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy