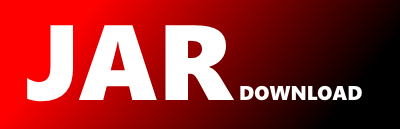
com.ibm.watson.assistant.v1.model.OutputData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of assistant Show documentation
Show all versions of assistant Show documentation
Java client library to use the IBM Assistant API
/*
* (C) Copyright IBM Corp. 2017, 2020.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*/
package com.ibm.watson.assistant.v1.model;
import com.google.gson.annotations.SerializedName;
import com.google.gson.reflect.TypeToken;
import com.ibm.cloud.sdk.core.service.model.DynamicModel;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* An output object that includes the response to the user, the dialog nodes that were triggered,
* and messages from the log.
*/
public class OutputData extends DynamicModel
© 2015 - 2025 Weber Informatics LLC | Privacy Policy