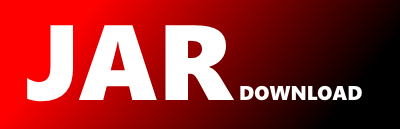
com.iceolive.util.ExcelExportUtil Maven / Gradle / Ivy
The newest version!
package com.iceolive.util;
import com.iceolive.util.enums.ColumnType;
import com.iceolive.util.enums.RuleType;
import com.iceolive.util.model.BaseInfo;
import com.iceolive.util.model.ColumnInfo;
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.ss.util.CellRangeAddressList;
import org.apache.poi.ss.util.CellReference;
import org.apache.poi.xssf.usermodel.XSSFClientAnchor;
import org.apache.poi.xssf.usermodel.XSSFDataValidationConstraint;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.awt.image.BufferedImage;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import org.apache.poi.util.Units;
/**
* @author wangmianzhe
*/
public class ExcelExportUtil {
/**
* 导出excel
*
* @param inputStream 导出模板
* @param data 导出数据
* @param columnInfos 导出列配置
* @param startRow 导出数据开始行(从1开始)
* @param onlyData 是否只导出数据(不含标题)
* @return
*/
public static byte[] exportExcel(
InputStream inputStream,
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy